Getting matrixL and matrixU from Eigen::SuperLU?
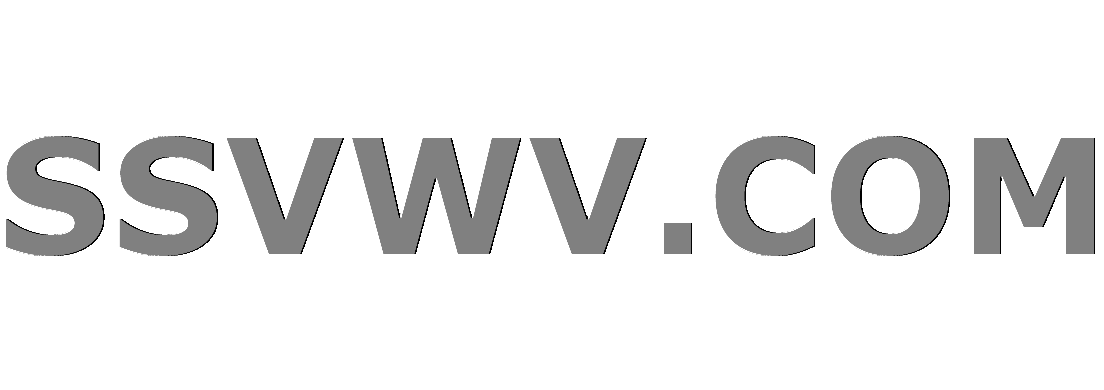
Multi tool use
I need to get matrices L and U from Eigen::SuperLU. The method matrixU() returns a UMatrixType. When I call this method, I get the following error
:cannot convert from
'Eigen::SparseMatrix<std::complex<double>,0,int>'
to'const Eigen::TriangularView<Eigen::SparseMatrix<std::complex<double>,0,int>,2> &'
.
If I replace UMatrixType by LUMAtrixType in SuperLUSupport.h, everything compiles but the following code does not give the expected result.
using SpMatc = Eigen::SparseMatrix<std::complex<double>>;
Eigen::MatrixXcd matDense = Eigen::MatrixXd::Identity(n, n);
matDense(13, 15) = std::complex<double>(5., 3.);
matDense(8, 11) = std::complex<double>(3., 9.);
SpMatc mat = matDense.sparseView();
Eigen::VectorXcd b = Eigen::VectorXcd::Random(n);
mat.makeCompressed();
Eigen::SuperLU<SpMatc> slu;
slu.compute(mat);
Eigen::VectorXcd y = slu.solve(b);
const auto& matL = slu.matrixL();
const auto& matU = slu.matrixU();
auto permutationMatrixP = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationP());
auto permutationMatrixQ = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationQ());
Eigen::VectorXcd res = permutationMatrixP*b;
matL.triangularView<Eigen::Lower | Eigen::UnitDiag>().solveInPlace(res);
matU.triangularView<Eigen::Upper>().solveInPlace(res);
Eigen::VectorXcd finalRes = permutationMatrixQ.inverse() * res;
Eigen::VectorXcd diff = finalRes - y;
Assert::IsTrue(!finalRes.isApprox(y));
If I replace Eigen::SuperLU by Eigen::SparseLU, the result is ok.
c++ eigen
add a comment |
I need to get matrices L and U from Eigen::SuperLU. The method matrixU() returns a UMatrixType. When I call this method, I get the following error
:cannot convert from
'Eigen::SparseMatrix<std::complex<double>,0,int>'
to'const Eigen::TriangularView<Eigen::SparseMatrix<std::complex<double>,0,int>,2> &'
.
If I replace UMatrixType by LUMAtrixType in SuperLUSupport.h, everything compiles but the following code does not give the expected result.
using SpMatc = Eigen::SparseMatrix<std::complex<double>>;
Eigen::MatrixXcd matDense = Eigen::MatrixXd::Identity(n, n);
matDense(13, 15) = std::complex<double>(5., 3.);
matDense(8, 11) = std::complex<double>(3., 9.);
SpMatc mat = matDense.sparseView();
Eigen::VectorXcd b = Eigen::VectorXcd::Random(n);
mat.makeCompressed();
Eigen::SuperLU<SpMatc> slu;
slu.compute(mat);
Eigen::VectorXcd y = slu.solve(b);
const auto& matL = slu.matrixL();
const auto& matU = slu.matrixU();
auto permutationMatrixP = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationP());
auto permutationMatrixQ = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationQ());
Eigen::VectorXcd res = permutationMatrixP*b;
matL.triangularView<Eigen::Lower | Eigen::UnitDiag>().solveInPlace(res);
matU.triangularView<Eigen::Upper>().solveInPlace(res);
Eigen::VectorXcd finalRes = permutationMatrixQ.inverse() * res;
Eigen::VectorXcd diff = finalRes - y;
Assert::IsTrue(!finalRes.isApprox(y));
If I replace Eigen::SuperLU by Eigen::SparseLU, the result is ok.
c++ eigen
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.
– ggael
Nov 28 '18 at 21:33
add a comment |
I need to get matrices L and U from Eigen::SuperLU. The method matrixU() returns a UMatrixType. When I call this method, I get the following error
:cannot convert from
'Eigen::SparseMatrix<std::complex<double>,0,int>'
to'const Eigen::TriangularView<Eigen::SparseMatrix<std::complex<double>,0,int>,2> &'
.
If I replace UMatrixType by LUMAtrixType in SuperLUSupport.h, everything compiles but the following code does not give the expected result.
using SpMatc = Eigen::SparseMatrix<std::complex<double>>;
Eigen::MatrixXcd matDense = Eigen::MatrixXd::Identity(n, n);
matDense(13, 15) = std::complex<double>(5., 3.);
matDense(8, 11) = std::complex<double>(3., 9.);
SpMatc mat = matDense.sparseView();
Eigen::VectorXcd b = Eigen::VectorXcd::Random(n);
mat.makeCompressed();
Eigen::SuperLU<SpMatc> slu;
slu.compute(mat);
Eigen::VectorXcd y = slu.solve(b);
const auto& matL = slu.matrixL();
const auto& matU = slu.matrixU();
auto permutationMatrixP = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationP());
auto permutationMatrixQ = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationQ());
Eigen::VectorXcd res = permutationMatrixP*b;
matL.triangularView<Eigen::Lower | Eigen::UnitDiag>().solveInPlace(res);
matU.triangularView<Eigen::Upper>().solveInPlace(res);
Eigen::VectorXcd finalRes = permutationMatrixQ.inverse() * res;
Eigen::VectorXcd diff = finalRes - y;
Assert::IsTrue(!finalRes.isApprox(y));
If I replace Eigen::SuperLU by Eigen::SparseLU, the result is ok.
c++ eigen
I need to get matrices L and U from Eigen::SuperLU. The method matrixU() returns a UMatrixType. When I call this method, I get the following error
:cannot convert from
'Eigen::SparseMatrix<std::complex<double>,0,int>'
to'const Eigen::TriangularView<Eigen::SparseMatrix<std::complex<double>,0,int>,2> &'
.
If I replace UMatrixType by LUMAtrixType in SuperLUSupport.h, everything compiles but the following code does not give the expected result.
using SpMatc = Eigen::SparseMatrix<std::complex<double>>;
Eigen::MatrixXcd matDense = Eigen::MatrixXd::Identity(n, n);
matDense(13, 15) = std::complex<double>(5., 3.);
matDense(8, 11) = std::complex<double>(3., 9.);
SpMatc mat = matDense.sparseView();
Eigen::VectorXcd b = Eigen::VectorXcd::Random(n);
mat.makeCompressed();
Eigen::SuperLU<SpMatc> slu;
slu.compute(mat);
Eigen::VectorXcd y = slu.solve(b);
const auto& matL = slu.matrixL();
const auto& matU = slu.matrixU();
auto permutationMatrixP = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationP());
auto permutationMatrixQ = Eigen::PermutationMatrix<Eigen::Dynamic>(slu.permutationQ());
Eigen::VectorXcd res = permutationMatrixP*b;
matL.triangularView<Eigen::Lower | Eigen::UnitDiag>().solveInPlace(res);
matU.triangularView<Eigen::Upper>().solveInPlace(res);
Eigen::VectorXcd finalRes = permutationMatrixQ.inverse() * res;
Eigen::VectorXcd diff = finalRes - y;
Assert::IsTrue(!finalRes.isApprox(y));
If I replace Eigen::SuperLU by Eigen::SparseLU, the result is ok.
c++ eigen
c++ eigen
edited Nov 28 '18 at 16:18


Öö Tiib
7,8442034
7,8442034
asked Nov 28 '18 at 16:12
kajezzkajezz
11
11
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.
– ggael
Nov 28 '18 at 21:33
add a comment |
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.
– ggael
Nov 28 '18 at 21:33
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.– ggael
Nov 28 '18 at 21:33
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.– ggael
Nov 28 '18 at 21:33
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523724%2fgetting-matrixl-and-matrixu-from-eigensuperlu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523724%2fgetting-matrixl-and-matrixu-from-eigensuperlu%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DlODvjgzJYh1lzRJo0jJxb XrpIiLYzEI8yNQfCRusO7NjWy,t 92CO7XJeL2pbjXNuCCk5Uq6T urG
This is expected. SuperLU uses dense matrices, SparseLU uses Sparse matrices, and you are using Sparse matrices. The two types you have are not compatible and cannot be converted from one to the other.
– Matthieu Brucher
Nov 28 '18 at 16:15
SparseLU
is a built-in rewrite of the external SuperLU solver, so better forget about SuperLU and stick with SparseLU.– ggael
Nov 28 '18 at 21:33