Cant get anything to show on the screen in OpenGL simple program only background color
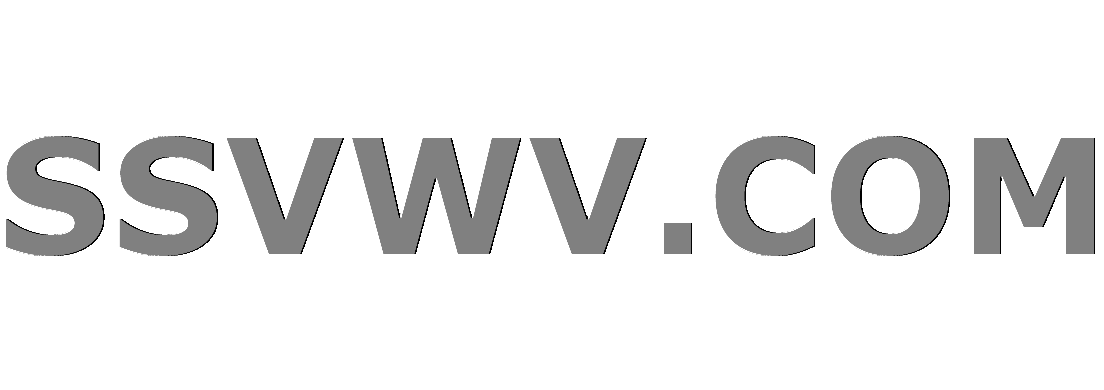
Multi tool use
.everyoneloves__top-leaderboard:empty,.everyoneloves__mid-leaderboard:empty,.everyoneloves__bot-mid-leaderboard:empty{ height:90px;width:728px;box-sizing:border-box;
}
I had glCreateProgram()
return error 1282 before but fixed it after removing context glfwWindowsHint
calls. But even after that nothing is showing up on the screen! Here is my shader code:
Shader.h
#pragma once
#include <iostream>
#include "file_utils.h"
#include "GL/glew.h"
class Shader {
private:
GLuint shaderID;
const char *vertPath;
const char *fragPath;
public:
Shader(const char *vertexPath, const char *fragmentPath);
~Shader();
void enable() const;
void disable() const;
private:
GLuint load();
};
Shader.cpp
#include "shader.h"
Shader::Shader(const char *vertexPath, const char *fragmentPath)
{
vertPath = vertexPath;
fragPath = fragmentPath;
shaderID = load();
}
Shader::~Shader()
{
glDeleteProgram(shaderID);
}
GLuint Shader::load()
{
GLuint program = glCreateProgram();
GLuint vertex = glCreateShader(GL_VERTEX_SHADER);
GLuint fragment = glCreateShader(GL_FRAGMENT_SHADER);
std::string g_vert = FileUtils::readFile(vertPath);
std::string g_frag = FileUtils::readFile(fragPath);
const char *vertSource = g_vert.c_str();
const char *fragSource = g_frag.c_str();
glShaderSource(vertex, 1, &vertSource, NULL);
glCompileShader(vertex);
GLchar infoL[1024];
GLint result;
glGetShaderiv(vertex, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(vertex, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING VERTEX SHADER: " << infoL << std::endl;
glDeleteShader(vertex);
return 0;
}
glShaderSource(fragment, 1, &fragSource, NULL);
glCompileShader(fragment);
glGetShaderiv(fragment, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(fragment, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING FRAGMENT SHADER: " << infoL << std::endl;
glDeleteShader(fragment);
return 0;
}
glAttachShader(program, vertex);
glAttachShader(program, fragment);
glLinkProgram(program);
glValidateProgram(program);
glDeleteShader(vertex);
glDeleteShader(fragment);
return program;
}
void Shader::enable() const
{
glUseProgram(shaderID);
}
void Shader::disable() const
{
glUseProgram(0);
}
vertex.glsl
#version 330 core
layout (location = 0) in vec4 position;
uniform mat4 pr_matrix;
uniform mat4 vw_matrix = mat4(1.0);
uniform mat4 ml_matrix = mat4(1.0);
void main()
{
gl_Position = position;
}
fragment.glsl
#version 330 core
layout (location = 0) out vec4 color;
void main()
{
color = vec4(1.0, 0.0, 1.0, 1.0);
}
And finally here is main:
#include <iostream>
#include "WindowCreation.h"
#include "Maths.h"
#include "shader.h"
const int SCREEN_WIDTH = 800, SCREEN_HEIGHT = 600;
int main(){
WindowCreation *mainWindow = new WindowCreation();
if (mainWindow->createWindow(&SCREEN_WIDTH, &SCREEN_HEIGHT, "GoldSpark Engine") == 0)
{
glClearColor(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
cout << "Could not create window. EXITING..." << endl;
return 1;
}
GLfloat vertices = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vao;
glGenVertexArrays(1, &vao);
glBindVertexArray(vao);
GLuint vbo = 0;
glGenBuffers(1, &vbo);
glBindBuffer(GL_ARRAY_BUFFER, vbo);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(0);
Shader shaders("resources/shaders/vertex.glsl", "resources/shaders/fragment.glsl");
shaders.enable();
while(!mainWindow->getShouldClose())
{
mainWindow->clear();
glDrawArrays(GL_TRIANGLES, 0, 6);
mainWindow->update();
}
shaders.disable();
glBindBuffer(GL_ARRAY_BUFFER, 0);
delete mainWindow;
return 0;
}
WindowCreation.h
#pragma once
#include <iostream>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
using namespace std;
class WindowCreation
{
public:
int bufferWidth, bufferHeight;
WindowCreation();
~WindowCreation();
bool getShouldClose() { return glfwWindowShouldClose(window); }
void createCallBacks();
GLfloat getXChange() {
GLfloat xCh = xChange;
xChange = 0.0f;
return xCh;
}
GLfloat getYChange() {
GLfloat yCh = yChange;
yChange = 0.0f;
return yCh;
}
bool *getKeyPress() { return keys; }
void update() {
glfwPollEvents();
glClear(GL_COLOR_BUFFER_BIT);
}
void clear() {
glfwSwapBuffers(window);
}
int createWindow(const int *screenWidth, const int *screenHeight, const char* title); // ovdje pravimo sve sto treba da se prozor pojavi
GLFWwindow *getWindow() { return window; }
private:
GLFWwindow *window;
bool firstTime;
bool keys[1024];
GLfloat lastX, lastY, xChange, yChange;
static void handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode);
static void handleMouse(GLFWwindow* window, double xPos, double yPos);
};
WindowCreation.cpp
#include "WindowCreation.h"
WindowCreation::WindowCreation()
{
//sve na 0 postavimo prvo
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
xChange = 0.0f;
yChange = 0.0f;
firstTime = true;
}
void WindowCreation::createCallBacks()
{
glfwSetKeyCallback(getWindow(), handleKeyboard);
glfwSetCursorPosCallback(getWindow(), handleMouse);
}
void WindowCreation::handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
{
glfwSetWindowShouldClose(thisWindow->window, GLFW_TRUE);
}
if (key >= 0 && key <= 1024)
{
if (action == GLFW_PRESS)
{
thisWindow->keys[key] = true;
}
else if (action == GLFW_RELEASE)
{
thisWindow->keys[key] = false;
}
}
}
void WindowCreation::handleMouse(GLFWwindow* window, double xPos, double yPos)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (thisWindow->firstTime)
{
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
thisWindow->firstTime = false;
}
thisWindow->xChange = xPos - thisWindow->lastX;
thisWindow->yChange = thisWindow->lastY - yPos;
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
}
int WindowCreation::createWindow(const int *screenWidth,const int *screenHeight, const char* title)
{
bufferWidth = 0;
bufferHeight = 0;
if (!glfwInit()) // inicijalizujemo glfw
{
cout << "Could not initialize glfw." << endl;
glfwTerminate();
return 1;
}
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GLFW_TRUE);
window = glfwCreateWindow(*screenWidth, *screenHeight, title, NULL, NULL);
glfwGetFramebufferSize(window, &bufferWidth, &bufferHeight);
if (!window)
{
cout << "Window could not be created." << endl;
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK)
{
cout << "Could not initialize GLEW";
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glewExperimental = true;
glViewport(0, 0, bufferWidth, bufferHeight);
glfwSetWindowUserPointer(window, this);
createCallBacks();
return 0;
}
WindowCreation::~WindowCreation()
{
glfwDestroyWindow(window);
glfwTerminate();
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
window = nullptr;
xChange = 0.0f;
yChange = 0.0f;
lastX = 0.0f;
lastY = 0.0f;
}
I have no idea what I did wrong here. This is very simple as you can see and shader code should be running . I've checked file loaders strings that it returns and it is correct just like in those .glsl files.
In function mainWindow->update()
is glfwSwapBuffers(window)
and in function mainWindow->clear()
is in order: glClear(GL_COLOR_BUFFER_BIT);
glfwPollEvents();
Also those info logs aren't returning anything. Window does show up but its all blue there is no square in the middle.
c++ opengl graphics shader graphics2d
|
show 3 more comments
I had glCreateProgram()
return error 1282 before but fixed it after removing context glfwWindowsHint
calls. But even after that nothing is showing up on the screen! Here is my shader code:
Shader.h
#pragma once
#include <iostream>
#include "file_utils.h"
#include "GL/glew.h"
class Shader {
private:
GLuint shaderID;
const char *vertPath;
const char *fragPath;
public:
Shader(const char *vertexPath, const char *fragmentPath);
~Shader();
void enable() const;
void disable() const;
private:
GLuint load();
};
Shader.cpp
#include "shader.h"
Shader::Shader(const char *vertexPath, const char *fragmentPath)
{
vertPath = vertexPath;
fragPath = fragmentPath;
shaderID = load();
}
Shader::~Shader()
{
glDeleteProgram(shaderID);
}
GLuint Shader::load()
{
GLuint program = glCreateProgram();
GLuint vertex = glCreateShader(GL_VERTEX_SHADER);
GLuint fragment = glCreateShader(GL_FRAGMENT_SHADER);
std::string g_vert = FileUtils::readFile(vertPath);
std::string g_frag = FileUtils::readFile(fragPath);
const char *vertSource = g_vert.c_str();
const char *fragSource = g_frag.c_str();
glShaderSource(vertex, 1, &vertSource, NULL);
glCompileShader(vertex);
GLchar infoL[1024];
GLint result;
glGetShaderiv(vertex, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(vertex, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING VERTEX SHADER: " << infoL << std::endl;
glDeleteShader(vertex);
return 0;
}
glShaderSource(fragment, 1, &fragSource, NULL);
glCompileShader(fragment);
glGetShaderiv(fragment, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(fragment, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING FRAGMENT SHADER: " << infoL << std::endl;
glDeleteShader(fragment);
return 0;
}
glAttachShader(program, vertex);
glAttachShader(program, fragment);
glLinkProgram(program);
glValidateProgram(program);
glDeleteShader(vertex);
glDeleteShader(fragment);
return program;
}
void Shader::enable() const
{
glUseProgram(shaderID);
}
void Shader::disable() const
{
glUseProgram(0);
}
vertex.glsl
#version 330 core
layout (location = 0) in vec4 position;
uniform mat4 pr_matrix;
uniform mat4 vw_matrix = mat4(1.0);
uniform mat4 ml_matrix = mat4(1.0);
void main()
{
gl_Position = position;
}
fragment.glsl
#version 330 core
layout (location = 0) out vec4 color;
void main()
{
color = vec4(1.0, 0.0, 1.0, 1.0);
}
And finally here is main:
#include <iostream>
#include "WindowCreation.h"
#include "Maths.h"
#include "shader.h"
const int SCREEN_WIDTH = 800, SCREEN_HEIGHT = 600;
int main(){
WindowCreation *mainWindow = new WindowCreation();
if (mainWindow->createWindow(&SCREEN_WIDTH, &SCREEN_HEIGHT, "GoldSpark Engine") == 0)
{
glClearColor(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
cout << "Could not create window. EXITING..." << endl;
return 1;
}
GLfloat vertices = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vao;
glGenVertexArrays(1, &vao);
glBindVertexArray(vao);
GLuint vbo = 0;
glGenBuffers(1, &vbo);
glBindBuffer(GL_ARRAY_BUFFER, vbo);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(0);
Shader shaders("resources/shaders/vertex.glsl", "resources/shaders/fragment.glsl");
shaders.enable();
while(!mainWindow->getShouldClose())
{
mainWindow->clear();
glDrawArrays(GL_TRIANGLES, 0, 6);
mainWindow->update();
}
shaders.disable();
glBindBuffer(GL_ARRAY_BUFFER, 0);
delete mainWindow;
return 0;
}
WindowCreation.h
#pragma once
#include <iostream>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
using namespace std;
class WindowCreation
{
public:
int bufferWidth, bufferHeight;
WindowCreation();
~WindowCreation();
bool getShouldClose() { return glfwWindowShouldClose(window); }
void createCallBacks();
GLfloat getXChange() {
GLfloat xCh = xChange;
xChange = 0.0f;
return xCh;
}
GLfloat getYChange() {
GLfloat yCh = yChange;
yChange = 0.0f;
return yCh;
}
bool *getKeyPress() { return keys; }
void update() {
glfwPollEvents();
glClear(GL_COLOR_BUFFER_BIT);
}
void clear() {
glfwSwapBuffers(window);
}
int createWindow(const int *screenWidth, const int *screenHeight, const char* title); // ovdje pravimo sve sto treba da se prozor pojavi
GLFWwindow *getWindow() { return window; }
private:
GLFWwindow *window;
bool firstTime;
bool keys[1024];
GLfloat lastX, lastY, xChange, yChange;
static void handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode);
static void handleMouse(GLFWwindow* window, double xPos, double yPos);
};
WindowCreation.cpp
#include "WindowCreation.h"
WindowCreation::WindowCreation()
{
//sve na 0 postavimo prvo
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
xChange = 0.0f;
yChange = 0.0f;
firstTime = true;
}
void WindowCreation::createCallBacks()
{
glfwSetKeyCallback(getWindow(), handleKeyboard);
glfwSetCursorPosCallback(getWindow(), handleMouse);
}
void WindowCreation::handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
{
glfwSetWindowShouldClose(thisWindow->window, GLFW_TRUE);
}
if (key >= 0 && key <= 1024)
{
if (action == GLFW_PRESS)
{
thisWindow->keys[key] = true;
}
else if (action == GLFW_RELEASE)
{
thisWindow->keys[key] = false;
}
}
}
void WindowCreation::handleMouse(GLFWwindow* window, double xPos, double yPos)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (thisWindow->firstTime)
{
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
thisWindow->firstTime = false;
}
thisWindow->xChange = xPos - thisWindow->lastX;
thisWindow->yChange = thisWindow->lastY - yPos;
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
}
int WindowCreation::createWindow(const int *screenWidth,const int *screenHeight, const char* title)
{
bufferWidth = 0;
bufferHeight = 0;
if (!glfwInit()) // inicijalizujemo glfw
{
cout << "Could not initialize glfw." << endl;
glfwTerminate();
return 1;
}
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GLFW_TRUE);
window = glfwCreateWindow(*screenWidth, *screenHeight, title, NULL, NULL);
glfwGetFramebufferSize(window, &bufferWidth, &bufferHeight);
if (!window)
{
cout << "Window could not be created." << endl;
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK)
{
cout << "Could not initialize GLEW";
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glewExperimental = true;
glViewport(0, 0, bufferWidth, bufferHeight);
glfwSetWindowUserPointer(window, this);
createCallBacks();
return 0;
}
WindowCreation::~WindowCreation()
{
glfwDestroyWindow(window);
glfwTerminate();
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
window = nullptr;
xChange = 0.0f;
yChange = 0.0f;
lastX = 0.0f;
lastY = 0.0f;
}
I have no idea what I did wrong here. This is very simple as you can see and shader code should be running . I've checked file loaders strings that it returns and it is correct just like in those .glsl files.
In function mainWindow->update()
is glfwSwapBuffers(window)
and in function mainWindow->clear()
is in order: glClear(GL_COLOR_BUFFER_BIT);
glfwPollEvents();
Also those info logs aren't returning anything. Window does show up but its all blue there is no square in the middle.
c++ opengl graphics shader graphics2d
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
1
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00
|
show 3 more comments
I had glCreateProgram()
return error 1282 before but fixed it after removing context glfwWindowsHint
calls. But even after that nothing is showing up on the screen! Here is my shader code:
Shader.h
#pragma once
#include <iostream>
#include "file_utils.h"
#include "GL/glew.h"
class Shader {
private:
GLuint shaderID;
const char *vertPath;
const char *fragPath;
public:
Shader(const char *vertexPath, const char *fragmentPath);
~Shader();
void enable() const;
void disable() const;
private:
GLuint load();
};
Shader.cpp
#include "shader.h"
Shader::Shader(const char *vertexPath, const char *fragmentPath)
{
vertPath = vertexPath;
fragPath = fragmentPath;
shaderID = load();
}
Shader::~Shader()
{
glDeleteProgram(shaderID);
}
GLuint Shader::load()
{
GLuint program = glCreateProgram();
GLuint vertex = glCreateShader(GL_VERTEX_SHADER);
GLuint fragment = glCreateShader(GL_FRAGMENT_SHADER);
std::string g_vert = FileUtils::readFile(vertPath);
std::string g_frag = FileUtils::readFile(fragPath);
const char *vertSource = g_vert.c_str();
const char *fragSource = g_frag.c_str();
glShaderSource(vertex, 1, &vertSource, NULL);
glCompileShader(vertex);
GLchar infoL[1024];
GLint result;
glGetShaderiv(vertex, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(vertex, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING VERTEX SHADER: " << infoL << std::endl;
glDeleteShader(vertex);
return 0;
}
glShaderSource(fragment, 1, &fragSource, NULL);
glCompileShader(fragment);
glGetShaderiv(fragment, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(fragment, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING FRAGMENT SHADER: " << infoL << std::endl;
glDeleteShader(fragment);
return 0;
}
glAttachShader(program, vertex);
glAttachShader(program, fragment);
glLinkProgram(program);
glValidateProgram(program);
glDeleteShader(vertex);
glDeleteShader(fragment);
return program;
}
void Shader::enable() const
{
glUseProgram(shaderID);
}
void Shader::disable() const
{
glUseProgram(0);
}
vertex.glsl
#version 330 core
layout (location = 0) in vec4 position;
uniform mat4 pr_matrix;
uniform mat4 vw_matrix = mat4(1.0);
uniform mat4 ml_matrix = mat4(1.0);
void main()
{
gl_Position = position;
}
fragment.glsl
#version 330 core
layout (location = 0) out vec4 color;
void main()
{
color = vec4(1.0, 0.0, 1.0, 1.0);
}
And finally here is main:
#include <iostream>
#include "WindowCreation.h"
#include "Maths.h"
#include "shader.h"
const int SCREEN_WIDTH = 800, SCREEN_HEIGHT = 600;
int main(){
WindowCreation *mainWindow = new WindowCreation();
if (mainWindow->createWindow(&SCREEN_WIDTH, &SCREEN_HEIGHT, "GoldSpark Engine") == 0)
{
glClearColor(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
cout << "Could not create window. EXITING..." << endl;
return 1;
}
GLfloat vertices = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vao;
glGenVertexArrays(1, &vao);
glBindVertexArray(vao);
GLuint vbo = 0;
glGenBuffers(1, &vbo);
glBindBuffer(GL_ARRAY_BUFFER, vbo);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(0);
Shader shaders("resources/shaders/vertex.glsl", "resources/shaders/fragment.glsl");
shaders.enable();
while(!mainWindow->getShouldClose())
{
mainWindow->clear();
glDrawArrays(GL_TRIANGLES, 0, 6);
mainWindow->update();
}
shaders.disable();
glBindBuffer(GL_ARRAY_BUFFER, 0);
delete mainWindow;
return 0;
}
WindowCreation.h
#pragma once
#include <iostream>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
using namespace std;
class WindowCreation
{
public:
int bufferWidth, bufferHeight;
WindowCreation();
~WindowCreation();
bool getShouldClose() { return glfwWindowShouldClose(window); }
void createCallBacks();
GLfloat getXChange() {
GLfloat xCh = xChange;
xChange = 0.0f;
return xCh;
}
GLfloat getYChange() {
GLfloat yCh = yChange;
yChange = 0.0f;
return yCh;
}
bool *getKeyPress() { return keys; }
void update() {
glfwPollEvents();
glClear(GL_COLOR_BUFFER_BIT);
}
void clear() {
glfwSwapBuffers(window);
}
int createWindow(const int *screenWidth, const int *screenHeight, const char* title); // ovdje pravimo sve sto treba da se prozor pojavi
GLFWwindow *getWindow() { return window; }
private:
GLFWwindow *window;
bool firstTime;
bool keys[1024];
GLfloat lastX, lastY, xChange, yChange;
static void handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode);
static void handleMouse(GLFWwindow* window, double xPos, double yPos);
};
WindowCreation.cpp
#include "WindowCreation.h"
WindowCreation::WindowCreation()
{
//sve na 0 postavimo prvo
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
xChange = 0.0f;
yChange = 0.0f;
firstTime = true;
}
void WindowCreation::createCallBacks()
{
glfwSetKeyCallback(getWindow(), handleKeyboard);
glfwSetCursorPosCallback(getWindow(), handleMouse);
}
void WindowCreation::handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
{
glfwSetWindowShouldClose(thisWindow->window, GLFW_TRUE);
}
if (key >= 0 && key <= 1024)
{
if (action == GLFW_PRESS)
{
thisWindow->keys[key] = true;
}
else if (action == GLFW_RELEASE)
{
thisWindow->keys[key] = false;
}
}
}
void WindowCreation::handleMouse(GLFWwindow* window, double xPos, double yPos)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (thisWindow->firstTime)
{
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
thisWindow->firstTime = false;
}
thisWindow->xChange = xPos - thisWindow->lastX;
thisWindow->yChange = thisWindow->lastY - yPos;
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
}
int WindowCreation::createWindow(const int *screenWidth,const int *screenHeight, const char* title)
{
bufferWidth = 0;
bufferHeight = 0;
if (!glfwInit()) // inicijalizujemo glfw
{
cout << "Could not initialize glfw." << endl;
glfwTerminate();
return 1;
}
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GLFW_TRUE);
window = glfwCreateWindow(*screenWidth, *screenHeight, title, NULL, NULL);
glfwGetFramebufferSize(window, &bufferWidth, &bufferHeight);
if (!window)
{
cout << "Window could not be created." << endl;
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK)
{
cout << "Could not initialize GLEW";
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glewExperimental = true;
glViewport(0, 0, bufferWidth, bufferHeight);
glfwSetWindowUserPointer(window, this);
createCallBacks();
return 0;
}
WindowCreation::~WindowCreation()
{
glfwDestroyWindow(window);
glfwTerminate();
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
window = nullptr;
xChange = 0.0f;
yChange = 0.0f;
lastX = 0.0f;
lastY = 0.0f;
}
I have no idea what I did wrong here. This is very simple as you can see and shader code should be running . I've checked file loaders strings that it returns and it is correct just like in those .glsl files.
In function mainWindow->update()
is glfwSwapBuffers(window)
and in function mainWindow->clear()
is in order: glClear(GL_COLOR_BUFFER_BIT);
glfwPollEvents();
Also those info logs aren't returning anything. Window does show up but its all blue there is no square in the middle.
c++ opengl graphics shader graphics2d
I had glCreateProgram()
return error 1282 before but fixed it after removing context glfwWindowsHint
calls. But even after that nothing is showing up on the screen! Here is my shader code:
Shader.h
#pragma once
#include <iostream>
#include "file_utils.h"
#include "GL/glew.h"
class Shader {
private:
GLuint shaderID;
const char *vertPath;
const char *fragPath;
public:
Shader(const char *vertexPath, const char *fragmentPath);
~Shader();
void enable() const;
void disable() const;
private:
GLuint load();
};
Shader.cpp
#include "shader.h"
Shader::Shader(const char *vertexPath, const char *fragmentPath)
{
vertPath = vertexPath;
fragPath = fragmentPath;
shaderID = load();
}
Shader::~Shader()
{
glDeleteProgram(shaderID);
}
GLuint Shader::load()
{
GLuint program = glCreateProgram();
GLuint vertex = glCreateShader(GL_VERTEX_SHADER);
GLuint fragment = glCreateShader(GL_FRAGMENT_SHADER);
std::string g_vert = FileUtils::readFile(vertPath);
std::string g_frag = FileUtils::readFile(fragPath);
const char *vertSource = g_vert.c_str();
const char *fragSource = g_frag.c_str();
glShaderSource(vertex, 1, &vertSource, NULL);
glCompileShader(vertex);
GLchar infoL[1024];
GLint result;
glGetShaderiv(vertex, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(vertex, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING VERTEX SHADER: " << infoL << std::endl;
glDeleteShader(vertex);
return 0;
}
glShaderSource(fragment, 1, &fragSource, NULL);
glCompileShader(fragment);
glGetShaderiv(fragment, GL_COMPILE_STATUS, &result);
if (result == GL_FALSE)
{
glGetShaderInfoLog(fragment, sizeof(infoL), 0, infoL);
std::cout << "ERROR COMPLING FRAGMENT SHADER: " << infoL << std::endl;
glDeleteShader(fragment);
return 0;
}
glAttachShader(program, vertex);
glAttachShader(program, fragment);
glLinkProgram(program);
glValidateProgram(program);
glDeleteShader(vertex);
glDeleteShader(fragment);
return program;
}
void Shader::enable() const
{
glUseProgram(shaderID);
}
void Shader::disable() const
{
glUseProgram(0);
}
vertex.glsl
#version 330 core
layout (location = 0) in vec4 position;
uniform mat4 pr_matrix;
uniform mat4 vw_matrix = mat4(1.0);
uniform mat4 ml_matrix = mat4(1.0);
void main()
{
gl_Position = position;
}
fragment.glsl
#version 330 core
layout (location = 0) out vec4 color;
void main()
{
color = vec4(1.0, 0.0, 1.0, 1.0);
}
And finally here is main:
#include <iostream>
#include "WindowCreation.h"
#include "Maths.h"
#include "shader.h"
const int SCREEN_WIDTH = 800, SCREEN_HEIGHT = 600;
int main(){
WindowCreation *mainWindow = new WindowCreation();
if (mainWindow->createWindow(&SCREEN_WIDTH, &SCREEN_HEIGHT, "GoldSpark Engine") == 0)
{
glClearColor(1.0f, 0.0f, 0.0f, 1.0f);
}
else
{
cout << "Could not create window. EXITING..." << endl;
return 1;
}
GLfloat vertices = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vao;
glGenVertexArrays(1, &vao);
glBindVertexArray(vao);
GLuint vbo = 0;
glGenBuffers(1, &vbo);
glBindBuffer(GL_ARRAY_BUFFER, vbo);
glBufferData(GL_ARRAY_BUFFER, sizeof(vertices), vertices, GL_STATIC_DRAW);
glVertexAttribPointer(0, 3, GL_FLOAT, GL_FALSE, 0, 0);
glEnableVertexAttribArray(0);
Shader shaders("resources/shaders/vertex.glsl", "resources/shaders/fragment.glsl");
shaders.enable();
while(!mainWindow->getShouldClose())
{
mainWindow->clear();
glDrawArrays(GL_TRIANGLES, 0, 6);
mainWindow->update();
}
shaders.disable();
glBindBuffer(GL_ARRAY_BUFFER, 0);
delete mainWindow;
return 0;
}
WindowCreation.h
#pragma once
#include <iostream>
#include "GL/glew.h"
#include "GLFW/glfw3.h"
using namespace std;
class WindowCreation
{
public:
int bufferWidth, bufferHeight;
WindowCreation();
~WindowCreation();
bool getShouldClose() { return glfwWindowShouldClose(window); }
void createCallBacks();
GLfloat getXChange() {
GLfloat xCh = xChange;
xChange = 0.0f;
return xCh;
}
GLfloat getYChange() {
GLfloat yCh = yChange;
yChange = 0.0f;
return yCh;
}
bool *getKeyPress() { return keys; }
void update() {
glfwPollEvents();
glClear(GL_COLOR_BUFFER_BIT);
}
void clear() {
glfwSwapBuffers(window);
}
int createWindow(const int *screenWidth, const int *screenHeight, const char* title); // ovdje pravimo sve sto treba da se prozor pojavi
GLFWwindow *getWindow() { return window; }
private:
GLFWwindow *window;
bool firstTime;
bool keys[1024];
GLfloat lastX, lastY, xChange, yChange;
static void handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode);
static void handleMouse(GLFWwindow* window, double xPos, double yPos);
};
WindowCreation.cpp
#include "WindowCreation.h"
WindowCreation::WindowCreation()
{
//sve na 0 postavimo prvo
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
xChange = 0.0f;
yChange = 0.0f;
firstTime = true;
}
void WindowCreation::createCallBacks()
{
glfwSetKeyCallback(getWindow(), handleKeyboard);
glfwSetCursorPosCallback(getWindow(), handleMouse);
}
void WindowCreation::handleKeyboard(GLFWwindow* window, int key, int code, int action, int mode)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (key == GLFW_KEY_ESCAPE && action == GLFW_PRESS)
{
glfwSetWindowShouldClose(thisWindow->window, GLFW_TRUE);
}
if (key >= 0 && key <= 1024)
{
if (action == GLFW_PRESS)
{
thisWindow->keys[key] = true;
}
else if (action == GLFW_RELEASE)
{
thisWindow->keys[key] = false;
}
}
}
void WindowCreation::handleMouse(GLFWwindow* window, double xPos, double yPos)
{
WindowCreation *thisWindow = static_cast<WindowCreation*>(glfwGetWindowUserPointer(window));
if (thisWindow->firstTime)
{
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
thisWindow->firstTime = false;
}
thisWindow->xChange = xPos - thisWindow->lastX;
thisWindow->yChange = thisWindow->lastY - yPos;
thisWindow->lastX = xPos;
thisWindow->lastY = yPos;
}
int WindowCreation::createWindow(const int *screenWidth,const int *screenHeight, const char* title)
{
bufferWidth = 0;
bufferHeight = 0;
if (!glfwInit()) // inicijalizujemo glfw
{
cout << "Could not initialize glfw." << endl;
glfwTerminate();
return 1;
}
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GLFW_TRUE);
window = glfwCreateWindow(*screenWidth, *screenHeight, title, NULL, NULL);
glfwGetFramebufferSize(window, &bufferWidth, &bufferHeight);
if (!window)
{
cout << "Window could not be created." << endl;
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glfwMakeContextCurrent(window);
if (glewInit() != GLEW_OK)
{
cout << "Could not initialize GLEW";
system("pause");
glfwDestroyWindow(window);
glfwTerminate();
return 1;
}
glewExperimental = true;
glViewport(0, 0, bufferWidth, bufferHeight);
glfwSetWindowUserPointer(window, this);
createCallBacks();
return 0;
}
WindowCreation::~WindowCreation()
{
glfwDestroyWindow(window);
glfwTerminate();
for (size_t i = 0; i < 1024; i++)
{
keys[i] = 0;
}
window = nullptr;
xChange = 0.0f;
yChange = 0.0f;
lastX = 0.0f;
lastY = 0.0f;
}
I have no idea what I did wrong here. This is very simple as you can see and shader code should be running . I've checked file loaders strings that it returns and it is correct just like in those .glsl files.
In function mainWindow->update()
is glfwSwapBuffers(window)
and in function mainWindow->clear()
is in order: glClear(GL_COLOR_BUFFER_BIT);
glfwPollEvents();
Also those info logs aren't returning anything. Window does show up but its all blue there is no square in the middle.
c++ opengl graphics shader graphics2d
c++ opengl graphics shader graphics2d
edited Dec 9 '18 at 12:41


Rabbid76
43.1k123354
43.1k123354
asked Nov 29 '18 at 1:26


GoldSparkGoldSpark
65
65
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
1
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00
|
show 3 more comments
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
1
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
1
1
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00
|
show 3 more comments
3 Answers
3
active
oldest
votes
Update: Now you have provided WindowCreation code, check your WindowCreation->update method where you call glClear, remove the glClear call and you will see your rendered rectangle.
As noted in comments you had not provided all the code required for window creation and as robthebloke answered you had not created a vertex array, below is a working mash of your main code with the tutorial code for window creation and triangle rendering from http://www.opengl-tutorial.org/beginners-tutorials/tutorial-1-opening-a-window/ that sucessfully renders a square and utilises your Shader class, after modifying to bypass the missing File_Utils code. Compiled and verified under Visual Studio 2017 on Windows 10.
#include "shader.h"
#include "GL/glew.h"
#include "GLFW/glfw3.h"
GLFWwindow* window;
int main(void)
{
// Initialise GLFW
if (!glfwInit())
{
fprintf(stderr, "Failed to initialize GLFWn");
getchar();
return -1;
}
glfwWindowHint(GLFW_SAMPLES, 4);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// Open a window and create its OpenGL context
window = glfwCreateWindow(1024, 768, "Tutorial 02 - Purple Square", NULL, NULL);
if (window == NULL) {
fprintf(stderr, "Failed to open GLFW window. If you have an Intel GPU, they are not 3.3 compatible. Try the 2.1 version of the tutorials.n");
getchar();
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Initialize GLEW
glewExperimental = true; // Needed for core profile
if (glewInit() != GLEW_OK) {
fprintf(stderr, "Failed to initialize GLEWn");
getchar();
glfwTerminate();
return -1;
}
// Ensure we can capture the escape key being pressed below
glfwSetInputMode(window, GLFW_STICKY_KEYS, GL_TRUE);
// Dark blue background
glClearColor(0.0f, 0.0f, 0.4f, 0.0f);
GLuint VertexArrayID;
glGenVertexArrays(1, &VertexArrayID);
glBindVertexArray(VertexArrayID);
// Create and compile our GLSL program from the shaders
Shader shader("vertex.glsl", "fragment.glsl");
static const GLfloat g_vertex_buffer_data = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vertexbuffer;
glGenBuffers(1, &vertexbuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(g_vertex_buffer_data), g_vertex_buffer_data, GL_STATIC_DRAW);
do {
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Use our shader
//glUseProgram(programID);
shader.enable();
// 1rst attribute buffer : vertices
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glVertexAttribPointer(
0, // attribute 0. No particular reason for 0, but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
);
// Draw the square !
glDrawArrays(GL_TRIANGLES, 0, 6); //
glDisableVertexAttribArray(0);
// Swap buffers
glfwSwapBuffers(window);
glfwPollEvents();
} // Check if the ESC key was pressed or the window was closed
while (glfwGetKey(window, GLFW_KEY_ESCAPE) != GLFW_PRESS &&
glfwWindowShouldClose(window) == 0);
// Cleanup VBO
glDeleteBuffers(1, &vertexbuffer);
glDeleteVertexArrays(1, &VertexArrayID);
shader.disable();
shader.~Shader();
// Close OpenGL window and terminate GLFW
glfwTerminate();
return 0;
}
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
add a comment |
Well apparently the whole time I had wrong vertex indices. Nice!
It even did not show up using old opengl drawing which was glBegin()
etc... glClearColor
was showing the color but triangle was not drawing just because I put minus on a vertex that was supposed to be UP.
add a comment |
Whilst you are setting the program to use (by the looks of it), you're not setting any of the uniform matrix parameters in the program. The vertex shader can't transform your vertices as a result.
As mentioned, always check the GL errors of your program (or enable the debug profile, and use the newer debugging API). At minimum, you should have something like this:
void printError(int err, const char* str, const char* file, const int line)
{
std::cout << "gl_error: " << str << ": " << file << "." << line << " > ";
switch(err)
{
case GL_INVALID_ENUM: std::cout << "GL_INVALID_ENUM" << std::endl;
case GL_INVALID_VALUE: std::cout << "GL_INVALID_VALUE" << std::endl;
case GL_INVALID_OPERATION: std::cout << "GL_INVALID_OPERATION" << std::endl;
case GL_INVALID_FRAMEBUFFER_OPERATION: std::cout << "GL_INVALID_FRAMEBUFFER_OPERATION" << std::endl;
case GL_OUT_OF_MEMORY: std::cout << "GL_OUT_OF_MEMORY" << std::endl;
case GL_STACK_UNDERFLOW: std::cout << "GL_STACK_UNDERFLOW" << std::endl;
case GL_STACK_OVERFLOW: std::cout << "GL_STACK_OVERFLOW" << std::endl;
default: break;
}
}
#define CHECK_GL(X) X; {
int __err = glGetError();
if(__err != GL_NO_ERROR) printError(__err, #X, __FILE__, __LINE__);
}
Which you can use in your GL calls like so:
CHECK_GL(glDeleteProgram(1, &prog));
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530546%2fcant-get-anything-to-show-on-the-screen-in-opengl-simple-program-only-background%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
Update: Now you have provided WindowCreation code, check your WindowCreation->update method where you call glClear, remove the glClear call and you will see your rendered rectangle.
As noted in comments you had not provided all the code required for window creation and as robthebloke answered you had not created a vertex array, below is a working mash of your main code with the tutorial code for window creation and triangle rendering from http://www.opengl-tutorial.org/beginners-tutorials/tutorial-1-opening-a-window/ that sucessfully renders a square and utilises your Shader class, after modifying to bypass the missing File_Utils code. Compiled and verified under Visual Studio 2017 on Windows 10.
#include "shader.h"
#include "GL/glew.h"
#include "GLFW/glfw3.h"
GLFWwindow* window;
int main(void)
{
// Initialise GLFW
if (!glfwInit())
{
fprintf(stderr, "Failed to initialize GLFWn");
getchar();
return -1;
}
glfwWindowHint(GLFW_SAMPLES, 4);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// Open a window and create its OpenGL context
window = glfwCreateWindow(1024, 768, "Tutorial 02 - Purple Square", NULL, NULL);
if (window == NULL) {
fprintf(stderr, "Failed to open GLFW window. If you have an Intel GPU, they are not 3.3 compatible. Try the 2.1 version of the tutorials.n");
getchar();
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Initialize GLEW
glewExperimental = true; // Needed for core profile
if (glewInit() != GLEW_OK) {
fprintf(stderr, "Failed to initialize GLEWn");
getchar();
glfwTerminate();
return -1;
}
// Ensure we can capture the escape key being pressed below
glfwSetInputMode(window, GLFW_STICKY_KEYS, GL_TRUE);
// Dark blue background
glClearColor(0.0f, 0.0f, 0.4f, 0.0f);
GLuint VertexArrayID;
glGenVertexArrays(1, &VertexArrayID);
glBindVertexArray(VertexArrayID);
// Create and compile our GLSL program from the shaders
Shader shader("vertex.glsl", "fragment.glsl");
static const GLfloat g_vertex_buffer_data = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vertexbuffer;
glGenBuffers(1, &vertexbuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(g_vertex_buffer_data), g_vertex_buffer_data, GL_STATIC_DRAW);
do {
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Use our shader
//glUseProgram(programID);
shader.enable();
// 1rst attribute buffer : vertices
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glVertexAttribPointer(
0, // attribute 0. No particular reason for 0, but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
);
// Draw the square !
glDrawArrays(GL_TRIANGLES, 0, 6); //
glDisableVertexAttribArray(0);
// Swap buffers
glfwSwapBuffers(window);
glfwPollEvents();
} // Check if the ESC key was pressed or the window was closed
while (glfwGetKey(window, GLFW_KEY_ESCAPE) != GLFW_PRESS &&
glfwWindowShouldClose(window) == 0);
// Cleanup VBO
glDeleteBuffers(1, &vertexbuffer);
glDeleteVertexArrays(1, &VertexArrayID);
shader.disable();
shader.~Shader();
// Close OpenGL window and terminate GLFW
glfwTerminate();
return 0;
}
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
add a comment |
Update: Now you have provided WindowCreation code, check your WindowCreation->update method where you call glClear, remove the glClear call and you will see your rendered rectangle.
As noted in comments you had not provided all the code required for window creation and as robthebloke answered you had not created a vertex array, below is a working mash of your main code with the tutorial code for window creation and triangle rendering from http://www.opengl-tutorial.org/beginners-tutorials/tutorial-1-opening-a-window/ that sucessfully renders a square and utilises your Shader class, after modifying to bypass the missing File_Utils code. Compiled and verified under Visual Studio 2017 on Windows 10.
#include "shader.h"
#include "GL/glew.h"
#include "GLFW/glfw3.h"
GLFWwindow* window;
int main(void)
{
// Initialise GLFW
if (!glfwInit())
{
fprintf(stderr, "Failed to initialize GLFWn");
getchar();
return -1;
}
glfwWindowHint(GLFW_SAMPLES, 4);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// Open a window and create its OpenGL context
window = glfwCreateWindow(1024, 768, "Tutorial 02 - Purple Square", NULL, NULL);
if (window == NULL) {
fprintf(stderr, "Failed to open GLFW window. If you have an Intel GPU, they are not 3.3 compatible. Try the 2.1 version of the tutorials.n");
getchar();
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Initialize GLEW
glewExperimental = true; // Needed for core profile
if (glewInit() != GLEW_OK) {
fprintf(stderr, "Failed to initialize GLEWn");
getchar();
glfwTerminate();
return -1;
}
// Ensure we can capture the escape key being pressed below
glfwSetInputMode(window, GLFW_STICKY_KEYS, GL_TRUE);
// Dark blue background
glClearColor(0.0f, 0.0f, 0.4f, 0.0f);
GLuint VertexArrayID;
glGenVertexArrays(1, &VertexArrayID);
glBindVertexArray(VertexArrayID);
// Create and compile our GLSL program from the shaders
Shader shader("vertex.glsl", "fragment.glsl");
static const GLfloat g_vertex_buffer_data = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vertexbuffer;
glGenBuffers(1, &vertexbuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(g_vertex_buffer_data), g_vertex_buffer_data, GL_STATIC_DRAW);
do {
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Use our shader
//glUseProgram(programID);
shader.enable();
// 1rst attribute buffer : vertices
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glVertexAttribPointer(
0, // attribute 0. No particular reason for 0, but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
);
// Draw the square !
glDrawArrays(GL_TRIANGLES, 0, 6); //
glDisableVertexAttribArray(0);
// Swap buffers
glfwSwapBuffers(window);
glfwPollEvents();
} // Check if the ESC key was pressed or the window was closed
while (glfwGetKey(window, GLFW_KEY_ESCAPE) != GLFW_PRESS &&
glfwWindowShouldClose(window) == 0);
// Cleanup VBO
glDeleteBuffers(1, &vertexbuffer);
glDeleteVertexArrays(1, &VertexArrayID);
shader.disable();
shader.~Shader();
// Close OpenGL window and terminate GLFW
glfwTerminate();
return 0;
}
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
add a comment |
Update: Now you have provided WindowCreation code, check your WindowCreation->update method where you call glClear, remove the glClear call and you will see your rendered rectangle.
As noted in comments you had not provided all the code required for window creation and as robthebloke answered you had not created a vertex array, below is a working mash of your main code with the tutorial code for window creation and triangle rendering from http://www.opengl-tutorial.org/beginners-tutorials/tutorial-1-opening-a-window/ that sucessfully renders a square and utilises your Shader class, after modifying to bypass the missing File_Utils code. Compiled and verified under Visual Studio 2017 on Windows 10.
#include "shader.h"
#include "GL/glew.h"
#include "GLFW/glfw3.h"
GLFWwindow* window;
int main(void)
{
// Initialise GLFW
if (!glfwInit())
{
fprintf(stderr, "Failed to initialize GLFWn");
getchar();
return -1;
}
glfwWindowHint(GLFW_SAMPLES, 4);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// Open a window and create its OpenGL context
window = glfwCreateWindow(1024, 768, "Tutorial 02 - Purple Square", NULL, NULL);
if (window == NULL) {
fprintf(stderr, "Failed to open GLFW window. If you have an Intel GPU, they are not 3.3 compatible. Try the 2.1 version of the tutorials.n");
getchar();
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Initialize GLEW
glewExperimental = true; // Needed for core profile
if (glewInit() != GLEW_OK) {
fprintf(stderr, "Failed to initialize GLEWn");
getchar();
glfwTerminate();
return -1;
}
// Ensure we can capture the escape key being pressed below
glfwSetInputMode(window, GLFW_STICKY_KEYS, GL_TRUE);
// Dark blue background
glClearColor(0.0f, 0.0f, 0.4f, 0.0f);
GLuint VertexArrayID;
glGenVertexArrays(1, &VertexArrayID);
glBindVertexArray(VertexArrayID);
// Create and compile our GLSL program from the shaders
Shader shader("vertex.glsl", "fragment.glsl");
static const GLfloat g_vertex_buffer_data = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vertexbuffer;
glGenBuffers(1, &vertexbuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(g_vertex_buffer_data), g_vertex_buffer_data, GL_STATIC_DRAW);
do {
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Use our shader
//glUseProgram(programID);
shader.enable();
// 1rst attribute buffer : vertices
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glVertexAttribPointer(
0, // attribute 0. No particular reason for 0, but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
);
// Draw the square !
glDrawArrays(GL_TRIANGLES, 0, 6); //
glDisableVertexAttribArray(0);
// Swap buffers
glfwSwapBuffers(window);
glfwPollEvents();
} // Check if the ESC key was pressed or the window was closed
while (glfwGetKey(window, GLFW_KEY_ESCAPE) != GLFW_PRESS &&
glfwWindowShouldClose(window) == 0);
// Cleanup VBO
glDeleteBuffers(1, &vertexbuffer);
glDeleteVertexArrays(1, &VertexArrayID);
shader.disable();
shader.~Shader();
// Close OpenGL window and terminate GLFW
glfwTerminate();
return 0;
}
Update: Now you have provided WindowCreation code, check your WindowCreation->update method where you call glClear, remove the glClear call and you will see your rendered rectangle.
As noted in comments you had not provided all the code required for window creation and as robthebloke answered you had not created a vertex array, below is a working mash of your main code with the tutorial code for window creation and triangle rendering from http://www.opengl-tutorial.org/beginners-tutorials/tutorial-1-opening-a-window/ that sucessfully renders a square and utilises your Shader class, after modifying to bypass the missing File_Utils code. Compiled and verified under Visual Studio 2017 on Windows 10.
#include "shader.h"
#include "GL/glew.h"
#include "GLFW/glfw3.h"
GLFWwindow* window;
int main(void)
{
// Initialise GLFW
if (!glfwInit())
{
fprintf(stderr, "Failed to initialize GLFWn");
getchar();
return -1;
}
glfwWindowHint(GLFW_SAMPLES, 4);
glfwWindowHint(GLFW_CONTEXT_VERSION_MAJOR, 3);
glfwWindowHint(GLFW_CONTEXT_VERSION_MINOR, 3);
glfwWindowHint(GLFW_OPENGL_FORWARD_COMPAT, GL_TRUE);
glfwWindowHint(GLFW_OPENGL_PROFILE, GLFW_OPENGL_CORE_PROFILE);
// Open a window and create its OpenGL context
window = glfwCreateWindow(1024, 768, "Tutorial 02 - Purple Square", NULL, NULL);
if (window == NULL) {
fprintf(stderr, "Failed to open GLFW window. If you have an Intel GPU, they are not 3.3 compatible. Try the 2.1 version of the tutorials.n");
getchar();
glfwTerminate();
return -1;
}
glfwMakeContextCurrent(window);
// Initialize GLEW
glewExperimental = true; // Needed for core profile
if (glewInit() != GLEW_OK) {
fprintf(stderr, "Failed to initialize GLEWn");
getchar();
glfwTerminate();
return -1;
}
// Ensure we can capture the escape key being pressed below
glfwSetInputMode(window, GLFW_STICKY_KEYS, GL_TRUE);
// Dark blue background
glClearColor(0.0f, 0.0f, 0.4f, 0.0f);
GLuint VertexArrayID;
glGenVertexArrays(1, &VertexArrayID);
glBindVertexArray(VertexArrayID);
// Create and compile our GLSL program from the shaders
Shader shader("vertex.glsl", "fragment.glsl");
static const GLfloat g_vertex_buffer_data = {
-0.5f, -0.5f, 0.0f,
-0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, 0.5f, 0.0f,
0.5f, -0.5f, 0.0f,
-0.5f, -0.5f, 0.0f
};
GLuint vertexbuffer;
glGenBuffers(1, &vertexbuffer);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glBufferData(GL_ARRAY_BUFFER, sizeof(g_vertex_buffer_data), g_vertex_buffer_data, GL_STATIC_DRAW);
do {
// Clear the screen
glClear(GL_COLOR_BUFFER_BIT);
// Use our shader
//glUseProgram(programID);
shader.enable();
// 1rst attribute buffer : vertices
glEnableVertexAttribArray(0);
glBindBuffer(GL_ARRAY_BUFFER, vertexbuffer);
glVertexAttribPointer(
0, // attribute 0. No particular reason for 0, but must match the layout in the shader.
3, // size
GL_FLOAT, // type
GL_FALSE, // normalized?
0, // stride
(void*)0 // array buffer offset
);
// Draw the square !
glDrawArrays(GL_TRIANGLES, 0, 6); //
glDisableVertexAttribArray(0);
// Swap buffers
glfwSwapBuffers(window);
glfwPollEvents();
} // Check if the ESC key was pressed or the window was closed
while (glfwGetKey(window, GLFW_KEY_ESCAPE) != GLFW_PRESS &&
glfwWindowShouldClose(window) == 0);
// Cleanup VBO
glDeleteBuffers(1, &vertexbuffer);
glDeleteVertexArrays(1, &VertexArrayID);
shader.disable();
shader.~Shader();
// Close OpenGL window and terminate GLFW
glfwTerminate();
return 0;
}
edited Dec 2 '18 at 22:11
answered Nov 29 '18 at 23:44
DanielsDaniels
1314
1314
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
add a comment |
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
Updated the code with WindownCreation.
– GoldSpark
Nov 30 '18 at 15:54
add a comment |
Well apparently the whole time I had wrong vertex indices. Nice!
It even did not show up using old opengl drawing which was glBegin()
etc... glClearColor
was showing the color but triangle was not drawing just because I put minus on a vertex that was supposed to be UP.
add a comment |
Well apparently the whole time I had wrong vertex indices. Nice!
It even did not show up using old opengl drawing which was glBegin()
etc... glClearColor
was showing the color but triangle was not drawing just because I put minus on a vertex that was supposed to be UP.
add a comment |
Well apparently the whole time I had wrong vertex indices. Nice!
It even did not show up using old opengl drawing which was glBegin()
etc... glClearColor
was showing the color but triangle was not drawing just because I put minus on a vertex that was supposed to be UP.
Well apparently the whole time I had wrong vertex indices. Nice!
It even did not show up using old opengl drawing which was glBegin()
etc... glClearColor
was showing the color but triangle was not drawing just because I put minus on a vertex that was supposed to be UP.
edited Dec 9 '18 at 12:41


Rabbid76
43.1k123354
43.1k123354
answered Dec 7 '18 at 1:05


GoldSparkGoldSpark
65
65
add a comment |
add a comment |
Whilst you are setting the program to use (by the looks of it), you're not setting any of the uniform matrix parameters in the program. The vertex shader can't transform your vertices as a result.
As mentioned, always check the GL errors of your program (or enable the debug profile, and use the newer debugging API). At minimum, you should have something like this:
void printError(int err, const char* str, const char* file, const int line)
{
std::cout << "gl_error: " << str << ": " << file << "." << line << " > ";
switch(err)
{
case GL_INVALID_ENUM: std::cout << "GL_INVALID_ENUM" << std::endl;
case GL_INVALID_VALUE: std::cout << "GL_INVALID_VALUE" << std::endl;
case GL_INVALID_OPERATION: std::cout << "GL_INVALID_OPERATION" << std::endl;
case GL_INVALID_FRAMEBUFFER_OPERATION: std::cout << "GL_INVALID_FRAMEBUFFER_OPERATION" << std::endl;
case GL_OUT_OF_MEMORY: std::cout << "GL_OUT_OF_MEMORY" << std::endl;
case GL_STACK_UNDERFLOW: std::cout << "GL_STACK_UNDERFLOW" << std::endl;
case GL_STACK_OVERFLOW: std::cout << "GL_STACK_OVERFLOW" << std::endl;
default: break;
}
}
#define CHECK_GL(X) X; {
int __err = glGetError();
if(__err != GL_NO_ERROR) printError(__err, #X, __FILE__, __LINE__);
}
Which you can use in your GL calls like so:
CHECK_GL(glDeleteProgram(1, &prog));
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
add a comment |
Whilst you are setting the program to use (by the looks of it), you're not setting any of the uniform matrix parameters in the program. The vertex shader can't transform your vertices as a result.
As mentioned, always check the GL errors of your program (or enable the debug profile, and use the newer debugging API). At minimum, you should have something like this:
void printError(int err, const char* str, const char* file, const int line)
{
std::cout << "gl_error: " << str << ": " << file << "." << line << " > ";
switch(err)
{
case GL_INVALID_ENUM: std::cout << "GL_INVALID_ENUM" << std::endl;
case GL_INVALID_VALUE: std::cout << "GL_INVALID_VALUE" << std::endl;
case GL_INVALID_OPERATION: std::cout << "GL_INVALID_OPERATION" << std::endl;
case GL_INVALID_FRAMEBUFFER_OPERATION: std::cout << "GL_INVALID_FRAMEBUFFER_OPERATION" << std::endl;
case GL_OUT_OF_MEMORY: std::cout << "GL_OUT_OF_MEMORY" << std::endl;
case GL_STACK_UNDERFLOW: std::cout << "GL_STACK_UNDERFLOW" << std::endl;
case GL_STACK_OVERFLOW: std::cout << "GL_STACK_OVERFLOW" << std::endl;
default: break;
}
}
#define CHECK_GL(X) X; {
int __err = glGetError();
if(__err != GL_NO_ERROR) printError(__err, #X, __FILE__, __LINE__);
}
Which you can use in your GL calls like so:
CHECK_GL(glDeleteProgram(1, &prog));
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
add a comment |
Whilst you are setting the program to use (by the looks of it), you're not setting any of the uniform matrix parameters in the program. The vertex shader can't transform your vertices as a result.
As mentioned, always check the GL errors of your program (or enable the debug profile, and use the newer debugging API). At minimum, you should have something like this:
void printError(int err, const char* str, const char* file, const int line)
{
std::cout << "gl_error: " << str << ": " << file << "." << line << " > ";
switch(err)
{
case GL_INVALID_ENUM: std::cout << "GL_INVALID_ENUM" << std::endl;
case GL_INVALID_VALUE: std::cout << "GL_INVALID_VALUE" << std::endl;
case GL_INVALID_OPERATION: std::cout << "GL_INVALID_OPERATION" << std::endl;
case GL_INVALID_FRAMEBUFFER_OPERATION: std::cout << "GL_INVALID_FRAMEBUFFER_OPERATION" << std::endl;
case GL_OUT_OF_MEMORY: std::cout << "GL_OUT_OF_MEMORY" << std::endl;
case GL_STACK_UNDERFLOW: std::cout << "GL_STACK_UNDERFLOW" << std::endl;
case GL_STACK_OVERFLOW: std::cout << "GL_STACK_OVERFLOW" << std::endl;
default: break;
}
}
#define CHECK_GL(X) X; {
int __err = glGetError();
if(__err != GL_NO_ERROR) printError(__err, #X, __FILE__, __LINE__);
}
Which you can use in your GL calls like so:
CHECK_GL(glDeleteProgram(1, &prog));
Whilst you are setting the program to use (by the looks of it), you're not setting any of the uniform matrix parameters in the program. The vertex shader can't transform your vertices as a result.
As mentioned, always check the GL errors of your program (or enable the debug profile, and use the newer debugging API). At minimum, you should have something like this:
void printError(int err, const char* str, const char* file, const int line)
{
std::cout << "gl_error: " << str << ": " << file << "." << line << " > ";
switch(err)
{
case GL_INVALID_ENUM: std::cout << "GL_INVALID_ENUM" << std::endl;
case GL_INVALID_VALUE: std::cout << "GL_INVALID_VALUE" << std::endl;
case GL_INVALID_OPERATION: std::cout << "GL_INVALID_OPERATION" << std::endl;
case GL_INVALID_FRAMEBUFFER_OPERATION: std::cout << "GL_INVALID_FRAMEBUFFER_OPERATION" << std::endl;
case GL_OUT_OF_MEMORY: std::cout << "GL_OUT_OF_MEMORY" << std::endl;
case GL_STACK_UNDERFLOW: std::cout << "GL_STACK_UNDERFLOW" << std::endl;
case GL_STACK_OVERFLOW: std::cout << "GL_STACK_OVERFLOW" << std::endl;
default: break;
}
}
#define CHECK_GL(X) X; {
int __err = glGetError();
if(__err != GL_NO_ERROR) printError(__err, #X, __FILE__, __LINE__);
}
Which you can use in your GL calls like so:
CHECK_GL(glDeleteProgram(1, &prog));
edited Dec 28 '18 at 16:31


Rabbid76
43.1k123354
43.1k123354
answered Nov 29 '18 at 3:42
robtheblokerobthebloke
29114
29114
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
add a comment |
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
Where is the question to this answer? By the way, read about OpenGL - Debug Output.
– Rabbid76
Nov 29 '18 at 6:42
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53530546%2fcant-get-anything-to-show-on-the-screen-in-opengl-simple-program-only-background%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
7Y,PY,iyAuERsNfzd0 2vsXu1ex7YE,lj qp,kl6,oo7 er VQjSTzVg,nBazYsuFH8K
glCreateProgram: "This function returns 0 if an error occurs creating the program object." I see no mention of 1282 as a return value for it.
– xaxxon
Nov 29 '18 at 1:36
That is why in the title it says glGetError returns 1282 and glCreateProgram returns 1 which is not an error. That is probably why for some weird reason nothing is showing up on the screen...
– GoldSpark
Nov 29 '18 at 1:40
you need to check for errors immediately after EVERY opengl call. After there's an error, everything else is suspect.
– xaxxon
Nov 29 '18 at 1:47
Yeah did that. Error 1282 is showing up after glCreateProgram() call and after VertexArrayAttrib()
– GoldSpark
Nov 29 '18 at 1:50
1
Your code sample is not complete and is missing content of WindowCreation and Maths headers and cpp which i assume to be your own code? you may get more help if your code is available in full. Tutorials 1 & 2 here opengl-tutorial.org/beginners-tutorials/… cover the basics of what you are trying to do and may be of some help.
– Daniels
Nov 29 '18 at 5:00