Issues finding average of elements
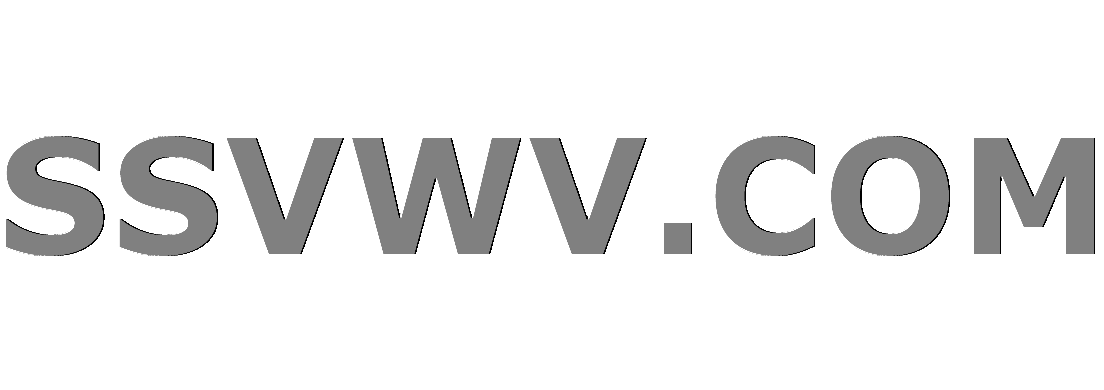
Multi tool use
Working with Repl.it and trying to use a function in C to average the elements in a variable length array. My program works fine in every other i/o area other than the average which returns:
The average for that day is: -nan. Any insight on what the issue may be?
The goal is to receive user input as a double(for example, how many pints of blood were taken per hour over a 7 hr period and then use a function call to calculate the average amount for that seven hour period.
New code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int size, float ary[*]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size;
float ave;
printf("Over how many hours do you want to view donation amounts?: ");
scanf("%d", &size);
if (size < 7 || size > 7)
size = 7;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayOne, ave);
}
printf("nnEnter day that you want to see average donation amount for: ");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0)
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayTwo, ave);
}
return 0;
}
double average(int size, float ary)
{
double sum = 0;
double ave;
for (int i = 0; i < size; i++)
sum += ary[i];
ave = (double)sum / size;
return ave;
}
c repl.it
add a comment |
Working with Repl.it and trying to use a function in C to average the elements in a variable length array. My program works fine in every other i/o area other than the average which returns:
The average for that day is: -nan. Any insight on what the issue may be?
The goal is to receive user input as a double(for example, how many pints of blood were taken per hour over a 7 hr period and then use a function call to calculate the average amount for that seven hour period.
New code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int size, float ary[*]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size;
float ave;
printf("Over how many hours do you want to view donation amounts?: ");
scanf("%d", &size);
if (size < 7 || size > 7)
size = 7;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayOne, ave);
}
printf("nnEnter day that you want to see average donation amount for: ");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0)
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayTwo, ave);
}
return 0;
}
double average(int size, float ary)
{
double sum = 0;
double ave;
for (int i = 0; i < size; i++)
sum += ary[i];
ave = (double)sum / size;
return ave;
}
c repl.it
2
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
2
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56
add a comment |
Working with Repl.it and trying to use a function in C to average the elements in a variable length array. My program works fine in every other i/o area other than the average which returns:
The average for that day is: -nan. Any insight on what the issue may be?
The goal is to receive user input as a double(for example, how many pints of blood were taken per hour over a 7 hr period and then use a function call to calculate the average amount for that seven hour period.
New code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int size, float ary[*]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size;
float ave;
printf("Over how many hours do you want to view donation amounts?: ");
scanf("%d", &size);
if (size < 7 || size > 7)
size = 7;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayOne, ave);
}
printf("nnEnter day that you want to see average donation amount for: ");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0)
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayTwo, ave);
}
return 0;
}
double average(int size, float ary)
{
double sum = 0;
double ave;
for (int i = 0; i < size; i++)
sum += ary[i];
ave = (double)sum / size;
return ave;
}
c repl.it
Working with Repl.it and trying to use a function in C to average the elements in a variable length array. My program works fine in every other i/o area other than the average which returns:
The average for that day is: -nan. Any insight on what the issue may be?
The goal is to receive user input as a double(for example, how many pints of blood were taken per hour over a 7 hr period and then use a function call to calculate the average amount for that seven hour period.
New code is as follows:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int size, float ary[*]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size;
float ave;
printf("Over how many hours do you want to view donation amounts?: ");
scanf("%d", &size);
if (size < 7 || size > 7)
size = 7;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayOne, ave);
}
printf("nnEnter day that you want to see average donation amount for: ");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0)
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
{
float ary[size];
for (int i = 0; i < size; i++)
{
printf("nEnter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
}
ave = average(size, ary);
printf("nThe average donated for %s is: %2.1f", dayTwo, ave);
}
return 0;
}
double average(int size, float ary)
{
double sum = 0;
double ave;
for (int i = 0; i < size; i++)
sum += ary[i];
ave = (double)sum / size;
return ave;
}
c repl.it
c repl.it
edited Nov 30 '18 at 0:17
Bwarr
asked Nov 28 '18 at 0:37
BwarrBwarr
42
42
2
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
2
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56
add a comment |
2
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
2
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56
2
2
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
2
2
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56
add a comment |
3 Answers
3
active
oldest
votes
This is wrong:
int ary[7];
…
scanf("%f", &ary[i]);
%f
is for scanning a float
, but ary[i]
is an int
. The resulting behavior is not defined.
This is wrong:
double size;
…
ave = average(size, ary);
Nothing in the “…” assigns a value to size
, so it has no defined value when average
is called.
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
add a comment |
Quite a few syntactical and logical changes to be made buddy. As Eric Postpischil has mentioned in his answer, your size
variable isn't initialized to anything before use. And using %f
for accepting integers is incorrect too. Along with these, there are a few other glitches which I will list below, with the corresponding fixes. I have also attached the full working code along with the output.
Error:
size
variable not initialized.
Fix:: Since you have a fixed number of hours, you can either use the
7
directly or throughsize
. Hence initialize the variable while declaring it. And just a suggestion buddy, useint
forsize
. ==>int size = 7;
Error: Accepting input for array values. The array
ary
is of typeint
, but you have used%f
which is a format specifier forfloat
.
Fix: Use
%d
which is the format specifier forint
data type. ==>scanf("%d", &ary[i]);
Error: Format specifier used for printing the average value. You have used
%f
again which is forfloat
while the variableave
for average if of typedouble
.
Fix: Use
%lf
which is the format specifier fordouble
. ==>printf("nThe average for the 2nd day is: %.3lf", ave);
The.3
beforelf
is just to limit the number of decimal points to 3. It is optional.
Error: You accept the second day and display a message if it is the same as the first day, but the
scanf
for this is out of theif
loop. hence it prompts the user to input another name of the day regardless of whether the second input day is same as the first or not.
Fix: just move that
scanf
into theif
loop where you check if that day is same as the previous one.
I wouldn't really call this one an error, because it's just an improvement which I find that needs to be done. You accept the second day which should be different from the first with the message that average would be found. But you are not calculating the average for this day. Hence I have included that part in the code.
CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int, int [7]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size = 7;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 1st day is: %.3lf", ave);
printf("nnEnter day that you want to see average donation amount for:");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0) {
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
}
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 2nd day is: %.3lf", ave);
return 0;
}
double average(int size, int ary[7])
{
int sum = 0;
double ave;
for (int i = 0; i < size; i++) {
sum = sum + ary[i];
}
ave = sum / size;
return ave;
}
OUTPUT:
Enter day that you want to see average donation amount for: Day1
Enter amount taken in hour 1: 1
Enter amount taken in hour 2: 2
Enter amount taken in hour 3: 3
Enter amount taken in hour 4: 4
Enter amount taken in hour 5: 5
Enter amount taken in hour 6: 6
Enter amount taken in hour 7: 7
The average for the 1st day is: 4.000
Enter day that you want to see average donation amount for: Day2
Enter amount taken in hour 1: 8
Enter amount taken in hour 2: 8
Enter amount taken in hour 3: 8
Enter amount taken in hour 4: 8
Enter amount taken in hour 5: 8
Enter amount taken in hour 6: 8
Enter amount taken in hour 7: 8
The average for the 2nd day is: 8.000
Hope this helps.
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
add a comment |
- double size;
The size variable has not been set before calling the average function.
ave = average(size, ary);
May be size can be initialized to 0 and incremented in the for loop as given below
double size = 0;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
size++;
}
ave = average(size, ary);
printf("nThe average for the day is: %f", ave);
}
- If the goal is to receive user input as a double, the array used to hold those values should be double.
double ary[7];
instead of
int ary[7];
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53510397%2fissues-finding-average-of-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
This is wrong:
int ary[7];
…
scanf("%f", &ary[i]);
%f
is for scanning a float
, but ary[i]
is an int
. The resulting behavior is not defined.
This is wrong:
double size;
…
ave = average(size, ary);
Nothing in the “…” assigns a value to size
, so it has no defined value when average
is called.
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
add a comment |
This is wrong:
int ary[7];
…
scanf("%f", &ary[i]);
%f
is for scanning a float
, but ary[i]
is an int
. The resulting behavior is not defined.
This is wrong:
double size;
…
ave = average(size, ary);
Nothing in the “…” assigns a value to size
, so it has no defined value when average
is called.
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
add a comment |
This is wrong:
int ary[7];
…
scanf("%f", &ary[i]);
%f
is for scanning a float
, but ary[i]
is an int
. The resulting behavior is not defined.
This is wrong:
double size;
…
ave = average(size, ary);
Nothing in the “…” assigns a value to size
, so it has no defined value when average
is called.
This is wrong:
int ary[7];
…
scanf("%f", &ary[i]);
%f
is for scanning a float
, but ary[i]
is an int
. The resulting behavior is not defined.
This is wrong:
double size;
…
ave = average(size, ary);
Nothing in the “…” assigns a value to size
, so it has no defined value when average
is called.
answered Nov 28 '18 at 1:54
Eric PostpischilEric Postpischil
78.5k883166
78.5k883166
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
add a comment |
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
Thank you. That gives me an idea on where to focus my efforts.
– Bwarr
Nov 28 '18 at 1:59
add a comment |
Quite a few syntactical and logical changes to be made buddy. As Eric Postpischil has mentioned in his answer, your size
variable isn't initialized to anything before use. And using %f
for accepting integers is incorrect too. Along with these, there are a few other glitches which I will list below, with the corresponding fixes. I have also attached the full working code along with the output.
Error:
size
variable not initialized.
Fix:: Since you have a fixed number of hours, you can either use the
7
directly or throughsize
. Hence initialize the variable while declaring it. And just a suggestion buddy, useint
forsize
. ==>int size = 7;
Error: Accepting input for array values. The array
ary
is of typeint
, but you have used%f
which is a format specifier forfloat
.
Fix: Use
%d
which is the format specifier forint
data type. ==>scanf("%d", &ary[i]);
Error: Format specifier used for printing the average value. You have used
%f
again which is forfloat
while the variableave
for average if of typedouble
.
Fix: Use
%lf
which is the format specifier fordouble
. ==>printf("nThe average for the 2nd day is: %.3lf", ave);
The.3
beforelf
is just to limit the number of decimal points to 3. It is optional.
Error: You accept the second day and display a message if it is the same as the first day, but the
scanf
for this is out of theif
loop. hence it prompts the user to input another name of the day regardless of whether the second input day is same as the first or not.
Fix: just move that
scanf
into theif
loop where you check if that day is same as the previous one.
I wouldn't really call this one an error, because it's just an improvement which I find that needs to be done. You accept the second day which should be different from the first with the message that average would be found. But you are not calculating the average for this day. Hence I have included that part in the code.
CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int, int [7]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size = 7;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 1st day is: %.3lf", ave);
printf("nnEnter day that you want to see average donation amount for:");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0) {
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
}
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 2nd day is: %.3lf", ave);
return 0;
}
double average(int size, int ary[7])
{
int sum = 0;
double ave;
for (int i = 0; i < size; i++) {
sum = sum + ary[i];
}
ave = sum / size;
return ave;
}
OUTPUT:
Enter day that you want to see average donation amount for: Day1
Enter amount taken in hour 1: 1
Enter amount taken in hour 2: 2
Enter amount taken in hour 3: 3
Enter amount taken in hour 4: 4
Enter amount taken in hour 5: 5
Enter amount taken in hour 6: 6
Enter amount taken in hour 7: 7
The average for the 1st day is: 4.000
Enter day that you want to see average donation amount for: Day2
Enter amount taken in hour 1: 8
Enter amount taken in hour 2: 8
Enter amount taken in hour 3: 8
Enter amount taken in hour 4: 8
Enter amount taken in hour 5: 8
Enter amount taken in hour 6: 8
Enter amount taken in hour 7: 8
The average for the 2nd day is: 8.000
Hope this helps.
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
add a comment |
Quite a few syntactical and logical changes to be made buddy. As Eric Postpischil has mentioned in his answer, your size
variable isn't initialized to anything before use. And using %f
for accepting integers is incorrect too. Along with these, there are a few other glitches which I will list below, with the corresponding fixes. I have also attached the full working code along with the output.
Error:
size
variable not initialized.
Fix:: Since you have a fixed number of hours, you can either use the
7
directly or throughsize
. Hence initialize the variable while declaring it. And just a suggestion buddy, useint
forsize
. ==>int size = 7;
Error: Accepting input for array values. The array
ary
is of typeint
, but you have used%f
which is a format specifier forfloat
.
Fix: Use
%d
which is the format specifier forint
data type. ==>scanf("%d", &ary[i]);
Error: Format specifier used for printing the average value. You have used
%f
again which is forfloat
while the variableave
for average if of typedouble
.
Fix: Use
%lf
which is the format specifier fordouble
. ==>printf("nThe average for the 2nd day is: %.3lf", ave);
The.3
beforelf
is just to limit the number of decimal points to 3. It is optional.
Error: You accept the second day and display a message if it is the same as the first day, but the
scanf
for this is out of theif
loop. hence it prompts the user to input another name of the day regardless of whether the second input day is same as the first or not.
Fix: just move that
scanf
into theif
loop where you check if that day is same as the previous one.
I wouldn't really call this one an error, because it's just an improvement which I find that needs to be done. You accept the second day which should be different from the first with the message that average would be found. But you are not calculating the average for this day. Hence I have included that part in the code.
CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int, int [7]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size = 7;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 1st day is: %.3lf", ave);
printf("nnEnter day that you want to see average donation amount for:");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0) {
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
}
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 2nd day is: %.3lf", ave);
return 0;
}
double average(int size, int ary[7])
{
int sum = 0;
double ave;
for (int i = 0; i < size; i++) {
sum = sum + ary[i];
}
ave = sum / size;
return ave;
}
OUTPUT:
Enter day that you want to see average donation amount for: Day1
Enter amount taken in hour 1: 1
Enter amount taken in hour 2: 2
Enter amount taken in hour 3: 3
Enter amount taken in hour 4: 4
Enter amount taken in hour 5: 5
Enter amount taken in hour 6: 6
Enter amount taken in hour 7: 7
The average for the 1st day is: 4.000
Enter day that you want to see average donation amount for: Day2
Enter amount taken in hour 1: 8
Enter amount taken in hour 2: 8
Enter amount taken in hour 3: 8
Enter amount taken in hour 4: 8
Enter amount taken in hour 5: 8
Enter amount taken in hour 6: 8
Enter amount taken in hour 7: 8
The average for the 2nd day is: 8.000
Hope this helps.
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
add a comment |
Quite a few syntactical and logical changes to be made buddy. As Eric Postpischil has mentioned in his answer, your size
variable isn't initialized to anything before use. And using %f
for accepting integers is incorrect too. Along with these, there are a few other glitches which I will list below, with the corresponding fixes. I have also attached the full working code along with the output.
Error:
size
variable not initialized.
Fix:: Since you have a fixed number of hours, you can either use the
7
directly or throughsize
. Hence initialize the variable while declaring it. And just a suggestion buddy, useint
forsize
. ==>int size = 7;
Error: Accepting input for array values. The array
ary
is of typeint
, but you have used%f
which is a format specifier forfloat
.
Fix: Use
%d
which is the format specifier forint
data type. ==>scanf("%d", &ary[i]);
Error: Format specifier used for printing the average value. You have used
%f
again which is forfloat
while the variableave
for average if of typedouble
.
Fix: Use
%lf
which is the format specifier fordouble
. ==>printf("nThe average for the 2nd day is: %.3lf", ave);
The.3
beforelf
is just to limit the number of decimal points to 3. It is optional.
Error: You accept the second day and display a message if it is the same as the first day, but the
scanf
for this is out of theif
loop. hence it prompts the user to input another name of the day regardless of whether the second input day is same as the first or not.
Fix: just move that
scanf
into theif
loop where you check if that day is same as the previous one.
I wouldn't really call this one an error, because it's just an improvement which I find that needs to be done. You accept the second day which should be different from the first with the message that average would be found. But you are not calculating the average for this day. Hence I have included that part in the code.
CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int, int [7]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size = 7;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 1st day is: %.3lf", ave);
printf("nnEnter day that you want to see average donation amount for:");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0) {
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
}
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 2nd day is: %.3lf", ave);
return 0;
}
double average(int size, int ary[7])
{
int sum = 0;
double ave;
for (int i = 0; i < size; i++) {
sum = sum + ary[i];
}
ave = sum / size;
return ave;
}
OUTPUT:
Enter day that you want to see average donation amount for: Day1
Enter amount taken in hour 1: 1
Enter amount taken in hour 2: 2
Enter amount taken in hour 3: 3
Enter amount taken in hour 4: 4
Enter amount taken in hour 5: 5
Enter amount taken in hour 6: 6
Enter amount taken in hour 7: 7
The average for the 1st day is: 4.000
Enter day that you want to see average donation amount for: Day2
Enter amount taken in hour 1: 8
Enter amount taken in hour 2: 8
Enter amount taken in hour 3: 8
Enter amount taken in hour 4: 8
Enter amount taken in hour 5: 8
Enter amount taken in hour 6: 8
Enter amount taken in hour 7: 8
The average for the 2nd day is: 8.000
Hope this helps.
Quite a few syntactical and logical changes to be made buddy. As Eric Postpischil has mentioned in his answer, your size
variable isn't initialized to anything before use. And using %f
for accepting integers is incorrect too. Along with these, there are a few other glitches which I will list below, with the corresponding fixes. I have also attached the full working code along with the output.
Error:
size
variable not initialized.
Fix:: Since you have a fixed number of hours, you can either use the
7
directly or throughsize
. Hence initialize the variable while declaring it. And just a suggestion buddy, useint
forsize
. ==>int size = 7;
Error: Accepting input for array values. The array
ary
is of typeint
, but you have used%f
which is a format specifier forfloat
.
Fix: Use
%d
which is the format specifier forint
data type. ==>scanf("%d", &ary[i]);
Error: Format specifier used for printing the average value. You have used
%f
again which is forfloat
while the variableave
for average if of typedouble
.
Fix: Use
%lf
which is the format specifier fordouble
. ==>printf("nThe average for the 2nd day is: %.3lf", ave);
The.3
beforelf
is just to limit the number of decimal points to 3. It is optional.
Error: You accept the second day and display a message if it is the same as the first day, but the
scanf
for this is out of theif
loop. hence it prompts the user to input another name of the day regardless of whether the second input day is same as the first or not.
Fix: just move that
scanf
into theif
loop where you check if that day is same as the previous one.
I wouldn't really call this one an error, because it's just an improvement which I find that needs to be done. You accept the second day which should be different from the first with the message that average would be found. But you are not calculating the average for this day. Hence I have included that part in the code.
CODE:
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
double average(int, int [7]);
int main(void)
{
char dayOne[8], dayTwo[8];
int size = 7;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 1st day is: %.3lf", ave);
printf("nnEnter day that you want to see average donation amount for:");
scanf("%s", dayTwo);
if(strcmp(dayOne, dayTwo)== 0) {
printf("nEnter a different day please: ");
scanf("%s", dayTwo);
}
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%d", &ary[i]);
}
ave = average(size, ary);
printf("nThe average for the 2nd day is: %.3lf", ave);
return 0;
}
double average(int size, int ary[7])
{
int sum = 0;
double ave;
for (int i = 0; i < size; i++) {
sum = sum + ary[i];
}
ave = sum / size;
return ave;
}
OUTPUT:
Enter day that you want to see average donation amount for: Day1
Enter amount taken in hour 1: 1
Enter amount taken in hour 2: 2
Enter amount taken in hour 3: 3
Enter amount taken in hour 4: 4
Enter amount taken in hour 5: 5
Enter amount taken in hour 6: 6
Enter amount taken in hour 7: 7
The average for the 1st day is: 4.000
Enter day that you want to see average donation amount for: Day2
Enter amount taken in hour 1: 8
Enter amount taken in hour 2: 8
Enter amount taken in hour 3: 8
Enter amount taken in hour 4: 8
Enter amount taken in hour 5: 8
Enter amount taken in hour 6: 8
Enter amount taken in hour 7: 8
The average for the 2nd day is: 8.000
Hope this helps.
answered Nov 28 '18 at 6:05


RaiRai
9831722
9831722
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
add a comment |
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
I was able to focus my attentions on the issues Eric highlighted and found the solution. Thank you very much for your input. I'll post my final code and compare it to yours to see where some changes could be made to mine to perhaps make it a bit cleaner if possible.
– Bwarr
Nov 30 '18 at 0:14
add a comment |
- double size;
The size variable has not been set before calling the average function.
ave = average(size, ary);
May be size can be initialized to 0 and incremented in the for loop as given below
double size = 0;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
size++;
}
ave = average(size, ary);
printf("nThe average for the day is: %f", ave);
}
- If the goal is to receive user input as a double, the array used to hold those values should be double.
double ary[7];
instead of
int ary[7];
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
add a comment |
- double size;
The size variable has not been set before calling the average function.
ave = average(size, ary);
May be size can be initialized to 0 and incremented in the for loop as given below
double size = 0;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
size++;
}
ave = average(size, ary);
printf("nThe average for the day is: %f", ave);
}
- If the goal is to receive user input as a double, the array used to hold those values should be double.
double ary[7];
instead of
int ary[7];
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
add a comment |
- double size;
The size variable has not been set before calling the average function.
ave = average(size, ary);
May be size can be initialized to 0 and incremented in the for loop as given below
double size = 0;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
size++;
}
ave = average(size, ary);
printf("nThe average for the day is: %f", ave);
}
- If the goal is to receive user input as a double, the array used to hold those values should be double.
double ary[7];
instead of
int ary[7];
- double size;
The size variable has not been set before calling the average function.
ave = average(size, ary);
May be size can be initialized to 0 and incremented in the for loop as given below
double size = 0;
double ave;
printf("Enter day that you want to see average donation amount for: ");
scanf("%s", dayOne);
{
int ary[7];
for (int i = 0; i < 7; i++)
{
printf("Enter amount taken in hour %d:", i + 1);
scanf("%f", &ary[i]);
size++;
}
ave = average(size, ary);
printf("nThe average for the day is: %f", ave);
}
- If the goal is to receive user input as a double, the array used to hold those values should be double.
double ary[7];
instead of
int ary[7];
answered Nov 28 '18 at 6:16


saroj pandasaroj panda
12
12
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
add a comment |
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
I was using an integer value as size of array to store a double value. For instance, an input of 5.4 for hour 1, 6.2 for hour 2.......and so on but yes, you are correct and that is what my issue was. I also used a variable length array and used an asterisk as the array size in the function definition and simply left it blank in the function definition which worked fine.
– Bwarr
Nov 30 '18 at 0:11
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53510397%2fissues-finding-average-of-elements%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
VI43g2GIrazU90 GQQ sDQvZDEG60 pNhMUqf1uPhvDwtgWcwhH xjJsvWq,eHDmOcNn J7x7W
2
We couldn't possibly diagnose the issue without seeing your code. Please edit your post and add your full/representative code in a code block. Also, give sample input/output and expected and actual behavior. See: stackoverflow.com/help/mcve
– Craig Estey
Nov 28 '18 at 0:45
Hello @Bwarr, would like to post your full code? So we can solve your problem easily.
– Alexander
Nov 28 '18 at 1:52
2
Both of the errors reported in my answer were found simply by compiling with warnings enabled. Enable warnings in your compiler. There is usually some default set of “most” warnings that provides a good selection of useful warnings. Find that setting and enable it. If you state which compiler you are using, people can help you with that.
– Eric Postpischil
Nov 28 '18 at 1:56