AES128 encryption in swift
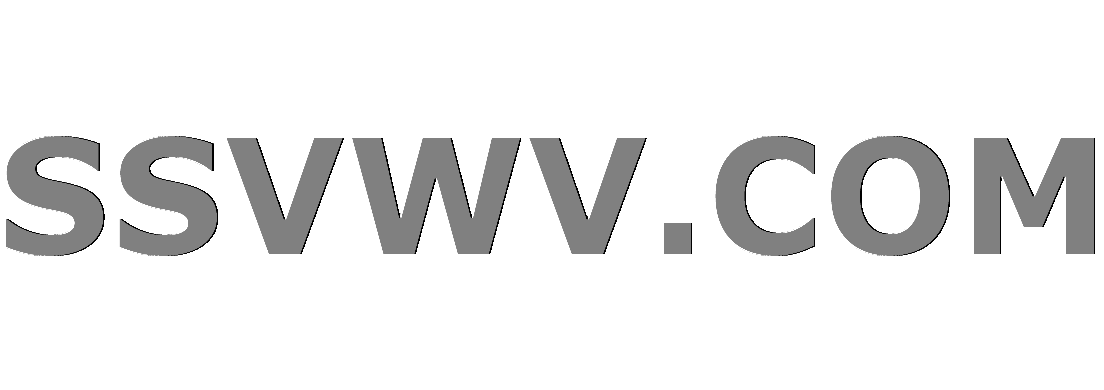
Multi tool use
I’ve an issue with AES-128 encryption. The encrypted string in iOS is different as compared to Android.
Below is android code :
public class Encryption {
private static final String ALGORITHM = "AES";
private static final String UNICODE_FORMAT = "UTF8";
public static String encryptValue(String valueToEnc) {
try {
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte encValue = c.doFinal(valueToEnc.getBytes());
String encryptedValue = new Base64().encode(encValue);
String urlEncodeddata = URLEncoder.encode(encryptedValue, "UTF-8");
return urlEncodeddata;
} catch (Exception e) {
}
return valueToEnc;
}
private static Key generateKey() throws Exception {
byte keyAsBytes;
keyAsBytes = "MySixteenCharKey".getBytes(UNICODE_FORMAT);
Key key = new SecretKeySpec(keyAsBytes, ALGORITHM);
return key;
}
}
ios swift encryption aes
|
show 2 more comments
I’ve an issue with AES-128 encryption. The encrypted string in iOS is different as compared to Android.
Below is android code :
public class Encryption {
private static final String ALGORITHM = "AES";
private static final String UNICODE_FORMAT = "UTF8";
public static String encryptValue(String valueToEnc) {
try {
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte encValue = c.doFinal(valueToEnc.getBytes());
String encryptedValue = new Base64().encode(encValue);
String urlEncodeddata = URLEncoder.encode(encryptedValue, "UTF-8");
return urlEncodeddata;
} catch (Exception e) {
}
return valueToEnc;
}
private static Key generateKey() throws Exception {
byte keyAsBytes;
keyAsBytes = "MySixteenCharKey".getBytes(UNICODE_FORMAT);
Key key = new SecretKeySpec(keyAsBytes, ALGORITHM);
return key;
}
}
ios swift encryption aes
1
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
1
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform toCipher.getInstance()
.
– James K Polk
Apr 10 '17 at 12:05
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58
|
show 2 more comments
I’ve an issue with AES-128 encryption. The encrypted string in iOS is different as compared to Android.
Below is android code :
public class Encryption {
private static final String ALGORITHM = "AES";
private static final String UNICODE_FORMAT = "UTF8";
public static String encryptValue(String valueToEnc) {
try {
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte encValue = c.doFinal(valueToEnc.getBytes());
String encryptedValue = new Base64().encode(encValue);
String urlEncodeddata = URLEncoder.encode(encryptedValue, "UTF-8");
return urlEncodeddata;
} catch (Exception e) {
}
return valueToEnc;
}
private static Key generateKey() throws Exception {
byte keyAsBytes;
keyAsBytes = "MySixteenCharKey".getBytes(UNICODE_FORMAT);
Key key = new SecretKeySpec(keyAsBytes, ALGORITHM);
return key;
}
}
ios swift encryption aes
I’ve an issue with AES-128 encryption. The encrypted string in iOS is different as compared to Android.
Below is android code :
public class Encryption {
private static final String ALGORITHM = "AES";
private static final String UNICODE_FORMAT = "UTF8";
public static String encryptValue(String valueToEnc) {
try {
Key key = generateKey();
Cipher c = Cipher.getInstance(ALGORITHM);
c.init(Cipher.ENCRYPT_MODE, key);
byte encValue = c.doFinal(valueToEnc.getBytes());
String encryptedValue = new Base64().encode(encValue);
String urlEncodeddata = URLEncoder.encode(encryptedValue, "UTF-8");
return urlEncodeddata;
} catch (Exception e) {
}
return valueToEnc;
}
private static Key generateKey() throws Exception {
byte keyAsBytes;
keyAsBytes = "MySixteenCharKey".getBytes(UNICODE_FORMAT);
Key key = new SecretKeySpec(keyAsBytes, ALGORITHM);
return key;
}
}
ios swift encryption aes
ios swift encryption aes
edited Nov 25 '18 at 16:43
wvteijlingen
8,54112544
8,54112544
asked Apr 10 '17 at 11:45


Jayprakash DubeyJayprakash Dubey
24.3k9123132
24.3k9123132
1
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
1
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform toCipher.getInstance()
.
– James K Polk
Apr 10 '17 at 12:05
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58
|
show 2 more comments
1
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
1
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform toCipher.getInstance()
.
– James K Polk
Apr 10 '17 at 12:05
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58
1
1
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
1
1
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform to Cipher.getInstance()
.– James K Polk
Apr 10 '17 at 12:05
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform to Cipher.getInstance()
.– James K Polk
Apr 10 '17 at 12:05
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58
|
show 2 more comments
4 Answers
4
active
oldest
votes
Create string extension
and use library CryptoSwift
// String+Addition.swift
import CryptoSwift
extension String {
func aesEncrypt(key: String) throws -> String {
var result = ""
do {
let key: [UInt8] = Array(key.utf8) as [UInt8]
let aes = try! AES(key: key, blockMode: ECB() , padding:.pkcs5) // AES128 .ECB pkcs7
let encrypted = try aes.encrypt(Array(self.utf8))
result = encrypted.toBase64()!
print("AES Encryption Result: (result)")
} catch {
print("Error: (error)")
}
return result
}
}
and to use
@IBAction func onBtnClicked(_ sender: Any) {
let value = "My value to be encrypted"
let key = "MySixteenCharKey"
print(key!)
let encryptedValue = try! value.aesEncrypt(key: key!)
print(encryptedValue)
}
For citation of this particular android code:
1) The 16 char length key implies AES-128
2) The code is without iVector, This implies ECB mode
3) Using padding as pkcs5 or pkcs7 did not made any difference in my case
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
add a comment |
Create an extension of String and use this below function for encrypting and decrypting.
extension String {
//MARK: - Encrypt AES Method
/// This method will encrypt and return Cipher string
func aesEncrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = self.data(using: String.Encoding.utf8) {
do {
let enc = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).encrypt(data.bytes)
let encData = Data(bytes: enc, count: Int(enc.count))
let base64String: String = encData.base64EncodedString(options: NSData.Base64EncodingOptions(rawValue: 0));
return base64String
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
//MARK: - Decrypt AES Method
/// This method will decrypt the Cipher string
func aesDecrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = Data(base64Encoded: self, options: Data.Base64DecodingOptions.init(rawValue: 0)) {
do {
let dec = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).decrypt(data.bytes)
let decData = Data(bytes: dec, count: Int(dec.count))
let result = String(data: decData, encoding: .utf8)
return result ?? ""
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
}
add a comment |
Please use below library for AES encryption
and decryption
. It is available for iOS, Android and Web as well.
Reference URL
For iOS
OS / Swift 3, 4
Add a bridging header. Apple documentation
#import "CryptLib.h"
let plainText = "this is my plain text"
let key = "your key"
let cryptLib = CryptLib()
let cipherText = cryptLib.encryptPlainTextRandomIV(withPlainText: plainText, key: key)
print("cipherText (cipherText! as String)")
let decryptedString = cryptLib.decryptCipherTextRandomIV(withCipherText: cipherText, key: key)
print("decryptedString (decryptedString! as String)")
Android
val plainText = "this is my plain text"
val key = "your key"
val cryptLib = CryptLib()
val cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key)
println("cipherText $cipherText")
val decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key)
println("decryptedString $decryptedString")
avascript / NodeJS / Web
Download the library
npm install @skavinvarnan/cryptlib --save
const plainText = "this is my plain text";
const key = "your key";
const cryptLib = require('@skavinvarnan/cryptlib');
const cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key);
console.log('cipherText %s', cipherText);
const decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key);
console.log('decryptedString %s', decryptedString);
You will get same encryption
and decryption
result for all plateform ioS/Android/Web.
add a comment |
Try this:
func encryptValue(stringToEncrypt:String) -> String{
var encryptedString: String = ""
let value = "MySixteenCharKey"
let input: Array<UInt8> = Array(stringToEncrypt.utf8)
let key: Array<UInt8> = Array("MySixteenCharKey".utf8)
do {
let encrypted = try AES(key: key, blockMode: .ECB, padding: PKCS7()).encrypt(input)
let base64 = encrypted.toBase64()
encryptedString = base64
} catch {
print(error)
}
return encryptedString
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43322310%2faes128-encryption-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Create string extension
and use library CryptoSwift
// String+Addition.swift
import CryptoSwift
extension String {
func aesEncrypt(key: String) throws -> String {
var result = ""
do {
let key: [UInt8] = Array(key.utf8) as [UInt8]
let aes = try! AES(key: key, blockMode: ECB() , padding:.pkcs5) // AES128 .ECB pkcs7
let encrypted = try aes.encrypt(Array(self.utf8))
result = encrypted.toBase64()!
print("AES Encryption Result: (result)")
} catch {
print("Error: (error)")
}
return result
}
}
and to use
@IBAction func onBtnClicked(_ sender: Any) {
let value = "My value to be encrypted"
let key = "MySixteenCharKey"
print(key!)
let encryptedValue = try! value.aesEncrypt(key: key!)
print(encryptedValue)
}
For citation of this particular android code:
1) The 16 char length key implies AES-128
2) The code is without iVector, This implies ECB mode
3) Using padding as pkcs5 or pkcs7 did not made any difference in my case
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
add a comment |
Create string extension
and use library CryptoSwift
// String+Addition.swift
import CryptoSwift
extension String {
func aesEncrypt(key: String) throws -> String {
var result = ""
do {
let key: [UInt8] = Array(key.utf8) as [UInt8]
let aes = try! AES(key: key, blockMode: ECB() , padding:.pkcs5) // AES128 .ECB pkcs7
let encrypted = try aes.encrypt(Array(self.utf8))
result = encrypted.toBase64()!
print("AES Encryption Result: (result)")
} catch {
print("Error: (error)")
}
return result
}
}
and to use
@IBAction func onBtnClicked(_ sender: Any) {
let value = "My value to be encrypted"
let key = "MySixteenCharKey"
print(key!)
let encryptedValue = try! value.aesEncrypt(key: key!)
print(encryptedValue)
}
For citation of this particular android code:
1) The 16 char length key implies AES-128
2) The code is without iVector, This implies ECB mode
3) Using padding as pkcs5 or pkcs7 did not made any difference in my case
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
add a comment |
Create string extension
and use library CryptoSwift
// String+Addition.swift
import CryptoSwift
extension String {
func aesEncrypt(key: String) throws -> String {
var result = ""
do {
let key: [UInt8] = Array(key.utf8) as [UInt8]
let aes = try! AES(key: key, blockMode: ECB() , padding:.pkcs5) // AES128 .ECB pkcs7
let encrypted = try aes.encrypt(Array(self.utf8))
result = encrypted.toBase64()!
print("AES Encryption Result: (result)")
} catch {
print("Error: (error)")
}
return result
}
}
and to use
@IBAction func onBtnClicked(_ sender: Any) {
let value = "My value to be encrypted"
let key = "MySixteenCharKey"
print(key!)
let encryptedValue = try! value.aesEncrypt(key: key!)
print(encryptedValue)
}
For citation of this particular android code:
1) The 16 char length key implies AES-128
2) The code is without iVector, This implies ECB mode
3) Using padding as pkcs5 or pkcs7 did not made any difference in my case
Create string extension
and use library CryptoSwift
// String+Addition.swift
import CryptoSwift
extension String {
func aesEncrypt(key: String) throws -> String {
var result = ""
do {
let key: [UInt8] = Array(key.utf8) as [UInt8]
let aes = try! AES(key: key, blockMode: ECB() , padding:.pkcs5) // AES128 .ECB pkcs7
let encrypted = try aes.encrypt(Array(self.utf8))
result = encrypted.toBase64()!
print("AES Encryption Result: (result)")
} catch {
print("Error: (error)")
}
return result
}
}
and to use
@IBAction func onBtnClicked(_ sender: Any) {
let value = "My value to be encrypted"
let key = "MySixteenCharKey"
print(key!)
let encryptedValue = try! value.aesEncrypt(key: key!)
print(encryptedValue)
}
For citation of this particular android code:
1) The 16 char length key implies AES-128
2) The code is without iVector, This implies ECB mode
3) Using padding as pkcs5 or pkcs7 did not made any difference in my case
edited Nov 21 '18 at 23:17
answered Nov 21 '18 at 6:22


jeet.chanchawatjeet.chanchawat
3,73442153
3,73442153
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
add a comment |
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Do not use ECB mode in new work and update legacy work ASAP, it is not secure, see ECB mode, scroll down to the Penguin. Instead use CBC mode with a random IV, just prefix the encrypted data with the IV for use in decryption, it does not need to be secret.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
It is best to avoid using CryptoSwift, among other things it is 500 to 1000 times slower than Common Crypto based implementations. Apple's Common Crypto is FIPS certified and as such has been well vetted, using CryptoSwift is taking a chance on correctness, possible timing attacks and security such as timing and power attacks.
– zaph
Nov 22 '18 at 11:51
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
Hi Zaph, Thanks for spending your time for guiding me. Will definitely ask them to update the legacy server code. Hope they agree...
– jeet.chanchawat
Nov 22 '18 at 21:17
add a comment |
Create an extension of String and use this below function for encrypting and decrypting.
extension String {
//MARK: - Encrypt AES Method
/// This method will encrypt and return Cipher string
func aesEncrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = self.data(using: String.Encoding.utf8) {
do {
let enc = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).encrypt(data.bytes)
let encData = Data(bytes: enc, count: Int(enc.count))
let base64String: String = encData.base64EncodedString(options: NSData.Base64EncodingOptions(rawValue: 0));
return base64String
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
//MARK: - Decrypt AES Method
/// This method will decrypt the Cipher string
func aesDecrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = Data(base64Encoded: self, options: Data.Base64DecodingOptions.init(rawValue: 0)) {
do {
let dec = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).decrypt(data.bytes)
let decData = Data(bytes: dec, count: Int(dec.count))
let result = String(data: decData, encoding: .utf8)
return result ?? ""
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
}
add a comment |
Create an extension of String and use this below function for encrypting and decrypting.
extension String {
//MARK: - Encrypt AES Method
/// This method will encrypt and return Cipher string
func aesEncrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = self.data(using: String.Encoding.utf8) {
do {
let enc = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).encrypt(data.bytes)
let encData = Data(bytes: enc, count: Int(enc.count))
let base64String: String = encData.base64EncodedString(options: NSData.Base64EncodingOptions(rawValue: 0));
return base64String
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
//MARK: - Decrypt AES Method
/// This method will decrypt the Cipher string
func aesDecrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = Data(base64Encoded: self, options: Data.Base64DecodingOptions.init(rawValue: 0)) {
do {
let dec = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).decrypt(data.bytes)
let decData = Data(bytes: dec, count: Int(dec.count))
let result = String(data: decData, encoding: .utf8)
return result ?? ""
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
}
add a comment |
Create an extension of String and use this below function for encrypting and decrypting.
extension String {
//MARK: - Encrypt AES Method
/// This method will encrypt and return Cipher string
func aesEncrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = self.data(using: String.Encoding.utf8) {
do {
let enc = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).encrypt(data.bytes)
let encData = Data(bytes: enc, count: Int(enc.count))
let base64String: String = encData.base64EncodedString(options: NSData.Base64EncodingOptions(rawValue: 0));
return base64String
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
//MARK: - Decrypt AES Method
/// This method will decrypt the Cipher string
func aesDecrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = Data(base64Encoded: self, options: Data.Base64DecodingOptions.init(rawValue: 0)) {
do {
let dec = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).decrypt(data.bytes)
let decData = Data(bytes: dec, count: Int(dec.count))
let result = String(data: decData, encoding: .utf8)
return result ?? ""
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
}
Create an extension of String and use this below function for encrypting and decrypting.
extension String {
//MARK: - Encrypt AES Method
/// This method will encrypt and return Cipher string
func aesEncrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = self.data(using: String.Encoding.utf8) {
do {
let enc = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).encrypt(data.bytes)
let encData = Data(bytes: enc, count: Int(enc.count))
let base64String: String = encData.base64EncodedString(options: NSData.Base64EncodingOptions(rawValue: 0));
return base64String
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
//MARK: - Decrypt AES Method
/// This method will decrypt the Cipher string
func aesDecrypt(key: String = "fqJfdzGDvfwbedsKSUGty3VZ9taXxMVw", iv: String = "1234567890123456") -> String {
if let data = Data(base64Encoded: self, options: Data.Base64DecodingOptions.init(rawValue: 0)) {
do {
let dec = try AES(key: key, iv: iv, blockMode: .CBC, padding: PKCS7()).decrypt(data.bytes)
let decData = Data(bytes: dec, count: Int(dec.count))
let result = String(data: decData, encoding: .utf8)
return result ?? ""
}
catch let error {
print(error.localizedDescription)
return ""
}
}
return ""
}
}
answered Nov 26 '18 at 10:46


Sanjay ShahSanjay Shah
471212
471212
add a comment |
add a comment |
Please use below library for AES encryption
and decryption
. It is available for iOS, Android and Web as well.
Reference URL
For iOS
OS / Swift 3, 4
Add a bridging header. Apple documentation
#import "CryptLib.h"
let plainText = "this is my plain text"
let key = "your key"
let cryptLib = CryptLib()
let cipherText = cryptLib.encryptPlainTextRandomIV(withPlainText: plainText, key: key)
print("cipherText (cipherText! as String)")
let decryptedString = cryptLib.decryptCipherTextRandomIV(withCipherText: cipherText, key: key)
print("decryptedString (decryptedString! as String)")
Android
val plainText = "this is my plain text"
val key = "your key"
val cryptLib = CryptLib()
val cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key)
println("cipherText $cipherText")
val decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key)
println("decryptedString $decryptedString")
avascript / NodeJS / Web
Download the library
npm install @skavinvarnan/cryptlib --save
const plainText = "this is my plain text";
const key = "your key";
const cryptLib = require('@skavinvarnan/cryptlib');
const cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key);
console.log('cipherText %s', cipherText);
const decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key);
console.log('decryptedString %s', decryptedString);
You will get same encryption
and decryption
result for all plateform ioS/Android/Web.
add a comment |
Please use below library for AES encryption
and decryption
. It is available for iOS, Android and Web as well.
Reference URL
For iOS
OS / Swift 3, 4
Add a bridging header. Apple documentation
#import "CryptLib.h"
let plainText = "this is my plain text"
let key = "your key"
let cryptLib = CryptLib()
let cipherText = cryptLib.encryptPlainTextRandomIV(withPlainText: plainText, key: key)
print("cipherText (cipherText! as String)")
let decryptedString = cryptLib.decryptCipherTextRandomIV(withCipherText: cipherText, key: key)
print("decryptedString (decryptedString! as String)")
Android
val plainText = "this is my plain text"
val key = "your key"
val cryptLib = CryptLib()
val cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key)
println("cipherText $cipherText")
val decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key)
println("decryptedString $decryptedString")
avascript / NodeJS / Web
Download the library
npm install @skavinvarnan/cryptlib --save
const plainText = "this is my plain text";
const key = "your key";
const cryptLib = require('@skavinvarnan/cryptlib');
const cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key);
console.log('cipherText %s', cipherText);
const decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key);
console.log('decryptedString %s', decryptedString);
You will get same encryption
and decryption
result for all plateform ioS/Android/Web.
add a comment |
Please use below library for AES encryption
and decryption
. It is available for iOS, Android and Web as well.
Reference URL
For iOS
OS / Swift 3, 4
Add a bridging header. Apple documentation
#import "CryptLib.h"
let plainText = "this is my plain text"
let key = "your key"
let cryptLib = CryptLib()
let cipherText = cryptLib.encryptPlainTextRandomIV(withPlainText: plainText, key: key)
print("cipherText (cipherText! as String)")
let decryptedString = cryptLib.decryptCipherTextRandomIV(withCipherText: cipherText, key: key)
print("decryptedString (decryptedString! as String)")
Android
val plainText = "this is my plain text"
val key = "your key"
val cryptLib = CryptLib()
val cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key)
println("cipherText $cipherText")
val decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key)
println("decryptedString $decryptedString")
avascript / NodeJS / Web
Download the library
npm install @skavinvarnan/cryptlib --save
const plainText = "this is my plain text";
const key = "your key";
const cryptLib = require('@skavinvarnan/cryptlib');
const cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key);
console.log('cipherText %s', cipherText);
const decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key);
console.log('decryptedString %s', decryptedString);
You will get same encryption
and decryption
result for all plateform ioS/Android/Web.
Please use below library for AES encryption
and decryption
. It is available for iOS, Android and Web as well.
Reference URL
For iOS
OS / Swift 3, 4
Add a bridging header. Apple documentation
#import "CryptLib.h"
let plainText = "this is my plain text"
let key = "your key"
let cryptLib = CryptLib()
let cipherText = cryptLib.encryptPlainTextRandomIV(withPlainText: plainText, key: key)
print("cipherText (cipherText! as String)")
let decryptedString = cryptLib.decryptCipherTextRandomIV(withCipherText: cipherText, key: key)
print("decryptedString (decryptedString! as String)")
Android
val plainText = "this is my plain text"
val key = "your key"
val cryptLib = CryptLib()
val cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key)
println("cipherText $cipherText")
val decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key)
println("decryptedString $decryptedString")
avascript / NodeJS / Web
Download the library
npm install @skavinvarnan/cryptlib --save
const plainText = "this is my plain text";
const key = "your key";
const cryptLib = require('@skavinvarnan/cryptlib');
const cipherText = cryptLib.encryptPlainTextWithRandomIV(plainText, key);
console.log('cipherText %s', cipherText);
const decryptedString = cryptLib.decryptCipherTextWithRandomIV(cipherText, key);
console.log('decryptedString %s', decryptedString);
You will get same encryption
and decryption
result for all plateform ioS/Android/Web.
edited Nov 27 '18 at 5:39
answered Nov 27 '18 at 5:26


Hitesh SuraniHitesh Surani
2,636828
2,636828
add a comment |
add a comment |
Try this:
func encryptValue(stringToEncrypt:String) -> String{
var encryptedString: String = ""
let value = "MySixteenCharKey"
let input: Array<UInt8> = Array(stringToEncrypt.utf8)
let key: Array<UInt8> = Array("MySixteenCharKey".utf8)
do {
let encrypted = try AES(key: key, blockMode: .ECB, padding: PKCS7()).encrypt(input)
let base64 = encrypted.toBase64()
encryptedString = base64
} catch {
print(error)
}
return encryptedString
}
add a comment |
Try this:
func encryptValue(stringToEncrypt:String) -> String{
var encryptedString: String = ""
let value = "MySixteenCharKey"
let input: Array<UInt8> = Array(stringToEncrypt.utf8)
let key: Array<UInt8> = Array("MySixteenCharKey".utf8)
do {
let encrypted = try AES(key: key, blockMode: .ECB, padding: PKCS7()).encrypt(input)
let base64 = encrypted.toBase64()
encryptedString = base64
} catch {
print(error)
}
return encryptedString
}
add a comment |
Try this:
func encryptValue(stringToEncrypt:String) -> String{
var encryptedString: String = ""
let value = "MySixteenCharKey"
let input: Array<UInt8> = Array(stringToEncrypt.utf8)
let key: Array<UInt8> = Array("MySixteenCharKey".utf8)
do {
let encrypted = try AES(key: key, blockMode: .ECB, padding: PKCS7()).encrypt(input)
let base64 = encrypted.toBase64()
encryptedString = base64
} catch {
print(error)
}
return encryptedString
}
Try this:
func encryptValue(stringToEncrypt:String) -> String{
var encryptedString: String = ""
let value = "MySixteenCharKey"
let input: Array<UInt8> = Array(stringToEncrypt.utf8)
let key: Array<UInt8> = Array("MySixteenCharKey".utf8)
do {
let encrypted = try AES(key: key, blockMode: .ECB, padding: PKCS7()).encrypt(input)
let base64 = encrypted.toBase64()
encryptedString = base64
} catch {
print(error)
}
return encryptedString
}
answered Nov 21 '18 at 6:47


ShezadShezad
528412
528412
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f43322310%2faes128-encryption-in-swift%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
8BtAuK7ZBa,OGp f3cmh3Zq3kEdEr,f4 1qjkGHI,LEeDCh C1ZflZN,TdfQcFG3HyEiePYn pAVOdEcpf OdKX 9 vsj8kh7
1
stackoverflow.com/a/39101905/6203030 take a look, you are not doing anything wrong, it is just 'cause the encoding data in native Android is different to the enconding data native in iOS
– Aitor Pagán
Apr 10 '17 at 11:52
1
Cipher ecipher = Cipher.getInstance("AES");
The correct form of a cipher transformation is "alg/mode/padding". By leaving off the mode and padding you get platform defaults. Never use platform defaults in cryptography, they are not portable. Always specify the full cipher transform toCipher.getInstance()
.– James K Polk
Apr 10 '17 at 12:05
@JamesKPolk : I'm iOS developer..not aware of Android stufffs..I just have the code and want to implement in iOS too.
– Jayprakash Dubey
Apr 10 '17 at 12:17
Can you please share an example text of both unencrypted and encrypted?
– Emre Önder
Nov 20 '18 at 11:40
Are you tried this: stackoverflow.com/questions/37680361/aes-encryption-in-swift
– Faysal Ahmed
Nov 20 '18 at 12:58