.net core BackgroundService Http Server/GRPC - Stop Method?
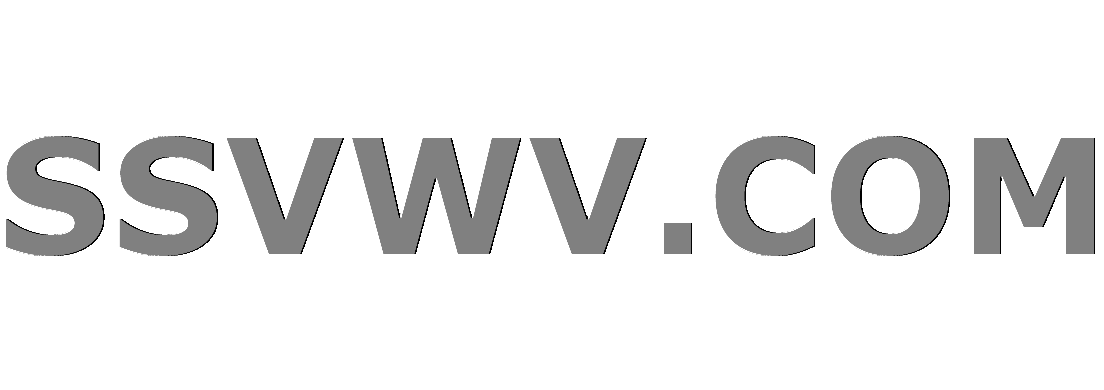
Multi tool use
currently what is the best way to actually run a Http Server inside a .net core BackgroundService
, i.e. running is easy, but how to actually correctly integrate the stopping method.
At the moment I've written the code like that:
var server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
var source = new TaskCompletionSource<bool>();
stoppingToken.Register(async () => await server.ShutdownAsync());
server.Start();
if (!stoppingToken.IsCancellationRequested)
{
await source.Task;
}
before I had something like
while(!stoppingToken.IsCancellationRequested) {}
await server.ShutdownAsync()
however the performance was really really bad.
is this actually the correct way of doing it?
or are there better ways? (IApplicationLifetime
)?
c# .net .net-core
add a comment |
currently what is the best way to actually run a Http Server inside a .net core BackgroundService
, i.e. running is easy, but how to actually correctly integrate the stopping method.
At the moment I've written the code like that:
var server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
var source = new TaskCompletionSource<bool>();
stoppingToken.Register(async () => await server.ShutdownAsync());
server.Start();
if (!stoppingToken.IsCancellationRequested)
{
await source.Task;
}
before I had something like
while(!stoppingToken.IsCancellationRequested) {}
await server.ShutdownAsync()
however the performance was really really bad.
is this actually the correct way of doing it?
or are there better ways? (IApplicationLifetime
)?
c# .net .net-core
add a comment |
currently what is the best way to actually run a Http Server inside a .net core BackgroundService
, i.e. running is easy, but how to actually correctly integrate the stopping method.
At the moment I've written the code like that:
var server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
var source = new TaskCompletionSource<bool>();
stoppingToken.Register(async () => await server.ShutdownAsync());
server.Start();
if (!stoppingToken.IsCancellationRequested)
{
await source.Task;
}
before I had something like
while(!stoppingToken.IsCancellationRequested) {}
await server.ShutdownAsync()
however the performance was really really bad.
is this actually the correct way of doing it?
or are there better ways? (IApplicationLifetime
)?
c# .net .net-core
currently what is the best way to actually run a Http Server inside a .net core BackgroundService
, i.e. running is easy, but how to actually correctly integrate the stopping method.
At the moment I've written the code like that:
var server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
var source = new TaskCompletionSource<bool>();
stoppingToken.Register(async () => await server.ShutdownAsync());
server.Start();
if (!stoppingToken.IsCancellationRequested)
{
await source.Task;
}
before I had something like
while(!stoppingToken.IsCancellationRequested) {}
await server.ShutdownAsync()
however the performance was really really bad.
is this actually the correct way of doing it?
or are there better ways? (IApplicationLifetime
)?
c# .net .net-core
c# .net .net-core
asked Nov 28 '18 at 16:01
Christian SchmittChristian Schmitt
630733
630733
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
BackgroundService
implements IHostedService
. You can implement you own tiny background service, that also implements IHostedService
:
public class YourBackgroundService : IHostedService
{
private Server _server;
public Task StartAsync(CancellationToken cancellationToken)
{
_server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
_server.Start();
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return server?.ShutdownAsync() ?? Task.CompletedTask;
}
}
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523523%2fnet-core-backgroundservice-http-server-grpc-stop-method%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
BackgroundService
implements IHostedService
. You can implement you own tiny background service, that also implements IHostedService
:
public class YourBackgroundService : IHostedService
{
private Server _server;
public Task StartAsync(CancellationToken cancellationToken)
{
_server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
_server.Start();
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return server?.ShutdownAsync() ?? Task.CompletedTask;
}
}
add a comment |
BackgroundService
implements IHostedService
. You can implement you own tiny background service, that also implements IHostedService
:
public class YourBackgroundService : IHostedService
{
private Server _server;
public Task StartAsync(CancellationToken cancellationToken)
{
_server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
_server.Start();
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return server?.ShutdownAsync() ?? Task.CompletedTask;
}
}
add a comment |
BackgroundService
implements IHostedService
. You can implement you own tiny background service, that also implements IHostedService
:
public class YourBackgroundService : IHostedService
{
private Server _server;
public Task StartAsync(CancellationToken cancellationToken)
{
_server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
_server.Start();
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return server?.ShutdownAsync() ?? Task.CompletedTask;
}
}
BackgroundService
implements IHostedService
. You can implement you own tiny background service, that also implements IHostedService
:
public class YourBackgroundService : IHostedService
{
private Server _server;
public Task StartAsync(CancellationToken cancellationToken)
{
_server = new Server
{
Services = {ConnectionHandler.BindService(_vpnConnectionHandler)},
Ports = {new ServerPort("0.0.0.0", 50055, ServerCredentials.Insecure)}
};
_server.Start();
return Task.CompletedTask;
}
public Task StopAsync(CancellationToken cancellationToken)
{
return server?.ShutdownAsync() ?? Task.CompletedTask;
}
}
answered Nov 28 '18 at 16:20


MarkeliMarkeli
487313
487313
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53523523%2fnet-core-backgroundservice-http-server-grpc-stop-method%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
UEP8QZfWu3U,0RxsLRkZCCptv Hkh e97vcdO5zczSBpnDambESuGfc sRkLq kcYbsOTP 8 F7X5u07i1Fhyrz22kVVjirpLIL