Add a property to a JavaScript object using a variable as the name?
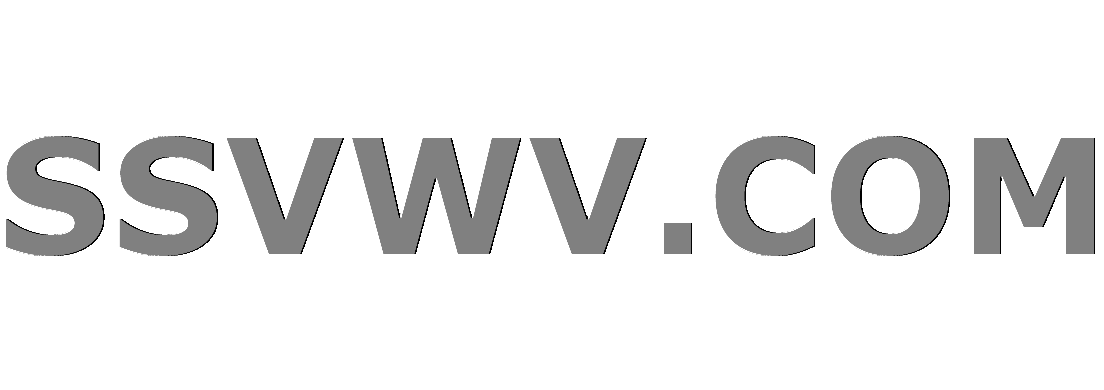
Multi tool use
I'm pulling items out of the DOM with jQuery and want to set a property on an object using the id
of the DOM element.
Example
const obj = {}
jQuery(itemsFromDom).each(function() {
const element = jQuery(this)
const name = element.attr('id')
const value = element.attr('value')
// Here is the problem
obj.name = value
})
If itemsFromDom
includes an element with an id
of "myId", I want obj
to have a property named "myId". The above gives me name
.
How do I name a property of an object using a variable using JavaScript?
javascript jquery object syntax
add a comment |
I'm pulling items out of the DOM with jQuery and want to set a property on an object using the id
of the DOM element.
Example
const obj = {}
jQuery(itemsFromDom).each(function() {
const element = jQuery(this)
const name = element.attr('id')
const value = element.attr('value')
// Here is the problem
obj.name = value
})
If itemsFromDom
includes an element with an id
of "myId", I want obj
to have a property named "myId". The above gives me name
.
How do I name a property of an object using a variable using JavaScript?
javascript jquery object syntax
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40
add a comment |
I'm pulling items out of the DOM with jQuery and want to set a property on an object using the id
of the DOM element.
Example
const obj = {}
jQuery(itemsFromDom).each(function() {
const element = jQuery(this)
const name = element.attr('id')
const value = element.attr('value')
// Here is the problem
obj.name = value
})
If itemsFromDom
includes an element with an id
of "myId", I want obj
to have a property named "myId". The above gives me name
.
How do I name a property of an object using a variable using JavaScript?
javascript jquery object syntax
I'm pulling items out of the DOM with jQuery and want to set a property on an object using the id
of the DOM element.
Example
const obj = {}
jQuery(itemsFromDom).each(function() {
const element = jQuery(this)
const name = element.attr('id')
const value = element.attr('value')
// Here is the problem
obj.name = value
})
If itemsFromDom
includes an element with an id
of "myId", I want obj
to have a property named "myId". The above gives me name
.
How do I name a property of an object using a variable using JavaScript?
javascript jquery object syntax
javascript jquery object syntax
edited Sep 17 at 6:26


KARTHIKEYAN.A
4,86733854
4,86733854
asked Mar 29 '09 at 18:00
Todd R
12.7k72433
12.7k72433
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40
add a comment |
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40
add a comment |
11 Answers
11
active
oldest
votes
You can use this equivalent syntax:
obj[name] = value
@melpomene That's incorrect;obj[name]
is different thanobj.name
in that the former expands thename
variable. See this jsfiddle
– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
add a comment |
With ECMAScript 2015 you can do it directly in object declaration using bracket notation:
var obj = {
[key]: value
}
Where key
can be any sort of expression (e.g. a variable) returning a value:
var obj = {
['hello']: 'World',
[x + 2]: 42,
[someObject.getId()]: someVar
}
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
@wOxxOm lol yeah why would I go through the hassle ofobj[name]=value
when I could just use your suggestion instead
– chiliNUT
Mar 17 '17 at 23:17
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
|
show 2 more comments
You can even make List of objects like this
var feeTypeList = ;
$('#feeTypeTable > tbody > tr').each(function (i, el) {
var feeType = {};
var $ID = $(this).find("input[id^=txtFeeType]").attr('id');
feeType["feeTypeID"] = $('#ddlTerm').val();
feeType["feeTypeName"] = $('#ddlProgram').val();
feeType["feeTypeDescription"] = $('#ddlBatch').val();
feeTypeList.push(feeType);
});
add a comment |
First we need to define key as variable and then we need to assign as key as object., for example
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
add a comment |
With lodash, you can create new object like this _.set:
obj = _.set({}, key, val);
Or you can set to existing object like this:
var existingObj = { a: 1 };
_.set(existingObj, 'a', 5); // existingObj will be: { a: 5 }
You should take care if you want to use dot (".") in your path, because lodash can set hierarchy, for example:
_.set({}, "a.b.c", "d"); // { "a": { "b": { "c": "d" } } }
add a comment |
There are two different notations to access object properties
Dot notation: myObj.prop1
Bracket notation: myObj["prop1"]
Dot notation is fast and easy but you must use the actual property name explicitly. No substitution, variables, etc.
Bracket notation is open ended. It uses a string but you can produce the string using any legal js code. You may specify the string as literal (though in this case dot notation would read easier) or use a variable or calculate in some way.
So, these all set the myObj property named prop1 to the value Hello:
// quick easy-on-the-eye dot notation
myObj.prop1 = "Hello";
// brackets+literal
myObj["prop1"] = "Hello";
// using a variable
var x = "prop1";
myObj[x] = "Hello";
// calculate the accessor string in some weird way
var numList = [0,1,2];
myObj[ "prop" + numList[1] ] = "Hello";
Pitfalls:
myObj.[xxxx] = "Hello"; // wrong: mixed notations, syntax fail
myObj[prop1] = "Hello"; // wrong: this expects a variable called prop1
tl;dnr: If you want to compute or reference the key you must use bracket notation. If you are using the key explicitly, then use dot notation for simple clear code.
Note: there are some other good and correct answers but I personally found them a bit brief coming from a low familiarity with JS on-the-fly quirkiness. This might be useful to some people.
add a comment |
With the advent of ES2015 Object.assign and computed property names the OP's code boils down to:
var obj = Object.assign.apply({}, $(itemsFromDom).map((i, el) => ({[el.id]: el.value})));
add a comment |
objectname.newProperty = value;
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
add a comment |
If you want to add fields to an object dynamically, simplest way to do it is as follows:
var params= [
{key: "k1", value=1},
{key: "k2", value=2},
{key: "k3", value=3}];
for(i=0; i< params.len; i++) {
data[params[i].key] = params[i].value
}
This will create data object which has following fields:
{k1:1, k2:2, k3:3}
add a comment |
Based on the wOxxOm's answer, this is an example of use:
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
add a comment |
const data = [{
name: 'BMW',
value: '25641'
}, {
name: 'Apple',
value: '45876'
},
{
name: 'Benz',
value: '65784'
},
{
name: 'Toyota',
value: '254'
}
]
const obj = {
carsList: [{
name: 'Ford',
value: '47563'
}, {
name: 'Toyota',
value: '254'
}],
pastriesList: ,
fruitsList: [{
name: 'Apple',
value: '45876'
}, {
name: 'Pineapple',
value: '84523'
}]
}
let keys = Object.keys(obj);
result = {};
for(key of keys){
let a = [...data,...obj[key]];
result[key] = a;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f695050%2fadd-a-property-to-a-javascript-object-using-a-variable-as-the-name%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
11 Answers
11
active
oldest
votes
11 Answers
11
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can use this equivalent syntax:
obj[name] = value
@melpomene That's incorrect;obj[name]
is different thanobj.name
in that the former expands thename
variable. See this jsfiddle
– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
add a comment |
You can use this equivalent syntax:
obj[name] = value
@melpomene That's incorrect;obj[name]
is different thanobj.name
in that the former expands thename
variable. See this jsfiddle
– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
add a comment |
You can use this equivalent syntax:
obj[name] = value
You can use this equivalent syntax:
obj[name] = value
edited Oct 17 '17 at 18:04


kube
4,75532035
4,75532035
answered Mar 29 '09 at 18:02
CMS
586k162841809
586k162841809
@melpomene That's incorrect;obj[name]
is different thanobj.name
in that the former expands thename
variable. See this jsfiddle
– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
add a comment |
@melpomene That's incorrect;obj[name]
is different thanobj.name
in that the former expands thename
variable. See this jsfiddle
– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
@melpomene That's incorrect;
obj[name]
is different than obj.name
in that the former expands the name
variable. See this jsfiddle– Lifz
Mar 19 at 22:30
@melpomene That's incorrect;
obj[name]
is different than obj.name
in that the former expands the name
variable. See this jsfiddle– Lifz
Mar 19 at 22:30
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
@Lifz The comment I was replying to was deleted.
– melpomene
Mar 20 at 19:27
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
Ah interesting, that's not how it was when I replied. At any rate I'll leave the fiddle for anyone who's curious.
– Lifz
Mar 21 at 0:19
add a comment |
With ECMAScript 2015 you can do it directly in object declaration using bracket notation:
var obj = {
[key]: value
}
Where key
can be any sort of expression (e.g. a variable) returning a value:
var obj = {
['hello']: 'World',
[x + 2]: 42,
[someObject.getId()]: someVar
}
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
@wOxxOm lol yeah why would I go through the hassle ofobj[name]=value
when I could just use your suggestion instead
– chiliNUT
Mar 17 '17 at 23:17
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
|
show 2 more comments
With ECMAScript 2015 you can do it directly in object declaration using bracket notation:
var obj = {
[key]: value
}
Where key
can be any sort of expression (e.g. a variable) returning a value:
var obj = {
['hello']: 'World',
[x + 2]: 42,
[someObject.getId()]: someVar
}
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
@wOxxOm lol yeah why would I go through the hassle ofobj[name]=value
when I could just use your suggestion instead
– chiliNUT
Mar 17 '17 at 23:17
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
|
show 2 more comments
With ECMAScript 2015 you can do it directly in object declaration using bracket notation:
var obj = {
[key]: value
}
Where key
can be any sort of expression (e.g. a variable) returning a value:
var obj = {
['hello']: 'World',
[x + 2]: 42,
[someObject.getId()]: someVar
}
With ECMAScript 2015 you can do it directly in object declaration using bracket notation:
var obj = {
[key]: value
}
Where key
can be any sort of expression (e.g. a variable) returning a value:
var obj = {
['hello']: 'World',
[x + 2]: 42,
[someObject.getId()]: someVar
}
edited Oct 17 '17 at 18:06
answered Jun 24 '15 at 11:02


kube
4,75532035
4,75532035
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
@wOxxOm lol yeah why would I go through the hassle ofobj[name]=value
when I could just use your suggestion instead
– chiliNUT
Mar 17 '17 at 23:17
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
|
show 2 more comments
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
@wOxxOm lol yeah why would I go through the hassle ofobj[name]=value
when I could just use your suggestion instead
– chiliNUT
Mar 17 '17 at 23:17
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
11
11
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
This question is about modifying existing object, not creating a new one.
– Michał Perłakowski
Dec 26 '15 at 10:16
14
14
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
This particular question might be about modifying but it's referenced by other questions that are about dynamically creating objects and so I ended up here and happily benefited from this answer.
– Oliver Lloyd
Oct 29 '16 at 17:40
1
1
@wOxxOm lol yeah why would I go through the hassle of
obj[name]=value
when I could just use your suggestion instead– chiliNUT
Mar 17 '17 at 23:17
@wOxxOm lol yeah why would I go through the hassle of
obj[name]=value
when I could just use your suggestion instead– chiliNUT
Mar 17 '17 at 23:17
1
1
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
I'm not sure what ECMAScript 6 is, but I appreciate it very much
– Arthur Tarasov
Apr 10 '17 at 12:23
1
1
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
@ArthurTarasov: ECMAScript 6 is more formally called ECMAScript 2015 ("ES2015") aka ECMAScript 6th edition ("ES6"). It's the specification for JavaScript released June 2015. Since then we've had ES2016 and soon we'll have ES2017, they're on a yearly cycle now.
– T.J. Crowder
Apr 18 '17 at 16:06
|
show 2 more comments
You can even make List of objects like this
var feeTypeList = ;
$('#feeTypeTable > tbody > tr').each(function (i, el) {
var feeType = {};
var $ID = $(this).find("input[id^=txtFeeType]").attr('id');
feeType["feeTypeID"] = $('#ddlTerm').val();
feeType["feeTypeName"] = $('#ddlProgram').val();
feeType["feeTypeDescription"] = $('#ddlBatch').val();
feeTypeList.push(feeType);
});
add a comment |
You can even make List of objects like this
var feeTypeList = ;
$('#feeTypeTable > tbody > tr').each(function (i, el) {
var feeType = {};
var $ID = $(this).find("input[id^=txtFeeType]").attr('id');
feeType["feeTypeID"] = $('#ddlTerm').val();
feeType["feeTypeName"] = $('#ddlProgram').val();
feeType["feeTypeDescription"] = $('#ddlBatch').val();
feeTypeList.push(feeType);
});
add a comment |
You can even make List of objects like this
var feeTypeList = ;
$('#feeTypeTable > tbody > tr').each(function (i, el) {
var feeType = {};
var $ID = $(this).find("input[id^=txtFeeType]").attr('id');
feeType["feeTypeID"] = $('#ddlTerm').val();
feeType["feeTypeName"] = $('#ddlProgram').val();
feeType["feeTypeDescription"] = $('#ddlBatch').val();
feeTypeList.push(feeType);
});
You can even make List of objects like this
var feeTypeList = ;
$('#feeTypeTable > tbody > tr').each(function (i, el) {
var feeType = {};
var $ID = $(this).find("input[id^=txtFeeType]").attr('id');
feeType["feeTypeID"] = $('#ddlTerm').val();
feeType["feeTypeName"] = $('#ddlProgram').val();
feeType["feeTypeDescription"] = $('#ddlBatch').val();
feeTypeList.push(feeType);
});
answered Feb 14 '14 at 6:55
dnxit
4,58921926
4,58921926
add a comment |
add a comment |
First we need to define key as variable and then we need to assign as key as object., for example
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
add a comment |
First we need to define key as variable and then we need to assign as key as object., for example
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
add a comment |
First we need to define key as variable and then we need to assign as key as object., for example
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
First we need to define key as variable and then we need to assign as key as object., for example
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
var data = {key:'dynamic_key',value:'dynamic_value'}
var key = data.key;
var obj = { [key]: data.value}
console.log(obj)
answered Oct 25 '17 at 9:04


KARTHIKEYAN.A
4,86733854
4,86733854
add a comment |
add a comment |
With lodash, you can create new object like this _.set:
obj = _.set({}, key, val);
Or you can set to existing object like this:
var existingObj = { a: 1 };
_.set(existingObj, 'a', 5); // existingObj will be: { a: 5 }
You should take care if you want to use dot (".") in your path, because lodash can set hierarchy, for example:
_.set({}, "a.b.c", "d"); // { "a": { "b": { "c": "d" } } }
add a comment |
With lodash, you can create new object like this _.set:
obj = _.set({}, key, val);
Or you can set to existing object like this:
var existingObj = { a: 1 };
_.set(existingObj, 'a', 5); // existingObj will be: { a: 5 }
You should take care if you want to use dot (".") in your path, because lodash can set hierarchy, for example:
_.set({}, "a.b.c", "d"); // { "a": { "b": { "c": "d" } } }
add a comment |
With lodash, you can create new object like this _.set:
obj = _.set({}, key, val);
Or you can set to existing object like this:
var existingObj = { a: 1 };
_.set(existingObj, 'a', 5); // existingObj will be: { a: 5 }
You should take care if you want to use dot (".") in your path, because lodash can set hierarchy, for example:
_.set({}, "a.b.c", "d"); // { "a": { "b": { "c": "d" } } }
With lodash, you can create new object like this _.set:
obj = _.set({}, key, val);
Or you can set to existing object like this:
var existingObj = { a: 1 };
_.set(existingObj, 'a', 5); // existingObj will be: { a: 5 }
You should take care if you want to use dot (".") in your path, because lodash can set hierarchy, for example:
_.set({}, "a.b.c", "d"); // { "a": { "b": { "c": "d" } } }
edited Dec 15 '16 at 12:33
answered Dec 15 '16 at 12:24
Momos Morbias
14813
14813
add a comment |
add a comment |
There are two different notations to access object properties
Dot notation: myObj.prop1
Bracket notation: myObj["prop1"]
Dot notation is fast and easy but you must use the actual property name explicitly. No substitution, variables, etc.
Bracket notation is open ended. It uses a string but you can produce the string using any legal js code. You may specify the string as literal (though in this case dot notation would read easier) or use a variable or calculate in some way.
So, these all set the myObj property named prop1 to the value Hello:
// quick easy-on-the-eye dot notation
myObj.prop1 = "Hello";
// brackets+literal
myObj["prop1"] = "Hello";
// using a variable
var x = "prop1";
myObj[x] = "Hello";
// calculate the accessor string in some weird way
var numList = [0,1,2];
myObj[ "prop" + numList[1] ] = "Hello";
Pitfalls:
myObj.[xxxx] = "Hello"; // wrong: mixed notations, syntax fail
myObj[prop1] = "Hello"; // wrong: this expects a variable called prop1
tl;dnr: If you want to compute or reference the key you must use bracket notation. If you are using the key explicitly, then use dot notation for simple clear code.
Note: there are some other good and correct answers but I personally found them a bit brief coming from a low familiarity with JS on-the-fly quirkiness. This might be useful to some people.
add a comment |
There are two different notations to access object properties
Dot notation: myObj.prop1
Bracket notation: myObj["prop1"]
Dot notation is fast and easy but you must use the actual property name explicitly. No substitution, variables, etc.
Bracket notation is open ended. It uses a string but you can produce the string using any legal js code. You may specify the string as literal (though in this case dot notation would read easier) or use a variable or calculate in some way.
So, these all set the myObj property named prop1 to the value Hello:
// quick easy-on-the-eye dot notation
myObj.prop1 = "Hello";
// brackets+literal
myObj["prop1"] = "Hello";
// using a variable
var x = "prop1";
myObj[x] = "Hello";
// calculate the accessor string in some weird way
var numList = [0,1,2];
myObj[ "prop" + numList[1] ] = "Hello";
Pitfalls:
myObj.[xxxx] = "Hello"; // wrong: mixed notations, syntax fail
myObj[prop1] = "Hello"; // wrong: this expects a variable called prop1
tl;dnr: If you want to compute or reference the key you must use bracket notation. If you are using the key explicitly, then use dot notation for simple clear code.
Note: there are some other good and correct answers but I personally found them a bit brief coming from a low familiarity with JS on-the-fly quirkiness. This might be useful to some people.
add a comment |
There are two different notations to access object properties
Dot notation: myObj.prop1
Bracket notation: myObj["prop1"]
Dot notation is fast and easy but you must use the actual property name explicitly. No substitution, variables, etc.
Bracket notation is open ended. It uses a string but you can produce the string using any legal js code. You may specify the string as literal (though in this case dot notation would read easier) or use a variable or calculate in some way.
So, these all set the myObj property named prop1 to the value Hello:
// quick easy-on-the-eye dot notation
myObj.prop1 = "Hello";
// brackets+literal
myObj["prop1"] = "Hello";
// using a variable
var x = "prop1";
myObj[x] = "Hello";
// calculate the accessor string in some weird way
var numList = [0,1,2];
myObj[ "prop" + numList[1] ] = "Hello";
Pitfalls:
myObj.[xxxx] = "Hello"; // wrong: mixed notations, syntax fail
myObj[prop1] = "Hello"; // wrong: this expects a variable called prop1
tl;dnr: If you want to compute or reference the key you must use bracket notation. If you are using the key explicitly, then use dot notation for simple clear code.
Note: there are some other good and correct answers but I personally found them a bit brief coming from a low familiarity with JS on-the-fly quirkiness. This might be useful to some people.
There are two different notations to access object properties
Dot notation: myObj.prop1
Bracket notation: myObj["prop1"]
Dot notation is fast and easy but you must use the actual property name explicitly. No substitution, variables, etc.
Bracket notation is open ended. It uses a string but you can produce the string using any legal js code. You may specify the string as literal (though in this case dot notation would read easier) or use a variable or calculate in some way.
So, these all set the myObj property named prop1 to the value Hello:
// quick easy-on-the-eye dot notation
myObj.prop1 = "Hello";
// brackets+literal
myObj["prop1"] = "Hello";
// using a variable
var x = "prop1";
myObj[x] = "Hello";
// calculate the accessor string in some weird way
var numList = [0,1,2];
myObj[ "prop" + numList[1] ] = "Hello";
Pitfalls:
myObj.[xxxx] = "Hello"; // wrong: mixed notations, syntax fail
myObj[prop1] = "Hello"; // wrong: this expects a variable called prop1
tl;dnr: If you want to compute or reference the key you must use bracket notation. If you are using the key explicitly, then use dot notation for simple clear code.
Note: there are some other good and correct answers but I personally found them a bit brief coming from a low familiarity with JS on-the-fly quirkiness. This might be useful to some people.
edited May 11 at 1:20
answered May 10 at 7:07


jim birch
936
936
add a comment |
add a comment |
With the advent of ES2015 Object.assign and computed property names the OP's code boils down to:
var obj = Object.assign.apply({}, $(itemsFromDom).map((i, el) => ({[el.id]: el.value})));
add a comment |
With the advent of ES2015 Object.assign and computed property names the OP's code boils down to:
var obj = Object.assign.apply({}, $(itemsFromDom).map((i, el) => ({[el.id]: el.value})));
add a comment |
With the advent of ES2015 Object.assign and computed property names the OP's code boils down to:
var obj = Object.assign.apply({}, $(itemsFromDom).map((i, el) => ({[el.id]: el.value})));
With the advent of ES2015 Object.assign and computed property names the OP's code boils down to:
var obj = Object.assign.apply({}, $(itemsFromDom).map((i, el) => ({[el.id]: el.value})));
answered Mar 5 '17 at 16:59


wOxxOm
26.1k34461
26.1k34461
add a comment |
add a comment |
objectname.newProperty = value;
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
add a comment |
objectname.newProperty = value;
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
add a comment |
objectname.newProperty = value;
objectname.newProperty = value;
answered May 29 '17 at 7:28
ARNAB
407
407
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
add a comment |
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
1
1
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
Did you read the question?
– Badacadabra
May 29 '17 at 13:45
add a comment |
If you want to add fields to an object dynamically, simplest way to do it is as follows:
var params= [
{key: "k1", value=1},
{key: "k2", value=2},
{key: "k3", value=3}];
for(i=0; i< params.len; i++) {
data[params[i].key] = params[i].value
}
This will create data object which has following fields:
{k1:1, k2:2, k3:3}
add a comment |
If you want to add fields to an object dynamically, simplest way to do it is as follows:
var params= [
{key: "k1", value=1},
{key: "k2", value=2},
{key: "k3", value=3}];
for(i=0; i< params.len; i++) {
data[params[i].key] = params[i].value
}
This will create data object which has following fields:
{k1:1, k2:2, k3:3}
add a comment |
If you want to add fields to an object dynamically, simplest way to do it is as follows:
var params= [
{key: "k1", value=1},
{key: "k2", value=2},
{key: "k3", value=3}];
for(i=0; i< params.len; i++) {
data[params[i].key] = params[i].value
}
This will create data object which has following fields:
{k1:1, k2:2, k3:3}
If you want to add fields to an object dynamically, simplest way to do it is as follows:
var params= [
{key: "k1", value=1},
{key: "k2", value=2},
{key: "k3", value=3}];
for(i=0; i< params.len; i++) {
data[params[i].key] = params[i].value
}
This will create data object which has following fields:
{k1:1, k2:2, k3:3}
answered Aug 10 '17 at 6:35
Sruthi Poddutur
45945
45945
add a comment |
add a comment |
Based on the wOxxOm's answer, this is an example of use:
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
add a comment |
Based on the wOxxOm's answer, this is an example of use:
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
add a comment |
Based on the wOxxOm's answer, this is an example of use:
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
Based on the wOxxOm's answer, this is an example of use:
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
const data = [
{"id": "z1", "val":10},
{"id": "z2", "val":20},
{"id": "z3", "val":30}
];
const obj = Object.assign.apply({}, data.map((el, i) => ({[el.id]: el.val})));
console.log(obj);
answered Apr 5 at 19:24
robe007
1,05521338
1,05521338
add a comment |
add a comment |
const data = [{
name: 'BMW',
value: '25641'
}, {
name: 'Apple',
value: '45876'
},
{
name: 'Benz',
value: '65784'
},
{
name: 'Toyota',
value: '254'
}
]
const obj = {
carsList: [{
name: 'Ford',
value: '47563'
}, {
name: 'Toyota',
value: '254'
}],
pastriesList: ,
fruitsList: [{
name: 'Apple',
value: '45876'
}, {
name: 'Pineapple',
value: '84523'
}]
}
let keys = Object.keys(obj);
result = {};
for(key of keys){
let a = [...data,...obj[key]];
result[key] = a;
}
add a comment |
const data = [{
name: 'BMW',
value: '25641'
}, {
name: 'Apple',
value: '45876'
},
{
name: 'Benz',
value: '65784'
},
{
name: 'Toyota',
value: '254'
}
]
const obj = {
carsList: [{
name: 'Ford',
value: '47563'
}, {
name: 'Toyota',
value: '254'
}],
pastriesList: ,
fruitsList: [{
name: 'Apple',
value: '45876'
}, {
name: 'Pineapple',
value: '84523'
}]
}
let keys = Object.keys(obj);
result = {};
for(key of keys){
let a = [...data,...obj[key]];
result[key] = a;
}
add a comment |
const data = [{
name: 'BMW',
value: '25641'
}, {
name: 'Apple',
value: '45876'
},
{
name: 'Benz',
value: '65784'
},
{
name: 'Toyota',
value: '254'
}
]
const obj = {
carsList: [{
name: 'Ford',
value: '47563'
}, {
name: 'Toyota',
value: '254'
}],
pastriesList: ,
fruitsList: [{
name: 'Apple',
value: '45876'
}, {
name: 'Pineapple',
value: '84523'
}]
}
let keys = Object.keys(obj);
result = {};
for(key of keys){
let a = [...data,...obj[key]];
result[key] = a;
}
const data = [{
name: 'BMW',
value: '25641'
}, {
name: 'Apple',
value: '45876'
},
{
name: 'Benz',
value: '65784'
},
{
name: 'Toyota',
value: '254'
}
]
const obj = {
carsList: [{
name: 'Ford',
value: '47563'
}, {
name: 'Toyota',
value: '254'
}],
pastriesList: ,
fruitsList: [{
name: 'Apple',
value: '45876'
}, {
name: 'Pineapple',
value: '84523'
}]
}
let keys = Object.keys(obj);
result = {};
for(key of keys){
let a = [...data,...obj[key]];
result[key] = a;
}
answered Nov 28 at 17:04


Shubham Asolkar
11
11
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f695050%2fadd-a-property-to-a-javascript-object-using-a-variable-as-the-name%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
EUiKgz1IPYORJ R,B4J LX6HbcCmjMFb yDF6x8O gH7v5Kuv2GIpc
See also property access: dot notation vs. brackets? and Dynamically access object property using variable
– Bergi
Nov 18 '14 at 6:11
See also How to create an object property from a variable value in JavaScript?
– Bergi
Aug 18 '15 at 23:40