My loop is populating my array with the number seven, 6 times, why?
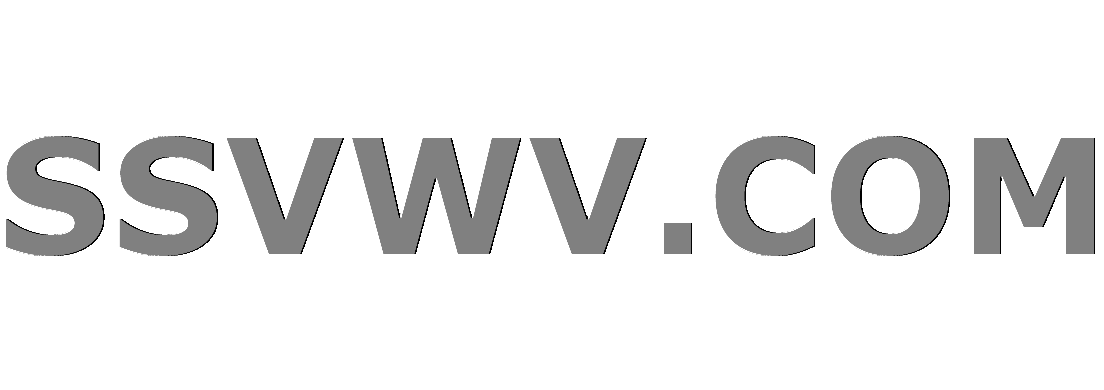
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
Im writing a program to read in a file and store the strings in arraylist and ints in an array. The file contains strings and ints in the format: String int
I have already got the string section to work, I'm looking to know why the following code is populating my array with the number 7, six times rather than the correct numbers. Correct output: 12, 14, 16, 31, 42, 7 but like I said, it gives, 7, 7, 7, 7, 7, 7
Code:
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
for(int i = 0; i <= arrayInt.length - 1; i++) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
}
}
}
buffy.close();
I can provide the rest of the code if you need me to,but anyways, any advice or tips are appreciated. Thanks in advanced.
java arrays loops output
add a comment |
Im writing a program to read in a file and store the strings in arraylist and ints in an array. The file contains strings and ints in the format: String int
I have already got the string section to work, I'm looking to know why the following code is populating my array with the number 7, six times rather than the correct numbers. Correct output: 12, 14, 16, 31, 42, 7 but like I said, it gives, 7, 7, 7, 7, 7, 7
Code:
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
for(int i = 0; i <= arrayInt.length - 1; i++) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
}
}
}
buffy.close();
I can provide the rest of the code if you need me to,but anyways, any advice or tips are appreciated. Thanks in advanced.
java arrays loops output
1
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53
add a comment |
Im writing a program to read in a file and store the strings in arraylist and ints in an array. The file contains strings and ints in the format: String int
I have already got the string section to work, I'm looking to know why the following code is populating my array with the number 7, six times rather than the correct numbers. Correct output: 12, 14, 16, 31, 42, 7 but like I said, it gives, 7, 7, 7, 7, 7, 7
Code:
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
for(int i = 0; i <= arrayInt.length - 1; i++) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
}
}
}
buffy.close();
I can provide the rest of the code if you need me to,but anyways, any advice or tips are appreciated. Thanks in advanced.
java arrays loops output
Im writing a program to read in a file and store the strings in arraylist and ints in an array. The file contains strings and ints in the format: String int
I have already got the string section to work, I'm looking to know why the following code is populating my array with the number 7, six times rather than the correct numbers. Correct output: 12, 14, 16, 31, 42, 7 but like I said, it gives, 7, 7, 7, 7, 7, 7
Code:
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
for(int i = 0; i <= arrayInt.length - 1; i++) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
}
}
}
buffy.close();
I can provide the rest of the code if you need me to,but anyways, any advice or tips are appreciated. Thanks in advanced.
java arrays loops output
java arrays loops output
asked Nov 29 '18 at 3:49
LootsLoots
82
82
1
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53
add a comment |
1
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53
1
1
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53
add a comment |
2 Answers
2
active
oldest
votes
This happens because for each line in file you fill whole array.
Try this:
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
break;
}
}
i++;
}
}
buffy.close();
Also you can use indexOf
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
int k = str.indexOf(" ");
if(k!=-1) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
i++;
}
}
buffy.close();
add a comment |
File read are typically batch/ETL kind of job and if this code is going to production and would be used multiple times instead of only once then I would like to stress on Performance and Ease of Maintenance:
Only read least characters to identify the space index
- @talex added a very good line of code i.e.
break;
inside the loop that way you won't need to read till the end of line but this would only work if the string has no spaces. If the string can contain spaces than you would needlastIndexOf
space(" ")
or nobreak;
at all.
I prefer using framework method
lastIndexOf
assuming you are using java because:
- it would start reading from right instead of left and assuming the numbers would be always less length than the string it would find index of space faster in most of the cases than reading from start.
2nd benefit is that there are lots of scenarios framework/utilities method already handled so why to reinvent the wheel
int k = str.lastIndexOf(" ");
last but not the least if someone else is going to maintain this code it would be easier for him/her as there would be enough documentation available.
Only read required lines from files
Seems like you only need certain number of lines to read arrayInt.length
if that's the case then you should 'break;' the while
loop once the counter i
is more than array length.
I/O operations are costly and though you will get right output you would end-up scanning whole file even if it's not required.
Dont forget try-catch-finally
The code assumes that there will not be any issue and it would be able to close the file after done but there could be n number of combinations that can result in error resulting the application to crash and locking the file.
See the below example:
private Integer readNumbers(String fileName) throws Exception {
Integer arrayInt = new Integer[7];
String str = null;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
try {
int i=0;
while ((str = buffy.readLine()) != null) {
if(i> arrayInt.length){
break;
}
//get last index of " "
int k = str.lastIndexOf(" ");
if(k > -1){
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
//increment the line counter
i++;
}
} catch (Exception ex) {
//handle exception
} finally {
buffy.close();
}
return arrayInt;
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531572%2fmy-loop-is-populating-my-array-with-the-number-seven-6-times-why%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
This happens because for each line in file you fill whole array.
Try this:
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
break;
}
}
i++;
}
}
buffy.close();
Also you can use indexOf
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
int k = str.indexOf(" ");
if(k!=-1) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
i++;
}
}
buffy.close();
add a comment |
This happens because for each line in file you fill whole array.
Try this:
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
break;
}
}
i++;
}
}
buffy.close();
Also you can use indexOf
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
int k = str.indexOf(" ");
if(k!=-1) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
i++;
}
}
buffy.close();
add a comment |
This happens because for each line in file you fill whole array.
Try this:
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
break;
}
}
i++;
}
}
buffy.close();
Also you can use indexOf
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
int k = str.indexOf(" ");
if(k!=-1) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
i++;
}
}
buffy.close();
This happens because for each line in file you fill whole array.
Try this:
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
for(int k = 0; k <= str.length()-1; k++) {
if(str.substring(k, k + 1).equals(" ")) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
break;
}
}
i++;
}
}
buffy.close();
Also you can use indexOf
int i = 0;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
while((str = buffy.readLine()) != null) {
if(i < arrayInt.length) {
int k = str.indexOf(" ");
if(k!=-1) {
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
i++;
}
}
buffy.close();
answered Nov 29 '18 at 4:02
talextalex
11.9k11749
11.9k11749
add a comment |
add a comment |
File read are typically batch/ETL kind of job and if this code is going to production and would be used multiple times instead of only once then I would like to stress on Performance and Ease of Maintenance:
Only read least characters to identify the space index
- @talex added a very good line of code i.e.
break;
inside the loop that way you won't need to read till the end of line but this would only work if the string has no spaces. If the string can contain spaces than you would needlastIndexOf
space(" ")
or nobreak;
at all.
I prefer using framework method
lastIndexOf
assuming you are using java because:
- it would start reading from right instead of left and assuming the numbers would be always less length than the string it would find index of space faster in most of the cases than reading from start.
2nd benefit is that there are lots of scenarios framework/utilities method already handled so why to reinvent the wheel
int k = str.lastIndexOf(" ");
last but not the least if someone else is going to maintain this code it would be easier for him/her as there would be enough documentation available.
Only read required lines from files
Seems like you only need certain number of lines to read arrayInt.length
if that's the case then you should 'break;' the while
loop once the counter i
is more than array length.
I/O operations are costly and though you will get right output you would end-up scanning whole file even if it's not required.
Dont forget try-catch-finally
The code assumes that there will not be any issue and it would be able to close the file after done but there could be n number of combinations that can result in error resulting the application to crash and locking the file.
See the below example:
private Integer readNumbers(String fileName) throws Exception {
Integer arrayInt = new Integer[7];
String str = null;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
try {
int i=0;
while ((str = buffy.readLine()) != null) {
if(i> arrayInt.length){
break;
}
//get last index of " "
int k = str.lastIndexOf(" ");
if(k > -1){
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
//increment the line counter
i++;
}
} catch (Exception ex) {
//handle exception
} finally {
buffy.close();
}
return arrayInt;
}
add a comment |
File read are typically batch/ETL kind of job and if this code is going to production and would be used multiple times instead of only once then I would like to stress on Performance and Ease of Maintenance:
Only read least characters to identify the space index
- @talex added a very good line of code i.e.
break;
inside the loop that way you won't need to read till the end of line but this would only work if the string has no spaces. If the string can contain spaces than you would needlastIndexOf
space(" ")
or nobreak;
at all.
I prefer using framework method
lastIndexOf
assuming you are using java because:
- it would start reading from right instead of left and assuming the numbers would be always less length than the string it would find index of space faster in most of the cases than reading from start.
2nd benefit is that there are lots of scenarios framework/utilities method already handled so why to reinvent the wheel
int k = str.lastIndexOf(" ");
last but not the least if someone else is going to maintain this code it would be easier for him/her as there would be enough documentation available.
Only read required lines from files
Seems like you only need certain number of lines to read arrayInt.length
if that's the case then you should 'break;' the while
loop once the counter i
is more than array length.
I/O operations are costly and though you will get right output you would end-up scanning whole file even if it's not required.
Dont forget try-catch-finally
The code assumes that there will not be any issue and it would be able to close the file after done but there could be n number of combinations that can result in error resulting the application to crash and locking the file.
See the below example:
private Integer readNumbers(String fileName) throws Exception {
Integer arrayInt = new Integer[7];
String str = null;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
try {
int i=0;
while ((str = buffy.readLine()) != null) {
if(i> arrayInt.length){
break;
}
//get last index of " "
int k = str.lastIndexOf(" ");
if(k > -1){
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
//increment the line counter
i++;
}
} catch (Exception ex) {
//handle exception
} finally {
buffy.close();
}
return arrayInt;
}
add a comment |
File read are typically batch/ETL kind of job and if this code is going to production and would be used multiple times instead of only once then I would like to stress on Performance and Ease of Maintenance:
Only read least characters to identify the space index
- @talex added a very good line of code i.e.
break;
inside the loop that way you won't need to read till the end of line but this would only work if the string has no spaces. If the string can contain spaces than you would needlastIndexOf
space(" ")
or nobreak;
at all.
I prefer using framework method
lastIndexOf
assuming you are using java because:
- it would start reading from right instead of left and assuming the numbers would be always less length than the string it would find index of space faster in most of the cases than reading from start.
2nd benefit is that there are lots of scenarios framework/utilities method already handled so why to reinvent the wheel
int k = str.lastIndexOf(" ");
last but not the least if someone else is going to maintain this code it would be easier for him/her as there would be enough documentation available.
Only read required lines from files
Seems like you only need certain number of lines to read arrayInt.length
if that's the case then you should 'break;' the while
loop once the counter i
is more than array length.
I/O operations are costly and though you will get right output you would end-up scanning whole file even if it's not required.
Dont forget try-catch-finally
The code assumes that there will not be any issue and it would be able to close the file after done but there could be n number of combinations that can result in error resulting the application to crash and locking the file.
See the below example:
private Integer readNumbers(String fileName) throws Exception {
Integer arrayInt = new Integer[7];
String str = null;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
try {
int i=0;
while ((str = buffy.readLine()) != null) {
if(i> arrayInt.length){
break;
}
//get last index of " "
int k = str.lastIndexOf(" ");
if(k > -1){
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
//increment the line counter
i++;
}
} catch (Exception ex) {
//handle exception
} finally {
buffy.close();
}
return arrayInt;
}
File read are typically batch/ETL kind of job and if this code is going to production and would be used multiple times instead of only once then I would like to stress on Performance and Ease of Maintenance:
Only read least characters to identify the space index
- @talex added a very good line of code i.e.
break;
inside the loop that way you won't need to read till the end of line but this would only work if the string has no spaces. If the string can contain spaces than you would needlastIndexOf
space(" ")
or nobreak;
at all.
I prefer using framework method
lastIndexOf
assuming you are using java because:
- it would start reading from right instead of left and assuming the numbers would be always less length than the string it would find index of space faster in most of the cases than reading from start.
2nd benefit is that there are lots of scenarios framework/utilities method already handled so why to reinvent the wheel
int k = str.lastIndexOf(" ");
last but not the least if someone else is going to maintain this code it would be easier for him/her as there would be enough documentation available.
Only read required lines from files
Seems like you only need certain number of lines to read arrayInt.length
if that's the case then you should 'break;' the while
loop once the counter i
is more than array length.
I/O operations are costly and though you will get right output you would end-up scanning whole file even if it's not required.
Dont forget try-catch-finally
The code assumes that there will not be any issue and it would be able to close the file after done but there could be n number of combinations that can result in error resulting the application to crash and locking the file.
See the below example:
private Integer readNumbers(String fileName) throws Exception {
Integer arrayInt = new Integer[7];
String str = null;
BufferedReader buffy = new BufferedReader(new FileReader(fileName));
try {
int i=0;
while ((str = buffy.readLine()) != null) {
if(i> arrayInt.length){
break;
}
//get last index of " "
int k = str.lastIndexOf(" ");
if(k > -1){
String nums = str.substring(k+1);
arrayInt[i] = Integer.parseInt(nums);
}
//increment the line counter
i++;
}
} catch (Exception ex) {
//handle exception
} finally {
buffy.close();
}
return arrayInt;
}
answered Nov 29 '18 at 7:17


Hemant SharmaHemant Sharma
305
305
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531572%2fmy-loop-is-populating-my-array-with-the-number-seven-6-times-why%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
wDU8J1LT4nz2NpHWAyqudR6fR6XGy3G0djfOyCVg3J9x wUizo,HSuKGH5JWkkvXWhH1E6IzFvAas
1
Hint: you only need 1 loop to populate a 1-dimensional array.
– shmosel
Nov 29 '18 at 3:53