Execute line of code n times without initializing a variable
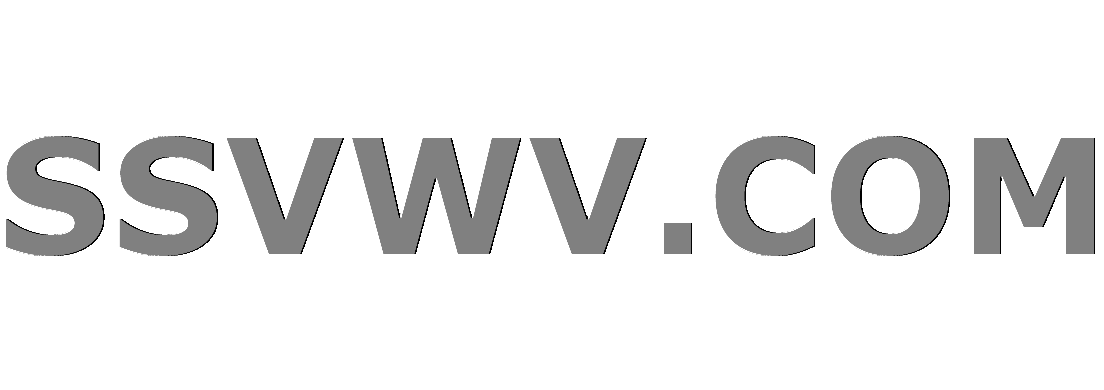
Multi tool use
I'm new to swift. I want to add 100 random integers to an array. I have the following working code:
var integers = [Int]()
for i in 1...100 {
integers.append((Int.random(in: 0 ..< 100)))
}
The compiler warns me that I did not use i
inside the scope of the for loop, which is indeed a sensible warning. Is there a way to do some line n
times without declaring a variable which I won't use anyway?
swift
add a comment |
I'm new to swift. I want to add 100 random integers to an array. I have the following working code:
var integers = [Int]()
for i in 1...100 {
integers.append((Int.random(in: 0 ..< 100)))
}
The compiler warns me that I did not use i
inside the scope of the for loop, which is indeed a sensible warning. Is there a way to do some line n
times without declaring a variable which I won't use anyway?
swift
add a comment |
I'm new to swift. I want to add 100 random integers to an array. I have the following working code:
var integers = [Int]()
for i in 1...100 {
integers.append((Int.random(in: 0 ..< 100)))
}
The compiler warns me that I did not use i
inside the scope of the for loop, which is indeed a sensible warning. Is there a way to do some line n
times without declaring a variable which I won't use anyway?
swift
I'm new to swift. I want to add 100 random integers to an array. I have the following working code:
var integers = [Int]()
for i in 1...100 {
integers.append((Int.random(in: 0 ..< 100)))
}
The compiler warns me that I did not use i
inside the scope of the for loop, which is indeed a sensible warning. Is there a way to do some line n
times without declaring a variable which I won't use anyway?
swift
swift
asked Nov 25 '18 at 15:15
K AbdulahiK Abdulahi
82
82
add a comment |
add a comment |
3 Answers
3
active
oldest
votes
Change the i
with _
.
_
is just a way of saying that you don't need a variable here. It is known as the "wildcard pattern" in the swift documentation:
A wildcard pattern matches and ignores any value and consists of an
underscore (_). Use a wildcard pattern when you don’t care about the
values being matched against. For example, the following code iterates
through the closed range 1...3, ignoring the current value of the
range on each iteration of the loop:
for _ in 1...3 {
// Do something three times.
}
add a comment |
Change i
to _
. The use of the underscore is a way to tell the Swift compiler that you don't care about the variable or return value.
You can find this in the Swift book in the Control Flow chapter under For-In Loops.
add a comment |
You could do it like so:
let integers = (1...100).map { _ in Int.random(in: 0..<100) }
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468895%2fexecute-line-of-code-n-times-without-initializing-a-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Change the i
with _
.
_
is just a way of saying that you don't need a variable here. It is known as the "wildcard pattern" in the swift documentation:
A wildcard pattern matches and ignores any value and consists of an
underscore (_). Use a wildcard pattern when you don’t care about the
values being matched against. For example, the following code iterates
through the closed range 1...3, ignoring the current value of the
range on each iteration of the loop:
for _ in 1...3 {
// Do something three times.
}
add a comment |
Change the i
with _
.
_
is just a way of saying that you don't need a variable here. It is known as the "wildcard pattern" in the swift documentation:
A wildcard pattern matches and ignores any value and consists of an
underscore (_). Use a wildcard pattern when you don’t care about the
values being matched against. For example, the following code iterates
through the closed range 1...3, ignoring the current value of the
range on each iteration of the loop:
for _ in 1...3 {
// Do something three times.
}
add a comment |
Change the i
with _
.
_
is just a way of saying that you don't need a variable here. It is known as the "wildcard pattern" in the swift documentation:
A wildcard pattern matches and ignores any value and consists of an
underscore (_). Use a wildcard pattern when you don’t care about the
values being matched against. For example, the following code iterates
through the closed range 1...3, ignoring the current value of the
range on each iteration of the loop:
for _ in 1...3 {
// Do something three times.
}
Change the i
with _
.
_
is just a way of saying that you don't need a variable here. It is known as the "wildcard pattern" in the swift documentation:
A wildcard pattern matches and ignores any value and consists of an
underscore (_). Use a wildcard pattern when you don’t care about the
values being matched against. For example, the following code iterates
through the closed range 1...3, ignoring the current value of the
range on each iteration of the loop:
for _ in 1...3 {
// Do something three times.
}
answered Nov 25 '18 at 15:19


SweeperSweeper
66.3k1073139
66.3k1073139
add a comment |
add a comment |
Change i
to _
. The use of the underscore is a way to tell the Swift compiler that you don't care about the variable or return value.
You can find this in the Swift book in the Control Flow chapter under For-In Loops.
add a comment |
Change i
to _
. The use of the underscore is a way to tell the Swift compiler that you don't care about the variable or return value.
You can find this in the Swift book in the Control Flow chapter under For-In Loops.
add a comment |
Change i
to _
. The use of the underscore is a way to tell the Swift compiler that you don't care about the variable or return value.
You can find this in the Swift book in the Control Flow chapter under For-In Loops.
Change i
to _
. The use of the underscore is a way to tell the Swift compiler that you don't care about the variable or return value.
You can find this in the Swift book in the Control Flow chapter under For-In Loops.
answered Nov 25 '18 at 15:17


rmaddyrmaddy
241k27316380
241k27316380
add a comment |
add a comment |
You could do it like so:
let integers = (1...100).map { _ in Int.random(in: 0..<100) }
add a comment |
You could do it like so:
let integers = (1...100).map { _ in Int.random(in: 0..<100) }
add a comment |
You could do it like so:
let integers = (1...100).map { _ in Int.random(in: 0..<100) }
You could do it like so:
let integers = (1...100).map { _ in Int.random(in: 0..<100) }
edited Nov 25 '18 at 15:34
answered Nov 25 '18 at 15:21


Carpsen90Carpsen90
7,49762760
7,49762760
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53468895%2fexecute-line-of-code-n-times-without-initializing-a-variable%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
fQtIl3aAd3JlLgqHEZ dDzJxj,3Mld