Reading JSON from a file?
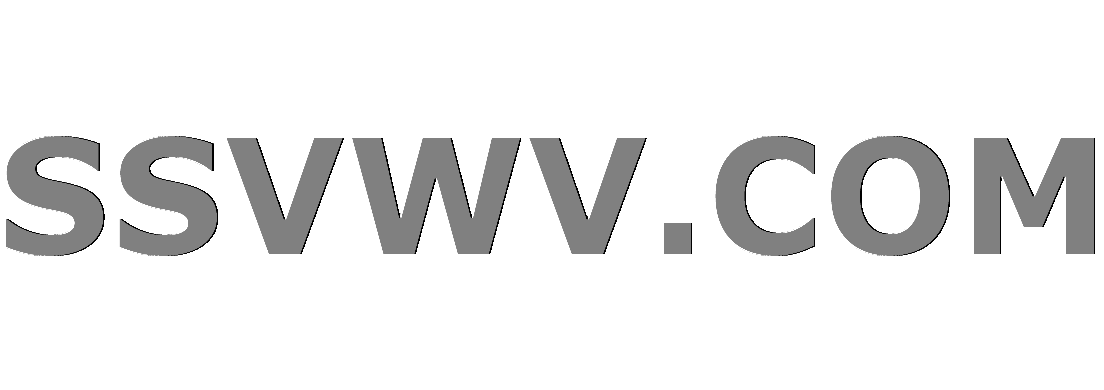
Multi tool use
I am getting a bit of headache just because a simple looking, easy statement is throwing some errors in my face.
I have a json file called strings.json like this:
"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ...,
{"-name": "address", "#text": "Address"}]
I want to read the json file, just that for now. I have these statements which I found out, but it's not working:
import json
from pprint import pprint
with open('strings.json') as json_data:
d = json.load(json_data)
json_data.close()
pprint(d)
The error displayed on the console was this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.loads(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 366, in decode
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
TypeError: expected string or buffer
[Finished in 0.1s with exit code 1]
Edited
Changed from json.loads
to json.load
and got this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.load(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 278, in load
**kw)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 369, in decode
raise ValueError(errmsg("Extra data", s, end, len(s)))
ValueError: Extra data: line 829 column 1 - line 829 column 2 (char 18476 - 18477)
[Finished in 0.1s with exit code 1]
python json
add a comment |
I am getting a bit of headache just because a simple looking, easy statement is throwing some errors in my face.
I have a json file called strings.json like this:
"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ...,
{"-name": "address", "#text": "Address"}]
I want to read the json file, just that for now. I have these statements which I found out, but it's not working:
import json
from pprint import pprint
with open('strings.json') as json_data:
d = json.load(json_data)
json_data.close()
pprint(d)
The error displayed on the console was this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.loads(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 366, in decode
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
TypeError: expected string or buffer
[Finished in 0.1s with exit code 1]
Edited
Changed from json.loads
to json.load
and got this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.load(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 278, in load
**kw)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 369, in decode
raise ValueError(errmsg("Extra data", s, end, len(s)))
ValueError: Extra data: line 829 column 1 - line 829 column 2 (char 18476 - 18477)
[Finished in 0.1s with exit code 1]
python json
6
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
1
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
Your file is an invalid json format. Change it to:{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11
add a comment |
I am getting a bit of headache just because a simple looking, easy statement is throwing some errors in my face.
I have a json file called strings.json like this:
"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ...,
{"-name": "address", "#text": "Address"}]
I want to read the json file, just that for now. I have these statements which I found out, but it's not working:
import json
from pprint import pprint
with open('strings.json') as json_data:
d = json.load(json_data)
json_data.close()
pprint(d)
The error displayed on the console was this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.loads(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 366, in decode
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
TypeError: expected string or buffer
[Finished in 0.1s with exit code 1]
Edited
Changed from json.loads
to json.load
and got this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.load(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 278, in load
**kw)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 369, in decode
raise ValueError(errmsg("Extra data", s, end, len(s)))
ValueError: Extra data: line 829 column 1 - line 829 column 2 (char 18476 - 18477)
[Finished in 0.1s with exit code 1]
python json
I am getting a bit of headache just because a simple looking, easy statement is throwing some errors in my face.
I have a json file called strings.json like this:
"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ...,
{"-name": "address", "#text": "Address"}]
I want to read the json file, just that for now. I have these statements which I found out, but it's not working:
import json
from pprint import pprint
with open('strings.json') as json_data:
d = json.load(json_data)
json_data.close()
pprint(d)
The error displayed on the console was this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.loads(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 366, in decode
obj, end = self.raw_decode(s, idx=_w(s, 0).end())
TypeError: expected string or buffer
[Finished in 0.1s with exit code 1]
Edited
Changed from json.loads
to json.load
and got this:
Traceback (most recent call last):
File "/home/.../android/values/manipulate_json.py", line 5, in <module>
d = json.load(json_data)
File "/usr/lib/python2.7/json/__init__.py", line 278, in load
**kw)
File "/usr/lib/python2.7/json/__init__.py", line 326, in loads
return _default_decoder.decode(s)
File "/usr/lib/python2.7/json/decoder.py", line 369, in decode
raise ValueError(errmsg("Extra data", s, end, len(s)))
ValueError: Extra data: line 829 column 1 - line 829 column 2 (char 18476 - 18477)
[Finished in 0.1s with exit code 1]
python json
python json
edited Jul 20 '18 at 19:05
Łukasz Sromek
2,55212440
2,55212440
asked Nov 25 '13 at 17:15


R.R.C.R.R.C.
1,5492917
1,5492917
6
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
1
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
Your file is an invalid json format. Change it to:{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11
add a comment |
6
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
1
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
Your file is an invalid json format. Change it to:{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11
6
6
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
1
1
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
Your file is an invalid json format. Change it to:
{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11
Your file is an invalid json format. Change it to:
{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11
add a comment |
5 Answers
5
active
oldest
votes
The json.load()
method (without "s" in "load") can read a file directly:
import json
with open('strings.json') as json_data:
d = json.load(json_data)
print(d)
You were using the json.loads()
method, which is used for string arguments only.
Edit:
The new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
There are also solutions for fixing json like for example How do I automatically fix an invalid JSON string?.
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
add a comment |
Here is a copy of code which works fine for me
import json
with open("test.json") as json_file:
json_data = json.load(json_file)
print(json_data)
with the data
{
"a": [1,3,"asdf",true],
"b": {
"Hello": "world"
}
}
you may want to wrap your json.load line with a try catch because invalid JSON will cause a stacktrace error message.
add a comment |
The problem is using with statement:
with open('strings.json') as json_data:
d = json.load(json_data)
pprint(d)
The file is going to be implicitly closed already. There is no need to call json_data.close()
again.
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
to pretty-print, I had to use:print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
add a comment |
In python 3, we can use below method.
Read from file and convert to JSON
import json
# Considering "json_list.json" is a json file
with open('json_list.json') as fd:
json_data = json.load(fd)
or
import json
json_data = json.load(open('json_list.json'))
Using with statement will automatically close the opened file descriptor.
String to JSON
import json
json_data = json.loads('{"name" : "myName", "age":24}')
add a comment |
To add on this, today you are able to use pandas to import json:
https://pandas.pydata.org/pandas-docs/stable/generated/pandas.read_json.html
You may want to do a careful use of the orient parameter.
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
add a comment |
protected by Community♦ Oct 13 '17 at 20:37
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
5 Answers
5
active
oldest
votes
5 Answers
5
active
oldest
votes
active
oldest
votes
active
oldest
votes
The json.load()
method (without "s" in "load") can read a file directly:
import json
with open('strings.json') as json_data:
d = json.load(json_data)
print(d)
You were using the json.loads()
method, which is used for string arguments only.
Edit:
The new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
There are also solutions for fixing json like for example How do I automatically fix an invalid JSON string?.
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
add a comment |
The json.load()
method (without "s" in "load") can read a file directly:
import json
with open('strings.json') as json_data:
d = json.load(json_data)
print(d)
You were using the json.loads()
method, which is used for string arguments only.
Edit:
The new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
There are also solutions for fixing json like for example How do I automatically fix an invalid JSON string?.
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
add a comment |
The json.load()
method (without "s" in "load") can read a file directly:
import json
with open('strings.json') as json_data:
d = json.load(json_data)
print(d)
You were using the json.loads()
method, which is used for string arguments only.
Edit:
The new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
There are also solutions for fixing json like for example How do I automatically fix an invalid JSON string?.
The json.load()
method (without "s" in "load") can read a file directly:
import json
with open('strings.json') as json_data:
d = json.load(json_data)
print(d)
You were using the json.loads()
method, which is used for string arguments only.
Edit:
The new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
There are also solutions for fixing json like for example How do I automatically fix an invalid JSON string?.
edited May 23 '17 at 11:33
Community♦
11
11
answered Nov 25 '13 at 17:19
ubombubomb
5,25721523
5,25721523
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
add a comment |
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
2
2
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
hm...I changed from json.loads to json.load but I get that nice msg.
– R.R.C.
Nov 25 '13 at 17:23
4
4
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
Ah, well the new message is a totally different problem. In that case, there is some invalid json in that file. For that, I would recommend running the file through a json validator.
– ubomb
Nov 25 '13 at 17:26
3
3
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
got it! The file was missing EOF. The file was not correctly ended. I wouldn't notice that if it wasn't your good recommendation! Thanks!
– R.R.C.
Nov 25 '13 at 17:32
1
1
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
ubomb, if you can change you answer to me to mark it as accepted. Be free! I'll mark it.
– R.R.C.
Nov 25 '13 at 17:33
add a comment |
Here is a copy of code which works fine for me
import json
with open("test.json") as json_file:
json_data = json.load(json_file)
print(json_data)
with the data
{
"a": [1,3,"asdf",true],
"b": {
"Hello": "world"
}
}
you may want to wrap your json.load line with a try catch because invalid JSON will cause a stacktrace error message.
add a comment |
Here is a copy of code which works fine for me
import json
with open("test.json") as json_file:
json_data = json.load(json_file)
print(json_data)
with the data
{
"a": [1,3,"asdf",true],
"b": {
"Hello": "world"
}
}
you may want to wrap your json.load line with a try catch because invalid JSON will cause a stacktrace error message.
add a comment |
Here is a copy of code which works fine for me
import json
with open("test.json") as json_file:
json_data = json.load(json_file)
print(json_data)
with the data
{
"a": [1,3,"asdf",true],
"b": {
"Hello": "world"
}
}
you may want to wrap your json.load line with a try catch because invalid JSON will cause a stacktrace error message.
Here is a copy of code which works fine for me
import json
with open("test.json") as json_file:
json_data = json.load(json_file)
print(json_data)
with the data
{
"a": [1,3,"asdf",true],
"b": {
"Hello": "world"
}
}
you may want to wrap your json.load line with a try catch because invalid JSON will cause a stacktrace error message.
answered Nov 25 '13 at 18:46


user1876508user1876508
6,204165289
6,204165289
add a comment |
add a comment |
The problem is using with statement:
with open('strings.json') as json_data:
d = json.load(json_data)
pprint(d)
The file is going to be implicitly closed already. There is no need to call json_data.close()
again.
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
to pretty-print, I had to use:print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
add a comment |
The problem is using with statement:
with open('strings.json') as json_data:
d = json.load(json_data)
pprint(d)
The file is going to be implicitly closed already. There is no need to call json_data.close()
again.
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
to pretty-print, I had to use:print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
add a comment |
The problem is using with statement:
with open('strings.json') as json_data:
d = json.load(json_data)
pprint(d)
The file is going to be implicitly closed already. There is no need to call json_data.close()
again.
The problem is using with statement:
with open('strings.json') as json_data:
d = json.load(json_data)
pprint(d)
The file is going to be implicitly closed already. There is no need to call json_data.close()
again.
edited Jun 7 '15 at 18:28


Corey Goldberg
37.5k22108124
37.5k22108124
answered Sep 22 '14 at 22:38
ZongjunZongjun
50947
50947
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
to pretty-print, I had to use:print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
add a comment |
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
to pretty-print, I had to use:print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
1
1
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
Please remove the json_data.close(). As mentioned, it will be called implicitly.
– Bonnie Varghese
Nov 22 '14 at 7:31
1
1
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
Thanks @BonnieVarghese for pointing out. I corrected above
– Zongjun
Dec 2 '14 at 20:28
1
1
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
@Zongjun : Please correct loads to json.load(json_data).
– Knight71
May 18 '15 at 15:28
2
2
to pretty-print, I had to use:
print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
to pretty-print, I had to use:
print(json.dumps(d,sort_keys=True,indent=2))
– Mike D
Mar 14 '16 at 22:46
add a comment |
In python 3, we can use below method.
Read from file and convert to JSON
import json
# Considering "json_list.json" is a json file
with open('json_list.json') as fd:
json_data = json.load(fd)
or
import json
json_data = json.load(open('json_list.json'))
Using with statement will automatically close the opened file descriptor.
String to JSON
import json
json_data = json.loads('{"name" : "myName", "age":24}')
add a comment |
In python 3, we can use below method.
Read from file and convert to JSON
import json
# Considering "json_list.json" is a json file
with open('json_list.json') as fd:
json_data = json.load(fd)
or
import json
json_data = json.load(open('json_list.json'))
Using with statement will automatically close the opened file descriptor.
String to JSON
import json
json_data = json.loads('{"name" : "myName", "age":24}')
add a comment |
In python 3, we can use below method.
Read from file and convert to JSON
import json
# Considering "json_list.json" is a json file
with open('json_list.json') as fd:
json_data = json.load(fd)
or
import json
json_data = json.load(open('json_list.json'))
Using with statement will automatically close the opened file descriptor.
String to JSON
import json
json_data = json.loads('{"name" : "myName", "age":24}')
In python 3, we can use below method.
Read from file and convert to JSON
import json
# Considering "json_list.json" is a json file
with open('json_list.json') as fd:
json_data = json.load(fd)
or
import json
json_data = json.load(open('json_list.json'))
Using with statement will automatically close the opened file descriptor.
String to JSON
import json
json_data = json.loads('{"name" : "myName", "age":24}')
edited Nov 27 '18 at 9:02
answered Feb 7 '17 at 10:52
Thejesh PRThejesh PR
349310
349310
add a comment |
add a comment |
To add on this, today you are able to use pandas to import json:
https://pandas.pydata.org/pandas-docs/stable/generated/pandas.read_json.html
You may want to do a careful use of the orient parameter.
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
add a comment |
To add on this, today you are able to use pandas to import json:
https://pandas.pydata.org/pandas-docs/stable/generated/pandas.read_json.html
You may want to do a careful use of the orient parameter.
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
add a comment |
To add on this, today you are able to use pandas to import json:
https://pandas.pydata.org/pandas-docs/stable/generated/pandas.read_json.html
You may want to do a careful use of the orient parameter.
To add on this, today you are able to use pandas to import json:
https://pandas.pydata.org/pandas-docs/stable/generated/pandas.read_json.html
You may want to do a careful use of the orient parameter.
answered Jan 17 '18 at 10:17
Ando JuraiAndo Jurai
4701618
4701618
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
add a comment |
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
This answer would be better if you add code examples as well as the url...
– David McCorrie
Oct 15 '18 at 11:58
add a comment |
protected by Community♦ Oct 13 '17 at 20:37
Thank you for your interest in this question.
Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
FvmHKaqGlZFOgvTlM48QQsio5LWuc
6
Are you sure that the file contains valid JSON?
– Explosion Pills
Nov 25 '13 at 17:16
1
possible duplicate of Parsing values from a JSON file in Python
– Pureferret
May 19 '15 at 9:39
See also: Read & Write example for JSON
– Martin Thoma
Mar 9 '17 at 10:50
Your file is an invalid json format. Change it to:
{"strings": [{"-name": "city", "#text": "City"}, {"-name": "phone", "#text": "Phone"}, ..., {"-name": "address", "#text": "Address"}]}
– krizex
Nov 27 '18 at 9:11