Checking file existence on FTP server
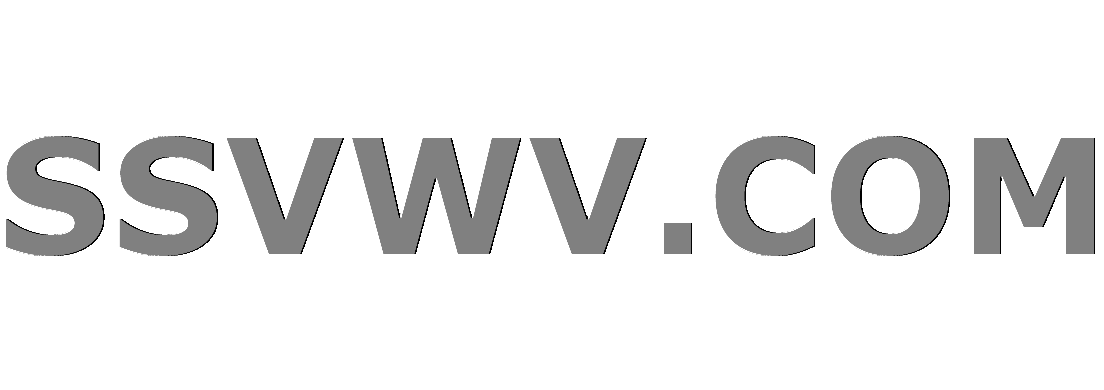
Multi tool use
Is there an efficient way to check the existence of a file on a FTP server? I'm using Apache Commons Net. I know that I can use the listNames
method of FTPClient
to get all the files in a specific directory and then I can go over this list to check if a given file exists, but I don't think it's efficient especially when the server contains a lot of files.
java ftp apache-commons-net
add a comment |
Is there an efficient way to check the existence of a file on a FTP server? I'm using Apache Commons Net. I know that I can use the listNames
method of FTPClient
to get all the files in a specific directory and then I can go over this list to check if a given file exists, but I don't think it's efficient especially when the server contains a lot of files.
java ftp apache-commons-net
add a comment |
Is there an efficient way to check the existence of a file on a FTP server? I'm using Apache Commons Net. I know that I can use the listNames
method of FTPClient
to get all the files in a specific directory and then I can go over this list to check if a given file exists, but I don't think it's efficient especially when the server contains a lot of files.
java ftp apache-commons-net
Is there an efficient way to check the existence of a file on a FTP server? I'm using Apache Commons Net. I know that I can use the listNames
method of FTPClient
to get all the files in a specific directory and then I can go over this list to check if a given file exists, but I don't think it's efficient especially when the server contains a lot of files.
java ftp apache-commons-net
java ftp apache-commons-net
edited May 18 '12 at 15:27


skaffman
339k85733721
339k85733721
asked May 7 '12 at 12:36
Mickael Marrache
2,96464996
2,96464996
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
listFiles(String pathName)
should work just fine for a single file.
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
add a comment |
Using a full path to a file in listFiles
(or mlistDir
) call, as the accepted answer shows, will indeed work for many FTP servers:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFiles = ftpClient.listFiles(remotePath );
if (remoteFiles.length > 0)
{
System.out.println("File " + remoteFiles[0].getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
But it actually violates an FTP specification as it maps to an FTP command
LIST /remote/path/file.txt
According to the specification, the FTP LIST
command accepts a path to a folder only.
Indeed most FTP servers can accept a file mask in the LIST
command (and an exact file name is kind of a mask too). But this is beyond the standard and not all FTP servers do support it (rightfully).
A portable code that works on any FTP server has to filter files locally:
FTPFile remoteFiles = ftpClient.listFiles("/remote/path");
Optional<FTPFile> remoteFile =
Arrays.stream(remoteFiles).filter(
(FTPFile remoteFile2) -> remoteFile2.getName().equals("file.txt")).findFirst();
if (remoteFile.isPresent())
{
System.out.println("File " + remoteFile.get().getName() + " exists");
}
else
{
System.out.println("File does not exists");
}
More efficient is to use mlistFile
(MLST
command), if the server supports it:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFile = ftpClient.mlistFile(remotePath);
if (remoteFile != null)
{
System.out.println("File " + remoteFile.getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method can be used to test an existence of a directory.
If the server does not support MLST
command, you can abuse getModificationTime
(MDTM
command):
String timestamp = ftpClient.getModificationTime(remotePath);
if (timestamp != null)
{
System.out.println("File " + remotePath + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method cannot be used to test an existence of a directory.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10482204%2fchecking-file-existence-on-ftp-server%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
listFiles(String pathName)
should work just fine for a single file.
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
add a comment |
listFiles(String pathName)
should work just fine for a single file.
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
add a comment |
listFiles(String pathName)
should work just fine for a single file.
listFiles(String pathName)
should work just fine for a single file.
answered May 7 '12 at 12:54
Christopher Creutzig
7,8202740
7,8202740
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
add a comment |
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
4
4
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
Thanks for your fast answer, I check the FTPFile array length to know if the file exists.
– Mickael Marrache
May 7 '12 at 13:29
add a comment |
Using a full path to a file in listFiles
(or mlistDir
) call, as the accepted answer shows, will indeed work for many FTP servers:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFiles = ftpClient.listFiles(remotePath );
if (remoteFiles.length > 0)
{
System.out.println("File " + remoteFiles[0].getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
But it actually violates an FTP specification as it maps to an FTP command
LIST /remote/path/file.txt
According to the specification, the FTP LIST
command accepts a path to a folder only.
Indeed most FTP servers can accept a file mask in the LIST
command (and an exact file name is kind of a mask too). But this is beyond the standard and not all FTP servers do support it (rightfully).
A portable code that works on any FTP server has to filter files locally:
FTPFile remoteFiles = ftpClient.listFiles("/remote/path");
Optional<FTPFile> remoteFile =
Arrays.stream(remoteFiles).filter(
(FTPFile remoteFile2) -> remoteFile2.getName().equals("file.txt")).findFirst();
if (remoteFile.isPresent())
{
System.out.println("File " + remoteFile.get().getName() + " exists");
}
else
{
System.out.println("File does not exists");
}
More efficient is to use mlistFile
(MLST
command), if the server supports it:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFile = ftpClient.mlistFile(remotePath);
if (remoteFile != null)
{
System.out.println("File " + remoteFile.getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method can be used to test an existence of a directory.
If the server does not support MLST
command, you can abuse getModificationTime
(MDTM
command):
String timestamp = ftpClient.getModificationTime(remotePath);
if (timestamp != null)
{
System.out.println("File " + remotePath + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method cannot be used to test an existence of a directory.
add a comment |
Using a full path to a file in listFiles
(or mlistDir
) call, as the accepted answer shows, will indeed work for many FTP servers:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFiles = ftpClient.listFiles(remotePath );
if (remoteFiles.length > 0)
{
System.out.println("File " + remoteFiles[0].getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
But it actually violates an FTP specification as it maps to an FTP command
LIST /remote/path/file.txt
According to the specification, the FTP LIST
command accepts a path to a folder only.
Indeed most FTP servers can accept a file mask in the LIST
command (and an exact file name is kind of a mask too). But this is beyond the standard and not all FTP servers do support it (rightfully).
A portable code that works on any FTP server has to filter files locally:
FTPFile remoteFiles = ftpClient.listFiles("/remote/path");
Optional<FTPFile> remoteFile =
Arrays.stream(remoteFiles).filter(
(FTPFile remoteFile2) -> remoteFile2.getName().equals("file.txt")).findFirst();
if (remoteFile.isPresent())
{
System.out.println("File " + remoteFile.get().getName() + " exists");
}
else
{
System.out.println("File does not exists");
}
More efficient is to use mlistFile
(MLST
command), if the server supports it:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFile = ftpClient.mlistFile(remotePath);
if (remoteFile != null)
{
System.out.println("File " + remoteFile.getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method can be used to test an existence of a directory.
If the server does not support MLST
command, you can abuse getModificationTime
(MDTM
command):
String timestamp = ftpClient.getModificationTime(remotePath);
if (timestamp != null)
{
System.out.println("File " + remotePath + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method cannot be used to test an existence of a directory.
add a comment |
Using a full path to a file in listFiles
(or mlistDir
) call, as the accepted answer shows, will indeed work for many FTP servers:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFiles = ftpClient.listFiles(remotePath );
if (remoteFiles.length > 0)
{
System.out.println("File " + remoteFiles[0].getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
But it actually violates an FTP specification as it maps to an FTP command
LIST /remote/path/file.txt
According to the specification, the FTP LIST
command accepts a path to a folder only.
Indeed most FTP servers can accept a file mask in the LIST
command (and an exact file name is kind of a mask too). But this is beyond the standard and not all FTP servers do support it (rightfully).
A portable code that works on any FTP server has to filter files locally:
FTPFile remoteFiles = ftpClient.listFiles("/remote/path");
Optional<FTPFile> remoteFile =
Arrays.stream(remoteFiles).filter(
(FTPFile remoteFile2) -> remoteFile2.getName().equals("file.txt")).findFirst();
if (remoteFile.isPresent())
{
System.out.println("File " + remoteFile.get().getName() + " exists");
}
else
{
System.out.println("File does not exists");
}
More efficient is to use mlistFile
(MLST
command), if the server supports it:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFile = ftpClient.mlistFile(remotePath);
if (remoteFile != null)
{
System.out.println("File " + remoteFile.getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method can be used to test an existence of a directory.
If the server does not support MLST
command, you can abuse getModificationTime
(MDTM
command):
String timestamp = ftpClient.getModificationTime(remotePath);
if (timestamp != null)
{
System.out.println("File " + remotePath + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method cannot be used to test an existence of a directory.
Using a full path to a file in listFiles
(or mlistDir
) call, as the accepted answer shows, will indeed work for many FTP servers:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFiles = ftpClient.listFiles(remotePath );
if (remoteFiles.length > 0)
{
System.out.println("File " + remoteFiles[0].getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
But it actually violates an FTP specification as it maps to an FTP command
LIST /remote/path/file.txt
According to the specification, the FTP LIST
command accepts a path to a folder only.
Indeed most FTP servers can accept a file mask in the LIST
command (and an exact file name is kind of a mask too). But this is beyond the standard and not all FTP servers do support it (rightfully).
A portable code that works on any FTP server has to filter files locally:
FTPFile remoteFiles = ftpClient.listFiles("/remote/path");
Optional<FTPFile> remoteFile =
Arrays.stream(remoteFiles).filter(
(FTPFile remoteFile2) -> remoteFile2.getName().equals("file.txt")).findFirst();
if (remoteFile.isPresent())
{
System.out.println("File " + remoteFile.get().getName() + " exists");
}
else
{
System.out.println("File does not exists");
}
More efficient is to use mlistFile
(MLST
command), if the server supports it:
String remotePath = "/remote/path/file.txt";
FTPFile remoteFile = ftpClient.mlistFile(remotePath);
if (remoteFile != null)
{
System.out.println("File " + remoteFile.getName() + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method can be used to test an existence of a directory.
If the server does not support MLST
command, you can abuse getModificationTime
(MDTM
command):
String timestamp = ftpClient.getModificationTime(remotePath);
if (timestamp != null)
{
System.out.println("File " + remotePath + " exists");
}
else
{
System.out.println("File " + remotePath + " does not exists");
}
This method cannot be used to test an existence of a directory.
edited Nov 7 '18 at 15:57
answered Apr 17 '18 at 11:46
Martin Prikryl
85.6k22164357
85.6k22164357
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f10482204%2fchecking-file-existence-on-ftp-server%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
FhqINSHE,onoJdm7kv87Ged0fdO,RIXHk9ECv mKWPeTwV,wyJPsjrk7LsF4Vd1euTmpTk1dU,f 3z