Storing inputs into an array from text file
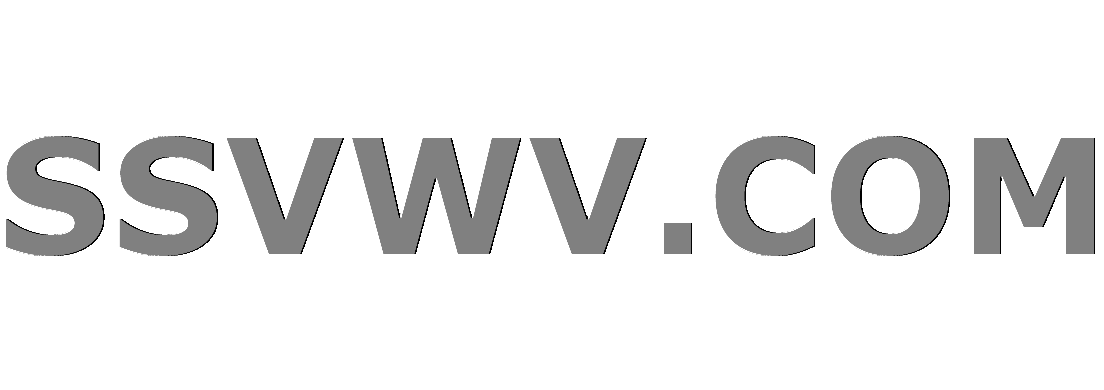
Multi tool use
forgive me for my ignorance as I'm still learning. This is my first assignment with try blocks and catch statements, and I'm confused on how to store the values into an array and display them correctly. According to my instructor's notes, my code looks correct but there's obviously something I'm missing since the console is only printing null. I'm assuming that somewhere the month files just aren't being stored in the array, but I'm not sure where they are supposed to be in my code.
String months;
StringArray1 = new String [4][3];
//fileReader object
try
{
FileReader fr = new FileReader ("months.txt");
BufferedReader inFile = new BufferedReader (fr);
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
months = inFile.readLine();
Array1[i][j] = months;
}
}
inFile.close();
}
catch (FileNotFoundException exception) {
}
catch (IOException exception) {
}
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
System.out.println(Array1[i][j]+ " ");
}
System.out.println();
The text file I'm trying to use is months and just has the months each separated by a new line.
EDIT: My text file and console output:
here
java arrays text
|
show 3 more comments
forgive me for my ignorance as I'm still learning. This is my first assignment with try blocks and catch statements, and I'm confused on how to store the values into an array and display them correctly. According to my instructor's notes, my code looks correct but there's obviously something I'm missing since the console is only printing null. I'm assuming that somewhere the month files just aren't being stored in the array, but I'm not sure where they are supposed to be in my code.
String months;
StringArray1 = new String [4][3];
//fileReader object
try
{
FileReader fr = new FileReader ("months.txt");
BufferedReader inFile = new BufferedReader (fr);
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
months = inFile.readLine();
Array1[i][j] = months;
}
}
inFile.close();
}
catch (FileNotFoundException exception) {
}
catch (IOException exception) {
}
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
System.out.println(Array1[i][j]+ " ");
}
System.out.println();
The text file I'm trying to use is months and just has the months each separated by a new line.
EDIT: My text file and console output:
here
java arrays text
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44
|
show 3 more comments
forgive me for my ignorance as I'm still learning. This is my first assignment with try blocks and catch statements, and I'm confused on how to store the values into an array and display them correctly. According to my instructor's notes, my code looks correct but there's obviously something I'm missing since the console is only printing null. I'm assuming that somewhere the month files just aren't being stored in the array, but I'm not sure where they are supposed to be in my code.
String months;
StringArray1 = new String [4][3];
//fileReader object
try
{
FileReader fr = new FileReader ("months.txt");
BufferedReader inFile = new BufferedReader (fr);
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
months = inFile.readLine();
Array1[i][j] = months;
}
}
inFile.close();
}
catch (FileNotFoundException exception) {
}
catch (IOException exception) {
}
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
System.out.println(Array1[i][j]+ " ");
}
System.out.println();
The text file I'm trying to use is months and just has the months each separated by a new line.
EDIT: My text file and console output:
here
java arrays text
forgive me for my ignorance as I'm still learning. This is my first assignment with try blocks and catch statements, and I'm confused on how to store the values into an array and display them correctly. According to my instructor's notes, my code looks correct but there's obviously something I'm missing since the console is only printing null. I'm assuming that somewhere the month files just aren't being stored in the array, but I'm not sure where they are supposed to be in my code.
String months;
StringArray1 = new String [4][3];
//fileReader object
try
{
FileReader fr = new FileReader ("months.txt");
BufferedReader inFile = new BufferedReader (fr);
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
months = inFile.readLine();
Array1[i][j] = months;
}
}
inFile.close();
}
catch (FileNotFoundException exception) {
}
catch (IOException exception) {
}
for (int i = 0; i < 4; i++)
{
for (int j = 0; j < 3; j++)
{
System.out.println(Array1[i][j]+ " ");
}
System.out.println();
The text file I'm trying to use is months and just has the months each separated by a new line.
EDIT: My text file and console output:
here
java arrays text
java arrays text
edited Nov 29 '18 at 0:56
Trevor Oakes
asked Nov 29 '18 at 0:23


Trevor OakesTrevor Oakes
73
73
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44
|
show 3 more comments
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44
|
show 3 more comments
1 Answer
1
active
oldest
votes
I put your code in my IDE, and I ran it, and i got
JAN
FEB
MAR
APR
MAY
JUN
JUL
AUG
SEP
OCT
NOV
DEC
Process finished with exit code 0
can you tell us, what it's the result that you are getting?
this is the content of mi file months.txt
Your project structure must be:
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530092%2fstoring-inputs-into-an-array-from-text-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I put your code in my IDE, and I ran it, and i got
JAN
FEB
MAR
APR
MAY
JUN
JUL
AUG
SEP
OCT
NOV
DEC
Process finished with exit code 0
can you tell us, what it's the result that you are getting?
this is the content of mi file months.txt
Your project structure must be:
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
|
show 3 more comments
I put your code in my IDE, and I ran it, and i got
JAN
FEB
MAR
APR
MAY
JUN
JUL
AUG
SEP
OCT
NOV
DEC
Process finished with exit code 0
can you tell us, what it's the result that you are getting?
this is the content of mi file months.txt
Your project structure must be:
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
|
show 3 more comments
I put your code in my IDE, and I ran it, and i got
JAN
FEB
MAR
APR
MAY
JUN
JUL
AUG
SEP
OCT
NOV
DEC
Process finished with exit code 0
can you tell us, what it's the result that you are getting?
this is the content of mi file months.txt
Your project structure must be:
I put your code in my IDE, and I ran it, and i got
JAN
FEB
MAR
APR
MAY
JUN
JUL
AUG
SEP
OCT
NOV
DEC
Process finished with exit code 0
can you tell us, what it's the result that you are getting?
this is the content of mi file months.txt
Your project structure must be:
edited Nov 29 '18 at 1:07
answered Nov 29 '18 at 0:50


Marco PensMarco Pens
916
916
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
|
show 3 more comments
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
I put my image in the edit on my OP.
– Trevor Oakes
Nov 29 '18 at 0:56
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
is Your file in the path of your project?, YourProjectFolder/months.txt?
– Marco Pens
Nov 29 '18 at 1:00
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
yes it is......
– Trevor Oakes
Nov 29 '18 at 1:05
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
i updated, my comment, can you check if your project is like that?
– Marco Pens
Nov 29 '18 at 1:07
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
exactly the same. man this is frustrating lol.
– Trevor Oakes
Nov 29 '18 at 1:17
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530092%2fstoring-inputs-into-an-array-from-text-file%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
aU R1H rCFlUXj6 ji PlYJHZj1a HNIGLB8cC OG1GgCrocoBcwHRy YeKzGMdscPDsNp ta
Can you share with us, the format of your file too?
– Marco Pens
Nov 29 '18 at 0:32
Sorry, what format are you referring to?
– Trevor Oakes
Nov 29 '18 at 0:37
i meant , its only like 01 02 03
– Marco Pens
Nov 29 '18 at 0:39
oh, yea each month is on a new line in the text file. January is line 1, february is line 2, and so on.
– Trevor Oakes
Nov 29 '18 at 0:44
Is your months.txt in the correct path?
– mettleap
Nov 29 '18 at 0:44