Access private struct within class c++
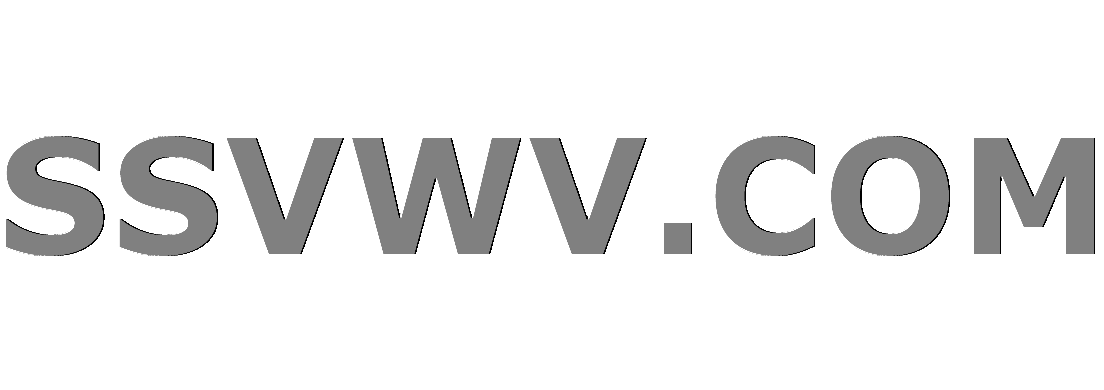
Multi tool use
Trying to implement an adjacency matrix graph and practice OOP. I've been stuck on implementing the inserNode(string ) method.
My troubles are with accessing the private data fields. What am I completely missing?
Some of the errors:
Graph.cpp:30:26: error: unknown type name 'node'
graph[id] = new node;
^
Graph.cpp:35:10: error: use of undeclared identifier 'numnodes'
numnodes++;
Graph.cpp:34:19: error: expected ';' at end of declaration
graph[id]->nodename = name;
Graph.cpp:34:15: error: decomposition declaration '[id]' requires an initializer
graph.h
#include <iostream>
using namespace std;
class Graph {
public:
Graph();
int insertNode(string name);
private:
static const int vertices = 20;
int nodeCount;
struct node {
int nodeid; // node position in graph
string nodename; // username
};
// pointers to the graph nodes
node *graph[vertices];
// adjacency matrix for graph. True if edge is going from node i to j.
bool edges[vertices][vertices];
};
#endif
graph.cpp
#include "Graph.h"
Graph::Graph() {
for (int i = 0; i < vertices; i++) {
graph[i] = 0;
for (int j = 0; j < vertices; j++ )
edges[i][j] = 0;
}
}
/* create node and insert pointer in first available graph position. Returns id value, -1 if unsuccessful. */
int insertNode(string name) {
int id = 0;
while (id < vertices) {
if (graph[id] == NULL) {
graph[id] = new node;
if (!graph[id])
return -1;
graph[id]->nodeid = id;
graph[id]->nodename = name;
numnodes++;
return id;
}
id++;
}
return -1;
}
c++
add a comment |
Trying to implement an adjacency matrix graph and practice OOP. I've been stuck on implementing the inserNode(string ) method.
My troubles are with accessing the private data fields. What am I completely missing?
Some of the errors:
Graph.cpp:30:26: error: unknown type name 'node'
graph[id] = new node;
^
Graph.cpp:35:10: error: use of undeclared identifier 'numnodes'
numnodes++;
Graph.cpp:34:19: error: expected ';' at end of declaration
graph[id]->nodename = name;
Graph.cpp:34:15: error: decomposition declaration '[id]' requires an initializer
graph.h
#include <iostream>
using namespace std;
class Graph {
public:
Graph();
int insertNode(string name);
private:
static const int vertices = 20;
int nodeCount;
struct node {
int nodeid; // node position in graph
string nodename; // username
};
// pointers to the graph nodes
node *graph[vertices];
// adjacency matrix for graph. True if edge is going from node i to j.
bool edges[vertices][vertices];
};
#endif
graph.cpp
#include "Graph.h"
Graph::Graph() {
for (int i = 0; i < vertices; i++) {
graph[i] = 0;
for (int j = 0; j < vertices; j++ )
edges[i][j] = 0;
}
}
/* create node and insert pointer in first available graph position. Returns id value, -1 if unsuccessful. */
int insertNode(string name) {
int id = 0;
while (id < vertices) {
if (graph[id] == NULL) {
graph[id] = new node;
if (!graph[id])
return -1;
graph[id]->nodeid = id;
graph[id]->nodename = name;
numnodes++;
return id;
}
id++;
}
return -1;
}
c++
Don't useusing namespace std;
. Especially not in header files.
– Swordfish
Nov 26 '18 at 3:38
Usenode *graph[vertices]{};
andbool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you canGraph() = default;
your constructor and remove the definition of it from graph.cpp.
– Swordfish
Nov 26 '18 at 3:43
add a comment |
Trying to implement an adjacency matrix graph and practice OOP. I've been stuck on implementing the inserNode(string ) method.
My troubles are with accessing the private data fields. What am I completely missing?
Some of the errors:
Graph.cpp:30:26: error: unknown type name 'node'
graph[id] = new node;
^
Graph.cpp:35:10: error: use of undeclared identifier 'numnodes'
numnodes++;
Graph.cpp:34:19: error: expected ';' at end of declaration
graph[id]->nodename = name;
Graph.cpp:34:15: error: decomposition declaration '[id]' requires an initializer
graph.h
#include <iostream>
using namespace std;
class Graph {
public:
Graph();
int insertNode(string name);
private:
static const int vertices = 20;
int nodeCount;
struct node {
int nodeid; // node position in graph
string nodename; // username
};
// pointers to the graph nodes
node *graph[vertices];
// adjacency matrix for graph. True if edge is going from node i to j.
bool edges[vertices][vertices];
};
#endif
graph.cpp
#include "Graph.h"
Graph::Graph() {
for (int i = 0; i < vertices; i++) {
graph[i] = 0;
for (int j = 0; j < vertices; j++ )
edges[i][j] = 0;
}
}
/* create node and insert pointer in first available graph position. Returns id value, -1 if unsuccessful. */
int insertNode(string name) {
int id = 0;
while (id < vertices) {
if (graph[id] == NULL) {
graph[id] = new node;
if (!graph[id])
return -1;
graph[id]->nodeid = id;
graph[id]->nodename = name;
numnodes++;
return id;
}
id++;
}
return -1;
}
c++
Trying to implement an adjacency matrix graph and practice OOP. I've been stuck on implementing the inserNode(string ) method.
My troubles are with accessing the private data fields. What am I completely missing?
Some of the errors:
Graph.cpp:30:26: error: unknown type name 'node'
graph[id] = new node;
^
Graph.cpp:35:10: error: use of undeclared identifier 'numnodes'
numnodes++;
Graph.cpp:34:19: error: expected ';' at end of declaration
graph[id]->nodename = name;
Graph.cpp:34:15: error: decomposition declaration '[id]' requires an initializer
graph.h
#include <iostream>
using namespace std;
class Graph {
public:
Graph();
int insertNode(string name);
private:
static const int vertices = 20;
int nodeCount;
struct node {
int nodeid; // node position in graph
string nodename; // username
};
// pointers to the graph nodes
node *graph[vertices];
// adjacency matrix for graph. True if edge is going from node i to j.
bool edges[vertices][vertices];
};
#endif
graph.cpp
#include "Graph.h"
Graph::Graph() {
for (int i = 0; i < vertices; i++) {
graph[i] = 0;
for (int j = 0; j < vertices; j++ )
edges[i][j] = 0;
}
}
/* create node and insert pointer in first available graph position. Returns id value, -1 if unsuccessful. */
int insertNode(string name) {
int id = 0;
while (id < vertices) {
if (graph[id] == NULL) {
graph[id] = new node;
if (!graph[id])
return -1;
graph[id]->nodeid = id;
graph[id]->nodename = name;
numnodes++;
return id;
}
id++;
}
return -1;
}
c++
c++
asked Nov 26 '18 at 3:32
addbunny123addbunny123
13
13
Don't useusing namespace std;
. Especially not in header files.
– Swordfish
Nov 26 '18 at 3:38
Usenode *graph[vertices]{};
andbool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you canGraph() = default;
your constructor and remove the definition of it from graph.cpp.
– Swordfish
Nov 26 '18 at 3:43
add a comment |
Don't useusing namespace std;
. Especially not in header files.
– Swordfish
Nov 26 '18 at 3:38
Usenode *graph[vertices]{};
andbool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you canGraph() = default;
your constructor and remove the definition of it from graph.cpp.
– Swordfish
Nov 26 '18 at 3:43
Don't use
using namespace std;
. Especially not in header files.– Swordfish
Nov 26 '18 at 3:38
Don't use
using namespace std;
. Especially not in header files.– Swordfish
Nov 26 '18 at 3:38
Use
node *graph[vertices]{};
and bool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you can Graph() = default;
your constructor and remove the definition of it from graph.cpp.– Swordfish
Nov 26 '18 at 3:43
Use
node *graph[vertices]{};
and bool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you can Graph() = default;
your constructor and remove the definition of it from graph.cpp.– Swordfish
Nov 26 '18 at 3:43
add a comment |
1 Answer
1
active
oldest
votes
The insertNode
you've defined is not the same way you declared in Graph
. You've just made a free function called insertNode
, which isn't a member of Graph
and therefore can't access Graph
. You need to define it like so:
int Graph::insertNode(string name)
{
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474455%2faccess-private-struct-within-class-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The insertNode
you've defined is not the same way you declared in Graph
. You've just made a free function called insertNode
, which isn't a member of Graph
and therefore can't access Graph
. You need to define it like so:
int Graph::insertNode(string name)
{
}
add a comment |
The insertNode
you've defined is not the same way you declared in Graph
. You've just made a free function called insertNode
, which isn't a member of Graph
and therefore can't access Graph
. You need to define it like so:
int Graph::insertNode(string name)
{
}
add a comment |
The insertNode
you've defined is not the same way you declared in Graph
. You've just made a free function called insertNode
, which isn't a member of Graph
and therefore can't access Graph
. You need to define it like so:
int Graph::insertNode(string name)
{
}
The insertNode
you've defined is not the same way you declared in Graph
. You've just made a free function called insertNode
, which isn't a member of Graph
and therefore can't access Graph
. You need to define it like so:
int Graph::insertNode(string name)
{
}
answered Nov 26 '18 at 3:38


TasTas
5,35832542
5,35832542
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53474455%2faccess-private-struct-within-class-c%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
6Bjavr,ln8,Z9FTMrrjs5X Ns9oGOZZqG0S dCgg57z1,qQ51
Don't use
using namespace std;
. Especially not in header files.– Swordfish
Nov 26 '18 at 3:38
Use
node *graph[vertices]{};
andbool edges[vertices][vertices]{};
(mind the curly brackets) instead of the for-loops in the constructor. Then you canGraph() = default;
your constructor and remove the definition of it from graph.cpp.– Swordfish
Nov 26 '18 at 3:43