Is there an easy way to change pop up windows to modals (with minimal changes to JS code)
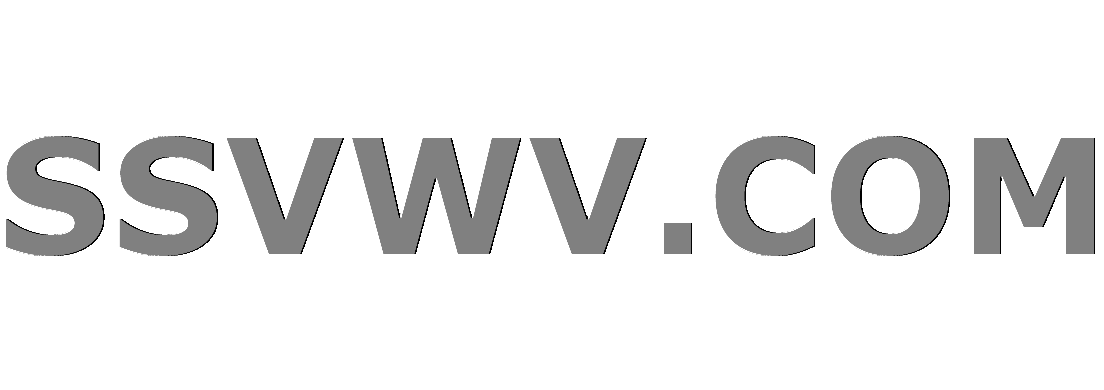
Multi tool use
I've seen several examples on SO of using modals rather than pop up windows and I'm trying to update some old JS code to do this without having to rewrite everything (as I'm very new to JS and don't understand a 99% of it yet).
The code we are using at the moment is included below. We're basically trying to get a christmas calender up and running for a local charity, so want the calender 'windows' to only open after specific dates and not before. Each 'window' has it's own html page with an image, a puzzle and clue, then a link to the charity (I've included one as an example, as all 24 are different). The calender is working great at the moment by using pop up windows. But we want to update the code to use modals (or something like that) instead, as having the window header/url bar looks clunky.
I suggested switching to modals as they look a lot 'cleaner' than windows, but if anyone has any better alternatives then I'd be happy to hear them.
Code for the main part of the calender:
<body>
<script>
function dooropen(door)
{
today=new Date();
daynow=today.getDate();
monthnow=today.getMonth();
if (monthnow!=11 && monthnow!=0) {alert("This calender unlocks in December. Please come back then."); return false;}
if (daynow==door-1) {alert("Come back tomorrow to see what's behind the door!");return false;}
if (door>daynow) {alert("You'll have to wait "+(door-daynow)+" days before this door will unlock!"); return false;}
oNewWindow=window.open(urlsarray[door], '_blank','directories=no,height='+heightarray[door]+',width='+widtharray[door]+',location=0,menubar=0,resizable=yes,scrollbars='+scrollbarsarray[door]+',status=no,titlebar=0,toolbar=0');
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray= new Array();
var x=285;
var y=50;
var rows=6;
var cols=4;
var spacinghoriz=120;
var spacingvert=100;
urlsarray[1]="1.html"; widtharray[1]="420"; heightarray[1]="550";
urlsarray[2]="2.html"; widtharray[2]="420"; heightarray[2]="550";
urlsarray[3]="3.html"; widtharray[3]="420"; heightarray[3]="550";
urlsarray[4]="4.html"; widtharray[4]="420"; heightarray[4]="550";
urlsarray[5]="5.html"; widtharray[5]="420"; heightarray[5]="550";
urlsarray[6]="6.html"; widtharray[6]="420"; heightarray[6]="550";
urlsarray[7]="7.html"; widtharray[7]="420"; heightarray[7]="550";
urlsarray[8]="8.html"; widtharray[8]="420"; heightarray[8]="550";
urlsarray[9]="9.html"; widtharray[9]="420"; heightarray[9]="550";
urlsarray[10]="10.html"; widtharray[10]="420"; heightarray[10]="550";
urlsarray[11]="11.html"; widtharray[11]="420"; heightarray[11]="550";
urlsarray[12]="12.html"; widtharray[12]="420"; heightarray[12]="550";
urlsarray[13]="13.html"; widtharray[13]="420"; heightarray[13]="550";
urlsarray[14]="14.html"; widtharray[14]="420"; heightarray[14]="550";
urlsarray[15]="15.html"; widtharray[15]="420"; heightarray[15]="550";
urlsarray[16]="16.html"; widtharray[16]="420"; heightarray[16]="550";
urlsarray[17]="17.html"; widtharray[17]="420"; heightarray[17]="550";
urlsarray[18]="18.html"; widtharray[18]="420"; heightarray[18]="550";
urlsarray[19]="19.html"; widtharray[19]="420"; heightarray[19]="550";
urlsarray[20]="20.html"; widtharray[20]="420"; heightarray[20]="550";
urlsarray[21]="21.html"; widtharray[21]="420"; heightarray[21]="550";
urlsarray[22]="22.html"; widtharray[22]="420"; heightarray[22]="550";
urlsarray[23]="23.html"; widtharray[23]="420"; heightarray[23]="550";
urlsarray[24]="24.html"; widtharray[24]="420"; heightarray[24]="550";
var doorno=1;
for (h=0;h<rows;++h)
{
for(g=0; g<cols; ++g, ++doorno)
{
document.write('<a class="button" onClick="dooropen('+doorno+');" href="#" style="position: absolute; left:'+(x+(g*spacinghoriz))+'px; top:'+(y+(h*spacingvert))+'px;">'+doorno+'</a>');}
}
</script>
</body>
Code for the main page, where the iframe will appear:
<html>
<head>
<title>Christmas Calender</title>
<link rel="shorcut icon" href="favicon.jpg" type="image/jpg">
<link rel="icon" href="favicon.jpg" type="image/x-icon">
</head>
<body style="background-color:#000000">
<center>
<iframe src="calendarcode.html" title="Christmas Calendar 2018" width=980 height=650 frameborder=0>
</iframe>
</center>
<!-- Button to return to previous room -->
<center>
<p><a href="room1.html"><img src="arrowleft.png" alt="Room1" width=97 height=97 border=0></a>
</center>
<!-- Logo -->
<center>
<a href=""><img src="logo.png" alt="charity name" width=40 height=40 border=0></a>
</center>
</body>
</html>
Code for example pop up window:
<html>
<head>
<title>Puzzle 1</title>
</head>
<body background-color="#000000">
<center>
<img src="images/puzzle1.gif" alt="Puzzle1" width=155 height=189 border=0>
<p>Clue text...</p>
<p>_ _ _ _ _</p>
<p><a href=""><img src="logo.png" alt="Logo" width=33 height=33 border=0></a></p>
</center>
</body>
</html>
javascript css html5
|
show 5 more comments
I've seen several examples on SO of using modals rather than pop up windows and I'm trying to update some old JS code to do this without having to rewrite everything (as I'm very new to JS and don't understand a 99% of it yet).
The code we are using at the moment is included below. We're basically trying to get a christmas calender up and running for a local charity, so want the calender 'windows' to only open after specific dates and not before. Each 'window' has it's own html page with an image, a puzzle and clue, then a link to the charity (I've included one as an example, as all 24 are different). The calender is working great at the moment by using pop up windows. But we want to update the code to use modals (or something like that) instead, as having the window header/url bar looks clunky.
I suggested switching to modals as they look a lot 'cleaner' than windows, but if anyone has any better alternatives then I'd be happy to hear them.
Code for the main part of the calender:
<body>
<script>
function dooropen(door)
{
today=new Date();
daynow=today.getDate();
monthnow=today.getMonth();
if (monthnow!=11 && monthnow!=0) {alert("This calender unlocks in December. Please come back then."); return false;}
if (daynow==door-1) {alert("Come back tomorrow to see what's behind the door!");return false;}
if (door>daynow) {alert("You'll have to wait "+(door-daynow)+" days before this door will unlock!"); return false;}
oNewWindow=window.open(urlsarray[door], '_blank','directories=no,height='+heightarray[door]+',width='+widtharray[door]+',location=0,menubar=0,resizable=yes,scrollbars='+scrollbarsarray[door]+',status=no,titlebar=0,toolbar=0');
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray= new Array();
var x=285;
var y=50;
var rows=6;
var cols=4;
var spacinghoriz=120;
var spacingvert=100;
urlsarray[1]="1.html"; widtharray[1]="420"; heightarray[1]="550";
urlsarray[2]="2.html"; widtharray[2]="420"; heightarray[2]="550";
urlsarray[3]="3.html"; widtharray[3]="420"; heightarray[3]="550";
urlsarray[4]="4.html"; widtharray[4]="420"; heightarray[4]="550";
urlsarray[5]="5.html"; widtharray[5]="420"; heightarray[5]="550";
urlsarray[6]="6.html"; widtharray[6]="420"; heightarray[6]="550";
urlsarray[7]="7.html"; widtharray[7]="420"; heightarray[7]="550";
urlsarray[8]="8.html"; widtharray[8]="420"; heightarray[8]="550";
urlsarray[9]="9.html"; widtharray[9]="420"; heightarray[9]="550";
urlsarray[10]="10.html"; widtharray[10]="420"; heightarray[10]="550";
urlsarray[11]="11.html"; widtharray[11]="420"; heightarray[11]="550";
urlsarray[12]="12.html"; widtharray[12]="420"; heightarray[12]="550";
urlsarray[13]="13.html"; widtharray[13]="420"; heightarray[13]="550";
urlsarray[14]="14.html"; widtharray[14]="420"; heightarray[14]="550";
urlsarray[15]="15.html"; widtharray[15]="420"; heightarray[15]="550";
urlsarray[16]="16.html"; widtharray[16]="420"; heightarray[16]="550";
urlsarray[17]="17.html"; widtharray[17]="420"; heightarray[17]="550";
urlsarray[18]="18.html"; widtharray[18]="420"; heightarray[18]="550";
urlsarray[19]="19.html"; widtharray[19]="420"; heightarray[19]="550";
urlsarray[20]="20.html"; widtharray[20]="420"; heightarray[20]="550";
urlsarray[21]="21.html"; widtharray[21]="420"; heightarray[21]="550";
urlsarray[22]="22.html"; widtharray[22]="420"; heightarray[22]="550";
urlsarray[23]="23.html"; widtharray[23]="420"; heightarray[23]="550";
urlsarray[24]="24.html"; widtharray[24]="420"; heightarray[24]="550";
var doorno=1;
for (h=0;h<rows;++h)
{
for(g=0; g<cols; ++g, ++doorno)
{
document.write('<a class="button" onClick="dooropen('+doorno+');" href="#" style="position: absolute; left:'+(x+(g*spacinghoriz))+'px; top:'+(y+(h*spacingvert))+'px;">'+doorno+'</a>');}
}
</script>
</body>
Code for the main page, where the iframe will appear:
<html>
<head>
<title>Christmas Calender</title>
<link rel="shorcut icon" href="favicon.jpg" type="image/jpg">
<link rel="icon" href="favicon.jpg" type="image/x-icon">
</head>
<body style="background-color:#000000">
<center>
<iframe src="calendarcode.html" title="Christmas Calendar 2018" width=980 height=650 frameborder=0>
</iframe>
</center>
<!-- Button to return to previous room -->
<center>
<p><a href="room1.html"><img src="arrowleft.png" alt="Room1" width=97 height=97 border=0></a>
</center>
<!-- Logo -->
<center>
<a href=""><img src="logo.png" alt="charity name" width=40 height=40 border=0></a>
</center>
</body>
</html>
Code for example pop up window:
<html>
<head>
<title>Puzzle 1</title>
</head>
<body background-color="#000000">
<center>
<img src="images/puzzle1.gif" alt="Puzzle1" width=155 height=189 border=0>
<p>Clue text...</p>
<p>_ _ _ _ _</p>
<p><a href=""><img src="logo.png" alt="Logo" width=33 height=33 border=0></a></p>
</center>
</body>
</html>
javascript css html5
Is it just thealerts
that you are looking to change (to modals)?
– OliverRadini
Nov 23 '18 at 14:01
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
It seems like those are also done withalert
s at the moment?
– OliverRadini
Nov 23 '18 at 14:08
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
1
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code usesalert
to display certain warnings, andwindow.open
to show the additional content
– OliverRadini
Nov 23 '18 at 14:28
|
show 5 more comments
I've seen several examples on SO of using modals rather than pop up windows and I'm trying to update some old JS code to do this without having to rewrite everything (as I'm very new to JS and don't understand a 99% of it yet).
The code we are using at the moment is included below. We're basically trying to get a christmas calender up and running for a local charity, so want the calender 'windows' to only open after specific dates and not before. Each 'window' has it's own html page with an image, a puzzle and clue, then a link to the charity (I've included one as an example, as all 24 are different). The calender is working great at the moment by using pop up windows. But we want to update the code to use modals (or something like that) instead, as having the window header/url bar looks clunky.
I suggested switching to modals as they look a lot 'cleaner' than windows, but if anyone has any better alternatives then I'd be happy to hear them.
Code for the main part of the calender:
<body>
<script>
function dooropen(door)
{
today=new Date();
daynow=today.getDate();
monthnow=today.getMonth();
if (monthnow!=11 && monthnow!=0) {alert("This calender unlocks in December. Please come back then."); return false;}
if (daynow==door-1) {alert("Come back tomorrow to see what's behind the door!");return false;}
if (door>daynow) {alert("You'll have to wait "+(door-daynow)+" days before this door will unlock!"); return false;}
oNewWindow=window.open(urlsarray[door], '_blank','directories=no,height='+heightarray[door]+',width='+widtharray[door]+',location=0,menubar=0,resizable=yes,scrollbars='+scrollbarsarray[door]+',status=no,titlebar=0,toolbar=0');
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray= new Array();
var x=285;
var y=50;
var rows=6;
var cols=4;
var spacinghoriz=120;
var spacingvert=100;
urlsarray[1]="1.html"; widtharray[1]="420"; heightarray[1]="550";
urlsarray[2]="2.html"; widtharray[2]="420"; heightarray[2]="550";
urlsarray[3]="3.html"; widtharray[3]="420"; heightarray[3]="550";
urlsarray[4]="4.html"; widtharray[4]="420"; heightarray[4]="550";
urlsarray[5]="5.html"; widtharray[5]="420"; heightarray[5]="550";
urlsarray[6]="6.html"; widtharray[6]="420"; heightarray[6]="550";
urlsarray[7]="7.html"; widtharray[7]="420"; heightarray[7]="550";
urlsarray[8]="8.html"; widtharray[8]="420"; heightarray[8]="550";
urlsarray[9]="9.html"; widtharray[9]="420"; heightarray[9]="550";
urlsarray[10]="10.html"; widtharray[10]="420"; heightarray[10]="550";
urlsarray[11]="11.html"; widtharray[11]="420"; heightarray[11]="550";
urlsarray[12]="12.html"; widtharray[12]="420"; heightarray[12]="550";
urlsarray[13]="13.html"; widtharray[13]="420"; heightarray[13]="550";
urlsarray[14]="14.html"; widtharray[14]="420"; heightarray[14]="550";
urlsarray[15]="15.html"; widtharray[15]="420"; heightarray[15]="550";
urlsarray[16]="16.html"; widtharray[16]="420"; heightarray[16]="550";
urlsarray[17]="17.html"; widtharray[17]="420"; heightarray[17]="550";
urlsarray[18]="18.html"; widtharray[18]="420"; heightarray[18]="550";
urlsarray[19]="19.html"; widtharray[19]="420"; heightarray[19]="550";
urlsarray[20]="20.html"; widtharray[20]="420"; heightarray[20]="550";
urlsarray[21]="21.html"; widtharray[21]="420"; heightarray[21]="550";
urlsarray[22]="22.html"; widtharray[22]="420"; heightarray[22]="550";
urlsarray[23]="23.html"; widtharray[23]="420"; heightarray[23]="550";
urlsarray[24]="24.html"; widtharray[24]="420"; heightarray[24]="550";
var doorno=1;
for (h=0;h<rows;++h)
{
for(g=0; g<cols; ++g, ++doorno)
{
document.write('<a class="button" onClick="dooropen('+doorno+');" href="#" style="position: absolute; left:'+(x+(g*spacinghoriz))+'px; top:'+(y+(h*spacingvert))+'px;">'+doorno+'</a>');}
}
</script>
</body>
Code for the main page, where the iframe will appear:
<html>
<head>
<title>Christmas Calender</title>
<link rel="shorcut icon" href="favicon.jpg" type="image/jpg">
<link rel="icon" href="favicon.jpg" type="image/x-icon">
</head>
<body style="background-color:#000000">
<center>
<iframe src="calendarcode.html" title="Christmas Calendar 2018" width=980 height=650 frameborder=0>
</iframe>
</center>
<!-- Button to return to previous room -->
<center>
<p><a href="room1.html"><img src="arrowleft.png" alt="Room1" width=97 height=97 border=0></a>
</center>
<!-- Logo -->
<center>
<a href=""><img src="logo.png" alt="charity name" width=40 height=40 border=0></a>
</center>
</body>
</html>
Code for example pop up window:
<html>
<head>
<title>Puzzle 1</title>
</head>
<body background-color="#000000">
<center>
<img src="images/puzzle1.gif" alt="Puzzle1" width=155 height=189 border=0>
<p>Clue text...</p>
<p>_ _ _ _ _</p>
<p><a href=""><img src="logo.png" alt="Logo" width=33 height=33 border=0></a></p>
</center>
</body>
</html>
javascript css html5
I've seen several examples on SO of using modals rather than pop up windows and I'm trying to update some old JS code to do this without having to rewrite everything (as I'm very new to JS and don't understand a 99% of it yet).
The code we are using at the moment is included below. We're basically trying to get a christmas calender up and running for a local charity, so want the calender 'windows' to only open after specific dates and not before. Each 'window' has it's own html page with an image, a puzzle and clue, then a link to the charity (I've included one as an example, as all 24 are different). The calender is working great at the moment by using pop up windows. But we want to update the code to use modals (or something like that) instead, as having the window header/url bar looks clunky.
I suggested switching to modals as they look a lot 'cleaner' than windows, but if anyone has any better alternatives then I'd be happy to hear them.
Code for the main part of the calender:
<body>
<script>
function dooropen(door)
{
today=new Date();
daynow=today.getDate();
monthnow=today.getMonth();
if (monthnow!=11 && monthnow!=0) {alert("This calender unlocks in December. Please come back then."); return false;}
if (daynow==door-1) {alert("Come back tomorrow to see what's behind the door!");return false;}
if (door>daynow) {alert("You'll have to wait "+(door-daynow)+" days before this door will unlock!"); return false;}
oNewWindow=window.open(urlsarray[door], '_blank','directories=no,height='+heightarray[door]+',width='+widtharray[door]+',location=0,menubar=0,resizable=yes,scrollbars='+scrollbarsarray[door]+',status=no,titlebar=0,toolbar=0');
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray= new Array();
var x=285;
var y=50;
var rows=6;
var cols=4;
var spacinghoriz=120;
var spacingvert=100;
urlsarray[1]="1.html"; widtharray[1]="420"; heightarray[1]="550";
urlsarray[2]="2.html"; widtharray[2]="420"; heightarray[2]="550";
urlsarray[3]="3.html"; widtharray[3]="420"; heightarray[3]="550";
urlsarray[4]="4.html"; widtharray[4]="420"; heightarray[4]="550";
urlsarray[5]="5.html"; widtharray[5]="420"; heightarray[5]="550";
urlsarray[6]="6.html"; widtharray[6]="420"; heightarray[6]="550";
urlsarray[7]="7.html"; widtharray[7]="420"; heightarray[7]="550";
urlsarray[8]="8.html"; widtharray[8]="420"; heightarray[8]="550";
urlsarray[9]="9.html"; widtharray[9]="420"; heightarray[9]="550";
urlsarray[10]="10.html"; widtharray[10]="420"; heightarray[10]="550";
urlsarray[11]="11.html"; widtharray[11]="420"; heightarray[11]="550";
urlsarray[12]="12.html"; widtharray[12]="420"; heightarray[12]="550";
urlsarray[13]="13.html"; widtharray[13]="420"; heightarray[13]="550";
urlsarray[14]="14.html"; widtharray[14]="420"; heightarray[14]="550";
urlsarray[15]="15.html"; widtharray[15]="420"; heightarray[15]="550";
urlsarray[16]="16.html"; widtharray[16]="420"; heightarray[16]="550";
urlsarray[17]="17.html"; widtharray[17]="420"; heightarray[17]="550";
urlsarray[18]="18.html"; widtharray[18]="420"; heightarray[18]="550";
urlsarray[19]="19.html"; widtharray[19]="420"; heightarray[19]="550";
urlsarray[20]="20.html"; widtharray[20]="420"; heightarray[20]="550";
urlsarray[21]="21.html"; widtharray[21]="420"; heightarray[21]="550";
urlsarray[22]="22.html"; widtharray[22]="420"; heightarray[22]="550";
urlsarray[23]="23.html"; widtharray[23]="420"; heightarray[23]="550";
urlsarray[24]="24.html"; widtharray[24]="420"; heightarray[24]="550";
var doorno=1;
for (h=0;h<rows;++h)
{
for(g=0; g<cols; ++g, ++doorno)
{
document.write('<a class="button" onClick="dooropen('+doorno+');" href="#" style="position: absolute; left:'+(x+(g*spacinghoriz))+'px; top:'+(y+(h*spacingvert))+'px;">'+doorno+'</a>');}
}
</script>
</body>
Code for the main page, where the iframe will appear:
<html>
<head>
<title>Christmas Calender</title>
<link rel="shorcut icon" href="favicon.jpg" type="image/jpg">
<link rel="icon" href="favicon.jpg" type="image/x-icon">
</head>
<body style="background-color:#000000">
<center>
<iframe src="calendarcode.html" title="Christmas Calendar 2018" width=980 height=650 frameborder=0>
</iframe>
</center>
<!-- Button to return to previous room -->
<center>
<p><a href="room1.html"><img src="arrowleft.png" alt="Room1" width=97 height=97 border=0></a>
</center>
<!-- Logo -->
<center>
<a href=""><img src="logo.png" alt="charity name" width=40 height=40 border=0></a>
</center>
</body>
</html>
Code for example pop up window:
<html>
<head>
<title>Puzzle 1</title>
</head>
<body background-color="#000000">
<center>
<img src="images/puzzle1.gif" alt="Puzzle1" width=155 height=189 border=0>
<p>Clue text...</p>
<p>_ _ _ _ _</p>
<p><a href=""><img src="logo.png" alt="Logo" width=33 height=33 border=0></a></p>
</center>
</body>
</html>
javascript css html5
javascript css html5
asked Nov 23 '18 at 13:46
Sudoku
287
287
Is it just thealerts
that you are looking to change (to modals)?
– OliverRadini
Nov 23 '18 at 14:01
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
It seems like those are also done withalert
s at the moment?
– OliverRadini
Nov 23 '18 at 14:08
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
1
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code usesalert
to display certain warnings, andwindow.open
to show the additional content
– OliverRadini
Nov 23 '18 at 14:28
|
show 5 more comments
Is it just thealerts
that you are looking to change (to modals)?
– OliverRadini
Nov 23 '18 at 14:01
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
It seems like those are also done withalert
s at the moment?
– OliverRadini
Nov 23 '18 at 14:08
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
1
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code usesalert
to display certain warnings, andwindow.open
to show the additional content
– OliverRadini
Nov 23 '18 at 14:28
Is it just the
alerts
that you are looking to change (to modals)?– OliverRadini
Nov 23 '18 at 14:01
Is it just the
alerts
that you are looking to change (to modals)?– OliverRadini
Nov 23 '18 at 14:01
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
It seems like those are also done with
alert
s at the moment?– OliverRadini
Nov 23 '18 at 14:08
It seems like those are also done with
alert
s at the moment?– OliverRadini
Nov 23 '18 at 14:08
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
1
1
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code uses
alert
to display certain warnings, and window.open
to show the additional content– OliverRadini
Nov 23 '18 at 14:28
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code uses
alert
to display certain warnings, and window.open
to show the additional content– OliverRadini
Nov 23 '18 at 14:28
|
show 5 more comments
1 Answer
1
active
oldest
votes
I'd recommend that you use a library here if you don't want to edit the code too heavily. I can't say that this is particularly good code, but I've tried to edit it as little as possible.
Here a new function, openModal
is being called instead of alert. You could replace the default window.alert
function but I think that'd complicate matters further.
This uses the tingle library for creating modals in javascript.
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
|
show 3 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447876%2fis-there-an-easy-way-to-change-pop-up-windows-to-modals-with-minimal-changes-to%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I'd recommend that you use a library here if you don't want to edit the code too heavily. I can't say that this is particularly good code, but I've tried to edit it as little as possible.
Here a new function, openModal
is being called instead of alert. You could replace the default window.alert
function but I think that'd complicate matters further.
This uses the tingle library for creating modals in javascript.
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
|
show 3 more comments
I'd recommend that you use a library here if you don't want to edit the code too heavily. I can't say that this is particularly good code, but I've tried to edit it as little as possible.
Here a new function, openModal
is being called instead of alert. You could replace the default window.alert
function but I think that'd complicate matters further.
This uses the tingle library for creating modals in javascript.
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
|
show 3 more comments
I'd recommend that you use a library here if you don't want to edit the code too heavily. I can't say that this is particularly good code, but I've tried to edit it as little as possible.
Here a new function, openModal
is being called instead of alert. You could replace the default window.alert
function but I think that'd complicate matters further.
This uses the tingle library for creating modals in javascript.
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
I'd recommend that you use a library here if you don't want to edit the code too heavily. I can't say that this is particularly good code, but I've tried to edit it as little as possible.
Here a new function, openModal
is being called instead of alert. You could replace the default window.alert
function but I think that'd complicate matters further.
This uses the tingle library for creating modals in javascript.
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
function createModal() {
return new tingle.modal({
footer: false,
stickyFooter: false,
closeMethods: ['overlay', 'button', 'escape'],
closeLabel: "Close",
cssClass: ['custom-class-1', 'custom-class-2'],
});
}
function createUrlModal(url) {
var newModal = createModal();
newModal.setContent('<iframe src="' + url + '"></iframe>');
return newModal;
}
function createTextModal(text) {
var newModal = createModal();
newModal.setContent('<h1>' + text + '</h1>');
return newModal;
}
function openModal(textOrUrl) {
var modalToOpen = textOrUrl.indexOf('html') > -1 || textOrUrl.indexOf('.com') > -1 ?
createUrlModal(textOrUrl) :
createTextModal(textOrUrl);
modalToOpen.open();
}
function dooropen(door) {
today = new Date();
daynow = today.getDate();
monthnow = today.getMonth();
if (monthnow != 11 && monthnow != 0) {
openModal("This calender unlocks in December. Please come back then.");
return false;
}
if (daynow == door - 1) {
openModal("Come back tomorrow to see what's behind the door!");
return false;
}
if (door > daynow) {
openModal("You'll have to wait " + (door - daynow) + " days before this door will unlock!");
return false;
}
oNewWindow = openModal(urlsarray[door]);
}
urlsarray = new Array();
widtharray = new Array();
heightarray = new Array();
scrollbarsarray = new Array();
var x = 285;
var y = 50;
var rows = 6;
var cols = 4;
var spacinghoriz = 120;
var spacingvert = 100;
urlsarray[1] = "https://fr.wikipedia.org/wiki/Main_Page";
widtharray[1] = "420";
heightarray[1] = "550";
urlsarray[2] = "2.html";
widtharray[2] = "420";
heightarray[2] = "550";
urlsarray[3] = "3.html";
widtharray[3] = "420";
heightarray[3] = "550";
urlsarray[4] = "4.html";
widtharray[4] = "420";
heightarray[4] = "550";
urlsarray[5] = "5.html";
widtharray[5] = "420";
heightarray[5] = "550";
urlsarray[6] = "6.html";
widtharray[6] = "420";
heightarray[6] = "550";
urlsarray[7] = "7.html";
widtharray[7] = "420";
heightarray[7] = "550";
urlsarray[8] = "8.html";
widtharray[8] = "420";
heightarray[8] = "550";
urlsarray[9] = "9.html";
widtharray[9] = "420";
heightarray[9] = "550";
urlsarray[10] = "10.html";
widtharray[10] = "420";
heightarray[10] = "550";
urlsarray[11] = "11.html";
widtharray[11] = "420";
heightarray[11] = "550";
urlsarray[12] = "12.html";
widtharray[12] = "420";
heightarray[12] = "550";
urlsarray[13] = "13.html";
widtharray[13] = "420";
heightarray[13] = "550";
urlsarray[14] = "14.html";
widtharray[14] = "420";
heightarray[14] = "550";
urlsarray[15] = "15.html";
widtharray[15] = "420";
heightarray[15] = "550";
urlsarray[16] = "16.html";
widtharray[16] = "420";
heightarray[16] = "550";
urlsarray[17] = "17.html";
widtharray[17] = "420";
heightarray[17] = "550";
urlsarray[18] = "18.html";
widtharray[18] = "420";
heightarray[18] = "550";
urlsarray[19] = "19.html";
widtharray[19] = "420";
heightarray[19] = "550";
urlsarray[20] = "20.html";
widtharray[20] = "420";
heightarray[20] = "550";
urlsarray[21] = "21.html";
widtharray[21] = "420";
heightarray[21] = "550";
urlsarray[22] = "22.html";
widtharray[22] = "420";
heightarray[22] = "550";
urlsarray[23] = "23.html";
widtharray[23] = "420";
heightarray[23] = "550";
urlsarray[24] = "24.html";
widtharray[24] = "420";
heightarray[24] = "550";
var doorno = 1;
for (h = 0; h < rows; ++h) {
for (g = 0; g < cols; ++g, ++doorno) {
document.write('<a class="button" onClick="dooropen(' + doorno + ');" href="#" style="position: absolute; left:' + (x + (g * spacinghoriz)) + 'px; top:' + (y + (h * spacingvert)) + 'px;">' + doorno + '</a>');
}
}
.tingle-modal-box__content {
padding: 0!important;
}
iframe {
width: 100%;
}
/* You can use the styles of the modal to adjust its size: */
.tingle-modal-box {
height: 500px;
width: 90%;
}
<link href="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.min.css" rel="stylesheet" />
<script src="https://cdnjs.cloudflare.com/ajax/libs/tingle/0.13.2/tingle.js"></script>
edited Nov 26 '18 at 8:28
answered Nov 23 '18 at 15:33


OliverRadini
2,653627
2,653627
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
|
show 3 more comments
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
This is amazing! I've 'sort of' got this working. Just trying to work out how to update the attributes/content of the modal windows so they look how the pop up windows on the original code looked. I've had a look at the tingle.js page and found the css to change the style of the contents. But can't work out how to change the size/shape of the modal box itself. Right now they are all the same size and not displaying things correctly. dropbox.com/sh/7h89j0rpnza06r6/AADldLrOSiPi7oeddA00SMBSa?dl=0
– Sudoku
Nov 23 '18 at 17:30
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I'm glad that's working; I've updated the answer to show how you might go about adjusting the size of the modal using css.
– OliverRadini
Nov 26 '18 at 8:29
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I tried the above already, but it doesn't seem to change the height. I've been playing around with different .css settings but it's as if the modal box is fixed to a specific shape. : (
– Sudoku
Nov 26 '18 at 22:52
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
I've marked your main answer as correct, as technically you solved my main issue (thank you!). If I can't get the modal windows to resize then I'll write another seperate question about the .css, as I guess that's another specific issue. I appreciate your help though! : )
– Sudoku
Nov 26 '18 at 23:14
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
@Sudoku I'm glad that helped; it probably is best to discuss the height issues as a separate question, as you'll likely get better help that way. For what it's worth, I've had problems styling the height of modals before, and I've yet to find a very good solution to the problem
– OliverRadini
Nov 27 '18 at 8:35
|
show 3 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447876%2fis-there-an-easy-way-to-change-pop-up-windows-to-modals-with-minimal-changes-to%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vZ2 w4vlbWyO3FN3kChQl3Kvlw wN24ahJ yS cqYwMfXI8jxCmm1hsURYAI 1iFURvc,qqAaY0S8,wu0hJA Ds8
Is it just the
alerts
that you are looking to change (to modals)?– OliverRadini
Nov 23 '18 at 14:01
And the individual pop ups for each day of the calender (in the array).
– Sudoku
Nov 23 '18 at 14:07
It seems like those are also done with
alert
s at the moment?– OliverRadini
Nov 23 '18 at 14:08
Oh, I see what you mean. Yep, all the alerts create pop up windows. We're trying to change those to modal boxes (or something similar) instead. Or perhaps there is a way to just hide the top of a browser window (the title bar, url bar, buttons, etc) in javascript like you can do with scroll bars?
– Sudoku
Nov 23 '18 at 14:10
1
The code does two separate things which, could, in theory be grouped into a single function, though it's likely to require fairly significant additions to the code. Currently the code uses
alert
to display certain warnings, andwindow.open
to show the additional content– OliverRadini
Nov 23 '18 at 14:28