Getting several buttons to stay active when the page refreshes?
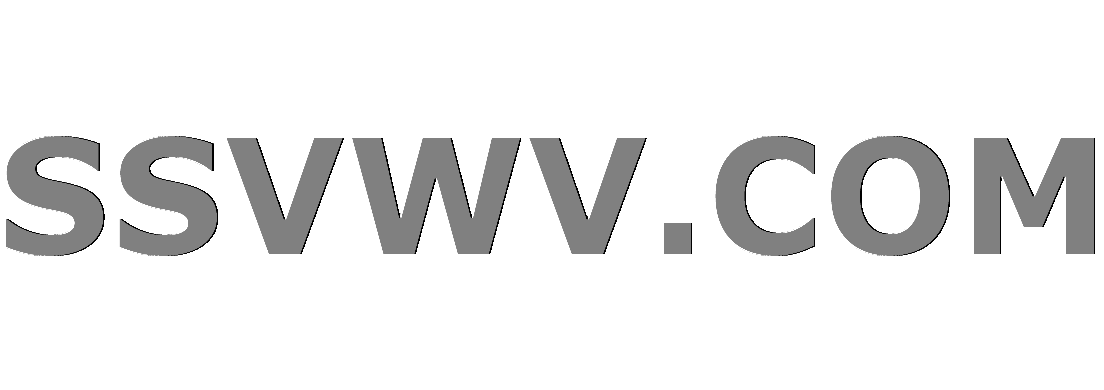
Multi tool use
I've been working on a table for a class project for awhile, and it is working pretty well (thanks to a lot of contributions I found here)
I am having problems getting buttons to stay active when the page is reloaded. I am using jQuery, and have worked with localStorage
and sessionStorage
quite a bit without results. Any help would be greatly appreciated!
Here's the snippet:
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
javascript jquery html session-storage
add a comment |
I've been working on a table for a class project for awhile, and it is working pretty well (thanks to a lot of contributions I found here)
I am having problems getting buttons to stay active when the page is reloaded. I am using jQuery, and have worked with localStorage
and sessionStorage
quite a bit without results. Any help would be greatly appreciated!
Here's the snippet:
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
javascript jquery html session-storage
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08
add a comment |
I've been working on a table for a class project for awhile, and it is working pretty well (thanks to a lot of contributions I found here)
I am having problems getting buttons to stay active when the page is reloaded. I am using jQuery, and have worked with localStorage
and sessionStorage
quite a bit without results. Any help would be greatly appreciated!
Here's the snippet:
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
javascript jquery html session-storage
I've been working on a table for a class project for awhile, and it is working pretty well (thanks to a lot of contributions I found here)
I am having problems getting buttons to stay active when the page is reloaded. I am using jQuery, and have worked with localStorage
and sessionStorage
quite a bit without results. Any help would be greatly appreciated!
Here's the snippet:
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
function toggle(event) { //this is what happens when you click on a button
$(this).toggleClass('active'); //adds an "active" class (color changes)
sessionStorage.setItem("activeDiv", $(this).index('.btn')); //i need help with this
}
$(function() {
"use strict";
$(".btn").on("click ", toggle); // you can set the buttons active or not
var activebtn = sessionStorage.getItem("activeDiv");
if (activebtn) { //I need help with this
$('.btn').toggleClass('active').eq(activebtn).toggleClass('active');
}
});
table,
tr,
td {
background-color: #a3bfd9;
border: solid 2px #41403E;
border-collapse: collapse;
}
.btn {
vertical-align: top;
background-color: #ebd3eb;
border-color: #93e2ff;
font-size: 10px;
width: 100px;
height: 30px;
text-align: center;
text-decoration: none;
display: inline-block;
cursor: pointer;
border-radius: 5px;
}
.btn:hover {
background-color: #93e2ff;
opacity: 0.7;
/*color: #5fc0e3;*/
border-color: #5fc0e3;
transition: 0.3s;
}
.btn.active {
background-color: #899c30;
color: #fff;
border-color: #005fbf;
transition: 0.3s;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/2.1.1/jquery.min.js"></script>
<div class="obsimotable">
<table>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="13">Some class 13op</button>
<br>
</td>
<td></td>
</tr>
<tr>
<td>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="5">Some class 5op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
</tr>
<tr>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="6">Some class 6op</button>
<br>
</td>
<td>
<button class="btn" value="6">Some class 6op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="4">Some class 4op</button>
<br>
<button class="btn" value="4">Some class 4op</button>
<br>
</td>
<td>
<button class="btn" value="3">Some class 3op</button>
<br>
</td>
</tr>
</table>
javascript jquery html session-storage
javascript jquery html session-storage
asked Nov 23 '18 at 13:33
I_Literally_Cannot
185
185
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08
add a comment |
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08
add a comment |
2 Answers
2
active
oldest
votes
As I said in my earlier comment, one way would be to use JSON.stringify
and JSON.parse
as you need to store multiple values (multiple buttons), but local (and session) storage only allows to save strings.
Code is commented so it should be easy to understand what is going on.
function toggle(event) {
let btnIndex = $(this).index('.btn');
let activeButtons = ;
if (!sessionStorage.getItem("activeButtons")) {
// Session storage item doesn't exist yet -> Create it
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
// Get active buttons from session storage
activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if ($(this).hasClass('active')) {
// Button has active class -> Remove from activeButtons array
var index = activeButtons.indexOf(btnIndex);
if (index !== -1) activeButtons.splice(index, 1);
} else {
// Button doesn't have active class -> Push to activeButtons array
activeButtons.push(btnIndex);
}
// Toggle active class
$(this).toggleClass('active');
// Set items in session storage
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
$(function() {
//sessionStorage.clear(); // Uncomment this line to clear session storage
$(".btn").on("click ", toggle);
// Load active buttons
let activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if (activeButtons.length) {
// Loop through active buttons
for (var i = 0; i < activeButtons.length; i++) {
// Add active class for each active button
$('.btn').eq(activeButtons[i]).addClass('active');
}
}
});
Here is a working fiddle.
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
add a comment |
Here's my solution: https://codepen.io/HerrSerker/pen/vQjYGw?editors=1011
This is in a codepen, because the SO snippets don't allow to use sessionStorage (for apparent reasons).
Anyway the JS code is like this:
jQuery(function($) {
"use strict";
var activeButtons =
var selector = '.btn';
var storageKey = "activeButtons";
var activeClassName = 'active';
readStorage();
$(selector).on('click', reactClick);
function readStorage() {
var storage = sessionStorage.getItem(storageKey);
if (storage) {
activeButtons = storage.split(',');
}
$(selector)
.filter(function(i, e) {
return activeButtons.indexOf(""+i) !== -1
})
.toggleClass(activeClassName)
}
function reactClick() {
$(this).toggleClass(activeClassName);
var index = $(this).index(selector);
toggleArrayItem(activeButtons, ""+index);
sessionStorage.setItem(storageKey, activeButtons)
}
function toggleArrayItem(a, v) {
var i = a.indexOf(v);
if (i === -1)
a.push(v);
else
a.splice(i,1);
}
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447691%2fgetting-several-buttons-to-stay-active-when-the-page-refreshes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
As I said in my earlier comment, one way would be to use JSON.stringify
and JSON.parse
as you need to store multiple values (multiple buttons), but local (and session) storage only allows to save strings.
Code is commented so it should be easy to understand what is going on.
function toggle(event) {
let btnIndex = $(this).index('.btn');
let activeButtons = ;
if (!sessionStorage.getItem("activeButtons")) {
// Session storage item doesn't exist yet -> Create it
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
// Get active buttons from session storage
activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if ($(this).hasClass('active')) {
// Button has active class -> Remove from activeButtons array
var index = activeButtons.indexOf(btnIndex);
if (index !== -1) activeButtons.splice(index, 1);
} else {
// Button doesn't have active class -> Push to activeButtons array
activeButtons.push(btnIndex);
}
// Toggle active class
$(this).toggleClass('active');
// Set items in session storage
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
$(function() {
//sessionStorage.clear(); // Uncomment this line to clear session storage
$(".btn").on("click ", toggle);
// Load active buttons
let activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if (activeButtons.length) {
// Loop through active buttons
for (var i = 0; i < activeButtons.length; i++) {
// Add active class for each active button
$('.btn').eq(activeButtons[i]).addClass('active');
}
}
});
Here is a working fiddle.
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
add a comment |
As I said in my earlier comment, one way would be to use JSON.stringify
and JSON.parse
as you need to store multiple values (multiple buttons), but local (and session) storage only allows to save strings.
Code is commented so it should be easy to understand what is going on.
function toggle(event) {
let btnIndex = $(this).index('.btn');
let activeButtons = ;
if (!sessionStorage.getItem("activeButtons")) {
// Session storage item doesn't exist yet -> Create it
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
// Get active buttons from session storage
activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if ($(this).hasClass('active')) {
// Button has active class -> Remove from activeButtons array
var index = activeButtons.indexOf(btnIndex);
if (index !== -1) activeButtons.splice(index, 1);
} else {
// Button doesn't have active class -> Push to activeButtons array
activeButtons.push(btnIndex);
}
// Toggle active class
$(this).toggleClass('active');
// Set items in session storage
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
$(function() {
//sessionStorage.clear(); // Uncomment this line to clear session storage
$(".btn").on("click ", toggle);
// Load active buttons
let activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if (activeButtons.length) {
// Loop through active buttons
for (var i = 0; i < activeButtons.length; i++) {
// Add active class for each active button
$('.btn').eq(activeButtons[i]).addClass('active');
}
}
});
Here is a working fiddle.
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
add a comment |
As I said in my earlier comment, one way would be to use JSON.stringify
and JSON.parse
as you need to store multiple values (multiple buttons), but local (and session) storage only allows to save strings.
Code is commented so it should be easy to understand what is going on.
function toggle(event) {
let btnIndex = $(this).index('.btn');
let activeButtons = ;
if (!sessionStorage.getItem("activeButtons")) {
// Session storage item doesn't exist yet -> Create it
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
// Get active buttons from session storage
activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if ($(this).hasClass('active')) {
// Button has active class -> Remove from activeButtons array
var index = activeButtons.indexOf(btnIndex);
if (index !== -1) activeButtons.splice(index, 1);
} else {
// Button doesn't have active class -> Push to activeButtons array
activeButtons.push(btnIndex);
}
// Toggle active class
$(this).toggleClass('active');
// Set items in session storage
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
$(function() {
//sessionStorage.clear(); // Uncomment this line to clear session storage
$(".btn").on("click ", toggle);
// Load active buttons
let activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if (activeButtons.length) {
// Loop through active buttons
for (var i = 0; i < activeButtons.length; i++) {
// Add active class for each active button
$('.btn').eq(activeButtons[i]).addClass('active');
}
}
});
Here is a working fiddle.
As I said in my earlier comment, one way would be to use JSON.stringify
and JSON.parse
as you need to store multiple values (multiple buttons), but local (and session) storage only allows to save strings.
Code is commented so it should be easy to understand what is going on.
function toggle(event) {
let btnIndex = $(this).index('.btn');
let activeButtons = ;
if (!sessionStorage.getItem("activeButtons")) {
// Session storage item doesn't exist yet -> Create it
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
// Get active buttons from session storage
activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if ($(this).hasClass('active')) {
// Button has active class -> Remove from activeButtons array
var index = activeButtons.indexOf(btnIndex);
if (index !== -1) activeButtons.splice(index, 1);
} else {
// Button doesn't have active class -> Push to activeButtons array
activeButtons.push(btnIndex);
}
// Toggle active class
$(this).toggleClass('active');
// Set items in session storage
sessionStorage.setItem("activeButtons", JSON.stringify(activeButtons));
}
$(function() {
//sessionStorage.clear(); // Uncomment this line to clear session storage
$(".btn").on("click ", toggle);
// Load active buttons
let activeButtons = JSON.parse(sessionStorage.getItem("activeButtons"));
if (activeButtons.length) {
// Loop through active buttons
for (var i = 0; i < activeButtons.length; i++) {
// Add active class for each active button
$('.btn').eq(activeButtons[i]).addClass('active');
}
}
});
Here is a working fiddle.
answered Nov 23 '18 at 14:41


MrUpsidown
14.7k74894
14.7k74894
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
add a comment |
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
1
1
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
Oh wow. I did sort of get something from setting a cookie and calling the cookies on reload, but this makes a lot more sense!
– I_Literally_Cannot
Nov 23 '18 at 21:41
add a comment |
Here's my solution: https://codepen.io/HerrSerker/pen/vQjYGw?editors=1011
This is in a codepen, because the SO snippets don't allow to use sessionStorage (for apparent reasons).
Anyway the JS code is like this:
jQuery(function($) {
"use strict";
var activeButtons =
var selector = '.btn';
var storageKey = "activeButtons";
var activeClassName = 'active';
readStorage();
$(selector).on('click', reactClick);
function readStorage() {
var storage = sessionStorage.getItem(storageKey);
if (storage) {
activeButtons = storage.split(',');
}
$(selector)
.filter(function(i, e) {
return activeButtons.indexOf(""+i) !== -1
})
.toggleClass(activeClassName)
}
function reactClick() {
$(this).toggleClass(activeClassName);
var index = $(this).index(selector);
toggleArrayItem(activeButtons, ""+index);
sessionStorage.setItem(storageKey, activeButtons)
}
function toggleArrayItem(a, v) {
var i = a.indexOf(v);
if (i === -1)
a.push(v);
else
a.splice(i,1);
}
});
add a comment |
Here's my solution: https://codepen.io/HerrSerker/pen/vQjYGw?editors=1011
This is in a codepen, because the SO snippets don't allow to use sessionStorage (for apparent reasons).
Anyway the JS code is like this:
jQuery(function($) {
"use strict";
var activeButtons =
var selector = '.btn';
var storageKey = "activeButtons";
var activeClassName = 'active';
readStorage();
$(selector).on('click', reactClick);
function readStorage() {
var storage = sessionStorage.getItem(storageKey);
if (storage) {
activeButtons = storage.split(',');
}
$(selector)
.filter(function(i, e) {
return activeButtons.indexOf(""+i) !== -1
})
.toggleClass(activeClassName)
}
function reactClick() {
$(this).toggleClass(activeClassName);
var index = $(this).index(selector);
toggleArrayItem(activeButtons, ""+index);
sessionStorage.setItem(storageKey, activeButtons)
}
function toggleArrayItem(a, v) {
var i = a.indexOf(v);
if (i === -1)
a.push(v);
else
a.splice(i,1);
}
});
add a comment |
Here's my solution: https://codepen.io/HerrSerker/pen/vQjYGw?editors=1011
This is in a codepen, because the SO snippets don't allow to use sessionStorage (for apparent reasons).
Anyway the JS code is like this:
jQuery(function($) {
"use strict";
var activeButtons =
var selector = '.btn';
var storageKey = "activeButtons";
var activeClassName = 'active';
readStorage();
$(selector).on('click', reactClick);
function readStorage() {
var storage = sessionStorage.getItem(storageKey);
if (storage) {
activeButtons = storage.split(',');
}
$(selector)
.filter(function(i, e) {
return activeButtons.indexOf(""+i) !== -1
})
.toggleClass(activeClassName)
}
function reactClick() {
$(this).toggleClass(activeClassName);
var index = $(this).index(selector);
toggleArrayItem(activeButtons, ""+index);
sessionStorage.setItem(storageKey, activeButtons)
}
function toggleArrayItem(a, v) {
var i = a.indexOf(v);
if (i === -1)
a.push(v);
else
a.splice(i,1);
}
});
Here's my solution: https://codepen.io/HerrSerker/pen/vQjYGw?editors=1011
This is in a codepen, because the SO snippets don't allow to use sessionStorage (for apparent reasons).
Anyway the JS code is like this:
jQuery(function($) {
"use strict";
var activeButtons =
var selector = '.btn';
var storageKey = "activeButtons";
var activeClassName = 'active';
readStorage();
$(selector).on('click', reactClick);
function readStorage() {
var storage = sessionStorage.getItem(storageKey);
if (storage) {
activeButtons = storage.split(',');
}
$(selector)
.filter(function(i, e) {
return activeButtons.indexOf(""+i) !== -1
})
.toggleClass(activeClassName)
}
function reactClick() {
$(this).toggleClass(activeClassName);
var index = $(this).index(selector);
toggleArrayItem(activeButtons, ""+index);
sessionStorage.setItem(storageKey, activeButtons)
}
function toggleArrayItem(a, v) {
var i = a.indexOf(v);
if (i === -1)
a.push(v);
else
a.splice(i,1);
}
});
edited Nov 23 '18 at 14:29
answered Nov 23 '18 at 14:17
HerrSerker
19.8k84778
19.8k84778
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53447691%2fgetting-several-buttons-to-stay-active-when-the-page-refreshes%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Co 4OkGRtf,iS50nJ5bY1Sau,0sQB6t7scvzhnRVdCl3fuIfDFdu,kqj76,0b88uZATyHM0mQN
You would need to save an array containing all indexes of the selected buttons. Session storage can only hold strings. You would need to JSON stringify your array to store the values as a string. On reloading you need to parse that string and check which button needs to be toggled and handle selecting/unselecting an item... so that sounds a lot more complex than what you are doing in the code you shared.
– MrUpsidown
Nov 23 '18 at 14:08