Using drools rule of one entity to invoke methods related to second entity
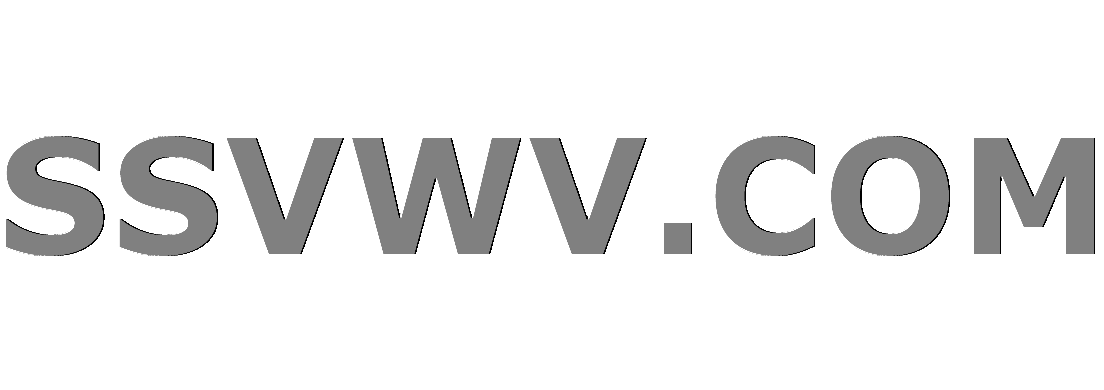
Multi tool use
Using the rules for one entity, is it possible in drools to invoke the methods related to a second entity?
Using Spring boot, I have the following Task entity.
@Entity
public class Task extends AbstractPersistable<Long> {
private String title;
private String description;
@ManyToOne
@JoinColumn(name = "assignee_group_id")
private UserGroup assigneeGroup;
@Enumerated(EnumType.STRING)
private TaskType type;
//getters and setters
}
And the UserGroup entity
@Entity
public class UserGroup extends AbstractPersistable<Long> {
private String name;
@OneToMany(targetEntity = Task.class, mappedBy = "assigneeGroup", fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private Set<Task> tasks;
//getters and setters
}
The TaskType enum is defined as
public enum TaskType {
STORE("Store"), ACCOUNTS("Accounts");
private String formattedName;
TaskType(String formattedName) {
this.formattedName = formattedName;
}
public String getFormattedName() {
return formattedName;
}
}
I also have two UserGroups Store
and Accounts
in the database. Now what I want to do is when a user creates a Task
of type Accounts
, I would like to set the assignee group as the Accounts
group, and similarly for the Task
of type Store
.
I have defined the rules as
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
System.out.println("task for store invoked");
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
System.out.println("task for accounts invoked");
end
This works perfectly fine. But now, I want to do something like
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
//find user group with name Store
//set this user group as the assignee group
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
//find user group with name Accounts
//set this user group as the assignee group
end
So far I've only found examples that either print out message in drools rule file like I've done above or invoke setters for fields of primitive data type. How can I (or is it even possible) to invoke setters for fields referring to a different entity? Please let me know if this is not even considered as best practice. I am just starting on drools so I don't have much idea here.
spring-boot drools kie
add a comment |
Using the rules for one entity, is it possible in drools to invoke the methods related to a second entity?
Using Spring boot, I have the following Task entity.
@Entity
public class Task extends AbstractPersistable<Long> {
private String title;
private String description;
@ManyToOne
@JoinColumn(name = "assignee_group_id")
private UserGroup assigneeGroup;
@Enumerated(EnumType.STRING)
private TaskType type;
//getters and setters
}
And the UserGroup entity
@Entity
public class UserGroup extends AbstractPersistable<Long> {
private String name;
@OneToMany(targetEntity = Task.class, mappedBy = "assigneeGroup", fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private Set<Task> tasks;
//getters and setters
}
The TaskType enum is defined as
public enum TaskType {
STORE("Store"), ACCOUNTS("Accounts");
private String formattedName;
TaskType(String formattedName) {
this.formattedName = formattedName;
}
public String getFormattedName() {
return formattedName;
}
}
I also have two UserGroups Store
and Accounts
in the database. Now what I want to do is when a user creates a Task
of type Accounts
, I would like to set the assignee group as the Accounts
group, and similarly for the Task
of type Store
.
I have defined the rules as
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
System.out.println("task for store invoked");
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
System.out.println("task for accounts invoked");
end
This works perfectly fine. But now, I want to do something like
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
//find user group with name Store
//set this user group as the assignee group
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
//find user group with name Accounts
//set this user group as the assignee group
end
So far I've only found examples that either print out message in drools rule file like I've done above or invoke setters for fields of primitive data type. How can I (or is it even possible) to invoke setters for fields referring to a different entity? Please let me know if this is not even considered as best practice. I am just starting on drools so I don't have much idea here.
spring-boot drools kie
add a comment |
Using the rules for one entity, is it possible in drools to invoke the methods related to a second entity?
Using Spring boot, I have the following Task entity.
@Entity
public class Task extends AbstractPersistable<Long> {
private String title;
private String description;
@ManyToOne
@JoinColumn(name = "assignee_group_id")
private UserGroup assigneeGroup;
@Enumerated(EnumType.STRING)
private TaskType type;
//getters and setters
}
And the UserGroup entity
@Entity
public class UserGroup extends AbstractPersistable<Long> {
private String name;
@OneToMany(targetEntity = Task.class, mappedBy = "assigneeGroup", fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private Set<Task> tasks;
//getters and setters
}
The TaskType enum is defined as
public enum TaskType {
STORE("Store"), ACCOUNTS("Accounts");
private String formattedName;
TaskType(String formattedName) {
this.formattedName = formattedName;
}
public String getFormattedName() {
return formattedName;
}
}
I also have two UserGroups Store
and Accounts
in the database. Now what I want to do is when a user creates a Task
of type Accounts
, I would like to set the assignee group as the Accounts
group, and similarly for the Task
of type Store
.
I have defined the rules as
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
System.out.println("task for store invoked");
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
System.out.println("task for accounts invoked");
end
This works perfectly fine. But now, I want to do something like
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
//find user group with name Store
//set this user group as the assignee group
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
//find user group with name Accounts
//set this user group as the assignee group
end
So far I've only found examples that either print out message in drools rule file like I've done above or invoke setters for fields of primitive data type. How can I (or is it even possible) to invoke setters for fields referring to a different entity? Please let me know if this is not even considered as best practice. I am just starting on drools so I don't have much idea here.
spring-boot drools kie
Using the rules for one entity, is it possible in drools to invoke the methods related to a second entity?
Using Spring boot, I have the following Task entity.
@Entity
public class Task extends AbstractPersistable<Long> {
private String title;
private String description;
@ManyToOne
@JoinColumn(name = "assignee_group_id")
private UserGroup assigneeGroup;
@Enumerated(EnumType.STRING)
private TaskType type;
//getters and setters
}
And the UserGroup entity
@Entity
public class UserGroup extends AbstractPersistable<Long> {
private String name;
@OneToMany(targetEntity = Task.class, mappedBy = "assigneeGroup", fetch = FetchType.LAZY, cascade = CascadeType.ALL)
private Set<Task> tasks;
//getters and setters
}
The TaskType enum is defined as
public enum TaskType {
STORE("Store"), ACCOUNTS("Accounts");
private String formattedName;
TaskType(String formattedName) {
this.formattedName = formattedName;
}
public String getFormattedName() {
return formattedName;
}
}
I also have two UserGroups Store
and Accounts
in the database. Now what I want to do is when a user creates a Task
of type Accounts
, I would like to set the assignee group as the Accounts
group, and similarly for the Task
of type Store
.
I have defined the rules as
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
System.out.println("task for store invoked");
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
System.out.println("task for accounts invoked");
end
This works perfectly fine. But now, I want to do something like
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store")
then
//find user group with name Store
//set this user group as the assignee group
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts")
then
//find user group with name Accounts
//set this user group as the assignee group
end
So far I've only found examples that either print out message in drools rule file like I've done above or invoke setters for fields of primitive data type. How can I (or is it even possible) to invoke setters for fields referring to a different entity? Please let me know if this is not even considered as best practice. I am just starting on drools so I don't have much idea here.
spring-boot drools kie
spring-boot drools kie
asked Nov 23 '18 at 12:18


Kshitij Bajracharya
103111
103111
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
I've solved the problem after some research. This post was really helpful. I defined a findByNameOrCreate
method in UserGroupService
that accepts the userGroup name and returns a userGroup instance. Then where I defined the kieSession
, I set a global to UserGroupService
like the following:
public Task save(Task task) {
KieSession kieSession = kieContainer.newKieSession("rulesSession");
KieRuntime kieRuntime = (KieRuntime) kieSession;
kieRuntime.setGlobal("userGroupService", userGroupService);
kieSession.insert(task);
kieSession.fireAllRules();
kieSession.dispose();
return taskRepository.save(task);
}
Then I defined the rules as
package rules
import com.packagename.domain.Task
import com.packagename.service.UserGroupService
global UserGroupService userGroupService;
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Store"));
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Accounts"));
end
Now whenever the save
method is invoked, using the name, the task is assigned to the correct userGroup.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446593%2fusing-drools-rule-of-one-entity-to-invoke-methods-related-to-second-entity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I've solved the problem after some research. This post was really helpful. I defined a findByNameOrCreate
method in UserGroupService
that accepts the userGroup name and returns a userGroup instance. Then where I defined the kieSession
, I set a global to UserGroupService
like the following:
public Task save(Task task) {
KieSession kieSession = kieContainer.newKieSession("rulesSession");
KieRuntime kieRuntime = (KieRuntime) kieSession;
kieRuntime.setGlobal("userGroupService", userGroupService);
kieSession.insert(task);
kieSession.fireAllRules();
kieSession.dispose();
return taskRepository.save(task);
}
Then I defined the rules as
package rules
import com.packagename.domain.Task
import com.packagename.service.UserGroupService
global UserGroupService userGroupService;
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Store"));
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Accounts"));
end
Now whenever the save
method is invoked, using the name, the task is assigned to the correct userGroup.
add a comment |
I've solved the problem after some research. This post was really helpful. I defined a findByNameOrCreate
method in UserGroupService
that accepts the userGroup name and returns a userGroup instance. Then where I defined the kieSession
, I set a global to UserGroupService
like the following:
public Task save(Task task) {
KieSession kieSession = kieContainer.newKieSession("rulesSession");
KieRuntime kieRuntime = (KieRuntime) kieSession;
kieRuntime.setGlobal("userGroupService", userGroupService);
kieSession.insert(task);
kieSession.fireAllRules();
kieSession.dispose();
return taskRepository.save(task);
}
Then I defined the rules as
package rules
import com.packagename.domain.Task
import com.packagename.service.UserGroupService
global UserGroupService userGroupService;
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Store"));
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Accounts"));
end
Now whenever the save
method is invoked, using the name, the task is assigned to the correct userGroup.
add a comment |
I've solved the problem after some research. This post was really helpful. I defined a findByNameOrCreate
method in UserGroupService
that accepts the userGroup name and returns a userGroup instance. Then where I defined the kieSession
, I set a global to UserGroupService
like the following:
public Task save(Task task) {
KieSession kieSession = kieContainer.newKieSession("rulesSession");
KieRuntime kieRuntime = (KieRuntime) kieSession;
kieRuntime.setGlobal("userGroupService", userGroupService);
kieSession.insert(task);
kieSession.fireAllRules();
kieSession.dispose();
return taskRepository.save(task);
}
Then I defined the rules as
package rules
import com.packagename.domain.Task
import com.packagename.service.UserGroupService
global UserGroupService userGroupService;
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Store"));
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Accounts"));
end
Now whenever the save
method is invoked, using the name, the task is assigned to the correct userGroup.
I've solved the problem after some research. This post was really helpful. I defined a findByNameOrCreate
method in UserGroupService
that accepts the userGroup name and returns a userGroup instance. Then where I defined the kieSession
, I set a global to UserGroupService
like the following:
public Task save(Task task) {
KieSession kieSession = kieContainer.newKieSession("rulesSession");
KieRuntime kieRuntime = (KieRuntime) kieSession;
kieRuntime.setGlobal("userGroupService", userGroupService);
kieSession.insert(task);
kieSession.fireAllRules();
kieSession.dispose();
return taskRepository.save(task);
}
Then I defined the rules as
package rules
import com.packagename.domain.Task
import com.packagename.service.UserGroupService
global UserGroupService userGroupService;
rule "Task for Store"
when
taskObject: Task(type.formattedName=="Store");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Store"));
end
rule "Task for Accounts"
when
taskObject: Task(type.formattedName=="Accounts");
then
taskObject.setAssigneeGroup(userGroupService.findByNameOrCreate("Accounts"));
end
Now whenever the save
method is invoked, using the name, the task is assigned to the correct userGroup.
answered Nov 26 '18 at 4:48


Kshitij Bajracharya
103111
103111
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53446593%2fusing-drools-rule-of-one-entity-to-invoke-methods-related-to-second-entity%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
5p3 NrO7ud c