C# JSON deserialize into complex class
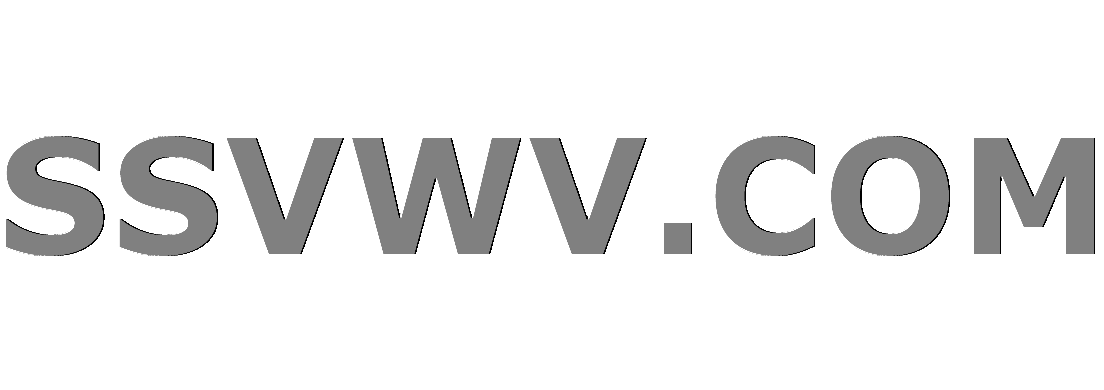
Multi tool use
I have a JSON like this
{
"Application": [
{
"Office": "London",
"LogPath": [
"\\server1filepath\"
]
},
{
"Office": "Paris",
"LogPath": [
"\\server2\logpath1\",
"\\server2\logpath2\"
]
}
],
"MailSettings": {
"MailTo": "mymail@mydomain.it",
"MailSubject" : "Log Checker"
}
}
and i have create a custom class to read the json content :
public class RootObject
{
public Application Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public List<Offices> Offices { get; set; }
}
public class Offices
{
public string Office{ get; set; }
public IList<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
But when i try to deserialize the json with
RootObject rootObject = JsonConvert.DeserializeObject<RootObject>(json);
I return the error :
the type requires a JSON object (e.g. {"name":"value"}) to deserialize correctly.
Which is wrong with my custom class ?
javascript c# json serialization json.net
add a comment |
I have a JSON like this
{
"Application": [
{
"Office": "London",
"LogPath": [
"\\server1filepath\"
]
},
{
"Office": "Paris",
"LogPath": [
"\\server2\logpath1\",
"\\server2\logpath2\"
]
}
],
"MailSettings": {
"MailTo": "mymail@mydomain.it",
"MailSubject" : "Log Checker"
}
}
and i have create a custom class to read the json content :
public class RootObject
{
public Application Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public List<Offices> Offices { get; set; }
}
public class Offices
{
public string Office{ get; set; }
public IList<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
But when i try to deserialize the json with
RootObject rootObject = JsonConvert.DeserializeObject<RootObject>(json);
I return the error :
the type requires a JSON object (e.g. {"name":"value"}) to deserialize correctly.
Which is wrong with my custom class ?
javascript c# json serialization json.net
Your JSON does not match your class structure. For example, your JSON has an array ofApplication
properties while your class structure has oneApplication
which has aList<String> offices
.
– jAC
Nov 27 '18 at 11:15
add a comment |
I have a JSON like this
{
"Application": [
{
"Office": "London",
"LogPath": [
"\\server1filepath\"
]
},
{
"Office": "Paris",
"LogPath": [
"\\server2\logpath1\",
"\\server2\logpath2\"
]
}
],
"MailSettings": {
"MailTo": "mymail@mydomain.it",
"MailSubject" : "Log Checker"
}
}
and i have create a custom class to read the json content :
public class RootObject
{
public Application Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public List<Offices> Offices { get; set; }
}
public class Offices
{
public string Office{ get; set; }
public IList<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
But when i try to deserialize the json with
RootObject rootObject = JsonConvert.DeserializeObject<RootObject>(json);
I return the error :
the type requires a JSON object (e.g. {"name":"value"}) to deserialize correctly.
Which is wrong with my custom class ?
javascript c# json serialization json.net
I have a JSON like this
{
"Application": [
{
"Office": "London",
"LogPath": [
"\\server1filepath\"
]
},
{
"Office": "Paris",
"LogPath": [
"\\server2\logpath1\",
"\\server2\logpath2\"
]
}
],
"MailSettings": {
"MailTo": "mymail@mydomain.it",
"MailSubject" : "Log Checker"
}
}
and i have create a custom class to read the json content :
public class RootObject
{
public Application Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public List<Offices> Offices { get; set; }
}
public class Offices
{
public string Office{ get; set; }
public IList<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
But when i try to deserialize the json with
RootObject rootObject = JsonConvert.DeserializeObject<RootObject>(json);
I return the error :
the type requires a JSON object (e.g. {"name":"value"}) to deserialize correctly.
Which is wrong with my custom class ?
javascript c# json serialization json.net
javascript c# json serialization json.net
asked Nov 27 '18 at 11:09


lpernicelpernice
4018
4018
Your JSON does not match your class structure. For example, your JSON has an array ofApplication
properties while your class structure has oneApplication
which has aList<String> offices
.
– jAC
Nov 27 '18 at 11:15
add a comment |
Your JSON does not match your class structure. For example, your JSON has an array ofApplication
properties while your class structure has oneApplication
which has aList<String> offices
.
– jAC
Nov 27 '18 at 11:15
Your JSON does not match your class structure. For example, your JSON has an array of
Application
properties while your class structure has one Application
which has a List<String> offices
.– jAC
Nov 27 '18 at 11:15
Your JSON does not match your class structure. For example, your JSON has an array of
Application
properties while your class structure has one Application
which has a List<String> offices
.– jAC
Nov 27 '18 at 11:15
add a comment |
4 Answers
4
active
oldest
votes
Your custom classes should be like it.
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
add a comment |
You can generate types using json2csharp - to save hand-crafting a representation of the JSON object.
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
Using Visual Studio "Paste JSON as Classes" gives you the equally functional, but slightly different:
public class Rootobject
{
public Application Application { get; set; }
public Mailsettings MailSettings { get; set; }
}
public class Mailsettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class Application
{
public string Office { get; set; }
public string LogPath { get; set; }
}
Visual Studio Code users can get the same feature using the JSON as Code extension, powered by QuickType.
add a comment |
Copy your json string and use visual studio inbuild feature to create custom class for respective json.
- Goto Edit -> Special Paste -> Paste JSON as Class
This will create custom class for you.
Now using Newtonsoft.Json library, you can deserialize your json.
add a comment |
I tested your code.your problem is in your RootObject model.
In your RootObject you have a collection of application not single application.
i changed it and it wrok correctly.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53498345%2fc-sharp-json-deserialize-into-complex-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your custom classes should be like it.
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
add a comment |
Your custom classes should be like it.
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
add a comment |
Your custom classes should be like it.
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
Your custom classes should be like it.
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
answered Nov 27 '18 at 11:17
OfficalMesutOfficalMesut
327413
327413
add a comment |
add a comment |
You can generate types using json2csharp - to save hand-crafting a representation of the JSON object.
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
Using Visual Studio "Paste JSON as Classes" gives you the equally functional, but slightly different:
public class Rootobject
{
public Application Application { get; set; }
public Mailsettings MailSettings { get; set; }
}
public class Mailsettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class Application
{
public string Office { get; set; }
public string LogPath { get; set; }
}
Visual Studio Code users can get the same feature using the JSON as Code extension, powered by QuickType.
add a comment |
You can generate types using json2csharp - to save hand-crafting a representation of the JSON object.
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
Using Visual Studio "Paste JSON as Classes" gives you the equally functional, but slightly different:
public class Rootobject
{
public Application Application { get; set; }
public Mailsettings MailSettings { get; set; }
}
public class Mailsettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class Application
{
public string Office { get; set; }
public string LogPath { get; set; }
}
Visual Studio Code users can get the same feature using the JSON as Code extension, powered by QuickType.
add a comment |
You can generate types using json2csharp - to save hand-crafting a representation of the JSON object.
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
Using Visual Studio "Paste JSON as Classes" gives you the equally functional, but slightly different:
public class Rootobject
{
public Application Application { get; set; }
public Mailsettings MailSettings { get; set; }
}
public class Mailsettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class Application
{
public string Office { get; set; }
public string LogPath { get; set; }
}
Visual Studio Code users can get the same feature using the JSON as Code extension, powered by QuickType.
You can generate types using json2csharp - to save hand-crafting a representation of the JSON object.
public class RootObject
{
public List<Application> Application { get; set; }
public MailSettings MailSettings { get; set; }
}
public class Application
{
public string Office { get; set; }
public List<string> LogPath { get; set; }
}
public class MailSettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
Using Visual Studio "Paste JSON as Classes" gives you the equally functional, but slightly different:
public class Rootobject
{
public Application Application { get; set; }
public Mailsettings MailSettings { get; set; }
}
public class Mailsettings
{
public string MailTo { get; set; }
public string MailSubject { get; set; }
}
public class Application
{
public string Office { get; set; }
public string LogPath { get; set; }
}
Visual Studio Code users can get the same feature using the JSON as Code extension, powered by QuickType.
edited Nov 27 '18 at 11:28
answered Nov 27 '18 at 11:20


FentonFenton
155k44291315
155k44291315
add a comment |
add a comment |
Copy your json string and use visual studio inbuild feature to create custom class for respective json.
- Goto Edit -> Special Paste -> Paste JSON as Class
This will create custom class for you.
Now using Newtonsoft.Json library, you can deserialize your json.
add a comment |
Copy your json string and use visual studio inbuild feature to create custom class for respective json.
- Goto Edit -> Special Paste -> Paste JSON as Class
This will create custom class for you.
Now using Newtonsoft.Json library, you can deserialize your json.
add a comment |
Copy your json string and use visual studio inbuild feature to create custom class for respective json.
- Goto Edit -> Special Paste -> Paste JSON as Class
This will create custom class for you.
Now using Newtonsoft.Json library, you can deserialize your json.
Copy your json string and use visual studio inbuild feature to create custom class for respective json.
- Goto Edit -> Special Paste -> Paste JSON as Class
This will create custom class for you.
Now using Newtonsoft.Json library, you can deserialize your json.
answered Nov 27 '18 at 11:22


Prasad TelkikarPrasad Telkikar
2,030419
2,030419
add a comment |
add a comment |
I tested your code.your problem is in your RootObject model.
In your RootObject you have a collection of application not single application.
i changed it and it wrok correctly.
add a comment |
I tested your code.your problem is in your RootObject model.
In your RootObject you have a collection of application not single application.
i changed it and it wrok correctly.
add a comment |
I tested your code.your problem is in your RootObject model.
In your RootObject you have a collection of application not single application.
i changed it and it wrok correctly.
I tested your code.your problem is in your RootObject model.
In your RootObject you have a collection of application not single application.
i changed it and it wrok correctly.
answered Nov 27 '18 at 11:36
MRMFMRMF
357
357
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53498345%2fc-sharp-json-deserialize-into-complex-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
kp0NUlLKEee,4bmf,zXdgsPN pw,zOea2aT,6So 3e mZepB,gb776Tcza6BDYP9J0xYphpawOYBi
Your JSON does not match your class structure. For example, your JSON has an array of
Application
properties while your class structure has oneApplication
which has aList<String> offices
.– jAC
Nov 27 '18 at 11:15