Sort a set in Java
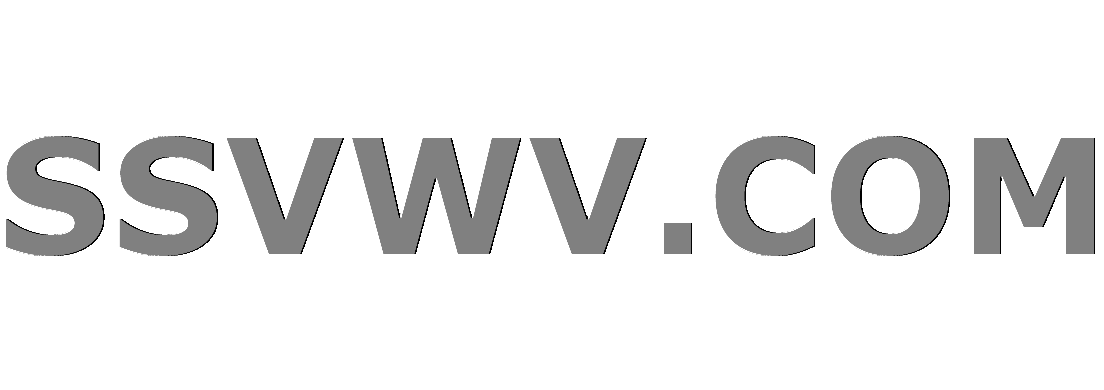
Multi tool use
up vote
2
down vote
favorite
Framework used: Spring
ORM used: Hibernate
I have two classes
class BatchExceptionDetails{
...
private Set<BatchExceptionComments> batchExceptionComments;
}
class BatchExceptionComments implements Comparable<BatchExceptionComments>{
...
@Override
public int compareTo(BatchExceptionComments o) {
// TODO Auto-generated method stub
return this.getAddedOn().compareTo(o.getAddedOn());
}
}
They are mapped with one to many mapping.
There is a set of BatchExceptionComments in BatchExceptionDetails.
I want to sort the set on the basis of Date. BatchExcpetionComment has an attribute of type java.util.Date i.e. addedOn. I want the latest comment to be the first element of set.
The set I am receiving is not sorted. Will you please guide me where I am going wrong.
Thanks in advance
java spring hibernate sorting collections
|
show 4 more comments
up vote
2
down vote
favorite
Framework used: Spring
ORM used: Hibernate
I have two classes
class BatchExceptionDetails{
...
private Set<BatchExceptionComments> batchExceptionComments;
}
class BatchExceptionComments implements Comparable<BatchExceptionComments>{
...
@Override
public int compareTo(BatchExceptionComments o) {
// TODO Auto-generated method stub
return this.getAddedOn().compareTo(o.getAddedOn());
}
}
They are mapped with one to many mapping.
There is a set of BatchExceptionComments in BatchExceptionDetails.
I want to sort the set on the basis of Date. BatchExcpetionComment has an attribute of type java.util.Date i.e. addedOn. I want the latest comment to be the first element of set.
The set I am receiving is not sorted. Will you please guide me where I am going wrong.
Thanks in advance
java spring hibernate sorting collections
Are you using aSortedSet
? Or some other type ofSet
?
– Joe C
Nov 22 at 7:45
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
2
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
2
You can't be using justSet
, becauseSet
is an interface. What implementation ofSet
are you using?
– Joe C
Nov 22 at 7:50
|
show 4 more comments
up vote
2
down vote
favorite
up vote
2
down vote
favorite
Framework used: Spring
ORM used: Hibernate
I have two classes
class BatchExceptionDetails{
...
private Set<BatchExceptionComments> batchExceptionComments;
}
class BatchExceptionComments implements Comparable<BatchExceptionComments>{
...
@Override
public int compareTo(BatchExceptionComments o) {
// TODO Auto-generated method stub
return this.getAddedOn().compareTo(o.getAddedOn());
}
}
They are mapped with one to many mapping.
There is a set of BatchExceptionComments in BatchExceptionDetails.
I want to sort the set on the basis of Date. BatchExcpetionComment has an attribute of type java.util.Date i.e. addedOn. I want the latest comment to be the first element of set.
The set I am receiving is not sorted. Will you please guide me where I am going wrong.
Thanks in advance
java spring hibernate sorting collections
Framework used: Spring
ORM used: Hibernate
I have two classes
class BatchExceptionDetails{
...
private Set<BatchExceptionComments> batchExceptionComments;
}
class BatchExceptionComments implements Comparable<BatchExceptionComments>{
...
@Override
public int compareTo(BatchExceptionComments o) {
// TODO Auto-generated method stub
return this.getAddedOn().compareTo(o.getAddedOn());
}
}
They are mapped with one to many mapping.
There is a set of BatchExceptionComments in BatchExceptionDetails.
I want to sort the set on the basis of Date. BatchExcpetionComment has an attribute of type java.util.Date i.e. addedOn. I want the latest comment to be the first element of set.
The set I am receiving is not sorted. Will you please guide me where I am going wrong.
Thanks in advance
java spring hibernate sorting collections
java spring hibernate sorting collections
asked Nov 22 at 7:44
Ayush
658
658
Are you using aSortedSet
? Or some other type ofSet
?
– Joe C
Nov 22 at 7:45
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
2
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
2
You can't be using justSet
, becauseSet
is an interface. What implementation ofSet
are you using?
– Joe C
Nov 22 at 7:50
|
show 4 more comments
Are you using aSortedSet
? Or some other type ofSet
?
– Joe C
Nov 22 at 7:45
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
2
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
2
You can't be using justSet
, becauseSet
is an interface. What implementation ofSet
are you using?
– Joe C
Nov 22 at 7:50
Are you using a
SortedSet
? Or some other type of Set
?– Joe C
Nov 22 at 7:45
Are you using a
SortedSet
? Or some other type of Set
?– Joe C
Nov 22 at 7:45
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
2
2
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
2
2
You can't be using just
Set
, because Set
is an interface. What implementation of Set
are you using?– Joe C
Nov 22 at 7:50
You can't be using just
Set
, because Set
is an interface. What implementation of Set
are you using?– Joe C
Nov 22 at 7:50
|
show 4 more comments
2 Answers
2
active
oldest
votes
up vote
5
down vote
Set
is an interface, so it is not possible to establish if it is sortable or not. You have to use the correct implementation, like TreeSet. If you want to
emphasize that it is a sorted set, you should use the SortedSet
interface. TreeSet
implements SortedSet
.
Alternatively you can use a List
and then you can sort it using Collections.sort
.
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type asSortedSet
instead ofSet
should work though.
– Robby Cornelissen
Nov 22 at 7:59
1
No need to initialize it. Hibernate will automatically initialize it with its ownSortedSet
implementation.
– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
|
show 2 more comments
up vote
1
down vote
accepted
After some goofing around I found a solution.
I just declared the set
private Set<BatchExceptionComments> batchExceptionComments;
Instead of using Comparable I used Order By to arrange the set.
<set name="batchExceptionComments" table="BATCH_EXCEPTION_COMMENTS"
inverse="true" fetch="select" lazy="false" order-by="commentId">
<key>
<column name="EXCEPTION_ID" not-null="true" />
</key>
<one-to-many class="com.beans.BatchExceptionComments" />
</set>
I believe ordering by Id will be better.
P.S. I am using hbm.xml instead of annotation
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
5
down vote
Set
is an interface, so it is not possible to establish if it is sortable or not. You have to use the correct implementation, like TreeSet. If you want to
emphasize that it is a sorted set, you should use the SortedSet
interface. TreeSet
implements SortedSet
.
Alternatively you can use a List
and then you can sort it using Collections.sort
.
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type asSortedSet
instead ofSet
should work though.
– Robby Cornelissen
Nov 22 at 7:59
1
No need to initialize it. Hibernate will automatically initialize it with its ownSortedSet
implementation.
– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
|
show 2 more comments
up vote
5
down vote
Set
is an interface, so it is not possible to establish if it is sortable or not. You have to use the correct implementation, like TreeSet. If you want to
emphasize that it is a sorted set, you should use the SortedSet
interface. TreeSet
implements SortedSet
.
Alternatively you can use a List
and then you can sort it using Collections.sort
.
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type asSortedSet
instead ofSet
should work though.
– Robby Cornelissen
Nov 22 at 7:59
1
No need to initialize it. Hibernate will automatically initialize it with its ownSortedSet
implementation.
– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
|
show 2 more comments
up vote
5
down vote
up vote
5
down vote
Set
is an interface, so it is not possible to establish if it is sortable or not. You have to use the correct implementation, like TreeSet. If you want to
emphasize that it is a sorted set, you should use the SortedSet
interface. TreeSet
implements SortedSet
.
Alternatively you can use a List
and then you can sort it using Collections.sort
.
Set
is an interface, so it is not possible to establish if it is sortable or not. You have to use the correct implementation, like TreeSet. If you want to
emphasize that it is a sorted set, you should use the SortedSet
interface. TreeSet
implements SortedSet
.
Alternatively you can use a List
and then you can sort it using Collections.sort
.
answered Nov 22 at 7:53


Lorelorelore
1,75451124
1,75451124
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type asSortedSet
instead ofSet
should work though.
– Robby Cornelissen
Nov 22 at 7:59
1
No need to initialize it. Hibernate will automatically initialize it with its ownSortedSet
implementation.
– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
|
show 2 more comments
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type asSortedSet
instead ofSet
should work though.
– Robby Cornelissen
Nov 22 at 7:59
1
No need to initialize it. Hibernate will automatically initialize it with its ownSortedSet
implementation.
– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
Can I use other collections instead of Set ? I am in assumption that if we are using Spring then we have to use Set for Containment.
– Ayush
Nov 22 at 7:58
3
3
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type as
SortedSet
instead of Set
should work though.– Robby Cornelissen
Nov 22 at 7:59
The problem is that Hibernate requires collections to be specified as an interface type. In terms of implementation classes, it uses its own to support things like lazy loading. Specifying the type as
SortedSet
instead of Set
should work though.– Robby Cornelissen
Nov 22 at 7:59
1
1
No need to initialize it. Hibernate will automatically initialize it with its own
SortedSet
implementation.– Robby Cornelissen
Nov 22 at 8:04
No need to initialize it. Hibernate will automatically initialize it with its own
SortedSet
implementation.– Robby Cornelissen
Nov 22 at 8:04
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, I used SortedSet and I am getting an exception IllegalArgumentException occurred while calling setter for property [combeans.BatchExceptionDetails.batchExceptionComments (expected type = java.util.SortedSet)];
– Ayush
Nov 22 at 8:15
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
@RobbyCornelissen, my setter method is public void setBatchExceptionComments( SortedSet<BatchExceptionComments> batchExceptionComments) { this.batchExceptionComments = batchExceptionComments; }
– Ayush
Nov 22 at 8:17
|
show 2 more comments
up vote
1
down vote
accepted
After some goofing around I found a solution.
I just declared the set
private Set<BatchExceptionComments> batchExceptionComments;
Instead of using Comparable I used Order By to arrange the set.
<set name="batchExceptionComments" table="BATCH_EXCEPTION_COMMENTS"
inverse="true" fetch="select" lazy="false" order-by="commentId">
<key>
<column name="EXCEPTION_ID" not-null="true" />
</key>
<one-to-many class="com.beans.BatchExceptionComments" />
</set>
I believe ordering by Id will be better.
P.S. I am using hbm.xml instead of annotation
add a comment |
up vote
1
down vote
accepted
After some goofing around I found a solution.
I just declared the set
private Set<BatchExceptionComments> batchExceptionComments;
Instead of using Comparable I used Order By to arrange the set.
<set name="batchExceptionComments" table="BATCH_EXCEPTION_COMMENTS"
inverse="true" fetch="select" lazy="false" order-by="commentId">
<key>
<column name="EXCEPTION_ID" not-null="true" />
</key>
<one-to-many class="com.beans.BatchExceptionComments" />
</set>
I believe ordering by Id will be better.
P.S. I am using hbm.xml instead of annotation
add a comment |
up vote
1
down vote
accepted
up vote
1
down vote
accepted
After some goofing around I found a solution.
I just declared the set
private Set<BatchExceptionComments> batchExceptionComments;
Instead of using Comparable I used Order By to arrange the set.
<set name="batchExceptionComments" table="BATCH_EXCEPTION_COMMENTS"
inverse="true" fetch="select" lazy="false" order-by="commentId">
<key>
<column name="EXCEPTION_ID" not-null="true" />
</key>
<one-to-many class="com.beans.BatchExceptionComments" />
</set>
I believe ordering by Id will be better.
P.S. I am using hbm.xml instead of annotation
After some goofing around I found a solution.
I just declared the set
private Set<BatchExceptionComments> batchExceptionComments;
Instead of using Comparable I used Order By to arrange the set.
<set name="batchExceptionComments" table="BATCH_EXCEPTION_COMMENTS"
inverse="true" fetch="select" lazy="false" order-by="commentId">
<key>
<column name="EXCEPTION_ID" not-null="true" />
</key>
<one-to-many class="com.beans.BatchExceptionComments" />
</set>
I believe ordering by Id will be better.
P.S. I am using hbm.xml instead of annotation
answered Nov 22 at 14:37
Ayush
658
658
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53426073%2fsort-a-set-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
3iKI YUfGYQ T2LRg2fPMztVF s34Np YL t 2PFE1cKx64e7KWRv 7IUI0T5,mFs bBHr Eo7cf,KwV
Are you using a
SortedSet
? Or some other type ofSet
?– Joe C
Nov 22 at 7:45
Collections.sort(batchExceptionComments);
– Lorelorelore
Nov 22 at 7:46
@Lorelorelore That's only available for lists, not for sets.
– Joe C
Nov 22 at 7:48
2
Sets are unsorted. Use a list instead.
– Robby Cornelissen
Nov 22 at 7:49
2
You can't be using just
Set
, becauseSet
is an interface. What implementation ofSet
are you using?– Joe C
Nov 22 at 7:50