Add values in independent dictionaries when the values are (2x2) numpy arrays
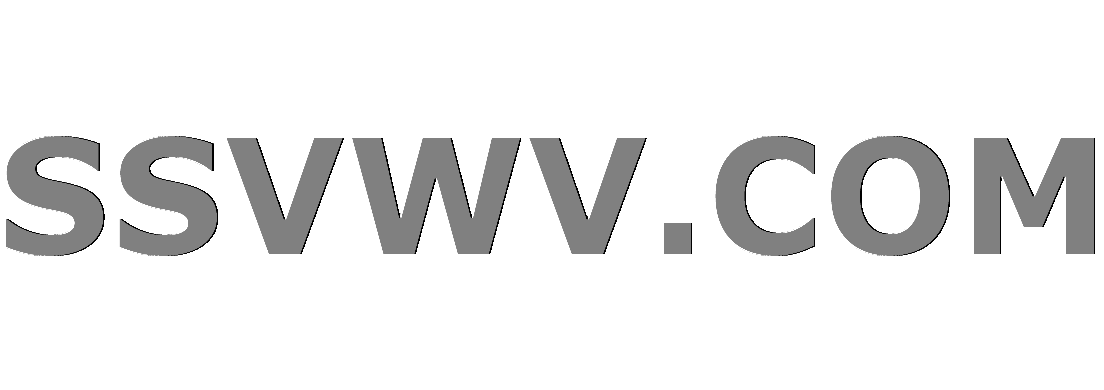
Multi tool use
Suppose I have two dictionaries with the same keys and all the values are 2x2 numpy arrays. Assumptions:
- dictionaries have the same keys
- every value is a 2x2 numpy array for all dictionaries and keys.
x1 and x2 are sample dictionaries.
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
I would like to add both x1 and x2 together by their keys and the result would be a new dictionary.
So if...
x1[0] = [[1,2],[3,4]]
and...
x2[0] = [[10,20],[30,40]]
a new dictionary value when key = 0, would be...
x_total[0] = [[11,22],[33,44]]
The next step would be to do this for many dictionaries with this structure. I was thinking of doing this in a for loop, but If there are more efficient solutions, I'd love to learn about them.
I've tried the approach below using the collections library
from collections import Counter
a = Counter(x1[0])
b = Counter(x2[0])
c = dict(a + b)
but I think this may not apply if the values are arrays.
I also know that np.add(x1[0], x2[0])
will result in the addition of the arrays but I'd like to do it across all keys at once.. if possible.
python dictionary numpy-ndarray
add a comment |
Suppose I have two dictionaries with the same keys and all the values are 2x2 numpy arrays. Assumptions:
- dictionaries have the same keys
- every value is a 2x2 numpy array for all dictionaries and keys.
x1 and x2 are sample dictionaries.
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
I would like to add both x1 and x2 together by their keys and the result would be a new dictionary.
So if...
x1[0] = [[1,2],[3,4]]
and...
x2[0] = [[10,20],[30,40]]
a new dictionary value when key = 0, would be...
x_total[0] = [[11,22],[33,44]]
The next step would be to do this for many dictionaries with this structure. I was thinking of doing this in a for loop, but If there are more efficient solutions, I'd love to learn about them.
I've tried the approach below using the collections library
from collections import Counter
a = Counter(x1[0])
b = Counter(x2[0])
c = dict(a + b)
but I think this may not apply if the values are arrays.
I also know that np.add(x1[0], x2[0])
will result in the addition of the arrays but I'd like to do it across all keys at once.. if possible.
python dictionary numpy-ndarray
add a comment |
Suppose I have two dictionaries with the same keys and all the values are 2x2 numpy arrays. Assumptions:
- dictionaries have the same keys
- every value is a 2x2 numpy array for all dictionaries and keys.
x1 and x2 are sample dictionaries.
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
I would like to add both x1 and x2 together by their keys and the result would be a new dictionary.
So if...
x1[0] = [[1,2],[3,4]]
and...
x2[0] = [[10,20],[30,40]]
a new dictionary value when key = 0, would be...
x_total[0] = [[11,22],[33,44]]
The next step would be to do this for many dictionaries with this structure. I was thinking of doing this in a for loop, but If there are more efficient solutions, I'd love to learn about them.
I've tried the approach below using the collections library
from collections import Counter
a = Counter(x1[0])
b = Counter(x2[0])
c = dict(a + b)
but I think this may not apply if the values are arrays.
I also know that np.add(x1[0], x2[0])
will result in the addition of the arrays but I'd like to do it across all keys at once.. if possible.
python dictionary numpy-ndarray
Suppose I have two dictionaries with the same keys and all the values are 2x2 numpy arrays. Assumptions:
- dictionaries have the same keys
- every value is a 2x2 numpy array for all dictionaries and keys.
x1 and x2 are sample dictionaries.
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
I would like to add both x1 and x2 together by their keys and the result would be a new dictionary.
So if...
x1[0] = [[1,2],[3,4]]
and...
x2[0] = [[10,20],[30,40]]
a new dictionary value when key = 0, would be...
x_total[0] = [[11,22],[33,44]]
The next step would be to do this for many dictionaries with this structure. I was thinking of doing this in a for loop, but If there are more efficient solutions, I'd love to learn about them.
I've tried the approach below using the collections library
from collections import Counter
a = Counter(x1[0])
b = Counter(x2[0])
c = dict(a + b)
but I think this may not apply if the values are arrays.
I also know that np.add(x1[0], x2[0])
will result in the addition of the arrays but I'd like to do it across all keys at once.. if possible.
python dictionary numpy-ndarray
python dictionary numpy-ndarray
edited Nov 28 '18 at 20:17
Sheila
asked Nov 28 '18 at 19:56
SheilaSheila
86071329
86071329
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Simply use a dictionary comprehension:
{k: x1.get(k,0) + x2.get(k,0) for k in set(x1)}
For example:
import numpy as np
np.random.seed(0)
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
Yields:
{0: array([[12, 15],
[ 0, 3]]), 1: array([[ 3, 7],
[ 9, 19]]), 2: array([[18, 4],
[ 6, 12]]), 3: array([[ 1, 6],
[ 7, 14]]), 4: array([[17, 5],
[13, 8]])}
{0: array([[ 9, 19],
[16, 19]]), 1: array([[ 5, 15],
[15, 0]]), 2: array([[18, 3],
[17, 19]]), 3: array([[19, 19],
[14, 7]]), 4: array([[0, 1],
[9, 0]])}
Then applying our solution, we get:
{0: array([[21, 34],
[16, 22]]), 1: array([[ 8, 22],
[24, 19]]), 2: array([[36, 7],
[23, 31]]), 3: array([[20, 25],
[21, 21]]), 4: array([[17, 6],
[22, 8]])}
add a comment |
Assuming all dictionaries are complete (they all have the same keys) a dict comprehension should be an efficient solution:
x3 = {key: sum(x1[key] + x2[key]) for key in x1}
add a comment |
I'm using shorter datastructures for readability and assume that x1
and x2
share the same keys.
>>> x1 = {k:np.arange(k, k+2) for k in range(2)}
>>> x2 = {k:np.arange(k+1, k+3) for k in range(2)}
>>>
>>> x1
{0: array([0, 1]), 1: array([1, 2])}
>>> x2
{0: array([1, 2]), 1: array([2, 3])}
>>>
>>> x_total = {k: x1[k] + x2[k] for k in x1}
>>> x_total
{0: array([1, 3]), 1: array([3, 5])}
Note that dictionaries with consecutive integer keys, especially when they start from zero, are a waste of memory (sparseness) and time (hashing). Why not use an array into which you can index with integers much more efficiently?
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53527149%2fadd-values-in-independent-dictionaries-when-the-values-are-2x2-numpy-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Simply use a dictionary comprehension:
{k: x1.get(k,0) + x2.get(k,0) for k in set(x1)}
For example:
import numpy as np
np.random.seed(0)
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
Yields:
{0: array([[12, 15],
[ 0, 3]]), 1: array([[ 3, 7],
[ 9, 19]]), 2: array([[18, 4],
[ 6, 12]]), 3: array([[ 1, 6],
[ 7, 14]]), 4: array([[17, 5],
[13, 8]])}
{0: array([[ 9, 19],
[16, 19]]), 1: array([[ 5, 15],
[15, 0]]), 2: array([[18, 3],
[17, 19]]), 3: array([[19, 19],
[14, 7]]), 4: array([[0, 1],
[9, 0]])}
Then applying our solution, we get:
{0: array([[21, 34],
[16, 22]]), 1: array([[ 8, 22],
[24, 19]]), 2: array([[36, 7],
[23, 31]]), 3: array([[20, 25],
[21, 21]]), 4: array([[17, 6],
[22, 8]])}
add a comment |
Simply use a dictionary comprehension:
{k: x1.get(k,0) + x2.get(k,0) for k in set(x1)}
For example:
import numpy as np
np.random.seed(0)
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
Yields:
{0: array([[12, 15],
[ 0, 3]]), 1: array([[ 3, 7],
[ 9, 19]]), 2: array([[18, 4],
[ 6, 12]]), 3: array([[ 1, 6],
[ 7, 14]]), 4: array([[17, 5],
[13, 8]])}
{0: array([[ 9, 19],
[16, 19]]), 1: array([[ 5, 15],
[15, 0]]), 2: array([[18, 3],
[17, 19]]), 3: array([[19, 19],
[14, 7]]), 4: array([[0, 1],
[9, 0]])}
Then applying our solution, we get:
{0: array([[21, 34],
[16, 22]]), 1: array([[ 8, 22],
[24, 19]]), 2: array([[36, 7],
[23, 31]]), 3: array([[20, 25],
[21, 21]]), 4: array([[17, 6],
[22, 8]])}
add a comment |
Simply use a dictionary comprehension:
{k: x1.get(k,0) + x2.get(k,0) for k in set(x1)}
For example:
import numpy as np
np.random.seed(0)
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
Yields:
{0: array([[12, 15],
[ 0, 3]]), 1: array([[ 3, 7],
[ 9, 19]]), 2: array([[18, 4],
[ 6, 12]]), 3: array([[ 1, 6],
[ 7, 14]]), 4: array([[17, 5],
[13, 8]])}
{0: array([[ 9, 19],
[16, 19]]), 1: array([[ 5, 15],
[15, 0]]), 2: array([[18, 3],
[17, 19]]), 3: array([[19, 19],
[14, 7]]), 4: array([[0, 1],
[9, 0]])}
Then applying our solution, we get:
{0: array([[21, 34],
[16, 22]]), 1: array([[ 8, 22],
[24, 19]]), 2: array([[36, 7],
[23, 31]]), 3: array([[20, 25],
[21, 21]]), 4: array([[17, 6],
[22, 8]])}
Simply use a dictionary comprehension:
{k: x1.get(k,0) + x2.get(k,0) for k in set(x1)}
For example:
import numpy as np
np.random.seed(0)
x1 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
x2 = {k: np.random.randint(20, size=(2, 2)) for k in range(5)}
Yields:
{0: array([[12, 15],
[ 0, 3]]), 1: array([[ 3, 7],
[ 9, 19]]), 2: array([[18, 4],
[ 6, 12]]), 3: array([[ 1, 6],
[ 7, 14]]), 4: array([[17, 5],
[13, 8]])}
{0: array([[ 9, 19],
[16, 19]]), 1: array([[ 5, 15],
[15, 0]]), 2: array([[18, 3],
[17, 19]]), 3: array([[19, 19],
[14, 7]]), 4: array([[0, 1],
[9, 0]])}
Then applying our solution, we get:
{0: array([[21, 34],
[16, 22]]), 1: array([[ 8, 22],
[24, 19]]), 2: array([[36, 7],
[23, 31]]), 3: array([[20, 25],
[21, 21]]), 4: array([[17, 6],
[22, 8]])}
answered Nov 28 '18 at 20:01
rahlf23rahlf23
5,3363731
5,3363731
add a comment |
add a comment |
Assuming all dictionaries are complete (they all have the same keys) a dict comprehension should be an efficient solution:
x3 = {key: sum(x1[key] + x2[key]) for key in x1}
add a comment |
Assuming all dictionaries are complete (they all have the same keys) a dict comprehension should be an efficient solution:
x3 = {key: sum(x1[key] + x2[key]) for key in x1}
add a comment |
Assuming all dictionaries are complete (they all have the same keys) a dict comprehension should be an efficient solution:
x3 = {key: sum(x1[key] + x2[key]) for key in x1}
Assuming all dictionaries are complete (they all have the same keys) a dict comprehension should be an efficient solution:
x3 = {key: sum(x1[key] + x2[key]) for key in x1}
answered Nov 28 '18 at 20:01
artonaartona
71248
71248
add a comment |
add a comment |
I'm using shorter datastructures for readability and assume that x1
and x2
share the same keys.
>>> x1 = {k:np.arange(k, k+2) for k in range(2)}
>>> x2 = {k:np.arange(k+1, k+3) for k in range(2)}
>>>
>>> x1
{0: array([0, 1]), 1: array([1, 2])}
>>> x2
{0: array([1, 2]), 1: array([2, 3])}
>>>
>>> x_total = {k: x1[k] + x2[k] for k in x1}
>>> x_total
{0: array([1, 3]), 1: array([3, 5])}
Note that dictionaries with consecutive integer keys, especially when they start from zero, are a waste of memory (sparseness) and time (hashing). Why not use an array into which you can index with integers much more efficiently?
add a comment |
I'm using shorter datastructures for readability and assume that x1
and x2
share the same keys.
>>> x1 = {k:np.arange(k, k+2) for k in range(2)}
>>> x2 = {k:np.arange(k+1, k+3) for k in range(2)}
>>>
>>> x1
{0: array([0, 1]), 1: array([1, 2])}
>>> x2
{0: array([1, 2]), 1: array([2, 3])}
>>>
>>> x_total = {k: x1[k] + x2[k] for k in x1}
>>> x_total
{0: array([1, 3]), 1: array([3, 5])}
Note that dictionaries with consecutive integer keys, especially when they start from zero, are a waste of memory (sparseness) and time (hashing). Why not use an array into which you can index with integers much more efficiently?
add a comment |
I'm using shorter datastructures for readability and assume that x1
and x2
share the same keys.
>>> x1 = {k:np.arange(k, k+2) for k in range(2)}
>>> x2 = {k:np.arange(k+1, k+3) for k in range(2)}
>>>
>>> x1
{0: array([0, 1]), 1: array([1, 2])}
>>> x2
{0: array([1, 2]), 1: array([2, 3])}
>>>
>>> x_total = {k: x1[k] + x2[k] for k in x1}
>>> x_total
{0: array([1, 3]), 1: array([3, 5])}
Note that dictionaries with consecutive integer keys, especially when they start from zero, are a waste of memory (sparseness) and time (hashing). Why not use an array into which you can index with integers much more efficiently?
I'm using shorter datastructures for readability and assume that x1
and x2
share the same keys.
>>> x1 = {k:np.arange(k, k+2) for k in range(2)}
>>> x2 = {k:np.arange(k+1, k+3) for k in range(2)}
>>>
>>> x1
{0: array([0, 1]), 1: array([1, 2])}
>>> x2
{0: array([1, 2]), 1: array([2, 3])}
>>>
>>> x_total = {k: x1[k] + x2[k] for k in x1}
>>> x_total
{0: array([1, 3]), 1: array([3, 5])}
Note that dictionaries with consecutive integer keys, especially when they start from zero, are a waste of memory (sparseness) and time (hashing). Why not use an array into which you can index with integers much more efficiently?
edited Nov 28 '18 at 20:16
answered Nov 28 '18 at 20:03


timgebtimgeb
51.3k126794
51.3k126794
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53527149%2fadd-values-in-independent-dictionaries-when-the-values-are-2x2-numpy-arrays%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0hUF,ia,g7Zs04CaG,fXZt4gmKxW 3l 1HIwv