JQuery scroll button not working on certain browsers and mobile
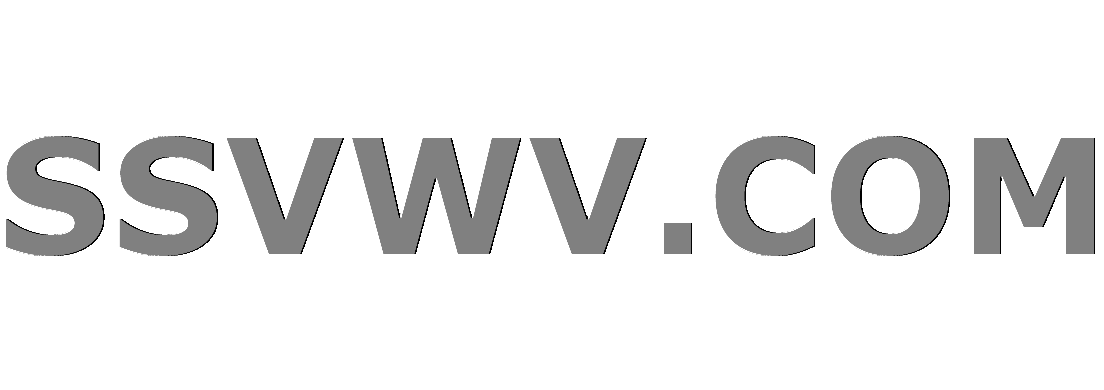
Multi tool use
EDIT: It now works on Edge thanks to Bowie, but the problem still persists on mobile, where the button doesn't appear at all.
I'm completely new to web development and have been tasked to create a website from scratch. I've learned a fair deal of HTML and CSS and have started to get into Javascript and JQuery. I have tried to implement a fixed button that scrolls the page back up to the top that appears only after a certain distance has been scrolled. It works perfectly fine in Chrome, Internet Explorer and Firefoxon desktop, however in Edge the button appears but doesn't scroll the page at all. And on mobile the button doesn't even appear at all...
Here is the code for it:
HTML
<link href="Styles.css" rel="stylesheet" type="text/css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"> </script>
<script src="Scripts.js"></script>
<a href="#" id="scrollTop"><img src="RFImages/arrow.png" height="102%" width="98%"></a>
The link and scripts are in "head"and the a href is just underneath the closing tag for body.
CSS (File is Styles.css)
#scrollTop {
border-radius: 10px;
border: 5px solid #333333;
width:80px;
height:80px;
background-color: #000000;
position:fixed;
display: none;
opacity: 0.5;
right: 40px;
top: 30px;
}
#scrollTop:hover {
opacity: 1;
}
Most of the CSS is just styling and opacity change when hovering over it.
JS (File is Scripts.js)
$(document).ready(function () {
$(document).scroll(function() {
if ( $(document).scrollTop() < 300 ) {
$("#scrollTop").fadeOut();
}
if ( $(document).scrollTop() >= 300 ) {
$("#scrollTop").fadeIn();
}
});
$('#scrollTop').click(function () {
$("html").animate({
scrollTop: 10
}, 600);
return false;
});
});
This is JS that I learned and implemented myself.
This is a really frustrating compatibility issue that I've been trying to fix, however even though I've been trying really hard to learn JS and JQuery, I'm finding it difficult to find a simple and easy to understand solution that is not many 10s of lines long, and even then some of those ones don't work on mobile.
I tried using the jQuery Mobile library too hosted by Google but that did nothing to fix the issue.
So here is my question:
How can I go about fixing this code and make it work on mobile and in Edge in the simplest and easiest way to understand for someone who has only just started web development.
Thanks, Alex.
jquery html css cross-browser compatibility
add a comment |
EDIT: It now works on Edge thanks to Bowie, but the problem still persists on mobile, where the button doesn't appear at all.
I'm completely new to web development and have been tasked to create a website from scratch. I've learned a fair deal of HTML and CSS and have started to get into Javascript and JQuery. I have tried to implement a fixed button that scrolls the page back up to the top that appears only after a certain distance has been scrolled. It works perfectly fine in Chrome, Internet Explorer and Firefoxon desktop, however in Edge the button appears but doesn't scroll the page at all. And on mobile the button doesn't even appear at all...
Here is the code for it:
HTML
<link href="Styles.css" rel="stylesheet" type="text/css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"> </script>
<script src="Scripts.js"></script>
<a href="#" id="scrollTop"><img src="RFImages/arrow.png" height="102%" width="98%"></a>
The link and scripts are in "head"and the a href is just underneath the closing tag for body.
CSS (File is Styles.css)
#scrollTop {
border-radius: 10px;
border: 5px solid #333333;
width:80px;
height:80px;
background-color: #000000;
position:fixed;
display: none;
opacity: 0.5;
right: 40px;
top: 30px;
}
#scrollTop:hover {
opacity: 1;
}
Most of the CSS is just styling and opacity change when hovering over it.
JS (File is Scripts.js)
$(document).ready(function () {
$(document).scroll(function() {
if ( $(document).scrollTop() < 300 ) {
$("#scrollTop").fadeOut();
}
if ( $(document).scrollTop() >= 300 ) {
$("#scrollTop").fadeIn();
}
});
$('#scrollTop').click(function () {
$("html").animate({
scrollTop: 10
}, 600);
return false;
});
});
This is JS that I learned and implemented myself.
This is a really frustrating compatibility issue that I've been trying to fix, however even though I've been trying really hard to learn JS and JQuery, I'm finding it difficult to find a simple and easy to understand solution that is not many 10s of lines long, and even then some of those ones don't work on mobile.
I tried using the jQuery Mobile library too hosted by Google but that did nothing to fix the issue.
So here is my question:
How can I go about fixing this code and make it work on mobile and in Edge in the simplest and easiest way to understand for someone who has only just started web development.
Thanks, Alex.
jquery html css cross-browser compatibility
add a comment |
EDIT: It now works on Edge thanks to Bowie, but the problem still persists on mobile, where the button doesn't appear at all.
I'm completely new to web development and have been tasked to create a website from scratch. I've learned a fair deal of HTML and CSS and have started to get into Javascript and JQuery. I have tried to implement a fixed button that scrolls the page back up to the top that appears only after a certain distance has been scrolled. It works perfectly fine in Chrome, Internet Explorer and Firefoxon desktop, however in Edge the button appears but doesn't scroll the page at all. And on mobile the button doesn't even appear at all...
Here is the code for it:
HTML
<link href="Styles.css" rel="stylesheet" type="text/css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"> </script>
<script src="Scripts.js"></script>
<a href="#" id="scrollTop"><img src="RFImages/arrow.png" height="102%" width="98%"></a>
The link and scripts are in "head"and the a href is just underneath the closing tag for body.
CSS (File is Styles.css)
#scrollTop {
border-radius: 10px;
border: 5px solid #333333;
width:80px;
height:80px;
background-color: #000000;
position:fixed;
display: none;
opacity: 0.5;
right: 40px;
top: 30px;
}
#scrollTop:hover {
opacity: 1;
}
Most of the CSS is just styling and opacity change when hovering over it.
JS (File is Scripts.js)
$(document).ready(function () {
$(document).scroll(function() {
if ( $(document).scrollTop() < 300 ) {
$("#scrollTop").fadeOut();
}
if ( $(document).scrollTop() >= 300 ) {
$("#scrollTop").fadeIn();
}
});
$('#scrollTop').click(function () {
$("html").animate({
scrollTop: 10
}, 600);
return false;
});
});
This is JS that I learned and implemented myself.
This is a really frustrating compatibility issue that I've been trying to fix, however even though I've been trying really hard to learn JS and JQuery, I'm finding it difficult to find a simple and easy to understand solution that is not many 10s of lines long, and even then some of those ones don't work on mobile.
I tried using the jQuery Mobile library too hosted by Google but that did nothing to fix the issue.
So here is my question:
How can I go about fixing this code and make it work on mobile and in Edge in the simplest and easiest way to understand for someone who has only just started web development.
Thanks, Alex.
jquery html css cross-browser compatibility
EDIT: It now works on Edge thanks to Bowie, but the problem still persists on mobile, where the button doesn't appear at all.
I'm completely new to web development and have been tasked to create a website from scratch. I've learned a fair deal of HTML and CSS and have started to get into Javascript and JQuery. I have tried to implement a fixed button that scrolls the page back up to the top that appears only after a certain distance has been scrolled. It works perfectly fine in Chrome, Internet Explorer and Firefoxon desktop, however in Edge the button appears but doesn't scroll the page at all. And on mobile the button doesn't even appear at all...
Here is the code for it:
HTML
<link href="Styles.css" rel="stylesheet" type="text/css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"> </script>
<script src="Scripts.js"></script>
<a href="#" id="scrollTop"><img src="RFImages/arrow.png" height="102%" width="98%"></a>
The link and scripts are in "head"and the a href is just underneath the closing tag for body.
CSS (File is Styles.css)
#scrollTop {
border-radius: 10px;
border: 5px solid #333333;
width:80px;
height:80px;
background-color: #000000;
position:fixed;
display: none;
opacity: 0.5;
right: 40px;
top: 30px;
}
#scrollTop:hover {
opacity: 1;
}
Most of the CSS is just styling and opacity change when hovering over it.
JS (File is Scripts.js)
$(document).ready(function () {
$(document).scroll(function() {
if ( $(document).scrollTop() < 300 ) {
$("#scrollTop").fadeOut();
}
if ( $(document).scrollTop() >= 300 ) {
$("#scrollTop").fadeIn();
}
});
$('#scrollTop').click(function () {
$("html").animate({
scrollTop: 10
}, 600);
return false;
});
});
This is JS that I learned and implemented myself.
This is a really frustrating compatibility issue that I've been trying to fix, however even though I've been trying really hard to learn JS and JQuery, I'm finding it difficult to find a simple and easy to understand solution that is not many 10s of lines long, and even then some of those ones don't work on mobile.
I tried using the jQuery Mobile library too hosted by Google but that did nothing to fix the issue.
So here is my question:
How can I go about fixing this code and make it work on mobile and in Edge in the simplest and easiest way to understand for someone who has only just started web development.
Thanks, Alex.
jquery html css cross-browser compatibility
jquery html css cross-browser compatibility
edited Nov 24 '18 at 8:27
asked Nov 23 '18 at 17:52
Alex
33
33
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Change $("html").animate
to $("html,body").animate
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451100%2fjquery-scroll-button-not-working-on-certain-browsers-and-mobile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Change $("html").animate
to $("html,body").animate
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
add a comment |
Change $("html").animate
to $("html,body").animate
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
add a comment |
Change $("html").animate
to $("html,body").animate
Change $("html").animate
to $("html,body").animate
answered Nov 23 '18 at 20:50
Bowie
1064
1064
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
add a comment |
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
Hi, I had that originally but that didn't work either. EDIT: I just tried it again and it worked. Maybe I had the syntax incorrect the first time? Dunno. At least it works on Edge now. Still need to fix it for mobile devices though. Thanks for the tip :)
– Alex
Nov 23 '18 at 21:58
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
No worries, different browsers use different controls regarding HTML or Body. If you could mark this correct I'd appreciate it!
– Bowie
Nov 23 '18 at 22:29
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53451100%2fjquery-scroll-button-not-working-on-certain-browsers-and-mobile%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0E9b2ygxL BUh25VR92hKjGqhT640l54mv DjrAPTCjJXBFG MTB