How to use CORS cross domain on Edge browser?
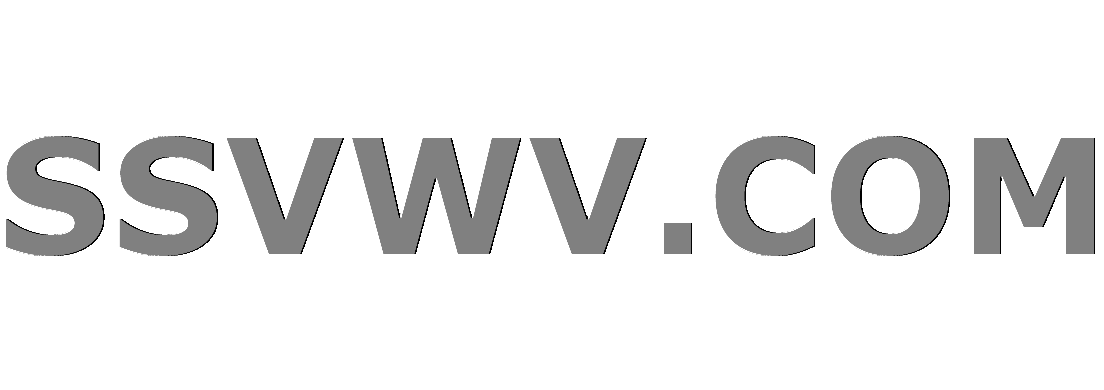
Multi tool use
I'm experiencing a weird issue when using the Edge browser. I cannot seem to figure out why on Chrome and Firefox this snippet performs correctly as a POST request. However on the Edge browser it comes up at a GET request. I've consulted other questions like this on SO, but I haven't found anything which is providing me the answer I need to circumvent this issue.
Why do I need cross domain? Well I'm posting to a server which is pushing the work to another port. I'm unable to perform the request without cross domain in the request. I can't seem to get around Edge default behavior. Any tips? There's nothing in the jQuery documentation about it
For reference: We're using a React front end with a Java backend. Our front end and backend are completely decoupled, so I'll need to be able to handle this in react.
$.ajax({
method: "POST",
type: "POST",
url: "http://www.example.com:8082",
crossDomain: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
dataType: 'json',
data: payload,
success: (data, status, jqxr) => {
successCB(data, status, jqxr)
},
error: (jqxr, textStatus, errorThrown) => {
if(errorCB) {
errorCB(jqxr, textStatus, errorThrown)
} else {
return errorThrown
}
}
})
jquery ajax reactjs cors microsoft-edge
add a comment |
I'm experiencing a weird issue when using the Edge browser. I cannot seem to figure out why on Chrome and Firefox this snippet performs correctly as a POST request. However on the Edge browser it comes up at a GET request. I've consulted other questions like this on SO, but I haven't found anything which is providing me the answer I need to circumvent this issue.
Why do I need cross domain? Well I'm posting to a server which is pushing the work to another port. I'm unable to perform the request without cross domain in the request. I can't seem to get around Edge default behavior. Any tips? There's nothing in the jQuery documentation about it
For reference: We're using a React front end with a Java backend. Our front end and backend are completely decoupled, so I'll need to be able to handle this in react.
$.ajax({
method: "POST",
type: "POST",
url: "http://www.example.com:8082",
crossDomain: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
dataType: 'json',
data: payload,
success: (data, status, jqxr) => {
successCB(data, status, jqxr)
},
error: (jqxr, textStatus, errorThrown) => {
if(errorCB) {
errorCB(jqxr, textStatus, errorThrown)
} else {
return errorThrown
}
}
})
jquery ajax reactjs cors microsoft-edge
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42
add a comment |
I'm experiencing a weird issue when using the Edge browser. I cannot seem to figure out why on Chrome and Firefox this snippet performs correctly as a POST request. However on the Edge browser it comes up at a GET request. I've consulted other questions like this on SO, but I haven't found anything which is providing me the answer I need to circumvent this issue.
Why do I need cross domain? Well I'm posting to a server which is pushing the work to another port. I'm unable to perform the request without cross domain in the request. I can't seem to get around Edge default behavior. Any tips? There's nothing in the jQuery documentation about it
For reference: We're using a React front end with a Java backend. Our front end and backend are completely decoupled, so I'll need to be able to handle this in react.
$.ajax({
method: "POST",
type: "POST",
url: "http://www.example.com:8082",
crossDomain: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
dataType: 'json',
data: payload,
success: (data, status, jqxr) => {
successCB(data, status, jqxr)
},
error: (jqxr, textStatus, errorThrown) => {
if(errorCB) {
errorCB(jqxr, textStatus, errorThrown)
} else {
return errorThrown
}
}
})
jquery ajax reactjs cors microsoft-edge
I'm experiencing a weird issue when using the Edge browser. I cannot seem to figure out why on Chrome and Firefox this snippet performs correctly as a POST request. However on the Edge browser it comes up at a GET request. I've consulted other questions like this on SO, but I haven't found anything which is providing me the answer I need to circumvent this issue.
Why do I need cross domain? Well I'm posting to a server which is pushing the work to another port. I'm unable to perform the request without cross domain in the request. I can't seem to get around Edge default behavior. Any tips? There's nothing in the jQuery documentation about it
For reference: We're using a React front end with a Java backend. Our front end and backend are completely decoupled, so I'll need to be able to handle this in react.
$.ajax({
method: "POST",
type: "POST",
url: "http://www.example.com:8082",
crossDomain: true,
contentType: "application/x-www-form-urlencoded; charset=UTF-8",
dataType: 'json',
data: payload,
success: (data, status, jqxr) => {
successCB(data, status, jqxr)
},
error: (jqxr, textStatus, errorThrown) => {
if(errorCB) {
errorCB(jqxr, textStatus, errorThrown)
} else {
return errorThrown
}
}
})
jquery ajax reactjs cors microsoft-edge
jquery ajax reactjs cors microsoft-edge
edited Jan 26 at 22:21


TylerH
16.1k105569
16.1k105569
asked Nov 29 '18 at 0:01


Sean KellySean Kelly
623216
623216
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42
add a comment |
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53529948%2fhow-to-use-cors-cross-domain-on-edge-browser%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53529948%2fhow-to-use-cors-cross-domain-on-edge-browser%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
HUjs,HuKYM5gxTbPlizgnR DvZFmvtPS73DOFj9mx27 rk7MwCf4AOYwJFE7c4nDAfb8zMiUq,5WeIQsQJYh
Please refer to this article to learn more about CORS, then, you could try to use Fiddler to capture the post request, and check whether it is correct.
– Zhi Lv - MSFT
Nov 30 '18 at 9:42