Firebase Observer Event Type
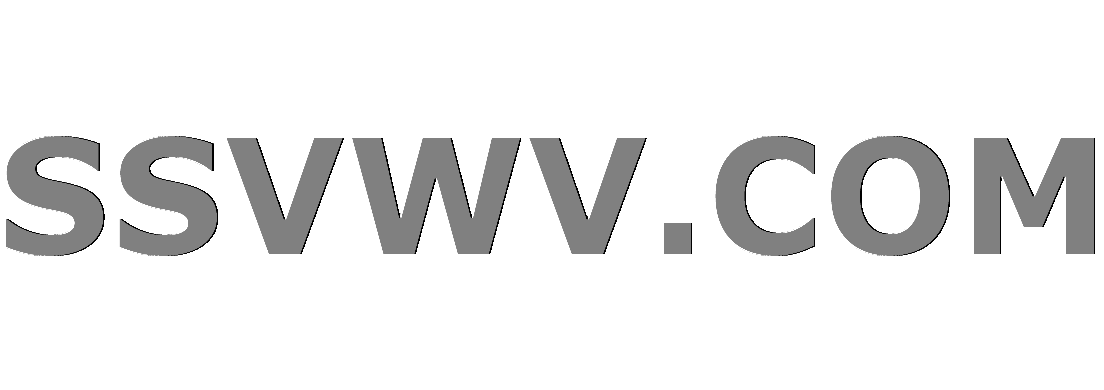
Multi tool use
I’m trying to modify code written a by a previous programmer. He wrote a getPostFromFirebase()
function where it updates the tableview when 1) the app loads up due to it’s presence in viewDidLoad
and 2) when there is a new post add by a user. The problem is that he used a .observe(.childAdded)
event type which means when a post is deleted or modified, the tableView will not update(my end goal to do). When I change .childAdded
to .value
, the current data doesn’t get loaded upon launch. I’ve been banging my head against the wall to figure out a if let
statement to add a .value
event type so the view can refresh after any change(if that’s even possible). I’m familiar with Firebase RT DB hence how I was able to ID the observer issue but I’m no where near close to as good as I’d like to be so any help is appreciated.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.childAdded) { (snapshot: DataSnapshot) in
/*parse method in the PostFetcher class
that returns the post data or an error by way of a tuple.*/
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: snapshot)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Edit:
Thanks to Franks help I was able to implement his suggestion and added a .removeAll()
to remove the current state and have the view append a fresh snapshot. Whether a post is added or deleted, the view now updates as I'd like it to do.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
self.latestPosts.removeAll()
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
self.tableView.reloadData()
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
}
ios swift firebase-realtime-database
add a comment |
I’m trying to modify code written a by a previous programmer. He wrote a getPostFromFirebase()
function where it updates the tableview when 1) the app loads up due to it’s presence in viewDidLoad
and 2) when there is a new post add by a user. The problem is that he used a .observe(.childAdded)
event type which means when a post is deleted or modified, the tableView will not update(my end goal to do). When I change .childAdded
to .value
, the current data doesn’t get loaded upon launch. I’ve been banging my head against the wall to figure out a if let
statement to add a .value
event type so the view can refresh after any change(if that’s even possible). I’m familiar with Firebase RT DB hence how I was able to ID the observer issue but I’m no where near close to as good as I’d like to be so any help is appreciated.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.childAdded) { (snapshot: DataSnapshot) in
/*parse method in the PostFetcher class
that returns the post data or an error by way of a tuple.*/
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: snapshot)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Edit:
Thanks to Franks help I was able to implement his suggestion and added a .removeAll()
to remove the current state and have the view append a fresh snapshot. Whether a post is added or deleted, the view now updates as I'd like it to do.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
self.latestPosts.removeAll()
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
self.tableView.reloadData()
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
}
ios swift firebase-realtime-database
add a comment |
I’m trying to modify code written a by a previous programmer. He wrote a getPostFromFirebase()
function where it updates the tableview when 1) the app loads up due to it’s presence in viewDidLoad
and 2) when there is a new post add by a user. The problem is that he used a .observe(.childAdded)
event type which means when a post is deleted or modified, the tableView will not update(my end goal to do). When I change .childAdded
to .value
, the current data doesn’t get loaded upon launch. I’ve been banging my head against the wall to figure out a if let
statement to add a .value
event type so the view can refresh after any change(if that’s even possible). I’m familiar with Firebase RT DB hence how I was able to ID the observer issue but I’m no where near close to as good as I’d like to be so any help is appreciated.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.childAdded) { (snapshot: DataSnapshot) in
/*parse method in the PostFetcher class
that returns the post data or an error by way of a tuple.*/
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: snapshot)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Edit:
Thanks to Franks help I was able to implement his suggestion and added a .removeAll()
to remove the current state and have the view append a fresh snapshot. Whether a post is added or deleted, the view now updates as I'd like it to do.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
self.latestPosts.removeAll()
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
self.tableView.reloadData()
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
}
ios swift firebase-realtime-database
I’m trying to modify code written a by a previous programmer. He wrote a getPostFromFirebase()
function where it updates the tableview when 1) the app loads up due to it’s presence in viewDidLoad
and 2) when there is a new post add by a user. The problem is that he used a .observe(.childAdded)
event type which means when a post is deleted or modified, the tableView will not update(my end goal to do). When I change .childAdded
to .value
, the current data doesn’t get loaded upon launch. I’ve been banging my head against the wall to figure out a if let
statement to add a .value
event type so the view can refresh after any change(if that’s even possible). I’m familiar with Firebase RT DB hence how I was able to ID the observer issue but I’m no where near close to as good as I’d like to be so any help is appreciated.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.childAdded) { (snapshot: DataSnapshot) in
/*parse method in the PostFetcher class
that returns the post data or an error by way of a tuple.*/
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: snapshot)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Edit:
Thanks to Franks help I was able to implement his suggestion and added a .removeAll()
to remove the current state and have the view append a fresh snapshot. Whether a post is added or deleted, the view now updates as I'd like it to do.
func getPostFromFirebase() {
let mostRecent = dbRef.lastestPostsQuery(count: 10)
mostRecent.keepSynced(true)
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
self.latestPosts.removeAll()
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
self.tableView.reloadData()
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
}
ios swift firebase-realtime-database
ios swift firebase-realtime-database
edited Nov 26 '18 at 0:16
Joe Vargas
asked Nov 24 '18 at 16:16
Joe VargasJoe Vargas
307
307
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
The .child*
events fire on child nodes of the location/query that you observe, while .value
fires on the location/query itself. This means that the value you get is one level higher up in the JSON, and you'll need to loop over the results:
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Alternatively, you can listen to the .childChanged
and .childRemoved
events (in addition to .childAdded
that you already have) and handle them separately. This may be better in cases where you need to update the UI efficiently, since it allows you to handle each individual case (new node, changed, node, removed node) in the most efficient way.
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460035%2ffirebase-observer-event-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
The .child*
events fire on child nodes of the location/query that you observe, while .value
fires on the location/query itself. This means that the value you get is one level higher up in the JSON, and you'll need to loop over the results:
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Alternatively, you can listen to the .childChanged
and .childRemoved
events (in addition to .childAdded
that you already have) and handle them separately. This may be better in cases where you need to update the UI efficiently, since it allows you to handle each individual case (new node, changed, node, removed node) in the most efficient way.
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
add a comment |
The .child*
events fire on child nodes of the location/query that you observe, while .value
fires on the location/query itself. This means that the value you get is one level higher up in the JSON, and you'll need to loop over the results:
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Alternatively, you can listen to the .childChanged
and .childRemoved
events (in addition to .childAdded
that you already have) and handle them separately. This may be better in cases where you need to update the UI efficiently, since it allows you to handle each individual case (new node, changed, node, removed node) in the most efficient way.
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
add a comment |
The .child*
events fire on child nodes of the location/query that you observe, while .value
fires on the location/query itself. This means that the value you get is one level higher up in the JSON, and you'll need to loop over the results:
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Alternatively, you can listen to the .childChanged
and .childRemoved
events (in addition to .childAdded
that you already have) and handle them separately. This may be better in cases where you need to update the UI efficiently, since it allows you to handle each individual case (new node, changed, node, removed node) in the most efficient way.
The .child*
events fire on child nodes of the location/query that you observe, while .value
fires on the location/query itself. This means that the value you get is one level higher up in the JSON, and you'll need to loop over the results:
mostRecent.observe(.value) { (snapshot: DataSnapshot) in
for child in snapshot.children.allObjects as! [DataSnapshot] {
let (post, error) = PostFetcher.parsePostSnapshot(snapshot: child)
if let post = post {
self.latestPosts.append(post)
if let postId = post.postId { print("PostId = (postId)") }
}
if let error = error {
print("(#function) - (error)")
}
}
}
Alternatively, you can listen to the .childChanged
and .childRemoved
events (in addition to .childAdded
that you already have) and handle them separately. This may be better in cases where you need to update the UI efficiently, since it allows you to handle each individual case (new node, changed, node, removed node) in the most efficient way.
answered Nov 24 '18 at 18:18
Frank van PuffelenFrank van Puffelen
230k28376400
230k28376400
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
add a comment |
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
Frank, thank you so much!! I have edited my post implenting your suggestion.
– Joe Vargas
Nov 26 '18 at 0:18
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53460035%2ffirebase-observer-event-type%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
48s6dhQZ0,cIxu1MN7N,LV ASG35F4N6LJ5av,SMW CyH Xnv9y,8,ZOyJ