Value from radio button to int and calculate
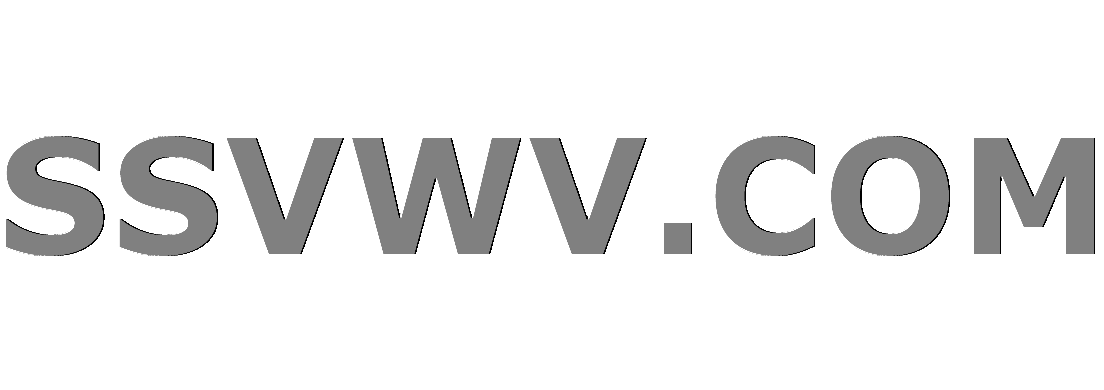
Multi tool use
up vote
1
down vote
favorite
I have just started with programming, so the answer may be obvious. My code has a radio button (price of a service) and a dropdown menu (quantity of orders), i want to calculate with these values. So far so good, it took me almost a day to get this short code.
My problem is now, I need the result to be shown realtime, possibly in an input tag like
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
Maybe there is also a better way?
So, one of the two radio buttons should always be checked and show its price. When the user chooses the quantity from the dropdown menu, the result should automatically refresh.
Can anyone help me?
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input class="test" type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input class="test" type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
</body>
Thx for your help!
edit: Ok, even the calculation is wrong. I want to get the value of a checked radio button and turn it into an int. After this I need the int to be multiplied with the value from the checkbox. I dont know what I am missing, but its always calculating with the first radio button value, even if I check the second one.
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
$(".test").click(function(event) {
var total = 0;
$(".test:checked").each(function() {
total += parseInt($(this).val());
});
if (total == 0) {
$('#amount').val('');
} else {
$('#amount').val(total);
}
});
</script>
I still got the problem, that I need the results to be shown realtime, depending which radio button and dropdown value is checked. So I added a class "test" to the radio buttons and added the above function. Now I got the result in realtime, depending on the checked radio button, but the calculation is still wrong and i need to combine it somehow.
javascript jquery html radio-button
add a comment |
up vote
1
down vote
favorite
I have just started with programming, so the answer may be obvious. My code has a radio button (price of a service) and a dropdown menu (quantity of orders), i want to calculate with these values. So far so good, it took me almost a day to get this short code.
My problem is now, I need the result to be shown realtime, possibly in an input tag like
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
Maybe there is also a better way?
So, one of the two radio buttons should always be checked and show its price. When the user chooses the quantity from the dropdown menu, the result should automatically refresh.
Can anyone help me?
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input class="test" type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input class="test" type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
</body>
Thx for your help!
edit: Ok, even the calculation is wrong. I want to get the value of a checked radio button and turn it into an int. After this I need the int to be multiplied with the value from the checkbox. I dont know what I am missing, but its always calculating with the first radio button value, even if I check the second one.
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
$(".test").click(function(event) {
var total = 0;
$(".test:checked").each(function() {
total += parseInt($(this).val());
});
if (total == 0) {
$('#amount').val('');
} else {
$('#amount').val(total);
}
});
</script>
I still got the problem, that I need the results to be shown realtime, depending which radio button and dropdown value is checked. So I added a class "test" to the radio buttons and added the above function. Now I got the result in realtime, depending on the checked radio button, but the calculation is still wrong and i need to combine it somehow.
javascript jquery html radio-button
add a comment |
up vote
1
down vote
favorite
up vote
1
down vote
favorite
I have just started with programming, so the answer may be obvious. My code has a radio button (price of a service) and a dropdown menu (quantity of orders), i want to calculate with these values. So far so good, it took me almost a day to get this short code.
My problem is now, I need the result to be shown realtime, possibly in an input tag like
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
Maybe there is also a better way?
So, one of the two radio buttons should always be checked and show its price. When the user chooses the quantity from the dropdown menu, the result should automatically refresh.
Can anyone help me?
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input class="test" type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input class="test" type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
</body>
Thx for your help!
edit: Ok, even the calculation is wrong. I want to get the value of a checked radio button and turn it into an int. After this I need the int to be multiplied with the value from the checkbox. I dont know what I am missing, but its always calculating with the first radio button value, even if I check the second one.
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
$(".test").click(function(event) {
var total = 0;
$(".test:checked").each(function() {
total += parseInt($(this).val());
});
if (total == 0) {
$('#amount').val('');
} else {
$('#amount').val(total);
}
});
</script>
I still got the problem, that I need the results to be shown realtime, depending which radio button and dropdown value is checked. So I added a class "test" to the radio buttons and added the above function. Now I got the result in realtime, depending on the checked radio button, but the calculation is still wrong and i need to combine it somehow.
javascript jquery html radio-button
I have just started with programming, so the answer may be obvious. My code has a radio button (price of a service) and a dropdown menu (quantity of orders), i want to calculate with these values. So far so good, it took me almost a day to get this short code.
My problem is now, I need the result to be shown realtime, possibly in an input tag like
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
Maybe there is also a better way?
So, one of the two radio buttons should always be checked and show its price. When the user chooses the quantity from the dropdown menu, the result should automatically refresh.
Can anyone help me?
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input class="test" type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input class="test" type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" /></p>
</body>
Thx for your help!
edit: Ok, even the calculation is wrong. I want to get the value of a checked radio button and turn it into an int. After this I need the int to be multiplied with the value from the checkbox. I dont know what I am missing, but its always calculating with the first radio button value, even if I check the second one.
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
$(".test").click(function(event) {
var total = 0;
$(".test:checked").each(function() {
total += parseInt($(this).val());
});
if (total == 0) {
$('#amount').val('');
} else {
$('#amount').val(total);
}
});
</script>
I still got the problem, that I need the results to be shown realtime, depending which radio button and dropdown value is checked. So I added a class "test" to the radio buttons and added the above function. Now I got the result in realtime, depending on the checked radio button, but the calculation is still wrong and i need to combine it somehow.
javascript jquery html radio-button
javascript jquery html radio-button
edited Nov 21 at 12:18
asked Nov 21 at 1:19
Jonah
103
103
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
up vote
0
down vote
accepted
Based off the comments above
To convert strings to int use parseInt()
as for to detecting change you need to detect to the radio buttons. Now you can go through and add onChange =
or onClick =
to all of them manually. With two why not but if you intend on having more and they will all change the outcome. You could just loop through them.
Add this to the bottom of the code
//Loop through the radio buttons, first find all the elements with that Name then you can
//loop through to to detect the onClick.
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
console.log(parseInt(this.value));
}
}
You may need to rewrite the code a bit differently, as you are dealing with two varaiblies into the code but your function is only accepting one.
So to avoid doing another loop, easier to just give it an Id and go forward. So now everytime you change the check boxes it will re run the code with whatever the current value from dropdown is.
The below does what I think you want.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" id='dropdown' name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
//Get the input Box you want to print to
var amountPrint = document.getElementById('amount');
//All the radio boxes which you named test
var radios = document.getElementsByName('test')
function calculate(val) {
var result = val * xInt;
//var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
xInt = parseInt(this.value, 10)
calculate(document.getElementById('dropdown').value)
}
}
</script>
add a comment |
up vote
2
down vote
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
</script>
You just need to push a new value to the amount area.
All I have done is added a value=""
to the Amount input, which could be skipped but it is good practice IMO.
Then in the js beneath. Get the element by id which you provided earlier.
Then use your calculation, and with that tell it to change the value of id 'amount'
You could also add <p id='printResultsHere'></p>
for example and call in much the same way.
I hope this helps. Please mark answer as accepted if so.
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
add a comment |
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
accepted
Based off the comments above
To convert strings to int use parseInt()
as for to detecting change you need to detect to the radio buttons. Now you can go through and add onChange =
or onClick =
to all of them manually. With two why not but if you intend on having more and they will all change the outcome. You could just loop through them.
Add this to the bottom of the code
//Loop through the radio buttons, first find all the elements with that Name then you can
//loop through to to detect the onClick.
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
console.log(parseInt(this.value));
}
}
You may need to rewrite the code a bit differently, as you are dealing with two varaiblies into the code but your function is only accepting one.
So to avoid doing another loop, easier to just give it an Id and go forward. So now everytime you change the check boxes it will re run the code with whatever the current value from dropdown is.
The below does what I think you want.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" id='dropdown' name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
//Get the input Box you want to print to
var amountPrint = document.getElementById('amount');
//All the radio boxes which you named test
var radios = document.getElementsByName('test')
function calculate(val) {
var result = val * xInt;
//var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
xInt = parseInt(this.value, 10)
calculate(document.getElementById('dropdown').value)
}
}
</script>
add a comment |
up vote
0
down vote
accepted
Based off the comments above
To convert strings to int use parseInt()
as for to detecting change you need to detect to the radio buttons. Now you can go through and add onChange =
or onClick =
to all of them manually. With two why not but if you intend on having more and they will all change the outcome. You could just loop through them.
Add this to the bottom of the code
//Loop through the radio buttons, first find all the elements with that Name then you can
//loop through to to detect the onClick.
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
console.log(parseInt(this.value));
}
}
You may need to rewrite the code a bit differently, as you are dealing with two varaiblies into the code but your function is only accepting one.
So to avoid doing another loop, easier to just give it an Id and go forward. So now everytime you change the check boxes it will re run the code with whatever the current value from dropdown is.
The below does what I think you want.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" id='dropdown' name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
//Get the input Box you want to print to
var amountPrint = document.getElementById('amount');
//All the radio boxes which you named test
var radios = document.getElementsByName('test')
function calculate(val) {
var result = val * xInt;
//var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
xInt = parseInt(this.value, 10)
calculate(document.getElementById('dropdown').value)
}
}
</script>
add a comment |
up vote
0
down vote
accepted
up vote
0
down vote
accepted
Based off the comments above
To convert strings to int use parseInt()
as for to detecting change you need to detect to the radio buttons. Now you can go through and add onChange =
or onClick =
to all of them manually. With two why not but if you intend on having more and they will all change the outcome. You could just loop through them.
Add this to the bottom of the code
//Loop through the radio buttons, first find all the elements with that Name then you can
//loop through to to detect the onClick.
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
console.log(parseInt(this.value));
}
}
You may need to rewrite the code a bit differently, as you are dealing with two varaiblies into the code but your function is only accepting one.
So to avoid doing another loop, easier to just give it an Id and go forward. So now everytime you change the check boxes it will re run the code with whatever the current value from dropdown is.
The below does what I think you want.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" id='dropdown' name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
//Get the input Box you want to print to
var amountPrint = document.getElementById('amount');
//All the radio boxes which you named test
var radios = document.getElementsByName('test')
function calculate(val) {
var result = val * xInt;
//var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
xInt = parseInt(this.value, 10)
calculate(document.getElementById('dropdown').value)
}
}
</script>
Based off the comments above
To convert strings to int use parseInt()
as for to detecting change you need to detect to the radio buttons. Now you can go through and add onChange =
or onClick =
to all of them manually. With two why not but if you intend on having more and they will all change the outcome. You could just loop through them.
Add this to the bottom of the code
//Loop through the radio buttons, first find all the elements with that Name then you can
//loop through to to detect the onClick.
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
console.log(parseInt(this.value));
}
}
You may need to rewrite the code a bit differently, as you are dealing with two varaiblies into the code but your function is only accepting one.
So to avoid doing another loop, easier to just give it an Id and go forward. So now everytime you change the check boxes it will re run the code with whatever the current value from dropdown is.
The below does what I think you want.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" id='dropdown' name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
//Get the input Box you want to print to
var amountPrint = document.getElementById('amount');
//All the radio boxes which you named test
var radios = document.getElementsByName('test')
function calculate(val) {
var result = val * xInt;
//var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
var radios = document.getElementsByName('test')
for (var i = 0, max = radios.length; i < max; i++) {
radios[i].onclick = function () {
xInt = parseInt(this.value, 10)
calculate(document.getElementById('dropdown').value)
}
}
</script>
edited Nov 22 at 7:01
answered Nov 22 at 6:28


Achmann
949
949
add a comment |
add a comment |
up vote
2
down vote
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
</script>
You just need to push a new value to the amount area.
All I have done is added a value=""
to the Amount input, which could be skipped but it is good practice IMO.
Then in the js beneath. Get the element by id which you provided earlier.
Then use your calculation, and with that tell it to change the value of id 'amount'
You could also add <p id='printResultsHere'></p>
for example and call in much the same way.
I hope this helps. Please mark answer as accepted if so.
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
add a comment |
up vote
2
down vote
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
</script>
You just need to push a new value to the amount area.
All I have done is added a value=""
to the Amount input, which could be skipped but it is good practice IMO.
Then in the js beneath. Get the element by id which you provided earlier.
Then use your calculation, and with that tell it to change the value of id 'amount'
You could also add <p id='printResultsHere'></p>
for example and call in much the same way.
I hope this helps. Please mark answer as accepted if so.
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
add a comment |
up vote
2
down vote
up vote
2
down vote
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
</script>
You just need to push a new value to the amount area.
All I have done is added a value=""
to the Amount input, which could be skipped but it is good practice IMO.
Then in the js beneath. Get the element by id which you provided earlier.
Then use your calculation, and with that tell it to change the value of id 'amount'
You could also add <p id='printResultsHere'></p>
for example and call in much the same way.
I hope this helps. Please mark answer as accepted if so.
<html lang="en">
<head>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js"></script>
</head>
<body>
<label>ABC
<input type="radio" checked="checked" name="test" value="500">
</label>
<label>DEF
<input type="radio" name="test" value="800">
</label>
<select size="1" name="dropdown" onchange='calculate(this.value);'>
<option value="1">1</option>
<option value="2">2</option>
<option value="3">3</option>
</select>
<p><strong>Amount (US$)</strong>: <input type="text" name="amount" id="amount" value="" /></p>
</body>
<script>
var x = $('input[name="test"]:checked').val();
var xInt = parseInt(x, 10);
function calculate (val) {
var result = val * xInt ;
var amountPrint = document.getElementById('amount');
amountPrint.value = result;
}
</script>
You just need to push a new value to the amount area.
All I have done is added a value=""
to the Amount input, which could be skipped but it is good practice IMO.
Then in the js beneath. Get the element by id which you provided earlier.
Then use your calculation, and with that tell it to change the value of id 'amount'
You could also add <p id='printResultsHere'></p>
for example and call in much the same way.
I hope this helps. Please mark answer as accepted if so.
edited Nov 21 at 3:41
Ryan Lee
564
564
answered Nov 21 at 1:24


Achmann
949
949
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
add a comment |
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
Thanks for your help. It got me one step further, but I m still missing something. I dont know why, but I got the wrong results in my calculation, apparently I was too tired when I wrote my question. I need the results always to be shown, depending which radio button is checked. Now it just shows, when I manipulate the drop down menu. And the problem with the wrong calculation still apears, it's calculating only with the first radio button value ("500"). I will edit my question....
– Jonah
Nov 21 at 11:50
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
You need to run your code again when a change is detected in the radio buttons.
– Achmann
Nov 22 at 6:10
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53403989%2fvalue-from-radio-button-to-int-and-calculate%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fWv0FHjRxMe9ATYzNnTGH7cgo nL3Ao10sbqyBl8Du364K7g3vMcQ81fCrgO FDSB ovt9aG1