Java MySQLSyntaxErrorException? Why is my SELECT statement incorrect?
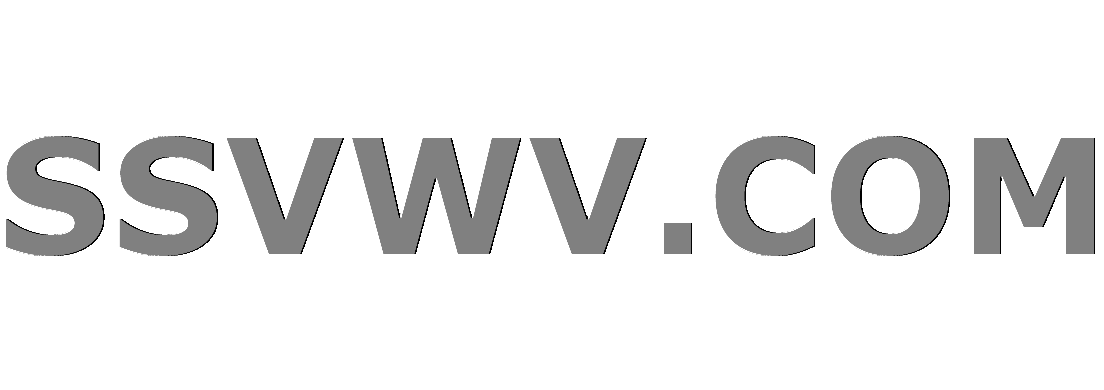
Multi tool use
This is my code for a web application that connects to a mySQL database. It is supposed to display the entire contents of one table in the database using a drop down menu on another page. I have another .jsp that does basically the same thing with a different SQL statement that performs a search operation, that works as it is supposed to. I figured that if anything I would have a problem with procedural generation of the output html tables, but I am getting a com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException exception for the mySQL statement syntax.
<%--
Document : display
Created on : Nov 22, 2018, 11:43:54 AM
Author : Scott
--%>
<%@page import="java.sql.*" %>
<%
try {
Class.forName("com.mysql.jdbc.Driver");
} catch(ClassNotFoundException e) {
System.out.println("Unable to load driver class!");
}
%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Henry Books</title>
</head>
<body>
<h1>Henry Books Store Database Table View </h1>
<%!
public class Table {
String URL = "jdbc:mysql://localhost:3306/HenryBooks?autoReconnect=true&useSSL=false";
String USERNAME = "root";
String PASSWORD = "SAvick4078100";
Connection connection = null;
PreparedStatement viewTable = null;
ResultSet resultSet = null;
public Table(){
try{
connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
viewTable = connection.prepareStatement(
"SELECT * FROM ?"
);
} catch (SQLException e) {
e.printStackTrace();
}
}
public ResultSet getTable(String tableSelection) {
try{
viewTable.setString(1, tableSelection);
resultSet = viewTable.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
return resultSet;
}
}
%>
<%
String tableSelect = new String();
//recieves input value from dropdown menu name "table option" in index.jsp
if(request.getParameter("table option") != null) {
tableSelect = request.getParameter("table option");
}
Table view = new Table();
ResultSet tableView = view.getTable(tableSelect);
ResultSetMetaData rsMetaData = tableView.getMetaData();
int cols = rsMetaData.getColumnCount();
String colNames = new String[cols];
for(int i = 0; i < cols; i++) {
colNames[i] = rsMetaData.getColumnName(i+1); //i+1 because the column name index in the ResultSetMetaData begins at 1, not 0
}
%>
<table border="1">
<thead>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<th><%= tableView.getString(colNames[i])%></th>
<% } %>
</tr>
</thead>
<tbody>
<% while(tableView.next()) { %>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<td><%= tableView.getString(colNames[i])%></td>
<% } %>
</tr>
<% } %>
</tbody>
</table>
</body>
</html>
However, I am receiving these error messages and I am not sure what is wrong with my SQL syntax here.
Severe: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''Author'' at line 1
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:62)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.lang.reflect.Constructor.newInstance(Constructor.java:423)
at com.mysql.jdbc.Util.handleNewInstance(Util.java:425)
at com.mysql.jdbc.Util.getInstance(Util.java:408)
at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:944)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3978)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3914)
at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:2530)
at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2683)
at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2495)
at com.mysql.jdbc.PreparedStatement.executeInternal(PreparedStatement.java:1903)
at com.mysql.jdbc.PreparedStatement.executeQuery(PreparedStatement.java:2011)
at org.apache.jsp.display_jsp$Table.getTable(display_jsp.java:36)
at org.apache.jsp.display_jsp._jspService(display_jsp.java:113)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception
java.lang.NullPointerException
at org.apache.jsp.display_jsp._jspService(display_jsp.java:114)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
java html mysql jsp web-applications
add a comment |
This is my code for a web application that connects to a mySQL database. It is supposed to display the entire contents of one table in the database using a drop down menu on another page. I have another .jsp that does basically the same thing with a different SQL statement that performs a search operation, that works as it is supposed to. I figured that if anything I would have a problem with procedural generation of the output html tables, but I am getting a com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException exception for the mySQL statement syntax.
<%--
Document : display
Created on : Nov 22, 2018, 11:43:54 AM
Author : Scott
--%>
<%@page import="java.sql.*" %>
<%
try {
Class.forName("com.mysql.jdbc.Driver");
} catch(ClassNotFoundException e) {
System.out.println("Unable to load driver class!");
}
%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Henry Books</title>
</head>
<body>
<h1>Henry Books Store Database Table View </h1>
<%!
public class Table {
String URL = "jdbc:mysql://localhost:3306/HenryBooks?autoReconnect=true&useSSL=false";
String USERNAME = "root";
String PASSWORD = "SAvick4078100";
Connection connection = null;
PreparedStatement viewTable = null;
ResultSet resultSet = null;
public Table(){
try{
connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
viewTable = connection.prepareStatement(
"SELECT * FROM ?"
);
} catch (SQLException e) {
e.printStackTrace();
}
}
public ResultSet getTable(String tableSelection) {
try{
viewTable.setString(1, tableSelection);
resultSet = viewTable.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
return resultSet;
}
}
%>
<%
String tableSelect = new String();
//recieves input value from dropdown menu name "table option" in index.jsp
if(request.getParameter("table option") != null) {
tableSelect = request.getParameter("table option");
}
Table view = new Table();
ResultSet tableView = view.getTable(tableSelect);
ResultSetMetaData rsMetaData = tableView.getMetaData();
int cols = rsMetaData.getColumnCount();
String colNames = new String[cols];
for(int i = 0; i < cols; i++) {
colNames[i] = rsMetaData.getColumnName(i+1); //i+1 because the column name index in the ResultSetMetaData begins at 1, not 0
}
%>
<table border="1">
<thead>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<th><%= tableView.getString(colNames[i])%></th>
<% } %>
</tr>
</thead>
<tbody>
<% while(tableView.next()) { %>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<td><%= tableView.getString(colNames[i])%></td>
<% } %>
</tr>
<% } %>
</tbody>
</table>
</body>
</html>
However, I am receiving these error messages and I am not sure what is wrong with my SQL syntax here.
Severe: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''Author'' at line 1
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:62)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.lang.reflect.Constructor.newInstance(Constructor.java:423)
at com.mysql.jdbc.Util.handleNewInstance(Util.java:425)
at com.mysql.jdbc.Util.getInstance(Util.java:408)
at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:944)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3978)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3914)
at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:2530)
at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2683)
at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2495)
at com.mysql.jdbc.PreparedStatement.executeInternal(PreparedStatement.java:1903)
at com.mysql.jdbc.PreparedStatement.executeQuery(PreparedStatement.java:2011)
at org.apache.jsp.display_jsp$Table.getTable(display_jsp.java:36)
at org.apache.jsp.display_jsp._jspService(display_jsp.java:113)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception
java.lang.NullPointerException
at org.apache.jsp.display_jsp._jspService(display_jsp.java:114)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
java html mysql jsp web-applications
1
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query asselect * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30
add a comment |
This is my code for a web application that connects to a mySQL database. It is supposed to display the entire contents of one table in the database using a drop down menu on another page. I have another .jsp that does basically the same thing with a different SQL statement that performs a search operation, that works as it is supposed to. I figured that if anything I would have a problem with procedural generation of the output html tables, but I am getting a com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException exception for the mySQL statement syntax.
<%--
Document : display
Created on : Nov 22, 2018, 11:43:54 AM
Author : Scott
--%>
<%@page import="java.sql.*" %>
<%
try {
Class.forName("com.mysql.jdbc.Driver");
} catch(ClassNotFoundException e) {
System.out.println("Unable to load driver class!");
}
%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Henry Books</title>
</head>
<body>
<h1>Henry Books Store Database Table View </h1>
<%!
public class Table {
String URL = "jdbc:mysql://localhost:3306/HenryBooks?autoReconnect=true&useSSL=false";
String USERNAME = "root";
String PASSWORD = "SAvick4078100";
Connection connection = null;
PreparedStatement viewTable = null;
ResultSet resultSet = null;
public Table(){
try{
connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
viewTable = connection.prepareStatement(
"SELECT * FROM ?"
);
} catch (SQLException e) {
e.printStackTrace();
}
}
public ResultSet getTable(String tableSelection) {
try{
viewTable.setString(1, tableSelection);
resultSet = viewTable.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
return resultSet;
}
}
%>
<%
String tableSelect = new String();
//recieves input value from dropdown menu name "table option" in index.jsp
if(request.getParameter("table option") != null) {
tableSelect = request.getParameter("table option");
}
Table view = new Table();
ResultSet tableView = view.getTable(tableSelect);
ResultSetMetaData rsMetaData = tableView.getMetaData();
int cols = rsMetaData.getColumnCount();
String colNames = new String[cols];
for(int i = 0; i < cols; i++) {
colNames[i] = rsMetaData.getColumnName(i+1); //i+1 because the column name index in the ResultSetMetaData begins at 1, not 0
}
%>
<table border="1">
<thead>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<th><%= tableView.getString(colNames[i])%></th>
<% } %>
</tr>
</thead>
<tbody>
<% while(tableView.next()) { %>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<td><%= tableView.getString(colNames[i])%></td>
<% } %>
</tr>
<% } %>
</tbody>
</table>
</body>
</html>
However, I am receiving these error messages and I am not sure what is wrong with my SQL syntax here.
Severe: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''Author'' at line 1
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:62)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.lang.reflect.Constructor.newInstance(Constructor.java:423)
at com.mysql.jdbc.Util.handleNewInstance(Util.java:425)
at com.mysql.jdbc.Util.getInstance(Util.java:408)
at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:944)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3978)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3914)
at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:2530)
at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2683)
at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2495)
at com.mysql.jdbc.PreparedStatement.executeInternal(PreparedStatement.java:1903)
at com.mysql.jdbc.PreparedStatement.executeQuery(PreparedStatement.java:2011)
at org.apache.jsp.display_jsp$Table.getTable(display_jsp.java:36)
at org.apache.jsp.display_jsp._jspService(display_jsp.java:113)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception
java.lang.NullPointerException
at org.apache.jsp.display_jsp._jspService(display_jsp.java:114)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
java html mysql jsp web-applications
This is my code for a web application that connects to a mySQL database. It is supposed to display the entire contents of one table in the database using a drop down menu on another page. I have another .jsp that does basically the same thing with a different SQL statement that performs a search operation, that works as it is supposed to. I figured that if anything I would have a problem with procedural generation of the output html tables, but I am getting a com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException exception for the mySQL statement syntax.
<%--
Document : display
Created on : Nov 22, 2018, 11:43:54 AM
Author : Scott
--%>
<%@page import="java.sql.*" %>
<%
try {
Class.forName("com.mysql.jdbc.Driver");
} catch(ClassNotFoundException e) {
System.out.println("Unable to load driver class!");
}
%>
<%@page contentType="text/html" pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Henry Books</title>
</head>
<body>
<h1>Henry Books Store Database Table View </h1>
<%!
public class Table {
String URL = "jdbc:mysql://localhost:3306/HenryBooks?autoReconnect=true&useSSL=false";
String USERNAME = "root";
String PASSWORD = "SAvick4078100";
Connection connection = null;
PreparedStatement viewTable = null;
ResultSet resultSet = null;
public Table(){
try{
connection = DriverManager.getConnection(URL, USERNAME, PASSWORD);
viewTable = connection.prepareStatement(
"SELECT * FROM ?"
);
} catch (SQLException e) {
e.printStackTrace();
}
}
public ResultSet getTable(String tableSelection) {
try{
viewTable.setString(1, tableSelection);
resultSet = viewTable.executeQuery();
} catch (SQLException e) {
e.printStackTrace();
}
return resultSet;
}
}
%>
<%
String tableSelect = new String();
//recieves input value from dropdown menu name "table option" in index.jsp
if(request.getParameter("table option") != null) {
tableSelect = request.getParameter("table option");
}
Table view = new Table();
ResultSet tableView = view.getTable(tableSelect);
ResultSetMetaData rsMetaData = tableView.getMetaData();
int cols = rsMetaData.getColumnCount();
String colNames = new String[cols];
for(int i = 0; i < cols; i++) {
colNames[i] = rsMetaData.getColumnName(i+1); //i+1 because the column name index in the ResultSetMetaData begins at 1, not 0
}
%>
<table border="1">
<thead>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<th><%= tableView.getString(colNames[i])%></th>
<% } %>
</tr>
</thead>
<tbody>
<% while(tableView.next()) { %>
<tr>
<% for(int i = 0; i < cols; i++) { %>
<td><%= tableView.getString(colNames[i])%></td>
<% } %>
</tr>
<% } %>
</tbody>
</table>
</body>
</html>
However, I am receiving these error messages and I am not sure what is wrong with my SQL syntax here.
Severe: com.mysql.jdbc.exceptions.jdbc4.MySQLSyntaxErrorException: You have an error in your SQL syntax; check the manual that corresponds to your MySQL server version for the right syntax to use near ''Author'' at line 1
at sun.reflect.NativeConstructorAccessorImpl.newInstance0(Native Method)
at sun.reflect.NativeConstructorAccessorImpl.newInstance(NativeConstructorAccessorImpl.java:62)
at sun.reflect.DelegatingConstructorAccessorImpl.newInstance(DelegatingConstructorAccessorImpl.java:45)
at java.lang.reflect.Constructor.newInstance(Constructor.java:423)
at com.mysql.jdbc.Util.handleNewInstance(Util.java:425)
at com.mysql.jdbc.Util.getInstance(Util.java:408)
at com.mysql.jdbc.SQLError.createSQLException(SQLError.java:944)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3978)
at com.mysql.jdbc.MysqlIO.checkErrorPacket(MysqlIO.java:3914)
at com.mysql.jdbc.MysqlIO.sendCommand(MysqlIO.java:2530)
at com.mysql.jdbc.MysqlIO.sqlQueryDirect(MysqlIO.java:2683)
at com.mysql.jdbc.ConnectionImpl.execSQL(ConnectionImpl.java:2495)
at com.mysql.jdbc.PreparedStatement.executeInternal(PreparedStatement.java:1903)
at com.mysql.jdbc.PreparedStatement.executeQuery(PreparedStatement.java:2011)
at org.apache.jsp.display_jsp$Table.getTable(display_jsp.java:36)
at org.apache.jsp.display_jsp._jspService(display_jsp.java:113)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception
java.lang.NullPointerException
at org.apache.jsp.display_jsp._jspService(display_jsp.java:114)
at org.apache.jasper.runtime.HttpJspBase.service(HttpJspBase.java:111)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.jasper.servlet.JspServletWrapper.service(JspServletWrapper.java:411)
at org.apache.jasper.servlet.JspServlet.serviceJspFile(JspServlet.java:473)
at org.apache.jasper.servlet.JspServlet.service(JspServlet.java:377)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:790)
at org.apache.catalina.core.StandardWrapper.service(StandardWrapper.java:1682)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:318)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:160)
at org.apache.catalina.core.StandardPipeline.doInvoke(StandardPipeline.java:734)
at org.apache.catalina.core.StandardPipeline.invoke(StandardPipeline.java:673)
at com.sun.enterprise.web.WebPipeline.invoke(WebPipeline.java:99)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:174)
at org.apache.catalina.connector.CoyoteAdapter.doService(CoyoteAdapter.java:416)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:283)
at com.sun.enterprise.v3.services.impl.ContainerMapper$HttpHandlerCallable.call(ContainerMapper.java:459)
at com.sun.enterprise.v3.services.impl.ContainerMapper.service(ContainerMapper.java:167)
at org.glassfish.grizzly.http.server.HttpHandler.runService(HttpHandler.java:206)
at org.glassfish.grizzly.http.server.HttpHandler.doHandle(HttpHandler.java:180)
at org.glassfish.grizzly.http.server.HttpServerFilter.handleRead(HttpServerFilter.java:235)
at org.glassfish.grizzly.filterchain.ExecutorResolver$9.execute(ExecutorResolver.java:119)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeFilter(DefaultFilterChain.java:283)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.executeChainPart(DefaultFilterChain.java:200)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.execute(DefaultFilterChain.java:132)
at org.glassfish.grizzly.filterchain.DefaultFilterChain.process(DefaultFilterChain.java:111)
at org.glassfish.grizzly.ProcessorExecutor.execute(ProcessorExecutor.java:77)
at org.glassfish.grizzly.nio.transport.TCPNIOTransport.fireIOEvent(TCPNIOTransport.java:536)
at org.glassfish.grizzly.strategies.AbstractIOStrategy.fireIOEvent(AbstractIOStrategy.java:112)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.run0(WorkerThreadIOStrategy.java:117)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy.access$100(WorkerThreadIOStrategy.java:56)
at org.glassfish.grizzly.strategies.WorkerThreadIOStrategy$WorkerThreadRunnable.run(WorkerThreadIOStrategy.java:137)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.doWork(AbstractThreadPool.java:591)
at org.glassfish.grizzly.threadpool.AbstractThreadPool$Worker.run(AbstractThreadPool.java:571)
at java.lang.Thread.run(Thread.java:748)
java html mysql jsp web-applications
java html mysql jsp web-applications
asked Nov 22 at 20:28


Scott
82
82
1
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query asselect * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30
add a comment |
1
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query asselect * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30
1
1
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query as
select * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query as
select * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30
add a comment |
1 Answer
1
active
oldest
votes
using
"SELECT * FROM ?"
for dynamically adding table name will lede to query being
SELECT * FROM 'TABLE_NAME'
instead append table name dynamically by just contacting the string like this:
"SELECT * FROM "+tableName
where tableName will be a variable of type string.
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437652%2fjava-mysqlsyntaxerrorexception-why-is-my-select-statement-incorrect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
using
"SELECT * FROM ?"
for dynamically adding table name will lede to query being
SELECT * FROM 'TABLE_NAME'
instead append table name dynamically by just contacting the string like this:
"SELECT * FROM "+tableName
where tableName will be a variable of type string.
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
add a comment |
using
"SELECT * FROM ?"
for dynamically adding table name will lede to query being
SELECT * FROM 'TABLE_NAME'
instead append table name dynamically by just contacting the string like this:
"SELECT * FROM "+tableName
where tableName will be a variable of type string.
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
add a comment |
using
"SELECT * FROM ?"
for dynamically adding table name will lede to query being
SELECT * FROM 'TABLE_NAME'
instead append table name dynamically by just contacting the string like this:
"SELECT * FROM "+tableName
where tableName will be a variable of type string.
using
"SELECT * FROM ?"
for dynamically adding table name will lede to query being
SELECT * FROM 'TABLE_NAME'
instead append table name dynamically by just contacting the string like this:
"SELECT * FROM "+tableName
where tableName will be a variable of type string.
answered Nov 22 at 21:00


Harshal
42627
42627
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
add a comment |
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Thanks, that seemed to get rid of the SQL syntax error, but now I have this exception Warning: StandardWrapperValve[jsp]: Servlet.service() for servlet jsp threw exception java.sql.SQLException: Before start of result set
– Scott
Nov 24 at 16:31
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
Nevermind, figured that one out. Thanks for the help!
– Scott
Nov 24 at 17:24
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53437652%2fjava-mysqlsyntaxerrorexception-why-is-my-select-statement-incorrect%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
ueeDJr3Kv6zKK6QG
1
Don't think you can have ? as tablename. ? is only for value of column parameters in query. So you should write your query as
select * from tablename
– Pushpesh Kumar Rajwanshi
Nov 22 at 20:30