How to reference and modify a std::unique_ptr's underlying value in another function?
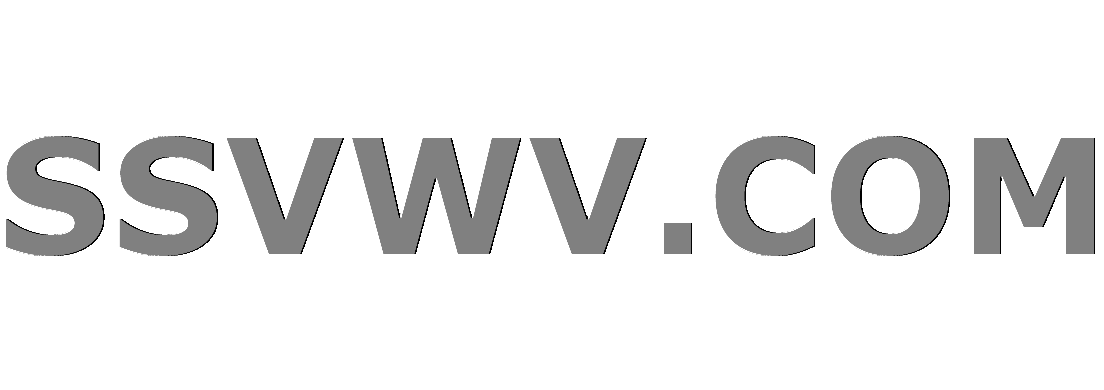
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I am trying to modify the value within a unique_ptr, but inside another function which doesn't actually own the unique_ptr, as follows:
void my_func(Foo* foo) {
foo->set_bar("a");
}
int main() {
auto foo = std::MakeUnique<Foo>();
my_func(foo.get());
cout << foo->bar;
}
I thought this would work, except I'm met with an error complaining of a deleted constructor. Is there a way to make this pattern work?
c++ unique-ptr
add a comment |
I am trying to modify the value within a unique_ptr, but inside another function which doesn't actually own the unique_ptr, as follows:
void my_func(Foo* foo) {
foo->set_bar("a");
}
int main() {
auto foo = std::MakeUnique<Foo>();
my_func(foo.get());
cout << foo->bar;
}
I thought this would work, except I'm met with an error complaining of a deleted constructor. Is there a way to make this pattern work?
c++ unique-ptr
what is aMakeUnique
? What is aFoo
? Please post a Minimal, Complete, and Verifiable example
– xaxxon
Nov 29 '18 at 1:33
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Unrelated suggestion: If you makemy_func
look more likevoid my_func(const std::unique_ptr<Foo> & foo)
, the ownership offoo
remains clear. Last thing you want is some knucklehead coming along and dropping adelete foo;
somewhere in there.
– user4581301
Nov 29 '18 at 1:51
add a comment |
I am trying to modify the value within a unique_ptr, but inside another function which doesn't actually own the unique_ptr, as follows:
void my_func(Foo* foo) {
foo->set_bar("a");
}
int main() {
auto foo = std::MakeUnique<Foo>();
my_func(foo.get());
cout << foo->bar;
}
I thought this would work, except I'm met with an error complaining of a deleted constructor. Is there a way to make this pattern work?
c++ unique-ptr
I am trying to modify the value within a unique_ptr, but inside another function which doesn't actually own the unique_ptr, as follows:
void my_func(Foo* foo) {
foo->set_bar("a");
}
int main() {
auto foo = std::MakeUnique<Foo>();
my_func(foo.get());
cout << foo->bar;
}
I thought this would work, except I'm met with an error complaining of a deleted constructor. Is there a way to make this pattern work?
c++ unique-ptr
c++ unique-ptr
asked Nov 29 '18 at 1:29
bonnoz05bonnoz05
71
71
what is aMakeUnique
? What is aFoo
? Please post a Minimal, Complete, and Verifiable example
– xaxxon
Nov 29 '18 at 1:33
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Unrelated suggestion: If you makemy_func
look more likevoid my_func(const std::unique_ptr<Foo> & foo)
, the ownership offoo
remains clear. Last thing you want is some knucklehead coming along and dropping adelete foo;
somewhere in there.
– user4581301
Nov 29 '18 at 1:51
add a comment |
what is aMakeUnique
? What is aFoo
? Please post a Minimal, Complete, and Verifiable example
– xaxxon
Nov 29 '18 at 1:33
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Unrelated suggestion: If you makemy_func
look more likevoid my_func(const std::unique_ptr<Foo> & foo)
, the ownership offoo
remains clear. Last thing you want is some knucklehead coming along and dropping adelete foo;
somewhere in there.
– user4581301
Nov 29 '18 at 1:51
what is a
MakeUnique
? What is a Foo
? Please post a Minimal, Complete, and Verifiable example– xaxxon
Nov 29 '18 at 1:33
what is a
MakeUnique
? What is a Foo
? Please post a Minimal, Complete, and Verifiable example– xaxxon
Nov 29 '18 at 1:33
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Unrelated suggestion: If you make
my_func
look more like void my_func(const std::unique_ptr<Foo> & foo)
, the ownership of foo
remains clear. Last thing you want is some knucklehead coming along and dropping a delete foo;
somewhere in there.– user4581301
Nov 29 '18 at 1:51
Unrelated suggestion: If you make
my_func
look more like void my_func(const std::unique_ptr<Foo> & foo)
, the ownership of foo
remains clear. Last thing you want is some knucklehead coming along and dropping a delete foo;
somewhere in there.– user4581301
Nov 29 '18 at 1:51
add a comment |
1 Answer
1
active
oldest
votes
I will assume you are actually using std::make_unique<Foo>()
.
Since it is an error with construction, this is most likely a problem with the Foo
type, not with what you are trying to do with it. Although, it could also stem from the set_bar()
function.
Using std::make_unique<Foo>()
will attempt to use the default constructor (the one with no arguments). It would appear that your Foo
type does not have a default constructor. Although, without the implementation of Foo
at hand, this is just a guess. You need to give std::make_unique<Foo>()
arguments that correspond to one of the defined constructors of Foo 1.
Re: What you are trying to do - this seems like it should work. Although, since it is taking a raw pointer, you should consider doing some nullptr
checks.
1: If there is at least one constructor defined, where one is not the default constructor, the default constructor is deleted.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530571%2fhow-to-reference-and-modify-a-stdunique-ptrs-underlying-value-in-another-func%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
I will assume you are actually using std::make_unique<Foo>()
.
Since it is an error with construction, this is most likely a problem with the Foo
type, not with what you are trying to do with it. Although, it could also stem from the set_bar()
function.
Using std::make_unique<Foo>()
will attempt to use the default constructor (the one with no arguments). It would appear that your Foo
type does not have a default constructor. Although, without the implementation of Foo
at hand, this is just a guess. You need to give std::make_unique<Foo>()
arguments that correspond to one of the defined constructors of Foo 1.
Re: What you are trying to do - this seems like it should work. Although, since it is taking a raw pointer, you should consider doing some nullptr
checks.
1: If there is at least one constructor defined, where one is not the default constructor, the default constructor is deleted.
add a comment |
I will assume you are actually using std::make_unique<Foo>()
.
Since it is an error with construction, this is most likely a problem with the Foo
type, not with what you are trying to do with it. Although, it could also stem from the set_bar()
function.
Using std::make_unique<Foo>()
will attempt to use the default constructor (the one with no arguments). It would appear that your Foo
type does not have a default constructor. Although, without the implementation of Foo
at hand, this is just a guess. You need to give std::make_unique<Foo>()
arguments that correspond to one of the defined constructors of Foo 1.
Re: What you are trying to do - this seems like it should work. Although, since it is taking a raw pointer, you should consider doing some nullptr
checks.
1: If there is at least one constructor defined, where one is not the default constructor, the default constructor is deleted.
add a comment |
I will assume you are actually using std::make_unique<Foo>()
.
Since it is an error with construction, this is most likely a problem with the Foo
type, not with what you are trying to do with it. Although, it could also stem from the set_bar()
function.
Using std::make_unique<Foo>()
will attempt to use the default constructor (the one with no arguments). It would appear that your Foo
type does not have a default constructor. Although, without the implementation of Foo
at hand, this is just a guess. You need to give std::make_unique<Foo>()
arguments that correspond to one of the defined constructors of Foo 1.
Re: What you are trying to do - this seems like it should work. Although, since it is taking a raw pointer, you should consider doing some nullptr
checks.
1: If there is at least one constructor defined, where one is not the default constructor, the default constructor is deleted.
I will assume you are actually using std::make_unique<Foo>()
.
Since it is an error with construction, this is most likely a problem with the Foo
type, not with what you are trying to do with it. Although, it could also stem from the set_bar()
function.
Using std::make_unique<Foo>()
will attempt to use the default constructor (the one with no arguments). It would appear that your Foo
type does not have a default constructor. Although, without the implementation of Foo
at hand, this is just a guess. You need to give std::make_unique<Foo>()
arguments that correspond to one of the defined constructors of Foo 1.
Re: What you are trying to do - this seems like it should work. Although, since it is taking a raw pointer, you should consider doing some nullptr
checks.
1: If there is at least one constructor defined, where one is not the default constructor, the default constructor is deleted.
edited Nov 29 '18 at 2:01
Remy Lebeau
342k19267461
342k19267461
answered Nov 29 '18 at 1:53
Bar StoolBar Stool
1735
1735
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530571%2fhow-to-reference-and-modify-a-stdunique-ptrs-underlying-value-in-another-func%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
vGAibLVM1sObUCr1R1jB bA8IZxTTU3 VzVc4ri s,g2Nrj1BJhzADr jDHXBThQ OTl3J6OUSp1LXUmPdf
what is a
MakeUnique
? What is aFoo
? Please post a Minimal, Complete, and Verifiable example– xaxxon
Nov 29 '18 at 1:33
Inside? That's a pointer. Managed by? That's what the pointer refers to.
– Deduplicator
Nov 29 '18 at 1:41
Once I make the required corrections and fill in the blanks I'm not seeing any problems: ideone.com/zZwgZv . Minimal, Complete, and Verifiable example definitely required.
– user4581301
Nov 29 '18 at 1:45
Unrelated suggestion: If you make
my_func
look more likevoid my_func(const std::unique_ptr<Foo> & foo)
, the ownership offoo
remains clear. Last thing you want is some knucklehead coming along and dropping adelete foo;
somewhere in there.– user4581301
Nov 29 '18 at 1:51