Java question on building HashMap for multiple enums
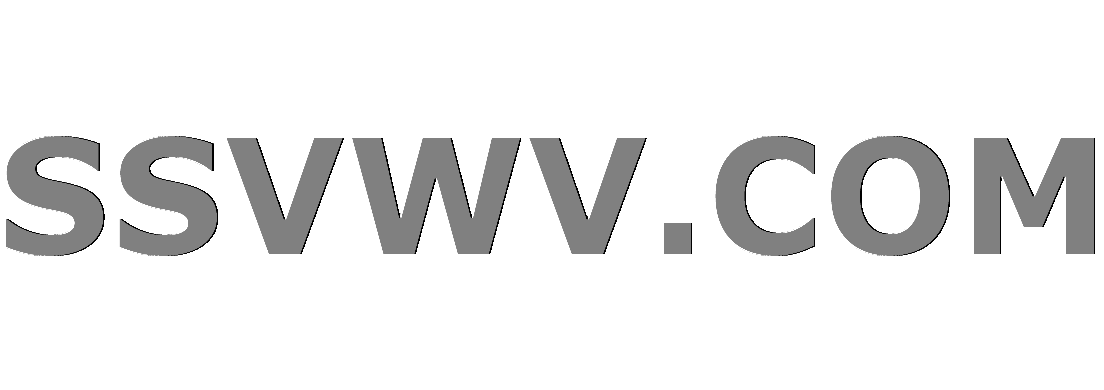
Multi tool use
I'm making an RPG game in Java for a school assignment. In the game I take user input and the first word is the "command word", so I create an enum to convert the strings for user input to enum constants:
public enum CommandWord
{
GO("go"), QUIT("quit"), HELP("help"), BACK("back"), LOOK("look"), DROP("drop"), GRAB("grab"), USE("use"), UNKNOWN("?");
private String commandString;
/*
* Initialize with the corresponding command string.
* @param commandString the command string.
*/
CommandWord(String commandString) {
this.commandString = commandString;
}
public String toString()
{
return commandString;
}
Sometimes the second word is a direction following "go" so I have a second enum for directions with more constants:
UP("up"), DOWN("down"), NORTH("north"), SOUTH("south"), EAST("east"), WEST("west"), UNKNOWN("unknown");
I'm trying to come up with the best method for building a HashMap to store strings and the related enum constants. For command words I have this class:
public class CommandWords
{
// A mapping between a command word and the CommandWord
// that is associated with it
private HashMap<String, CommandWord> validCommands;
/**
* Constructor - initialise the command words.
*/
public CommandWords()
{
validCommands = new HashMap<>();
for (CommandWord command : CommandWord.values()) {
if(command != CommandWord.UNKNOWN) {
validCommands.put(command.toString(), command);
}
}
}
/**
* Searches the HashMap of valid commands for the supplied word.
* @param commandWord The word we're searching for.
* @return The CommandWord that is mapped to the supplied string commandWord,
* or UNKNOWN if it is not in valid command.
*/
public CommandWord getCommandWord(String commandWord)
{
CommandWord command = validCommands.get(commandWord);
if (command!= null) {
return command;
}
else {
return CommandWord.UNKNOWN;
}
}
}
Then I can take userinput and search for the command word, but I can't reuse it for directions, or items, characters etc. I looked at using a generic class but I can't call methods like .values() on it, is there a good way to do this so I can reuse the CommandWords class on different enums?
java
add a comment |
I'm making an RPG game in Java for a school assignment. In the game I take user input and the first word is the "command word", so I create an enum to convert the strings for user input to enum constants:
public enum CommandWord
{
GO("go"), QUIT("quit"), HELP("help"), BACK("back"), LOOK("look"), DROP("drop"), GRAB("grab"), USE("use"), UNKNOWN("?");
private String commandString;
/*
* Initialize with the corresponding command string.
* @param commandString the command string.
*/
CommandWord(String commandString) {
this.commandString = commandString;
}
public String toString()
{
return commandString;
}
Sometimes the second word is a direction following "go" so I have a second enum for directions with more constants:
UP("up"), DOWN("down"), NORTH("north"), SOUTH("south"), EAST("east"), WEST("west"), UNKNOWN("unknown");
I'm trying to come up with the best method for building a HashMap to store strings and the related enum constants. For command words I have this class:
public class CommandWords
{
// A mapping between a command word and the CommandWord
// that is associated with it
private HashMap<String, CommandWord> validCommands;
/**
* Constructor - initialise the command words.
*/
public CommandWords()
{
validCommands = new HashMap<>();
for (CommandWord command : CommandWord.values()) {
if(command != CommandWord.UNKNOWN) {
validCommands.put(command.toString(), command);
}
}
}
/**
* Searches the HashMap of valid commands for the supplied word.
* @param commandWord The word we're searching for.
* @return The CommandWord that is mapped to the supplied string commandWord,
* or UNKNOWN if it is not in valid command.
*/
public CommandWord getCommandWord(String commandWord)
{
CommandWord command = validCommands.get(commandWord);
if (command!= null) {
return command;
}
else {
return CommandWord.UNKNOWN;
}
}
}
Then I can take userinput and search for the command word, but I can't reuse it for directions, or items, characters etc. I looked at using a generic class but I can't call methods like .values() on it, is there a good way to do this so I can reuse the CommandWords class on different enums?
java
Well, you could haveCommandWord
be an interface and let all enums implement that. Then you'd need to determine what typecommand
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you getgo down
then the first would be the command and the second would be the direction (which you know because of the "go" command).
– Thomas
Nov 26 '18 at 11:05
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21
add a comment |
I'm making an RPG game in Java for a school assignment. In the game I take user input and the first word is the "command word", so I create an enum to convert the strings for user input to enum constants:
public enum CommandWord
{
GO("go"), QUIT("quit"), HELP("help"), BACK("back"), LOOK("look"), DROP("drop"), GRAB("grab"), USE("use"), UNKNOWN("?");
private String commandString;
/*
* Initialize with the corresponding command string.
* @param commandString the command string.
*/
CommandWord(String commandString) {
this.commandString = commandString;
}
public String toString()
{
return commandString;
}
Sometimes the second word is a direction following "go" so I have a second enum for directions with more constants:
UP("up"), DOWN("down"), NORTH("north"), SOUTH("south"), EAST("east"), WEST("west"), UNKNOWN("unknown");
I'm trying to come up with the best method for building a HashMap to store strings and the related enum constants. For command words I have this class:
public class CommandWords
{
// A mapping between a command word and the CommandWord
// that is associated with it
private HashMap<String, CommandWord> validCommands;
/**
* Constructor - initialise the command words.
*/
public CommandWords()
{
validCommands = new HashMap<>();
for (CommandWord command : CommandWord.values()) {
if(command != CommandWord.UNKNOWN) {
validCommands.put(command.toString(), command);
}
}
}
/**
* Searches the HashMap of valid commands for the supplied word.
* @param commandWord The word we're searching for.
* @return The CommandWord that is mapped to the supplied string commandWord,
* or UNKNOWN if it is not in valid command.
*/
public CommandWord getCommandWord(String commandWord)
{
CommandWord command = validCommands.get(commandWord);
if (command!= null) {
return command;
}
else {
return CommandWord.UNKNOWN;
}
}
}
Then I can take userinput and search for the command word, but I can't reuse it for directions, or items, characters etc. I looked at using a generic class but I can't call methods like .values() on it, is there a good way to do this so I can reuse the CommandWords class on different enums?
java
I'm making an RPG game in Java for a school assignment. In the game I take user input and the first word is the "command word", so I create an enum to convert the strings for user input to enum constants:
public enum CommandWord
{
GO("go"), QUIT("quit"), HELP("help"), BACK("back"), LOOK("look"), DROP("drop"), GRAB("grab"), USE("use"), UNKNOWN("?");
private String commandString;
/*
* Initialize with the corresponding command string.
* @param commandString the command string.
*/
CommandWord(String commandString) {
this.commandString = commandString;
}
public String toString()
{
return commandString;
}
Sometimes the second word is a direction following "go" so I have a second enum for directions with more constants:
UP("up"), DOWN("down"), NORTH("north"), SOUTH("south"), EAST("east"), WEST("west"), UNKNOWN("unknown");
I'm trying to come up with the best method for building a HashMap to store strings and the related enum constants. For command words I have this class:
public class CommandWords
{
// A mapping between a command word and the CommandWord
// that is associated with it
private HashMap<String, CommandWord> validCommands;
/**
* Constructor - initialise the command words.
*/
public CommandWords()
{
validCommands = new HashMap<>();
for (CommandWord command : CommandWord.values()) {
if(command != CommandWord.UNKNOWN) {
validCommands.put(command.toString(), command);
}
}
}
/**
* Searches the HashMap of valid commands for the supplied word.
* @param commandWord The word we're searching for.
* @return The CommandWord that is mapped to the supplied string commandWord,
* or UNKNOWN if it is not in valid command.
*/
public CommandWord getCommandWord(String commandWord)
{
CommandWord command = validCommands.get(commandWord);
if (command!= null) {
return command;
}
else {
return CommandWord.UNKNOWN;
}
}
}
Then I can take userinput and search for the command word, but I can't reuse it for directions, or items, characters etc. I looked at using a generic class but I can't call methods like .values() on it, is there a good way to do this so I can reuse the CommandWords class on different enums?
java
java
asked Nov 26 '18 at 10:59
esperskiesperski
162
162
Well, you could haveCommandWord
be an interface and let all enums implement that. Then you'd need to determine what typecommand
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you getgo down
then the first would be the command and the second would be the direction (which you know because of the "go" command).
– Thomas
Nov 26 '18 at 11:05
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21
add a comment |
Well, you could haveCommandWord
be an interface and let all enums implement that. Then you'd need to determine what typecommand
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you getgo down
then the first would be the command and the second would be the direction (which you know because of the "go" command).
– Thomas
Nov 26 '18 at 11:05
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21
Well, you could have
CommandWord
be an interface and let all enums implement that. Then you'd need to determine what type command
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you get go down
then the first would be the command and the second would be the direction (which you know because of the "go" command).– Thomas
Nov 26 '18 at 11:05
Well, you could have
CommandWord
be an interface and let all enums implement that. Then you'd need to determine what type command
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you get go down
then the first would be the command and the second would be the direction (which you know because of the "go" command).– Thomas
Nov 26 '18 at 11:05
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21
add a comment |
1 Answer
1
active
oldest
votes
We have valueOf(String) method on Enum, you don't have to build that map.
For your case, you have a value, and you know which Enum type you would like to convert to. So, just use:
CommandWord.valueOf("QUIT");
Items.valueOf("GEM");
etc..
Enums must be determined at compile-time.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479671%2fjava-question-on-building-hashmap-for-multiple-enums%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
We have valueOf(String) method on Enum, you don't have to build that map.
For your case, you have a value, and you know which Enum type you would like to convert to. So, just use:
CommandWord.valueOf("QUIT");
Items.valueOf("GEM");
etc..
Enums must be determined at compile-time.
add a comment |
We have valueOf(String) method on Enum, you don't have to build that map.
For your case, you have a value, and you know which Enum type you would like to convert to. So, just use:
CommandWord.valueOf("QUIT");
Items.valueOf("GEM");
etc..
Enums must be determined at compile-time.
add a comment |
We have valueOf(String) method on Enum, you don't have to build that map.
For your case, you have a value, and you know which Enum type you would like to convert to. So, just use:
CommandWord.valueOf("QUIT");
Items.valueOf("GEM");
etc..
Enums must be determined at compile-time.
We have valueOf(String) method on Enum, you don't have to build that map.
For your case, you have a value, and you know which Enum type you would like to convert to. So, just use:
CommandWord.valueOf("QUIT");
Items.valueOf("GEM");
etc..
Enums must be determined at compile-time.
edited Nov 26 '18 at 11:56
answered Nov 26 '18 at 11:49


TheIronHeadTheIronHead
413
413
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53479671%2fjava-question-on-building-hashmap-for-multiple-enums%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
a1ga,p,B KGTtqS8uWLoe 7FB,0 2QLBKLUueYN5NxZGvQ7hv,pKXf7ZPg2hae0bfAnXX2 V2VXmjwJfz96680wVwTuQwqDWh w
Well, you could have
CommandWord
be an interface and let all enums implement that. Then you'd need to determine what typecommand
is or call methods that the interface defines. When doing that enums might not be the best way to implement commands but discussing that might be out of scope for this question. As an alternative you could have multiple maps that handle each individual type - after all you'd need to know the type anyways, i.e. if you getgo down
then the first would be the command and the second would be the direction (which you know because of the "go" command).– Thomas
Nov 26 '18 at 11:05
out of wonder, why you want to have sigle map for all your enums? what s your gain here?
– user902383
Nov 26 '18 at 11:14
sorry wasn't clear, I wanted to have multiple maps like <String, CommandWord> and <String, Direction> but I'm wondering if I can have a class like CommandWords that can make maps for all enums
– esperski
Nov 26 '18 at 11:21