Laravel: How to get records from a pivot table?
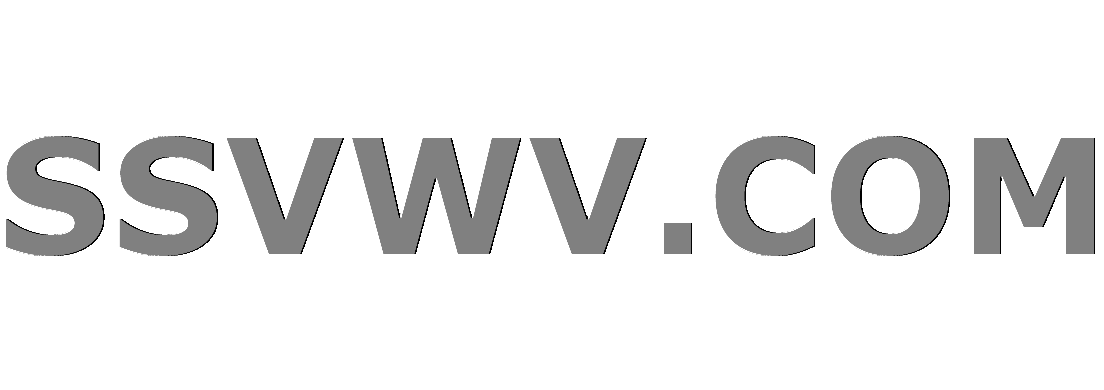
Multi tool use
I'm using Lumen 5.1, I have many to many relation between tasks and users
Task model
public function user()
{
return $this->belongsToMany('AppModelsAuthUser', 'task_user');
}
public function domain()
{
return $this->hasOne('AppModelsDomain', 'domain_id');
}
User model
public function tasks()
{
return $this->belongsToMany(Task::class, 'task_user');
}
UserTask model
class UserTask {}
I want to search the get the user of the task, my code is
$tasks = Task::Where(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->where("is_done", 0)
->orwherehas('tasks.user.id', Auth::id)
->orderBy('due_date', 'DESC');
})
->orWhere(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->Where("is_done", 1)
->Where("closed_dated", Carbon::today())
->orwherehas('tasks.user.id', Auth::id)
->orderBy('closed_date', 'ASC');
})
->get();
My question is whereHas
correct? Is tasks.user.id
correct? Can I get to the user's id that way? I did it that way because of this question
The tech lead tells me that my code is wrong, he would you use where
, he said that whereHas
when you want to run a closure.
Migrations:
Tasks
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->string('title')->nullable();
$table->dateTime('submit_date');
$table->dateTime('closed_date');
$table->dateTime('due_date');
$table->tinyInteger('is_done')->default(0);
$table->integer('domain_id')->unsigned()->nullable();
$table->foreign('domain_id')->references('id')
->on(self::getTableName('domains'))->onDelete('cascade');
$table->bigInteger('created_by')->unsigned()->nullable();
$table->foreign('created_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->bigInteger('closed_by')->unsigned()->nullable();
$table->foreign('closed_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->timestamps();
});
}
public function down()
{
Schema::drop($this->getTable());
}
task_user
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->integer('task_id')->unsigned()->nullable();
$table->foreign('task_id')->references('id')
->on(self::getTableName('tasks'))
->onDelete('cascade');
$table->bigInteger('user_id')->unsigned()->nullable();
$table->foreign('user_id')->references('id')
->on(self::getTableName('auth_users', false))
->onDelete('cascade');
});
}
public function down()
{
Schema::drop($this->getTable());
}
php laravel eloquent lumen
add a comment |
I'm using Lumen 5.1, I have many to many relation between tasks and users
Task model
public function user()
{
return $this->belongsToMany('AppModelsAuthUser', 'task_user');
}
public function domain()
{
return $this->hasOne('AppModelsDomain', 'domain_id');
}
User model
public function tasks()
{
return $this->belongsToMany(Task::class, 'task_user');
}
UserTask model
class UserTask {}
I want to search the get the user of the task, my code is
$tasks = Task::Where(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->where("is_done", 0)
->orwherehas('tasks.user.id', Auth::id)
->orderBy('due_date', 'DESC');
})
->orWhere(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->Where("is_done", 1)
->Where("closed_dated", Carbon::today())
->orwherehas('tasks.user.id', Auth::id)
->orderBy('closed_date', 'ASC');
})
->get();
My question is whereHas
correct? Is tasks.user.id
correct? Can I get to the user's id that way? I did it that way because of this question
The tech lead tells me that my code is wrong, he would you use where
, he said that whereHas
when you want to run a closure.
Migrations:
Tasks
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->string('title')->nullable();
$table->dateTime('submit_date');
$table->dateTime('closed_date');
$table->dateTime('due_date');
$table->tinyInteger('is_done')->default(0);
$table->integer('domain_id')->unsigned()->nullable();
$table->foreign('domain_id')->references('id')
->on(self::getTableName('domains'))->onDelete('cascade');
$table->bigInteger('created_by')->unsigned()->nullable();
$table->foreign('created_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->bigInteger('closed_by')->unsigned()->nullable();
$table->foreign('closed_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->timestamps();
});
}
public function down()
{
Schema::drop($this->getTable());
}
task_user
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->integer('task_id')->unsigned()->nullable();
$table->foreign('task_id')->references('id')
->on(self::getTableName('tasks'))
->onDelete('cascade');
$table->bigInteger('user_id')->unsigned()->nullable();
$table->foreign('user_id')->references('id')
->on(self::getTableName('auth_users', false))
->onDelete('cascade');
});
}
public function down()
{
Schema::drop($this->getTable());
}
php laravel eloquent lumen
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
Please could you add your migrations (or just the table structures) fortasks
andtask_user
?
– Ross Wilson
Nov 25 at 9:43
@RossWilson Just added them
– Lynob
Nov 25 at 10:08
add a comment |
I'm using Lumen 5.1, I have many to many relation between tasks and users
Task model
public function user()
{
return $this->belongsToMany('AppModelsAuthUser', 'task_user');
}
public function domain()
{
return $this->hasOne('AppModelsDomain', 'domain_id');
}
User model
public function tasks()
{
return $this->belongsToMany(Task::class, 'task_user');
}
UserTask model
class UserTask {}
I want to search the get the user of the task, my code is
$tasks = Task::Where(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->where("is_done", 0)
->orwherehas('tasks.user.id', Auth::id)
->orderBy('due_date', 'DESC');
})
->orWhere(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->Where("is_done", 1)
->Where("closed_dated", Carbon::today())
->orwherehas('tasks.user.id', Auth::id)
->orderBy('closed_date', 'ASC');
})
->get();
My question is whereHas
correct? Is tasks.user.id
correct? Can I get to the user's id that way? I did it that way because of this question
The tech lead tells me that my code is wrong, he would you use where
, he said that whereHas
when you want to run a closure.
Migrations:
Tasks
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->string('title')->nullable();
$table->dateTime('submit_date');
$table->dateTime('closed_date');
$table->dateTime('due_date');
$table->tinyInteger('is_done')->default(0);
$table->integer('domain_id')->unsigned()->nullable();
$table->foreign('domain_id')->references('id')
->on(self::getTableName('domains'))->onDelete('cascade');
$table->bigInteger('created_by')->unsigned()->nullable();
$table->foreign('created_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->bigInteger('closed_by')->unsigned()->nullable();
$table->foreign('closed_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->timestamps();
});
}
public function down()
{
Schema::drop($this->getTable());
}
task_user
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->integer('task_id')->unsigned()->nullable();
$table->foreign('task_id')->references('id')
->on(self::getTableName('tasks'))
->onDelete('cascade');
$table->bigInteger('user_id')->unsigned()->nullable();
$table->foreign('user_id')->references('id')
->on(self::getTableName('auth_users', false))
->onDelete('cascade');
});
}
public function down()
{
Schema::drop($this->getTable());
}
php laravel eloquent lumen
I'm using Lumen 5.1, I have many to many relation between tasks and users
Task model
public function user()
{
return $this->belongsToMany('AppModelsAuthUser', 'task_user');
}
public function domain()
{
return $this->hasOne('AppModelsDomain', 'domain_id');
}
User model
public function tasks()
{
return $this->belongsToMany(Task::class, 'task_user');
}
UserTask model
class UserTask {}
I want to search the get the user of the task, my code is
$tasks = Task::Where(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->where("is_done", 0)
->orwherehas('tasks.user.id', Auth::id)
->orderBy('due_date', 'DESC');
})
->orWhere(function ($query) use ($domainId) {
$query->where("domain_id", $domainId)
->Where("is_done", 1)
->Where("closed_dated", Carbon::today())
->orwherehas('tasks.user.id', Auth::id)
->orderBy('closed_date', 'ASC');
})
->get();
My question is whereHas
correct? Is tasks.user.id
correct? Can I get to the user's id that way? I did it that way because of this question
The tech lead tells me that my code is wrong, he would you use where
, he said that whereHas
when you want to run a closure.
Migrations:
Tasks
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->string('title')->nullable();
$table->dateTime('submit_date');
$table->dateTime('closed_date');
$table->dateTime('due_date');
$table->tinyInteger('is_done')->default(0);
$table->integer('domain_id')->unsigned()->nullable();
$table->foreign('domain_id')->references('id')
->on(self::getTableName('domains'))->onDelete('cascade');
$table->bigInteger('created_by')->unsigned()->nullable();
$table->foreign('created_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->bigInteger('closed_by')->unsigned()->nullable();
$table->foreign('closed_by')->references('id')
->on(self::getTableName('auth_users', false))->onDelete('cascade');
$table->timestamps();
});
}
public function down()
{
Schema::drop($this->getTable());
}
task_user
public function up()
{
Schema::create($this->getTable(), function (Blueprint $table) {
$table->increments('id');
$table->integer('task_id')->unsigned()->nullable();
$table->foreign('task_id')->references('id')
->on(self::getTableName('tasks'))
->onDelete('cascade');
$table->bigInteger('user_id')->unsigned()->nullable();
$table->foreign('user_id')->references('id')
->on(self::getTableName('auth_users', false))
->onDelete('cascade');
});
}
public function down()
{
Schema::drop($this->getTable());
}
php laravel eloquent lumen
php laravel eloquent lumen
edited Nov 25 at 10:07
asked Nov 23 at 10:02


Lynob
1,53573260
1,53573260
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
Please could you add your migrations (or just the table structures) fortasks
andtask_user
?
– Ross Wilson
Nov 25 at 9:43
@RossWilson Just added them
– Lynob
Nov 25 at 10:08
add a comment |
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
Please could you add your migrations (or just the table structures) fortasks
andtask_user
?
– Ross Wilson
Nov 25 at 9:43
@RossWilson Just added them
– Lynob
Nov 25 at 10:08
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
Please could you add your migrations (or just the table structures) for
tasks
and task_user
?– Ross Wilson
Nov 25 at 9:43
Please could you add your migrations (or just the table structures) for
tasks
and task_user
?– Ross Wilson
Nov 25 at 9:43
@RossWilson Just added them
– Lynob
Nov 25 at 10:08
@RossWilson Just added them
– Lynob
Nov 25 at 10:08
add a comment |
2 Answers
2
active
oldest
votes
No, whereHas
would not be correct for both here. Also, you wouldn't be saying whereHas('tasks...')
on the Task
model.
NB
The 2nd param for whereHas
should be a closure (function) and Auth::id
should be Auth::id()
. You can also use the auth()
helper function instead of the Auth
facade if you want to.
The following should give you what you want:
$tasks = Task::where("domain_id", $domainId)
->where(function ($query) use ($domainId) {
$query
->where("is_done", 0)
//whereHas - 1st arg = name of the relationship on the model, 2nd arg = closure
->whereHas('user', function ($query) {
$query->where('id', auth()->id());
});
})
->orWhere(function ($query) use ($domainId) {
$query
//If "is_done" = 1 only when it's been closed then you won't need to check "is_done"
->where("is_done", 1)
->where('closed_by', auth()->id())
->whereDate("closed_dated", '>=', Carbon::today()->startOfDay());
})
->orderBy('due_date', 'DESC')
->orderBy('closed_date', 'ASC')
->get();
add a comment |
The following blog post covers a one to many task assignment example.
https://medium.com/@brice_hartmann/building-a-user-based-task-list-application-in-laravel-eff4a07e2688
Getting tasks for current user, Relation on belongsToMany to the User model would be:
Auth::user()->tasks()->where('is_done',0) .... ->get();
Getting tasks with users:
Tasks::with(['user'])->where('is_done',1) ... ->get();
Authed user conclusion ... I am not 100% sure this is correct:
Auth::user()->tasks()
->where('domain_id', $domainId)
->where(function ($query) {
$query->where('is_done', 1)
->orWhere(function($query) {
$query->where('is_done', 0)
->where('closed_dated', Carbon::today())
});
});
})
->get();
so then, to get the task id from user, it becomestask.user.id
?
– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
|
show 1 more comment
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53444484%2flaravel-how-to-get-records-from-a-pivot-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
No, whereHas
would not be correct for both here. Also, you wouldn't be saying whereHas('tasks...')
on the Task
model.
NB
The 2nd param for whereHas
should be a closure (function) and Auth::id
should be Auth::id()
. You can also use the auth()
helper function instead of the Auth
facade if you want to.
The following should give you what you want:
$tasks = Task::where("domain_id", $domainId)
->where(function ($query) use ($domainId) {
$query
->where("is_done", 0)
//whereHas - 1st arg = name of the relationship on the model, 2nd arg = closure
->whereHas('user', function ($query) {
$query->where('id', auth()->id());
});
})
->orWhere(function ($query) use ($domainId) {
$query
//If "is_done" = 1 only when it's been closed then you won't need to check "is_done"
->where("is_done", 1)
->where('closed_by', auth()->id())
->whereDate("closed_dated", '>=', Carbon::today()->startOfDay());
})
->orderBy('due_date', 'DESC')
->orderBy('closed_date', 'ASC')
->get();
add a comment |
No, whereHas
would not be correct for both here. Also, you wouldn't be saying whereHas('tasks...')
on the Task
model.
NB
The 2nd param for whereHas
should be a closure (function) and Auth::id
should be Auth::id()
. You can also use the auth()
helper function instead of the Auth
facade if you want to.
The following should give you what you want:
$tasks = Task::where("domain_id", $domainId)
->where(function ($query) use ($domainId) {
$query
->where("is_done", 0)
//whereHas - 1st arg = name of the relationship on the model, 2nd arg = closure
->whereHas('user', function ($query) {
$query->where('id', auth()->id());
});
})
->orWhere(function ($query) use ($domainId) {
$query
//If "is_done" = 1 only when it's been closed then you won't need to check "is_done"
->where("is_done", 1)
->where('closed_by', auth()->id())
->whereDate("closed_dated", '>=', Carbon::today()->startOfDay());
})
->orderBy('due_date', 'DESC')
->orderBy('closed_date', 'ASC')
->get();
add a comment |
No, whereHas
would not be correct for both here. Also, you wouldn't be saying whereHas('tasks...')
on the Task
model.
NB
The 2nd param for whereHas
should be a closure (function) and Auth::id
should be Auth::id()
. You can also use the auth()
helper function instead of the Auth
facade if you want to.
The following should give you what you want:
$tasks = Task::where("domain_id", $domainId)
->where(function ($query) use ($domainId) {
$query
->where("is_done", 0)
//whereHas - 1st arg = name of the relationship on the model, 2nd arg = closure
->whereHas('user', function ($query) {
$query->where('id', auth()->id());
});
})
->orWhere(function ($query) use ($domainId) {
$query
//If "is_done" = 1 only when it's been closed then you won't need to check "is_done"
->where("is_done", 1)
->where('closed_by', auth()->id())
->whereDate("closed_dated", '>=', Carbon::today()->startOfDay());
})
->orderBy('due_date', 'DESC')
->orderBy('closed_date', 'ASC')
->get();
No, whereHas
would not be correct for both here. Also, you wouldn't be saying whereHas('tasks...')
on the Task
model.
NB
The 2nd param for whereHas
should be a closure (function) and Auth::id
should be Auth::id()
. You can also use the auth()
helper function instead of the Auth
facade if you want to.
The following should give you what you want:
$tasks = Task::where("domain_id", $domainId)
->where(function ($query) use ($domainId) {
$query
->where("is_done", 0)
//whereHas - 1st arg = name of the relationship on the model, 2nd arg = closure
->whereHas('user', function ($query) {
$query->where('id', auth()->id());
});
})
->orWhere(function ($query) use ($domainId) {
$query
//If "is_done" = 1 only when it's been closed then you won't need to check "is_done"
->where("is_done", 1)
->where('closed_by', auth()->id())
->whereDate("closed_dated", '>=', Carbon::today()->startOfDay());
})
->orderBy('due_date', 'DESC')
->orderBy('closed_date', 'ASC')
->get();
answered Nov 25 at 17:12
Ross Wilson
15.6k22539
15.6k22539
add a comment |
add a comment |
The following blog post covers a one to many task assignment example.
https://medium.com/@brice_hartmann/building-a-user-based-task-list-application-in-laravel-eff4a07e2688
Getting tasks for current user, Relation on belongsToMany to the User model would be:
Auth::user()->tasks()->where('is_done',0) .... ->get();
Getting tasks with users:
Tasks::with(['user'])->where('is_done',1) ... ->get();
Authed user conclusion ... I am not 100% sure this is correct:
Auth::user()->tasks()
->where('domain_id', $domainId)
->where(function ($query) {
$query->where('is_done', 1)
->orWhere(function($query) {
$query->where('is_done', 0)
->where('closed_dated', Carbon::today())
});
});
})
->get();
so then, to get the task id from user, it becomestask.user.id
?
– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
|
show 1 more comment
The following blog post covers a one to many task assignment example.
https://medium.com/@brice_hartmann/building-a-user-based-task-list-application-in-laravel-eff4a07e2688
Getting tasks for current user, Relation on belongsToMany to the User model would be:
Auth::user()->tasks()->where('is_done',0) .... ->get();
Getting tasks with users:
Tasks::with(['user'])->where('is_done',1) ... ->get();
Authed user conclusion ... I am not 100% sure this is correct:
Auth::user()->tasks()
->where('domain_id', $domainId)
->where(function ($query) {
$query->where('is_done', 1)
->orWhere(function($query) {
$query->where('is_done', 0)
->where('closed_dated', Carbon::today())
});
});
})
->get();
so then, to get the task id from user, it becomestask.user.id
?
– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
|
show 1 more comment
The following blog post covers a one to many task assignment example.
https://medium.com/@brice_hartmann/building-a-user-based-task-list-application-in-laravel-eff4a07e2688
Getting tasks for current user, Relation on belongsToMany to the User model would be:
Auth::user()->tasks()->where('is_done',0) .... ->get();
Getting tasks with users:
Tasks::with(['user'])->where('is_done',1) ... ->get();
Authed user conclusion ... I am not 100% sure this is correct:
Auth::user()->tasks()
->where('domain_id', $domainId)
->where(function ($query) {
$query->where('is_done', 1)
->orWhere(function($query) {
$query->where('is_done', 0)
->where('closed_dated', Carbon::today())
});
});
})
->get();
The following blog post covers a one to many task assignment example.
https://medium.com/@brice_hartmann/building-a-user-based-task-list-application-in-laravel-eff4a07e2688
Getting tasks for current user, Relation on belongsToMany to the User model would be:
Auth::user()->tasks()->where('is_done',0) .... ->get();
Getting tasks with users:
Tasks::with(['user'])->where('is_done',1) ... ->get();
Authed user conclusion ... I am not 100% sure this is correct:
Auth::user()->tasks()
->where('domain_id', $domainId)
->where(function ($query) {
$query->where('is_done', 1)
->orWhere(function($query) {
$query->where('is_done', 0)
->where('closed_dated', Carbon::today())
});
});
})
->get();
edited Nov 26 at 8:25
answered Nov 23 at 11:12
Marc Newton
1,8581320
1,8581320
so then, to get the task id from user, it becomestask.user.id
?
– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
|
show 1 more comment
so then, to get the task id from user, it becomestask.user.id
?
– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
so then, to get the task id from user, it becomes
task.user.id
?– Lynob
Nov 23 at 12:34
so then, to get the task id from user, it becomes
task.user.id
?– Lynob
Nov 23 at 12:34
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
I see, I went over what you posted again, I have updated my answer with what I think i can understand you want..
– Marc Newton
Nov 23 at 13:10
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
Your second answer is exactly what I want Sir, the only thing is that it gets all tasks for all users, I want to get all tasks for only the authorized user.
– Lynob
Nov 23 at 13:27
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
So you mean the date closed query? Auth:user()->tasks()->where('is_done',1)->orWhere('closed_dated', Carbon::today()) ? You gust need to mod that to group the OR to include domain_id
– Marc Newton
Nov 23 at 13:29
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
I added a conclusion, I think that is possible but i never had to write such a nested query before..
– Marc Newton
Nov 23 at 13:52
|
show 1 more comment
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53444484%2flaravel-how-to-get-records-from-a-pivot-table%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7HQsh,9RXYm6HNRd5k6DV9bvnMyf,e,nf52fE I4q0F52GW72ibC4i989MIUxI1u,Db52OM
Just to be clear, are you wanting to get the tasks that have been assigned to a user that have either been completed today or not completed at all?
– Ross Wilson
Nov 25 at 8:08
@RossWilson Please refer to this question (stackoverflow.com/questions/53464283/…) A task can be created by one user and assigned to many users, there's a many to many relationship between tasks and users
– Lynob
Nov 25 at 9:29
@RossWilson I want to find all tasks that are either created by or assigned to a user, if they are not completed or if they are closed today. So a user can see all of his open tasks + the tasks that he closed today, if the tasks are open, they should be sorted by the one having the worst due date, meaning by urgency
– Lynob
Nov 25 at 9:33
Please could you add your migrations (or just the table structures) for
tasks
andtask_user
?– Ross Wilson
Nov 25 at 9:43
@RossWilson Just added them
– Lynob
Nov 25 at 10:08