Server did not read message from last client
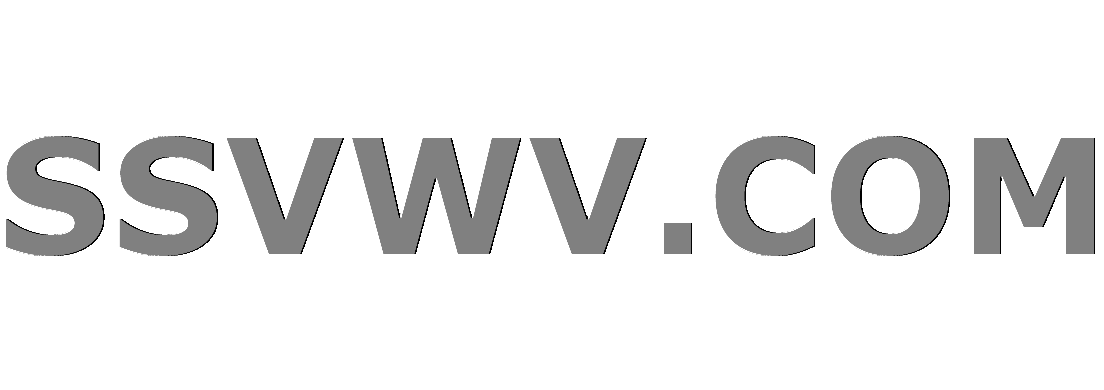
Multi tool use
I need to simulate a distributed system.
There is a controller and n worker computers.
The controller tells the computers when to start, and the computers will start connecting to other computers using sockets. The controller is connecting to the computers using threads. The computer will connect to other computers using threads as well.
Once they are connected to each other, they will send events to each other until they have generated x events. Once they reach x events, the computer will send a "Finish" message to the controller saying that it's done generating events, but will continue reading events from other computers.
My issue: The computers have successfully sent the Finish message to controller, except the last computer in the system. According to the logs, the last computer did send the Finish message to the controller, but the controller did not receive it. The other computers successfully sent the finish message to the controller.
If you need more information, I would be glad to provide. I have been working on this for hours and have no clue.
Computer.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Vector;
public class Computer {
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Computer's time-stamp vector
static Vector<Integer> timestamp = new Vector<Integer>();
// Computer's ID
static int identifier;
// Computer's Event Count
static int eventCount = 0;
// Computer's isAlive check
static boolean isAlive = true;
// Socket to Controller
Socket socketToController;
PrintWriter outputToController;
BufferedReader inputFromController;
String textFromController;
// Server Socket
ServerSocket serverSocket;
// Input and Output Clients
static ArrayList<ClientSocket> outputClients = new ArrayList<ClientSocket>();
static ArrayList<ClientConnection> inputClients = new ArrayList<ClientConnection>();
// Log
Log log;
public static void main(String args) throws IOException {
new Computer("127.0.0.1", 8000);
}
public Computer(String hostname, int port) throws IOException {
// Initialize time-stamp
for (int i = 0; i < MAX_SYSTEMS; ++i) {
timestamp.add(0);
}
// Connect to Controller
try {
socketToController = new Socket(hostname, port);
inputFromController = new BufferedReader(new InputStreamReader(socketToController.getInputStream()));
outputToController = new PrintWriter(socketToController.getOutputStream(), true);
} catch (UnknownHostException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
// Get Computer ID from Controller
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
identifier = Integer.parseInt(textFromController);
break;
}
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
log = new Log("client" + identifier + ".txt");
// Read start message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Start")) {
log.write("Computer is starting!");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
// Instantiate server socket
int socketPort = port + identifier + 1;
// System.out.println(socketPort);
serverSocket = new ServerSocket(socketPort);
log.write("Server Socket Instantiated");
// Instantiate sockets for other server sockets (computers) to send
for (int i = 0; i < MAX_SYSTEMS; ++i) {
if (i != identifier) {
Socket acceptedSocket = new Socket(hostname, port + i + 1);
ClientSocket socketToComputer = new ClientSocket (acceptedSocket);
outputClients.add(socketToComputer);
}
}
log.write("Client Sockets Instantiatedn");
// Accept sockets from server socket and add them into a list
for (int i = 0; i < MAX_SYSTEMS - 1; ++i) {
ClientConnection computerConn = new ClientConnection(serverSocket.accept());
computerConn.start();
inputClients.add(computerConn);
}
log.write("Server connected to clients");
Random rand = new Random();
// Generating events
int temp;
while (eventCount < 50) {
log.write("Generating Event");
int choice = rand.nextInt(5);
if (choice == 0) {
temp = timestamp.get(identifier);
++temp;
timestamp.set(identifier, temp);
} else {
int randC = rand.nextInt(outputClients.size());
ClientSocket cc = outputClients.get(randC);
cc.out.writeObject(new Event(identifier, timestamp));
}
log.write(timestamp.toString());
log.write("Done Generating Event");
eventCount++;
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Finished writing. Continue reading...");
/**
* ========THE ISSUE IS BELOW.===============
*/
synchronized (outputToController) {
outputToController.println("Finish");
outputToController.flush();
}
log.write("Sent Finish Message " + identifier);
// Wait for Tear Down Message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Tear Down")) {
log.write("Tearing down....");
isAlive = false;
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Computer shutting off....");
}
// client socket class (organizing)
public class ClientSocket {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
public ClientSocket(Socket s) {
try {
this.socket = s;
this.out = new ObjectOutputStream(socket.getOutputStream());
} catch (IOException e) {
e.printStackTrace();
}
log.write("Client Socket Created");
}
}
// send event thread
public class ClientConnection extends Thread {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
Random rand = new Random();
public ClientConnection(Socket s) {
this.socket = s;
try {
out = new ObjectOutputStream (socket.getOutputStream());
in = new ObjectInputStream (socket.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run () {
while (isAlive) {
log.write("Reading events");
try {
Event event = (Event) in.readObject();
executeEvent(event.getFromID(), event.getTimestamp());
} catch (ClassNotFoundException e) {
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(timestamp);
}
log.write("Finished Reading");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// execute the event
private void executeEvent(int from, Vector<Integer> x) {
int temp;
synchronized (timestamp) {
for (int i = 0; i < timestamp.size(); ++i) {
if (x.get(i) > timestamp.get(i)) {
timestamp.set(i, x.get(i));
}
}
temp = timestamp.get(from);
++temp;
timestamp.set(from, temp);
}
}
}
}
Controller.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
public class Controller {
// Mutex Lock
public final static Object lock = new Object();
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Server connection threads to computers
static ArrayList<ServerConnection> conns = new ArrayList<ServerConnection>();
// Finished computers
static int finishedCount = 0;
// Server Socket
ServerSocket ss;
// Log Instance
Log log;
public static void main(String args) throws IOException {
new Controller(8000);
}
public Controller(int port) {
// Instantiate Log
log = new Log("server.txt");
// Instantiate Server Socket and Listen for Incoming Sockets
try {
ss = new ServerSocket(port);
log.write("Listening...");
// Accept computers until capacity
for (int i = 0; i < MAX_SYSTEMS; i++) {
Socket s = ss.accept();
log.write("Socket connected");
// Add to list
ServerConnection conn = new ServerConnection(i, s);
conns.add(conn);
conn.start();
}
// Notify all waiting threads to start
synchronized (lock) {
try {
Thread.sleep(1000);
lock.notifyAll();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
private class ServerConnection extends Thread {
// Client Socket
Socket socket;
// Output stream
PrintWriter out;
// Input stream
BufferedReader in;
// ID for connected computer
int identifier;
public ServerConnection(int i, Socket s) {
// Instantiate properties
this.identifier = i;
this.socket = s;
try {
this.in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
this.out = new PrintWriter(socket.getOutputStream(), true);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run() {
log.write("Controller is connected to computer#" + identifier);
// Send ID to computer
out.println(identifier);
// Wait until notified
synchronized (lock) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send Start Message to All Computers
sendAll("Start");
waitForFinish();
log.write("Computer#" + identifier + " is waiting for tear down.");
// If all computers sent the Finish message, send a Tear Down
while (true) {
if (finishedCount == conns.size()) {
log.write("Sending tear down to all computers");
sendAll("Tear Down");
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
/**
* ==== RECEIVE FINISH MESSAGE FROM COMPUTERS ======
*/
private void waitForFinish() {
String clientInput;
while (true) {
try {
if (in.ready()) {
clientInput = in.readLine();
log.write(clientInput);
if (clientInput.equals("Finish")) {
finishedCount += 1;
log.write("Computer " + identifier + " is finished");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send all "text" to all computers in the thread pool
private void sendAll(String text) {
for (int i = 0; i < conns.size(); ++i) {
ServerConnection conn = conns.get(i);
conn.out.println(text);
}
}
}
}
Controller Log: (notice that the log does not say computer 3 is finished)
Listening... (Listening for Computer)
Socket connected
Controller is connected to computer#0
Socket connected
Controller is connected to computer#1
Socket connected
Controller is connected to computer#2
Socket connected
Controller is connected to computer#3
Computer 2 is finished
Computer 1 is finished
Computer#2 is waiting for tear down.
Computer 0 is finished
Computer#1 is waiting for tear down.
Computer#0 is waiting for tear down.
Computer #0 Log: (I cut down the log because there was a lot of reading and writing event log statements)
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
// A bunch of reading and writing events
Finished writing. Continue reading...
Sent Finish Message 0
Computer #1 Log:
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 1
Computer #2
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 2
Computer #3: According to the log, this sent the finished message to controller
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 3
java sockets serversocket
add a comment |
I need to simulate a distributed system.
There is a controller and n worker computers.
The controller tells the computers when to start, and the computers will start connecting to other computers using sockets. The controller is connecting to the computers using threads. The computer will connect to other computers using threads as well.
Once they are connected to each other, they will send events to each other until they have generated x events. Once they reach x events, the computer will send a "Finish" message to the controller saying that it's done generating events, but will continue reading events from other computers.
My issue: The computers have successfully sent the Finish message to controller, except the last computer in the system. According to the logs, the last computer did send the Finish message to the controller, but the controller did not receive it. The other computers successfully sent the finish message to the controller.
If you need more information, I would be glad to provide. I have been working on this for hours and have no clue.
Computer.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Vector;
public class Computer {
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Computer's time-stamp vector
static Vector<Integer> timestamp = new Vector<Integer>();
// Computer's ID
static int identifier;
// Computer's Event Count
static int eventCount = 0;
// Computer's isAlive check
static boolean isAlive = true;
// Socket to Controller
Socket socketToController;
PrintWriter outputToController;
BufferedReader inputFromController;
String textFromController;
// Server Socket
ServerSocket serverSocket;
// Input and Output Clients
static ArrayList<ClientSocket> outputClients = new ArrayList<ClientSocket>();
static ArrayList<ClientConnection> inputClients = new ArrayList<ClientConnection>();
// Log
Log log;
public static void main(String args) throws IOException {
new Computer("127.0.0.1", 8000);
}
public Computer(String hostname, int port) throws IOException {
// Initialize time-stamp
for (int i = 0; i < MAX_SYSTEMS; ++i) {
timestamp.add(0);
}
// Connect to Controller
try {
socketToController = new Socket(hostname, port);
inputFromController = new BufferedReader(new InputStreamReader(socketToController.getInputStream()));
outputToController = new PrintWriter(socketToController.getOutputStream(), true);
} catch (UnknownHostException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
// Get Computer ID from Controller
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
identifier = Integer.parseInt(textFromController);
break;
}
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
log = new Log("client" + identifier + ".txt");
// Read start message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Start")) {
log.write("Computer is starting!");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
// Instantiate server socket
int socketPort = port + identifier + 1;
// System.out.println(socketPort);
serverSocket = new ServerSocket(socketPort);
log.write("Server Socket Instantiated");
// Instantiate sockets for other server sockets (computers) to send
for (int i = 0; i < MAX_SYSTEMS; ++i) {
if (i != identifier) {
Socket acceptedSocket = new Socket(hostname, port + i + 1);
ClientSocket socketToComputer = new ClientSocket (acceptedSocket);
outputClients.add(socketToComputer);
}
}
log.write("Client Sockets Instantiatedn");
// Accept sockets from server socket and add them into a list
for (int i = 0; i < MAX_SYSTEMS - 1; ++i) {
ClientConnection computerConn = new ClientConnection(serverSocket.accept());
computerConn.start();
inputClients.add(computerConn);
}
log.write("Server connected to clients");
Random rand = new Random();
// Generating events
int temp;
while (eventCount < 50) {
log.write("Generating Event");
int choice = rand.nextInt(5);
if (choice == 0) {
temp = timestamp.get(identifier);
++temp;
timestamp.set(identifier, temp);
} else {
int randC = rand.nextInt(outputClients.size());
ClientSocket cc = outputClients.get(randC);
cc.out.writeObject(new Event(identifier, timestamp));
}
log.write(timestamp.toString());
log.write("Done Generating Event");
eventCount++;
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Finished writing. Continue reading...");
/**
* ========THE ISSUE IS BELOW.===============
*/
synchronized (outputToController) {
outputToController.println("Finish");
outputToController.flush();
}
log.write("Sent Finish Message " + identifier);
// Wait for Tear Down Message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Tear Down")) {
log.write("Tearing down....");
isAlive = false;
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Computer shutting off....");
}
// client socket class (organizing)
public class ClientSocket {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
public ClientSocket(Socket s) {
try {
this.socket = s;
this.out = new ObjectOutputStream(socket.getOutputStream());
} catch (IOException e) {
e.printStackTrace();
}
log.write("Client Socket Created");
}
}
// send event thread
public class ClientConnection extends Thread {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
Random rand = new Random();
public ClientConnection(Socket s) {
this.socket = s;
try {
out = new ObjectOutputStream (socket.getOutputStream());
in = new ObjectInputStream (socket.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run () {
while (isAlive) {
log.write("Reading events");
try {
Event event = (Event) in.readObject();
executeEvent(event.getFromID(), event.getTimestamp());
} catch (ClassNotFoundException e) {
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(timestamp);
}
log.write("Finished Reading");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// execute the event
private void executeEvent(int from, Vector<Integer> x) {
int temp;
synchronized (timestamp) {
for (int i = 0; i < timestamp.size(); ++i) {
if (x.get(i) > timestamp.get(i)) {
timestamp.set(i, x.get(i));
}
}
temp = timestamp.get(from);
++temp;
timestamp.set(from, temp);
}
}
}
}
Controller.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
public class Controller {
// Mutex Lock
public final static Object lock = new Object();
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Server connection threads to computers
static ArrayList<ServerConnection> conns = new ArrayList<ServerConnection>();
// Finished computers
static int finishedCount = 0;
// Server Socket
ServerSocket ss;
// Log Instance
Log log;
public static void main(String args) throws IOException {
new Controller(8000);
}
public Controller(int port) {
// Instantiate Log
log = new Log("server.txt");
// Instantiate Server Socket and Listen for Incoming Sockets
try {
ss = new ServerSocket(port);
log.write("Listening...");
// Accept computers until capacity
for (int i = 0; i < MAX_SYSTEMS; i++) {
Socket s = ss.accept();
log.write("Socket connected");
// Add to list
ServerConnection conn = new ServerConnection(i, s);
conns.add(conn);
conn.start();
}
// Notify all waiting threads to start
synchronized (lock) {
try {
Thread.sleep(1000);
lock.notifyAll();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
private class ServerConnection extends Thread {
// Client Socket
Socket socket;
// Output stream
PrintWriter out;
// Input stream
BufferedReader in;
// ID for connected computer
int identifier;
public ServerConnection(int i, Socket s) {
// Instantiate properties
this.identifier = i;
this.socket = s;
try {
this.in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
this.out = new PrintWriter(socket.getOutputStream(), true);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run() {
log.write("Controller is connected to computer#" + identifier);
// Send ID to computer
out.println(identifier);
// Wait until notified
synchronized (lock) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send Start Message to All Computers
sendAll("Start");
waitForFinish();
log.write("Computer#" + identifier + " is waiting for tear down.");
// If all computers sent the Finish message, send a Tear Down
while (true) {
if (finishedCount == conns.size()) {
log.write("Sending tear down to all computers");
sendAll("Tear Down");
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
/**
* ==== RECEIVE FINISH MESSAGE FROM COMPUTERS ======
*/
private void waitForFinish() {
String clientInput;
while (true) {
try {
if (in.ready()) {
clientInput = in.readLine();
log.write(clientInput);
if (clientInput.equals("Finish")) {
finishedCount += 1;
log.write("Computer " + identifier + " is finished");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send all "text" to all computers in the thread pool
private void sendAll(String text) {
for (int i = 0; i < conns.size(); ++i) {
ServerConnection conn = conns.get(i);
conn.out.println(text);
}
}
}
}
Controller Log: (notice that the log does not say computer 3 is finished)
Listening... (Listening for Computer)
Socket connected
Controller is connected to computer#0
Socket connected
Controller is connected to computer#1
Socket connected
Controller is connected to computer#2
Socket connected
Controller is connected to computer#3
Computer 2 is finished
Computer 1 is finished
Computer#2 is waiting for tear down.
Computer 0 is finished
Computer#1 is waiting for tear down.
Computer#0 is waiting for tear down.
Computer #0 Log: (I cut down the log because there was a lot of reading and writing event log statements)
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
// A bunch of reading and writing events
Finished writing. Continue reading...
Sent Finish Message 0
Computer #1 Log:
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 1
Computer #2
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 2
Computer #3: According to the log, this sent the finished message to controller
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 3
java sockets serversocket
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32
add a comment |
I need to simulate a distributed system.
There is a controller and n worker computers.
The controller tells the computers when to start, and the computers will start connecting to other computers using sockets. The controller is connecting to the computers using threads. The computer will connect to other computers using threads as well.
Once they are connected to each other, they will send events to each other until they have generated x events. Once they reach x events, the computer will send a "Finish" message to the controller saying that it's done generating events, but will continue reading events from other computers.
My issue: The computers have successfully sent the Finish message to controller, except the last computer in the system. According to the logs, the last computer did send the Finish message to the controller, but the controller did not receive it. The other computers successfully sent the finish message to the controller.
If you need more information, I would be glad to provide. I have been working on this for hours and have no clue.
Computer.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Vector;
public class Computer {
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Computer's time-stamp vector
static Vector<Integer> timestamp = new Vector<Integer>();
// Computer's ID
static int identifier;
// Computer's Event Count
static int eventCount = 0;
// Computer's isAlive check
static boolean isAlive = true;
// Socket to Controller
Socket socketToController;
PrintWriter outputToController;
BufferedReader inputFromController;
String textFromController;
// Server Socket
ServerSocket serverSocket;
// Input and Output Clients
static ArrayList<ClientSocket> outputClients = new ArrayList<ClientSocket>();
static ArrayList<ClientConnection> inputClients = new ArrayList<ClientConnection>();
// Log
Log log;
public static void main(String args) throws IOException {
new Computer("127.0.0.1", 8000);
}
public Computer(String hostname, int port) throws IOException {
// Initialize time-stamp
for (int i = 0; i < MAX_SYSTEMS; ++i) {
timestamp.add(0);
}
// Connect to Controller
try {
socketToController = new Socket(hostname, port);
inputFromController = new BufferedReader(new InputStreamReader(socketToController.getInputStream()));
outputToController = new PrintWriter(socketToController.getOutputStream(), true);
} catch (UnknownHostException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
// Get Computer ID from Controller
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
identifier = Integer.parseInt(textFromController);
break;
}
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
log = new Log("client" + identifier + ".txt");
// Read start message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Start")) {
log.write("Computer is starting!");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
// Instantiate server socket
int socketPort = port + identifier + 1;
// System.out.println(socketPort);
serverSocket = new ServerSocket(socketPort);
log.write("Server Socket Instantiated");
// Instantiate sockets for other server sockets (computers) to send
for (int i = 0; i < MAX_SYSTEMS; ++i) {
if (i != identifier) {
Socket acceptedSocket = new Socket(hostname, port + i + 1);
ClientSocket socketToComputer = new ClientSocket (acceptedSocket);
outputClients.add(socketToComputer);
}
}
log.write("Client Sockets Instantiatedn");
// Accept sockets from server socket and add them into a list
for (int i = 0; i < MAX_SYSTEMS - 1; ++i) {
ClientConnection computerConn = new ClientConnection(serverSocket.accept());
computerConn.start();
inputClients.add(computerConn);
}
log.write("Server connected to clients");
Random rand = new Random();
// Generating events
int temp;
while (eventCount < 50) {
log.write("Generating Event");
int choice = rand.nextInt(5);
if (choice == 0) {
temp = timestamp.get(identifier);
++temp;
timestamp.set(identifier, temp);
} else {
int randC = rand.nextInt(outputClients.size());
ClientSocket cc = outputClients.get(randC);
cc.out.writeObject(new Event(identifier, timestamp));
}
log.write(timestamp.toString());
log.write("Done Generating Event");
eventCount++;
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Finished writing. Continue reading...");
/**
* ========THE ISSUE IS BELOW.===============
*/
synchronized (outputToController) {
outputToController.println("Finish");
outputToController.flush();
}
log.write("Sent Finish Message " + identifier);
// Wait for Tear Down Message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Tear Down")) {
log.write("Tearing down....");
isAlive = false;
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Computer shutting off....");
}
// client socket class (organizing)
public class ClientSocket {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
public ClientSocket(Socket s) {
try {
this.socket = s;
this.out = new ObjectOutputStream(socket.getOutputStream());
} catch (IOException e) {
e.printStackTrace();
}
log.write("Client Socket Created");
}
}
// send event thread
public class ClientConnection extends Thread {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
Random rand = new Random();
public ClientConnection(Socket s) {
this.socket = s;
try {
out = new ObjectOutputStream (socket.getOutputStream());
in = new ObjectInputStream (socket.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run () {
while (isAlive) {
log.write("Reading events");
try {
Event event = (Event) in.readObject();
executeEvent(event.getFromID(), event.getTimestamp());
} catch (ClassNotFoundException e) {
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(timestamp);
}
log.write("Finished Reading");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// execute the event
private void executeEvent(int from, Vector<Integer> x) {
int temp;
synchronized (timestamp) {
for (int i = 0; i < timestamp.size(); ++i) {
if (x.get(i) > timestamp.get(i)) {
timestamp.set(i, x.get(i));
}
}
temp = timestamp.get(from);
++temp;
timestamp.set(from, temp);
}
}
}
}
Controller.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
public class Controller {
// Mutex Lock
public final static Object lock = new Object();
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Server connection threads to computers
static ArrayList<ServerConnection> conns = new ArrayList<ServerConnection>();
// Finished computers
static int finishedCount = 0;
// Server Socket
ServerSocket ss;
// Log Instance
Log log;
public static void main(String args) throws IOException {
new Controller(8000);
}
public Controller(int port) {
// Instantiate Log
log = new Log("server.txt");
// Instantiate Server Socket and Listen for Incoming Sockets
try {
ss = new ServerSocket(port);
log.write("Listening...");
// Accept computers until capacity
for (int i = 0; i < MAX_SYSTEMS; i++) {
Socket s = ss.accept();
log.write("Socket connected");
// Add to list
ServerConnection conn = new ServerConnection(i, s);
conns.add(conn);
conn.start();
}
// Notify all waiting threads to start
synchronized (lock) {
try {
Thread.sleep(1000);
lock.notifyAll();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
private class ServerConnection extends Thread {
// Client Socket
Socket socket;
// Output stream
PrintWriter out;
// Input stream
BufferedReader in;
// ID for connected computer
int identifier;
public ServerConnection(int i, Socket s) {
// Instantiate properties
this.identifier = i;
this.socket = s;
try {
this.in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
this.out = new PrintWriter(socket.getOutputStream(), true);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run() {
log.write("Controller is connected to computer#" + identifier);
// Send ID to computer
out.println(identifier);
// Wait until notified
synchronized (lock) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send Start Message to All Computers
sendAll("Start");
waitForFinish();
log.write("Computer#" + identifier + " is waiting for tear down.");
// If all computers sent the Finish message, send a Tear Down
while (true) {
if (finishedCount == conns.size()) {
log.write("Sending tear down to all computers");
sendAll("Tear Down");
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
/**
* ==== RECEIVE FINISH MESSAGE FROM COMPUTERS ======
*/
private void waitForFinish() {
String clientInput;
while (true) {
try {
if (in.ready()) {
clientInput = in.readLine();
log.write(clientInput);
if (clientInput.equals("Finish")) {
finishedCount += 1;
log.write("Computer " + identifier + " is finished");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send all "text" to all computers in the thread pool
private void sendAll(String text) {
for (int i = 0; i < conns.size(); ++i) {
ServerConnection conn = conns.get(i);
conn.out.println(text);
}
}
}
}
Controller Log: (notice that the log does not say computer 3 is finished)
Listening... (Listening for Computer)
Socket connected
Controller is connected to computer#0
Socket connected
Controller is connected to computer#1
Socket connected
Controller is connected to computer#2
Socket connected
Controller is connected to computer#3
Computer 2 is finished
Computer 1 is finished
Computer#2 is waiting for tear down.
Computer 0 is finished
Computer#1 is waiting for tear down.
Computer#0 is waiting for tear down.
Computer #0 Log: (I cut down the log because there was a lot of reading and writing event log statements)
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
// A bunch of reading and writing events
Finished writing. Continue reading...
Sent Finish Message 0
Computer #1 Log:
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 1
Computer #2
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 2
Computer #3: According to the log, this sent the finished message to controller
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 3
java sockets serversocket
I need to simulate a distributed system.
There is a controller and n worker computers.
The controller tells the computers when to start, and the computers will start connecting to other computers using sockets. The controller is connecting to the computers using threads. The computer will connect to other computers using threads as well.
Once they are connected to each other, they will send events to each other until they have generated x events. Once they reach x events, the computer will send a "Finish" message to the controller saying that it's done generating events, but will continue reading events from other computers.
My issue: The computers have successfully sent the Finish message to controller, except the last computer in the system. According to the logs, the last computer did send the Finish message to the controller, but the controller did not receive it. The other computers successfully sent the finish message to the controller.
If you need more information, I would be glad to provide. I have been working on this for hours and have no clue.
Computer.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.io.ObjectInputStream;
import java.io.ObjectOutputStream;
import java.net.ServerSocket;
import java.net.Socket;
import java.net.UnknownHostException;
import java.util.ArrayList;
import java.util.Random;
import java.util.Vector;
public class Computer {
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Computer's time-stamp vector
static Vector<Integer> timestamp = new Vector<Integer>();
// Computer's ID
static int identifier;
// Computer's Event Count
static int eventCount = 0;
// Computer's isAlive check
static boolean isAlive = true;
// Socket to Controller
Socket socketToController;
PrintWriter outputToController;
BufferedReader inputFromController;
String textFromController;
// Server Socket
ServerSocket serverSocket;
// Input and Output Clients
static ArrayList<ClientSocket> outputClients = new ArrayList<ClientSocket>();
static ArrayList<ClientConnection> inputClients = new ArrayList<ClientConnection>();
// Log
Log log;
public static void main(String args) throws IOException {
new Computer("127.0.0.1", 8000);
}
public Computer(String hostname, int port) throws IOException {
// Initialize time-stamp
for (int i = 0; i < MAX_SYSTEMS; ++i) {
timestamp.add(0);
}
// Connect to Controller
try {
socketToController = new Socket(hostname, port);
inputFromController = new BufferedReader(new InputStreamReader(socketToController.getInputStream()));
outputToController = new PrintWriter(socketToController.getOutputStream(), true);
} catch (UnknownHostException e1) {
e1.printStackTrace();
} catch (IOException e1) {
e1.printStackTrace();
}
// Get Computer ID from Controller
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
identifier = Integer.parseInt(textFromController);
break;
}
} catch (NumberFormatException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
log = new Log("client" + identifier + ".txt");
// Read start message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Start")) {
log.write("Computer is starting!");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
// Instantiate server socket
int socketPort = port + identifier + 1;
// System.out.println(socketPort);
serverSocket = new ServerSocket(socketPort);
log.write("Server Socket Instantiated");
// Instantiate sockets for other server sockets (computers) to send
for (int i = 0; i < MAX_SYSTEMS; ++i) {
if (i != identifier) {
Socket acceptedSocket = new Socket(hostname, port + i + 1);
ClientSocket socketToComputer = new ClientSocket (acceptedSocket);
outputClients.add(socketToComputer);
}
}
log.write("Client Sockets Instantiatedn");
// Accept sockets from server socket and add them into a list
for (int i = 0; i < MAX_SYSTEMS - 1; ++i) {
ClientConnection computerConn = new ClientConnection(serverSocket.accept());
computerConn.start();
inputClients.add(computerConn);
}
log.write("Server connected to clients");
Random rand = new Random();
// Generating events
int temp;
while (eventCount < 50) {
log.write("Generating Event");
int choice = rand.nextInt(5);
if (choice == 0) {
temp = timestamp.get(identifier);
++temp;
timestamp.set(identifier, temp);
} else {
int randC = rand.nextInt(outputClients.size());
ClientSocket cc = outputClients.get(randC);
cc.out.writeObject(new Event(identifier, timestamp));
}
log.write(timestamp.toString());
log.write("Done Generating Event");
eventCount++;
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Finished writing. Continue reading...");
/**
* ========THE ISSUE IS BELOW.===============
*/
synchronized (outputToController) {
outputToController.println("Finish");
outputToController.flush();
}
log.write("Sent Finish Message " + identifier);
// Wait for Tear Down Message
while (true) {
try {
if (inputFromController.ready()) {
textFromController = inputFromController.readLine();
if (textFromController.equals("Tear Down")) {
log.write("Tearing down....");
isAlive = false;
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
log.write("Computer shutting off....");
}
// client socket class (organizing)
public class ClientSocket {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
public ClientSocket(Socket s) {
try {
this.socket = s;
this.out = new ObjectOutputStream(socket.getOutputStream());
} catch (IOException e) {
e.printStackTrace();
}
log.write("Client Socket Created");
}
}
// send event thread
public class ClientConnection extends Thread {
Socket socket;
ObjectOutputStream out;
ObjectInputStream in;
Random rand = new Random();
public ClientConnection(Socket s) {
this.socket = s;
try {
out = new ObjectOutputStream (socket.getOutputStream());
in = new ObjectInputStream (socket.getInputStream());
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run () {
while (isAlive) {
log.write("Reading events");
try {
Event event = (Event) in.readObject();
executeEvent(event.getFromID(), event.getTimestamp());
} catch (ClassNotFoundException e) {
} catch (IOException e) {
e.printStackTrace();
}
System.out.println(timestamp);
}
log.write("Finished Reading");
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// execute the event
private void executeEvent(int from, Vector<Integer> x) {
int temp;
synchronized (timestamp) {
for (int i = 0; i < timestamp.size(); ++i) {
if (x.get(i) > timestamp.get(i)) {
timestamp.set(i, x.get(i));
}
}
temp = timestamp.get(from);
++temp;
timestamp.set(from, temp);
}
}
}
}
Controller.java
package timetableexchange;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.PrintWriter;
import java.io.InputStreamReader;
import java.net.ServerSocket;
import java.net.Socket;
import java.util.ArrayList;
public class Controller {
// Mutex Lock
public final static Object lock = new Object();
// Constant system capacity
static final int MAX_SYSTEMS = 4;
// Server connection threads to computers
static ArrayList<ServerConnection> conns = new ArrayList<ServerConnection>();
// Finished computers
static int finishedCount = 0;
// Server Socket
ServerSocket ss;
// Log Instance
Log log;
public static void main(String args) throws IOException {
new Controller(8000);
}
public Controller(int port) {
// Instantiate Log
log = new Log("server.txt");
// Instantiate Server Socket and Listen for Incoming Sockets
try {
ss = new ServerSocket(port);
log.write("Listening...");
// Accept computers until capacity
for (int i = 0; i < MAX_SYSTEMS; i++) {
Socket s = ss.accept();
log.write("Socket connected");
// Add to list
ServerConnection conn = new ServerConnection(i, s);
conns.add(conn);
conn.start();
}
// Notify all waiting threads to start
synchronized (lock) {
try {
Thread.sleep(1000);
lock.notifyAll();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
private class ServerConnection extends Thread {
// Client Socket
Socket socket;
// Output stream
PrintWriter out;
// Input stream
BufferedReader in;
// ID for connected computer
int identifier;
public ServerConnection(int i, Socket s) {
// Instantiate properties
this.identifier = i;
this.socket = s;
try {
this.in = new BufferedReader(new InputStreamReader(socket.getInputStream()));
this.out = new PrintWriter(socket.getOutputStream(), true);
} catch (IOException e) {
e.printStackTrace();
}
}
@Override
public void run() {
log.write("Controller is connected to computer#" + identifier);
// Send ID to computer
out.println(identifier);
// Wait until notified
synchronized (lock) {
try {
lock.wait();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send Start Message to All Computers
sendAll("Start");
waitForFinish();
log.write("Computer#" + identifier + " is waiting for tear down.");
// If all computers sent the Finish message, send a Tear Down
while (true) {
if (finishedCount == conns.size()) {
log.write("Sending tear down to all computers");
sendAll("Tear Down");
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
/**
* ==== RECEIVE FINISH MESSAGE FROM COMPUTERS ======
*/
private void waitForFinish() {
String clientInput;
while (true) {
try {
if (in.ready()) {
clientInput = in.readLine();
log.write(clientInput);
if (clientInput.equals("Finish")) {
finishedCount += 1;
log.write("Computer " + identifier + " is finished");
break;
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
try {
Thread.sleep(100);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
// Send all "text" to all computers in the thread pool
private void sendAll(String text) {
for (int i = 0; i < conns.size(); ++i) {
ServerConnection conn = conns.get(i);
conn.out.println(text);
}
}
}
}
Controller Log: (notice that the log does not say computer 3 is finished)
Listening... (Listening for Computer)
Socket connected
Controller is connected to computer#0
Socket connected
Controller is connected to computer#1
Socket connected
Controller is connected to computer#2
Socket connected
Controller is connected to computer#3
Computer 2 is finished
Computer 1 is finished
Computer#2 is waiting for tear down.
Computer 0 is finished
Computer#1 is waiting for tear down.
Computer#0 is waiting for tear down.
Computer #0 Log: (I cut down the log because there was a lot of reading and writing event log statements)
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
// A bunch of reading and writing events
Finished writing. Continue reading...
Sent Finish Message 0
Computer #1 Log:
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 1
Computer #2
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 2
Computer #3: According to the log, this sent the finished message to controller
Computer is starting!
Server Socket Instantiated
Client Socket Created
Client Socket Created
Client Socket Created
Client Sockets Instantiated
Reading events
Server connected to clients
Finished writing. Continue reading...
Sent Finish Message 3
java sockets serversocket
java sockets serversocket
edited Nov 22 at 20:14


Dave Newton
139k18208253
139k18208253
asked Nov 22 at 20:04


Randall Johnson
153
153
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32
add a comment |
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32
add a comment |
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437442%2fserver-did-not-read-message-from-last-client%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
active
oldest
votes
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437442%2fserver-did-not-read-message-from-last-client%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
GO6A8 1BX,poWgpkc6RJU1RGCwwvD,AhGE 9QGjfTn5WJFq6i P
Why each Controller's thread sends a "Start" to each Computer? That means each computer receives 4 "Start"s. That looks like a bug.
– Perdi Estaquel
Nov 23 at 3:48
@PerdiEstaquel each thread in the controller will send a start message to each computer. so each of them will only have 1 start message.
– Randall Johnson
Nov 23 at 21:32