Public instance of FactoryClass
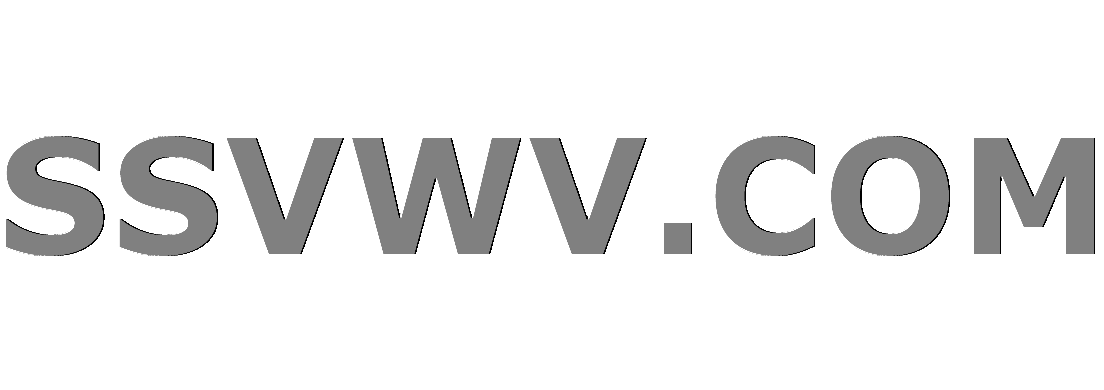
Multi tool use
I created two classes, one for the object I need (action), and a second as a factory class that I use to keep track of every instance of each class I created through arrays (ACTIONS(), OTHER(), etc()).
Actions are created from a userform and by the factory.
How could I do so that the same factory (I need the arrays) could be used by another userform (first userform is used to create the action, second to sort the ActionFactory actions array)?
I can't manage to share my ActionFactory
between the two forms, declaring it public returns an error. I feel like I'm missing something obvious.
Sub question: Whats the best design --> Factory class or factory method in the class itself?
Edit additional info
Here is how I create the Action object from my Userform
Option Explicit
Private ActionFactory As New Factory
Private Sub CommandButton1_Click()
Dim test As Action
Set test = ActionFactory.CreateAction(Tit.value, ComboBox1.ListIndex, ComboBox2.ListIndex, Startdate.value, EndDate.value, Owner.value)
test.draw
Dim act() As Action
act = ActionFactory.GetTheActionsArray
Debug.Print UBound(act)
End Sub
This is my factory and the Actions array & creator
Option Explicit
Private ACTIONS() As Action
Private OTHERS() as Other
Private counter As Integer
Public Function CreateAction(t As String, d As Integer, c As Integer, std As String, edt As String, ownr As String) As Object
counter = Sheets(Sheets.Count - 1).Cells(1, 1).value
ReDim Preserve ACTIONS(counter)
With New Action
.SetTitle = t
.SetDepartement = d
.SetCategory = c
.SetStartdate = std
.SetEndDate = edt
.SetOwner = ownr
.SetIndexValue = counter
Set ACTIONS(counter) = .Self
Set CreateAction = .Self
End With
Sheets(Sheets.Count - 1).Cells(1, 1).value = Sheets(Sheets.Count - 1).Cells(1, 1).value + 1
End Function
The code works, What I can't manage to do is to retrieve the values stored in ACTIONS() of ActionFactory on another userform that userform1.
This also lead me to another question I have.
How to get rid of that counter on the Factory class.
I would prefer something in the lines of :
If ACTIONS() = null Then
ReDim ACTIONS(0)
Else
ReDim preserve ACTIONS(ubound(ACTIONS) + 1)
end if
obviously, this does not work.
BR,
Bob
excel vba
add a comment |
I created two classes, one for the object I need (action), and a second as a factory class that I use to keep track of every instance of each class I created through arrays (ACTIONS(), OTHER(), etc()).
Actions are created from a userform and by the factory.
How could I do so that the same factory (I need the arrays) could be used by another userform (first userform is used to create the action, second to sort the ActionFactory actions array)?
I can't manage to share my ActionFactory
between the two forms, declaring it public returns an error. I feel like I'm missing something obvious.
Sub question: Whats the best design --> Factory class or factory method in the class itself?
Edit additional info
Here is how I create the Action object from my Userform
Option Explicit
Private ActionFactory As New Factory
Private Sub CommandButton1_Click()
Dim test As Action
Set test = ActionFactory.CreateAction(Tit.value, ComboBox1.ListIndex, ComboBox2.ListIndex, Startdate.value, EndDate.value, Owner.value)
test.draw
Dim act() As Action
act = ActionFactory.GetTheActionsArray
Debug.Print UBound(act)
End Sub
This is my factory and the Actions array & creator
Option Explicit
Private ACTIONS() As Action
Private OTHERS() as Other
Private counter As Integer
Public Function CreateAction(t As String, d As Integer, c As Integer, std As String, edt As String, ownr As String) As Object
counter = Sheets(Sheets.Count - 1).Cells(1, 1).value
ReDim Preserve ACTIONS(counter)
With New Action
.SetTitle = t
.SetDepartement = d
.SetCategory = c
.SetStartdate = std
.SetEndDate = edt
.SetOwner = ownr
.SetIndexValue = counter
Set ACTIONS(counter) = .Self
Set CreateAction = .Self
End With
Sheets(Sheets.Count - 1).Cells(1, 1).value = Sheets(Sheets.Count - 1).Cells(1, 1).value + 1
End Function
The code works, What I can't manage to do is to retrieve the values stored in ACTIONS() of ActionFactory on another userform that userform1.
This also lead me to another question I have.
How to get rid of that counter on the Factory class.
I would prefer something in the lines of :
If ACTIONS() = null Then
ReDim ACTIONS(0)
Else
ReDim preserve ACTIONS(ubound(ACTIONS) + 1)
end if
obviously, this does not work.
BR,
Bob
excel vba
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection classActions
which has the sole purpose of tracking/managing instances of theAction
class. Can you agree with this @RobRkt? There could surely be an additional classActionFactory
, that, depending on the circumstances, could be stand-alone or integrated intoActions
.
– Inarion
Nov 27 '18 at 13:07
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring yourActionFactory
public and what error happens?
– Inarion
Nov 27 '18 at 13:15
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34
add a comment |
I created two classes, one for the object I need (action), and a second as a factory class that I use to keep track of every instance of each class I created through arrays (ACTIONS(), OTHER(), etc()).
Actions are created from a userform and by the factory.
How could I do so that the same factory (I need the arrays) could be used by another userform (first userform is used to create the action, second to sort the ActionFactory actions array)?
I can't manage to share my ActionFactory
between the two forms, declaring it public returns an error. I feel like I'm missing something obvious.
Sub question: Whats the best design --> Factory class or factory method in the class itself?
Edit additional info
Here is how I create the Action object from my Userform
Option Explicit
Private ActionFactory As New Factory
Private Sub CommandButton1_Click()
Dim test As Action
Set test = ActionFactory.CreateAction(Tit.value, ComboBox1.ListIndex, ComboBox2.ListIndex, Startdate.value, EndDate.value, Owner.value)
test.draw
Dim act() As Action
act = ActionFactory.GetTheActionsArray
Debug.Print UBound(act)
End Sub
This is my factory and the Actions array & creator
Option Explicit
Private ACTIONS() As Action
Private OTHERS() as Other
Private counter As Integer
Public Function CreateAction(t As String, d As Integer, c As Integer, std As String, edt As String, ownr As String) As Object
counter = Sheets(Sheets.Count - 1).Cells(1, 1).value
ReDim Preserve ACTIONS(counter)
With New Action
.SetTitle = t
.SetDepartement = d
.SetCategory = c
.SetStartdate = std
.SetEndDate = edt
.SetOwner = ownr
.SetIndexValue = counter
Set ACTIONS(counter) = .Self
Set CreateAction = .Self
End With
Sheets(Sheets.Count - 1).Cells(1, 1).value = Sheets(Sheets.Count - 1).Cells(1, 1).value + 1
End Function
The code works, What I can't manage to do is to retrieve the values stored in ACTIONS() of ActionFactory on another userform that userform1.
This also lead me to another question I have.
How to get rid of that counter on the Factory class.
I would prefer something in the lines of :
If ACTIONS() = null Then
ReDim ACTIONS(0)
Else
ReDim preserve ACTIONS(ubound(ACTIONS) + 1)
end if
obviously, this does not work.
BR,
Bob
excel vba
I created two classes, one for the object I need (action), and a second as a factory class that I use to keep track of every instance of each class I created through arrays (ACTIONS(), OTHER(), etc()).
Actions are created from a userform and by the factory.
How could I do so that the same factory (I need the arrays) could be used by another userform (first userform is used to create the action, second to sort the ActionFactory actions array)?
I can't manage to share my ActionFactory
between the two forms, declaring it public returns an error. I feel like I'm missing something obvious.
Sub question: Whats the best design --> Factory class or factory method in the class itself?
Edit additional info
Here is how I create the Action object from my Userform
Option Explicit
Private ActionFactory As New Factory
Private Sub CommandButton1_Click()
Dim test As Action
Set test = ActionFactory.CreateAction(Tit.value, ComboBox1.ListIndex, ComboBox2.ListIndex, Startdate.value, EndDate.value, Owner.value)
test.draw
Dim act() As Action
act = ActionFactory.GetTheActionsArray
Debug.Print UBound(act)
End Sub
This is my factory and the Actions array & creator
Option Explicit
Private ACTIONS() As Action
Private OTHERS() as Other
Private counter As Integer
Public Function CreateAction(t As String, d As Integer, c As Integer, std As String, edt As String, ownr As String) As Object
counter = Sheets(Sheets.Count - 1).Cells(1, 1).value
ReDim Preserve ACTIONS(counter)
With New Action
.SetTitle = t
.SetDepartement = d
.SetCategory = c
.SetStartdate = std
.SetEndDate = edt
.SetOwner = ownr
.SetIndexValue = counter
Set ACTIONS(counter) = .Self
Set CreateAction = .Self
End With
Sheets(Sheets.Count - 1).Cells(1, 1).value = Sheets(Sheets.Count - 1).Cells(1, 1).value + 1
End Function
The code works, What I can't manage to do is to retrieve the values stored in ACTIONS() of ActionFactory on another userform that userform1.
This also lead me to another question I have.
How to get rid of that counter on the Factory class.
I would prefer something in the lines of :
If ACTIONS() = null Then
ReDim ACTIONS(0)
Else
ReDim preserve ACTIONS(ubound(ACTIONS) + 1)
end if
obviously, this does not work.
BR,
Bob
excel vba
excel vba
edited Nov 27 '18 at 18:11
a1a1a1a1a1
asked Nov 27 '18 at 12:24
a1a1a1a1a1a1a1a1a1a1
68
68
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection classActions
which has the sole purpose of tracking/managing instances of theAction
class. Can you agree with this @RobRkt? There could surely be an additional classActionFactory
, that, depending on the circumstances, could be stand-alone or integrated intoActions
.
– Inarion
Nov 27 '18 at 13:07
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring yourActionFactory
public and what error happens?
– Inarion
Nov 27 '18 at 13:15
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34
add a comment |
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection classActions
which has the sole purpose of tracking/managing instances of theAction
class. Can you agree with this @RobRkt? There could surely be an additional classActionFactory
, that, depending on the circumstances, could be stand-alone or integrated intoActions
.
– Inarion
Nov 27 '18 at 13:07
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring yourActionFactory
public and what error happens?
– Inarion
Nov 27 '18 at 13:15
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection class
Actions
which has the sole purpose of tracking/managing instances of the Action
class. Can you agree with this @RobRkt? There could surely be an additional class ActionFactory
, that, depending on the circumstances, could be stand-alone or integrated into Actions
.– Inarion
Nov 27 '18 at 13:07
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection class
Actions
which has the sole purpose of tracking/managing instances of the Action
class. Can you agree with this @RobRkt? There could surely be an additional class ActionFactory
, that, depending on the circumstances, could be stand-alone or integrated into Actions
.– Inarion
Nov 27 '18 at 13:07
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring your
ActionFactory
public and what error happens?– Inarion
Nov 27 '18 at 13:15
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring your
ActionFactory
public and what error happens?– Inarion
Nov 27 '18 at 13:15
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53499655%2fpublic-instance-of-factoryclass%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53499655%2fpublic-instance-of-factoryclass%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
0X49Y,ZyIOb5K0XlB73Hd5301Xr At544a,fH6Q9IpNVakh6,3I0YZ,o,supJedknEumwXMZqwiNP2,hpWgc,61ruWCU
Your factory should not be storing reference to the instances it creates. Its sole function should be to provide an instance of the class. The actions array should be created and stored by the object that used the actions. Best design is what is best for your solution. You might find this an interesting read rubberduckvba.wordpress.com/2018/04/24/…
– Freeflow
Nov 27 '18 at 12:46
I feel there might just be a misunderstanding or a wrong word here. What you describe is not so much a factory but more of a collection class
Actions
which has the sole purpose of tracking/managing instances of theAction
class. Can you agree with this @RobRkt? There could surely be an additional classActionFactory
, that, depending on the circumstances, could be stand-alone or integrated intoActions
.– Inarion
Nov 27 '18 at 13:07
Also could you share some more details regarding the forms: What connects them? There has to be some code that will provide the forms with data and have them show up. Where are you declaring your
ActionFactory
public and what error happens?– Inarion
Nov 27 '18 at 13:15
It does both, factory and collection. It can create & store multiple class I created. The term factory may be wrong but it does create the action, however the main role in our situation is the collection role.
– a1a1a1a1a1
Nov 27 '18 at 13:34