RecylverView not able to send messages after onClick
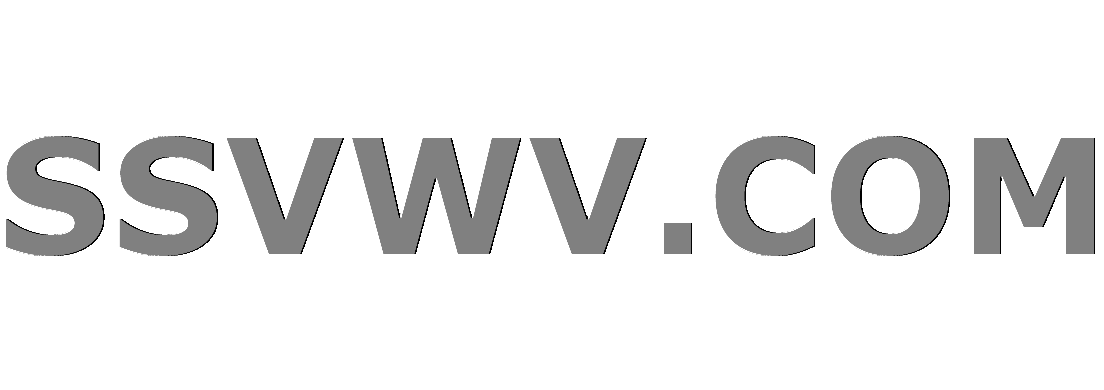
Multi tool use
I have integrated a simple messaging service in my application. I am the first stage where I can only send messages and not receive. The problem is that when I try to send a message, my send_button
does not do anything. I am using a custom MessagesAdapter
and sending static messages
that appear when the user goes to the messages
section of the app. Any help would be greatly appreciated. I will post my code
and xml
down below.
xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Messages">
<android.support.v7.widget.RecyclerView
android:id="@+id/reyclerview_message_list"
android:layout_width="0dp"
android:layout_height="439dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"></android.support.v7.widget.RecyclerView>
<!-- A horizontal line between the chatbox and RecyclerView -->
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="2dp"
android:background="#dfdfdf"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<LinearLayout
android:id="@+id/layout_chatbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:minHeight="48dp"
android:background="#ffffff"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent">
<EditText
android:id="@+id/edittext_chatbox"
android:hint="Enter message"
android:background="@android:color/transparent"
android:layout_gravity="center"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:maxLines="6"/>
<Button
android:id="@+id/button_chatbox_send"
android:text="SEND"
android:textSize="14dp"
android:background="?attr/selectableItemBackground"
android:clickable="true"
android:layout_width="64dp"
android:layout_height="48dp"
android:gravity="center"
android:layout_gravity="bottom" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
Messages.java
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import java.util.ArrayList;
public class Messages extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
public ArrayList<MessagesModel> initMessages() {
ArrayList<MessagesModel> mm = new ArrayList<>();
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
return mm;
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.activity_messages, container, false);
// setContentView(R.layout.activity_messages);
ArrayList<MessagesModel> mm = initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
// btn = myView.findViewById(R.id.button_chatbox_send);
// btn.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View view) {
// txtview = myView.findViewById(R.id.edittext_chatbox);
// rview = myView.findViewById(R.id.reyclerview_message_list);
// txtview.getText();
// }
// });
return myView;
}
}
MessagesAdapter.java
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import java.util.ArrayList;
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public static ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.sent_messages, viewGroup, false);
return new ViewHolder(view);
}
// @Override
// public void onBindViewHolder(@NonNull MessagesAdapter.ViewHolder viewHolder, int i) {
//
// MessagesModel model = mm.get(i);
// viewHolder.message.setText(model.getMessage());
// viewHolder.time.setText(model.getTime());
//
// }
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).getMessage());
viewHolder.time.setText(mm.get(i).getTime());
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
// public final View view;
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
// this.view = view;
message = view.findViewById(R.id.text_message_body);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.text_message_time);
}
}
}
java

add a comment |
I have integrated a simple messaging service in my application. I am the first stage where I can only send messages and not receive. The problem is that when I try to send a message, my send_button
does not do anything. I am using a custom MessagesAdapter
and sending static messages
that appear when the user goes to the messages
section of the app. Any help would be greatly appreciated. I will post my code
and xml
down below.
xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Messages">
<android.support.v7.widget.RecyclerView
android:id="@+id/reyclerview_message_list"
android:layout_width="0dp"
android:layout_height="439dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"></android.support.v7.widget.RecyclerView>
<!-- A horizontal line between the chatbox and RecyclerView -->
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="2dp"
android:background="#dfdfdf"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<LinearLayout
android:id="@+id/layout_chatbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:minHeight="48dp"
android:background="#ffffff"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent">
<EditText
android:id="@+id/edittext_chatbox"
android:hint="Enter message"
android:background="@android:color/transparent"
android:layout_gravity="center"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:maxLines="6"/>
<Button
android:id="@+id/button_chatbox_send"
android:text="SEND"
android:textSize="14dp"
android:background="?attr/selectableItemBackground"
android:clickable="true"
android:layout_width="64dp"
android:layout_height="48dp"
android:gravity="center"
android:layout_gravity="bottom" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
Messages.java
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import java.util.ArrayList;
public class Messages extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
public ArrayList<MessagesModel> initMessages() {
ArrayList<MessagesModel> mm = new ArrayList<>();
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
return mm;
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.activity_messages, container, false);
// setContentView(R.layout.activity_messages);
ArrayList<MessagesModel> mm = initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
// btn = myView.findViewById(R.id.button_chatbox_send);
// btn.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View view) {
// txtview = myView.findViewById(R.id.edittext_chatbox);
// rview = myView.findViewById(R.id.reyclerview_message_list);
// txtview.getText();
// }
// });
return myView;
}
}
MessagesAdapter.java
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import java.util.ArrayList;
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public static ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.sent_messages, viewGroup, false);
return new ViewHolder(view);
}
// @Override
// public void onBindViewHolder(@NonNull MessagesAdapter.ViewHolder viewHolder, int i) {
//
// MessagesModel model = mm.get(i);
// viewHolder.message.setText(model.getMessage());
// viewHolder.time.setText(model.getTime());
//
// }
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).getMessage());
viewHolder.time.setText(mm.get(i).getTime());
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
// public final View view;
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
// this.view = view;
message = view.findViewById(R.id.text_message_body);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.text_message_time);
}
}
}
java

add a comment |
I have integrated a simple messaging service in my application. I am the first stage where I can only send messages and not receive. The problem is that when I try to send a message, my send_button
does not do anything. I am using a custom MessagesAdapter
and sending static messages
that appear when the user goes to the messages
section of the app. Any help would be greatly appreciated. I will post my code
and xml
down below.
xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Messages">
<android.support.v7.widget.RecyclerView
android:id="@+id/reyclerview_message_list"
android:layout_width="0dp"
android:layout_height="439dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"></android.support.v7.widget.RecyclerView>
<!-- A horizontal line between the chatbox and RecyclerView -->
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="2dp"
android:background="#dfdfdf"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<LinearLayout
android:id="@+id/layout_chatbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:minHeight="48dp"
android:background="#ffffff"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent">
<EditText
android:id="@+id/edittext_chatbox"
android:hint="Enter message"
android:background="@android:color/transparent"
android:layout_gravity="center"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:maxLines="6"/>
<Button
android:id="@+id/button_chatbox_send"
android:text="SEND"
android:textSize="14dp"
android:background="?attr/selectableItemBackground"
android:clickable="true"
android:layout_width="64dp"
android:layout_height="48dp"
android:gravity="center"
android:layout_gravity="bottom" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
Messages.java
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import java.util.ArrayList;
public class Messages extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
public ArrayList<MessagesModel> initMessages() {
ArrayList<MessagesModel> mm = new ArrayList<>();
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
return mm;
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.activity_messages, container, false);
// setContentView(R.layout.activity_messages);
ArrayList<MessagesModel> mm = initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
// btn = myView.findViewById(R.id.button_chatbox_send);
// btn.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View view) {
// txtview = myView.findViewById(R.id.edittext_chatbox);
// rview = myView.findViewById(R.id.reyclerview_message_list);
// txtview.getText();
// }
// });
return myView;
}
}
MessagesAdapter.java
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import java.util.ArrayList;
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public static ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.sent_messages, viewGroup, false);
return new ViewHolder(view);
}
// @Override
// public void onBindViewHolder(@NonNull MessagesAdapter.ViewHolder viewHolder, int i) {
//
// MessagesModel model = mm.get(i);
// viewHolder.message.setText(model.getMessage());
// viewHolder.time.setText(model.getTime());
//
// }
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).getMessage());
viewHolder.time.setText(mm.get(i).getTime());
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
// public final View view;
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
// this.view = view;
message = view.findViewById(R.id.text_message_body);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.text_message_time);
}
}
}
java

I have integrated a simple messaging service in my application. I am the first stage where I can only send messages and not receive. The problem is that when I try to send a message, my send_button
does not do anything. I am using a custom MessagesAdapter
and sending static messages
that appear when the user goes to the messages
section of the app. Any help would be greatly appreciated. I will post my code
and xml
down below.
xml
<?xml version="1.0" encoding="utf-8"?>
<android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".Messages">
<android.support.v7.widget.RecyclerView
android:id="@+id/reyclerview_message_list"
android:layout_width="0dp"
android:layout_height="439dp"
android:layout_marginBottom="8dp"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintTop_toTopOf="parent"></android.support.v7.widget.RecyclerView>
<!-- A horizontal line between the chatbox and RecyclerView -->
<View
android:id="@+id/view3"
android:layout_width="0dp"
android:layout_height="2dp"
android:background="#dfdfdf"
app:layout_constraintBottom_toTopOf="@+id/layout_chatbox"
app:layout_constraintLeft_toLeftOf="parent"
app:layout_constraintRight_toRightOf="parent" />
<LinearLayout
android:id="@+id/layout_chatbox"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:orientation="horizontal"
android:minHeight="48dp"
android:background="#ffffff"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintRight_toRightOf="parent"
app:layout_constraintLeft_toLeftOf="parent">
<EditText
android:id="@+id/edittext_chatbox"
android:hint="Enter message"
android:background="@android:color/transparent"
android:layout_gravity="center"
android:layout_marginLeft="16dp"
android:layout_marginRight="16dp"
android:layout_width="0dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:maxLines="6"/>
<Button
android:id="@+id/button_chatbox_send"
android:text="SEND"
android:textSize="14dp"
android:background="?attr/selectableItemBackground"
android:clickable="true"
android:layout_width="64dp"
android:layout_height="48dp"
android:gravity="center"
android:layout_gravity="bottom" />
</LinearLayout>
</android.support.constraint.ConstraintLayout>
Messages.java
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.support.v4.app.Fragment;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.TextView;
import java.util.ArrayList;
public class Messages extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
public ArrayList<MessagesModel> initMessages() {
ArrayList<MessagesModel> mm = new ArrayList<>();
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
return mm;
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.activity_messages, container, false);
// setContentView(R.layout.activity_messages);
ArrayList<MessagesModel> mm = initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
// btn = myView.findViewById(R.id.button_chatbox_send);
// btn.setOnClickListener(new View.OnClickListener() {
// @Override
// public void onClick(View view) {
// txtview = myView.findViewById(R.id.edittext_chatbox);
// rview = myView.findViewById(R.id.reyclerview_message_list);
// txtview.getText();
// }
// });
return myView;
}
}
MessagesAdapter.java
import android.support.annotation.NonNull;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.TextView;
import java.util.ArrayList;
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public static ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.sent_messages, viewGroup, false);
return new ViewHolder(view);
}
// @Override
// public void onBindViewHolder(@NonNull MessagesAdapter.ViewHolder viewHolder, int i) {
//
// MessagesModel model = mm.get(i);
// viewHolder.message.setText(model.getMessage());
// viewHolder.time.setText(model.getTime());
//
// }
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).getMessage());
viewHolder.time.setText(mm.get(i).getTime());
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
// public final View view;
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
// this.view = view;
message = view.findViewById(R.id.text_message_body);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.text_message_time);
}
}
}
java

java

edited Nov 28 '18 at 17:15


Fantômas
32.8k156490
32.8k156490
asked Nov 28 '18 at 16:06
moegizzlemoegizzle
479
479
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
i have added your Messages fragment class replace it with your existing one hopefully it works for you
public class Help extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
ArrayList<MessagesModel> mm = new ArrayList<>();
public void initMessages() {
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.help, container, false);
initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
txtview = myView.findViewById(R.id.edittext_chatbox);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
btn = myView.findViewById(R.id.button_chatbox_send);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mm.add(new MessagesModel(""+txtview.getText().toString(), "12:41"));
rviewadap.notifyDataSetChanged();
}
});
return myView;
}
public class MessagesModel{
String varMessage,varTime;
public MessagesModel(String varMessage,String varTime){
this.varMessage = varMessage;
this.varTime = varTime;
}
}
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.row_weather, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).varMessage);
viewHolder.time.setText(mm.get(i).varTime);
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
message = view.findViewById(R.id.day_txt);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.temp_txt);
}
}
}
}
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
|
show 2 more comments
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523611%2frecylverview-not-able-to-send-messages-after-onclick%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
i have added your Messages fragment class replace it with your existing one hopefully it works for you
public class Help extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
ArrayList<MessagesModel> mm = new ArrayList<>();
public void initMessages() {
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.help, container, false);
initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
txtview = myView.findViewById(R.id.edittext_chatbox);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
btn = myView.findViewById(R.id.button_chatbox_send);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mm.add(new MessagesModel(""+txtview.getText().toString(), "12:41"));
rviewadap.notifyDataSetChanged();
}
});
return myView;
}
public class MessagesModel{
String varMessage,varTime;
public MessagesModel(String varMessage,String varTime){
this.varMessage = varMessage;
this.varTime = varTime;
}
}
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.row_weather, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).varMessage);
viewHolder.time.setText(mm.get(i).varTime);
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
message = view.findViewById(R.id.day_txt);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.temp_txt);
}
}
}
}
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
|
show 2 more comments
i have added your Messages fragment class replace it with your existing one hopefully it works for you
public class Help extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
ArrayList<MessagesModel> mm = new ArrayList<>();
public void initMessages() {
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.help, container, false);
initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
txtview = myView.findViewById(R.id.edittext_chatbox);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
btn = myView.findViewById(R.id.button_chatbox_send);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mm.add(new MessagesModel(""+txtview.getText().toString(), "12:41"));
rviewadap.notifyDataSetChanged();
}
});
return myView;
}
public class MessagesModel{
String varMessage,varTime;
public MessagesModel(String varMessage,String varTime){
this.varMessage = varMessage;
this.varTime = varTime;
}
}
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.row_weather, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).varMessage);
viewHolder.time.setText(mm.get(i).varTime);
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
message = view.findViewById(R.id.day_txt);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.temp_txt);
}
}
}
}
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
|
show 2 more comments
i have added your Messages fragment class replace it with your existing one hopefully it works for you
public class Help extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
ArrayList<MessagesModel> mm = new ArrayList<>();
public void initMessages() {
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.help, container, false);
initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
txtview = myView.findViewById(R.id.edittext_chatbox);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
btn = myView.findViewById(R.id.button_chatbox_send);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mm.add(new MessagesModel(""+txtview.getText().toString(), "12:41"));
rviewadap.notifyDataSetChanged();
}
});
return myView;
}
public class MessagesModel{
String varMessage,varTime;
public MessagesModel(String varMessage,String varTime){
this.varMessage = varMessage;
this.varTime = varTime;
}
}
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.row_weather, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).varMessage);
viewHolder.time.setText(mm.get(i).varTime);
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
message = view.findViewById(R.id.day_txt);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.temp_txt);
}
}
}
}
i have added your Messages fragment class replace it with your existing one hopefully it works for you
public class Help extends Fragment {
View myView;
Button btn;
TextView txtview;
private RecyclerView rview;
private RecyclerView.Adapter rviewadap;
ArrayList<MessagesModel> mm = new ArrayList<>();
public void initMessages() {
mm.add(new MessagesModel("Hello this is a test", "12:41"));
mm.add(new MessagesModel("This is another test", "12:43"));
mm.add(new MessagesModel("How are you doing today", "12:43"));
}
public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
myView = inflater.inflate(R.layout.help, container, false);
initMessages();
this.rview = myView.findViewById(R.id.reyclerview_message_list);
txtview = myView.findViewById(R.id.edittext_chatbox);
RecyclerView.LayoutManager layout = new LinearLayoutManager(this.getActivity());
this.rview.setLayoutManager(layout);
rviewadap = new MessagesAdapter(mm);
this.rview.setAdapter(rviewadap);
btn = myView.findViewById(R.id.button_chatbox_send);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
mm.add(new MessagesModel(""+txtview.getText().toString(), "12:41"));
rviewadap.notifyDataSetChanged();
}
});
return myView;
}
public class MessagesModel{
String varMessage,varTime;
public MessagesModel(String varMessage,String varTime){
this.varMessage = varMessage;
this.varTime = varTime;
}
}
public class MessagesAdapter extends RecyclerView.Adapter<MessagesAdapter.ViewHolder> {
public ArrayList<MessagesModel> mm;
public MessagesAdapter(ArrayList<MessagesModel> mm) {
this.mm = mm;
}
@NonNull
@Override
public ViewHolder onCreateViewHolder(@NonNull ViewGroup viewGroup, int i) {
View view = (View) LayoutInflater.from(viewGroup.getContext()).inflate(R.layout.row_weather, viewGroup, false);
return new ViewHolder(view);
}
@Override
public void onBindViewHolder(@NonNull final ViewHolder viewHolder, int i) {
viewHolder.message.setText(mm.get(i).varMessage);
viewHolder.time.setText(mm.get(i).varTime);
}
@Override
public int getItemCount() {
if(mm != null) {
return mm.size();
}else {
return 0;
}
}
public class ViewHolder extends RecyclerView.ViewHolder {
public TextView message;
public TextView time;
public ViewHolder(@NonNull View view) {
super(view);
message = view.findViewById(R.id.day_txt);
System.out.println("body message id is: "+message);
time = view.findViewById(R.id.temp_txt);
}
}
}
}
edited Nov 28 '18 at 17:21
answered Nov 28 '18 at 16:24
Zahoor SaleemZahoor Saleem
32229
32229
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
|
show 2 more comments
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
when I click send it doesn't work..
– moegizzle
Nov 28 '18 at 16:30
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
your click listener is fired or not?
– Zahoor Saleem
Nov 28 '18 at 16:34
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
It should fire but I don't see why it isn't
– moegizzle
Nov 28 '18 at 16:36
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
replace private RecyclerView.Adapter rviewadap; with MessagesAdapter rviewadap; and try again
– Zahoor Saleem
Nov 28 '18 at 16:39
no still not working...
– moegizzle
Nov 28 '18 at 16:44
no still not working...
– moegizzle
Nov 28 '18 at 16:44
|
show 2 more comments
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523611%2frecylverview-not-able-to-send-messages-after-onclick%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
suQ,sDkmKQhEyr2OkS,SmLclCU 3VZjlN,CrtbJLIamC,5RxywV5,no,a w,g,tFw