distribute nodes around the tree root with d3-hierarchy with animation
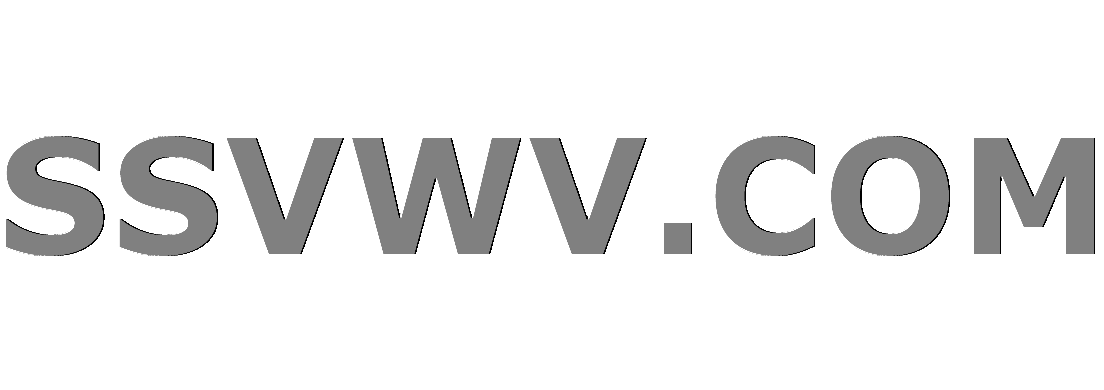
Multi tool use
I have the attached a code sandbox showing my solution.
I feel my solution is not right as I have done all my calculations in the NodesFanout
component.
import * as React from "react";
import NodeGroup from "react-move/NodeGroup";
import { StructureItem } from "../../types";
import { Node } from "../Node";
import { HierarchyPointNode } from "d3-hierarchy";
import { HierarchyLabelProps } from "../HierarchyLabel";
import { findIndex } from "lodash";
import { NodeHeight } from "../Tree";
import { findCollapsedParent } from "../../util/node";
const { Group } = require("@vx/group");
export interface NodesProps {
nodes: HierarchyPointNode<StructureItem>;
clickHandler: any;
shapeLength: number;
collapse: boolean;
Label: React.ComponentType<HierarchyLabelProps>;
LabelCollapsed: React.ComponentType<HierarchyLabelProps>;
}
const positionNodes = (
node: HierarchyPointNode<StructureItem>,
nodes: HierarchyPointNode<StructureItem>
) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else if (node.data && node.data.isRight) {
const index = findIndex(nodes, node);
const lastLeft = nodes[index - 1];
top = lastLeft.x;
left = NodeHeight;
} else {
top = node.x;
left = node.y;
}
return {
top: [top],
left: [left],
opacity: [1]
};
};
export const NodesFanout: React.SFC<NodesProps> = ({
Label,
LabelCollapsed,
nodes,
shapeLength,
clickHandler,
collapse
}) => {
return (
<NodeGroup
data={nodes}
keyAccessor={(d: HierarchyPointNode<StructureItem>) => d.data.id}
start={(node: HierarchyPointNode<StructureItem>) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else {
left = node.parent.y / 2;
top = node.parent.x;
}
return {
top,
left,
opacity: 0
};
}}
enter={node => positionNodes(node, nodes)}
update={node => positionNodes(node, nodes)}
leave={node => {
let left: number;
let top: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
left = collapsedParent.y / 2;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
>
{nodes => (
<Group>
{nodes.map(({ key, data: node, state }, index) => {
return (
<Group
top={state.top}
left={state.left}
key={key}
opacity={state.opacity}
className={`node__${index}`}
>
<Node
Label={Label}
LabelCollapsed={LabelCollapsed}
node={node}
shapeLength={shapeLength}
clickHandler={(e: any) => {
clickHandler({ e, node });
}}
key={key}
collapse={collapse}
/>
</Group>
);
})}
</Group>
)}
</NodeGroup>
);
};
The animation is also not fanning out from the center correctly.
I am trying to achieve something like this where all the nodes spread out from the center.
Is there a better way of doing this?
reactjs d3.js
add a comment |
I have the attached a code sandbox showing my solution.
I feel my solution is not right as I have done all my calculations in the NodesFanout
component.
import * as React from "react";
import NodeGroup from "react-move/NodeGroup";
import { StructureItem } from "../../types";
import { Node } from "../Node";
import { HierarchyPointNode } from "d3-hierarchy";
import { HierarchyLabelProps } from "../HierarchyLabel";
import { findIndex } from "lodash";
import { NodeHeight } from "../Tree";
import { findCollapsedParent } from "../../util/node";
const { Group } = require("@vx/group");
export interface NodesProps {
nodes: HierarchyPointNode<StructureItem>;
clickHandler: any;
shapeLength: number;
collapse: boolean;
Label: React.ComponentType<HierarchyLabelProps>;
LabelCollapsed: React.ComponentType<HierarchyLabelProps>;
}
const positionNodes = (
node: HierarchyPointNode<StructureItem>,
nodes: HierarchyPointNode<StructureItem>
) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else if (node.data && node.data.isRight) {
const index = findIndex(nodes, node);
const lastLeft = nodes[index - 1];
top = lastLeft.x;
left = NodeHeight;
} else {
top = node.x;
left = node.y;
}
return {
top: [top],
left: [left],
opacity: [1]
};
};
export const NodesFanout: React.SFC<NodesProps> = ({
Label,
LabelCollapsed,
nodes,
shapeLength,
clickHandler,
collapse
}) => {
return (
<NodeGroup
data={nodes}
keyAccessor={(d: HierarchyPointNode<StructureItem>) => d.data.id}
start={(node: HierarchyPointNode<StructureItem>) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else {
left = node.parent.y / 2;
top = node.parent.x;
}
return {
top,
left,
opacity: 0
};
}}
enter={node => positionNodes(node, nodes)}
update={node => positionNodes(node, nodes)}
leave={node => {
let left: number;
let top: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
left = collapsedParent.y / 2;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
>
{nodes => (
<Group>
{nodes.map(({ key, data: node, state }, index) => {
return (
<Group
top={state.top}
left={state.left}
key={key}
opacity={state.opacity}
className={`node__${index}`}
>
<Node
Label={Label}
LabelCollapsed={LabelCollapsed}
node={node}
shapeLength={shapeLength}
clickHandler={(e: any) => {
clickHandler({ e, node });
}}
key={key}
collapse={collapse}
/>
</Group>
);
})}
</Group>
)}
</NodeGroup>
);
};
The animation is also not fanning out from the center correctly.
I am trying to achieve something like this where all the nodes spread out from the center.
Is there a better way of doing this?
reactjs d3.js
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
@Root I have updated the question.
– dagda1
Nov 26 at 19:55
add a comment |
I have the attached a code sandbox showing my solution.
I feel my solution is not right as I have done all my calculations in the NodesFanout
component.
import * as React from "react";
import NodeGroup from "react-move/NodeGroup";
import { StructureItem } from "../../types";
import { Node } from "../Node";
import { HierarchyPointNode } from "d3-hierarchy";
import { HierarchyLabelProps } from "../HierarchyLabel";
import { findIndex } from "lodash";
import { NodeHeight } from "../Tree";
import { findCollapsedParent } from "../../util/node";
const { Group } = require("@vx/group");
export interface NodesProps {
nodes: HierarchyPointNode<StructureItem>;
clickHandler: any;
shapeLength: number;
collapse: boolean;
Label: React.ComponentType<HierarchyLabelProps>;
LabelCollapsed: React.ComponentType<HierarchyLabelProps>;
}
const positionNodes = (
node: HierarchyPointNode<StructureItem>,
nodes: HierarchyPointNode<StructureItem>
) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else if (node.data && node.data.isRight) {
const index = findIndex(nodes, node);
const lastLeft = nodes[index - 1];
top = lastLeft.x;
left = NodeHeight;
} else {
top = node.x;
left = node.y;
}
return {
top: [top],
left: [left],
opacity: [1]
};
};
export const NodesFanout: React.SFC<NodesProps> = ({
Label,
LabelCollapsed,
nodes,
shapeLength,
clickHandler,
collapse
}) => {
return (
<NodeGroup
data={nodes}
keyAccessor={(d: HierarchyPointNode<StructureItem>) => d.data.id}
start={(node: HierarchyPointNode<StructureItem>) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else {
left = node.parent.y / 2;
top = node.parent.x;
}
return {
top,
left,
opacity: 0
};
}}
enter={node => positionNodes(node, nodes)}
update={node => positionNodes(node, nodes)}
leave={node => {
let left: number;
let top: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
left = collapsedParent.y / 2;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
>
{nodes => (
<Group>
{nodes.map(({ key, data: node, state }, index) => {
return (
<Group
top={state.top}
left={state.left}
key={key}
opacity={state.opacity}
className={`node__${index}`}
>
<Node
Label={Label}
LabelCollapsed={LabelCollapsed}
node={node}
shapeLength={shapeLength}
clickHandler={(e: any) => {
clickHandler({ e, node });
}}
key={key}
collapse={collapse}
/>
</Group>
);
})}
</Group>
)}
</NodeGroup>
);
};
The animation is also not fanning out from the center correctly.
I am trying to achieve something like this where all the nodes spread out from the center.
Is there a better way of doing this?
reactjs d3.js
I have the attached a code sandbox showing my solution.
I feel my solution is not right as I have done all my calculations in the NodesFanout
component.
import * as React from "react";
import NodeGroup from "react-move/NodeGroup";
import { StructureItem } from "../../types";
import { Node } from "../Node";
import { HierarchyPointNode } from "d3-hierarchy";
import { HierarchyLabelProps } from "../HierarchyLabel";
import { findIndex } from "lodash";
import { NodeHeight } from "../Tree";
import { findCollapsedParent } from "../../util/node";
const { Group } = require("@vx/group");
export interface NodesProps {
nodes: HierarchyPointNode<StructureItem>;
clickHandler: any;
shapeLength: number;
collapse: boolean;
Label: React.ComponentType<HierarchyLabelProps>;
LabelCollapsed: React.ComponentType<HierarchyLabelProps>;
}
const positionNodes = (
node: HierarchyPointNode<StructureItem>,
nodes: HierarchyPointNode<StructureItem>
) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else if (node.data && node.data.isRight) {
const index = findIndex(nodes, node);
const lastLeft = nodes[index - 1];
top = lastLeft.x;
left = NodeHeight;
} else {
top = node.x;
left = node.y;
}
return {
top: [top],
left: [left],
opacity: [1]
};
};
export const NodesFanout: React.SFC<NodesProps> = ({
Label,
LabelCollapsed,
nodes,
shapeLength,
clickHandler,
collapse
}) => {
return (
<NodeGroup
data={nodes}
keyAccessor={(d: HierarchyPointNode<StructureItem>) => d.data.id}
start={(node: HierarchyPointNode<StructureItem>) => {
let left: number;
let top: number;
if (!node.parent) {
left = node.y / 2 - NodeHeight;
top = node.x - NodeHeight;
} else {
left = node.parent.y / 2;
top = node.parent.x;
}
return {
top,
left,
opacity: 0
};
}}
enter={node => positionNodes(node, nodes)}
update={node => positionNodes(node, nodes)}
leave={node => {
let left: number;
let top: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
left = collapsedParent.y / 2;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
>
{nodes => (
<Group>
{nodes.map(({ key, data: node, state }, index) => {
return (
<Group
top={state.top}
left={state.left}
key={key}
opacity={state.opacity}
className={`node__${index}`}
>
<Node
Label={Label}
LabelCollapsed={LabelCollapsed}
node={node}
shapeLength={shapeLength}
clickHandler={(e: any) => {
clickHandler({ e, node });
}}
key={key}
collapse={collapse}
/>
</Group>
);
})}
</Group>
)}
</NodeGroup>
);
};
The animation is also not fanning out from the center correctly.
I am trying to achieve something like this where all the nodes spread out from the center.
Is there a better way of doing this?
reactjs d3.js
reactjs d3.js
edited Nov 26 at 19:55
asked Nov 22 at 22:21
dagda1
9,65031129259
9,65031129259
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
@Root I have updated the question.
– dagda1
Nov 26 at 19:55
add a comment |
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
@Root I have updated the question.
– dagda1
Nov 26 at 19:55
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
@Root I have updated the question.
– dagda1
Nov 26 at 19:55
@Root I have updated the question.
– dagda1
Nov 26 at 19:55
add a comment |
1 Answer
1
active
oldest
votes
I believe this achieves the goal: https://codesandbox.io/s/w4n1v3xvw
I don't think the code is problematic, its just the example you based on was one-sided, so the leave
animation needed to be updated based on the side of each node.
This is the code changed with comments before changes:
leave={node => {
let left: number;
let top: number;
let nodeIndex: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
// Get this nodes index from its' position in the parent
nodeIndex = findIndex(node.parent.children, node);
// Even indices go one side and odd go to the other
// So we add a modifier which changes sign based on NodeHeight
let modifier: number = NodeHeight;
if (nodeIndex % 2 == 1) {
modifier *= -1;
}
// We add the modifier in the left calculation
left = collapsedParent.y / 2 + modifier;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
withopacity[0]
there is no difference visible. withopacity[1]
it is very hard to see an improvement.
– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438604%2fdistribute-nodes-around-the-tree-root-with-d3-hierarchy-with-animation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I believe this achieves the goal: https://codesandbox.io/s/w4n1v3xvw
I don't think the code is problematic, its just the example you based on was one-sided, so the leave
animation needed to be updated based on the side of each node.
This is the code changed with comments before changes:
leave={node => {
let left: number;
let top: number;
let nodeIndex: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
// Get this nodes index from its' position in the parent
nodeIndex = findIndex(node.parent.children, node);
// Even indices go one side and odd go to the other
// So we add a modifier which changes sign based on NodeHeight
let modifier: number = NodeHeight;
if (nodeIndex % 2 == 1) {
modifier *= -1;
}
// We add the modifier in the left calculation
left = collapsedParent.y / 2 + modifier;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
withopacity[0]
there is no difference visible. withopacity[1]
it is very hard to see an improvement.
– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
add a comment |
I believe this achieves the goal: https://codesandbox.io/s/w4n1v3xvw
I don't think the code is problematic, its just the example you based on was one-sided, so the leave
animation needed to be updated based on the side of each node.
This is the code changed with comments before changes:
leave={node => {
let left: number;
let top: number;
let nodeIndex: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
// Get this nodes index from its' position in the parent
nodeIndex = findIndex(node.parent.children, node);
// Even indices go one side and odd go to the other
// So we add a modifier which changes sign based on NodeHeight
let modifier: number = NodeHeight;
if (nodeIndex % 2 == 1) {
modifier *= -1;
}
// We add the modifier in the left calculation
left = collapsedParent.y / 2 + modifier;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
withopacity[0]
there is no difference visible. withopacity[1]
it is very hard to see an improvement.
– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
add a comment |
I believe this achieves the goal: https://codesandbox.io/s/w4n1v3xvw
I don't think the code is problematic, its just the example you based on was one-sided, so the leave
animation needed to be updated based on the side of each node.
This is the code changed with comments before changes:
leave={node => {
let left: number;
let top: number;
let nodeIndex: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
// Get this nodes index from its' position in the parent
nodeIndex = findIndex(node.parent.children, node);
// Even indices go one side and odd go to the other
// So we add a modifier which changes sign based on NodeHeight
let modifier: number = NodeHeight;
if (nodeIndex % 2 == 1) {
modifier *= -1;
}
// We add the modifier in the left calculation
left = collapsedParent.y / 2 + modifier;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
I believe this achieves the goal: https://codesandbox.io/s/w4n1v3xvw
I don't think the code is problematic, its just the example you based on was one-sided, so the leave
animation needed to be updated based on the side of each node.
This is the code changed with comments before changes:
leave={node => {
let left: number;
let top: number;
let nodeIndex: number;
const collapsedParent = findCollapsedParent(
node.parent
) as HierarchyPointNode<StructureItem>;
if (!collapsedParent.parent) {
// Get this nodes index from its' position in the parent
nodeIndex = findIndex(node.parent.children, node);
// Even indices go one side and odd go to the other
// So we add a modifier which changes sign based on NodeHeight
let modifier: number = NodeHeight;
if (nodeIndex % 2 == 1) {
modifier *= -1;
}
// We add the modifier in the left calculation
left = collapsedParent.y / 2 + modifier;
top = collapsedParent.x - NodeHeight;
} else {
left = collapsedParent.parent.y / 2;
top = collapsedParent.parent.x - NodeHeight;
}
return {
top: [top],
left: [left],
opacity: [0]
};
}}
answered Nov 28 at 17:50
Shushan
1,1171714
1,1171714
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
withopacity[0]
there is no difference visible. withopacity[1]
it is very hard to see an improvement.
– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
add a comment |
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
withopacity[0]
there is no difference visible. withopacity[1]
it is very hard to see an improvement.
– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@dagda1 I don't see anything different in the animation from your sandbox and this answer sandbox, I'm using Chrome
– rioV8
Nov 30 at 18:44
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
@rioV8 You can see that before the nodes fade away on the collapse animation, they aren't exactly on top of the center node but somewhat around it. This is really apparent when viewing the minimized view, but also apparent in the full window if you look carefully. You can also change the opacity: [0] to opacity: [1] to see the problem in the original animation more carefully.
– Shushan
Dec 1 at 9:52
with
opacity[0]
there is no difference visible. with opacity[1]
it is very hard to see an improvement.– rioV8
Dec 1 at 12:40
with
opacity[0]
there is no difference visible. with opacity[1]
it is very hard to see an improvement.– rioV8
Dec 1 at 12:40
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
Here is a screenshot from a few frames before the nodes disappear, on the left you see the initial situation and on the right the fixed one. Its quite obvious when you actually look for it that the nodes don't converge at the exact center. (And as I said the distortion depends on window size)
– Shushan
Dec 1 at 13:04
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53438604%2fdistribute-nodes-around-the-tree-root-with-d3-hierarchy-with-animation%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Z2ILO p8Cb4F9WC,h H5 44KpyFL q lZ6DP xvQob1yNLx9dxku 16ZxWbwz4HKcZfcRrEzk6aMGJo1,9QUBuctXi,Bi bke210jOMJGkn
Please share what animation do you exactly want.
– Root
Nov 26 at 5:56
@Root I have updated the question.
– dagda1
Nov 26 at 19:55