How to make Material-UI Snackbar not take up the whole screen width using anchorOrigin?
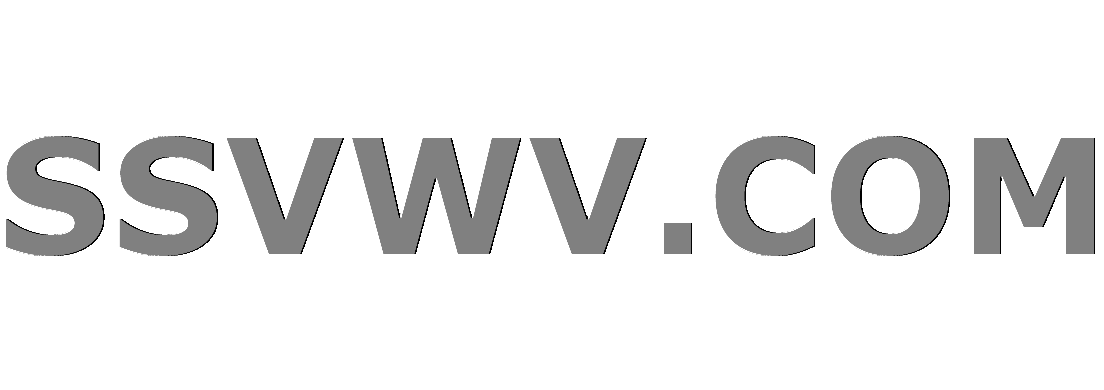
Multi tool use
up vote
0
down vote
favorite
I have a class in React which uses an input field which is part of the website header:
If the input is invalid then I want to display a snackbar. I'm using Material-UI components.
The problem is I defined anchorOrigin
to be center and top as per Material-UI API. However the snackbar takes up the whole screen width while I want it to only take up the top center location of the screen. My message is quite short, for example "Value invalid" but if it's longer then I should be able to use newlines. I'm not sure if there's some setting in Material-UI API to alter this (I couldn't find one) or I need to use CSS.
This is my code:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import InputBase from '@material-ui/core/InputBase';
import Snackbar from '@material-ui/core/Snackbar';
import SnackbarMessage from './SnackbarMessage.js';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class Test extends Component {
state = {
appId: '',
snackBarOpen: false
}
render() {
return (
<div>
<InputBase
placeholder="Search…"
classes={{
root: classes.inputRoot,
input: classes.inputInput,
}}
value={'test'} />
<Snackbar
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<SnackbarMessage
variant="warning"
message={"test message"}
/>
</Snackbar>
</div>
)
}
}
css reactjs
add a comment |
up vote
0
down vote
favorite
I have a class in React which uses an input field which is part of the website header:
If the input is invalid then I want to display a snackbar. I'm using Material-UI components.
The problem is I defined anchorOrigin
to be center and top as per Material-UI API. However the snackbar takes up the whole screen width while I want it to only take up the top center location of the screen. My message is quite short, for example "Value invalid" but if it's longer then I should be able to use newlines. I'm not sure if there's some setting in Material-UI API to alter this (I couldn't find one) or I need to use CSS.
This is my code:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import InputBase from '@material-ui/core/InputBase';
import Snackbar from '@material-ui/core/Snackbar';
import SnackbarMessage from './SnackbarMessage.js';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class Test extends Component {
state = {
appId: '',
snackBarOpen: false
}
render() {
return (
<div>
<InputBase
placeholder="Search…"
classes={{
root: classes.inputRoot,
input: classes.inputInput,
}}
value={'test'} />
<Snackbar
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<SnackbarMessage
variant="warning"
message={"test message"}
/>
</Snackbar>
</div>
)
}
}
css reactjs
1
I don't see import statements forSnackbar
andSnackbarMessage
... How is the above code even running? Also, in the material-ui examples forSnackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.
– o4ohel
Nov 22 at 5:06
@o4ohel I found from Chrome's inspect tool thatSnackbarMessage
has the following CSS:@media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disableflex-grow
the snackbar doesn't take up the whole width. I tried adding style toSnackbarMessage
to manually setflex-grow
to 0 but this doesn't work.
– hitchhiker
Nov 22 at 6:20
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
I have a class in React which uses an input field which is part of the website header:
If the input is invalid then I want to display a snackbar. I'm using Material-UI components.
The problem is I defined anchorOrigin
to be center and top as per Material-UI API. However the snackbar takes up the whole screen width while I want it to only take up the top center location of the screen. My message is quite short, for example "Value invalid" but if it's longer then I should be able to use newlines. I'm not sure if there's some setting in Material-UI API to alter this (I couldn't find one) or I need to use CSS.
This is my code:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import InputBase from '@material-ui/core/InputBase';
import Snackbar from '@material-ui/core/Snackbar';
import SnackbarMessage from './SnackbarMessage.js';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class Test extends Component {
state = {
appId: '',
snackBarOpen: false
}
render() {
return (
<div>
<InputBase
placeholder="Search…"
classes={{
root: classes.inputRoot,
input: classes.inputInput,
}}
value={'test'} />
<Snackbar
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<SnackbarMessage
variant="warning"
message={"test message"}
/>
</Snackbar>
</div>
)
}
}
css reactjs
I have a class in React which uses an input field which is part of the website header:
If the input is invalid then I want to display a snackbar. I'm using Material-UI components.
The problem is I defined anchorOrigin
to be center and top as per Material-UI API. However the snackbar takes up the whole screen width while I want it to only take up the top center location of the screen. My message is quite short, for example "Value invalid" but if it's longer then I should be able to use newlines. I'm not sure if there's some setting in Material-UI API to alter this (I couldn't find one) or I need to use CSS.
This is my code:
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import InputBase from '@material-ui/core/InputBase';
import Snackbar from '@material-ui/core/Snackbar';
import SnackbarMessage from './SnackbarMessage.js';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class Test extends Component {
state = {
appId: '',
snackBarOpen: false
}
render() {
return (
<div>
<InputBase
placeholder="Search…"
classes={{
root: classes.inputRoot,
input: classes.inputInput,
}}
value={'test'} />
<Snackbar
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<SnackbarMessage
variant="warning"
message={"test message"}
/>
</Snackbar>
</div>
)
}
}
css reactjs
css reactjs
edited Nov 22 at 5:34
asked Nov 21 at 21:31
hitchhiker
727
727
1
I don't see import statements forSnackbar
andSnackbarMessage
... How is the above code even running? Also, in the material-ui examples forSnackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.
– o4ohel
Nov 22 at 5:06
@o4ohel I found from Chrome's inspect tool thatSnackbarMessage
has the following CSS:@media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disableflex-grow
the snackbar doesn't take up the whole width. I tried adding style toSnackbarMessage
to manually setflex-grow
to 0 but this doesn't work.
– hitchhiker
Nov 22 at 6:20
add a comment |
1
I don't see import statements forSnackbar
andSnackbarMessage
... How is the above code even running? Also, in the material-ui examples forSnackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.
– o4ohel
Nov 22 at 5:06
@o4ohel I found from Chrome's inspect tool thatSnackbarMessage
has the following CSS:@media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disableflex-grow
the snackbar doesn't take up the whole width. I tried adding style toSnackbarMessage
to manually setflex-grow
to 0 but this doesn't work.
– hitchhiker
Nov 22 at 6:20
1
1
I don't see import statements for
Snackbar
and SnackbarMessage
... How is the above code even running? Also, in the material-ui examples for Snackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.– o4ohel
Nov 22 at 5:06
I don't see import statements for
Snackbar
and SnackbarMessage
... How is the above code even running? Also, in the material-ui examples for Snackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.– o4ohel
Nov 22 at 5:06
@o4ohel I found from Chrome's inspect tool that
SnackbarMessage
has the following CSS: @media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disable flex-grow
the snackbar doesn't take up the whole width. I tried adding style to SnackbarMessage
to manually set flex-grow
to 0 but this doesn't work.– hitchhiker
Nov 22 at 6:20
@o4ohel I found from Chrome's inspect tool that
SnackbarMessage
has the following CSS: @media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disable flex-grow
the snackbar doesn't take up the whole width. I tried adding style to SnackbarMessage
to manually set flex-grow
to 0 but this doesn't work.– hitchhiker
Nov 22 at 6:20
add a comment |
1 Answer
1
active
oldest
votes
up vote
0
down vote
I do not know if we can add some style to the component anchor origin field. I think the div needs to be managed using CSS. It's an anchor, not style.
<Snakbar
className = "my-snakbar"
{/*All your other stuff*/}
>
{//Stuff}
</Snakbar>
CSS
.my-snakbar {
width: 200px;
//Maybe use flexbox for positioning then
}
Let me know your thoughts
Daniel
Improved Answer
Code copied from origional question and modified
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Snackbar from '@material-ui/core/Snackbar';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class ComingSoon extends Component {
render() {
const styles = {
container: {
position: "fixed",
top: "0px",
width: "100%",
height: "30px"
},
snakbar: {
background: "black",
color: "white",
width: "100px",
height: "100%",
display: "flex",
justifyContent: "center",
alignContent: "center",
margin: "0 auto"
}
};
return (
<div className = "snakbar-container" style = {styles.container}>
<Snackbar
className = "my-snakbar"
style = {styles.snakbar}
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<span>My Message</span>
</Snackbar>
</div>
)
}
}
export default ComingSoon;
Screen shot
enter image description here
Let me know if this helped
Daniel
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
0
down vote
I do not know if we can add some style to the component anchor origin field. I think the div needs to be managed using CSS. It's an anchor, not style.
<Snakbar
className = "my-snakbar"
{/*All your other stuff*/}
>
{//Stuff}
</Snakbar>
CSS
.my-snakbar {
width: 200px;
//Maybe use flexbox for positioning then
}
Let me know your thoughts
Daniel
Improved Answer
Code copied from origional question and modified
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Snackbar from '@material-ui/core/Snackbar';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class ComingSoon extends Component {
render() {
const styles = {
container: {
position: "fixed",
top: "0px",
width: "100%",
height: "30px"
},
snakbar: {
background: "black",
color: "white",
width: "100px",
height: "100%",
display: "flex",
justifyContent: "center",
alignContent: "center",
margin: "0 auto"
}
};
return (
<div className = "snakbar-container" style = {styles.container}>
<Snackbar
className = "my-snakbar"
style = {styles.snakbar}
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<span>My Message</span>
</Snackbar>
</div>
)
}
}
export default ComingSoon;
Screen shot
enter image description here
Let me know if this helped
Daniel
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
add a comment |
up vote
0
down vote
I do not know if we can add some style to the component anchor origin field. I think the div needs to be managed using CSS. It's an anchor, not style.
<Snakbar
className = "my-snakbar"
{/*All your other stuff*/}
>
{//Stuff}
</Snakbar>
CSS
.my-snakbar {
width: 200px;
//Maybe use flexbox for positioning then
}
Let me know your thoughts
Daniel
Improved Answer
Code copied from origional question and modified
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Snackbar from '@material-ui/core/Snackbar';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class ComingSoon extends Component {
render() {
const styles = {
container: {
position: "fixed",
top: "0px",
width: "100%",
height: "30px"
},
snakbar: {
background: "black",
color: "white",
width: "100px",
height: "100%",
display: "flex",
justifyContent: "center",
alignContent: "center",
margin: "0 auto"
}
};
return (
<div className = "snakbar-container" style = {styles.container}>
<Snackbar
className = "my-snakbar"
style = {styles.snakbar}
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<span>My Message</span>
</Snackbar>
</div>
)
}
}
export default ComingSoon;
Screen shot
enter image description here
Let me know if this helped
Daniel
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
add a comment |
up vote
0
down vote
up vote
0
down vote
I do not know if we can add some style to the component anchor origin field. I think the div needs to be managed using CSS. It's an anchor, not style.
<Snakbar
className = "my-snakbar"
{/*All your other stuff*/}
>
{//Stuff}
</Snakbar>
CSS
.my-snakbar {
width: 200px;
//Maybe use flexbox for positioning then
}
Let me know your thoughts
Daniel
Improved Answer
Code copied from origional question and modified
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Snackbar from '@material-ui/core/Snackbar';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class ComingSoon extends Component {
render() {
const styles = {
container: {
position: "fixed",
top: "0px",
width: "100%",
height: "30px"
},
snakbar: {
background: "black",
color: "white",
width: "100px",
height: "100%",
display: "flex",
justifyContent: "center",
alignContent: "center",
margin: "0 auto"
}
};
return (
<div className = "snakbar-container" style = {styles.container}>
<Snackbar
className = "my-snakbar"
style = {styles.snakbar}
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<span>My Message</span>
</Snackbar>
</div>
)
}
}
export default ComingSoon;
Screen shot
enter image description here
Let me know if this helped
Daniel
I do not know if we can add some style to the component anchor origin field. I think the div needs to be managed using CSS. It's an anchor, not style.
<Snakbar
className = "my-snakbar"
{/*All your other stuff*/}
>
{//Stuff}
</Snakbar>
CSS
.my-snakbar {
width: 200px;
//Maybe use flexbox for positioning then
}
Let me know your thoughts
Daniel
Improved Answer
Code copied from origional question and modified
import React, { Component } from 'react';
import PropTypes from 'prop-types';
import Snackbar from '@material-ui/core/Snackbar';
const classes = theme => ({
inputRoot: {
color: 'inherit',
width: '100%',
},
inputInput: {
paddingTop: theme.spacing.unit,
paddingRight: theme.spacing.unit,
paddingBottom: theme.spacing.unit,
paddingLeft: theme.spacing.unit * 10,
transition: theme.transitions.create('width'),
width: '100%',
[theme.breakpoints.up('sm')]: {
width: 120,
'&:focus': {
width: 200,
},
},
}
});
class ComingSoon extends Component {
render() {
const styles = {
container: {
position: "fixed",
top: "0px",
width: "100%",
height: "30px"
},
snakbar: {
background: "black",
color: "white",
width: "100px",
height: "100%",
display: "flex",
justifyContent: "center",
alignContent: "center",
margin: "0 auto"
}
};
return (
<div className = "snakbar-container" style = {styles.container}>
<Snackbar
className = "my-snakbar"
style = {styles.snakbar}
anchorOrigin={{
vertical: 'top',
horizontal: 'center'
}}
open={true}
autoHideDuration={5000}
>
<span>My Message</span>
</Snackbar>
</div>
)
}
}
export default ComingSoon;
Screen shot
enter image description here
Let me know if this helped
Daniel
edited Nov 24 at 12:25
answered Nov 21 at 22:11
GaddMaster
11
11
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
add a comment |
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
This didn't help.
– hitchhiker
Nov 22 at 6:17
This didn't help.
– hitchhiker
Nov 22 at 6:17
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
I amended answer and made it work. Screenshot demonstrates. Also in the comments above, it mentions that the demos on the material UI site show how style is handled.
– GaddMaster
Nov 22 at 20:21
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
Your second suggestion didn't work either, moreover even in the image your provided you can see that it didn't work (the message is in the left top corner while in the OP it says "I defined anchorOrigin to be center and top").
– hitchhiker
Nov 23 at 7:42
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
I see. that's an easy fix. Ill amend right now on fly
– GaddMaster
Nov 24 at 12:19
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
That should do it. I imagine there is a more professional way through. But that CSS will do it
– GaddMaster
Nov 24 at 12:26
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420736%2fhow-to-make-material-ui-snackbar-not-take-up-the-whole-screen-width-using-anchor%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
khpTltJKrup4r7kF8NG9td5o4g4GytHcC,oQzSIIX3rpJglO iIg8Svo5t t8 G38FwH jytfN kQ,w7HW8
1
I don't see import statements for
Snackbar
andSnackbarMessage
... How is the above code even running? Also, in the material-ui examples forSnackbar
it does not take the entire screen width. Try to inspect the element and see where the 100% width is coming from.– o4ohel
Nov 22 at 5:06
@o4ohel I found from Chrome's inspect tool that
SnackbarMessage
has the following CSS:@media (max-width: 959.95px) <style>…</style> .MuiSnackbarContent-root-169 { flex-grow: 1; }
. When I disableflex-grow
the snackbar doesn't take up the whole width. I tried adding style toSnackbarMessage
to manually setflex-grow
to 0 but this doesn't work.– hitchhiker
Nov 22 at 6:20