Howto decorate doctrine collection
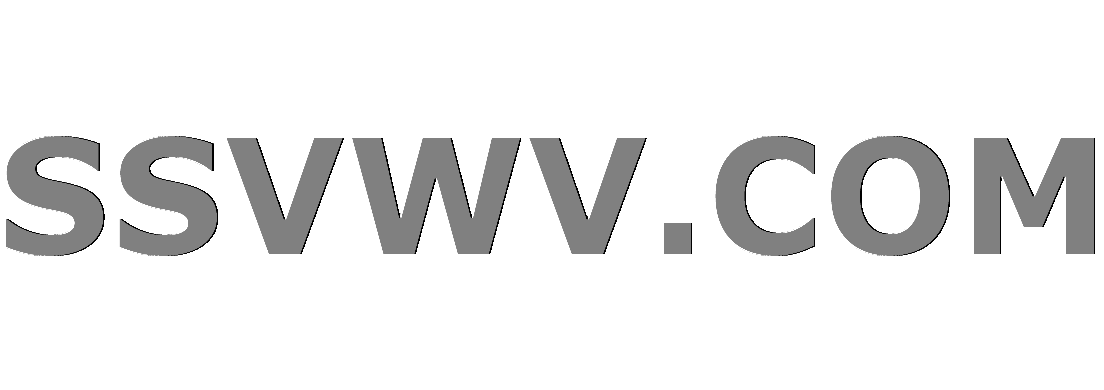
Multi tool use
I want to decorate a doctrine2 collection and working with symfony4.
So i had an entity
class Entry
{
protected $title;
public function getTitle(): ?string
{
return $this->title;
}
}
And a decorator:
class EntryDecorator
{
protected $entry;
public function __construct(Entry $entry)
{
$this->entry = $entry;
}
public function getTitle()
{
return '----'; // just for testing ;-)
}
}
And in my Controller i load the complete entries from the database using:
$entries = $this->entryRepository->findAll()
And my view is simple as this:
{% for entry in entries %}
{{ entry.getTitle }}
{% endfor %}
So, now i want to decorate the whole collection.
In SF4 i found How to Decorate Services which looks fine, but this doesn't work for this situation.
Because: doctrine returns an immutable collection (see this topic Immutable collections in Doctrine 2?)
So i thought something like this will be fine:
array_walk($entries, function($entry) {
return new EntryDecorator($entry);
});
But doesn't work, because the collection is immutable.
Now i have the following which is working:
$entries = ;
foreach($this->entryRepository->findAll() as $entry) {
$entries = new EntryDecorator($entry);
}
But it looks hm, ok.
So my question is:
Is there a better solution to decorate a doctrine collection?
Update:
currently, im using array_map()
for this.
array_map(function ($entry) {
$decorator = new EntryDecorator($entry);
return $decorator;
}, $entries);
php symfony doctrine decorator symfony4
add a comment |
I want to decorate a doctrine2 collection and working with symfony4.
So i had an entity
class Entry
{
protected $title;
public function getTitle(): ?string
{
return $this->title;
}
}
And a decorator:
class EntryDecorator
{
protected $entry;
public function __construct(Entry $entry)
{
$this->entry = $entry;
}
public function getTitle()
{
return '----'; // just for testing ;-)
}
}
And in my Controller i load the complete entries from the database using:
$entries = $this->entryRepository->findAll()
And my view is simple as this:
{% for entry in entries %}
{{ entry.getTitle }}
{% endfor %}
So, now i want to decorate the whole collection.
In SF4 i found How to Decorate Services which looks fine, but this doesn't work for this situation.
Because: doctrine returns an immutable collection (see this topic Immutable collections in Doctrine 2?)
So i thought something like this will be fine:
array_walk($entries, function($entry) {
return new EntryDecorator($entry);
});
But doesn't work, because the collection is immutable.
Now i have the following which is working:
$entries = ;
foreach($this->entryRepository->findAll() as $entry) {
$entries = new EntryDecorator($entry);
}
But it looks hm, ok.
So my question is:
Is there a better solution to decorate a doctrine collection?
Update:
currently, im using array_map()
for this.
array_map(function ($entry) {
$decorator = new EntryDecorator($entry);
return $decorator;
}, $entries);
php symfony doctrine decorator symfony4
add a comment |
I want to decorate a doctrine2 collection and working with symfony4.
So i had an entity
class Entry
{
protected $title;
public function getTitle(): ?string
{
return $this->title;
}
}
And a decorator:
class EntryDecorator
{
protected $entry;
public function __construct(Entry $entry)
{
$this->entry = $entry;
}
public function getTitle()
{
return '----'; // just for testing ;-)
}
}
And in my Controller i load the complete entries from the database using:
$entries = $this->entryRepository->findAll()
And my view is simple as this:
{% for entry in entries %}
{{ entry.getTitle }}
{% endfor %}
So, now i want to decorate the whole collection.
In SF4 i found How to Decorate Services which looks fine, but this doesn't work for this situation.
Because: doctrine returns an immutable collection (see this topic Immutable collections in Doctrine 2?)
So i thought something like this will be fine:
array_walk($entries, function($entry) {
return new EntryDecorator($entry);
});
But doesn't work, because the collection is immutable.
Now i have the following which is working:
$entries = ;
foreach($this->entryRepository->findAll() as $entry) {
$entries = new EntryDecorator($entry);
}
But it looks hm, ok.
So my question is:
Is there a better solution to decorate a doctrine collection?
Update:
currently, im using array_map()
for this.
array_map(function ($entry) {
$decorator = new EntryDecorator($entry);
return $decorator;
}, $entries);
php symfony doctrine decorator symfony4
I want to decorate a doctrine2 collection and working with symfony4.
So i had an entity
class Entry
{
protected $title;
public function getTitle(): ?string
{
return $this->title;
}
}
And a decorator:
class EntryDecorator
{
protected $entry;
public function __construct(Entry $entry)
{
$this->entry = $entry;
}
public function getTitle()
{
return '----'; // just for testing ;-)
}
}
And in my Controller i load the complete entries from the database using:
$entries = $this->entryRepository->findAll()
And my view is simple as this:
{% for entry in entries %}
{{ entry.getTitle }}
{% endfor %}
So, now i want to decorate the whole collection.
In SF4 i found How to Decorate Services which looks fine, but this doesn't work for this situation.
Because: doctrine returns an immutable collection (see this topic Immutable collections in Doctrine 2?)
So i thought something like this will be fine:
array_walk($entries, function($entry) {
return new EntryDecorator($entry);
});
But doesn't work, because the collection is immutable.
Now i have the following which is working:
$entries = ;
foreach($this->entryRepository->findAll() as $entry) {
$entries = new EntryDecorator($entry);
}
But it looks hm, ok.
So my question is:
Is there a better solution to decorate a doctrine collection?
Update:
currently, im using array_map()
for this.
array_map(function ($entry) {
$decorator = new EntryDecorator($entry);
return $decorator;
}, $entries);
php symfony doctrine decorator symfony4
php symfony doctrine decorator symfony4
edited Nov 28 '18 at 8:09
rob
asked Nov 27 '18 at 13:25


robrob
1,32351526
1,32351526
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
If you want "transform" the data in your twig template, if suggest you use a TwigExtension and create a custom filter (or function).
{# filter #}
{% for entry in entries %}
{{ entry.getTitle|my_decorator }}
{% endfor %}
{# function #}
{% for entry in entries %}
{{ my_decorator(entry) }}
{% endfor %}
That's a good idea, but it will only work forTwig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.
– rob
Nov 28 '18 at 7:09
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53500770%2fhowto-decorate-doctrine-collection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
If you want "transform" the data in your twig template, if suggest you use a TwigExtension and create a custom filter (or function).
{# filter #}
{% for entry in entries %}
{{ entry.getTitle|my_decorator }}
{% endfor %}
{# function #}
{% for entry in entries %}
{{ my_decorator(entry) }}
{% endfor %}
That's a good idea, but it will only work forTwig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.
– rob
Nov 28 '18 at 7:09
add a comment |
If you want "transform" the data in your twig template, if suggest you use a TwigExtension and create a custom filter (or function).
{# filter #}
{% for entry in entries %}
{{ entry.getTitle|my_decorator }}
{% endfor %}
{# function #}
{% for entry in entries %}
{{ my_decorator(entry) }}
{% endfor %}
That's a good idea, but it will only work forTwig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.
– rob
Nov 28 '18 at 7:09
add a comment |
If you want "transform" the data in your twig template, if suggest you use a TwigExtension and create a custom filter (or function).
{# filter #}
{% for entry in entries %}
{{ entry.getTitle|my_decorator }}
{% endfor %}
{# function #}
{% for entry in entries %}
{{ my_decorator(entry) }}
{% endfor %}
If you want "transform" the data in your twig template, if suggest you use a TwigExtension and create a custom filter (or function).
{# filter #}
{% for entry in entries %}
{{ entry.getTitle|my_decorator }}
{% endfor %}
{# function #}
{% for entry in entries %}
{{ my_decorator(entry) }}
{% endfor %}
edited Nov 27 '18 at 13:45
answered Nov 27 '18 at 13:37


EquaProEquaPro
3195
3195
That's a good idea, but it will only work forTwig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.
– rob
Nov 28 '18 at 7:09
add a comment |
That's a good idea, but it will only work forTwig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.
– rob
Nov 28 '18 at 7:09
That's a good idea, but it will only work for
Twig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.– rob
Nov 28 '18 at 7:09
That's a good idea, but it will only work for
Twig
. If i want to use another template engine or build an json response, then this will not work. i'm searching for a solution to "inject" the decorator into doctrine e.g.– rob
Nov 28 '18 at 7:09
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53500770%2fhowto-decorate-doctrine-collection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
w,NIfNcRsaGe9JwNAx4,HRK29PD7hF 7M65m43gFO0FX5mxK p0 aIzuQwYUvuV4ZAa0SD5ViXU,tdcLcH vh8QSw KbewV