Send mail with nodemailer in NodeJS with Angular5 front-end
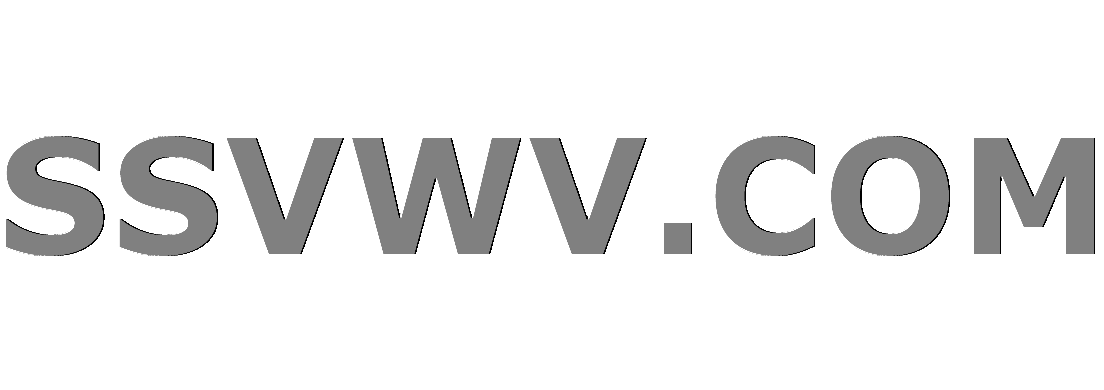
Multi tool use
I want to send an email from my nodejs server whenever i register a user. I'm using Angular5 as front-end. It doesn't return any error, but it doesn't work either. It's like my function is not being called at all. This is what I tried(email not real in this example):
server.js
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
return console.log(error);
res.json({error: "API Error"});
}
else{
console.log("The message is sent: " + response.message);
res.json({response: "sent"})
}
})
});
from Angular
UserService.ts (relevant parts)
constructor(private http: HttpClient) { }
sendMail(user: User){
console.log("sendMail() was called.")
return this.http.post("http://localhost:3000/sendMails", user.email);
}
This is called in my registration component on signup
registration.component.ts
public signUp(){
//marks all fields as touched.
this.fs.markFormGroupTouched(this.registerForm);
if(this.registerForm.valid) {
let user: User = this.registerForm.value;
console.log(user)
this.userService.createUser(user);
this.userService.sendMail(user); //<--- only this is relevant for this case.
}
else {
//shows the error messages, for the not valid input fields.
this.formErrors = this.fs.validateForm(this.registerForm,
this.formErrors, false)
}
}
When i run this code there's no errors. In the inspector/ client-side console window i see the message "sendMail() was called.", which means it's definetly calling my service. However, I recieve no mail nor any error
node.js email angular5 nodemailer
add a comment |
I want to send an email from my nodejs server whenever i register a user. I'm using Angular5 as front-end. It doesn't return any error, but it doesn't work either. It's like my function is not being called at all. This is what I tried(email not real in this example):
server.js
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
return console.log(error);
res.json({error: "API Error"});
}
else{
console.log("The message is sent: " + response.message);
res.json({response: "sent"})
}
})
});
from Angular
UserService.ts (relevant parts)
constructor(private http: HttpClient) { }
sendMail(user: User){
console.log("sendMail() was called.")
return this.http.post("http://localhost:3000/sendMails", user.email);
}
This is called in my registration component on signup
registration.component.ts
public signUp(){
//marks all fields as touched.
this.fs.markFormGroupTouched(this.registerForm);
if(this.registerForm.valid) {
let user: User = this.registerForm.value;
console.log(user)
this.userService.createUser(user);
this.userService.sendMail(user); //<--- only this is relevant for this case.
}
else {
//shows the error messages, for the not valid input fields.
this.formErrors = this.fs.validateForm(this.registerForm,
this.formErrors, false)
}
}
When i run this code there's no errors. In the inspector/ client-side console window i see the message "sendMail() was called.", which means it's definetly calling my service. However, I recieve no mail nor any error
node.js email angular5 nodemailer
add a comment |
I want to send an email from my nodejs server whenever i register a user. I'm using Angular5 as front-end. It doesn't return any error, but it doesn't work either. It's like my function is not being called at all. This is what I tried(email not real in this example):
server.js
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
return console.log(error);
res.json({error: "API Error"});
}
else{
console.log("The message is sent: " + response.message);
res.json({response: "sent"})
}
})
});
from Angular
UserService.ts (relevant parts)
constructor(private http: HttpClient) { }
sendMail(user: User){
console.log("sendMail() was called.")
return this.http.post("http://localhost:3000/sendMails", user.email);
}
This is called in my registration component on signup
registration.component.ts
public signUp(){
//marks all fields as touched.
this.fs.markFormGroupTouched(this.registerForm);
if(this.registerForm.valid) {
let user: User = this.registerForm.value;
console.log(user)
this.userService.createUser(user);
this.userService.sendMail(user); //<--- only this is relevant for this case.
}
else {
//shows the error messages, for the not valid input fields.
this.formErrors = this.fs.validateForm(this.registerForm,
this.formErrors, false)
}
}
When i run this code there's no errors. In the inspector/ client-side console window i see the message "sendMail() was called.", which means it's definetly calling my service. However, I recieve no mail nor any error
node.js email angular5 nodemailer
I want to send an email from my nodejs server whenever i register a user. I'm using Angular5 as front-end. It doesn't return any error, but it doesn't work either. It's like my function is not being called at all. This is what I tried(email not real in this example):
server.js
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
return console.log(error);
res.json({error: "API Error"});
}
else{
console.log("The message is sent: " + response.message);
res.json({response: "sent"})
}
})
});
from Angular
UserService.ts (relevant parts)
constructor(private http: HttpClient) { }
sendMail(user: User){
console.log("sendMail() was called.")
return this.http.post("http://localhost:3000/sendMails", user.email);
}
This is called in my registration component on signup
registration.component.ts
public signUp(){
//marks all fields as touched.
this.fs.markFormGroupTouched(this.registerForm);
if(this.registerForm.valid) {
let user: User = this.registerForm.value;
console.log(user)
this.userService.createUser(user);
this.userService.sendMail(user); //<--- only this is relevant for this case.
}
else {
//shows the error messages, for the not valid input fields.
this.formErrors = this.fs.validateForm(this.registerForm,
this.formErrors, false)
}
}
When i run this code there's no errors. In the inspector/ client-side console window i see the message "sendMail() was called.", which means it's definetly calling my service. However, I recieve no mail nor any error
node.js email angular5 nodemailer
node.js email angular5 nodemailer
asked Nov 22 at 22:25
Thomas
516
516
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The http.post()
inside sendMail()
returns an observable. You must subscribe to this observable, otherwise it will not be executed.
So in registration.component.ts
you need something like:
this.userService.sendMail(user).subscribe(
response => {
console.log(response);
}
);
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
add a comment |
You also need to have a return statements in your sendMail function call. In the error block, the res.send is unreachable code. The edit code below:
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438632%2fsend-mail-with-nodemailer-in-nodejs-with-angular5-front-end%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The http.post()
inside sendMail()
returns an observable. You must subscribe to this observable, otherwise it will not be executed.
So in registration.component.ts
you need something like:
this.userService.sendMail(user).subscribe(
response => {
console.log(response);
}
);
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
add a comment |
The http.post()
inside sendMail()
returns an observable. You must subscribe to this observable, otherwise it will not be executed.
So in registration.component.ts
you need something like:
this.userService.sendMail(user).subscribe(
response => {
console.log(response);
}
);
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
add a comment |
The http.post()
inside sendMail()
returns an observable. You must subscribe to this observable, otherwise it will not be executed.
So in registration.component.ts
you need something like:
this.userService.sendMail(user).subscribe(
response => {
console.log(response);
}
);
The http.post()
inside sendMail()
returns an observable. You must subscribe to this observable, otherwise it will not be executed.
So in registration.component.ts
you need something like:
this.userService.sendMail(user).subscribe(
response => {
console.log(response);
}
);
answered Nov 22 at 23:06


Boji
807
807
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
add a comment |
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
Thank you! This worked and solved the issue
– Thomas
Nov 22 at 23:27
add a comment |
You also need to have a return statements in your sendMail function call. In the error block, the res.send is unreachable code. The edit code below:
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
add a comment |
You also need to have a return statements in your sendMail function call. In the error block, the res.send is unreachable code. The edit code below:
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
add a comment |
You also need to have a return statements in your sendMail function call. In the error block, the res.send is unreachable code. The edit code below:
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
You also need to have a return statements in your sendMail function call. In the error block, the res.send is unreachable code. The edit code below:
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
app.post('/sendMails', function(req,res) {
console.log("WENT INTO SEND MAILS!!!")
let transporter = nodemailer.createTransport({
service: 'gmail',
secure: false,
port: 25,
auth: {
user: 'someemail@gmail.com',
pass: process.env.MAIL_PASSWORD
},
tls: {
rejectUnauthorized: false
},
});
let HelperOptions = {
from: '"Babababa" <someemail@gmail.com>',
to: 'someemail@gmail.com',
subject: 'Hello world',
text: 'hey dude'
};
transporter.sendMail(HelperOptions, (error, response) => {
if(error) {
console.log(error);
return res.json({error: "API Error"}); // return should be here instead of at console.log
}
else{
console.log("The message is sent: " + response.message);
return res.json({response: "sent"})
}
})
});
answered Nov 22 at 23:25
shmit
44239
44239
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438632%2fsend-mail-with-nodemailer-in-nodejs-with-angular5-front-end%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
I hiirE,7GDMz4x