JavaScript UTC to localtime conversion not working in IE & Firefox, but works fine for Chrome
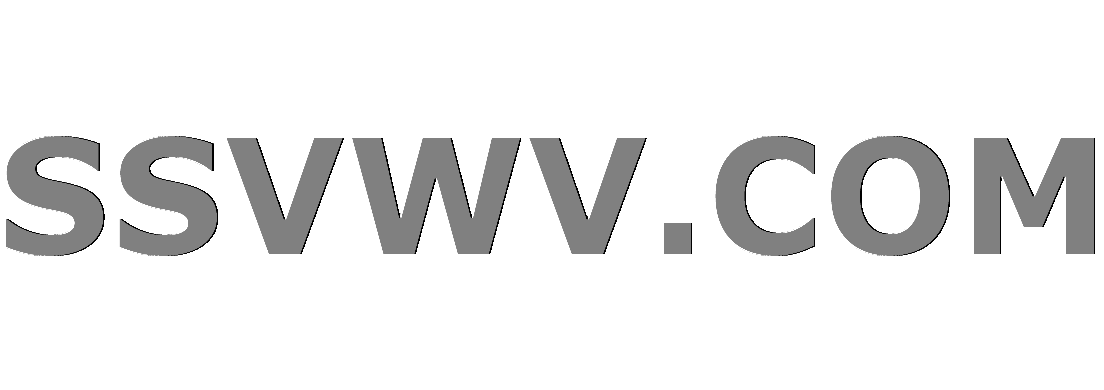
Multi tool use
I'm facing an issue.
I'm saving the UTC time in my server using Java code:
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
I persist the time with this ->
time = timeFormat.format(new Date()).toString()
This is persisted as the UTC time.
Now, while displaying it in a browser, I convert it into local time :
var date = new Date(time);
convertToLocal(date).toLocaleString();
function convertToLocal(date) {
var newDate = new Date(date.getTime() +date.getTimezoneOffset()*60*1000);
var offset = date.getTimezoneOffset() / 60;
var hours = date.getHours();
newDate.setHours(hours - offset);
return newDate;
}
console.log("Time : " + convertToLocal(date).toLocaleString())
This works fine in Chrome but in Firefox & IE , I get "Invalid Date" instead of the timestamp which I expect to see.
Please help.
javascript
|
show 5 more comments
I'm facing an issue.
I'm saving the UTC time in my server using Java code:
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
I persist the time with this ->
time = timeFormat.format(new Date()).toString()
This is persisted as the UTC time.
Now, while displaying it in a browser, I convert it into local time :
var date = new Date(time);
convertToLocal(date).toLocaleString();
function convertToLocal(date) {
var newDate = new Date(date.getTime() +date.getTimezoneOffset()*60*1000);
var offset = date.getTimezoneOffset() / 60;
var hours = date.getHours();
newDate.setHours(hours - offset);
return newDate;
}
console.log("Time : " + convertToLocal(date).toLocaleString())
This works fine in Chrome but in Firefox & IE , I get "Invalid Date" instead of the timestamp which I expect to see.
Please help.
javascript
2
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
2
I guess the Java output as string does not contain theT
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.
– trincot
Nov 26 '18 at 14:54
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
1
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to dotoLocaleString()
in JavaScript which will take the local timezone into account in the stringification.
– trincot
Nov 26 '18 at 15:12
|
show 5 more comments
I'm facing an issue.
I'm saving the UTC time in my server using Java code:
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
I persist the time with this ->
time = timeFormat.format(new Date()).toString()
This is persisted as the UTC time.
Now, while displaying it in a browser, I convert it into local time :
var date = new Date(time);
convertToLocal(date).toLocaleString();
function convertToLocal(date) {
var newDate = new Date(date.getTime() +date.getTimezoneOffset()*60*1000);
var offset = date.getTimezoneOffset() / 60;
var hours = date.getHours();
newDate.setHours(hours - offset);
return newDate;
}
console.log("Time : " + convertToLocal(date).toLocaleString())
This works fine in Chrome but in Firefox & IE , I get "Invalid Date" instead of the timestamp which I expect to see.
Please help.
javascript
I'm facing an issue.
I'm saving the UTC time in my server using Java code:
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
I persist the time with this ->
time = timeFormat.format(new Date()).toString()
This is persisted as the UTC time.
Now, while displaying it in a browser, I convert it into local time :
var date = new Date(time);
convertToLocal(date).toLocaleString();
function convertToLocal(date) {
var newDate = new Date(date.getTime() +date.getTimezoneOffset()*60*1000);
var offset = date.getTimezoneOffset() / 60;
var hours = date.getHours();
newDate.setHours(hours - offset);
return newDate;
}
console.log("Time : " + convertToLocal(date).toLocaleString())
This works fine in Chrome but in Firefox & IE , I get "Invalid Date" instead of the timestamp which I expect to see.
Please help.
javascript
javascript
asked Nov 26 '18 at 14:49
pep8pep8
437
437
2
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
2
I guess the Java output as string does not contain theT
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.
– trincot
Nov 26 '18 at 14:54
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
1
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to dotoLocaleString()
in JavaScript which will take the local timezone into account in the stringification.
– trincot
Nov 26 '18 at 15:12
|
show 5 more comments
2
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
2
I guess the Java output as string does not contain theT
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.
– trincot
Nov 26 '18 at 14:54
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
1
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to dotoLocaleString()
in JavaScript which will take the local timezone into account in the stringification.
– trincot
Nov 26 '18 at 15:12
2
2
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
2
2
I guess the Java output as string does not contain the
T
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.– trincot
Nov 26 '18 at 14:54
I guess the Java output as string does not contain the
T
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.– trincot
Nov 26 '18 at 14:54
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
1
1
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to do
toLocaleString()
in JavaScript which will take the local timezone into account in the stringification.– trincot
Nov 26 '18 at 15:12
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to do
toLocaleString()
in JavaScript which will take the local timezone into account in the stringification.– trincot
Nov 26 '18 at 15:12
|
show 5 more comments
2 Answers
2
active
oldest
votes
The problem is that your Java code produces a date/time string that does not adhere to the
ISO 8601 format. How a JavaScript engine must deal with that is not defined (and thus differs from one browser to another).
See How to get current moment in ISO 8601 format with date, hour, and minute? for how you can change the Java code to produce a date that uses the ISO format. You can just pass the desired format (ISO) to SimpleDateFormat
and configure it to mean UTC:
DateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
timeFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
That output should look like 2018-11-26T19:41:48Z (several variations of this are accepted). Notice the "T" and terminating "Z" (for UTC).
Once that is done, JavaScript will correctly interpret the date/time as being specified in UTC time zone, and then you only need to do the following in JavaScript, without any need of explicitly adding time zone differences:
console.log("Time : " + date.toLocaleString())
JavaScript which will take the local time zone into account in the stringification.
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specifyyyyy-MM-dd'T'HH:mm:ss'Z'
as argument toSimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.
– trincot
Nov 27 '18 at 7:36
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
|
show 1 more comment
tl;dr
Use modern java.time classes, not terrible legacy classes.
Send a string in standard ISO 8601 format.
Instant
.now()
.truncatedTo(
ChronoUnit.SECONDS
)
.toString()
Instant.now().toString(): 2018-11-27T00:57:25Z
Flawed code
This is persisted as the UTC time.
Nope, incorrect. You are not recording a moment in UTC.
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
String time = timeFormat.format(new Date()).toString() ;
You did specify a time zone in your SimpleDateFormat
class. By default that class implicitly applies the JVM’s current default time zone. So the string generated will vary at runtime.
For example, here in my current default time zone of America/Los_Angeles
it is not quite 5 PM. When I run that code, I get:
26-November-18 16:57:25
That is not UTC. UTC is several hours later, after midnight tomorrow, as shown next:
Instant.now().toString(): 2018-11-27T00:57:25.389849Z
If you want whole seconds, drop the fractional second by calling truncatedTo
.
Instant.now().truncatedTo( ChronoUnit.SECONDS ).toString(): 2018-11-27T00:57:25Z
java.time
You are using terrible old date-time classes that were supplanted years ago by the modern java.time classes.
Instant
The Instant
class represents a moment on the timeline in UTC with a resolution of nanoseconds (up to nine (9) digits of a decimal fraction).
Instant nowInUtc = Instant.now() ; // Capture the current moment in UTC.
This Instant
class is a basic building-block class of java.time. You can think of OffsetDateTime
as an Instant
plus a ZoneOffset
. Likewise, you can think of ZonedDateTime
as an Instant
plus a ZoneId
.
Never use LocalDateTime
to track a moment, as it purposely lacks any concept of time zone or offset-from-UTC.
ISO 8601
As trincot stated in his correct Answer, date-time values are best exchanged as text in standard ISO 8601 format.
For a moment in UTC, that would be either:
2018-11-27T00:57:25.389849Z
where theZ
on the end means UTC, and is pronounced “Zulu”.
2018-11-27T00:57:25.389849+00:00
where the+00:00
means an offset from UTC of zero hours-minutes-seconds, or in other words, UTC itself.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
Java SE 8, Java SE 9, Java SE 10, Java SE 11, and later - Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
Java SE 6 and Java SE 7
- Most of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
Android
- Later versions of Android bundle implementations of the java.time classes.
- For earlier Android (<26), the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53483634%2fjavascript-utc-to-localtime-conversion-not-working-in-ie-firefox-but-works-fi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
The problem is that your Java code produces a date/time string that does not adhere to the
ISO 8601 format. How a JavaScript engine must deal with that is not defined (and thus differs from one browser to another).
See How to get current moment in ISO 8601 format with date, hour, and minute? for how you can change the Java code to produce a date that uses the ISO format. You can just pass the desired format (ISO) to SimpleDateFormat
and configure it to mean UTC:
DateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
timeFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
That output should look like 2018-11-26T19:41:48Z (several variations of this are accepted). Notice the "T" and terminating "Z" (for UTC).
Once that is done, JavaScript will correctly interpret the date/time as being specified in UTC time zone, and then you only need to do the following in JavaScript, without any need of explicitly adding time zone differences:
console.log("Time : " + date.toLocaleString())
JavaScript which will take the local time zone into account in the stringification.
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specifyyyyy-MM-dd'T'HH:mm:ss'Z'
as argument toSimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.
– trincot
Nov 27 '18 at 7:36
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
|
show 1 more comment
The problem is that your Java code produces a date/time string that does not adhere to the
ISO 8601 format. How a JavaScript engine must deal with that is not defined (and thus differs from one browser to another).
See How to get current moment in ISO 8601 format with date, hour, and minute? for how you can change the Java code to produce a date that uses the ISO format. You can just pass the desired format (ISO) to SimpleDateFormat
and configure it to mean UTC:
DateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
timeFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
That output should look like 2018-11-26T19:41:48Z (several variations of this are accepted). Notice the "T" and terminating "Z" (for UTC).
Once that is done, JavaScript will correctly interpret the date/time as being specified in UTC time zone, and then you only need to do the following in JavaScript, without any need of explicitly adding time zone differences:
console.log("Time : " + date.toLocaleString())
JavaScript which will take the local time zone into account in the stringification.
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specifyyyyy-MM-dd'T'HH:mm:ss'Z'
as argument toSimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.
– trincot
Nov 27 '18 at 7:36
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
|
show 1 more comment
The problem is that your Java code produces a date/time string that does not adhere to the
ISO 8601 format. How a JavaScript engine must deal with that is not defined (and thus differs from one browser to another).
See How to get current moment in ISO 8601 format with date, hour, and minute? for how you can change the Java code to produce a date that uses the ISO format. You can just pass the desired format (ISO) to SimpleDateFormat
and configure it to mean UTC:
DateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
timeFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
That output should look like 2018-11-26T19:41:48Z (several variations of this are accepted). Notice the "T" and terminating "Z" (for UTC).
Once that is done, JavaScript will correctly interpret the date/time as being specified in UTC time zone, and then you only need to do the following in JavaScript, without any need of explicitly adding time zone differences:
console.log("Time : " + date.toLocaleString())
JavaScript which will take the local time zone into account in the stringification.
The problem is that your Java code produces a date/time string that does not adhere to the
ISO 8601 format. How a JavaScript engine must deal with that is not defined (and thus differs from one browser to another).
See How to get current moment in ISO 8601 format with date, hour, and minute? for how you can change the Java code to produce a date that uses the ISO format. You can just pass the desired format (ISO) to SimpleDateFormat
and configure it to mean UTC:
DateFormat timeFormat = new SimpleDateFormat("yyyy-MM-dd'T'HH:mm:ss'Z'");
timeFormat.setTimeZone(TimeZone.getTimeZone("UTC"));
That output should look like 2018-11-26T19:41:48Z (several variations of this are accepted). Notice the "T" and terminating "Z" (for UTC).
Once that is done, JavaScript will correctly interpret the date/time as being specified in UTC time zone, and then you only need to do the following in JavaScript, without any need of explicitly adding time zone differences:
console.log("Time : " + date.toLocaleString())
JavaScript which will take the local time zone into account in the stringification.
edited Nov 27 '18 at 13:34
answered Nov 26 '18 at 20:34


trincottrincot
123k1587121
123k1587121
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specifyyyyy-MM-dd'T'HH:mm:ss'Z'
as argument toSimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.
– trincot
Nov 27 '18 at 7:36
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
|
show 1 more comment
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specifyyyyy-MM-dd'T'HH:mm:ss'Z'
as argument toSimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.
– trincot
Nov 27 '18 at 7:36
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
After formatting the date as per the answer shared, and then doing toLocaleString() , everything works fine. But I didn't have any "T" or "Z" in my date when I persisted it through java code with that format. Is that expected? Do I need to be worried ?
– pep8
Nov 27 '18 at 7:00
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
For being 100% sure that JavaScript interprets the date string correctly, it would need to have that "T" and "Z". Just check the wikipedia page on what ISO 8601 specifies. If it complies with that, then every JavaScript engine will interpret it correctly. Other formats may work, but there is no language specification backing that up.
– trincot
Nov 27 '18 at 7:04
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
The answer you mentioned in the link , has this format in the accepted answer : "new SimpleDateFormat("MM/dd/yyyy KK:mm:ss a Z").format(dateObj)". And this doesn't contain a "T" or "Z" when you print a date with that format.
– pep8
Nov 27 '18 at 7:10
Specify
yyyy-MM-dd'T'HH:mm:ss'Z'
as argument to SimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.– trincot
Nov 27 '18 at 7:36
Specify
yyyy-MM-dd'T'HH:mm:ss'Z'
as argument to SimpleDateFormat
. I replaced the reference with a better Q&A on StackOverflow.– trincot
Nov 27 '18 at 7:36
1
1
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
Yes, that is an issue, because with a string formatted as MM/dd/yyyy, JS cannot always determine with certainty whether the format of the received date string was MM/dd/yyyy or dd/MM/yyyy. It is not documented how JS should deal with this. It is always the safest to have yyyy-MM-dd. JS also supports RFC 2822 compliant timestamps, but there the day should come before(!) the month.
– trincot
Nov 27 '18 at 13:09
|
show 1 more comment
tl;dr
Use modern java.time classes, not terrible legacy classes.
Send a string in standard ISO 8601 format.
Instant
.now()
.truncatedTo(
ChronoUnit.SECONDS
)
.toString()
Instant.now().toString(): 2018-11-27T00:57:25Z
Flawed code
This is persisted as the UTC time.
Nope, incorrect. You are not recording a moment in UTC.
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
String time = timeFormat.format(new Date()).toString() ;
You did specify a time zone in your SimpleDateFormat
class. By default that class implicitly applies the JVM’s current default time zone. So the string generated will vary at runtime.
For example, here in my current default time zone of America/Los_Angeles
it is not quite 5 PM. When I run that code, I get:
26-November-18 16:57:25
That is not UTC. UTC is several hours later, after midnight tomorrow, as shown next:
Instant.now().toString(): 2018-11-27T00:57:25.389849Z
If you want whole seconds, drop the fractional second by calling truncatedTo
.
Instant.now().truncatedTo( ChronoUnit.SECONDS ).toString(): 2018-11-27T00:57:25Z
java.time
You are using terrible old date-time classes that were supplanted years ago by the modern java.time classes.
Instant
The Instant
class represents a moment on the timeline in UTC with a resolution of nanoseconds (up to nine (9) digits of a decimal fraction).
Instant nowInUtc = Instant.now() ; // Capture the current moment in UTC.
This Instant
class is a basic building-block class of java.time. You can think of OffsetDateTime
as an Instant
plus a ZoneOffset
. Likewise, you can think of ZonedDateTime
as an Instant
plus a ZoneId
.
Never use LocalDateTime
to track a moment, as it purposely lacks any concept of time zone or offset-from-UTC.
ISO 8601
As trincot stated in his correct Answer, date-time values are best exchanged as text in standard ISO 8601 format.
For a moment in UTC, that would be either:
2018-11-27T00:57:25.389849Z
where theZ
on the end means UTC, and is pronounced “Zulu”.
2018-11-27T00:57:25.389849+00:00
where the+00:00
means an offset from UTC of zero hours-minutes-seconds, or in other words, UTC itself.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
Java SE 8, Java SE 9, Java SE 10, Java SE 11, and later - Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
Java SE 6 and Java SE 7
- Most of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
Android
- Later versions of Android bundle implementations of the java.time classes.
- For earlier Android (<26), the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
add a comment |
tl;dr
Use modern java.time classes, not terrible legacy classes.
Send a string in standard ISO 8601 format.
Instant
.now()
.truncatedTo(
ChronoUnit.SECONDS
)
.toString()
Instant.now().toString(): 2018-11-27T00:57:25Z
Flawed code
This is persisted as the UTC time.
Nope, incorrect. You are not recording a moment in UTC.
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
String time = timeFormat.format(new Date()).toString() ;
You did specify a time zone in your SimpleDateFormat
class. By default that class implicitly applies the JVM’s current default time zone. So the string generated will vary at runtime.
For example, here in my current default time zone of America/Los_Angeles
it is not quite 5 PM. When I run that code, I get:
26-November-18 16:57:25
That is not UTC. UTC is several hours later, after midnight tomorrow, as shown next:
Instant.now().toString(): 2018-11-27T00:57:25.389849Z
If you want whole seconds, drop the fractional second by calling truncatedTo
.
Instant.now().truncatedTo( ChronoUnit.SECONDS ).toString(): 2018-11-27T00:57:25Z
java.time
You are using terrible old date-time classes that were supplanted years ago by the modern java.time classes.
Instant
The Instant
class represents a moment on the timeline in UTC with a resolution of nanoseconds (up to nine (9) digits of a decimal fraction).
Instant nowInUtc = Instant.now() ; // Capture the current moment in UTC.
This Instant
class is a basic building-block class of java.time. You can think of OffsetDateTime
as an Instant
plus a ZoneOffset
. Likewise, you can think of ZonedDateTime
as an Instant
plus a ZoneId
.
Never use LocalDateTime
to track a moment, as it purposely lacks any concept of time zone or offset-from-UTC.
ISO 8601
As trincot stated in his correct Answer, date-time values are best exchanged as text in standard ISO 8601 format.
For a moment in UTC, that would be either:
2018-11-27T00:57:25.389849Z
where theZ
on the end means UTC, and is pronounced “Zulu”.
2018-11-27T00:57:25.389849+00:00
where the+00:00
means an offset from UTC of zero hours-minutes-seconds, or in other words, UTC itself.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
Java SE 8, Java SE 9, Java SE 10, Java SE 11, and later - Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
Java SE 6 and Java SE 7
- Most of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
Android
- Later versions of Android bundle implementations of the java.time classes.
- For earlier Android (<26), the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
add a comment |
tl;dr
Use modern java.time classes, not terrible legacy classes.
Send a string in standard ISO 8601 format.
Instant
.now()
.truncatedTo(
ChronoUnit.SECONDS
)
.toString()
Instant.now().toString(): 2018-11-27T00:57:25Z
Flawed code
This is persisted as the UTC time.
Nope, incorrect. You are not recording a moment in UTC.
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
String time = timeFormat.format(new Date()).toString() ;
You did specify a time zone in your SimpleDateFormat
class. By default that class implicitly applies the JVM’s current default time zone. So the string generated will vary at runtime.
For example, here in my current default time zone of America/Los_Angeles
it is not quite 5 PM. When I run that code, I get:
26-November-18 16:57:25
That is not UTC. UTC is several hours later, after midnight tomorrow, as shown next:
Instant.now().toString(): 2018-11-27T00:57:25.389849Z
If you want whole seconds, drop the fractional second by calling truncatedTo
.
Instant.now().truncatedTo( ChronoUnit.SECONDS ).toString(): 2018-11-27T00:57:25Z
java.time
You are using terrible old date-time classes that were supplanted years ago by the modern java.time classes.
Instant
The Instant
class represents a moment on the timeline in UTC with a resolution of nanoseconds (up to nine (9) digits of a decimal fraction).
Instant nowInUtc = Instant.now() ; // Capture the current moment in UTC.
This Instant
class is a basic building-block class of java.time. You can think of OffsetDateTime
as an Instant
plus a ZoneOffset
. Likewise, you can think of ZonedDateTime
as an Instant
plus a ZoneId
.
Never use LocalDateTime
to track a moment, as it purposely lacks any concept of time zone or offset-from-UTC.
ISO 8601
As trincot stated in his correct Answer, date-time values are best exchanged as text in standard ISO 8601 format.
For a moment in UTC, that would be either:
2018-11-27T00:57:25.389849Z
where theZ
on the end means UTC, and is pronounced “Zulu”.
2018-11-27T00:57:25.389849+00:00
where the+00:00
means an offset from UTC of zero hours-minutes-seconds, or in other words, UTC itself.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
Java SE 8, Java SE 9, Java SE 10, Java SE 11, and later - Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
Java SE 6 and Java SE 7
- Most of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
Android
- Later versions of Android bundle implementations of the java.time classes.
- For earlier Android (<26), the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
tl;dr
Use modern java.time classes, not terrible legacy classes.
Send a string in standard ISO 8601 format.
Instant
.now()
.truncatedTo(
ChronoUnit.SECONDS
)
.toString()
Instant.now().toString(): 2018-11-27T00:57:25Z
Flawed code
This is persisted as the UTC time.
Nope, incorrect. You are not recording a moment in UTC.
DateFormat timeFormat = new SimpleDateFormat("dd-MMMM-yy HH:mm:ss");
String time = timeFormat.format(new Date()).toString() ;
You did specify a time zone in your SimpleDateFormat
class. By default that class implicitly applies the JVM’s current default time zone. So the string generated will vary at runtime.
For example, here in my current default time zone of America/Los_Angeles
it is not quite 5 PM. When I run that code, I get:
26-November-18 16:57:25
That is not UTC. UTC is several hours later, after midnight tomorrow, as shown next:
Instant.now().toString(): 2018-11-27T00:57:25.389849Z
If you want whole seconds, drop the fractional second by calling truncatedTo
.
Instant.now().truncatedTo( ChronoUnit.SECONDS ).toString(): 2018-11-27T00:57:25Z
java.time
You are using terrible old date-time classes that were supplanted years ago by the modern java.time classes.
Instant
The Instant
class represents a moment on the timeline in UTC with a resolution of nanoseconds (up to nine (9) digits of a decimal fraction).
Instant nowInUtc = Instant.now() ; // Capture the current moment in UTC.
This Instant
class is a basic building-block class of java.time. You can think of OffsetDateTime
as an Instant
plus a ZoneOffset
. Likewise, you can think of ZonedDateTime
as an Instant
plus a ZoneId
.
Never use LocalDateTime
to track a moment, as it purposely lacks any concept of time zone or offset-from-UTC.
ISO 8601
As trincot stated in his correct Answer, date-time values are best exchanged as text in standard ISO 8601 format.
For a moment in UTC, that would be either:
2018-11-27T00:57:25.389849Z
where theZ
on the end means UTC, and is pronounced “Zulu”.
2018-11-27T00:57:25.389849+00:00
where the+00:00
means an offset from UTC of zero hours-minutes-seconds, or in other words, UTC itself.
About java.time
The java.time framework is built into Java 8 and later. These classes supplant the troublesome old legacy date-time classes such as java.util.Date
, Calendar
, & SimpleDateFormat
.
The Joda-Time project, now in maintenance mode, advises migration to the java.time classes.
To learn more, see the Oracle Tutorial. And search Stack Overflow for many examples and explanations. Specification is JSR 310.
You may exchange java.time objects directly with your database. Use a JDBC driver compliant with JDBC 4.2 or later. No need for strings, no need for java.sql.*
classes.
Where to obtain the java.time classes?
Java SE 8, Java SE 9, Java SE 10, Java SE 11, and later - Part of the standard Java API with a bundled implementation.
- Java 9 adds some minor features and fixes.
Java SE 6 and Java SE 7
- Most of the java.time functionality is back-ported to Java 6 & 7 in ThreeTen-Backport.
Android
- Later versions of Android bundle implementations of the java.time classes.
- For earlier Android (<26), the ThreeTenABP project adapts ThreeTen-Backport (mentioned above). See How to use ThreeTenABP….
The ThreeTen-Extra project extends java.time with additional classes. This project is a proving ground for possible future additions to java.time. You may find some useful classes here such as Interval
, YearWeek
, YearQuarter
, and more.
edited Nov 27 '18 at 1:15
answered Nov 27 '18 at 1:00


Basil BourqueBasil Bourque
111k27378542
111k27378542
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53483634%2fjavascript-utc-to-localtime-conversion-not-working-in-ie-firefox-but-works-fi%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
68w8zs1Pl4hkq6PDgP,uRGAfaJ39ong,XaPOYxcJwBR,aqVDbqL Mgh,IQck J9x2h,k,yEbIsX67nom4IzzbJb8GF6BH,FbeVNhQK
2
Browsers are not consistent in the date formats they accept beyond ISO standard (which yours isn't).
– Pointy
Nov 26 '18 at 14:54
2
I guess the Java output as string does not contain the
T
between date and time parts. Also make sure the timezone is added, which can be a plain "Z" suffix for UTC. Make sure it has that so it complies with ISO standards. With that you wont need to add timezone offsets in JavaScript. Just use the appropriate rendering.– trincot
Nov 26 '18 at 14:54
Also if send proper ISO UTC date string won't need to do local conversion
– charlietfl
Nov 26 '18 at 15:00
@trincot : Can you please explain with some snippets how I can modify the code?
– pep8
Nov 26 '18 at 15:01
1
See stackoverflow.com/questions/20238280/date-in-to-utc-format-java for the change in Java code. Once that is done you only need to do
toLocaleString()
in JavaScript which will take the local timezone into account in the stringification.– trincot
Nov 26 '18 at 15:12