How to remove non-numeric from a string but retain decimal in C#
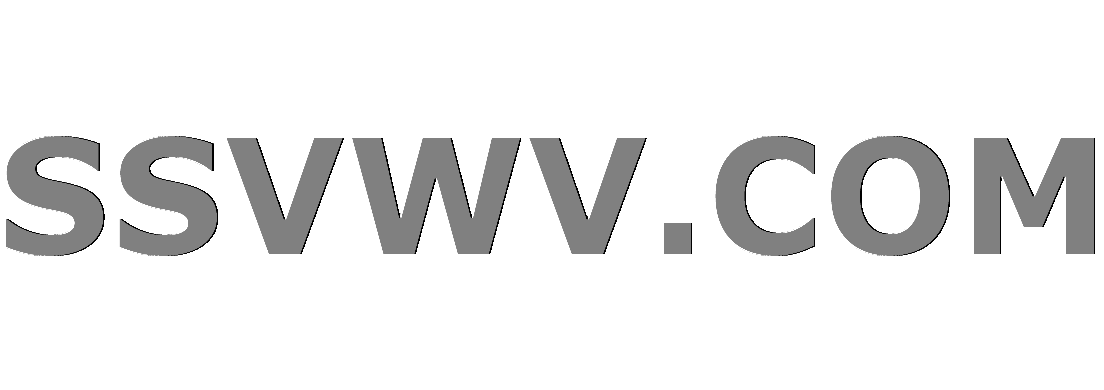
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am using Selenium(C#) on NUnit Framework and is getting a string from UI as $4850.19.
I want to compare above string with the value from backend (DB) to assert they are equal.
I am using a below method to parse my dollar amount from front-end, but the issue is that is also stripping the decimal point; and obviously the comparison with backend is failing.
Method used:
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]))
sb.Append(s[i]);
return sb.ToString();
}
How to strip out any '$' or ',' but keep '.' in the value?
c# selenium selenium-webdriver
add a comment |
I am using Selenium(C#) on NUnit Framework and is getting a string from UI as $4850.19.
I want to compare above string with the value from backend (DB) to assert they are equal.
I am using a below method to parse my dollar amount from front-end, but the issue is that is also stripping the decimal point; and obviously the comparison with backend is failing.
Method used:
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]))
sb.Append(s[i]);
return sb.ToString();
}
How to strip out any '$' or ',' but keep '.' in the value?
c# selenium selenium-webdriver
5
Isn't it as simple asreturn s.Replace("$", String.Empty)
?
– spender
Nov 29 '18 at 4:05
1
Or possiblyreturn s.Substring(1);
?
– Enigmativity
Nov 29 '18 at 4:09
Those methods won't remove the','
character, though...
– Rufus L
Nov 29 '18 at 13:37
add a comment |
I am using Selenium(C#) on NUnit Framework and is getting a string from UI as $4850.19.
I want to compare above string with the value from backend (DB) to assert they are equal.
I am using a below method to parse my dollar amount from front-end, but the issue is that is also stripping the decimal point; and obviously the comparison with backend is failing.
Method used:
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]))
sb.Append(s[i]);
return sb.ToString();
}
How to strip out any '$' or ',' but keep '.' in the value?
c# selenium selenium-webdriver
I am using Selenium(C#) on NUnit Framework and is getting a string from UI as $4850.19.
I want to compare above string with the value from backend (DB) to assert they are equal.
I am using a below method to parse my dollar amount from front-end, but the issue is that is also stripping the decimal point; and obviously the comparison with backend is failing.
Method used:
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]))
sb.Append(s[i]);
return sb.ToString();
}
How to strip out any '$' or ',' but keep '.' in the value?
c# selenium selenium-webdriver
c# selenium selenium-webdriver
asked Nov 29 '18 at 4:00


SymboCoderSymboCoder
558
558
5
Isn't it as simple asreturn s.Replace("$", String.Empty)
?
– spender
Nov 29 '18 at 4:05
1
Or possiblyreturn s.Substring(1);
?
– Enigmativity
Nov 29 '18 at 4:09
Those methods won't remove the','
character, though...
– Rufus L
Nov 29 '18 at 13:37
add a comment |
5
Isn't it as simple asreturn s.Replace("$", String.Empty)
?
– spender
Nov 29 '18 at 4:05
1
Or possiblyreturn s.Substring(1);
?
– Enigmativity
Nov 29 '18 at 4:09
Those methods won't remove the','
character, though...
– Rufus L
Nov 29 '18 at 13:37
5
5
Isn't it as simple as
return s.Replace("$", String.Empty)
?– spender
Nov 29 '18 at 4:05
Isn't it as simple as
return s.Replace("$", String.Empty)
?– spender
Nov 29 '18 at 4:05
1
1
Or possibly
return s.Substring(1);
?– Enigmativity
Nov 29 '18 at 4:09
Or possibly
return s.Substring(1);
?– Enigmativity
Nov 29 '18 at 4:09
Those methods won't remove the
','
character, though...– Rufus L
Nov 29 '18 at 13:37
Those methods won't remove the
','
character, though...– Rufus L
Nov 29 '18 at 13:37
add a comment |
4 Answers
4
active
oldest
votes
With Reg ex it's trivial
Regex.Replace(s, "[^0-9.]", "")
add a comment |
You can also use the decimal.Parse
method to parse a string
formatted as currency into a decimal
type:
string input = "$4,850.19";
decimal result = decimal.Parse(input, NumberStyles.Currency);
Console.WriteLine($"{input} => {result}");
Output:
add a comment |
Another way to do it if you don't want to go the decimal.Parse
route is to simply return only numeric and '.'
characters from the string:
public static string RemoveNonNumeric2(string s)
{
return string.Concat(s?.Where(c => char.IsNumber(c) || c == '.') ?? "");
}
add a comment |
Thanks for all inputs above, I kind of figured out an easy way to handle this for now (as shown below) -
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]) || s[i] == '.')
sb.Append(s[i]);
return sb.ToString();
}
I would like to try other ways of handling this as well soon.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531638%2fhow-to-remove-non-numeric-from-a-string-but-retain-decimal-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
4 Answers
4
active
oldest
votes
4 Answers
4
active
oldest
votes
active
oldest
votes
active
oldest
votes
With Reg ex it's trivial
Regex.Replace(s, "[^0-9.]", "")
add a comment |
With Reg ex it's trivial
Regex.Replace(s, "[^0-9.]", "")
add a comment |
With Reg ex it's trivial
Regex.Replace(s, "[^0-9.]", "")
With Reg ex it's trivial
Regex.Replace(s, "[^0-9.]", "")
answered Nov 29 '18 at 4:19
Vadim AlekseevskyVadim Alekseevsky
38219
38219
add a comment |
add a comment |
You can also use the decimal.Parse
method to parse a string
formatted as currency into a decimal
type:
string input = "$4,850.19";
decimal result = decimal.Parse(input, NumberStyles.Currency);
Console.WriteLine($"{input} => {result}");
Output:
add a comment |
You can also use the decimal.Parse
method to parse a string
formatted as currency into a decimal
type:
string input = "$4,850.19";
decimal result = decimal.Parse(input, NumberStyles.Currency);
Console.WriteLine($"{input} => {result}");
Output:
add a comment |
You can also use the decimal.Parse
method to parse a string
formatted as currency into a decimal
type:
string input = "$4,850.19";
decimal result = decimal.Parse(input, NumberStyles.Currency);
Console.WriteLine($"{input} => {result}");
Output:
You can also use the decimal.Parse
method to parse a string
formatted as currency into a decimal
type:
string input = "$4,850.19";
decimal result = decimal.Parse(input, NumberStyles.Currency);
Console.WriteLine($"{input} => {result}");
Output:
answered Nov 29 '18 at 13:36


Rufus LRufus L
19.3k31732
19.3k31732
add a comment |
add a comment |
Another way to do it if you don't want to go the decimal.Parse
route is to simply return only numeric and '.'
characters from the string:
public static string RemoveNonNumeric2(string s)
{
return string.Concat(s?.Where(c => char.IsNumber(c) || c == '.') ?? "");
}
add a comment |
Another way to do it if you don't want to go the decimal.Parse
route is to simply return only numeric and '.'
characters from the string:
public static string RemoveNonNumeric2(string s)
{
return string.Concat(s?.Where(c => char.IsNumber(c) || c == '.') ?? "");
}
add a comment |
Another way to do it if you don't want to go the decimal.Parse
route is to simply return only numeric and '.'
characters from the string:
public static string RemoveNonNumeric2(string s)
{
return string.Concat(s?.Where(c => char.IsNumber(c) || c == '.') ?? "");
}
Another way to do it if you don't want to go the decimal.Parse
route is to simply return only numeric and '.'
characters from the string:
public static string RemoveNonNumeric2(string s)
{
return string.Concat(s?.Where(c => char.IsNumber(c) || c == '.') ?? "");
}
answered Dec 3 '18 at 5:29


Rufus LRufus L
19.3k31732
19.3k31732
add a comment |
add a comment |
Thanks for all inputs above, I kind of figured out an easy way to handle this for now (as shown below) -
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]) || s[i] == '.')
sb.Append(s[i]);
return sb.ToString();
}
I would like to try other ways of handling this as well soon.
add a comment |
Thanks for all inputs above, I kind of figured out an easy way to handle this for now (as shown below) -
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]) || s[i] == '.')
sb.Append(s[i]);
return sb.ToString();
}
I would like to try other ways of handling this as well soon.
add a comment |
Thanks for all inputs above, I kind of figured out an easy way to handle this for now (as shown below) -
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]) || s[i] == '.')
sb.Append(s[i]);
return sb.ToString();
}
I would like to try other ways of handling this as well soon.
Thanks for all inputs above, I kind of figured out an easy way to handle this for now (as shown below) -
public static string RemoveNonNumeric(string s)
{
StringBuilder sb = new StringBuilder();
for (int i = 0; i < s.Length; i++)
if (Char.IsNumber(s[i]) || s[i] == '.')
sb.Append(s[i]);
return sb.ToString();
}
I would like to try other ways of handling this as well soon.
answered Dec 3 '18 at 1:01


SymboCoderSymboCoder
558
558
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53531638%2fhow-to-remove-non-numeric-from-a-string-but-retain-decimal-in-c-sharp%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
2XxoaWgCgHkeM5q w bKm3GNQn,7,Npl8 zYXbmYGs
5
Isn't it as simple as
return s.Replace("$", String.Empty)
?– spender
Nov 29 '18 at 4:05
1
Or possibly
return s.Substring(1);
?– Enigmativity
Nov 29 '18 at 4:09
Those methods won't remove the
','
character, though...– Rufus L
Nov 29 '18 at 13:37