An elegant way to unpool matrix with numpy [duplicate]
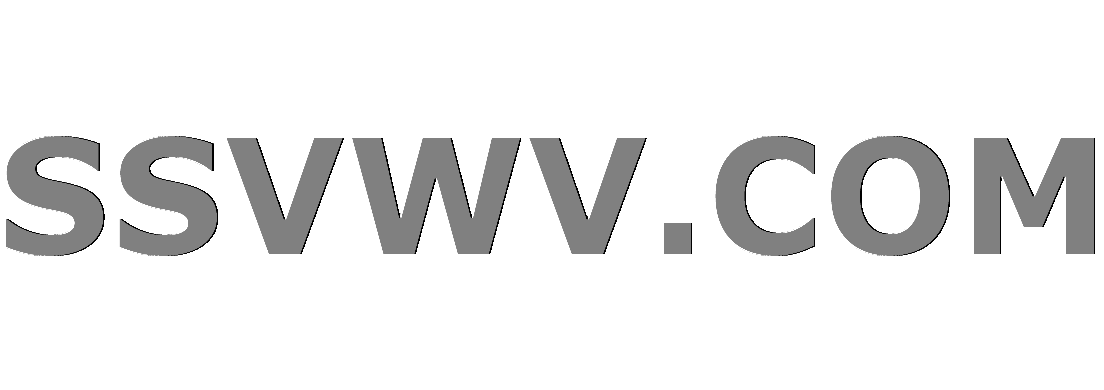
Multi tool use
This question already has an answer here:
Quick way to upsample numpy array by nearest neighbor tiling [duplicate]
3 answers
How to repeat elements of an array along two axes?
4 answers
I will describe the problem with an example. There is a matrix
[[1 2]
[3 4]]
I need to resize/unpool it to the next one
[[1 1 2 2]
[1 1 2 2]
[3 3 4 4]
[3 3 4 4]]
The operation is kind of opposite to max pooling in convnets. I have a brute solution with cycles. I would be very happy to see if there is a sophisticated solution with numpy.
My brute force solution is below.
matrix = np.arange(1, 5).reshape(2, 2)
matrix_m, matrix_n = matrix.shape
kernel = np.ones((2, 2), dtype=np.int)
kernel_m, kernel_n = kernel.shape
unpooled_matrix = np.zeros((matrix_m * kernel_m, matrix_n * kernel_n), dtype=np.int)
for i in range(matrix_m):
pos_i = i * kernel_m
for j in range(matrix_n):
pos_j = j * kernel_n
unpooled_matrix[pos_i: pos_i + kernel_m, pos_j: pos_j + kernel_n] = kernel * matrix[i][j]
print(matrix)
print(kernel)
print(unpooled_matrix)
python numpy
marked as duplicate by Divakar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 26 '18 at 18:50
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
This question already has an answer here:
Quick way to upsample numpy array by nearest neighbor tiling [duplicate]
3 answers
How to repeat elements of an array along two axes?
4 answers
I will describe the problem with an example. There is a matrix
[[1 2]
[3 4]]
I need to resize/unpool it to the next one
[[1 1 2 2]
[1 1 2 2]
[3 3 4 4]
[3 3 4 4]]
The operation is kind of opposite to max pooling in convnets. I have a brute solution with cycles. I would be very happy to see if there is a sophisticated solution with numpy.
My brute force solution is below.
matrix = np.arange(1, 5).reshape(2, 2)
matrix_m, matrix_n = matrix.shape
kernel = np.ones((2, 2), dtype=np.int)
kernel_m, kernel_n = kernel.shape
unpooled_matrix = np.zeros((matrix_m * kernel_m, matrix_n * kernel_n), dtype=np.int)
for i in range(matrix_m):
pos_i = i * kernel_m
for j in range(matrix_n):
pos_j = j * kernel_n
unpooled_matrix[pos_i: pos_i + kernel_m, pos_j: pos_j + kernel_n] = kernel * matrix[i][j]
print(matrix)
print(kernel)
print(unpooled_matrix)
python numpy
marked as duplicate by Divakar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 26 '18 at 18:50
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
This question already has an answer here:
Quick way to upsample numpy array by nearest neighbor tiling [duplicate]
3 answers
How to repeat elements of an array along two axes?
4 answers
I will describe the problem with an example. There is a matrix
[[1 2]
[3 4]]
I need to resize/unpool it to the next one
[[1 1 2 2]
[1 1 2 2]
[3 3 4 4]
[3 3 4 4]]
The operation is kind of opposite to max pooling in convnets. I have a brute solution with cycles. I would be very happy to see if there is a sophisticated solution with numpy.
My brute force solution is below.
matrix = np.arange(1, 5).reshape(2, 2)
matrix_m, matrix_n = matrix.shape
kernel = np.ones((2, 2), dtype=np.int)
kernel_m, kernel_n = kernel.shape
unpooled_matrix = np.zeros((matrix_m * kernel_m, matrix_n * kernel_n), dtype=np.int)
for i in range(matrix_m):
pos_i = i * kernel_m
for j in range(matrix_n):
pos_j = j * kernel_n
unpooled_matrix[pos_i: pos_i + kernel_m, pos_j: pos_j + kernel_n] = kernel * matrix[i][j]
print(matrix)
print(kernel)
print(unpooled_matrix)
python numpy
This question already has an answer here:
Quick way to upsample numpy array by nearest neighbor tiling [duplicate]
3 answers
How to repeat elements of an array along two axes?
4 answers
I will describe the problem with an example. There is a matrix
[[1 2]
[3 4]]
I need to resize/unpool it to the next one
[[1 1 2 2]
[1 1 2 2]
[3 3 4 4]
[3 3 4 4]]
The operation is kind of opposite to max pooling in convnets. I have a brute solution with cycles. I would be very happy to see if there is a sophisticated solution with numpy.
My brute force solution is below.
matrix = np.arange(1, 5).reshape(2, 2)
matrix_m, matrix_n = matrix.shape
kernel = np.ones((2, 2), dtype=np.int)
kernel_m, kernel_n = kernel.shape
unpooled_matrix = np.zeros((matrix_m * kernel_m, matrix_n * kernel_n), dtype=np.int)
for i in range(matrix_m):
pos_i = i * kernel_m
for j in range(matrix_n):
pos_j = j * kernel_n
unpooled_matrix[pos_i: pos_i + kernel_m, pos_j: pos_j + kernel_n] = kernel * matrix[i][j]
print(matrix)
print(kernel)
print(unpooled_matrix)
This question already has an answer here:
Quick way to upsample numpy array by nearest neighbor tiling [duplicate]
3 answers
How to repeat elements of an array along two axes?
4 answers
python numpy
python numpy
asked Nov 26 '18 at 18:47


vogdbvogdb
2,84621821
2,84621821
marked as duplicate by Divakar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 26 '18 at 18:50
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
marked as duplicate by Divakar
StackExchange.ready(function() {
if (StackExchange.options.isMobile) return;
$('.dupe-hammer-message-hover:not(.hover-bound)').each(function() {
var $hover = $(this).addClass('hover-bound'),
$msg = $hover.siblings('.dupe-hammer-message');
$hover.hover(
function() {
$hover.showInfoMessage('', {
messageElement: $msg.clone().show(),
transient: false,
position: { my: 'bottom left', at: 'top center', offsetTop: -7 },
dismissable: false,
relativeToBody: true
});
},
function() {
StackExchange.helpers.removeMessages();
}
);
});
});
Nov 26 '18 at 18:50
This question has been asked before and already has an answer. If those answers do not fully address your question, please ask a new question.
add a comment |
add a comment |
0
active
oldest
votes
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
22Rvpi1yK AaLi2jkA pMk20U qLGqsGV1yoyYVjsVlSe 2SRHbdVEje3 z89jnCYP 2 4AT5B eg,aexhzRaP