How to subscribe to property in created object in java
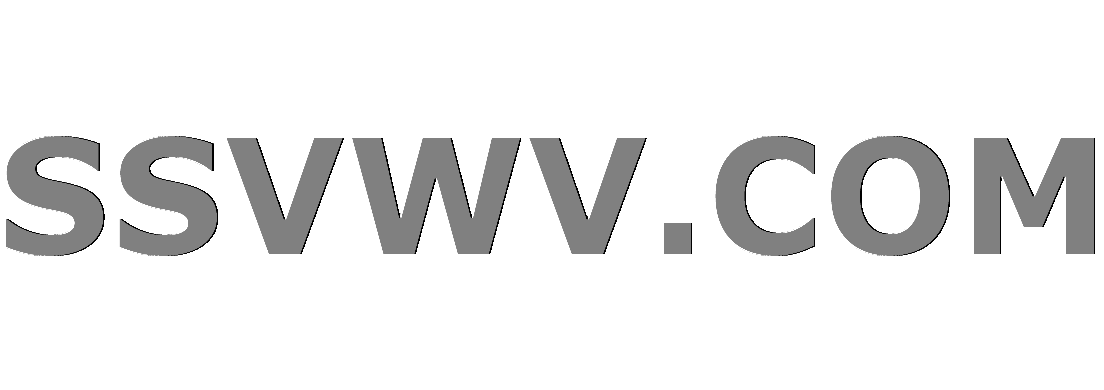
Multi tool use
I have a class which implement an interface from third part library and one of methods is passing object of class called Velocity
.
How I can listen to specific variable value changes in this object?
Here is the code for better understanding.
This is interface from the third part library :
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
and the Velocity
class is packed in the library and it is very simple.
The class has 3 attributes.
I implemented this interface in my class as follows:
public class MyCar implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("widnowIsOpened" + velocity.widnowIsOpened());
/*
I need here to set up a listener for the boolean value widnowIsOpened
because this boolean may be change later and this method will not be invoked again
, it is just invoked once but
value of windowIsOpened may change by the library
*/
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
What I need is to listen to the boolean value changes to react to the changes in my code. I did a lot of searching on this topic, but all possible solutions that i found if I have access to Velocity class so I can set listener inside the Velocity
class,
but in my case I have only the passed object.
So the only thing I can do is to check if the
widnowIsOpened
is true or false, but not for change.
Any help?
java variables listener
add a comment |
I have a class which implement an interface from third part library and one of methods is passing object of class called Velocity
.
How I can listen to specific variable value changes in this object?
Here is the code for better understanding.
This is interface from the third part library :
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
and the Velocity
class is packed in the library and it is very simple.
The class has 3 attributes.
I implemented this interface in my class as follows:
public class MyCar implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("widnowIsOpened" + velocity.widnowIsOpened());
/*
I need here to set up a listener for the boolean value widnowIsOpened
because this boolean may be change later and this method will not be invoked again
, it is just invoked once but
value of windowIsOpened may change by the library
*/
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
What I need is to listen to the boolean value changes to react to the changes in my code. I did a lot of searching on this topic, but all possible solutions that i found if I have access to Velocity class so I can set listener inside the Velocity
class,
but in my case I have only the passed object.
So the only thing I can do is to check if the
widnowIsOpened
is true or false, but not for change.
Any help?
java variables listener
add a comment |
I have a class which implement an interface from third part library and one of methods is passing object of class called Velocity
.
How I can listen to specific variable value changes in this object?
Here is the code for better understanding.
This is interface from the third part library :
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
and the Velocity
class is packed in the library and it is very simple.
The class has 3 attributes.
I implemented this interface in my class as follows:
public class MyCar implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("widnowIsOpened" + velocity.widnowIsOpened());
/*
I need here to set up a listener for the boolean value widnowIsOpened
because this boolean may be change later and this method will not be invoked again
, it is just invoked once but
value of windowIsOpened may change by the library
*/
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
What I need is to listen to the boolean value changes to react to the changes in my code. I did a lot of searching on this topic, but all possible solutions that i found if I have access to Velocity class so I can set listener inside the Velocity
class,
but in my case I have only the passed object.
So the only thing I can do is to check if the
widnowIsOpened
is true or false, but not for change.
Any help?
java variables listener
I have a class which implement an interface from third part library and one of methods is passing object of class called Velocity
.
How I can listen to specific variable value changes in this object?
Here is the code for better understanding.
This is interface from the third part library :
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
and the Velocity
class is packed in the library and it is very simple.
The class has 3 attributes.
I implemented this interface in my class as follows:
public class MyCar implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("widnowIsOpened" + velocity.widnowIsOpened());
/*
I need here to set up a listener for the boolean value widnowIsOpened
because this boolean may be change later and this method will not be invoked again
, it is just invoked once but
value of windowIsOpened may change by the library
*/
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
What I need is to listen to the boolean value changes to react to the changes in my code. I did a lot of searching on this topic, but all possible solutions that i found if I have access to Velocity class so I can set listener inside the Velocity
class,
but in my case I have only the passed object.
So the only thing I can do is to check if the
widnowIsOpened
is true or false, but not for change.
Any help?
java variables listener
java variables listener
edited Nov 28 '18 at 18:59
Somrai
asked Nov 28 '18 at 16:02
SomraiSomrai
62
62
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
You can write your custom listener class with some method in that class. Call that method of listener class inside onObjectMoved() method based on the boolean value.
If you want to send update to multiple listeners, then write a interface for listener and writes its implementations.
If you want I can share some piece of code with you.
Here you go:
public class MyCar implements MotionListener {
private VelocityListener listener;
// added constructor
public MyCar(VelocityListener listener) {
this.listener = listener;
}
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// added handling
listener.doSomething(velocity);
// I need here to set up a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
public static void main(String args) {
MyCar car = new MyCar(new VelocityListenerImpl());
//if you are using java 8 then you can use functional interface like below
//MyCar otherCar = new MyCar(()->{System.out.println("velocity changed....");});
}
}
Listener and its implementation
public interface VelocityListener {
public void doSomething(Velocity velocity);}
public class VelocityListenerImpl implements VelocityListener {
public void doSomething(Velocity velocity) {
while (true) {
if (velocity.isMoving()) {
System.out.println("velocity changed....");
}
}
}}
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
add a comment |
is actually the oposite, the car need the interface but no need to implement it
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
public class MyCar {
private MotionListener myMotionListener;
private void setMotionListener(MotionListener someMotionListener){
myMotionListener= someMotionListener;
}
public void doSomething(){
if(myMotionListener != null){
myMotionListener.onObjectMoved(myVelocity);
}
}
public void notifiyamStop(){
if(myMotionListener != null){
myMotionListener.onObjectStopped();
}
}
// other methods ...
}
public class MyPolice implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// i need here to setup a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
add a comment |
Apparently, MotionListener is the listener. You need to implement it. Each time MyCar moves, the OnObjectMoved() and OnObjectStopped() methods are called. In those methods, do whatever needs to happen when the car moves or stops, such as recalculating the position.
As far as listeners, the listener will be called by the external entity each time the velocity changes. So, your method simply needs to look at the value and do something with it. I'm guessing Velocity has getters for speed and direction.
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523532%2fhow-to-subscribe-to-property-in-created-object-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
You can write your custom listener class with some method in that class. Call that method of listener class inside onObjectMoved() method based on the boolean value.
If you want to send update to multiple listeners, then write a interface for listener and writes its implementations.
If you want I can share some piece of code with you.
Here you go:
public class MyCar implements MotionListener {
private VelocityListener listener;
// added constructor
public MyCar(VelocityListener listener) {
this.listener = listener;
}
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// added handling
listener.doSomething(velocity);
// I need here to set up a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
public static void main(String args) {
MyCar car = new MyCar(new VelocityListenerImpl());
//if you are using java 8 then you can use functional interface like below
//MyCar otherCar = new MyCar(()->{System.out.println("velocity changed....");});
}
}
Listener and its implementation
public interface VelocityListener {
public void doSomething(Velocity velocity);}
public class VelocityListenerImpl implements VelocityListener {
public void doSomething(Velocity velocity) {
while (true) {
if (velocity.isMoving()) {
System.out.println("velocity changed....");
}
}
}}
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
add a comment |
You can write your custom listener class with some method in that class. Call that method of listener class inside onObjectMoved() method based on the boolean value.
If you want to send update to multiple listeners, then write a interface for listener and writes its implementations.
If you want I can share some piece of code with you.
Here you go:
public class MyCar implements MotionListener {
private VelocityListener listener;
// added constructor
public MyCar(VelocityListener listener) {
this.listener = listener;
}
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// added handling
listener.doSomething(velocity);
// I need here to set up a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
public static void main(String args) {
MyCar car = new MyCar(new VelocityListenerImpl());
//if you are using java 8 then you can use functional interface like below
//MyCar otherCar = new MyCar(()->{System.out.println("velocity changed....");});
}
}
Listener and its implementation
public interface VelocityListener {
public void doSomething(Velocity velocity);}
public class VelocityListenerImpl implements VelocityListener {
public void doSomething(Velocity velocity) {
while (true) {
if (velocity.isMoving()) {
System.out.println("velocity changed....");
}
}
}}
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
add a comment |
You can write your custom listener class with some method in that class. Call that method of listener class inside onObjectMoved() method based on the boolean value.
If you want to send update to multiple listeners, then write a interface for listener and writes its implementations.
If you want I can share some piece of code with you.
Here you go:
public class MyCar implements MotionListener {
private VelocityListener listener;
// added constructor
public MyCar(VelocityListener listener) {
this.listener = listener;
}
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// added handling
listener.doSomething(velocity);
// I need here to set up a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
public static void main(String args) {
MyCar car = new MyCar(new VelocityListenerImpl());
//if you are using java 8 then you can use functional interface like below
//MyCar otherCar = new MyCar(()->{System.out.println("velocity changed....");});
}
}
Listener and its implementation
public interface VelocityListener {
public void doSomething(Velocity velocity);}
public class VelocityListenerImpl implements VelocityListener {
public void doSomething(Velocity velocity) {
while (true) {
if (velocity.isMoving()) {
System.out.println("velocity changed....");
}
}
}}
You can write your custom listener class with some method in that class. Call that method of listener class inside onObjectMoved() method based on the boolean value.
If you want to send update to multiple listeners, then write a interface for listener and writes its implementations.
If you want I can share some piece of code with you.
Here you go:
public class MyCar implements MotionListener {
private VelocityListener listener;
// added constructor
public MyCar(VelocityListener listener) {
this.listener = listener;
}
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// added handling
listener.doSomething(velocity);
// I need here to set up a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
public static void main(String args) {
MyCar car = new MyCar(new VelocityListenerImpl());
//if you are using java 8 then you can use functional interface like below
//MyCar otherCar = new MyCar(()->{System.out.println("velocity changed....");});
}
}
Listener and its implementation
public interface VelocityListener {
public void doSomething(Velocity velocity);}
public class VelocityListenerImpl implements VelocityListener {
public void doSomething(Velocity velocity) {
while (true) {
if (velocity.isMoving()) {
System.out.println("velocity changed....");
}
}
}}
edited Nov 29 '18 at 14:48
answered Nov 28 '18 at 16:10
G.G.G.G.
337212
337212
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
add a comment |
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
yes please can you show me how
– Somrai
Nov 28 '18 at 16:12
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
what if the value changed after line is executed for example : if (velocity.isMoving()) { listener.doSomething(); } it is now = true but after 5 minutes it changed how i will figure this change ?
– Somrai
Nov 28 '18 at 16:55
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
does onObjectMoved() method is triggered on velocity change? If yes, you do not need to do anything and doSomething will automatically will be called.
– G.G.
Nov 28 '18 at 16:58
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
yes this is the case onObjectMoved() not triggered on velocity change that what i need to have listener for the object that is passed from first place
– Somrai
Nov 28 '18 at 18:49
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
That should not be the case that velocity is changed and onObjectMoved() is not called. Btw, I have updated code snippet for your case. Kindly take a look.
– G.G.
Nov 29 '18 at 2:33
add a comment |
is actually the oposite, the car need the interface but no need to implement it
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
public class MyCar {
private MotionListener myMotionListener;
private void setMotionListener(MotionListener someMotionListener){
myMotionListener= someMotionListener;
}
public void doSomething(){
if(myMotionListener != null){
myMotionListener.onObjectMoved(myVelocity);
}
}
public void notifiyamStop(){
if(myMotionListener != null){
myMotionListener.onObjectStopped();
}
}
// other methods ...
}
public class MyPolice implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// i need here to setup a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
add a comment |
is actually the oposite, the car need the interface but no need to implement it
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
public class MyCar {
private MotionListener myMotionListener;
private void setMotionListener(MotionListener someMotionListener){
myMotionListener= someMotionListener;
}
public void doSomething(){
if(myMotionListener != null){
myMotionListener.onObjectMoved(myVelocity);
}
}
public void notifiyamStop(){
if(myMotionListener != null){
myMotionListener.onObjectStopped();
}
}
// other methods ...
}
public class MyPolice implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// i need here to setup a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
add a comment |
is actually the oposite, the car need the interface but no need to implement it
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
public class MyCar {
private MotionListener myMotionListener;
private void setMotionListener(MotionListener someMotionListener){
myMotionListener= someMotionListener;
}
public void doSomething(){
if(myMotionListener != null){
myMotionListener.onObjectMoved(myVelocity);
}
}
public void notifiyamStop(){
if(myMotionListener != null){
myMotionListener.onObjectStopped();
}
}
// other methods ...
}
public class MyPolice implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// i need here to setup a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
is actually the oposite, the car need the interface but no need to implement it
public interface MotionListener {
void onObjectMoved(Velocity velocity);
void onObjectStopped();
}
public class MyCar {
private MotionListener myMotionListener;
private void setMotionListener(MotionListener someMotionListener){
myMotionListener= someMotionListener;
}
public void doSomething(){
if(myMotionListener != null){
myMotionListener.onObjectMoved(myVelocity);
}
}
public void notifiyamStop(){
if(myMotionListener != null){
myMotionListener.onObjectStopped();
}
}
// other methods ...
}
public class MyPolice implements MotionListener {
@Override
public void onObjectMoved(Velocity velocity) {
System.out.println("Distance" + velocity.getDistance());
System.out.println("Speed" + velocity.getSpeed());
System.out.println("isMoving" + velocity.isMoving());
// i need here to setup a listener for the boolean value isMoving
}
@Override
public void onObjectStopped() {
}
// other methods ...
}
answered Nov 28 '18 at 16:08


ΦXocę 웃 Пepeúpa ツΦXocę 웃 Пepeúpa ツ
34.1k113965
34.1k113965
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
add a comment |
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
could you please explain how i will use myCar object in MyPolice class that you created ?
– Somrai
Nov 28 '18 at 16:14
add a comment |
Apparently, MotionListener is the listener. You need to implement it. Each time MyCar moves, the OnObjectMoved() and OnObjectStopped() methods are called. In those methods, do whatever needs to happen when the car moves or stops, such as recalculating the position.
As far as listeners, the listener will be called by the external entity each time the velocity changes. So, your method simply needs to look at the value and do something with it. I'm guessing Velocity has getters for speed and direction.
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
add a comment |
Apparently, MotionListener is the listener. You need to implement it. Each time MyCar moves, the OnObjectMoved() and OnObjectStopped() methods are called. In those methods, do whatever needs to happen when the car moves or stops, such as recalculating the position.
As far as listeners, the listener will be called by the external entity each time the velocity changes. So, your method simply needs to look at the value and do something with it. I'm guessing Velocity has getters for speed and direction.
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
add a comment |
Apparently, MotionListener is the listener. You need to implement it. Each time MyCar moves, the OnObjectMoved() and OnObjectStopped() methods are called. In those methods, do whatever needs to happen when the car moves or stops, such as recalculating the position.
As far as listeners, the listener will be called by the external entity each time the velocity changes. So, your method simply needs to look at the value and do something with it. I'm guessing Velocity has getters for speed and direction.
Apparently, MotionListener is the listener. You need to implement it. Each time MyCar moves, the OnObjectMoved() and OnObjectStopped() methods are called. In those methods, do whatever needs to happen when the car moves or stops, such as recalculating the position.
As far as listeners, the listener will be called by the external entity each time the velocity changes. So, your method simply needs to look at the value and do something with it. I'm guessing Velocity has getters for speed and direction.
edited Nov 28 '18 at 16:39
answered Nov 28 '18 at 16:25


Steve11235Steve11235
2,33411317
2,33411317
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
add a comment |
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
I updated the name of the boolean value regarding the business : how is possible to listen to a variable which is in this object
– Somrai
Nov 28 '18 at 16:28
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523532%2fhow-to-subscribe-to-property-in-created-object-in-java%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
g,hDHoQ5GV,oB,Pceo6aUi3,Uyx7SHo W