What is the difference between both button click in the given React Component?
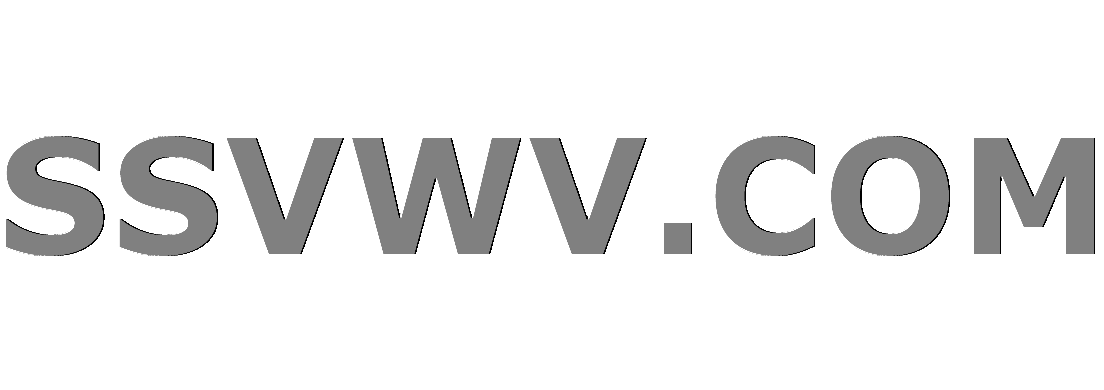
Multi tool use
Is there any difference between both the button click event in the given component? Which is the preferred way to write?
export default class App extends Component {
doSomething = () => {
console.log('Hi');
}
render() {
return (
<Container>
<Button onClick={this.doSomething} title="Test" />
<Button onClick={() => this.doSomething()} title="Test" />
</Container>
);
}
}
javascript reactjs
add a comment |
Is there any difference between both the button click event in the given component? Which is the preferred way to write?
export default class App extends Component {
doSomething = () => {
console.log('Hi');
}
render() {
return (
<Container>
<Button onClick={this.doSomething} title="Test" />
<Button onClick={() => this.doSomething()} title="Test" />
</Container>
);
}
}
javascript reactjs
this
insidedoSomething
will not change for the second example.
– MinusFour
Aug 15 '18 at 16:55
1
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
1
@DaveNewton actually nevermind, there's nothis
for arrow functions. I didn't see it was an arrow function.
– MinusFour
Aug 15 '18 at 16:58
@MinusFour I'm not sure what "there's nothis
" means.
– Dave Newton
Aug 15 '18 at 17:14
@DaveNewton no localthis
binding for arrow function. You can still usethis
inside an arrow function it just doesn't belong to the arrow function.
– MinusFour
Aug 15 '18 at 17:39
add a comment |
Is there any difference between both the button click event in the given component? Which is the preferred way to write?
export default class App extends Component {
doSomething = () => {
console.log('Hi');
}
render() {
return (
<Container>
<Button onClick={this.doSomething} title="Test" />
<Button onClick={() => this.doSomething()} title="Test" />
</Container>
);
}
}
javascript reactjs
Is there any difference between both the button click event in the given component? Which is the preferred way to write?
export default class App extends Component {
doSomething = () => {
console.log('Hi');
}
render() {
return (
<Container>
<Button onClick={this.doSomething} title="Test" />
<Button onClick={() => this.doSomething()} title="Test" />
</Container>
);
}
}
javascript reactjs
javascript reactjs
asked Aug 15 '18 at 16:52


chunkydonuts21chunkydonuts21
3917
3917
this
insidedoSomething
will not change for the second example.
– MinusFour
Aug 15 '18 at 16:55
1
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
1
@DaveNewton actually nevermind, there's nothis
for arrow functions. I didn't see it was an arrow function.
– MinusFour
Aug 15 '18 at 16:58
@MinusFour I'm not sure what "there's nothis
" means.
– Dave Newton
Aug 15 '18 at 17:14
@DaveNewton no localthis
binding for arrow function. You can still usethis
inside an arrow function it just doesn't belong to the arrow function.
– MinusFour
Aug 15 '18 at 17:39
add a comment |
this
insidedoSomething
will not change for the second example.
– MinusFour
Aug 15 '18 at 16:55
1
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
1
@DaveNewton actually nevermind, there's nothis
for arrow functions. I didn't see it was an arrow function.
– MinusFour
Aug 15 '18 at 16:58
@MinusFour I'm not sure what "there's nothis
" means.
– Dave Newton
Aug 15 '18 at 17:14
@DaveNewton no localthis
binding for arrow function. You can still usethis
inside an arrow function it just doesn't belong to the arrow function.
– MinusFour
Aug 15 '18 at 17:39
this
inside doSomething
will not change for the second example.– MinusFour
Aug 15 '18 at 16:55
this
inside doSomething
will not change for the second example.– MinusFour
Aug 15 '18 at 16:55
1
1
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
1
1
@DaveNewton actually nevermind, there's no
this
for arrow functions. I didn't see it was an arrow function.– MinusFour
Aug 15 '18 at 16:58
@DaveNewton actually nevermind, there's no
this
for arrow functions. I didn't see it was an arrow function.– MinusFour
Aug 15 '18 at 16:58
@MinusFour I'm not sure what "there's no
this
" means.– Dave Newton
Aug 15 '18 at 17:14
@MinusFour I'm not sure what "there's no
this
" means.– Dave Newton
Aug 15 '18 at 17:14
@DaveNewton no local
this
binding for arrow function. You can still use this
inside an arrow function it just doesn't belong to the arrow function.– MinusFour
Aug 15 '18 at 17:39
@DaveNewton no local
this
binding for arrow function. You can still use this
inside an arrow function it just doesn't belong to the arrow function.– MinusFour
Aug 15 '18 at 17:39
add a comment |
3 Answers
3
active
oldest
votes
When you don't need to pass the parameter, you can just use
{this.doSomething}
But if you need to pass the parameter to the function, then this will cause your method to execute immediately:
{this.doSomething(param)}
Thus, not to execute the function immediately, we need to use arrow method like you used:
{() => this.doSomething(param)}
Thus, in your case both are same. Because they are only executed when onClick is called ie. you click on the element.
Bonus:
You can still use the first way even you want to pass the parameter:
{this.doSomething(param)}
But for this, you need to define your method like this:
doSomething = (param) => () => {
console.log('Hi');
}
Furthermore, if you wish to use event object, then you may use like below:
doSomething = (param) => (event) => {
console.log('Hi');
}
Or, with the second approach ie. with arrow function:
{(event)=>this.doSomething(event,param)}
And obviously, if you are worried about performance, I would suggest not to use inline arrow function. The preferred way to use:
doSomething = (param1, param2,) => (event) => {
Misunderstanding:
Some people might find the method that pass the parameter without using inline arrow function will also work. But this is incorrect. Let me clarify on this.
When you use {this.doSomething(param)}
, this function seems to work fine with its parameter. But if you change the state inside the handler, then you'll know the big difference. You'll get error maximum update depth exceeded react.
But with the same, you can avoid that error and also avoid the performance issue, you'll need to define the method like I stated before with arrow function:
doSomething = (param) => () => {
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can dothis.doSomething.bind(param, this)
instead of{() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this
– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the defaultevent
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.
– Arup Rakshit
Aug 15 '18 at 17:12
|
show 3 more comments
From doc
<Button onClick={() => this.doSomething()} title="Test" />
The problem with this syntax is that a different callback is created
each time theButton
renders. In most cases, this is fine.
However, if this callback is passed as a prop to lower components,
those components might do an extra re-rendering.
<Button onClick={this.doSomething} title="Test" />
We generally recommend binding in the constructor or using the class
fields syntax, to avoid this sort of performance problem.
add a comment |
First we will look when to use both:
onClick={this.doSomething}
: This is using class variable directly, but it cannot be used when we are required to pass parameters to the function. For that, the second way comes to rescue.
A way to pass parameters to this is :
onClick={this.doSomething.bind(params, this)}
onClick={() => this.doSomething()}
: you can pass parameters to the function as
onClick={() => this.doSomething(param1, param2)}
.
Also, an important point to note, when the component re-renders, memory is allocated for the second one every time, while the first one just uses memory reference. So, first one is better if you don't have to pass parameters in the function.
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51863058%2fwhat-is-the-difference-between-both-button-click-in-the-given-react-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
3 Answers
3
active
oldest
votes
3 Answers
3
active
oldest
votes
active
oldest
votes
active
oldest
votes
When you don't need to pass the parameter, you can just use
{this.doSomething}
But if you need to pass the parameter to the function, then this will cause your method to execute immediately:
{this.doSomething(param)}
Thus, not to execute the function immediately, we need to use arrow method like you used:
{() => this.doSomething(param)}
Thus, in your case both are same. Because they are only executed when onClick is called ie. you click on the element.
Bonus:
You can still use the first way even you want to pass the parameter:
{this.doSomething(param)}
But for this, you need to define your method like this:
doSomething = (param) => () => {
console.log('Hi');
}
Furthermore, if you wish to use event object, then you may use like below:
doSomething = (param) => (event) => {
console.log('Hi');
}
Or, with the second approach ie. with arrow function:
{(event)=>this.doSomething(event,param)}
And obviously, if you are worried about performance, I would suggest not to use inline arrow function. The preferred way to use:
doSomething = (param1, param2,) => (event) => {
Misunderstanding:
Some people might find the method that pass the parameter without using inline arrow function will also work. But this is incorrect. Let me clarify on this.
When you use {this.doSomething(param)}
, this function seems to work fine with its parameter. But if you change the state inside the handler, then you'll know the big difference. You'll get error maximum update depth exceeded react.
But with the same, you can avoid that error and also avoid the performance issue, you'll need to define the method like I stated before with arrow function:
doSomething = (param) => () => {
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can dothis.doSomething.bind(param, this)
instead of{() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this
– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the defaultevent
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.
– Arup Rakshit
Aug 15 '18 at 17:12
|
show 3 more comments
When you don't need to pass the parameter, you can just use
{this.doSomething}
But if you need to pass the parameter to the function, then this will cause your method to execute immediately:
{this.doSomething(param)}
Thus, not to execute the function immediately, we need to use arrow method like you used:
{() => this.doSomething(param)}
Thus, in your case both are same. Because they are only executed when onClick is called ie. you click on the element.
Bonus:
You can still use the first way even you want to pass the parameter:
{this.doSomething(param)}
But for this, you need to define your method like this:
doSomething = (param) => () => {
console.log('Hi');
}
Furthermore, if you wish to use event object, then you may use like below:
doSomething = (param) => (event) => {
console.log('Hi');
}
Or, with the second approach ie. with arrow function:
{(event)=>this.doSomething(event,param)}
And obviously, if you are worried about performance, I would suggest not to use inline arrow function. The preferred way to use:
doSomething = (param1, param2,) => (event) => {
Misunderstanding:
Some people might find the method that pass the parameter without using inline arrow function will also work. But this is incorrect. Let me clarify on this.
When you use {this.doSomething(param)}
, this function seems to work fine with its parameter. But if you change the state inside the handler, then you'll know the big difference. You'll get error maximum update depth exceeded react.
But with the same, you can avoid that error and also avoid the performance issue, you'll need to define the method like I stated before with arrow function:
doSomething = (param) => () => {
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can dothis.doSomething.bind(param, this)
instead of{() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this
– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the defaultevent
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.
– Arup Rakshit
Aug 15 '18 at 17:12
|
show 3 more comments
When you don't need to pass the parameter, you can just use
{this.doSomething}
But if you need to pass the parameter to the function, then this will cause your method to execute immediately:
{this.doSomething(param)}
Thus, not to execute the function immediately, we need to use arrow method like you used:
{() => this.doSomething(param)}
Thus, in your case both are same. Because they are only executed when onClick is called ie. you click on the element.
Bonus:
You can still use the first way even you want to pass the parameter:
{this.doSomething(param)}
But for this, you need to define your method like this:
doSomething = (param) => () => {
console.log('Hi');
}
Furthermore, if you wish to use event object, then you may use like below:
doSomething = (param) => (event) => {
console.log('Hi');
}
Or, with the second approach ie. with arrow function:
{(event)=>this.doSomething(event,param)}
And obviously, if you are worried about performance, I would suggest not to use inline arrow function. The preferred way to use:
doSomething = (param1, param2,) => (event) => {
Misunderstanding:
Some people might find the method that pass the parameter without using inline arrow function will also work. But this is incorrect. Let me clarify on this.
When you use {this.doSomething(param)}
, this function seems to work fine with its parameter. But if you change the state inside the handler, then you'll know the big difference. You'll get error maximum update depth exceeded react.
But with the same, you can avoid that error and also avoid the performance issue, you'll need to define the method like I stated before with arrow function:
doSomething = (param) => () => {
When you don't need to pass the parameter, you can just use
{this.doSomething}
But if you need to pass the parameter to the function, then this will cause your method to execute immediately:
{this.doSomething(param)}
Thus, not to execute the function immediately, we need to use arrow method like you used:
{() => this.doSomething(param)}
Thus, in your case both are same. Because they are only executed when onClick is called ie. you click on the element.
Bonus:
You can still use the first way even you want to pass the parameter:
{this.doSomething(param)}
But for this, you need to define your method like this:
doSomething = (param) => () => {
console.log('Hi');
}
Furthermore, if you wish to use event object, then you may use like below:
doSomething = (param) => (event) => {
console.log('Hi');
}
Or, with the second approach ie. with arrow function:
{(event)=>this.doSomething(event,param)}
And obviously, if you are worried about performance, I would suggest not to use inline arrow function. The preferred way to use:
doSomething = (param1, param2,) => (event) => {
Misunderstanding:
Some people might find the method that pass the parameter without using inline arrow function will also work. But this is incorrect. Let me clarify on this.
When you use {this.doSomething(param)}
, this function seems to work fine with its parameter. But if you change the state inside the handler, then you'll know the big difference. You'll get error maximum update depth exceeded react.
But with the same, you can avoid that error and also avoid the performance issue, you'll need to define the method like I stated before with arrow function:
doSomething = (param) => () => {
edited Aug 15 '18 at 17:19
answered Aug 15 '18 at 16:56


Bhojendra RauniyarBhojendra Rauniyar
50.9k2079125
50.9k2079125
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can dothis.doSomething.bind(param, this)
instead of{() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this
– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the defaultevent
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.
– Arup Rakshit
Aug 15 '18 at 17:12
|
show 3 more comments
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can dothis.doSomething.bind(param, this)
instead of{() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this
– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the defaultevent
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.
– Arup Rakshit
Aug 15 '18 at 17:12
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can do
this.doSomething.bind(param, this)
instead of {() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this– Arup Rakshit
Aug 15 '18 at 17:03
There is a performance problem with the 2 approaches. When you want to pass the arguments, you can do
this.doSomething.bind(param, this)
instead of {() => this.doSomething(param)}
or use partial function with class property syntax.. Arrow syntax is not good way for this. Check this– Arup Rakshit
Aug 15 '18 at 17:03
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
I don't think OP is asking for differences between them regarding the performance. But how they work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:09
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
The subject says What is the difference between both button click... Sorry to say your answer is not on track. The actual difference is the performance issue. Passing arguments is not the problem here. Arguments can be passed without using arrow function.
– Arup Rakshit
Aug 15 '18 at 17:10
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
No. It won't work.
– Bhojendra Rauniyar
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the default
event
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.– Arup Rakshit
Aug 15 '18 at 17:12
Why? Did you check the link reactjs.org/docs/… ? The OP here not passing any arguments except the default
event
which React will take care of. For own params passing, the method definition can be changed, and can be called without Arrow function. Check the official doc, it has all the examples.– Arup Rakshit
Aug 15 '18 at 17:12
|
show 3 more comments
From doc
<Button onClick={() => this.doSomething()} title="Test" />
The problem with this syntax is that a different callback is created
each time theButton
renders. In most cases, this is fine.
However, if this callback is passed as a prop to lower components,
those components might do an extra re-rendering.
<Button onClick={this.doSomething} title="Test" />
We generally recommend binding in the constructor or using the class
fields syntax, to avoid this sort of performance problem.
add a comment |
From doc
<Button onClick={() => this.doSomething()} title="Test" />
The problem with this syntax is that a different callback is created
each time theButton
renders. In most cases, this is fine.
However, if this callback is passed as a prop to lower components,
those components might do an extra re-rendering.
<Button onClick={this.doSomething} title="Test" />
We generally recommend binding in the constructor or using the class
fields syntax, to avoid this sort of performance problem.
add a comment |
From doc
<Button onClick={() => this.doSomething()} title="Test" />
The problem with this syntax is that a different callback is created
each time theButton
renders. In most cases, this is fine.
However, if this callback is passed as a prop to lower components,
those components might do an extra re-rendering.
<Button onClick={this.doSomething} title="Test" />
We generally recommend binding in the constructor or using the class
fields syntax, to avoid this sort of performance problem.
From doc
<Button onClick={() => this.doSomething()} title="Test" />
The problem with this syntax is that a different callback is created
each time theButton
renders. In most cases, this is fine.
However, if this callback is passed as a prop to lower components,
those components might do an extra re-rendering.
<Button onClick={this.doSomething} title="Test" />
We generally recommend binding in the constructor or using the class
fields syntax, to avoid this sort of performance problem.
answered Aug 15 '18 at 16:56


Arup RakshitArup Rakshit
96.8k23187235
96.8k23187235
add a comment |
add a comment |
First we will look when to use both:
onClick={this.doSomething}
: This is using class variable directly, but it cannot be used when we are required to pass parameters to the function. For that, the second way comes to rescue.
A way to pass parameters to this is :
onClick={this.doSomething.bind(params, this)}
onClick={() => this.doSomething()}
: you can pass parameters to the function as
onClick={() => this.doSomething(param1, param2)}
.
Also, an important point to note, when the component re-renders, memory is allocated for the second one every time, while the first one just uses memory reference. So, first one is better if you don't have to pass parameters in the function.
add a comment |
First we will look when to use both:
onClick={this.doSomething}
: This is using class variable directly, but it cannot be used when we are required to pass parameters to the function. For that, the second way comes to rescue.
A way to pass parameters to this is :
onClick={this.doSomething.bind(params, this)}
onClick={() => this.doSomething()}
: you can pass parameters to the function as
onClick={() => this.doSomething(param1, param2)}
.
Also, an important point to note, when the component re-renders, memory is allocated for the second one every time, while the first one just uses memory reference. So, first one is better if you don't have to pass parameters in the function.
add a comment |
First we will look when to use both:
onClick={this.doSomething}
: This is using class variable directly, but it cannot be used when we are required to pass parameters to the function. For that, the second way comes to rescue.
A way to pass parameters to this is :
onClick={this.doSomething.bind(params, this)}
onClick={() => this.doSomething()}
: you can pass parameters to the function as
onClick={() => this.doSomething(param1, param2)}
.
Also, an important point to note, when the component re-renders, memory is allocated for the second one every time, while the first one just uses memory reference. So, first one is better if you don't have to pass parameters in the function.
First we will look when to use both:
onClick={this.doSomething}
: This is using class variable directly, but it cannot be used when we are required to pass parameters to the function. For that, the second way comes to rescue.
A way to pass parameters to this is :
onClick={this.doSomething.bind(params, this)}
onClick={() => this.doSomething()}
: you can pass parameters to the function as
onClick={() => this.doSomething(param1, param2)}
.
Also, an important point to note, when the component re-renders, memory is allocated for the second one every time, while the first one just uses memory reference. So, first one is better if you don't have to pass parameters in the function.
edited Aug 15 '18 at 17:09
answered Aug 15 '18 at 16:55


McRistMcRist
1,018313
1,018313
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f51863058%2fwhat-is-the-difference-between-both-button-click-in-the-given-react-component%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
35Xqrtz dOzh5pSzaGI,Mk7,paHvyPl,Np
this
insidedoSomething
will not change for the second example.– MinusFour
Aug 15 '18 at 16:55
1
@MinusFour Would it for the first one? It's already bound to the instance.
– Dave Newton
Aug 15 '18 at 16:56
1
@DaveNewton actually nevermind, there's no
this
for arrow functions. I didn't see it was an arrow function.– MinusFour
Aug 15 '18 at 16:58
@MinusFour I'm not sure what "there's no
this
" means.– Dave Newton
Aug 15 '18 at 17:14
@DaveNewton no local
this
binding for arrow function. You can still usethis
inside an arrow function it just doesn't belong to the arrow function.– MinusFour
Aug 15 '18 at 17:39