How to use ProjectTo with Automapper 8.0 Dependency injection
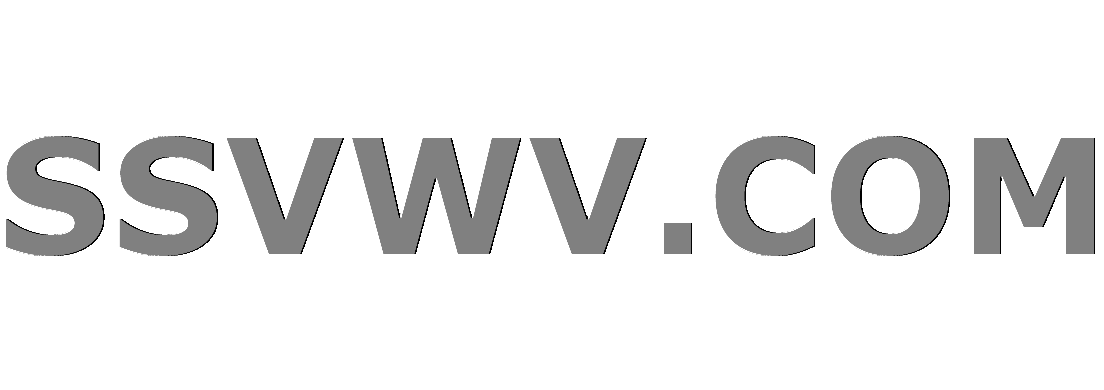
Multi tool use
I'm using Automapper's (8.0) DI pattern in my project and am looking to start using ProjectTo in my Entity Framework Core entity queries.
Here is an example of what I have managed to get to work:
public async Task<IEnumerable<SomeViewModel>> GetStuffAsync() {
return await _dbContext.SomeEntity
.ProjectTo<SomeViewModel>(_mapper.ConfigurationProvider)
.ToListAsync();
}
The above call returns all the expected records but it requires both injecting IMapper in the constructor of the repository as well as a Using reference to AutoMapper.QueryableExtensions.
There are two versions of the AutoMapper docs that I have found and they seem to provide conflicting info.
These docs https://automapperdocs.readthedocs.io/en/latest/Dependency-injection.html state the following:
Using DI is effectively mutually exclusive with using the
IQueryable.ProjectTo extension method. Use
IEnumerable.Select(_mapper.Map).ToList() instead.
And these docs http://docs.automapper.org/en/stable/Dependency-injection.html state this:
Starting with 8.0 you can use IMapper.ProjectTo. For older versions
you need to pass the configuration to the extension method
IQueryable.ProjectTo(IConfigurationProvider).
Following the first documentation's example, I converted my query to this:
public IEnumerable<SomeViewModel> GetStuff() {
return _dbContext.SomeEntity
.Select(_mapper.Map<SomeViewModel>)
.ToList();
}
However, that method returns 0 records (the previous returned everything) and the method needed to be converted from Async to synchronous as ToListAsync() wasn't supported by the extended Select. Clearly I'm missing something. Also, I'm not sure if that is the correct technique as the second set of docs talk about using IMapper.ProjectTo for version 8.0 without passing the configuaration to the extension method. How do I do that?
entity-framework-core automapper
add a comment |
I'm using Automapper's (8.0) DI pattern in my project and am looking to start using ProjectTo in my Entity Framework Core entity queries.
Here is an example of what I have managed to get to work:
public async Task<IEnumerable<SomeViewModel>> GetStuffAsync() {
return await _dbContext.SomeEntity
.ProjectTo<SomeViewModel>(_mapper.ConfigurationProvider)
.ToListAsync();
}
The above call returns all the expected records but it requires both injecting IMapper in the constructor of the repository as well as a Using reference to AutoMapper.QueryableExtensions.
There are two versions of the AutoMapper docs that I have found and they seem to provide conflicting info.
These docs https://automapperdocs.readthedocs.io/en/latest/Dependency-injection.html state the following:
Using DI is effectively mutually exclusive with using the
IQueryable.ProjectTo extension method. Use
IEnumerable.Select(_mapper.Map).ToList() instead.
And these docs http://docs.automapper.org/en/stable/Dependency-injection.html state this:
Starting with 8.0 you can use IMapper.ProjectTo. For older versions
you need to pass the configuration to the extension method
IQueryable.ProjectTo(IConfigurationProvider).
Following the first documentation's example, I converted my query to this:
public IEnumerable<SomeViewModel> GetStuff() {
return _dbContext.SomeEntity
.Select(_mapper.Map<SomeViewModel>)
.ToList();
}
However, that method returns 0 records (the previous returned everything) and the method needed to be converted from Async to synchronous as ToListAsync() wasn't supported by the extended Select. Clearly I'm missing something. Also, I'm not sure if that is the correct technique as the second set of docs talk about using IMapper.ProjectTo for version 8.0 without passing the configuaration to the extension method. How do I do that?
entity-framework-core automapper
add a comment |
I'm using Automapper's (8.0) DI pattern in my project and am looking to start using ProjectTo in my Entity Framework Core entity queries.
Here is an example of what I have managed to get to work:
public async Task<IEnumerable<SomeViewModel>> GetStuffAsync() {
return await _dbContext.SomeEntity
.ProjectTo<SomeViewModel>(_mapper.ConfigurationProvider)
.ToListAsync();
}
The above call returns all the expected records but it requires both injecting IMapper in the constructor of the repository as well as a Using reference to AutoMapper.QueryableExtensions.
There are two versions of the AutoMapper docs that I have found and they seem to provide conflicting info.
These docs https://automapperdocs.readthedocs.io/en/latest/Dependency-injection.html state the following:
Using DI is effectively mutually exclusive with using the
IQueryable.ProjectTo extension method. Use
IEnumerable.Select(_mapper.Map).ToList() instead.
And these docs http://docs.automapper.org/en/stable/Dependency-injection.html state this:
Starting with 8.0 you can use IMapper.ProjectTo. For older versions
you need to pass the configuration to the extension method
IQueryable.ProjectTo(IConfigurationProvider).
Following the first documentation's example, I converted my query to this:
public IEnumerable<SomeViewModel> GetStuff() {
return _dbContext.SomeEntity
.Select(_mapper.Map<SomeViewModel>)
.ToList();
}
However, that method returns 0 records (the previous returned everything) and the method needed to be converted from Async to synchronous as ToListAsync() wasn't supported by the extended Select. Clearly I'm missing something. Also, I'm not sure if that is the correct technique as the second set of docs talk about using IMapper.ProjectTo for version 8.0 without passing the configuaration to the extension method. How do I do that?
entity-framework-core automapper
I'm using Automapper's (8.0) DI pattern in my project and am looking to start using ProjectTo in my Entity Framework Core entity queries.
Here is an example of what I have managed to get to work:
public async Task<IEnumerable<SomeViewModel>> GetStuffAsync() {
return await _dbContext.SomeEntity
.ProjectTo<SomeViewModel>(_mapper.ConfigurationProvider)
.ToListAsync();
}
The above call returns all the expected records but it requires both injecting IMapper in the constructor of the repository as well as a Using reference to AutoMapper.QueryableExtensions.
There are two versions of the AutoMapper docs that I have found and they seem to provide conflicting info.
These docs https://automapperdocs.readthedocs.io/en/latest/Dependency-injection.html state the following:
Using DI is effectively mutually exclusive with using the
IQueryable.ProjectTo extension method. Use
IEnumerable.Select(_mapper.Map).ToList() instead.
And these docs http://docs.automapper.org/en/stable/Dependency-injection.html state this:
Starting with 8.0 you can use IMapper.ProjectTo. For older versions
you need to pass the configuration to the extension method
IQueryable.ProjectTo(IConfigurationProvider).
Following the first documentation's example, I converted my query to this:
public IEnumerable<SomeViewModel> GetStuff() {
return _dbContext.SomeEntity
.Select(_mapper.Map<SomeViewModel>)
.ToList();
}
However, that method returns 0 records (the previous returned everything) and the method needed to be converted from Async to synchronous as ToListAsync() wasn't supported by the extended Select. Clearly I'm missing something. Also, I'm not sure if that is the correct technique as the second set of docs talk about using IMapper.ProjectTo for version 8.0 without passing the configuaration to the extension method. How do I do that?
entity-framework-core automapper
entity-framework-core automapper
asked Nov 28 '18 at 22:19
AlexAlex
443417
443417
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
Starting with 8.0 you can use IMapper.ProjectTo
This means that now IMapper
interface has a method ProjectTo
(similar to Map
). So while you still need injecting IMapper
(but you need it anyway if you were using Map
, so no difference), you don't need QueryableExtensions
and ProjectTo
extension method - you simply use the interface method (similar to Map
):
return await _mapper.ProjectTo<SomeViewModel>(dbContext.SomeEntity)
.ToListAsync();
Please note that there is fundamental difference between _mapper.ProjectTo
and Select(_mapper.Map)
- the former is translated to SQL and executed server side, while the later leads to client evaluation and needs Include
/ ThenInclude
(or lazy loading) in order to function properly.
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So theIMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)
– Ivan Stoev
Nov 29 '18 at 17:54
add a comment |
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53528967%2fhow-to-use-projectto-with-automapper-8-0-dependency-injection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Starting with 8.0 you can use IMapper.ProjectTo
This means that now IMapper
interface has a method ProjectTo
(similar to Map
). So while you still need injecting IMapper
(but you need it anyway if you were using Map
, so no difference), you don't need QueryableExtensions
and ProjectTo
extension method - you simply use the interface method (similar to Map
):
return await _mapper.ProjectTo<SomeViewModel>(dbContext.SomeEntity)
.ToListAsync();
Please note that there is fundamental difference between _mapper.ProjectTo
and Select(_mapper.Map)
- the former is translated to SQL and executed server side, while the later leads to client evaluation and needs Include
/ ThenInclude
(or lazy loading) in order to function properly.
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So theIMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)
– Ivan Stoev
Nov 29 '18 at 17:54
add a comment |
Starting with 8.0 you can use IMapper.ProjectTo
This means that now IMapper
interface has a method ProjectTo
(similar to Map
). So while you still need injecting IMapper
(but you need it anyway if you were using Map
, so no difference), you don't need QueryableExtensions
and ProjectTo
extension method - you simply use the interface method (similar to Map
):
return await _mapper.ProjectTo<SomeViewModel>(dbContext.SomeEntity)
.ToListAsync();
Please note that there is fundamental difference between _mapper.ProjectTo
and Select(_mapper.Map)
- the former is translated to SQL and executed server side, while the later leads to client evaluation and needs Include
/ ThenInclude
(or lazy loading) in order to function properly.
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So theIMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)
– Ivan Stoev
Nov 29 '18 at 17:54
add a comment |
Starting with 8.0 you can use IMapper.ProjectTo
This means that now IMapper
interface has a method ProjectTo
(similar to Map
). So while you still need injecting IMapper
(but you need it anyway if you were using Map
, so no difference), you don't need QueryableExtensions
and ProjectTo
extension method - you simply use the interface method (similar to Map
):
return await _mapper.ProjectTo<SomeViewModel>(dbContext.SomeEntity)
.ToListAsync();
Please note that there is fundamental difference between _mapper.ProjectTo
and Select(_mapper.Map)
- the former is translated to SQL and executed server side, while the later leads to client evaluation and needs Include
/ ThenInclude
(or lazy loading) in order to function properly.
Starting with 8.0 you can use IMapper.ProjectTo
This means that now IMapper
interface has a method ProjectTo
(similar to Map
). So while you still need injecting IMapper
(but you need it anyway if you were using Map
, so no difference), you don't need QueryableExtensions
and ProjectTo
extension method - you simply use the interface method (similar to Map
):
return await _mapper.ProjectTo<SomeViewModel>(dbContext.SomeEntity)
.ToListAsync();
Please note that there is fundamental difference between _mapper.ProjectTo
and Select(_mapper.Map)
- the former is translated to SQL and executed server side, while the later leads to client evaluation and needs Include
/ ThenInclude
(or lazy loading) in order to function properly.
edited Nov 29 '18 at 4:45
answered Nov 29 '18 at 4:34


Ivan StoevIvan Stoev
108k788142
108k788142
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So theIMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)
– Ivan Stoev
Nov 29 '18 at 17:54
add a comment |
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So theIMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)
– Ivan Stoev
Nov 29 '18 at 17:54
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
That did the trick. Is there a reference or example in the docs for this function that I missed?
– Alex
Nov 29 '18 at 17:33
Nope. As you know, there is no AutoMapper API reference. So the
IMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)– Ivan Stoev
Nov 29 '18 at 17:54
Nope. As you know, there is no AutoMapper API reference. So the
IMapper.ProjectTo
is only mentioned in the second link from your post. And of course the VS Intellisense :)– Ivan Stoev
Nov 29 '18 at 17:54
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53528967%2fhow-to-use-projectto-with-automapper-8-0-dependency-injection%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UCkrJ M1eAOsGSoCtspV2,2zc,WBz1NddO0DzmdDD6I6,k