Vue js Form Field should be empty
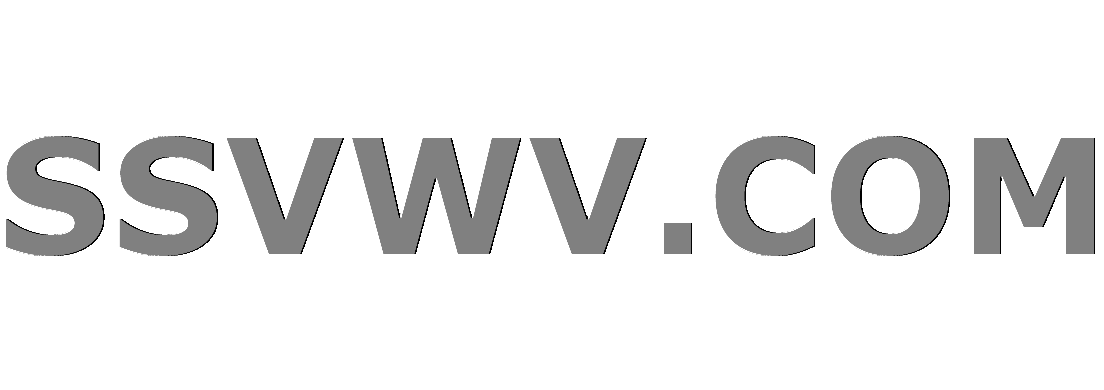
Multi tool use
I have a parent component and I am assigning values in child component form. But after submitting the form I want form field should be empty.
I am using resetFields function for resetting value but when I am using these function it is setting last value, what I send it from parent component instead of reset value I want form fields should be empty.
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
vue.js vuejs2 element-ui
add a comment |
I have a parent component and I am assigning values in child component form. But after submitting the form I want form field should be empty.
I am using resetFields function for resetting value but when I am using these function it is setting last value, what I send it from parent component instead of reset value I want form fields should be empty.
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
vue.js vuejs2 element-ui
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method oncreated()
and then recall this method on reset or after the data changes.
– ssc-hrep3
Nov 24 '18 at 8:30
add a comment |
I have a parent component and I am assigning values in child component form. But after submitting the form I want form field should be empty.
I am using resetFields function for resetting value but when I am using these function it is setting last value, what I send it from parent component instead of reset value I want form fields should be empty.
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
vue.js vuejs2 element-ui
I have a parent component and I am assigning values in child component form. But after submitting the form I want form field should be empty.
I am using resetFields function for resetting value but when I am using these function it is setting last value, what I send it from parent component instead of reset value I want form fields should be empty.
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
<script>
export default {
props: ["empData"],
data {
return() {
empInfo: {
name: "",
id: null,
age: null
}
}
},
methods: {
getUserData() {
if (response.status == "SUCCESS") {
this.$refs['empInfo'].resetFields();
}
}
}
watch: {
empData: immediate: true,
handler() {
this.empInfo = this.empData;
}
}
}
</script>
<template>
<div>
<el-form :label-position="labelPosition" :model="empInfo" status-icon :rules="rules" ref="empInfo">
<el-form-item label="Employee Name" prop="name">
<el-input v-model="empInfo.markupName"></el-input>
</el-form-item>
<el-form-item label="EMployee Id" prop="id">
<el-input v-model="empInfo.id"></el-input>
</el-form-item>
<el-form-item label="Employee Age" prop="age">
<el-input v-model="empInfo.age"></el-input>
</el-form-item>
<el-button @click="getUserData">Submit</el-button>
</div>
</template>
vue.js vuejs2 element-ui
vue.js vuejs2 element-ui
edited Nov 25 '18 at 15:41


tony19
23.5k44175
23.5k44175
asked Nov 24 '18 at 8:13
user3739946user3739946
62
62
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method oncreated()
and then recall this method on reset or after the data changes.
– ssc-hrep3
Nov 24 '18 at 8:30
add a comment |
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method oncreated()
and then recall this method on reset or after the data changes.
– ssc-hrep3
Nov 24 '18 at 8:30
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method on
created()
and then recall this method on reset or after the data changes.– ssc-hrep3
Nov 24 '18 at 8:30
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method on
created()
and then recall this method on reset or after the data changes.– ssc-hrep3
Nov 24 '18 at 8:30
add a comment |
2 Answers
2
active
oldest
votes
The Element UI form seems to latch onto the data it gets initialized with, and resetFields()
reinitializes the form with that data. You could defer the watcher until the next tick so that the component's initially empty empInfo
could be propagated to the Element UI form as initial data, which would then be used in resetFields()
.
watch: {
empData: {
immediate: true,
handler(value) {
this.$nextTick(() => {
if (value) {
this.empInfo = value;
}
});
}
}
}
demo
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
add a comment |
If you use Parent and Child component, it is good practise to emit data as output to parent component and then process data. This parent child pattern is good for making dynamic form. Reset method cleares your form.
getUserData() {
if (this.$refs.empInfo.validate()) {
this.$emit('get-user-data', this.empInfo);
this.$refs.empInfo.reset();
}
}
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456387%2fvue-js-form-field-should-be-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The Element UI form seems to latch onto the data it gets initialized with, and resetFields()
reinitializes the form with that data. You could defer the watcher until the next tick so that the component's initially empty empInfo
could be propagated to the Element UI form as initial data, which would then be used in resetFields()
.
watch: {
empData: {
immediate: true,
handler(value) {
this.$nextTick(() => {
if (value) {
this.empInfo = value;
}
});
}
}
}
demo
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
add a comment |
The Element UI form seems to latch onto the data it gets initialized with, and resetFields()
reinitializes the form with that data. You could defer the watcher until the next tick so that the component's initially empty empInfo
could be propagated to the Element UI form as initial data, which would then be used in resetFields()
.
watch: {
empData: {
immediate: true,
handler(value) {
this.$nextTick(() => {
if (value) {
this.empInfo = value;
}
});
}
}
}
demo
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
add a comment |
The Element UI form seems to latch onto the data it gets initialized with, and resetFields()
reinitializes the form with that data. You could defer the watcher until the next tick so that the component's initially empty empInfo
could be propagated to the Element UI form as initial data, which would then be used in resetFields()
.
watch: {
empData: {
immediate: true,
handler(value) {
this.$nextTick(() => {
if (value) {
this.empInfo = value;
}
});
}
}
}
demo
The Element UI form seems to latch onto the data it gets initialized with, and resetFields()
reinitializes the form with that data. You could defer the watcher until the next tick so that the component's initially empty empInfo
could be propagated to the Element UI form as initial data, which would then be used in resetFields()
.
watch: {
empData: {
immediate: true,
handler(value) {
this.$nextTick(() => {
if (value) {
this.empInfo = value;
}
});
}
}
}
demo
answered Nov 25 '18 at 15:41


tony19tony19
23.5k44175
23.5k44175
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
add a comment |
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
Thanks for ur help...Its working Fine.
– user3739946
Nov 26 '18 at 8:38
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
@user3739946 No problem :)
– tony19
Nov 26 '18 at 17:02
add a comment |
If you use Parent and Child component, it is good practise to emit data as output to parent component and then process data. This parent child pattern is good for making dynamic form. Reset method cleares your form.
getUserData() {
if (this.$refs.empInfo.validate()) {
this.$emit('get-user-data', this.empInfo);
this.$refs.empInfo.reset();
}
}
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
add a comment |
If you use Parent and Child component, it is good practise to emit data as output to parent component and then process data. This parent child pattern is good for making dynamic form. Reset method cleares your form.
getUserData() {
if (this.$refs.empInfo.validate()) {
this.$emit('get-user-data', this.empInfo);
this.$refs.empInfo.reset();
}
}
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
add a comment |
If you use Parent and Child component, it is good practise to emit data as output to parent component and then process data. This parent child pattern is good for making dynamic form. Reset method cleares your form.
getUserData() {
if (this.$refs.empInfo.validate()) {
this.$emit('get-user-data', this.empInfo);
this.$refs.empInfo.reset();
}
}
If you use Parent and Child component, it is good practise to emit data as output to parent component and then process data. This parent child pattern is good for making dynamic form. Reset method cleares your form.
getUserData() {
if (this.$refs.empInfo.validate()) {
this.$emit('get-user-data', this.empInfo);
this.$refs.empInfo.reset();
}
}
edited Nov 26 '18 at 11:36
answered Nov 25 '18 at 20:21


DaaronDaaron
149
149
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
add a comment |
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
1
1
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
Although this code might solve the question, a good answer should always explain what the problem was and how this code helps.
– BDL
Nov 26 '18 at 9:16
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53456387%2fvue-js-form-field-should-be-empty%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vEc5xFNK5AU7GfrU4,ua6nvHW0r,EPw5,M q8UENr,Z4aC5 sIEt1JPj4V9Hp GDLveytft f1umM CLGgH,KaM90Vr,qgCQAMzjhF4YA,CKQX7
You could just write a reset method in your component and initialize the values (with either empData or empty values) and call this method on
created()
and then recall this method on reset or after the data changes.– ssc-hrep3
Nov 24 '18 at 8:30