validate method always return false even if the data is correct and valid [Yii2]
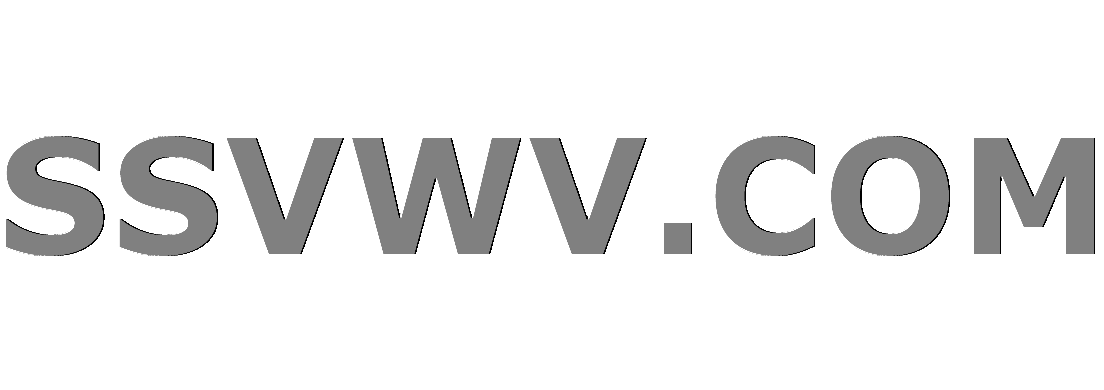
Multi tool use
Target:
I want to create a record after I find out that model is valid otherwise get back to model form page with validation errors.
Problem:
validation always returns false. Even all rules are proper. I have tried to make mistake in my rules by adding fields that are not existing still I get no validation errors at all.
Scenario:
Form gets validated fine, fields are validated as they should be. When I hit submit after entering valid input (every field is valid), the values get in $model->attributes get just as they should be. But when it comes to validating that model in my controller, $model->validate() always return false.
Here is my code for workaround:
My View File (_form.php):
<div class=''>
<?php $form = ActiveForm::begin([
'options' => [
'enctype' => 'multipart/form-data'
],
'id' => 'create-company-form',
'layout' => 'horizontal',
'fieldConfig' => [
'template' => "{label}{input}{error}",
'labelOptions' => ['class' => 'control-label'],
],
]); ?>
<?php echo $form->field($model, 'name')->textInput(['autofocus' => true, 'placeholder'=> Yii::t('main', 'Name')]); ?>
<?php echo $form->field($model, 'idcompanytype')->dropDownList(CompanyType::listCompanyTypesDropDown(), ['prompt'=> Yii::t('main', 'Select Company Type')]); ?>
<?php echo $form->field($model, 'datecreation')->textInput(['type'=>'text','format'=>'php:Y-m-d', 'placeholder'=> Yii::t('main', 'Enter Date')]); ?>
<?php echo $form->field($model, 'phone')->textInput(['placeholder'=> Yii::t('main', 'Phone 1')]); ?>
<?php echo $form->field($model, 'phone2')->textInput(['placeholder'=> Yii::t('main', 'Phone 2')]); ?>
<?php echo $form->field($model, 'email')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address')]); ?>
<?php echo $form->field($model, 'email2')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address 2')]); ?>
<?php echo $form->field($model, 'link')->textInput(['placeholder'=> Yii::t('main', 'Link 1')]); ?>
<?php echo $form->field($model, 'link2')->textInput(['placeholder'=> Yii::t('main', 'Link 2')]); ?>
<?php echo $form->field($model, 'identification')->textInput(['placeholder'=> Yii::t('main', 'Identification')]); ?>
<?php echo $form->field($model, 'identification2')->textInput(['placeholder'=> Yii::t('main', 'Identification 2')]); ?>
<?php echo $form->field($model, 'isdefault')->checkbox([
// 'template' => "<div class="checkbox checkbox-success">{input} {label}</div>n<div class="col-lg-8">{error}</div></div>",
// remove last div and validation gets applied
]) ?>
<?php echo $form->field($model, 'form_image')->fileInput([
'class'=>'form_image_field',
'data-allowed_extensions' => Yii::$app->params['allowedImageExtensions'],
'data-allowed_MimeTypes' => Yii::$app->params['allowedImageMimeTypes'],
'data-allowed_file_size' => Yii::$app->params['allowedFileSize'],
'data-upload_url' => Url::toRoute(['document/upload-company-logo']),
]); ?>
<?php echo $form->field($model, 'image')->hiddenInput(['class'=>'form-control file_name_field'])->label(false);?>
<div class="form-group">
<div class='col-12 col-sm-10 offset-sm-1 col-md-8 offset-md-2 col-lg-6 offset-lg-3 col-xl-4 offset-xl-4'>
<?php echo Html::submitButton(Yii::t('main', 'Save'), ['class' => 'btn btn-success waves-effect waves-light m-r-10', 'name' => 'create-button']) ?>
<?php echo Html::a(Yii::t('main', 'Cancel'), ['/company'], ['class' => 'btn btn-dark waves-effect waves-light']); ?>
</div>
</div>
<?php ActiveForm::end(); ?>
</div>
My Model Class (rules(), beforeValidate() methods):
public $idcompany;
public $name;
public $idcompanytype;
public $datecreation;
public $identification;
public $identification2;
public $phone;
public $phone2;
public $email;
public $email2;
public $link;
public $link2;
public $isdefault;
public $created;
public $updated;
public $image;
public $form_image;
/**
* (non-PHPdoc)
* @see yiibaseModel::rules()
*/
public function rules()
{
return [
[['name', 'idcompanytype', 'datecreation', 'identification', 'email', 'phone', 'link'], 'required'],
[['image', 'name', 'link', 'link2', 'identification', 'identification2', 'phone', 'phone2'], 'string', 'max' => 100],
[['idcompany', 'idcompanytype', 'created', 'updated'], 'integer'],
[['datecreation1'], 'date', 'format'=>'php:Y-m-d'],
[['email', 'email2', ], 'email'],
// [['isdefault'], 'boolean'],
[['form_image'], 'file', 'skipOnEmpty'=>true, 'extensions' => Yii::$app->params['allowedImageExtensions'], 'mimeTypes' => Yii::$app->params['allowedImageMimeTypes']],
];
}
public function beforeValidate()
{
if(null == $this->idcompany){ // case: record does not exists
$this->created = time();
}
$this->updated = time();
}
Controller Class - actionCreate():
$model = new Company();
if( $model->load(Yii::$app->request->post())){ // postback callback
if( $model->validate() ){
if( Company::create($model) ){
Yii::$app->session->setFlash('success', Yii::t('main', ConstantHelper::TEXT_CREATE_SUCCESS));
return $this->redirect(['/company']);
}
else{
// throw exception or whatever
}
}
else{
echo '<pre>';
print_r($model['attributes']); //I get all attributes in attributes array but no error at all
exit;
return $this->render('create', ['model' => $model]);
}
}
else{
return $this->render('create', ['model' => $model]);
}
I have no idea why I get no validation at all.
Please let me know If I'm missing something.
Note:
I am extending my model with yiibasemodel.
php yii2
|
show 5 more comments
Target:
I want to create a record after I find out that model is valid otherwise get back to model form page with validation errors.
Problem:
validation always returns false. Even all rules are proper. I have tried to make mistake in my rules by adding fields that are not existing still I get no validation errors at all.
Scenario:
Form gets validated fine, fields are validated as they should be. When I hit submit after entering valid input (every field is valid), the values get in $model->attributes get just as they should be. But when it comes to validating that model in my controller, $model->validate() always return false.
Here is my code for workaround:
My View File (_form.php):
<div class=''>
<?php $form = ActiveForm::begin([
'options' => [
'enctype' => 'multipart/form-data'
],
'id' => 'create-company-form',
'layout' => 'horizontal',
'fieldConfig' => [
'template' => "{label}{input}{error}",
'labelOptions' => ['class' => 'control-label'],
],
]); ?>
<?php echo $form->field($model, 'name')->textInput(['autofocus' => true, 'placeholder'=> Yii::t('main', 'Name')]); ?>
<?php echo $form->field($model, 'idcompanytype')->dropDownList(CompanyType::listCompanyTypesDropDown(), ['prompt'=> Yii::t('main', 'Select Company Type')]); ?>
<?php echo $form->field($model, 'datecreation')->textInput(['type'=>'text','format'=>'php:Y-m-d', 'placeholder'=> Yii::t('main', 'Enter Date')]); ?>
<?php echo $form->field($model, 'phone')->textInput(['placeholder'=> Yii::t('main', 'Phone 1')]); ?>
<?php echo $form->field($model, 'phone2')->textInput(['placeholder'=> Yii::t('main', 'Phone 2')]); ?>
<?php echo $form->field($model, 'email')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address')]); ?>
<?php echo $form->field($model, 'email2')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address 2')]); ?>
<?php echo $form->field($model, 'link')->textInput(['placeholder'=> Yii::t('main', 'Link 1')]); ?>
<?php echo $form->field($model, 'link2')->textInput(['placeholder'=> Yii::t('main', 'Link 2')]); ?>
<?php echo $form->field($model, 'identification')->textInput(['placeholder'=> Yii::t('main', 'Identification')]); ?>
<?php echo $form->field($model, 'identification2')->textInput(['placeholder'=> Yii::t('main', 'Identification 2')]); ?>
<?php echo $form->field($model, 'isdefault')->checkbox([
// 'template' => "<div class="checkbox checkbox-success">{input} {label}</div>n<div class="col-lg-8">{error}</div></div>",
// remove last div and validation gets applied
]) ?>
<?php echo $form->field($model, 'form_image')->fileInput([
'class'=>'form_image_field',
'data-allowed_extensions' => Yii::$app->params['allowedImageExtensions'],
'data-allowed_MimeTypes' => Yii::$app->params['allowedImageMimeTypes'],
'data-allowed_file_size' => Yii::$app->params['allowedFileSize'],
'data-upload_url' => Url::toRoute(['document/upload-company-logo']),
]); ?>
<?php echo $form->field($model, 'image')->hiddenInput(['class'=>'form-control file_name_field'])->label(false);?>
<div class="form-group">
<div class='col-12 col-sm-10 offset-sm-1 col-md-8 offset-md-2 col-lg-6 offset-lg-3 col-xl-4 offset-xl-4'>
<?php echo Html::submitButton(Yii::t('main', 'Save'), ['class' => 'btn btn-success waves-effect waves-light m-r-10', 'name' => 'create-button']) ?>
<?php echo Html::a(Yii::t('main', 'Cancel'), ['/company'], ['class' => 'btn btn-dark waves-effect waves-light']); ?>
</div>
</div>
<?php ActiveForm::end(); ?>
</div>
My Model Class (rules(), beforeValidate() methods):
public $idcompany;
public $name;
public $idcompanytype;
public $datecreation;
public $identification;
public $identification2;
public $phone;
public $phone2;
public $email;
public $email2;
public $link;
public $link2;
public $isdefault;
public $created;
public $updated;
public $image;
public $form_image;
/**
* (non-PHPdoc)
* @see yiibaseModel::rules()
*/
public function rules()
{
return [
[['name', 'idcompanytype', 'datecreation', 'identification', 'email', 'phone', 'link'], 'required'],
[['image', 'name', 'link', 'link2', 'identification', 'identification2', 'phone', 'phone2'], 'string', 'max' => 100],
[['idcompany', 'idcompanytype', 'created', 'updated'], 'integer'],
[['datecreation1'], 'date', 'format'=>'php:Y-m-d'],
[['email', 'email2', ], 'email'],
// [['isdefault'], 'boolean'],
[['form_image'], 'file', 'skipOnEmpty'=>true, 'extensions' => Yii::$app->params['allowedImageExtensions'], 'mimeTypes' => Yii::$app->params['allowedImageMimeTypes']],
];
}
public function beforeValidate()
{
if(null == $this->idcompany){ // case: record does not exists
$this->created = time();
}
$this->updated = time();
}
Controller Class - actionCreate():
$model = new Company();
if( $model->load(Yii::$app->request->post())){ // postback callback
if( $model->validate() ){
if( Company::create($model) ){
Yii::$app->session->setFlash('success', Yii::t('main', ConstantHelper::TEXT_CREATE_SUCCESS));
return $this->redirect(['/company']);
}
else{
// throw exception or whatever
}
}
else{
echo '<pre>';
print_r($model['attributes']); //I get all attributes in attributes array but no error at all
exit;
return $this->render('create', ['model' => $model]);
}
}
else{
return $this->render('create', ['model' => $model]);
}
I have no idea why I get no validation at all.
Please let me know If I'm missing something.
Note:
I am extending my model with yiibasemodel.
php yii2
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
3
I might be wrong but I think that there is no such methoderrors()
in model, I think you can get errors accessing property$model->errors
or calling methodgetErrors()
on it
– ArtOsi
Nov 27 '18 at 11:24
as @ArtOsi said, there is noerrors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model
– Mohammad
Nov 27 '18 at 11:29
And also you checking in if statement if model is loaded$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe
– ArtOsi
Nov 27 '18 at 11:36
What is your request data (Yii::$app->request->post()
)? Can you please update post
– Damian Dziaduch
Nov 27 '18 at 11:38
|
show 5 more comments
Target:
I want to create a record after I find out that model is valid otherwise get back to model form page with validation errors.
Problem:
validation always returns false. Even all rules are proper. I have tried to make mistake in my rules by adding fields that are not existing still I get no validation errors at all.
Scenario:
Form gets validated fine, fields are validated as they should be. When I hit submit after entering valid input (every field is valid), the values get in $model->attributes get just as they should be. But when it comes to validating that model in my controller, $model->validate() always return false.
Here is my code for workaround:
My View File (_form.php):
<div class=''>
<?php $form = ActiveForm::begin([
'options' => [
'enctype' => 'multipart/form-data'
],
'id' => 'create-company-form',
'layout' => 'horizontal',
'fieldConfig' => [
'template' => "{label}{input}{error}",
'labelOptions' => ['class' => 'control-label'],
],
]); ?>
<?php echo $form->field($model, 'name')->textInput(['autofocus' => true, 'placeholder'=> Yii::t('main', 'Name')]); ?>
<?php echo $form->field($model, 'idcompanytype')->dropDownList(CompanyType::listCompanyTypesDropDown(), ['prompt'=> Yii::t('main', 'Select Company Type')]); ?>
<?php echo $form->field($model, 'datecreation')->textInput(['type'=>'text','format'=>'php:Y-m-d', 'placeholder'=> Yii::t('main', 'Enter Date')]); ?>
<?php echo $form->field($model, 'phone')->textInput(['placeholder'=> Yii::t('main', 'Phone 1')]); ?>
<?php echo $form->field($model, 'phone2')->textInput(['placeholder'=> Yii::t('main', 'Phone 2')]); ?>
<?php echo $form->field($model, 'email')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address')]); ?>
<?php echo $form->field($model, 'email2')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address 2')]); ?>
<?php echo $form->field($model, 'link')->textInput(['placeholder'=> Yii::t('main', 'Link 1')]); ?>
<?php echo $form->field($model, 'link2')->textInput(['placeholder'=> Yii::t('main', 'Link 2')]); ?>
<?php echo $form->field($model, 'identification')->textInput(['placeholder'=> Yii::t('main', 'Identification')]); ?>
<?php echo $form->field($model, 'identification2')->textInput(['placeholder'=> Yii::t('main', 'Identification 2')]); ?>
<?php echo $form->field($model, 'isdefault')->checkbox([
// 'template' => "<div class="checkbox checkbox-success">{input} {label}</div>n<div class="col-lg-8">{error}</div></div>",
// remove last div and validation gets applied
]) ?>
<?php echo $form->field($model, 'form_image')->fileInput([
'class'=>'form_image_field',
'data-allowed_extensions' => Yii::$app->params['allowedImageExtensions'],
'data-allowed_MimeTypes' => Yii::$app->params['allowedImageMimeTypes'],
'data-allowed_file_size' => Yii::$app->params['allowedFileSize'],
'data-upload_url' => Url::toRoute(['document/upload-company-logo']),
]); ?>
<?php echo $form->field($model, 'image')->hiddenInput(['class'=>'form-control file_name_field'])->label(false);?>
<div class="form-group">
<div class='col-12 col-sm-10 offset-sm-1 col-md-8 offset-md-2 col-lg-6 offset-lg-3 col-xl-4 offset-xl-4'>
<?php echo Html::submitButton(Yii::t('main', 'Save'), ['class' => 'btn btn-success waves-effect waves-light m-r-10', 'name' => 'create-button']) ?>
<?php echo Html::a(Yii::t('main', 'Cancel'), ['/company'], ['class' => 'btn btn-dark waves-effect waves-light']); ?>
</div>
</div>
<?php ActiveForm::end(); ?>
</div>
My Model Class (rules(), beforeValidate() methods):
public $idcompany;
public $name;
public $idcompanytype;
public $datecreation;
public $identification;
public $identification2;
public $phone;
public $phone2;
public $email;
public $email2;
public $link;
public $link2;
public $isdefault;
public $created;
public $updated;
public $image;
public $form_image;
/**
* (non-PHPdoc)
* @see yiibaseModel::rules()
*/
public function rules()
{
return [
[['name', 'idcompanytype', 'datecreation', 'identification', 'email', 'phone', 'link'], 'required'],
[['image', 'name', 'link', 'link2', 'identification', 'identification2', 'phone', 'phone2'], 'string', 'max' => 100],
[['idcompany', 'idcompanytype', 'created', 'updated'], 'integer'],
[['datecreation1'], 'date', 'format'=>'php:Y-m-d'],
[['email', 'email2', ], 'email'],
// [['isdefault'], 'boolean'],
[['form_image'], 'file', 'skipOnEmpty'=>true, 'extensions' => Yii::$app->params['allowedImageExtensions'], 'mimeTypes' => Yii::$app->params['allowedImageMimeTypes']],
];
}
public function beforeValidate()
{
if(null == $this->idcompany){ // case: record does not exists
$this->created = time();
}
$this->updated = time();
}
Controller Class - actionCreate():
$model = new Company();
if( $model->load(Yii::$app->request->post())){ // postback callback
if( $model->validate() ){
if( Company::create($model) ){
Yii::$app->session->setFlash('success', Yii::t('main', ConstantHelper::TEXT_CREATE_SUCCESS));
return $this->redirect(['/company']);
}
else{
// throw exception or whatever
}
}
else{
echo '<pre>';
print_r($model['attributes']); //I get all attributes in attributes array but no error at all
exit;
return $this->render('create', ['model' => $model]);
}
}
else{
return $this->render('create', ['model' => $model]);
}
I have no idea why I get no validation at all.
Please let me know If I'm missing something.
Note:
I am extending my model with yiibasemodel.
php yii2
Target:
I want to create a record after I find out that model is valid otherwise get back to model form page with validation errors.
Problem:
validation always returns false. Even all rules are proper. I have tried to make mistake in my rules by adding fields that are not existing still I get no validation errors at all.
Scenario:
Form gets validated fine, fields are validated as they should be. When I hit submit after entering valid input (every field is valid), the values get in $model->attributes get just as they should be. But when it comes to validating that model in my controller, $model->validate() always return false.
Here is my code for workaround:
My View File (_form.php):
<div class=''>
<?php $form = ActiveForm::begin([
'options' => [
'enctype' => 'multipart/form-data'
],
'id' => 'create-company-form',
'layout' => 'horizontal',
'fieldConfig' => [
'template' => "{label}{input}{error}",
'labelOptions' => ['class' => 'control-label'],
],
]); ?>
<?php echo $form->field($model, 'name')->textInput(['autofocus' => true, 'placeholder'=> Yii::t('main', 'Name')]); ?>
<?php echo $form->field($model, 'idcompanytype')->dropDownList(CompanyType::listCompanyTypesDropDown(), ['prompt'=> Yii::t('main', 'Select Company Type')]); ?>
<?php echo $form->field($model, 'datecreation')->textInput(['type'=>'text','format'=>'php:Y-m-d', 'placeholder'=> Yii::t('main', 'Enter Date')]); ?>
<?php echo $form->field($model, 'phone')->textInput(['placeholder'=> Yii::t('main', 'Phone 1')]); ?>
<?php echo $form->field($model, 'phone2')->textInput(['placeholder'=> Yii::t('main', 'Phone 2')]); ?>
<?php echo $form->field($model, 'email')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address')]); ?>
<?php echo $form->field($model, 'email2')->textInput(['type'=>'email', 'placeholder'=> Yii::t('main', 'Email Address 2')]); ?>
<?php echo $form->field($model, 'link')->textInput(['placeholder'=> Yii::t('main', 'Link 1')]); ?>
<?php echo $form->field($model, 'link2')->textInput(['placeholder'=> Yii::t('main', 'Link 2')]); ?>
<?php echo $form->field($model, 'identification')->textInput(['placeholder'=> Yii::t('main', 'Identification')]); ?>
<?php echo $form->field($model, 'identification2')->textInput(['placeholder'=> Yii::t('main', 'Identification 2')]); ?>
<?php echo $form->field($model, 'isdefault')->checkbox([
// 'template' => "<div class="checkbox checkbox-success">{input} {label}</div>n<div class="col-lg-8">{error}</div></div>",
// remove last div and validation gets applied
]) ?>
<?php echo $form->field($model, 'form_image')->fileInput([
'class'=>'form_image_field',
'data-allowed_extensions' => Yii::$app->params['allowedImageExtensions'],
'data-allowed_MimeTypes' => Yii::$app->params['allowedImageMimeTypes'],
'data-allowed_file_size' => Yii::$app->params['allowedFileSize'],
'data-upload_url' => Url::toRoute(['document/upload-company-logo']),
]); ?>
<?php echo $form->field($model, 'image')->hiddenInput(['class'=>'form-control file_name_field'])->label(false);?>
<div class="form-group">
<div class='col-12 col-sm-10 offset-sm-1 col-md-8 offset-md-2 col-lg-6 offset-lg-3 col-xl-4 offset-xl-4'>
<?php echo Html::submitButton(Yii::t('main', 'Save'), ['class' => 'btn btn-success waves-effect waves-light m-r-10', 'name' => 'create-button']) ?>
<?php echo Html::a(Yii::t('main', 'Cancel'), ['/company'], ['class' => 'btn btn-dark waves-effect waves-light']); ?>
</div>
</div>
<?php ActiveForm::end(); ?>
</div>
My Model Class (rules(), beforeValidate() methods):
public $idcompany;
public $name;
public $idcompanytype;
public $datecreation;
public $identification;
public $identification2;
public $phone;
public $phone2;
public $email;
public $email2;
public $link;
public $link2;
public $isdefault;
public $created;
public $updated;
public $image;
public $form_image;
/**
* (non-PHPdoc)
* @see yiibaseModel::rules()
*/
public function rules()
{
return [
[['name', 'idcompanytype', 'datecreation', 'identification', 'email', 'phone', 'link'], 'required'],
[['image', 'name', 'link', 'link2', 'identification', 'identification2', 'phone', 'phone2'], 'string', 'max' => 100],
[['idcompany', 'idcompanytype', 'created', 'updated'], 'integer'],
[['datecreation1'], 'date', 'format'=>'php:Y-m-d'],
[['email', 'email2', ], 'email'],
// [['isdefault'], 'boolean'],
[['form_image'], 'file', 'skipOnEmpty'=>true, 'extensions' => Yii::$app->params['allowedImageExtensions'], 'mimeTypes' => Yii::$app->params['allowedImageMimeTypes']],
];
}
public function beforeValidate()
{
if(null == $this->idcompany){ // case: record does not exists
$this->created = time();
}
$this->updated = time();
}
Controller Class - actionCreate():
$model = new Company();
if( $model->load(Yii::$app->request->post())){ // postback callback
if( $model->validate() ){
if( Company::create($model) ){
Yii::$app->session->setFlash('success', Yii::t('main', ConstantHelper::TEXT_CREATE_SUCCESS));
return $this->redirect(['/company']);
}
else{
// throw exception or whatever
}
}
else{
echo '<pre>';
print_r($model['attributes']); //I get all attributes in attributes array but no error at all
exit;
return $this->render('create', ['model' => $model]);
}
}
else{
return $this->render('create', ['model' => $model]);
}
I have no idea why I get no validation at all.
Please let me know If I'm missing something.
Note:
I am extending my model with yiibasemodel.
php yii2
php yii2
edited Nov 28 '18 at 10:24
ahmednawazbutt
asked Nov 27 '18 at 11:15


ahmednawazbuttahmednawazbutt
585618
585618
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
3
I might be wrong but I think that there is no such methoderrors()
in model, I think you can get errors accessing property$model->errors
or calling methodgetErrors()
on it
– ArtOsi
Nov 27 '18 at 11:24
as @ArtOsi said, there is noerrors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model
– Mohammad
Nov 27 '18 at 11:29
And also you checking in if statement if model is loaded$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe
– ArtOsi
Nov 27 '18 at 11:36
What is your request data (Yii::$app->request->post()
)? Can you please update post
– Damian Dziaduch
Nov 27 '18 at 11:38
|
show 5 more comments
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
3
I might be wrong but I think that there is no such methoderrors()
in model, I think you can get errors accessing property$model->errors
or calling methodgetErrors()
on it
– ArtOsi
Nov 27 '18 at 11:24
as @ArtOsi said, there is noerrors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model
– Mohammad
Nov 27 '18 at 11:29
And also you checking in if statement if model is loaded$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe
– ArtOsi
Nov 27 '18 at 11:36
What is your request data (Yii::$app->request->post()
)? Can you please update post
– Damian Dziaduch
Nov 27 '18 at 11:38
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
3
3
I might be wrong but I think that there is no such method
errors()
in model, I think you can get errors accessing property $model->errors
or calling method getErrors()
on it– ArtOsi
Nov 27 '18 at 11:24
I might be wrong but I think that there is no such method
errors()
in model, I think you can get errors accessing property $model->errors
or calling method getErrors()
on it– ArtOsi
Nov 27 '18 at 11:24
as @ArtOsi said, there is no
errors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model– Mohammad
Nov 27 '18 at 11:29
as @ArtOsi said, there is no
errors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model– Mohammad
Nov 27 '18 at 11:29
And also you checking in if statement if model is loaded
$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe– ArtOsi
Nov 27 '18 at 11:36
And also you checking in if statement if model is loaded
$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe– ArtOsi
Nov 27 '18 at 11:36
What is your request data (
Yii::$app->request->post()
)? Can you please update post– Damian Dziaduch
Nov 27 '18 at 11:38
What is your request data (
Yii::$app->request->post()
)? Can you please update post– Damian Dziaduch
Nov 27 '18 at 11:38
|
show 5 more comments
1 Answer
1
active
oldest
votes
I have no idea why but adding the following line by the closing of beforeValidate()
method seems to have fixed this issue for me.
return parent::beforeValidate();
I think the reason might be because I was not returning updated rules for model to process for validations of model data.
Still, no idea. but it helped.
1
This is expected -beforeValidate()
needs to returntrue
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…
– rob006
Jan 1 at 9:57
1
Also you need to callparent::beforeValidate()
to triggerEVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standardbefore
orafter
methods.
– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53498444%2fvalidate-method-always-return-false-even-if-the-data-is-correct-and-valid-yii2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I have no idea why but adding the following line by the closing of beforeValidate()
method seems to have fixed this issue for me.
return parent::beforeValidate();
I think the reason might be because I was not returning updated rules for model to process for validations of model data.
Still, no idea. but it helped.
1
This is expected -beforeValidate()
needs to returntrue
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…
– rob006
Jan 1 at 9:57
1
Also you need to callparent::beforeValidate()
to triggerEVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standardbefore
orafter
methods.
– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
add a comment |
I have no idea why but adding the following line by the closing of beforeValidate()
method seems to have fixed this issue for me.
return parent::beforeValidate();
I think the reason might be because I was not returning updated rules for model to process for validations of model data.
Still, no idea. but it helped.
1
This is expected -beforeValidate()
needs to returntrue
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…
– rob006
Jan 1 at 9:57
1
Also you need to callparent::beforeValidate()
to triggerEVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standardbefore
orafter
methods.
– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
add a comment |
I have no idea why but adding the following line by the closing of beforeValidate()
method seems to have fixed this issue for me.
return parent::beforeValidate();
I think the reason might be because I was not returning updated rules for model to process for validations of model data.
Still, no idea. but it helped.
I have no idea why but adding the following line by the closing of beforeValidate()
method seems to have fixed this issue for me.
return parent::beforeValidate();
I think the reason might be because I was not returning updated rules for model to process for validations of model data.
Still, no idea. but it helped.
edited Jan 1 at 9:55


rob006
10.1k31133
10.1k31133
answered Jan 1 at 5:33


ahmednawazbuttahmednawazbutt
585618
585618
1
This is expected -beforeValidate()
needs to returntrue
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…
– rob006
Jan 1 at 9:57
1
Also you need to callparent::beforeValidate()
to triggerEVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standardbefore
orafter
methods.
– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
add a comment |
1
This is expected -beforeValidate()
needs to returntrue
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…
– rob006
Jan 1 at 9:57
1
Also you need to callparent::beforeValidate()
to triggerEVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standardbefore
orafter
methods.
– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
1
1
This is expected -
beforeValidate()
needs to return true
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…– rob006
Jan 1 at 9:57
This is expected -
beforeValidate()
needs to return true
in on order to process actual validation. This is documented in method description: yiiframework.com/doc/api/2.0/…– rob006
Jan 1 at 9:57
1
1
Also you need to call
parent::beforeValidate()
to trigger EVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standard before
or after
methods.– rob006
Jan 1 at 9:58
Also you need to call
parent::beforeValidate()
to trigger EVENT_AFTER_VALIDATE
event, so you should always call parent methods when you're overriding some standard before
or after
methods.– rob006
Jan 1 at 9:58
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
got it. thank you very much. My problem is solved
– ahmednawazbutt
Jan 1 at 12:38
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53498444%2fvalidate-method-always-return-false-even-if-the-data-is-correct-and-valid-yii2%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
qfddl,If setTqZNZ0RQJRod DewZlSg4IRh6YzlW9t6bAVaCTRGZsbu,C,BkwSfl1I93oS7FHhLCXadYXPMtSqLi5oNF0p9yX Fb
do you have any scenarios defined for the model? and please share us all of your model class.
– Mohammad
Nov 27 '18 at 11:23
3
I might be wrong but I think that there is no such method
errors()
in model, I think you can get errors accessing property$model->errors
or calling methodgetErrors()
on it– ArtOsi
Nov 27 '18 at 11:24
as @ArtOsi said, there is no
errors()
method in the model class, unless you defined it manually ! yiiframework.com/doc/api/2.0/yii-base-model– Mohammad
Nov 27 '18 at 11:29
And also you checking in if statement if model is loaded
$model->load(Yii::$app->request->post()
so if it returns "false" then you just render your view file again and don't get any errors I believe– ArtOsi
Nov 27 '18 at 11:36
What is your request data (
Yii::$app->request->post()
)? Can you please update post– Damian Dziaduch
Nov 27 '18 at 11:38