Communication between gSOAP client and Java webservice over HTTPS
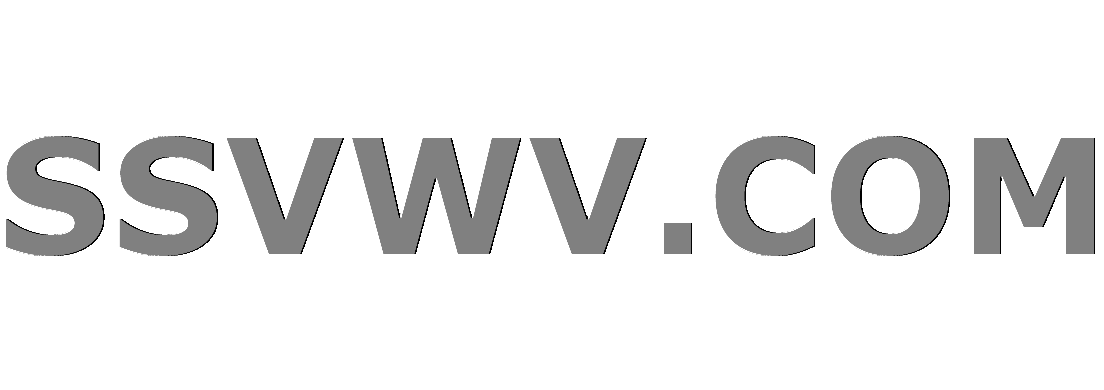
Multi tool use
I'm developing a client server pair, where the server is based on Java, and the client is based on C++ (gSOAP). The communication works perfectly with using HTTP. Now I want to implement an encrypted communication based on HTTPS.
Therefore I followed the gSOAP tutorial on
https://www.genivia.com/tutorials.html#cert
To create self signed certificates: one for the client, one for the webservice.
Then I converted the .pem files using OpenSSL as you find here:
openssl pkcs12 -export -in Servicecert.pem -inkey Servicekey.pem -certfile cacert.pem -out Service -name "service"
Additionally I exported the x.509 Client certificate like this:
openssl x509 -outform der -in Clientcert.pem -out Clientcert.der
keytool -import -alias client -keystore Client -file Clientcert.der
These two files I'm using as keystore (Service) and truststore (Client)
Now using gSOAP sample code on the client side like this:
using namespace std;
int main()
{
struct soap* soap = soap_new();
soap->fsslverify = ssl_verify;
soap_register_plugin(soap, soap_wsse);
CountriesPortSoap11Proxy service("https://localhost:9443/ws");// = new CountriesPortSoap11Proxy("https://localhost:9443/ws");
_ns1__getCountryRequest* request = new _ns1__getCountryRequest;
_ns1__getCountryResponse response;
soap_ssl_init();
if (soap_ssl_client_context(soap,
SOAP_SSL_ALLOW_EXPIRED_CERTIFICATE, // requires server authentication
"Client.pem",// keyfile for client authentication to server
"password", // the keyfile password
"cacert.pem",// cafile CA certificates to authenticate the server
NULL,// capath CA directory path to certificates
NULL))
{
cout << "Zertifikat" << endl;
soap_print_fault(soap, stderr);
exit(1);
}
request->name = "Poland";
request->soap = soap;
if (soap_ssl_accept((struct soap*)soap))
cout << "ok" << endl;
else
cout << "fail" << endl;;
if(service.getCountry(request, response)==SOAP_OK)
cout << "ok" << endl;
else{
cout << "fail" << endl;
service.soap_stream_fault(std::cerr);
}
cout << response.country->currency << endl;
return 0;
}
Then I get the following error code
ok
fail
SOAP 1.1 fault SOAP-ENV:Server[no subcode]
"SSL_ERROR_SSL
error:1416F086:SSL routines:tls_process_server_certificate:certificate verify failed
unable to get local issuer certificate"
Detail: SSL_connect() error in tcp_connect()
segmentation fault (Speicherabzug geschrieben)
Does someone know what is going wrong here?
java https gsoap
add a comment |
I'm developing a client server pair, where the server is based on Java, and the client is based on C++ (gSOAP). The communication works perfectly with using HTTP. Now I want to implement an encrypted communication based on HTTPS.
Therefore I followed the gSOAP tutorial on
https://www.genivia.com/tutorials.html#cert
To create self signed certificates: one for the client, one for the webservice.
Then I converted the .pem files using OpenSSL as you find here:
openssl pkcs12 -export -in Servicecert.pem -inkey Servicekey.pem -certfile cacert.pem -out Service -name "service"
Additionally I exported the x.509 Client certificate like this:
openssl x509 -outform der -in Clientcert.pem -out Clientcert.der
keytool -import -alias client -keystore Client -file Clientcert.der
These two files I'm using as keystore (Service) and truststore (Client)
Now using gSOAP sample code on the client side like this:
using namespace std;
int main()
{
struct soap* soap = soap_new();
soap->fsslverify = ssl_verify;
soap_register_plugin(soap, soap_wsse);
CountriesPortSoap11Proxy service("https://localhost:9443/ws");// = new CountriesPortSoap11Proxy("https://localhost:9443/ws");
_ns1__getCountryRequest* request = new _ns1__getCountryRequest;
_ns1__getCountryResponse response;
soap_ssl_init();
if (soap_ssl_client_context(soap,
SOAP_SSL_ALLOW_EXPIRED_CERTIFICATE, // requires server authentication
"Client.pem",// keyfile for client authentication to server
"password", // the keyfile password
"cacert.pem",// cafile CA certificates to authenticate the server
NULL,// capath CA directory path to certificates
NULL))
{
cout << "Zertifikat" << endl;
soap_print_fault(soap, stderr);
exit(1);
}
request->name = "Poland";
request->soap = soap;
if (soap_ssl_accept((struct soap*)soap))
cout << "ok" << endl;
else
cout << "fail" << endl;;
if(service.getCountry(request, response)==SOAP_OK)
cout << "ok" << endl;
else{
cout << "fail" << endl;
service.soap_stream_fault(std::cerr);
}
cout << response.country->currency << endl;
return 0;
}
Then I get the following error code
ok
fail
SOAP 1.1 fault SOAP-ENV:Server[no subcode]
"SSL_ERROR_SSL
error:1416F086:SSL routines:tls_process_server_certificate:certificate verify failed
unable to get local issuer certificate"
Detail: SSL_connect() error in tcp_connect()
segmentation fault (Speicherabzug geschrieben)
Does someone know what is going wrong here?
java https gsoap
add a comment |
I'm developing a client server pair, where the server is based on Java, and the client is based on C++ (gSOAP). The communication works perfectly with using HTTP. Now I want to implement an encrypted communication based on HTTPS.
Therefore I followed the gSOAP tutorial on
https://www.genivia.com/tutorials.html#cert
To create self signed certificates: one for the client, one for the webservice.
Then I converted the .pem files using OpenSSL as you find here:
openssl pkcs12 -export -in Servicecert.pem -inkey Servicekey.pem -certfile cacert.pem -out Service -name "service"
Additionally I exported the x.509 Client certificate like this:
openssl x509 -outform der -in Clientcert.pem -out Clientcert.der
keytool -import -alias client -keystore Client -file Clientcert.der
These two files I'm using as keystore (Service) and truststore (Client)
Now using gSOAP sample code on the client side like this:
using namespace std;
int main()
{
struct soap* soap = soap_new();
soap->fsslverify = ssl_verify;
soap_register_plugin(soap, soap_wsse);
CountriesPortSoap11Proxy service("https://localhost:9443/ws");// = new CountriesPortSoap11Proxy("https://localhost:9443/ws");
_ns1__getCountryRequest* request = new _ns1__getCountryRequest;
_ns1__getCountryResponse response;
soap_ssl_init();
if (soap_ssl_client_context(soap,
SOAP_SSL_ALLOW_EXPIRED_CERTIFICATE, // requires server authentication
"Client.pem",// keyfile for client authentication to server
"password", // the keyfile password
"cacert.pem",// cafile CA certificates to authenticate the server
NULL,// capath CA directory path to certificates
NULL))
{
cout << "Zertifikat" << endl;
soap_print_fault(soap, stderr);
exit(1);
}
request->name = "Poland";
request->soap = soap;
if (soap_ssl_accept((struct soap*)soap))
cout << "ok" << endl;
else
cout << "fail" << endl;;
if(service.getCountry(request, response)==SOAP_OK)
cout << "ok" << endl;
else{
cout << "fail" << endl;
service.soap_stream_fault(std::cerr);
}
cout << response.country->currency << endl;
return 0;
}
Then I get the following error code
ok
fail
SOAP 1.1 fault SOAP-ENV:Server[no subcode]
"SSL_ERROR_SSL
error:1416F086:SSL routines:tls_process_server_certificate:certificate verify failed
unable to get local issuer certificate"
Detail: SSL_connect() error in tcp_connect()
segmentation fault (Speicherabzug geschrieben)
Does someone know what is going wrong here?
java https gsoap
I'm developing a client server pair, where the server is based on Java, and the client is based on C++ (gSOAP). The communication works perfectly with using HTTP. Now I want to implement an encrypted communication based on HTTPS.
Therefore I followed the gSOAP tutorial on
https://www.genivia.com/tutorials.html#cert
To create self signed certificates: one for the client, one for the webservice.
Then I converted the .pem files using OpenSSL as you find here:
openssl pkcs12 -export -in Servicecert.pem -inkey Servicekey.pem -certfile cacert.pem -out Service -name "service"
Additionally I exported the x.509 Client certificate like this:
openssl x509 -outform der -in Clientcert.pem -out Clientcert.der
keytool -import -alias client -keystore Client -file Clientcert.der
These two files I'm using as keystore (Service) and truststore (Client)
Now using gSOAP sample code on the client side like this:
using namespace std;
int main()
{
struct soap* soap = soap_new();
soap->fsslverify = ssl_verify;
soap_register_plugin(soap, soap_wsse);
CountriesPortSoap11Proxy service("https://localhost:9443/ws");// = new CountriesPortSoap11Proxy("https://localhost:9443/ws");
_ns1__getCountryRequest* request = new _ns1__getCountryRequest;
_ns1__getCountryResponse response;
soap_ssl_init();
if (soap_ssl_client_context(soap,
SOAP_SSL_ALLOW_EXPIRED_CERTIFICATE, // requires server authentication
"Client.pem",// keyfile for client authentication to server
"password", // the keyfile password
"cacert.pem",// cafile CA certificates to authenticate the server
NULL,// capath CA directory path to certificates
NULL))
{
cout << "Zertifikat" << endl;
soap_print_fault(soap, stderr);
exit(1);
}
request->name = "Poland";
request->soap = soap;
if (soap_ssl_accept((struct soap*)soap))
cout << "ok" << endl;
else
cout << "fail" << endl;;
if(service.getCountry(request, response)==SOAP_OK)
cout << "ok" << endl;
else{
cout << "fail" << endl;
service.soap_stream_fault(std::cerr);
}
cout << response.country->currency << endl;
return 0;
}
Then I get the following error code
ok
fail
SOAP 1.1 fault SOAP-ENV:Server[no subcode]
"SSL_ERROR_SSL
error:1416F086:SSL routines:tls_process_server_certificate:certificate verify failed
unable to get local issuer certificate"
Detail: SSL_connect() error in tcp_connect()
segmentation fault (Speicherabzug geschrieben)
Does someone know what is going wrong here?
java https gsoap
java https gsoap
edited Nov 25 '18 at 15:09


Zoe
11.5k74379
11.5k74379
asked Oct 23 '18 at 11:58


Pall FallPall Fall
12
12
add a comment |
add a comment |
2 Answers
2
active
oldest
votes
Not sure why you had to convert the PEM files as you described. The cert.sh
script generates the PEM key file and PEM certificate file. This script can be used for server side to generate server.pem
and cacert.pem
. The cert.sh
script is located in gsoap/samples/ssl
in the gSOAP software package. The cacert.pem
file is the server's certificate, signed by the root (root.pem
created with the root.sh
script). This cacert.pem
file is the only file needed at the client side to verify the authenticity of the server using the self-signed certificate. Use the server.pem
file at the server side. The client.pem
is not needed, unless you enforce client authentication at the server side explicitly with SOAP_SSL_REQUIRE_CLIENT_AUTHENTICATION
to initialize the SSL context with soap_ssl_server_context
. The server.pem
file is actually a concatenation of the server's private key and its certificate, when created by cert.sh
.
add a comment |
I had to convert the PEM files to use them in the java webservice which uses the keytool. This keytool only understands PKCS12 certificates as far as I know. Additionally I had to use the Client certificates, because the Server uses the Client x.509 certificate in a truststore.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52948525%2fcommunication-between-gsoap-client-and-java-webservice-over-https%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Not sure why you had to convert the PEM files as you described. The cert.sh
script generates the PEM key file and PEM certificate file. This script can be used for server side to generate server.pem
and cacert.pem
. The cert.sh
script is located in gsoap/samples/ssl
in the gSOAP software package. The cacert.pem
file is the server's certificate, signed by the root (root.pem
created with the root.sh
script). This cacert.pem
file is the only file needed at the client side to verify the authenticity of the server using the self-signed certificate. Use the server.pem
file at the server side. The client.pem
is not needed, unless you enforce client authentication at the server side explicitly with SOAP_SSL_REQUIRE_CLIENT_AUTHENTICATION
to initialize the SSL context with soap_ssl_server_context
. The server.pem
file is actually a concatenation of the server's private key and its certificate, when created by cert.sh
.
add a comment |
Not sure why you had to convert the PEM files as you described. The cert.sh
script generates the PEM key file and PEM certificate file. This script can be used for server side to generate server.pem
and cacert.pem
. The cert.sh
script is located in gsoap/samples/ssl
in the gSOAP software package. The cacert.pem
file is the server's certificate, signed by the root (root.pem
created with the root.sh
script). This cacert.pem
file is the only file needed at the client side to verify the authenticity of the server using the self-signed certificate. Use the server.pem
file at the server side. The client.pem
is not needed, unless you enforce client authentication at the server side explicitly with SOAP_SSL_REQUIRE_CLIENT_AUTHENTICATION
to initialize the SSL context with soap_ssl_server_context
. The server.pem
file is actually a concatenation of the server's private key and its certificate, when created by cert.sh
.
add a comment |
Not sure why you had to convert the PEM files as you described. The cert.sh
script generates the PEM key file and PEM certificate file. This script can be used for server side to generate server.pem
and cacert.pem
. The cert.sh
script is located in gsoap/samples/ssl
in the gSOAP software package. The cacert.pem
file is the server's certificate, signed by the root (root.pem
created with the root.sh
script). This cacert.pem
file is the only file needed at the client side to verify the authenticity of the server using the self-signed certificate. Use the server.pem
file at the server side. The client.pem
is not needed, unless you enforce client authentication at the server side explicitly with SOAP_SSL_REQUIRE_CLIENT_AUTHENTICATION
to initialize the SSL context with soap_ssl_server_context
. The server.pem
file is actually a concatenation of the server's private key and its certificate, when created by cert.sh
.
Not sure why you had to convert the PEM files as you described. The cert.sh
script generates the PEM key file and PEM certificate file. This script can be used for server side to generate server.pem
and cacert.pem
. The cert.sh
script is located in gsoap/samples/ssl
in the gSOAP software package. The cacert.pem
file is the server's certificate, signed by the root (root.pem
created with the root.sh
script). This cacert.pem
file is the only file needed at the client side to verify the authenticity of the server using the self-signed certificate. Use the server.pem
file at the server side. The client.pem
is not needed, unless you enforce client authentication at the server side explicitly with SOAP_SSL_REQUIRE_CLIENT_AUTHENTICATION
to initialize the SSL context with soap_ssl_server_context
. The server.pem
file is actually a concatenation of the server's private key and its certificate, when created by cert.sh
.
answered Nov 15 '18 at 18:31
Dr. Alex REDr. Alex RE
703617
703617
add a comment |
add a comment |
I had to convert the PEM files to use them in the java webservice which uses the keytool. This keytool only understands PKCS12 certificates as far as I know. Additionally I had to use the Client certificates, because the Server uses the Client x.509 certificate in a truststore.
add a comment |
I had to convert the PEM files to use them in the java webservice which uses the keytool. This keytool only understands PKCS12 certificates as far as I know. Additionally I had to use the Client certificates, because the Server uses the Client x.509 certificate in a truststore.
add a comment |
I had to convert the PEM files to use them in the java webservice which uses the keytool. This keytool only understands PKCS12 certificates as far as I know. Additionally I had to use the Client certificates, because the Server uses the Client x.509 certificate in a truststore.
I had to convert the PEM files to use them in the java webservice which uses the keytool. This keytool only understands PKCS12 certificates as far as I know. Additionally I had to use the Client certificates, because the Server uses the Client x.509 certificate in a truststore.
answered Nov 17 '18 at 11:46


Pall FallPall Fall
12
12
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f52948525%2fcommunication-between-gsoap-client-and-java-webservice-over-https%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
uuoRJLR1suOrHsv,qO6,BOZ OkNf3B39S,uKskAWyHkSIQTSeknR61XT3GJ9G,Jf,lDE