Overloading of a non-member operator for a templated inner class
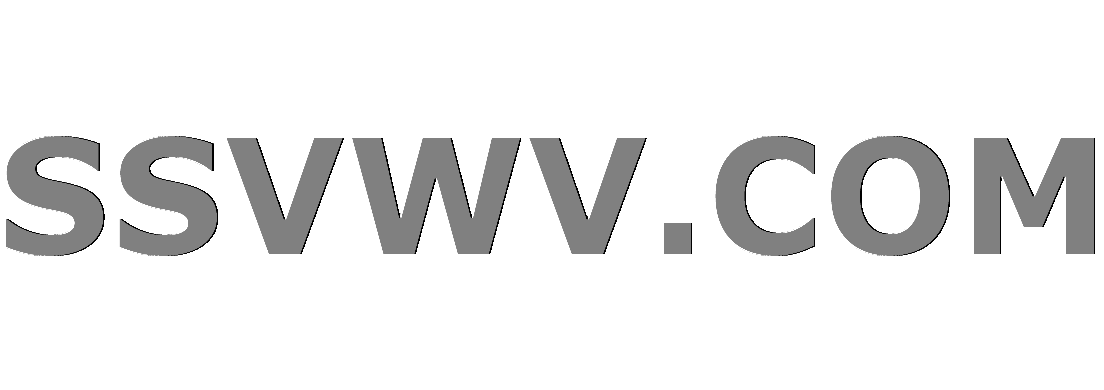
Multi tool use
I cannot figure out why the operator<< ( std::ostream & os, typename A<T>::B const& b )
can't be seen or used by the compiler.
#include <vector>
#include <iostream>
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
};
std::vector<B> outervec;
};
template <typename T>
auto operator<< ( std::ostream & os, typename A<T>::B const& b ) -> std::ostream&
{
for (auto e : b.innvervec)
os << "nt" << e;
return os;
}
template <typename T>
auto operator<< ( std::ostream & os, A<T> const& a ) -> std::ostream&
{
for (auto e : a.outervec)
os << 'n' << e;
return os;
}
int main()
{
A<int> a;
A<int>::B b1;
A<int>::B b2;
b1.innervec.push_back( 11 );
b1.innervec.push_back( 12 );
b2.innervec.push_back( 21 );
b2.innervec.push_back( 22 );
a.outervec.push_back( b1 );
a.outervec.push_back( b2 );
std::cout << a << std::endl;
}
gives me the following error on VC++15:
error C2679: binary '<<': no operator found which takes a right-hand operand of type 'A<int>::B' (or there is no acceptable conversion)
And it doesn't compile on GCC as well (only tried with an online compiler though).
I suppose that a rule about scope and deduction is involved, but I couldn't find out which precisely.
c++ templates
add a comment |
I cannot figure out why the operator<< ( std::ostream & os, typename A<T>::B const& b )
can't be seen or used by the compiler.
#include <vector>
#include <iostream>
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
};
std::vector<B> outervec;
};
template <typename T>
auto operator<< ( std::ostream & os, typename A<T>::B const& b ) -> std::ostream&
{
for (auto e : b.innvervec)
os << "nt" << e;
return os;
}
template <typename T>
auto operator<< ( std::ostream & os, A<T> const& a ) -> std::ostream&
{
for (auto e : a.outervec)
os << 'n' << e;
return os;
}
int main()
{
A<int> a;
A<int>::B b1;
A<int>::B b2;
b1.innervec.push_back( 11 );
b1.innervec.push_back( 12 );
b2.innervec.push_back( 21 );
b2.innervec.push_back( 22 );
a.outervec.push_back( b1 );
a.outervec.push_back( b2 );
std::cout << a << std::endl;
}
gives me the following error on VC++15:
error C2679: binary '<<': no operator found which takes a right-hand operand of type 'A<int>::B' (or there is no acceptable conversion)
And it doesn't compile on GCC as well (only tried with an online compiler though).
I suppose that a rule about scope and deduction is involved, but I couldn't find out which precisely.
c++ templates
add a comment |
I cannot figure out why the operator<< ( std::ostream & os, typename A<T>::B const& b )
can't be seen or used by the compiler.
#include <vector>
#include <iostream>
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
};
std::vector<B> outervec;
};
template <typename T>
auto operator<< ( std::ostream & os, typename A<T>::B const& b ) -> std::ostream&
{
for (auto e : b.innvervec)
os << "nt" << e;
return os;
}
template <typename T>
auto operator<< ( std::ostream & os, A<T> const& a ) -> std::ostream&
{
for (auto e : a.outervec)
os << 'n' << e;
return os;
}
int main()
{
A<int> a;
A<int>::B b1;
A<int>::B b2;
b1.innervec.push_back( 11 );
b1.innervec.push_back( 12 );
b2.innervec.push_back( 21 );
b2.innervec.push_back( 22 );
a.outervec.push_back( b1 );
a.outervec.push_back( b2 );
std::cout << a << std::endl;
}
gives me the following error on VC++15:
error C2679: binary '<<': no operator found which takes a right-hand operand of type 'A<int>::B' (or there is no acceptable conversion)
And it doesn't compile on GCC as well (only tried with an online compiler though).
I suppose that a rule about scope and deduction is involved, but I couldn't find out which precisely.
c++ templates
I cannot figure out why the operator<< ( std::ostream & os, typename A<T>::B const& b )
can't be seen or used by the compiler.
#include <vector>
#include <iostream>
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
};
std::vector<B> outervec;
};
template <typename T>
auto operator<< ( std::ostream & os, typename A<T>::B const& b ) -> std::ostream&
{
for (auto e : b.innvervec)
os << "nt" << e;
return os;
}
template <typename T>
auto operator<< ( std::ostream & os, A<T> const& a ) -> std::ostream&
{
for (auto e : a.outervec)
os << 'n' << e;
return os;
}
int main()
{
A<int> a;
A<int>::B b1;
A<int>::B b2;
b1.innervec.push_back( 11 );
b1.innervec.push_back( 12 );
b2.innervec.push_back( 21 );
b2.innervec.push_back( 22 );
a.outervec.push_back( b1 );
a.outervec.push_back( b2 );
std::cout << a << std::endl;
}
gives me the following error on VC++15:
error C2679: binary '<<': no operator found which takes a right-hand operand of type 'A<int>::B' (or there is no acceptable conversion)
And it doesn't compile on GCC as well (only tried with an online compiler though).
I suppose that a rule about scope and deduction is involved, but I couldn't find out which precisely.
c++ templates
c++ templates
asked Nov 25 '18 at 19:51


TarquiscaniTarquiscani
12119
12119
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
The error is because the overload for the nested type contain a non-deduced context parameter typename A<T>::B const& b
and for operators you can't provide the explicit template argument T
, you need to define the operator as friend for the nested A<T>::B
as:
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
friend auto operator<< ( std::ostream & os, B const& b ) -> std::ostream&
{
for (auto e : b.innervec)
os << "nt" << e;
return os;
}
};
std::vector<B> outervec;
};
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
add a comment |
The problematic part is A<T>::B
in the parameter list, as the compiler cannot deduce type expressions of this form, since existence and type of member B
depends on T
. I would take the type of b
argument as template argument T
directly, and use SFINAE to constrain accepted types.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471270%2foverloading-of-a-non-member-operator-for-a-templated-inner-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The error is because the overload for the nested type contain a non-deduced context parameter typename A<T>::B const& b
and for operators you can't provide the explicit template argument T
, you need to define the operator as friend for the nested A<T>::B
as:
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
friend auto operator<< ( std::ostream & os, B const& b ) -> std::ostream&
{
for (auto e : b.innervec)
os << "nt" << e;
return os;
}
};
std::vector<B> outervec;
};
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
add a comment |
The error is because the overload for the nested type contain a non-deduced context parameter typename A<T>::B const& b
and for operators you can't provide the explicit template argument T
, you need to define the operator as friend for the nested A<T>::B
as:
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
friend auto operator<< ( std::ostream & os, B const& b ) -> std::ostream&
{
for (auto e : b.innervec)
os << "nt" << e;
return os;
}
};
std::vector<B> outervec;
};
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
add a comment |
The error is because the overload for the nested type contain a non-deduced context parameter typename A<T>::B const& b
and for operators you can't provide the explicit template argument T
, you need to define the operator as friend for the nested A<T>::B
as:
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
friend auto operator<< ( std::ostream & os, B const& b ) -> std::ostream&
{
for (auto e : b.innervec)
os << "nt" << e;
return os;
}
};
std::vector<B> outervec;
};
The error is because the overload for the nested type contain a non-deduced context parameter typename A<T>::B const& b
and for operators you can't provide the explicit template argument T
, you need to define the operator as friend for the nested A<T>::B
as:
template <typename T>
struct A
{
struct B
{
std::vector<T> innervec;
friend auto operator<< ( std::ostream & os, B const& b ) -> std::ostream&
{
for (auto e : b.innervec)
os << "nt" << e;
return os;
}
};
std::vector<B> outervec;
};
edited Nov 25 '18 at 20:02
answered Nov 25 '18 at 19:57
JansJans
8,89422635
8,89422635
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
add a comment |
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
No need for friendship, just for membership
– Géza Török
Nov 25 '18 at 20:05
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
Thanks :) I didn't know about non-deduced contexts.
– Tarquiscani
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
@GézaTörök stream operators can't be members.
– Jans
Nov 25 '18 at 20:09
add a comment |
The problematic part is A<T>::B
in the parameter list, as the compiler cannot deduce type expressions of this form, since existence and type of member B
depends on T
. I would take the type of b
argument as template argument T
directly, and use SFINAE to constrain accepted types.
add a comment |
The problematic part is A<T>::B
in the parameter list, as the compiler cannot deduce type expressions of this form, since existence and type of member B
depends on T
. I would take the type of b
argument as template argument T
directly, and use SFINAE to constrain accepted types.
add a comment |
The problematic part is A<T>::B
in the parameter list, as the compiler cannot deduce type expressions of this form, since existence and type of member B
depends on T
. I would take the type of b
argument as template argument T
directly, and use SFINAE to constrain accepted types.
The problematic part is A<T>::B
in the parameter list, as the compiler cannot deduce type expressions of this form, since existence and type of member B
depends on T
. I would take the type of b
argument as template argument T
directly, and use SFINAE to constrain accepted types.
edited Nov 25 '18 at 20:18
answered Nov 25 '18 at 20:00
Géza TörökGéza Török
1,222515
1,222515
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53471270%2foverloading-of-a-non-member-operator-for-a-templated-inner-class%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UU1jX qK2YGVxV,dNDwZVSk8x,gjPdx yrdo