How to do an integration test on my Asp.Net core web api endpoint where I upload a file?
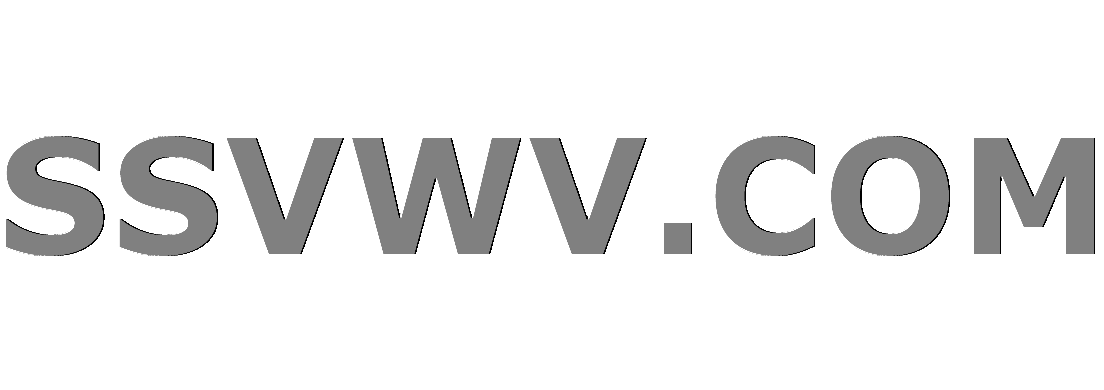
Multi tool use
I am writing an integration test that tests the upload of a file to one of my endpoints and checks if the request result is correct!
I am using IFormFile
in my controller to receive the request, but I am getting a 400 Bad request because apparently my file is null.
How do I allow an integration test to send a file to my endpoint? I found this post but that only talks about mocking IFormFile
, not an integration test.
My controller:
[HttpPost]
public async Task<IActionResult> AddFile(IFormFile file)
{
if (file== null)
{
return StatusCode(400, "A file must be supplied");
}
// ... code that does stuff with the file..
return CreatedAtAction("downloadFile", new { id = MADE_UP_ID }, { MADE_UP_ID };
}
My integration test:
public class IntegrationTest:
IClassFixture<CustomWebApplicationFactory<Startup>>
{
private readonly CustomWebApplicationFactory<Startup> _factory;
public IntegrationTest(CustomWebApplicationFactory<Startup> factory)
{
_factory = factory;
}
[Fact]
public async Task UploadFileTest()
{
// Arrange
var expectedContent = "1";
var expectedContentType = "application/json; charset=utf-8";
var url = "api/bijlages";
var client = _factory.CreateClient();
// Act
var file = System.IO.File.OpenRead(@"C:file.pdf");
HttpContent fileStreamContent = new StreamContent(file);
var formData = new MultipartFormDataContent
{
{ fileStreamContent, "file.pdf", "file.pdf" }
};
var response = await client.PostAsync(url, formData);
fileStreamContent.Dispose();
formData.Dispose();
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
// Assert
Assert.NotEmpty(responseString);
Assert.Equal(expectedContent, responseString);
Assert.Equal(expectedContentType, response.Content.Headers.ContentType.ToString());
}
I hope you guys can help me (and possibly others!) out here!
c# testing asp.net-core integration-testing
add a comment |
I am writing an integration test that tests the upload of a file to one of my endpoints and checks if the request result is correct!
I am using IFormFile
in my controller to receive the request, but I am getting a 400 Bad request because apparently my file is null.
How do I allow an integration test to send a file to my endpoint? I found this post but that only talks about mocking IFormFile
, not an integration test.
My controller:
[HttpPost]
public async Task<IActionResult> AddFile(IFormFile file)
{
if (file== null)
{
return StatusCode(400, "A file must be supplied");
}
// ... code that does stuff with the file..
return CreatedAtAction("downloadFile", new { id = MADE_UP_ID }, { MADE_UP_ID };
}
My integration test:
public class IntegrationTest:
IClassFixture<CustomWebApplicationFactory<Startup>>
{
private readonly CustomWebApplicationFactory<Startup> _factory;
public IntegrationTest(CustomWebApplicationFactory<Startup> factory)
{
_factory = factory;
}
[Fact]
public async Task UploadFileTest()
{
// Arrange
var expectedContent = "1";
var expectedContentType = "application/json; charset=utf-8";
var url = "api/bijlages";
var client = _factory.CreateClient();
// Act
var file = System.IO.File.OpenRead(@"C:file.pdf");
HttpContent fileStreamContent = new StreamContent(file);
var formData = new MultipartFormDataContent
{
{ fileStreamContent, "file.pdf", "file.pdf" }
};
var response = await client.PostAsync(url, formData);
fileStreamContent.Dispose();
formData.Dispose();
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
// Assert
Assert.NotEmpty(responseString);
Assert.Equal(expectedContent, responseString);
Assert.Equal(expectedContentType, response.Content.Headers.ContentType.ToString());
}
I hope you guys can help me (and possibly others!) out here!
c# testing asp.net-core integration-testing
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
MultipartFormDataContent
can sometimes be a little tricky to send correctly
– Nkosi
Nov 23 '18 at 18:02
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07
add a comment |
I am writing an integration test that tests the upload of a file to one of my endpoints and checks if the request result is correct!
I am using IFormFile
in my controller to receive the request, but I am getting a 400 Bad request because apparently my file is null.
How do I allow an integration test to send a file to my endpoint? I found this post but that only talks about mocking IFormFile
, not an integration test.
My controller:
[HttpPost]
public async Task<IActionResult> AddFile(IFormFile file)
{
if (file== null)
{
return StatusCode(400, "A file must be supplied");
}
// ... code that does stuff with the file..
return CreatedAtAction("downloadFile", new { id = MADE_UP_ID }, { MADE_UP_ID };
}
My integration test:
public class IntegrationTest:
IClassFixture<CustomWebApplicationFactory<Startup>>
{
private readonly CustomWebApplicationFactory<Startup> _factory;
public IntegrationTest(CustomWebApplicationFactory<Startup> factory)
{
_factory = factory;
}
[Fact]
public async Task UploadFileTest()
{
// Arrange
var expectedContent = "1";
var expectedContentType = "application/json; charset=utf-8";
var url = "api/bijlages";
var client = _factory.CreateClient();
// Act
var file = System.IO.File.OpenRead(@"C:file.pdf");
HttpContent fileStreamContent = new StreamContent(file);
var formData = new MultipartFormDataContent
{
{ fileStreamContent, "file.pdf", "file.pdf" }
};
var response = await client.PostAsync(url, formData);
fileStreamContent.Dispose();
formData.Dispose();
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
// Assert
Assert.NotEmpty(responseString);
Assert.Equal(expectedContent, responseString);
Assert.Equal(expectedContentType, response.Content.Headers.ContentType.ToString());
}
I hope you guys can help me (and possibly others!) out here!
c# testing asp.net-core integration-testing
I am writing an integration test that tests the upload of a file to one of my endpoints and checks if the request result is correct!
I am using IFormFile
in my controller to receive the request, but I am getting a 400 Bad request because apparently my file is null.
How do I allow an integration test to send a file to my endpoint? I found this post but that only talks about mocking IFormFile
, not an integration test.
My controller:
[HttpPost]
public async Task<IActionResult> AddFile(IFormFile file)
{
if (file== null)
{
return StatusCode(400, "A file must be supplied");
}
// ... code that does stuff with the file..
return CreatedAtAction("downloadFile", new { id = MADE_UP_ID }, { MADE_UP_ID };
}
My integration test:
public class IntegrationTest:
IClassFixture<CustomWebApplicationFactory<Startup>>
{
private readonly CustomWebApplicationFactory<Startup> _factory;
public IntegrationTest(CustomWebApplicationFactory<Startup> factory)
{
_factory = factory;
}
[Fact]
public async Task UploadFileTest()
{
// Arrange
var expectedContent = "1";
var expectedContentType = "application/json; charset=utf-8";
var url = "api/bijlages";
var client = _factory.CreateClient();
// Act
var file = System.IO.File.OpenRead(@"C:file.pdf");
HttpContent fileStreamContent = new StreamContent(file);
var formData = new MultipartFormDataContent
{
{ fileStreamContent, "file.pdf", "file.pdf" }
};
var response = await client.PostAsync(url, formData);
fileStreamContent.Dispose();
formData.Dispose();
response.EnsureSuccessStatusCode();
var responseString = await response.Content.ReadAsStringAsync();
// Assert
Assert.NotEmpty(responseString);
Assert.Equal(expectedContent, responseString);
Assert.Equal(expectedContentType, response.Content.Headers.ContentType.ToString());
}
I hope you guys can help me (and possibly others!) out here!
c# testing asp.net-core integration-testing
c# testing asp.net-core integration-testing
asked Nov 23 '18 at 17:36
S. ten Brinke
365418
365418
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
MultipartFormDataContent
can sometimes be a little tricky to send correctly
– Nkosi
Nov 23 '18 at 18:02
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07
add a comment |
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
MultipartFormDataContent
can sometimes be a little tricky to send correctly
– Nkosi
Nov 23 '18 at 18:02
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
MultipartFormDataContent
can sometimes be a little tricky to send correctly– Nkosi
Nov 23 '18 at 18:02
MultipartFormDataContent
can sometimes be a little tricky to send correctly– Nkosi
Nov 23 '18 at 18:02
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07
add a comment |
1 Answer
1
active
oldest
votes
Your code looks correct except the key in MultipartFormDataContent
should be file
& not file.pdf
Change the formdata to { fileStreamContent, "file", "file.pdf" }
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450941%2fhow-to-do-an-integration-test-on-my-asp-net-core-web-api-endpoint-where-i-upload%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
Your code looks correct except the key in MultipartFormDataContent
should be file
& not file.pdf
Change the formdata to { fileStreamContent, "file", "file.pdf" }
add a comment |
Your code looks correct except the key in MultipartFormDataContent
should be file
& not file.pdf
Change the formdata to { fileStreamContent, "file", "file.pdf" }
add a comment |
Your code looks correct except the key in MultipartFormDataContent
should be file
& not file.pdf
Change the formdata to { fileStreamContent, "file", "file.pdf" }
Your code looks correct except the key in MultipartFormDataContent
should be file
& not file.pdf
Change the formdata to { fileStreamContent, "file", "file.pdf" }
answered Nov 23 '18 at 18:07


Vivian Ajay Monis
746
746
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53450941%2fhow-to-do-an-integration-test-on-my-asp-net-core-web-api-endpoint-where-i-upload%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
V3mIBuChUmuQNaABlSOWBM52EjC8hoa
The referenced link was more an isolated unit test as apposed to an integration test
– Nkosi
Nov 23 '18 at 17:48
Take a look at how content is constructed here stackoverflow.com/a/42216413/5233410
– Nkosi
Nov 23 '18 at 17:55
MultipartFormDataContent
can sometimes be a little tricky to send correctly– Nkosi
Nov 23 '18 at 18:02
can you use a tool like Fiddler and check what is actually the being posted?
– Leonardo
Nov 23 '18 at 18:07