Test datetime object using flask and mockupdb (mongodb)
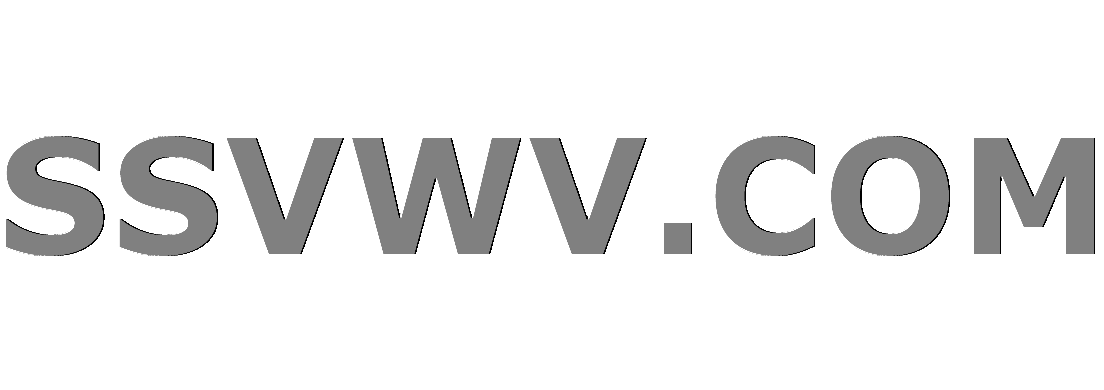
Multi tool use
I'm testing MongoDB as DB with a Flask's REST Server (and flask-pymongo), using the mockupdb module. I want to receive a DateTime in the json request, and store it as Date object, to perform some range query using this field in the future, so, I send the data as EJSON (BSON) to keep the data exactly as I.
This is the testcase:
@pytest.fixture()
def client_and_mongoserver():
random.seed()
mongo_server = MockupDB(auto_ismaster=True, verbose=True)
mongo_server.run()
config = Config()
config.MONGO_URI = mongo_server.uri + '/test'
flask_app = create_app(config)
flask_app.testing = True
client = flask_app.test_client()
yield client, mongo_server
mongo_server.stop()
def test_insert(client_and_mongoserver):
client, server = client_and_mongoserver
headers = [('Content-Type', 'application/json')]
id = str(uuid.uuid4()).encode('utf-8')[:12]
now = datetime.now()
obj_id = ObjectId(id)
toInsert = {
"_id": obj_id,
"datetime": now
}
toVerify = {
"_id": obj_id,
"datetime": now
}
future = go(client.post, '/api/insert', data=dumps(toInsert), headers=headers)
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': "test",
'$readPreference': {"mode": "primary"},
'documents': [
toVerify
]
}, namespace='test')
)
request.ok(cursor={'inserted_id': id})
# act
http_response = future()
# assert
data = http_response.get_data(as_text=True)
This is the endpoint. Before the insert call I convert the datetime string to datetime object:
from flask_restful import Resource
from bson import json_util
class Insert(Resource):
def post(self):
if not request.json:
abort(400)
json_data = json_util.loads(request.data)
result = mongo.db.test.insert_one(json_data)
return {'message': 'OK'}, 200
But the test generate this assertion:
self = MockupDB(localhost, 37213)
args = (OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"),)
kwargs = {}, timeout = 10, end = 1543504028.309115
matcher = Matcher(OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"))
request = OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
def receives(self, *args, **kwargs):
"""Pop the next `Request` and assert it matches.
Returns None if the server is stopped.
Pass a `Request` or request pattern to specify what client request to
expect. See the tutorial for examples. Pass ``timeout`` as a keyword
argument to override this server's ``request_timeout``.
"""
timeout = kwargs.pop('timeout', self._request_timeout)
end = time.time() + timeout
matcher = Matcher(*args, **kwargs)
while not self._stopped:
try:
# Short timeout so we notice if the server is stopped.
request = self._request_q.get(timeout=0.05)
except Empty:
if time.time() > end:
raise AssertionError('expected to receive %r, got nothing' % matcher.prototype)
else:
if matcher.matches(request):
return request
else:
raise AssertionError('expected to receive %r, got %r'
> % (matcher.prototype, request))
E AssertionError: expected to receive OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"), got OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
.venv/lib/python3.6/site-packages/mockupdb/__init__.py:1291: AssertionError
The value match but the assertion is raised either way.
How can I test the Date object using flask?
EDIT:
As pointed out by @bauman.space. The lack of:
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
Don't affect the validation made by mockupdb. I'd tested that in other test cases.
EDIT 2: Change question to prevent confusion
flask pymongo
add a comment |
I'm testing MongoDB as DB with a Flask's REST Server (and flask-pymongo), using the mockupdb module. I want to receive a DateTime in the json request, and store it as Date object, to perform some range query using this field in the future, so, I send the data as EJSON (BSON) to keep the data exactly as I.
This is the testcase:
@pytest.fixture()
def client_and_mongoserver():
random.seed()
mongo_server = MockupDB(auto_ismaster=True, verbose=True)
mongo_server.run()
config = Config()
config.MONGO_URI = mongo_server.uri + '/test'
flask_app = create_app(config)
flask_app.testing = True
client = flask_app.test_client()
yield client, mongo_server
mongo_server.stop()
def test_insert(client_and_mongoserver):
client, server = client_and_mongoserver
headers = [('Content-Type', 'application/json')]
id = str(uuid.uuid4()).encode('utf-8')[:12]
now = datetime.now()
obj_id = ObjectId(id)
toInsert = {
"_id": obj_id,
"datetime": now
}
toVerify = {
"_id": obj_id,
"datetime": now
}
future = go(client.post, '/api/insert', data=dumps(toInsert), headers=headers)
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': "test",
'$readPreference': {"mode": "primary"},
'documents': [
toVerify
]
}, namespace='test')
)
request.ok(cursor={'inserted_id': id})
# act
http_response = future()
# assert
data = http_response.get_data(as_text=True)
This is the endpoint. Before the insert call I convert the datetime string to datetime object:
from flask_restful import Resource
from bson import json_util
class Insert(Resource):
def post(self):
if not request.json:
abort(400)
json_data = json_util.loads(request.data)
result = mongo.db.test.insert_one(json_data)
return {'message': 'OK'}, 200
But the test generate this assertion:
self = MockupDB(localhost, 37213)
args = (OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"),)
kwargs = {}, timeout = 10, end = 1543504028.309115
matcher = Matcher(OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"))
request = OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
def receives(self, *args, **kwargs):
"""Pop the next `Request` and assert it matches.
Returns None if the server is stopped.
Pass a `Request` or request pattern to specify what client request to
expect. See the tutorial for examples. Pass ``timeout`` as a keyword
argument to override this server's ``request_timeout``.
"""
timeout = kwargs.pop('timeout', self._request_timeout)
end = time.time() + timeout
matcher = Matcher(*args, **kwargs)
while not self._stopped:
try:
# Short timeout so we notice if the server is stopped.
request = self._request_q.get(timeout=0.05)
except Empty:
if time.time() > end:
raise AssertionError('expected to receive %r, got nothing' % matcher.prototype)
else:
if matcher.matches(request):
return request
else:
raise AssertionError('expected to receive %r, got %r'
> % (matcher.prototype, request))
E AssertionError: expected to receive OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"), got OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
.venv/lib/python3.6/site-packages/mockupdb/__init__.py:1291: AssertionError
The value match but the assertion is raised either way.
How can I test the Date object using flask?
EDIT:
As pointed out by @bauman.space. The lack of:
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
Don't affect the validation made by mockupdb. I'd tested that in other test cases.
EDIT 2: Change question to prevent confusion
flask pymongo
add a comment |
I'm testing MongoDB as DB with a Flask's REST Server (and flask-pymongo), using the mockupdb module. I want to receive a DateTime in the json request, and store it as Date object, to perform some range query using this field in the future, so, I send the data as EJSON (BSON) to keep the data exactly as I.
This is the testcase:
@pytest.fixture()
def client_and_mongoserver():
random.seed()
mongo_server = MockupDB(auto_ismaster=True, verbose=True)
mongo_server.run()
config = Config()
config.MONGO_URI = mongo_server.uri + '/test'
flask_app = create_app(config)
flask_app.testing = True
client = flask_app.test_client()
yield client, mongo_server
mongo_server.stop()
def test_insert(client_and_mongoserver):
client, server = client_and_mongoserver
headers = [('Content-Type', 'application/json')]
id = str(uuid.uuid4()).encode('utf-8')[:12]
now = datetime.now()
obj_id = ObjectId(id)
toInsert = {
"_id": obj_id,
"datetime": now
}
toVerify = {
"_id": obj_id,
"datetime": now
}
future = go(client.post, '/api/insert', data=dumps(toInsert), headers=headers)
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': "test",
'$readPreference': {"mode": "primary"},
'documents': [
toVerify
]
}, namespace='test')
)
request.ok(cursor={'inserted_id': id})
# act
http_response = future()
# assert
data = http_response.get_data(as_text=True)
This is the endpoint. Before the insert call I convert the datetime string to datetime object:
from flask_restful import Resource
from bson import json_util
class Insert(Resource):
def post(self):
if not request.json:
abort(400)
json_data = json_util.loads(request.data)
result = mongo.db.test.insert_one(json_data)
return {'message': 'OK'}, 200
But the test generate this assertion:
self = MockupDB(localhost, 37213)
args = (OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"),)
kwargs = {}, timeout = 10, end = 1543504028.309115
matcher = Matcher(OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"))
request = OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
def receives(self, *args, **kwargs):
"""Pop the next `Request` and assert it matches.
Returns None if the server is stopped.
Pass a `Request` or request pattern to specify what client request to
expect. See the tutorial for examples. Pass ``timeout`` as a keyword
argument to override this server's ``request_timeout``.
"""
timeout = kwargs.pop('timeout', self._request_timeout)
end = time.time() + timeout
matcher = Matcher(*args, **kwargs)
while not self._stopped:
try:
# Short timeout so we notice if the server is stopped.
request = self._request_q.get(timeout=0.05)
except Empty:
if time.time() > end:
raise AssertionError('expected to receive %r, got nothing' % matcher.prototype)
else:
if matcher.matches(request):
return request
else:
raise AssertionError('expected to receive %r, got %r'
> % (matcher.prototype, request))
E AssertionError: expected to receive OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"), got OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
.venv/lib/python3.6/site-packages/mockupdb/__init__.py:1291: AssertionError
The value match but the assertion is raised either way.
How can I test the Date object using flask?
EDIT:
As pointed out by @bauman.space. The lack of:
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
Don't affect the validation made by mockupdb. I'd tested that in other test cases.
EDIT 2: Change question to prevent confusion
flask pymongo
I'm testing MongoDB as DB with a Flask's REST Server (and flask-pymongo), using the mockupdb module. I want to receive a DateTime in the json request, and store it as Date object, to perform some range query using this field in the future, so, I send the data as EJSON (BSON) to keep the data exactly as I.
This is the testcase:
@pytest.fixture()
def client_and_mongoserver():
random.seed()
mongo_server = MockupDB(auto_ismaster=True, verbose=True)
mongo_server.run()
config = Config()
config.MONGO_URI = mongo_server.uri + '/test'
flask_app = create_app(config)
flask_app.testing = True
client = flask_app.test_client()
yield client, mongo_server
mongo_server.stop()
def test_insert(client_and_mongoserver):
client, server = client_and_mongoserver
headers = [('Content-Type', 'application/json')]
id = str(uuid.uuid4()).encode('utf-8')[:12]
now = datetime.now()
obj_id = ObjectId(id)
toInsert = {
"_id": obj_id,
"datetime": now
}
toVerify = {
"_id": obj_id,
"datetime": now
}
future = go(client.post, '/api/insert', data=dumps(toInsert), headers=headers)
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': "test",
'$readPreference': {"mode": "primary"},
'documents': [
toVerify
]
}, namespace='test')
)
request.ok(cursor={'inserted_id': id})
# act
http_response = future()
# assert
data = http_response.get_data(as_text=True)
This is the endpoint. Before the insert call I convert the datetime string to datetime object:
from flask_restful import Resource
from bson import json_util
class Insert(Resource):
def post(self):
if not request.json:
abort(400)
json_data = json_util.loads(request.data)
result = mongo.db.test.insert_one(json_data)
return {'message': 'OK'}, 200
But the test generate this assertion:
self = MockupDB(localhost, 37213)
args = (OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"),)
kwargs = {}, timeout = 10, end = 1543504028.309115
matcher = Matcher(OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"))
request = OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
def receives(self, *args, **kwargs):
"""Pop the next `Request` and assert it matches.
Returns None if the server is stopped.
Pass a `Request` or request pattern to specify what client request to
expect. See the tutorial for examples. Pass ``timeout`` as a keyword
argument to override this server's ``request_timeout``.
"""
timeout = kwargs.pop('timeout', self._request_timeout)
end = time.time() + timeout
matcher = Matcher(*args, **kwargs)
while not self._stopped:
try:
# Short timeout so we notice if the server is stopped.
request = self._request_q.get(timeout=0.05)
except Empty:
if time.time() > end:
raise AssertionError('expected to receive %r, got nothing' % matcher.prototype)
else:
if matcher.matches(request):
return request
else:
raise AssertionError('expected to receive %r, got %r'
> % (matcher.prototype, request))
E AssertionError: expected to receive OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test"), got OpMsg({"insert": "test", "ordered": true, "$db": "test", "$readPreference": {"mode": "primary"}, "documents": [{"_id": {"$oid": "63343264363661622d393764"}, "datetime": {"$date": 1543493218306}}]}, namespace="test")
.venv/lib/python3.6/site-packages/mockupdb/__init__.py:1291: AssertionError
The value match but the assertion is raised either way.
How can I test the Date object using flask?
EDIT:
As pointed out by @bauman.space. The lack of:
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
Don't affect the validation made by mockupdb. I'd tested that in other test cases.
EDIT 2: Change question to prevent confusion
flask pymongo
flask pymongo
edited Nov 29 at 15:32
asked Nov 22 at 19:05
Jose Truyol
289
289
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
your assertion is quite descriptive
AssertionError:
expected to receive
OpMsg(
{"insert": "test",
"ordered": true,
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test"
),
got
OpMsg(
{"insert": "test",
"ordered": true,
"$db": "test",
"$readPreference": {"mode": "primary"},
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test")
looks like you simply need to include some of the standard MongoDB keys in your verification code.
Swap yours out with this and give it a try?
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
'documents': [
toVerify
]
}, namespace='test')
)
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436857%2ftest-datetime-object-using-flask-and-mockupdb-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
your assertion is quite descriptive
AssertionError:
expected to receive
OpMsg(
{"insert": "test",
"ordered": true,
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test"
),
got
OpMsg(
{"insert": "test",
"ordered": true,
"$db": "test",
"$readPreference": {"mode": "primary"},
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test")
looks like you simply need to include some of the standard MongoDB keys in your verification code.
Swap yours out with this and give it a try?
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
'documents': [
toVerify
]
}, namespace='test')
)
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
add a comment |
your assertion is quite descriptive
AssertionError:
expected to receive
OpMsg(
{"insert": "test",
"ordered": true,
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test"
),
got
OpMsg(
{"insert": "test",
"ordered": true,
"$db": "test",
"$readPreference": {"mode": "primary"},
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test")
looks like you simply need to include some of the standard MongoDB keys in your verification code.
Swap yours out with this and give it a try?
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
'documents': [
toVerify
]
}, namespace='test')
)
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
add a comment |
your assertion is quite descriptive
AssertionError:
expected to receive
OpMsg(
{"insert": "test",
"ordered": true,
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test"
),
got
OpMsg(
{"insert": "test",
"ordered": true,
"$db": "test",
"$readPreference": {"mode": "primary"},
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test")
looks like you simply need to include some of the standard MongoDB keys in your verification code.
Swap yours out with this and give it a try?
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
'documents': [
toVerify
]
}, namespace='test')
)
your assertion is quite descriptive
AssertionError:
expected to receive
OpMsg(
{"insert": "test",
"ordered": true,
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test"
),
got
OpMsg(
{"insert": "test",
"ordered": true,
"$db": "test",
"$readPreference": {"mode": "primary"},
"documents": [{"_id": "a3dbe8a7e1cc43469b706a8877b0a14a",
"datetime": {"$date": 1542901445120}}]
}, namespace="test")
looks like you simply need to include some of the standard MongoDB keys in your verification code.
Swap yours out with this and give it a try?
request = server.receives(
OpMsg({
'insert': 'test',
'ordered': True,
'$db': 'test', # this key appears somewhere at the driver
'$readPreference': {"mode": "primary"}, # so does this one
'documents': [
toVerify
]
}, namespace='test')
)
answered Nov 27 at 2:05
bauman.space
1,125612
1,125612
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
add a comment |
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
I'd edited the question. The '$db' and '$readPreference' keys don't affect the validation made by mockupdb.
– Jose Truyol
Nov 27 at 13:35
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
can you edit in the new exception once you put those keys in the server.receives? The exception raised looks extremely informative
– bauman.space
Nov 27 at 14:57
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
Edited with more code info
– Jose Truyol
Nov 29 at 15:33
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53436857%2ftest-datetime-object-using-flask-and-mockupdb-mongodb%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
9pzqNj7