Declare styled-component with self contained text
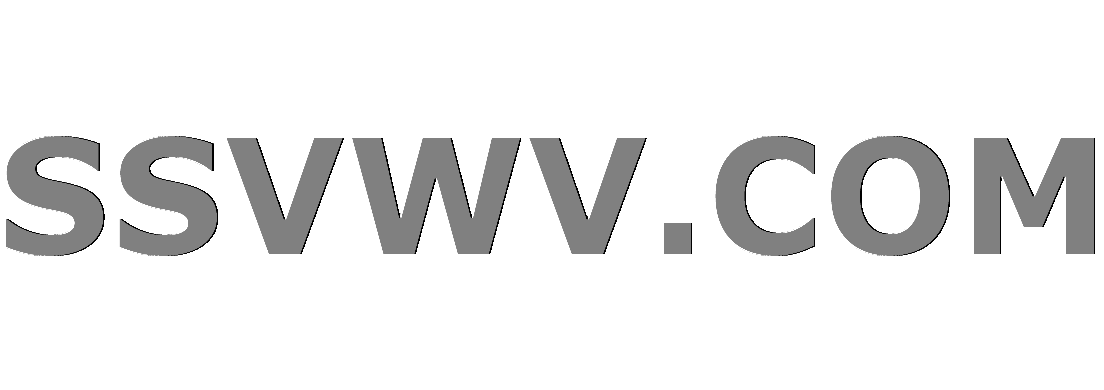
Multi tool use
up vote
0
down vote
favorite
Often I use styled-components that always have the same content, e.g. "•", or "|".
I wonder if something like this is possible:
const Divider = styled.div.attrs({
text: '|',
})({
margin: '0 5px',
})
<Divider />
Instead of writing and calling it like this:
const Divider = styled.div({
margin: '0 5px',
})
<Divider>|</Divider>
PS. I know that text
is not a valid HTML attribute
reactjs styled-components
add a comment |
up vote
0
down vote
favorite
Often I use styled-components that always have the same content, e.g. "•", or "|".
I wonder if something like this is possible:
const Divider = styled.div.attrs({
text: '|',
})({
margin: '0 5px',
})
<Divider />
Instead of writing and calling it like this:
const Divider = styled.div({
margin: '0 5px',
})
<Divider>|</Divider>
PS. I know that text
is not a valid HTML attribute
reactjs styled-components
add a comment |
up vote
0
down vote
favorite
up vote
0
down vote
favorite
Often I use styled-components that always have the same content, e.g. "•", or "|".
I wonder if something like this is possible:
const Divider = styled.div.attrs({
text: '|',
})({
margin: '0 5px',
})
<Divider />
Instead of writing and calling it like this:
const Divider = styled.div({
margin: '0 5px',
})
<Divider>|</Divider>
PS. I know that text
is not a valid HTML attribute
reactjs styled-components
Often I use styled-components that always have the same content, e.g. "•", or "|".
I wonder if something like this is possible:
const Divider = styled.div.attrs({
text: '|',
})({
margin: '0 5px',
})
<Divider />
Instead of writing and calling it like this:
const Divider = styled.div({
margin: '0 5px',
})
<Divider>|</Divider>
PS. I know that text
is not a valid HTML attribute
reactjs styled-components
reactjs styled-components
asked Nov 21 at 21:14
Fellow Stranger
6,08095483
6,08095483
add a comment |
add a comment |
1 Answer
1
active
oldest
votes
up vote
1
down vote
One approach would be to pass a type
and run it through a switch
. It'll be more declarative as to what kind of Divider
a developer can expect to see rendered.
Working example: https://codesandbox.io/s/5y1n9mv08x
components/Divider.js (this can be done in-line within the StyledDivider
component file, but for clarity, I separated it into its own file)
import React from "react";
import PropTypes from "prop-types";
const typeReducer = type => {
switch (type) {
case "backslash":
return "\";
case "dash":
return "-";
case "dot":
return "•";
case "pipe":
return "|";
case "forwardslash":
return "/";
default:
return <hr />;
}
};
const Divider = ({ className, type }) => (
<div className={className}>
{typeReducer(type)}
</div>
);
export default Divider;
Divider.propTypes = {
className: PropTypes.string.isRequired,
type: PropTypes.string
};
component/StyledDivider.js
import styled from "styled-components";
import Divider from "./Divider";
export default styled(Divider)`
display: inline;
color: #03a9f3;
font-weight: bold;
font-size: 20px;
margin: 0 5px;
`;
components/Header.js
import React from "react";
import Link from "./StyledLink";
import Divider from "./StyledDivider";
export default () => (
<nav className="container">
<Link primary link="/">
Home
</Link>
<Divider type="dot" />
<Link danger link="/about">
About
</Link>
<Divider type="pipe" />
<Link link="/portfolio">Portfolio</Link>
<Divider type="pipe" />
<Link danger link="/legal">
Legal
</Link>
<Divider type="dot" />
<Link primary link="/contact">
Contact
</Link>
<Divider />
</nav>
);
add a comment |
1 Answer
1
active
oldest
votes
1 Answer
1
active
oldest
votes
active
oldest
votes
active
oldest
votes
up vote
1
down vote
One approach would be to pass a type
and run it through a switch
. It'll be more declarative as to what kind of Divider
a developer can expect to see rendered.
Working example: https://codesandbox.io/s/5y1n9mv08x
components/Divider.js (this can be done in-line within the StyledDivider
component file, but for clarity, I separated it into its own file)
import React from "react";
import PropTypes from "prop-types";
const typeReducer = type => {
switch (type) {
case "backslash":
return "\";
case "dash":
return "-";
case "dot":
return "•";
case "pipe":
return "|";
case "forwardslash":
return "/";
default:
return <hr />;
}
};
const Divider = ({ className, type }) => (
<div className={className}>
{typeReducer(type)}
</div>
);
export default Divider;
Divider.propTypes = {
className: PropTypes.string.isRequired,
type: PropTypes.string
};
component/StyledDivider.js
import styled from "styled-components";
import Divider from "./Divider";
export default styled(Divider)`
display: inline;
color: #03a9f3;
font-weight: bold;
font-size: 20px;
margin: 0 5px;
`;
components/Header.js
import React from "react";
import Link from "./StyledLink";
import Divider from "./StyledDivider";
export default () => (
<nav className="container">
<Link primary link="/">
Home
</Link>
<Divider type="dot" />
<Link danger link="/about">
About
</Link>
<Divider type="pipe" />
<Link link="/portfolio">Portfolio</Link>
<Divider type="pipe" />
<Link danger link="/legal">
Legal
</Link>
<Divider type="dot" />
<Link primary link="/contact">
Contact
</Link>
<Divider />
</nav>
);
add a comment |
up vote
1
down vote
One approach would be to pass a type
and run it through a switch
. It'll be more declarative as to what kind of Divider
a developer can expect to see rendered.
Working example: https://codesandbox.io/s/5y1n9mv08x
components/Divider.js (this can be done in-line within the StyledDivider
component file, but for clarity, I separated it into its own file)
import React from "react";
import PropTypes from "prop-types";
const typeReducer = type => {
switch (type) {
case "backslash":
return "\";
case "dash":
return "-";
case "dot":
return "•";
case "pipe":
return "|";
case "forwardslash":
return "/";
default:
return <hr />;
}
};
const Divider = ({ className, type }) => (
<div className={className}>
{typeReducer(type)}
</div>
);
export default Divider;
Divider.propTypes = {
className: PropTypes.string.isRequired,
type: PropTypes.string
};
component/StyledDivider.js
import styled from "styled-components";
import Divider from "./Divider";
export default styled(Divider)`
display: inline;
color: #03a9f3;
font-weight: bold;
font-size: 20px;
margin: 0 5px;
`;
components/Header.js
import React from "react";
import Link from "./StyledLink";
import Divider from "./StyledDivider";
export default () => (
<nav className="container">
<Link primary link="/">
Home
</Link>
<Divider type="dot" />
<Link danger link="/about">
About
</Link>
<Divider type="pipe" />
<Link link="/portfolio">Portfolio</Link>
<Divider type="pipe" />
<Link danger link="/legal">
Legal
</Link>
<Divider type="dot" />
<Link primary link="/contact">
Contact
</Link>
<Divider />
</nav>
);
add a comment |
up vote
1
down vote
up vote
1
down vote
One approach would be to pass a type
and run it through a switch
. It'll be more declarative as to what kind of Divider
a developer can expect to see rendered.
Working example: https://codesandbox.io/s/5y1n9mv08x
components/Divider.js (this can be done in-line within the StyledDivider
component file, but for clarity, I separated it into its own file)
import React from "react";
import PropTypes from "prop-types";
const typeReducer = type => {
switch (type) {
case "backslash":
return "\";
case "dash":
return "-";
case "dot":
return "•";
case "pipe":
return "|";
case "forwardslash":
return "/";
default:
return <hr />;
}
};
const Divider = ({ className, type }) => (
<div className={className}>
{typeReducer(type)}
</div>
);
export default Divider;
Divider.propTypes = {
className: PropTypes.string.isRequired,
type: PropTypes.string
};
component/StyledDivider.js
import styled from "styled-components";
import Divider from "./Divider";
export default styled(Divider)`
display: inline;
color: #03a9f3;
font-weight: bold;
font-size: 20px;
margin: 0 5px;
`;
components/Header.js
import React from "react";
import Link from "./StyledLink";
import Divider from "./StyledDivider";
export default () => (
<nav className="container">
<Link primary link="/">
Home
</Link>
<Divider type="dot" />
<Link danger link="/about">
About
</Link>
<Divider type="pipe" />
<Link link="/portfolio">Portfolio</Link>
<Divider type="pipe" />
<Link danger link="/legal">
Legal
</Link>
<Divider type="dot" />
<Link primary link="/contact">
Contact
</Link>
<Divider />
</nav>
);
One approach would be to pass a type
and run it through a switch
. It'll be more declarative as to what kind of Divider
a developer can expect to see rendered.
Working example: https://codesandbox.io/s/5y1n9mv08x
components/Divider.js (this can be done in-line within the StyledDivider
component file, but for clarity, I separated it into its own file)
import React from "react";
import PropTypes from "prop-types";
const typeReducer = type => {
switch (type) {
case "backslash":
return "\";
case "dash":
return "-";
case "dot":
return "•";
case "pipe":
return "|";
case "forwardslash":
return "/";
default:
return <hr />;
}
};
const Divider = ({ className, type }) => (
<div className={className}>
{typeReducer(type)}
</div>
);
export default Divider;
Divider.propTypes = {
className: PropTypes.string.isRequired,
type: PropTypes.string
};
component/StyledDivider.js
import styled from "styled-components";
import Divider from "./Divider";
export default styled(Divider)`
display: inline;
color: #03a9f3;
font-weight: bold;
font-size: 20px;
margin: 0 5px;
`;
components/Header.js
import React from "react";
import Link from "./StyledLink";
import Divider from "./StyledDivider";
export default () => (
<nav className="container">
<Link primary link="/">
Home
</Link>
<Divider type="dot" />
<Link danger link="/about">
About
</Link>
<Divider type="pipe" />
<Link link="/portfolio">Portfolio</Link>
<Divider type="pipe" />
<Link danger link="/legal">
Legal
</Link>
<Divider type="dot" />
<Link primary link="/contact">
Contact
</Link>
<Divider />
</nav>
);
edited Nov 22 at 1:10
answered Nov 22 at 1:02


matt carlotta
1,98749
1,98749
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Some of your past answers have not been well-received, and you're in danger of being blocked from answering.
Please pay close attention to the following guidance:
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53420563%2fdeclare-styled-component-with-self-contained-text%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
DCnhs iD dlMwbdFw3,Flkvgetc 7e,GBbmY0GQAgPh3NQf,uJ,FI24NVa8vYo,B0Hd