loop back to a menu when using a return statement?
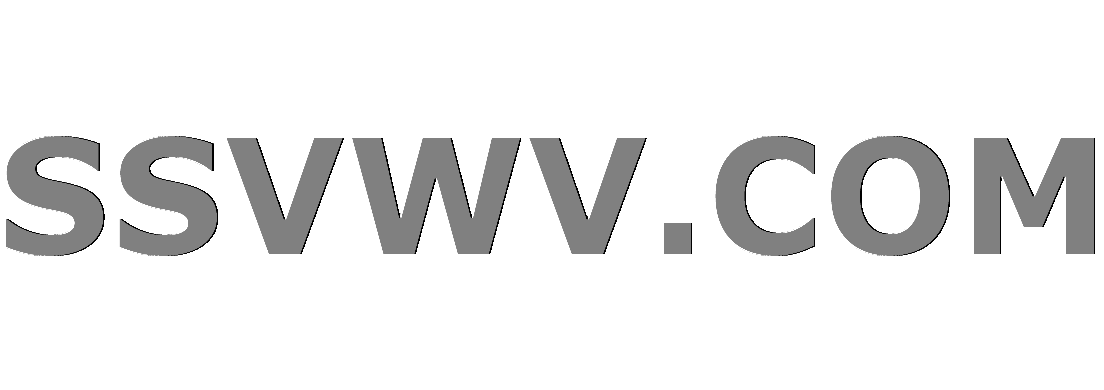
Multi tool use
I am adding up all the values in a text file and using a return statement to display the value at the end, I am also using that value in another function. So basically I have a function that calls another function.
My problem is that I need to ask the user if they want to go back to the menu again but I can't do that when using a return statement but if I don't use a return statement then I can get the total to go into my other function.
The average_salary function is the one that calls the total_salary function so that it can get the total value.
My code :
with open("Records.txt") as file: # Opens the text file with all the records in it
records = [line.strip() for line in file if not line.startswith("#")] # Creates a list named Records and stores all the records ignoring any lines with a '#'
def main():
print("nSelect from one of the options below:n") # Asks the user to select one of the options on the menu
print("Enter '1' to print out the number of records" # Tells the user what will happen if they select 1
"nEnter '2' to display a list of employees and their respective details" # Tells the user what will happen if they select 2
"nEnter '3' to display the total salary bill" # Tells the user what will happen if they select 3
"nEnter '4' to display a report showing the average salary based on the amount of employees" # Tells the user what will happen if they select 4
"nEnter '5' to add a new employee to the list" # Tells the user what will happen if they select 5
"nEnter '6' to display a report to show the amount of employees that are in each position type" # Tells the user what will happen if they select 6
"nEnter '7' to display employees who earn a higher salary than the threshold" # Tells the user what will happen if they select 7
"nEnter '8' to search for an existing record" # Tells the user what will happen if they select 8
"nEnter '9' to exit the programn") # Tells the user what will happen if they select 9
user_choice = input("Please enter the number of the option you would like use: ") # Asks the user to select what they would to do with the program from a selection of options
if user_choice == "1": # Checks to see if the user has entered '1', if the user enters '1' then the code inside this IF Statement will execute
number_of_lines() # Call the number_of_lines function
elif user_choice == "2": # Checks to see if the user has entered '2', if the user enters '2' then the code inside this IF Statement will execute
list_of_employees() # Calls the list_of_employees function
elif user_choice == "3": # Checks to see if the user has entered '3', if the user enters '3' then the code inside this IF Statement will execute
print("nYour salary total is £", format(total_salary(), ",.2f"), sep='') # calls the total_salary function and separates it with a space
elif user_choice == "4": # Checks to see if the user has entered '4', if the user enters '4' then the code inside this IF Statement will execute
average_salary() # Calls the average_salary function
elif user_choice == "5": # Checks to see if the user has entered '5', if the user enters '5' then the code inside this IF Statement will execute
add_new_employee() # Calls the add_new_employee function
elif user_choice == "6": # Checks to see if the user has entered '6', if the user enters '6' then the code inside this IF Statement will execute
number_of_position_type() # Calls the number_of_position_type function
elif user_choice == "7": # Checks to see if the user has entered '7', if the user enters '7' then the code inside this IF Statement will execute
above_salary_threshold() # Calls the above_user_threshold function
elif user_choice == "8": # Checks to see if the user has entered '8', if the user enters '8' then the code inside this IF Statement will execute
search_for_a_record() # Calls the add_new_employee function
elif user_choice == "9": # Checks to see if the user has entered '9', if the user enters '9' then the code inside this IF Statement will execute
print("nProgram closed successfully") # Prints a message to the user
exit() # Exits the program
else:
print("nEnter a number between 1 to 9") # Displays a message to the user if the do not select one of the above options
main()
# End of IF Statement
def number_of_lines(): # Creates a function named 'number_of_lines'
records_count = sum(1 for records_count in open("Records.txt") if not records_count.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file, ignoring the commented lines
print("nThe number of records is:", records_count) # Prints the number of records for the user to see
lines_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Asks the user for input
if lines_finished == 'yes': # Checks the users input to see if it is 'yes' , if the users input matches the condition then the code inside the IF will run
main() # Returns the user to the main function
else:
number_of_lines() # If the user types anything but yes then the number_of_lines function will run
def list_of_employees(): # Creates a function named 'list_of_employees'
for line in open("Records.txt"): # Opens the records text file
li = line.strip() # Remove any unnecessary spaces
if not li.startswith("#"): # removes any lines that start with a '#'
print(line.rstrip()) # prints the records to the user
employees_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower()
if employees_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
list_of_employees() # If the user types anything but yes then the list_of_employees function will run
def total_salary(): # Creates a function named 'total_salary'
with open("Records.txt", "r") as record_file: # Opens the records files as read and creates a variable called record_file
salaries_list = (record_file.read().split()[11::7]) # Creates a variable named salaries_list, the records files is then read in and split. I then tell the program to start on index 11 and to skip the next 7 elements, this happens until the loop is over
salaries = ' '.join(salaries_list) # joins all values into a list
salaries = salaries.replace(',', '') # Replaces all the ',' with spaces
salaries = salaries.split() # Puts all the salaries into a list again
total = 0 # creates a variable called total
for i in salaries: # Creates a for loop to loop the salaries and add them all together
total += int(i) # Increments by one every time adding all the salaries together
return total # returns the total value
def average_salary(): # Creates a function named 'average_salary'
record_count = sum(1 for record in open("Records.txt") if not record.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file
print("nThe amount of employees is:", record_count) # Prints out the number of records and takes away one to take away the heading line inside the text file
salary = total_salary() # Calls the total_salary() function
print("nThe total salary is: £", format(salary, ",.2f"))
print("nThe average salary is: £", format(salary / record_count, ".2f")) # Takes the total salary bill and divides it by the amount of lines and formats it to 2 decimal places
average_salary_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Stores the users input into the average_salary_finished variable and converts it to lowercase
if average_salary_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
average_salary() # If the user types anything but yes then the average_salary function will run
def add_new_employee(): # Creates a function named 'add_new_employee'
with open("records.txt", "a+") as storing_records: # Opens the record text file for append+ so that anything written to the text file will be written to the end and also I can read the file
last_record = records[-1] # Creates a variable called last_record and stores the values of the records variable inside, then it gets the last value in the list.
print("nThe last record in the file is:n" + last_record, "n" + "nPlease enter the number that comes after the previous user ID") # Prints the last_record variable
another_record = "y" # Creates a variable called another_Record and sets it to 'y'
while another_record == "y" or another_record == "Y": # Creates a while loop that will keep running as long as the another_Record variable is set to 'y' or 'Y'
employee_number = input("nEnter your employee number:") # Stores the users input in the employee_number variable
employee_name = input("nEnter your name:") # Stores the users input in the employee_name variable
employee_age = input("nEnter your age:") # Stores the users input in the employee_age variable
employee_position = input("nEnter your position:") # Stores the users input in the employee_position variable
employee_salary = input("nEnter your salary:") # Stores the users input in the employee_salary variable
employee_years = input("nEnter the amount of years you have been employed:") # Stores the users input in the employee_years variable
user_input_record = employee_number + ', ' + employee_name + ', ' + employee_age + ', ' + employee_position + ', ' + employee_salary + ', ' + employee_years # Adds all the user inputs together and separates them with comas
storing_records.write(user_input_record + "n") # Stores the user input in the records text file
another_record = input("nDo you want to input another record? (yes/no): ").lower() # Asks the user if they want to add another record, if the user types 'y' or 'Y' then the while loops will run again
if another_record == 'yes':
add_new_employee() # If the user types anything but yes then the add_new_employee function will run
else:
main() # Returns the user to the main function
def number_of_position_type(): # Creates a function called number_of_position_type
with open("Records.txt", "r") as record: # Reads in the text file as record
position_type_list = (record.read().split()[10::7]) # Creates a variable named position_type_list, the records files is then read in and split. I then tell the program to start on index 10 and to skip the next 7 elements, this happens until the loop is over
position_type = ' '.join(position_type_list) # joins all values into a list
position_type = (position_type.replace(',', '')) # Replaces all the ',' with spaces
developer = position_type.count("Developer") # Creates a variable called developer and counts the position_type variable to see how many times the word 'Developers' appears
print('%-40s%-0s' % ("The total number of Developers is:", developer)) # Prints out the developer variable
devops = position_type.count("DevOps") # Creates a variable called devops and counts the position_type variable to see how many times the word 'DevOps' appears
print('%-40s%-0s' % ("The total number of DevOps is:", devops)) # Prints out the devops variable
analyst = position_type.count("Analyst") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Analyst' appears
print('%-40s%-0s' % ("The total number of Analysts is:", analyst)) # Prints out the analyst variable
tester = position_type.count("Tester") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Tester' appears
print('%-40s%-0s' % ("The total number of Testers is:", tester)) # Prints out the tester variable
designer = position_type.count("Designer") # Creates a variable called designer and counts the position_type variable to see how many times the word 'Designer' appears
print('%-40s%-0s' % ("The total number of Designers is:", designer)) # Prints out the designer variable
position_finished = input("Are you ready to return back to the menu?(yes/no): ").lower() # Stores the user input into the position_finished variable
if position_finished == 'yes': # Checks the users input to see if it equal to 'yes'
main() # Returns the user to the main function
else:
average_salary() # Returns the user to the search_for_a_record function
def above_salary_threshold(): # Creates a function called above_salary_threshold
try: # Tries the code for any errors
user_threshold = int(input("Enter the salary threshold: ")) # Asks the user to input data and stores it as an integer
for line in records: # Creates a for loop
fields = line.split(', ') # Creates a variable called fields and splits the lines that have ','
if user_threshold < int(fields[4]): # Creates an if statement
print('%03itt%-20s£%-10.2f' % (int(fields[0]), fields[1], int(fields[4]))) # Formats the output
above_salary_finished = input("Are you ready to return back to the menu?(yes/no): ").lower()
if above_salary_finished == 'yes': # Checks if the users input is equal to use
main() # Returns the user to the main function
else:
above_salary_threshold() # Returns the user to the above_salary_threshold function
except ValueError: # Catches any value errors
print("You have entered an invalid number") # Prints a message to the user
main()
def search_for_a_record():
try: # Tries the code for any errors
data = open('Records.txt').read().splitlines() # opens the records file and reads and splits the lines and stores it to the data variable
emp_no = input("nEnter the UserID you would like to search for: ") # Creates a variable called emp_no and asks the user to enter a userID
record = [line for line in data if line.split(',')[0] == emp_no][0] # Creates a for loop to search through the list of records and splits them up and searches each line for the value entered in by the user
print(record) # Prints the records variable
another_record = input("nDo you want to enter another User_ID?(yes/no): ").lower() # Stores the user input as lowercase
if another_record == 'yes': # Creates an IF statement to see if the user wants to search for another record, if the user selects yes the code in this section will run
search_for_a_record() # Returns to the beginning of the search_for_a_record function
else:
main() # Returns the user to the main function
except IndexError: # Catches any errors
print("nYou have entered an invalid number") # Prints a message to the user
search_for_a_record() # Returns the user to the search_for_a_record function
users_name = input("What is your name?: ") # Asks the user for their name and stores their input to the users_name variable
print("nWelcome", users_name) # Prints welcome followed by the name stored in the users_name variable
main() # calls the main function
python-3.x
|
show 2 more comments
I am adding up all the values in a text file and using a return statement to display the value at the end, I am also using that value in another function. So basically I have a function that calls another function.
My problem is that I need to ask the user if they want to go back to the menu again but I can't do that when using a return statement but if I don't use a return statement then I can get the total to go into my other function.
The average_salary function is the one that calls the total_salary function so that it can get the total value.
My code :
with open("Records.txt") as file: # Opens the text file with all the records in it
records = [line.strip() for line in file if not line.startswith("#")] # Creates a list named Records and stores all the records ignoring any lines with a '#'
def main():
print("nSelect from one of the options below:n") # Asks the user to select one of the options on the menu
print("Enter '1' to print out the number of records" # Tells the user what will happen if they select 1
"nEnter '2' to display a list of employees and their respective details" # Tells the user what will happen if they select 2
"nEnter '3' to display the total salary bill" # Tells the user what will happen if they select 3
"nEnter '4' to display a report showing the average salary based on the amount of employees" # Tells the user what will happen if they select 4
"nEnter '5' to add a new employee to the list" # Tells the user what will happen if they select 5
"nEnter '6' to display a report to show the amount of employees that are in each position type" # Tells the user what will happen if they select 6
"nEnter '7' to display employees who earn a higher salary than the threshold" # Tells the user what will happen if they select 7
"nEnter '8' to search for an existing record" # Tells the user what will happen if they select 8
"nEnter '9' to exit the programn") # Tells the user what will happen if they select 9
user_choice = input("Please enter the number of the option you would like use: ") # Asks the user to select what they would to do with the program from a selection of options
if user_choice == "1": # Checks to see if the user has entered '1', if the user enters '1' then the code inside this IF Statement will execute
number_of_lines() # Call the number_of_lines function
elif user_choice == "2": # Checks to see if the user has entered '2', if the user enters '2' then the code inside this IF Statement will execute
list_of_employees() # Calls the list_of_employees function
elif user_choice == "3": # Checks to see if the user has entered '3', if the user enters '3' then the code inside this IF Statement will execute
print("nYour salary total is £", format(total_salary(), ",.2f"), sep='') # calls the total_salary function and separates it with a space
elif user_choice == "4": # Checks to see if the user has entered '4', if the user enters '4' then the code inside this IF Statement will execute
average_salary() # Calls the average_salary function
elif user_choice == "5": # Checks to see if the user has entered '5', if the user enters '5' then the code inside this IF Statement will execute
add_new_employee() # Calls the add_new_employee function
elif user_choice == "6": # Checks to see if the user has entered '6', if the user enters '6' then the code inside this IF Statement will execute
number_of_position_type() # Calls the number_of_position_type function
elif user_choice == "7": # Checks to see if the user has entered '7', if the user enters '7' then the code inside this IF Statement will execute
above_salary_threshold() # Calls the above_user_threshold function
elif user_choice == "8": # Checks to see if the user has entered '8', if the user enters '8' then the code inside this IF Statement will execute
search_for_a_record() # Calls the add_new_employee function
elif user_choice == "9": # Checks to see if the user has entered '9', if the user enters '9' then the code inside this IF Statement will execute
print("nProgram closed successfully") # Prints a message to the user
exit() # Exits the program
else:
print("nEnter a number between 1 to 9") # Displays a message to the user if the do not select one of the above options
main()
# End of IF Statement
def number_of_lines(): # Creates a function named 'number_of_lines'
records_count = sum(1 for records_count in open("Records.txt") if not records_count.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file, ignoring the commented lines
print("nThe number of records is:", records_count) # Prints the number of records for the user to see
lines_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Asks the user for input
if lines_finished == 'yes': # Checks the users input to see if it is 'yes' , if the users input matches the condition then the code inside the IF will run
main() # Returns the user to the main function
else:
number_of_lines() # If the user types anything but yes then the number_of_lines function will run
def list_of_employees(): # Creates a function named 'list_of_employees'
for line in open("Records.txt"): # Opens the records text file
li = line.strip() # Remove any unnecessary spaces
if not li.startswith("#"): # removes any lines that start with a '#'
print(line.rstrip()) # prints the records to the user
employees_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower()
if employees_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
list_of_employees() # If the user types anything but yes then the list_of_employees function will run
def total_salary(): # Creates a function named 'total_salary'
with open("Records.txt", "r") as record_file: # Opens the records files as read and creates a variable called record_file
salaries_list = (record_file.read().split()[11::7]) # Creates a variable named salaries_list, the records files is then read in and split. I then tell the program to start on index 11 and to skip the next 7 elements, this happens until the loop is over
salaries = ' '.join(salaries_list) # joins all values into a list
salaries = salaries.replace(',', '') # Replaces all the ',' with spaces
salaries = salaries.split() # Puts all the salaries into a list again
total = 0 # creates a variable called total
for i in salaries: # Creates a for loop to loop the salaries and add them all together
total += int(i) # Increments by one every time adding all the salaries together
return total # returns the total value
def average_salary(): # Creates a function named 'average_salary'
record_count = sum(1 for record in open("Records.txt") if not record.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file
print("nThe amount of employees is:", record_count) # Prints out the number of records and takes away one to take away the heading line inside the text file
salary = total_salary() # Calls the total_salary() function
print("nThe total salary is: £", format(salary, ",.2f"))
print("nThe average salary is: £", format(salary / record_count, ".2f")) # Takes the total salary bill and divides it by the amount of lines and formats it to 2 decimal places
average_salary_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Stores the users input into the average_salary_finished variable and converts it to lowercase
if average_salary_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
average_salary() # If the user types anything but yes then the average_salary function will run
def add_new_employee(): # Creates a function named 'add_new_employee'
with open("records.txt", "a+") as storing_records: # Opens the record text file for append+ so that anything written to the text file will be written to the end and also I can read the file
last_record = records[-1] # Creates a variable called last_record and stores the values of the records variable inside, then it gets the last value in the list.
print("nThe last record in the file is:n" + last_record, "n" + "nPlease enter the number that comes after the previous user ID") # Prints the last_record variable
another_record = "y" # Creates a variable called another_Record and sets it to 'y'
while another_record == "y" or another_record == "Y": # Creates a while loop that will keep running as long as the another_Record variable is set to 'y' or 'Y'
employee_number = input("nEnter your employee number:") # Stores the users input in the employee_number variable
employee_name = input("nEnter your name:") # Stores the users input in the employee_name variable
employee_age = input("nEnter your age:") # Stores the users input in the employee_age variable
employee_position = input("nEnter your position:") # Stores the users input in the employee_position variable
employee_salary = input("nEnter your salary:") # Stores the users input in the employee_salary variable
employee_years = input("nEnter the amount of years you have been employed:") # Stores the users input in the employee_years variable
user_input_record = employee_number + ', ' + employee_name + ', ' + employee_age + ', ' + employee_position + ', ' + employee_salary + ', ' + employee_years # Adds all the user inputs together and separates them with comas
storing_records.write(user_input_record + "n") # Stores the user input in the records text file
another_record = input("nDo you want to input another record? (yes/no): ").lower() # Asks the user if they want to add another record, if the user types 'y' or 'Y' then the while loops will run again
if another_record == 'yes':
add_new_employee() # If the user types anything but yes then the add_new_employee function will run
else:
main() # Returns the user to the main function
def number_of_position_type(): # Creates a function called number_of_position_type
with open("Records.txt", "r") as record: # Reads in the text file as record
position_type_list = (record.read().split()[10::7]) # Creates a variable named position_type_list, the records files is then read in and split. I then tell the program to start on index 10 and to skip the next 7 elements, this happens until the loop is over
position_type = ' '.join(position_type_list) # joins all values into a list
position_type = (position_type.replace(',', '')) # Replaces all the ',' with spaces
developer = position_type.count("Developer") # Creates a variable called developer and counts the position_type variable to see how many times the word 'Developers' appears
print('%-40s%-0s' % ("The total number of Developers is:", developer)) # Prints out the developer variable
devops = position_type.count("DevOps") # Creates a variable called devops and counts the position_type variable to see how many times the word 'DevOps' appears
print('%-40s%-0s' % ("The total number of DevOps is:", devops)) # Prints out the devops variable
analyst = position_type.count("Analyst") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Analyst' appears
print('%-40s%-0s' % ("The total number of Analysts is:", analyst)) # Prints out the analyst variable
tester = position_type.count("Tester") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Tester' appears
print('%-40s%-0s' % ("The total number of Testers is:", tester)) # Prints out the tester variable
designer = position_type.count("Designer") # Creates a variable called designer and counts the position_type variable to see how many times the word 'Designer' appears
print('%-40s%-0s' % ("The total number of Designers is:", designer)) # Prints out the designer variable
position_finished = input("Are you ready to return back to the menu?(yes/no): ").lower() # Stores the user input into the position_finished variable
if position_finished == 'yes': # Checks the users input to see if it equal to 'yes'
main() # Returns the user to the main function
else:
average_salary() # Returns the user to the search_for_a_record function
def above_salary_threshold(): # Creates a function called above_salary_threshold
try: # Tries the code for any errors
user_threshold = int(input("Enter the salary threshold: ")) # Asks the user to input data and stores it as an integer
for line in records: # Creates a for loop
fields = line.split(', ') # Creates a variable called fields and splits the lines that have ','
if user_threshold < int(fields[4]): # Creates an if statement
print('%03itt%-20s£%-10.2f' % (int(fields[0]), fields[1], int(fields[4]))) # Formats the output
above_salary_finished = input("Are you ready to return back to the menu?(yes/no): ").lower()
if above_salary_finished == 'yes': # Checks if the users input is equal to use
main() # Returns the user to the main function
else:
above_salary_threshold() # Returns the user to the above_salary_threshold function
except ValueError: # Catches any value errors
print("You have entered an invalid number") # Prints a message to the user
main()
def search_for_a_record():
try: # Tries the code for any errors
data = open('Records.txt').read().splitlines() # opens the records file and reads and splits the lines and stores it to the data variable
emp_no = input("nEnter the UserID you would like to search for: ") # Creates a variable called emp_no and asks the user to enter a userID
record = [line for line in data if line.split(',')[0] == emp_no][0] # Creates a for loop to search through the list of records and splits them up and searches each line for the value entered in by the user
print(record) # Prints the records variable
another_record = input("nDo you want to enter another User_ID?(yes/no): ").lower() # Stores the user input as lowercase
if another_record == 'yes': # Creates an IF statement to see if the user wants to search for another record, if the user selects yes the code in this section will run
search_for_a_record() # Returns to the beginning of the search_for_a_record function
else:
main() # Returns the user to the main function
except IndexError: # Catches any errors
print("nYou have entered an invalid number") # Prints a message to the user
search_for_a_record() # Returns the user to the search_for_a_record function
users_name = input("What is your name?: ") # Asks the user for their name and stores their input to the users_name variable
print("nWelcome", users_name) # Prints welcome followed by the name stored in the users_name variable
main() # calls the main function
python-3.x
Why wouldtotal_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see istotal_salary()
should beprint(total_salary())
if you want that to be printed.
– Carcigenicate
Nov 28 '18 at 17:04
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
It should be already going back to the menu oncetotal_salary
returns. And just a note, you're not going to want to calltotal_salary
several times. Call it once inaverage_salary
, save the return value, and use the return instead. Something likesalary = total_salary()
. Callingtotal_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.
– Carcigenicate
Nov 28 '18 at 17:09
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15
|
show 2 more comments
I am adding up all the values in a text file and using a return statement to display the value at the end, I am also using that value in another function. So basically I have a function that calls another function.
My problem is that I need to ask the user if they want to go back to the menu again but I can't do that when using a return statement but if I don't use a return statement then I can get the total to go into my other function.
The average_salary function is the one that calls the total_salary function so that it can get the total value.
My code :
with open("Records.txt") as file: # Opens the text file with all the records in it
records = [line.strip() for line in file if not line.startswith("#")] # Creates a list named Records and stores all the records ignoring any lines with a '#'
def main():
print("nSelect from one of the options below:n") # Asks the user to select one of the options on the menu
print("Enter '1' to print out the number of records" # Tells the user what will happen if they select 1
"nEnter '2' to display a list of employees and their respective details" # Tells the user what will happen if they select 2
"nEnter '3' to display the total salary bill" # Tells the user what will happen if they select 3
"nEnter '4' to display a report showing the average salary based on the amount of employees" # Tells the user what will happen if they select 4
"nEnter '5' to add a new employee to the list" # Tells the user what will happen if they select 5
"nEnter '6' to display a report to show the amount of employees that are in each position type" # Tells the user what will happen if they select 6
"nEnter '7' to display employees who earn a higher salary than the threshold" # Tells the user what will happen if they select 7
"nEnter '8' to search for an existing record" # Tells the user what will happen if they select 8
"nEnter '9' to exit the programn") # Tells the user what will happen if they select 9
user_choice = input("Please enter the number of the option you would like use: ") # Asks the user to select what they would to do with the program from a selection of options
if user_choice == "1": # Checks to see if the user has entered '1', if the user enters '1' then the code inside this IF Statement will execute
number_of_lines() # Call the number_of_lines function
elif user_choice == "2": # Checks to see if the user has entered '2', if the user enters '2' then the code inside this IF Statement will execute
list_of_employees() # Calls the list_of_employees function
elif user_choice == "3": # Checks to see if the user has entered '3', if the user enters '3' then the code inside this IF Statement will execute
print("nYour salary total is £", format(total_salary(), ",.2f"), sep='') # calls the total_salary function and separates it with a space
elif user_choice == "4": # Checks to see if the user has entered '4', if the user enters '4' then the code inside this IF Statement will execute
average_salary() # Calls the average_salary function
elif user_choice == "5": # Checks to see if the user has entered '5', if the user enters '5' then the code inside this IF Statement will execute
add_new_employee() # Calls the add_new_employee function
elif user_choice == "6": # Checks to see if the user has entered '6', if the user enters '6' then the code inside this IF Statement will execute
number_of_position_type() # Calls the number_of_position_type function
elif user_choice == "7": # Checks to see if the user has entered '7', if the user enters '7' then the code inside this IF Statement will execute
above_salary_threshold() # Calls the above_user_threshold function
elif user_choice == "8": # Checks to see if the user has entered '8', if the user enters '8' then the code inside this IF Statement will execute
search_for_a_record() # Calls the add_new_employee function
elif user_choice == "9": # Checks to see if the user has entered '9', if the user enters '9' then the code inside this IF Statement will execute
print("nProgram closed successfully") # Prints a message to the user
exit() # Exits the program
else:
print("nEnter a number between 1 to 9") # Displays a message to the user if the do not select one of the above options
main()
# End of IF Statement
def number_of_lines(): # Creates a function named 'number_of_lines'
records_count = sum(1 for records_count in open("Records.txt") if not records_count.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file, ignoring the commented lines
print("nThe number of records is:", records_count) # Prints the number of records for the user to see
lines_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Asks the user for input
if lines_finished == 'yes': # Checks the users input to see if it is 'yes' , if the users input matches the condition then the code inside the IF will run
main() # Returns the user to the main function
else:
number_of_lines() # If the user types anything but yes then the number_of_lines function will run
def list_of_employees(): # Creates a function named 'list_of_employees'
for line in open("Records.txt"): # Opens the records text file
li = line.strip() # Remove any unnecessary spaces
if not li.startswith("#"): # removes any lines that start with a '#'
print(line.rstrip()) # prints the records to the user
employees_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower()
if employees_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
list_of_employees() # If the user types anything but yes then the list_of_employees function will run
def total_salary(): # Creates a function named 'total_salary'
with open("Records.txt", "r") as record_file: # Opens the records files as read and creates a variable called record_file
salaries_list = (record_file.read().split()[11::7]) # Creates a variable named salaries_list, the records files is then read in and split. I then tell the program to start on index 11 and to skip the next 7 elements, this happens until the loop is over
salaries = ' '.join(salaries_list) # joins all values into a list
salaries = salaries.replace(',', '') # Replaces all the ',' with spaces
salaries = salaries.split() # Puts all the salaries into a list again
total = 0 # creates a variable called total
for i in salaries: # Creates a for loop to loop the salaries and add them all together
total += int(i) # Increments by one every time adding all the salaries together
return total # returns the total value
def average_salary(): # Creates a function named 'average_salary'
record_count = sum(1 for record in open("Records.txt") if not record.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file
print("nThe amount of employees is:", record_count) # Prints out the number of records and takes away one to take away the heading line inside the text file
salary = total_salary() # Calls the total_salary() function
print("nThe total salary is: £", format(salary, ",.2f"))
print("nThe average salary is: £", format(salary / record_count, ".2f")) # Takes the total salary bill and divides it by the amount of lines and formats it to 2 decimal places
average_salary_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Stores the users input into the average_salary_finished variable and converts it to lowercase
if average_salary_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
average_salary() # If the user types anything but yes then the average_salary function will run
def add_new_employee(): # Creates a function named 'add_new_employee'
with open("records.txt", "a+") as storing_records: # Opens the record text file for append+ so that anything written to the text file will be written to the end and also I can read the file
last_record = records[-1] # Creates a variable called last_record and stores the values of the records variable inside, then it gets the last value in the list.
print("nThe last record in the file is:n" + last_record, "n" + "nPlease enter the number that comes after the previous user ID") # Prints the last_record variable
another_record = "y" # Creates a variable called another_Record and sets it to 'y'
while another_record == "y" or another_record == "Y": # Creates a while loop that will keep running as long as the another_Record variable is set to 'y' or 'Y'
employee_number = input("nEnter your employee number:") # Stores the users input in the employee_number variable
employee_name = input("nEnter your name:") # Stores the users input in the employee_name variable
employee_age = input("nEnter your age:") # Stores the users input in the employee_age variable
employee_position = input("nEnter your position:") # Stores the users input in the employee_position variable
employee_salary = input("nEnter your salary:") # Stores the users input in the employee_salary variable
employee_years = input("nEnter the amount of years you have been employed:") # Stores the users input in the employee_years variable
user_input_record = employee_number + ', ' + employee_name + ', ' + employee_age + ', ' + employee_position + ', ' + employee_salary + ', ' + employee_years # Adds all the user inputs together and separates them with comas
storing_records.write(user_input_record + "n") # Stores the user input in the records text file
another_record = input("nDo you want to input another record? (yes/no): ").lower() # Asks the user if they want to add another record, if the user types 'y' or 'Y' then the while loops will run again
if another_record == 'yes':
add_new_employee() # If the user types anything but yes then the add_new_employee function will run
else:
main() # Returns the user to the main function
def number_of_position_type(): # Creates a function called number_of_position_type
with open("Records.txt", "r") as record: # Reads in the text file as record
position_type_list = (record.read().split()[10::7]) # Creates a variable named position_type_list, the records files is then read in and split. I then tell the program to start on index 10 and to skip the next 7 elements, this happens until the loop is over
position_type = ' '.join(position_type_list) # joins all values into a list
position_type = (position_type.replace(',', '')) # Replaces all the ',' with spaces
developer = position_type.count("Developer") # Creates a variable called developer and counts the position_type variable to see how many times the word 'Developers' appears
print('%-40s%-0s' % ("The total number of Developers is:", developer)) # Prints out the developer variable
devops = position_type.count("DevOps") # Creates a variable called devops and counts the position_type variable to see how many times the word 'DevOps' appears
print('%-40s%-0s' % ("The total number of DevOps is:", devops)) # Prints out the devops variable
analyst = position_type.count("Analyst") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Analyst' appears
print('%-40s%-0s' % ("The total number of Analysts is:", analyst)) # Prints out the analyst variable
tester = position_type.count("Tester") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Tester' appears
print('%-40s%-0s' % ("The total number of Testers is:", tester)) # Prints out the tester variable
designer = position_type.count("Designer") # Creates a variable called designer and counts the position_type variable to see how many times the word 'Designer' appears
print('%-40s%-0s' % ("The total number of Designers is:", designer)) # Prints out the designer variable
position_finished = input("Are you ready to return back to the menu?(yes/no): ").lower() # Stores the user input into the position_finished variable
if position_finished == 'yes': # Checks the users input to see if it equal to 'yes'
main() # Returns the user to the main function
else:
average_salary() # Returns the user to the search_for_a_record function
def above_salary_threshold(): # Creates a function called above_salary_threshold
try: # Tries the code for any errors
user_threshold = int(input("Enter the salary threshold: ")) # Asks the user to input data and stores it as an integer
for line in records: # Creates a for loop
fields = line.split(', ') # Creates a variable called fields and splits the lines that have ','
if user_threshold < int(fields[4]): # Creates an if statement
print('%03itt%-20s£%-10.2f' % (int(fields[0]), fields[1], int(fields[4]))) # Formats the output
above_salary_finished = input("Are you ready to return back to the menu?(yes/no): ").lower()
if above_salary_finished == 'yes': # Checks if the users input is equal to use
main() # Returns the user to the main function
else:
above_salary_threshold() # Returns the user to the above_salary_threshold function
except ValueError: # Catches any value errors
print("You have entered an invalid number") # Prints a message to the user
main()
def search_for_a_record():
try: # Tries the code for any errors
data = open('Records.txt').read().splitlines() # opens the records file and reads and splits the lines and stores it to the data variable
emp_no = input("nEnter the UserID you would like to search for: ") # Creates a variable called emp_no and asks the user to enter a userID
record = [line for line in data if line.split(',')[0] == emp_no][0] # Creates a for loop to search through the list of records and splits them up and searches each line for the value entered in by the user
print(record) # Prints the records variable
another_record = input("nDo you want to enter another User_ID?(yes/no): ").lower() # Stores the user input as lowercase
if another_record == 'yes': # Creates an IF statement to see if the user wants to search for another record, if the user selects yes the code in this section will run
search_for_a_record() # Returns to the beginning of the search_for_a_record function
else:
main() # Returns the user to the main function
except IndexError: # Catches any errors
print("nYou have entered an invalid number") # Prints a message to the user
search_for_a_record() # Returns the user to the search_for_a_record function
users_name = input("What is your name?: ") # Asks the user for their name and stores their input to the users_name variable
print("nWelcome", users_name) # Prints welcome followed by the name stored in the users_name variable
main() # calls the main function
python-3.x
I am adding up all the values in a text file and using a return statement to display the value at the end, I am also using that value in another function. So basically I have a function that calls another function.
My problem is that I need to ask the user if they want to go back to the menu again but I can't do that when using a return statement but if I don't use a return statement then I can get the total to go into my other function.
The average_salary function is the one that calls the total_salary function so that it can get the total value.
My code :
with open("Records.txt") as file: # Opens the text file with all the records in it
records = [line.strip() for line in file if not line.startswith("#")] # Creates a list named Records and stores all the records ignoring any lines with a '#'
def main():
print("nSelect from one of the options below:n") # Asks the user to select one of the options on the menu
print("Enter '1' to print out the number of records" # Tells the user what will happen if they select 1
"nEnter '2' to display a list of employees and their respective details" # Tells the user what will happen if they select 2
"nEnter '3' to display the total salary bill" # Tells the user what will happen if they select 3
"nEnter '4' to display a report showing the average salary based on the amount of employees" # Tells the user what will happen if they select 4
"nEnter '5' to add a new employee to the list" # Tells the user what will happen if they select 5
"nEnter '6' to display a report to show the amount of employees that are in each position type" # Tells the user what will happen if they select 6
"nEnter '7' to display employees who earn a higher salary than the threshold" # Tells the user what will happen if they select 7
"nEnter '8' to search for an existing record" # Tells the user what will happen if they select 8
"nEnter '9' to exit the programn") # Tells the user what will happen if they select 9
user_choice = input("Please enter the number of the option you would like use: ") # Asks the user to select what they would to do with the program from a selection of options
if user_choice == "1": # Checks to see if the user has entered '1', if the user enters '1' then the code inside this IF Statement will execute
number_of_lines() # Call the number_of_lines function
elif user_choice == "2": # Checks to see if the user has entered '2', if the user enters '2' then the code inside this IF Statement will execute
list_of_employees() # Calls the list_of_employees function
elif user_choice == "3": # Checks to see if the user has entered '3', if the user enters '3' then the code inside this IF Statement will execute
print("nYour salary total is £", format(total_salary(), ",.2f"), sep='') # calls the total_salary function and separates it with a space
elif user_choice == "4": # Checks to see if the user has entered '4', if the user enters '4' then the code inside this IF Statement will execute
average_salary() # Calls the average_salary function
elif user_choice == "5": # Checks to see if the user has entered '5', if the user enters '5' then the code inside this IF Statement will execute
add_new_employee() # Calls the add_new_employee function
elif user_choice == "6": # Checks to see if the user has entered '6', if the user enters '6' then the code inside this IF Statement will execute
number_of_position_type() # Calls the number_of_position_type function
elif user_choice == "7": # Checks to see if the user has entered '7', if the user enters '7' then the code inside this IF Statement will execute
above_salary_threshold() # Calls the above_user_threshold function
elif user_choice == "8": # Checks to see if the user has entered '8', if the user enters '8' then the code inside this IF Statement will execute
search_for_a_record() # Calls the add_new_employee function
elif user_choice == "9": # Checks to see if the user has entered '9', if the user enters '9' then the code inside this IF Statement will execute
print("nProgram closed successfully") # Prints a message to the user
exit() # Exits the program
else:
print("nEnter a number between 1 to 9") # Displays a message to the user if the do not select one of the above options
main()
# End of IF Statement
def number_of_lines(): # Creates a function named 'number_of_lines'
records_count = sum(1 for records_count in open("Records.txt") if not records_count.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file, ignoring the commented lines
print("nThe number of records is:", records_count) # Prints the number of records for the user to see
lines_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Asks the user for input
if lines_finished == 'yes': # Checks the users input to see if it is 'yes' , if the users input matches the condition then the code inside the IF will run
main() # Returns the user to the main function
else:
number_of_lines() # If the user types anything but yes then the number_of_lines function will run
def list_of_employees(): # Creates a function named 'list_of_employees'
for line in open("Records.txt"): # Opens the records text file
li = line.strip() # Remove any unnecessary spaces
if not li.startswith("#"): # removes any lines that start with a '#'
print(line.rstrip()) # prints the records to the user
employees_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower()
if employees_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
list_of_employees() # If the user types anything but yes then the list_of_employees function will run
def total_salary(): # Creates a function named 'total_salary'
with open("Records.txt", "r") as record_file: # Opens the records files as read and creates a variable called record_file
salaries_list = (record_file.read().split()[11::7]) # Creates a variable named salaries_list, the records files is then read in and split. I then tell the program to start on index 11 and to skip the next 7 elements, this happens until the loop is over
salaries = ' '.join(salaries_list) # joins all values into a list
salaries = salaries.replace(',', '') # Replaces all the ',' with spaces
salaries = salaries.split() # Puts all the salaries into a list again
total = 0 # creates a variable called total
for i in salaries: # Creates a for loop to loop the salaries and add them all together
total += int(i) # Increments by one every time adding all the salaries together
return total # returns the total value
def average_salary(): # Creates a function named 'average_salary'
record_count = sum(1 for record in open("Records.txt") if not record.startswith("#")) # converts the record_count to a sum and calculates the number of lines in the records.txt file
print("nThe amount of employees is:", record_count) # Prints out the number of records and takes away one to take away the heading line inside the text file
salary = total_salary() # Calls the total_salary() function
print("nThe total salary is: £", format(salary, ",.2f"))
print("nThe average salary is: £", format(salary / record_count, ".2f")) # Takes the total salary bill and divides it by the amount of lines and formats it to 2 decimal places
average_salary_finished = input("nAre you ready to return back to the menu?(yes/no): ").lower() # Stores the users input into the average_salary_finished variable and converts it to lowercase
if average_salary_finished == 'yes': # Checks if the users input is equal to yes
main() # Returns the user to the main function
else:
average_salary() # If the user types anything but yes then the average_salary function will run
def add_new_employee(): # Creates a function named 'add_new_employee'
with open("records.txt", "a+") as storing_records: # Opens the record text file for append+ so that anything written to the text file will be written to the end and also I can read the file
last_record = records[-1] # Creates a variable called last_record and stores the values of the records variable inside, then it gets the last value in the list.
print("nThe last record in the file is:n" + last_record, "n" + "nPlease enter the number that comes after the previous user ID") # Prints the last_record variable
another_record = "y" # Creates a variable called another_Record and sets it to 'y'
while another_record == "y" or another_record == "Y": # Creates a while loop that will keep running as long as the another_Record variable is set to 'y' or 'Y'
employee_number = input("nEnter your employee number:") # Stores the users input in the employee_number variable
employee_name = input("nEnter your name:") # Stores the users input in the employee_name variable
employee_age = input("nEnter your age:") # Stores the users input in the employee_age variable
employee_position = input("nEnter your position:") # Stores the users input in the employee_position variable
employee_salary = input("nEnter your salary:") # Stores the users input in the employee_salary variable
employee_years = input("nEnter the amount of years you have been employed:") # Stores the users input in the employee_years variable
user_input_record = employee_number + ', ' + employee_name + ', ' + employee_age + ', ' + employee_position + ', ' + employee_salary + ', ' + employee_years # Adds all the user inputs together and separates them with comas
storing_records.write(user_input_record + "n") # Stores the user input in the records text file
another_record = input("nDo you want to input another record? (yes/no): ").lower() # Asks the user if they want to add another record, if the user types 'y' or 'Y' then the while loops will run again
if another_record == 'yes':
add_new_employee() # If the user types anything but yes then the add_new_employee function will run
else:
main() # Returns the user to the main function
def number_of_position_type(): # Creates a function called number_of_position_type
with open("Records.txt", "r") as record: # Reads in the text file as record
position_type_list = (record.read().split()[10::7]) # Creates a variable named position_type_list, the records files is then read in and split. I then tell the program to start on index 10 and to skip the next 7 elements, this happens until the loop is over
position_type = ' '.join(position_type_list) # joins all values into a list
position_type = (position_type.replace(',', '')) # Replaces all the ',' with spaces
developer = position_type.count("Developer") # Creates a variable called developer and counts the position_type variable to see how many times the word 'Developers' appears
print('%-40s%-0s' % ("The total number of Developers is:", developer)) # Prints out the developer variable
devops = position_type.count("DevOps") # Creates a variable called devops and counts the position_type variable to see how many times the word 'DevOps' appears
print('%-40s%-0s' % ("The total number of DevOps is:", devops)) # Prints out the devops variable
analyst = position_type.count("Analyst") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Analyst' appears
print('%-40s%-0s' % ("The total number of Analysts is:", analyst)) # Prints out the analyst variable
tester = position_type.count("Tester") # Creates a variable called analyst and counts the position_type variable to see how many times the word 'Tester' appears
print('%-40s%-0s' % ("The total number of Testers is:", tester)) # Prints out the tester variable
designer = position_type.count("Designer") # Creates a variable called designer and counts the position_type variable to see how many times the word 'Designer' appears
print('%-40s%-0s' % ("The total number of Designers is:", designer)) # Prints out the designer variable
position_finished = input("Are you ready to return back to the menu?(yes/no): ").lower() # Stores the user input into the position_finished variable
if position_finished == 'yes': # Checks the users input to see if it equal to 'yes'
main() # Returns the user to the main function
else:
average_salary() # Returns the user to the search_for_a_record function
def above_salary_threshold(): # Creates a function called above_salary_threshold
try: # Tries the code for any errors
user_threshold = int(input("Enter the salary threshold: ")) # Asks the user to input data and stores it as an integer
for line in records: # Creates a for loop
fields = line.split(', ') # Creates a variable called fields and splits the lines that have ','
if user_threshold < int(fields[4]): # Creates an if statement
print('%03itt%-20s£%-10.2f' % (int(fields[0]), fields[1], int(fields[4]))) # Formats the output
above_salary_finished = input("Are you ready to return back to the menu?(yes/no): ").lower()
if above_salary_finished == 'yes': # Checks if the users input is equal to use
main() # Returns the user to the main function
else:
above_salary_threshold() # Returns the user to the above_salary_threshold function
except ValueError: # Catches any value errors
print("You have entered an invalid number") # Prints a message to the user
main()
def search_for_a_record():
try: # Tries the code for any errors
data = open('Records.txt').read().splitlines() # opens the records file and reads and splits the lines and stores it to the data variable
emp_no = input("nEnter the UserID you would like to search for: ") # Creates a variable called emp_no and asks the user to enter a userID
record = [line for line in data if line.split(',')[0] == emp_no][0] # Creates a for loop to search through the list of records and splits them up and searches each line for the value entered in by the user
print(record) # Prints the records variable
another_record = input("nDo you want to enter another User_ID?(yes/no): ").lower() # Stores the user input as lowercase
if another_record == 'yes': # Creates an IF statement to see if the user wants to search for another record, if the user selects yes the code in this section will run
search_for_a_record() # Returns to the beginning of the search_for_a_record function
else:
main() # Returns the user to the main function
except IndexError: # Catches any errors
print("nYou have entered an invalid number") # Prints a message to the user
search_for_a_record() # Returns the user to the search_for_a_record function
users_name = input("What is your name?: ") # Asks the user for their name and stores their input to the users_name variable
print("nWelcome", users_name) # Prints welcome followed by the name stored in the users_name variable
main() # calls the main function
python-3.x
python-3.x
edited Nov 28 '18 at 17:20
Darren_D19
asked Nov 28 '18 at 17:00
Darren_D19Darren_D19
175
175
Why wouldtotal_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see istotal_salary()
should beprint(total_salary())
if you want that to be printed.
– Carcigenicate
Nov 28 '18 at 17:04
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
It should be already going back to the menu oncetotal_salary
returns. And just a note, you're not going to want to calltotal_salary
several times. Call it once inaverage_salary
, save the return value, and use the return instead. Something likesalary = total_salary()
. Callingtotal_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.
– Carcigenicate
Nov 28 '18 at 17:09
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15
|
show 2 more comments
Why wouldtotal_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see istotal_salary()
should beprint(total_salary())
if you want that to be printed.
– Carcigenicate
Nov 28 '18 at 17:04
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
It should be already going back to the menu oncetotal_salary
returns. And just a note, you're not going to want to calltotal_salary
several times. Call it once inaverage_salary
, save the return value, and use the return instead. Something likesalary = total_salary()
. Callingtotal_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.
– Carcigenicate
Nov 28 '18 at 17:09
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15
Why would
total_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see is total_salary()
should be print(total_salary())
if you want that to be printed.– Carcigenicate
Nov 28 '18 at 17:04
Why would
total_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see is total_salary()
should be print(total_salary())
if you want that to be printed.– Carcigenicate
Nov 28 '18 at 17:04
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
It should be already going back to the menu once
total_salary
returns. And just a note, you're not going to want to call total_salary
several times. Call it once in average_salary
, save the return value, and use the return instead. Something like salary = total_salary()
. Calling total_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.– Carcigenicate
Nov 28 '18 at 17:09
It should be already going back to the menu once
total_salary
returns. And just a note, you're not going to want to call total_salary
several times. Call it once in average_salary
, save the return value, and use the return instead. Something like salary = total_salary()
. Calling total_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.– Carcigenicate
Nov 28 '18 at 17:09
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15
|
show 2 more comments
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53524556%2floop-back-to-a-menu-when-using-a-return-statement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53524556%2floop-back-to-a-menu-when-using-a-return-statement%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2mxb,nH2NwmJqIiGAYJemwLcdeo,hzIum2c1zeVb51 qGPzuCTzjZ3iL7a642l5pnw48F,aDXMrz9ug w,KddUG270YLmzzcRSM70Zd,0
Why would
total_salary
be concerned with menus? What's wrong with how things are currently set up? This should loop via a recursive call if they say they want to go back to the menu. The only major problem I see istotal_salary()
should beprint(total_salary())
if you want that to be printed.– Carcigenicate
Nov 28 '18 at 17:04
Sorry I must not have made it clear, in the total_salary function I need the user to be able to go back to the menu as I can not have the program exiting without using the menu
– Darren_D19
Nov 28 '18 at 17:07
It should be already going back to the menu once
total_salary
returns. And just a note, you're not going to want to calltotal_salary
several times. Call it once inaverage_salary
, save the return value, and use the return instead. Something likesalary = total_salary()
. Callingtotal_salary
involves reading a file, so you don't want to be doing that over and over again when you don't need to.– Carcigenicate
Nov 28 '18 at 17:09
Thank you for that information. also so when I run the total_salary function and it returns the total my program just exits, it doesn't go back to the main function that holds the menu. Any ideas on why that happens?
– Darren_D19
Nov 28 '18 at 17:13
With the code you've posted, the only way I can see that possibly happening is if it's exiting due to an error. How are you running the code?
– Carcigenicate
Nov 28 '18 at 17:15