Create a new node with the given parameter info & add a new node
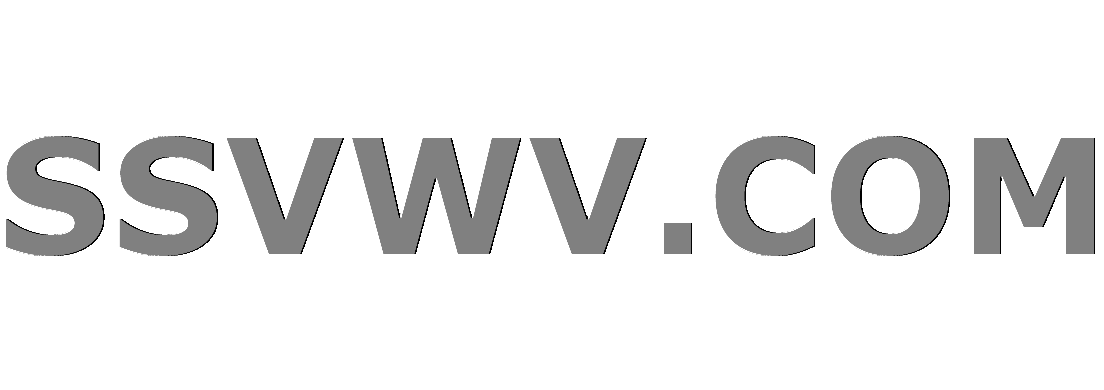
Multi tool use
I have a constructor, destructor, a function that returns to the head of the Linked list. I am not sure about the createNode function and couldn't figure out how to do the addNode function.
//constructor
LinkedList::LinkedList()
{
head = NULL;
}
//destructor
LinkedList::~LinkedList()
{
struct Node *temp = head;
int nodeCount = 0;
struct Node *nextNode;
while (temp != NULL)
{
nextNode = temp->next;
delete temp;
nodeCount++;
temp = nextNode;
}
head = NULL;
cout << "The number of deleted nodes is: " << nodeCount << "n";
}
//This function returns the head of the linked list
Node *LinkedList::getHead()
{
return head;
}
//This function creates a new node with the given parameter info. It returns a pointer that points to the newly created node.
Node * LinkedList::createNode(string name, int winScore, int loseScore)
{
Node *temp = new Node;
temp->name = name;
temp->winScore = winScore;
temp->loseScore = loseScore;
temp->next = NULL;
return new Node; // I think I have done this function right but please correct me if I'm wrong
}
//The function calls createnode first. It then insert the newly created node inside the linked list in alphabetical order. It also returns a pointer that
points to the newly added node.
Node *LinkedList::addNode(string name, int winScore, int loseScore)
{
//add code here which I couldn't do
}
c++ linked-list
add a comment |
I have a constructor, destructor, a function that returns to the head of the Linked list. I am not sure about the createNode function and couldn't figure out how to do the addNode function.
//constructor
LinkedList::LinkedList()
{
head = NULL;
}
//destructor
LinkedList::~LinkedList()
{
struct Node *temp = head;
int nodeCount = 0;
struct Node *nextNode;
while (temp != NULL)
{
nextNode = temp->next;
delete temp;
nodeCount++;
temp = nextNode;
}
head = NULL;
cout << "The number of deleted nodes is: " << nodeCount << "n";
}
//This function returns the head of the linked list
Node *LinkedList::getHead()
{
return head;
}
//This function creates a new node with the given parameter info. It returns a pointer that points to the newly created node.
Node * LinkedList::createNode(string name, int winScore, int loseScore)
{
Node *temp = new Node;
temp->name = name;
temp->winScore = winScore;
temp->loseScore = loseScore;
temp->next = NULL;
return new Node; // I think I have done this function right but please correct me if I'm wrong
}
//The function calls createnode first. It then insert the newly created node inside the linked list in alphabetical order. It also returns a pointer that
points to the newly added node.
Node *LinkedList::addNode(string name, int winScore, int loseScore)
{
//add code here which I couldn't do
}
c++ linked-list
addNode
will allocate a new node, set the data value andnext
pointer toNULL
then check ifhead = NULL
(if so just sethead = newnode;
for the 1st node case), then yourelse
case will use a temporary pointer set to head and then iteratewhile (iter->next != NULL)
when that loop fails,iter
points to the last node, so just setiter->next = newnode;
(there are many many examples of the logic) Also, if you keep alast
pointer, you can avoid iterating to find the last node -- up to you. (you can have separatecreateNode
andaddNode
functions - or combine them, up to you)
– David C. Rankin
Nov 25 '18 at 4:40
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49
add a comment |
I have a constructor, destructor, a function that returns to the head of the Linked list. I am not sure about the createNode function and couldn't figure out how to do the addNode function.
//constructor
LinkedList::LinkedList()
{
head = NULL;
}
//destructor
LinkedList::~LinkedList()
{
struct Node *temp = head;
int nodeCount = 0;
struct Node *nextNode;
while (temp != NULL)
{
nextNode = temp->next;
delete temp;
nodeCount++;
temp = nextNode;
}
head = NULL;
cout << "The number of deleted nodes is: " << nodeCount << "n";
}
//This function returns the head of the linked list
Node *LinkedList::getHead()
{
return head;
}
//This function creates a new node with the given parameter info. It returns a pointer that points to the newly created node.
Node * LinkedList::createNode(string name, int winScore, int loseScore)
{
Node *temp = new Node;
temp->name = name;
temp->winScore = winScore;
temp->loseScore = loseScore;
temp->next = NULL;
return new Node; // I think I have done this function right but please correct me if I'm wrong
}
//The function calls createnode first. It then insert the newly created node inside the linked list in alphabetical order. It also returns a pointer that
points to the newly added node.
Node *LinkedList::addNode(string name, int winScore, int loseScore)
{
//add code here which I couldn't do
}
c++ linked-list
I have a constructor, destructor, a function that returns to the head of the Linked list. I am not sure about the createNode function and couldn't figure out how to do the addNode function.
//constructor
LinkedList::LinkedList()
{
head = NULL;
}
//destructor
LinkedList::~LinkedList()
{
struct Node *temp = head;
int nodeCount = 0;
struct Node *nextNode;
while (temp != NULL)
{
nextNode = temp->next;
delete temp;
nodeCount++;
temp = nextNode;
}
head = NULL;
cout << "The number of deleted nodes is: " << nodeCount << "n";
}
//This function returns the head of the linked list
Node *LinkedList::getHead()
{
return head;
}
//This function creates a new node with the given parameter info. It returns a pointer that points to the newly created node.
Node * LinkedList::createNode(string name, int winScore, int loseScore)
{
Node *temp = new Node;
temp->name = name;
temp->winScore = winScore;
temp->loseScore = loseScore;
temp->next = NULL;
return new Node; // I think I have done this function right but please correct me if I'm wrong
}
//The function calls createnode first. It then insert the newly created node inside the linked list in alphabetical order. It also returns a pointer that
points to the newly added node.
Node *LinkedList::addNode(string name, int winScore, int loseScore)
{
//add code here which I couldn't do
}
c++ linked-list
c++ linked-list
asked Nov 25 '18 at 4:21
ConfusedProgrammerConfusedProgrammer
11
11
addNode
will allocate a new node, set the data value andnext
pointer toNULL
then check ifhead = NULL
(if so just sethead = newnode;
for the 1st node case), then yourelse
case will use a temporary pointer set to head and then iteratewhile (iter->next != NULL)
when that loop fails,iter
points to the last node, so just setiter->next = newnode;
(there are many many examples of the logic) Also, if you keep alast
pointer, you can avoid iterating to find the last node -- up to you. (you can have separatecreateNode
andaddNode
functions - or combine them, up to you)
– David C. Rankin
Nov 25 '18 at 4:40
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49
add a comment |
addNode
will allocate a new node, set the data value andnext
pointer toNULL
then check ifhead = NULL
(if so just sethead = newnode;
for the 1st node case), then yourelse
case will use a temporary pointer set to head and then iteratewhile (iter->next != NULL)
when that loop fails,iter
points to the last node, so just setiter->next = newnode;
(there are many many examples of the logic) Also, if you keep alast
pointer, you can avoid iterating to find the last node -- up to you. (you can have separatecreateNode
andaddNode
functions - or combine them, up to you)
– David C. Rankin
Nov 25 '18 at 4:40
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49
addNode
will allocate a new node, set the data value and next
pointer to NULL
then check if head = NULL
(if so just set head = newnode;
for the 1st node case), then your else
case will use a temporary pointer set to head and then iterate while (iter->next != NULL)
when that loop fails, iter
points to the last node, so just set iter->next = newnode;
(there are many many examples of the logic) Also, if you keep a last
pointer, you can avoid iterating to find the last node -- up to you. (you can have separate createNode
and addNode
functions - or combine them, up to you)– David C. Rankin
Nov 25 '18 at 4:40
addNode
will allocate a new node, set the data value and next
pointer to NULL
then check if head = NULL
(if so just set head = newnode;
for the 1st node case), then your else
case will use a temporary pointer set to head and then iterate while (iter->next != NULL)
when that loop fails, iter
points to the last node, so just set iter->next = newnode;
(there are many many examples of the logic) Also, if you keep a last
pointer, you can avoid iterating to find the last node -- up to you. (you can have separate createNode
and addNode
functions - or combine them, up to you)– David C. Rankin
Nov 25 '18 at 4:40
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49
add a comment |
1 Answer
1
active
oldest
votes
Linked lists are somewhat generic. With a simple implementation, you create a class instance for the list and the constuctor does nothing more than set the first node (head
) of the list to NULL
. (you can overload your constructor to take character data and call insert
as well)
You start with your data structure (or class, your choice):
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
The insert
function always creates and allocates storage for a new node and initializes the next
pointer NULL
, e.g.
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
...
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
...
At this point in insert
, all that remains is checking whether head == NULL
, and if so, you know this is your first node, so simply set head = node;
, e.g.
if (!head) /* if 1st node */
head = node;
If head != NULL
, then you know you need to set iter = head;
and iterate to the list-end to set the last->next = node;
. You can do this with a simple while
loop, e.g.
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
That's basically it other than returning a pointer to node
. However, if you keep a last
pointer, and always set last = node;
at the end of your insert
function, then there is no need to iterate to find the last node, you simply set last->next = node;
instead of iterating and then update last = node;
A short example putting it altogether may help:
#include <iostream>
#include <string>
using namespace std;
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
~linkedlist() { /* destructor - free list memory */
iter = head;
while (iter) {
node_t *victim = iter;
iter = iter->next;
delete victim;
}
}
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
void print (void) { /* simple print function */
iter = head;
while (iter) {
cout << iter->data;
iter = iter->next;
}
cout << 'n';
}
};
int main (void) {
string s = "my dog has fleas";
linkedlist l;
for (auto& c : s)
l.insert(c);
l.print();
}
Example Use/Output
$ ./bin/ll_insert
my dog has fleas
Memory Use/Error Check
Always use a memory/error checking program to verify you haven't done anything bizarre with your allocations and to confirm you have freed all the memory you allocate. For Linux, valgrind
is the normal choice. Just run your program through it:
$ valgrind ./bin/ll_insert
==9240== Memcheck, a memory error detector
==9240== Copyright (C) 2002-2015, and GNU GPL'd, by Julian Seward et al.
==9240== Using Valgrind-3.12.0 and LibVEX; rerun with -h for copyright info
==9240== Command: ./bin/ll_insert
==9240==
my dog has fleas
==9240==
==9240== HEAP SUMMARY:
==9240== in use at exit: 0 bytes in 0 blocks
==9240== total heap usage: 18 allocs, 18 frees, 73,001 bytes allocated
==9240==
==9240== All heap blocks were freed -- no leaks are possible
==9240==
==9240== For counts of detected and suppressed errors, rerun with: -v
==9240== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Look things over and let me know if you have further questions. I'll leave the last
pointer implementation to you.
Adding a Separate create
Node Function
You have the flexibility to decide how you want to factor your code into separate function. A create
function normally just handles allocating for the node
and setting the data
values and initializing next
to NULL
. I'm not sure how much that makes things easier here, but where you have a complex create
function, it does help tidy up the insert
function a great deal.
Here you simply move the call to new
and assignment to data
and next
to the new create
function and call create
at the top of insert
, e.g.
node_t *create (char data) { /* simply move allocation/initialization
to a create function */
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
return node;
}
node_t *insert (char data) {
node_t *node = create (data); /* call create function */
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
It is functionally identical to doing it all in insert
(and a smart compiler will probably optimize it identically as well). So it is entirely up to you what makes it easier to understand.
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separatelist.create()
before callinglist.insert (data)
inmain()
or whether you just wantedcreate
as a helper forinsert
(which is what I did above). Since you provide a constructor to sethead = NULL;
, there really isn't a need for a separate function just to allow a call tolist.create()
where a list is needed. Let me know which you meant.
– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464626%2fcreate-a-new-node-with-the-given-parameter-info-add-a-new-node%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Linked lists are somewhat generic. With a simple implementation, you create a class instance for the list and the constuctor does nothing more than set the first node (head
) of the list to NULL
. (you can overload your constructor to take character data and call insert
as well)
You start with your data structure (or class, your choice):
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
The insert
function always creates and allocates storage for a new node and initializes the next
pointer NULL
, e.g.
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
...
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
...
At this point in insert
, all that remains is checking whether head == NULL
, and if so, you know this is your first node, so simply set head = node;
, e.g.
if (!head) /* if 1st node */
head = node;
If head != NULL
, then you know you need to set iter = head;
and iterate to the list-end to set the last->next = node;
. You can do this with a simple while
loop, e.g.
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
That's basically it other than returning a pointer to node
. However, if you keep a last
pointer, and always set last = node;
at the end of your insert
function, then there is no need to iterate to find the last node, you simply set last->next = node;
instead of iterating and then update last = node;
A short example putting it altogether may help:
#include <iostream>
#include <string>
using namespace std;
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
~linkedlist() { /* destructor - free list memory */
iter = head;
while (iter) {
node_t *victim = iter;
iter = iter->next;
delete victim;
}
}
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
void print (void) { /* simple print function */
iter = head;
while (iter) {
cout << iter->data;
iter = iter->next;
}
cout << 'n';
}
};
int main (void) {
string s = "my dog has fleas";
linkedlist l;
for (auto& c : s)
l.insert(c);
l.print();
}
Example Use/Output
$ ./bin/ll_insert
my dog has fleas
Memory Use/Error Check
Always use a memory/error checking program to verify you haven't done anything bizarre with your allocations and to confirm you have freed all the memory you allocate. For Linux, valgrind
is the normal choice. Just run your program through it:
$ valgrind ./bin/ll_insert
==9240== Memcheck, a memory error detector
==9240== Copyright (C) 2002-2015, and GNU GPL'd, by Julian Seward et al.
==9240== Using Valgrind-3.12.0 and LibVEX; rerun with -h for copyright info
==9240== Command: ./bin/ll_insert
==9240==
my dog has fleas
==9240==
==9240== HEAP SUMMARY:
==9240== in use at exit: 0 bytes in 0 blocks
==9240== total heap usage: 18 allocs, 18 frees, 73,001 bytes allocated
==9240==
==9240== All heap blocks were freed -- no leaks are possible
==9240==
==9240== For counts of detected and suppressed errors, rerun with: -v
==9240== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Look things over and let me know if you have further questions. I'll leave the last
pointer implementation to you.
Adding a Separate create
Node Function
You have the flexibility to decide how you want to factor your code into separate function. A create
function normally just handles allocating for the node
and setting the data
values and initializing next
to NULL
. I'm not sure how much that makes things easier here, but where you have a complex create
function, it does help tidy up the insert
function a great deal.
Here you simply move the call to new
and assignment to data
and next
to the new create
function and call create
at the top of insert
, e.g.
node_t *create (char data) { /* simply move allocation/initialization
to a create function */
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
return node;
}
node_t *insert (char data) {
node_t *node = create (data); /* call create function */
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
It is functionally identical to doing it all in insert
(and a smart compiler will probably optimize it identically as well). So it is entirely up to you what makes it easier to understand.
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separatelist.create()
before callinglist.insert (data)
inmain()
or whether you just wantedcreate
as a helper forinsert
(which is what I did above). Since you provide a constructor to sethead = NULL;
, there really isn't a need for a separate function just to allow a call tolist.create()
where a list is needed. Let me know which you meant.
– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
|
show 2 more comments
Linked lists are somewhat generic. With a simple implementation, you create a class instance for the list and the constuctor does nothing more than set the first node (head
) of the list to NULL
. (you can overload your constructor to take character data and call insert
as well)
You start with your data structure (or class, your choice):
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
The insert
function always creates and allocates storage for a new node and initializes the next
pointer NULL
, e.g.
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
...
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
...
At this point in insert
, all that remains is checking whether head == NULL
, and if so, you know this is your first node, so simply set head = node;
, e.g.
if (!head) /* if 1st node */
head = node;
If head != NULL
, then you know you need to set iter = head;
and iterate to the list-end to set the last->next = node;
. You can do this with a simple while
loop, e.g.
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
That's basically it other than returning a pointer to node
. However, if you keep a last
pointer, and always set last = node;
at the end of your insert
function, then there is no need to iterate to find the last node, you simply set last->next = node;
instead of iterating and then update last = node;
A short example putting it altogether may help:
#include <iostream>
#include <string>
using namespace std;
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
~linkedlist() { /* destructor - free list memory */
iter = head;
while (iter) {
node_t *victim = iter;
iter = iter->next;
delete victim;
}
}
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
void print (void) { /* simple print function */
iter = head;
while (iter) {
cout << iter->data;
iter = iter->next;
}
cout << 'n';
}
};
int main (void) {
string s = "my dog has fleas";
linkedlist l;
for (auto& c : s)
l.insert(c);
l.print();
}
Example Use/Output
$ ./bin/ll_insert
my dog has fleas
Memory Use/Error Check
Always use a memory/error checking program to verify you haven't done anything bizarre with your allocations and to confirm you have freed all the memory you allocate. For Linux, valgrind
is the normal choice. Just run your program through it:
$ valgrind ./bin/ll_insert
==9240== Memcheck, a memory error detector
==9240== Copyright (C) 2002-2015, and GNU GPL'd, by Julian Seward et al.
==9240== Using Valgrind-3.12.0 and LibVEX; rerun with -h for copyright info
==9240== Command: ./bin/ll_insert
==9240==
my dog has fleas
==9240==
==9240== HEAP SUMMARY:
==9240== in use at exit: 0 bytes in 0 blocks
==9240== total heap usage: 18 allocs, 18 frees, 73,001 bytes allocated
==9240==
==9240== All heap blocks were freed -- no leaks are possible
==9240==
==9240== For counts of detected and suppressed errors, rerun with: -v
==9240== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Look things over and let me know if you have further questions. I'll leave the last
pointer implementation to you.
Adding a Separate create
Node Function
You have the flexibility to decide how you want to factor your code into separate function. A create
function normally just handles allocating for the node
and setting the data
values and initializing next
to NULL
. I'm not sure how much that makes things easier here, but where you have a complex create
function, it does help tidy up the insert
function a great deal.
Here you simply move the call to new
and assignment to data
and next
to the new create
function and call create
at the top of insert
, e.g.
node_t *create (char data) { /* simply move allocation/initialization
to a create function */
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
return node;
}
node_t *insert (char data) {
node_t *node = create (data); /* call create function */
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
It is functionally identical to doing it all in insert
(and a smart compiler will probably optimize it identically as well). So it is entirely up to you what makes it easier to understand.
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separatelist.create()
before callinglist.insert (data)
inmain()
or whether you just wantedcreate
as a helper forinsert
(which is what I did above). Since you provide a constructor to sethead = NULL;
, there really isn't a need for a separate function just to allow a call tolist.create()
where a list is needed. Let me know which you meant.
– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
|
show 2 more comments
Linked lists are somewhat generic. With a simple implementation, you create a class instance for the list and the constuctor does nothing more than set the first node (head
) of the list to NULL
. (you can overload your constructor to take character data and call insert
as well)
You start with your data structure (or class, your choice):
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
The insert
function always creates and allocates storage for a new node and initializes the next
pointer NULL
, e.g.
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
...
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
...
At this point in insert
, all that remains is checking whether head == NULL
, and if so, you know this is your first node, so simply set head = node;
, e.g.
if (!head) /* if 1st node */
head = node;
If head != NULL
, then you know you need to set iter = head;
and iterate to the list-end to set the last->next = node;
. You can do this with a simple while
loop, e.g.
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
That's basically it other than returning a pointer to node
. However, if you keep a last
pointer, and always set last = node;
at the end of your insert
function, then there is no need to iterate to find the last node, you simply set last->next = node;
instead of iterating and then update last = node;
A short example putting it altogether may help:
#include <iostream>
#include <string>
using namespace std;
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
~linkedlist() { /* destructor - free list memory */
iter = head;
while (iter) {
node_t *victim = iter;
iter = iter->next;
delete victim;
}
}
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
void print (void) { /* simple print function */
iter = head;
while (iter) {
cout << iter->data;
iter = iter->next;
}
cout << 'n';
}
};
int main (void) {
string s = "my dog has fleas";
linkedlist l;
for (auto& c : s)
l.insert(c);
l.print();
}
Example Use/Output
$ ./bin/ll_insert
my dog has fleas
Memory Use/Error Check
Always use a memory/error checking program to verify you haven't done anything bizarre with your allocations and to confirm you have freed all the memory you allocate. For Linux, valgrind
is the normal choice. Just run your program through it:
$ valgrind ./bin/ll_insert
==9240== Memcheck, a memory error detector
==9240== Copyright (C) 2002-2015, and GNU GPL'd, by Julian Seward et al.
==9240== Using Valgrind-3.12.0 and LibVEX; rerun with -h for copyright info
==9240== Command: ./bin/ll_insert
==9240==
my dog has fleas
==9240==
==9240== HEAP SUMMARY:
==9240== in use at exit: 0 bytes in 0 blocks
==9240== total heap usage: 18 allocs, 18 frees, 73,001 bytes allocated
==9240==
==9240== All heap blocks were freed -- no leaks are possible
==9240==
==9240== For counts of detected and suppressed errors, rerun with: -v
==9240== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Look things over and let me know if you have further questions. I'll leave the last
pointer implementation to you.
Adding a Separate create
Node Function
You have the flexibility to decide how you want to factor your code into separate function. A create
function normally just handles allocating for the node
and setting the data
values and initializing next
to NULL
. I'm not sure how much that makes things easier here, but where you have a complex create
function, it does help tidy up the insert
function a great deal.
Here you simply move the call to new
and assignment to data
and next
to the new create
function and call create
at the top of insert
, e.g.
node_t *create (char data) { /* simply move allocation/initialization
to a create function */
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
return node;
}
node_t *insert (char data) {
node_t *node = create (data); /* call create function */
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
It is functionally identical to doing it all in insert
(and a smart compiler will probably optimize it identically as well). So it is entirely up to you what makes it easier to understand.
Linked lists are somewhat generic. With a simple implementation, you create a class instance for the list and the constuctor does nothing more than set the first node (head
) of the list to NULL
. (you can overload your constructor to take character data and call insert
as well)
You start with your data structure (or class, your choice):
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
The insert
function always creates and allocates storage for a new node and initializes the next
pointer NULL
, e.g.
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
...
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
...
At this point in insert
, all that remains is checking whether head == NULL
, and if so, you know this is your first node, so simply set head = node;
, e.g.
if (!head) /* if 1st node */
head = node;
If head != NULL
, then you know you need to set iter = head;
and iterate to the list-end to set the last->next = node;
. You can do this with a simple while
loop, e.g.
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
That's basically it other than returning a pointer to node
. However, if you keep a last
pointer, and always set last = node;
at the end of your insert
function, then there is no need to iterate to find the last node, you simply set last->next = node;
instead of iterating and then update last = node;
A short example putting it altogether may help:
#include <iostream>
#include <string>
using namespace std;
struct node_t { /* simple struct holding char and next pointer */
char data;
node_t *next;
};
class linkedlist {
node_t *head, *iter; /* private members */
public:
linkedlist() { /* constructor - sets head NULL */
head = NULL;
}
~linkedlist() { /* destructor - free list memory */
iter = head;
while (iter) {
node_t *victim = iter;
iter = iter->next;
delete victim;
}
}
node_t *insert (char data) {
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
void print (void) { /* simple print function */
iter = head;
while (iter) {
cout << iter->data;
iter = iter->next;
}
cout << 'n';
}
};
int main (void) {
string s = "my dog has fleas";
linkedlist l;
for (auto& c : s)
l.insert(c);
l.print();
}
Example Use/Output
$ ./bin/ll_insert
my dog has fleas
Memory Use/Error Check
Always use a memory/error checking program to verify you haven't done anything bizarre with your allocations and to confirm you have freed all the memory you allocate. For Linux, valgrind
is the normal choice. Just run your program through it:
$ valgrind ./bin/ll_insert
==9240== Memcheck, a memory error detector
==9240== Copyright (C) 2002-2015, and GNU GPL'd, by Julian Seward et al.
==9240== Using Valgrind-3.12.0 and LibVEX; rerun with -h for copyright info
==9240== Command: ./bin/ll_insert
==9240==
my dog has fleas
==9240==
==9240== HEAP SUMMARY:
==9240== in use at exit: 0 bytes in 0 blocks
==9240== total heap usage: 18 allocs, 18 frees, 73,001 bytes allocated
==9240==
==9240== All heap blocks were freed -- no leaks are possible
==9240==
==9240== For counts of detected and suppressed errors, rerun with: -v
==9240== ERROR SUMMARY: 0 errors from 0 contexts (suppressed: 0 from 0)
Look things over and let me know if you have further questions. I'll leave the last
pointer implementation to you.
Adding a Separate create
Node Function
You have the flexibility to decide how you want to factor your code into separate function. A create
function normally just handles allocating for the node
and setting the data
values and initializing next
to NULL
. I'm not sure how much that makes things easier here, but where you have a complex create
function, it does help tidy up the insert
function a great deal.
Here you simply move the call to new
and assignment to data
and next
to the new create
function and call create
at the top of insert
, e.g.
node_t *create (char data) { /* simply move allocation/initialization
to a create function */
node_t *node = new node_t; /* allocate new node */
node->data = data; /* set node data */
node->next = NULL;
return node;
}
node_t *insert (char data) {
node_t *node = create (data); /* call create function */
if (!head) /* if 1st node */
head = node;
else { /* otherwise */
iter = head;
while (iter->next) /* traditional iterate to find last */
iter = iter->next;
iter->next = node; /* set new last */
}
return node; /* return node */
}
It is functionally identical to doing it all in insert
(and a smart compiler will probably optimize it identically as well). So it is entirely up to you what makes it easier to understand.
edited Nov 25 '18 at 6:41
answered Nov 25 '18 at 6:11


David C. RankinDavid C. Rankin
41.1k32648
41.1k32648
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separatelist.create()
before callinglist.insert (data)
inmain()
or whether you just wantedcreate
as a helper forinsert
(which is what I did above). Since you provide a constructor to sethead = NULL;
, there really isn't a need for a separate function just to allow a call tolist.create()
where a list is needed. Let me know which you meant.
– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
|
show 2 more comments
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separatelist.create()
before callinglist.insert (data)
inmain()
or whether you just wantedcreate
as a helper forinsert
(which is what I did above). Since you provide a constructor to sethead = NULL;
, there really isn't a need for a separate function just to allow a call tolist.create()
where a list is needed. Let me know which you meant.
– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
as much as I appreciate this explanation, could you modify my code accordingly create and add a new node in different functions. That would help me more, I think. Thanks.
– ConfusedProgrammer
Nov 25 '18 at 6:22
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
Sure, hold on I'll drop an edit.
– David C. Rankin
Nov 25 '18 at 6:26
And also, it is a bit ambiguous whether you wanted to have to call a separate
list.create()
before calling list.insert (data)
in main()
or whether you just wanted create
as a helper for insert
(which is what I did above). Since you provide a constructor to set head = NULL;
, there really isn't a need for a separate function just to allow a call to list.create()
where a list is needed. Let me know which you meant.– David C. Rankin
Nov 25 '18 at 6:47
And also, it is a bit ambiguous whether you wanted to have to call a separate
list.create()
before calling list.insert (data)
in main()
or whether you just wanted create
as a helper for insert
(which is what I did above). Since you provide a constructor to set head = NULL;
, there really isn't a need for a separate function just to allow a call to list.create()
where a list is needed. Let me know which you meant.– David C. Rankin
Nov 25 '18 at 6:47
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
AAHHH I GOT IT TO WORK. OMG THANK YOU. I actually understood this now!!! aah i can't thank you enouugh!!
– ConfusedProgrammer
Nov 25 '18 at 7:10
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
quick question: in the create function, if there is more than one data, then do i need to set the node data for every one of them right? example: Node * LinkedList::createNode(string name, int winScore, int loseScore) { Node *node = new Node; node->name = name; node->winScore = winScore; node->loseScore = loseScore; node->next = NULL; return node; }
– ConfusedProgrammer
Nov 25 '18 at 7:12
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53464626%2fcreate-a-new-node-with-the-given-parameter-info-add-a-new-node%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Japaj WUobY,VhioszIFFFaJ1qygAUl3ac4EnSqWx1f,UxbZLreq
addNode
will allocate a new node, set the data value andnext
pointer toNULL
then check ifhead = NULL
(if so just sethead = newnode;
for the 1st node case), then yourelse
case will use a temporary pointer set to head and then iteratewhile (iter->next != NULL)
when that loop fails,iter
points to the last node, so just setiter->next = newnode;
(there are many many examples of the logic) Also, if you keep alast
pointer, you can avoid iterating to find the last node -- up to you. (you can have separatecreateNode
andaddNode
functions - or combine them, up to you)– David C. Rankin
Nov 25 '18 at 4:40
@DavidC.Rankin could you write it out for me? I am still confused. been trying for half an hour since you replied. I really want the code to have separate createNode and addNode functions
– ConfusedProgrammer
Nov 25 '18 at 5:20
Sure give me a bit and I'll help you through it.
– David C. Rankin
Nov 25 '18 at 5:31
Sure thing! I'll hold up
– ConfusedProgrammer
Nov 25 '18 at 5:49