LinkedList - Creating function that takes a value, creates a node, and add it to end of list
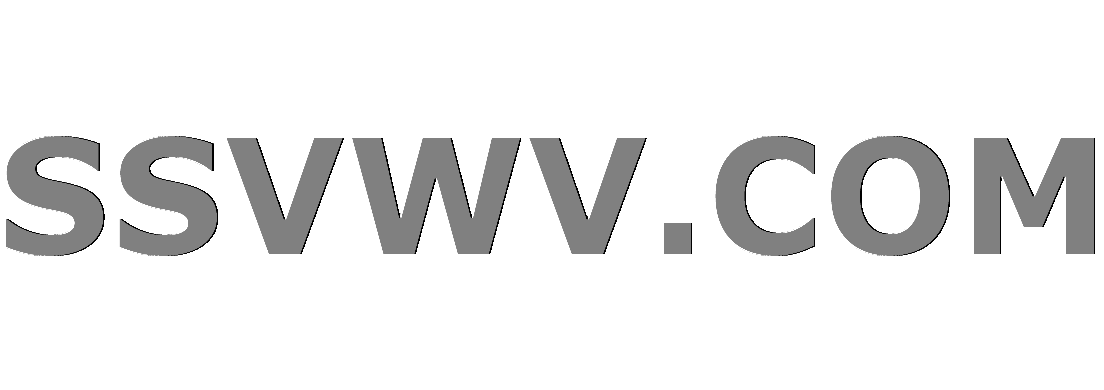
Multi tool use
This is the pseudocode we have to build the LL from:
FUNCTION push(element)
CREATE node
SET node.value TO element
SET node.next TO null
IF the head node does not exist
THEN SET head to node
ELSE
SET current to head
SET current.next to node
END IF
END FUNCTION
The pseudocode itself has an error in it as well.
Below is my attempt to follow that, but right now it's pointing
to the { right after value in the push function.
let head = null,
last,
node,
current,
value = element;
const linkedList = () => {
let node = new Node(value);
push(value) {
if(head === null) {
head = last = node;
} else {
last.next = node;
last = node;
}
}
}
Error : push(value) { <----- This curly bracket is throwing the error. Unexpected token.
javascript linked-list
|
show 1 more comment
This is the pseudocode we have to build the LL from:
FUNCTION push(element)
CREATE node
SET node.value TO element
SET node.next TO null
IF the head node does not exist
THEN SET head to node
ELSE
SET current to head
SET current.next to node
END IF
END FUNCTION
The pseudocode itself has an error in it as well.
Below is my attempt to follow that, but right now it's pointing
to the { right after value in the push function.
let head = null,
last,
node,
current,
value = element;
const linkedList = () => {
let node = new Node(value);
push(value) {
if(head === null) {
head = last = node;
} else {
last.next = node;
last = node;
}
}
}
Error : push(value) { <----- This curly bracket is throwing the error. Unexpected token.
javascript linked-list
1
Is this homework?
– Mawg
Nov 28 '18 at 8:31
1
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
1
What does "` it's pointing to the {" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several
– Mawg
Nov 28 '18 at 8:33
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
1
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44
|
show 1 more comment
This is the pseudocode we have to build the LL from:
FUNCTION push(element)
CREATE node
SET node.value TO element
SET node.next TO null
IF the head node does not exist
THEN SET head to node
ELSE
SET current to head
SET current.next to node
END IF
END FUNCTION
The pseudocode itself has an error in it as well.
Below is my attempt to follow that, but right now it's pointing
to the { right after value in the push function.
let head = null,
last,
node,
current,
value = element;
const linkedList = () => {
let node = new Node(value);
push(value) {
if(head === null) {
head = last = node;
} else {
last.next = node;
last = node;
}
}
}
Error : push(value) { <----- This curly bracket is throwing the error. Unexpected token.
javascript linked-list
This is the pseudocode we have to build the LL from:
FUNCTION push(element)
CREATE node
SET node.value TO element
SET node.next TO null
IF the head node does not exist
THEN SET head to node
ELSE
SET current to head
SET current.next to node
END IF
END FUNCTION
The pseudocode itself has an error in it as well.
Below is my attempt to follow that, but right now it's pointing
to the { right after value in the push function.
let head = null,
last,
node,
current,
value = element;
const linkedList = () => {
let node = new Node(value);
push(value) {
if(head === null) {
head = last = node;
} else {
last.next = node;
last = node;
}
}
}
Error : push(value) { <----- This curly bracket is throwing the error. Unexpected token.
javascript linked-list
javascript linked-list
edited Nov 28 '18 at 8:43
cjl85
asked Nov 28 '18 at 8:29


cjl85cjl85
7510
7510
1
Is this homework?
– Mawg
Nov 28 '18 at 8:31
1
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
1
What does "` it's pointing to the {" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several
– Mawg
Nov 28 '18 at 8:33
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
1
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44
|
show 1 more comment
1
Is this homework?
– Mawg
Nov 28 '18 at 8:31
1
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
1
What does "` it's pointing to the {" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several
– Mawg
Nov 28 '18 at 8:33
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
1
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44
1
1
Is this homework?
– Mawg
Nov 28 '18 at 8:31
Is this homework?
– Mawg
Nov 28 '18 at 8:31
1
1
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
1
1
What does "` it's pointing to the {
" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several– Mawg
Nov 28 '18 at 8:33
What does "` it's pointing to the {
" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several– Mawg
Nov 28 '18 at 8:33
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
1
1
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44
|
show 1 more comment
2 Answers
2
active
oldest
votes
Beside the syntax error, you have a logical error as well. You need to treat the head node different from any other node and set the head property to node if not set, but if set, then assign to the last node the next node.
If you no head node, you can not set the last node, because there is actually no one.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
With check for an already inserted value.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
|
show 4 more comments
And since we are at it.
Here is a version where you can insert append and remove nodes in ES6. No last node thougth since that would ruin the beauty of it. :)
class Node {
constructor(value) {
this.prev = null;
this.next = null;
this.value = value === undefined? null : value;
this.list = null;
}
remove() {
let prev = this.prev;
let next = this.next;
if (prev) {
prev.next = next;
}
if (next) {
next.prev = prev;
}
return this;
}
insert(node) {
let prev = this.prev;
if (prev) {
prev.next = node;
node.prev = prev;
}
this.prev = node;
node.next = this;
}
append(node) {
let next = this.next;
if (next) {
next.prev = node;
node.next = next;
}
this.next = node;
node.prev = this;
}
}
class LinkedList {
constructor() {
this.head = null;
}
get last() {
return this.list.length > 0 ? this.list[this.list.length] : null;
}
get values() {
let node = this.head;
let values = ;
while(node) {
values.push(node.value);
node = node.next;
};
return values;
}
push(node) {
node.prev = null;
node.next = null;
if (this.head) {
this.head.prev = node;
node.next = this.head;
}
this.head = node;
}
find(v) {
let node = this.head;
let fn = v;
if (!(v instanceof Function)) fn = (el) => el.value === v;
while(node && !fn(node)) {node = node.next;};
return node;
}
forEach(fn) {
let node = this.head;
while(node) {fn(node); node = node.next;};
}
}
Usable like so:
let ll = new LinkedList();
let n1= new Node(1);
let n2= new Node(2);
let n3= new Node(3);
ll.push(n1);
ll.push(n2);
ll.find(1).append(n3);
console.log(ll.values);
ll.find(3).remove();
console.log(ll.values);
ll.find(2).append(n3);
console.log(ll.values);
add a comment |
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53515119%2flinkedlist-creating-function-that-takes-a-value-creates-a-node-and-add-it-to%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
2 Answers
2
active
oldest
votes
2 Answers
2
active
oldest
votes
active
oldest
votes
active
oldest
votes
Beside the syntax error, you have a logical error as well. You need to treat the head node different from any other node and set the head property to node if not set, but if set, then assign to the last node the next node.
If you no head node, you can not set the last node, because there is actually no one.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
With check for an already inserted value.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
|
show 4 more comments
Beside the syntax error, you have a logical error as well. You need to treat the head node different from any other node and set the head property to node if not set, but if set, then assign to the last node the next node.
If you no head node, you can not set the last node, because there is actually no one.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
With check for an already inserted value.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
|
show 4 more comments
Beside the syntax error, you have a logical error as well. You need to treat the head node different from any other node and set the head property to node if not set, but if set, then assign to the last node the next node.
If you no head node, you can not set the last node, because there is actually no one.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
With check for an already inserted value.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
Beside the syntax error, you have a logical error as well. You need to treat the head node different from any other node and set the head property to node if not set, but if set, then assign to the last node the next node.
If you no head node, you can not set the last node, because there is actually no one.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
With check for an already inserted value.
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value);
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
class Node {
constructor(value) {
this.value = value;
this.next = null;
}
}
class LinkedList {
constructor() {
this.head = null;
this.last = null;
}
push(value) {
var node = new Node(value),
temp = this.head;
while (temp) {
if (temp.value === value) return;
temp = temp.next;
}
if (!this.head) {
this.head = node;
} else {
this.last.next = node;
}
this.last = node;
}
}
var ll = new LinkedList;
ll.push(10);
console.log(ll);
ll.push(11);
console.log(ll);
ll.push(12);
console.log(ll);
ll.push(11);
console.log(ll);
.as-console-wrapper { max-height: 100% !important; top: 0; }
edited Nov 28 '18 at 10:02
answered Nov 28 '18 at 8:59


Nina ScholzNina Scholz
192k15104176
192k15104176
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
|
show 4 more comments
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
thank you for that, i see where i was way off on
– cjl85
Nov 28 '18 at 9:04
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
do you have a moment to chat about linkedlists?
– cjl85
Nov 28 '18 at 9:26
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
yes, we can chat.
– Nina Scholz
Nov 28 '18 at 9:36
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
i don't know if i have the capabilities to create a chat room or how it works
– cjl85
Nov 28 '18 at 9:37
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
just ask here, and if the comments becomes more, the chat opportunity appears.
– Nina Scholz
Nov 28 '18 at 9:38
|
show 4 more comments
And since we are at it.
Here is a version where you can insert append and remove nodes in ES6. No last node thougth since that would ruin the beauty of it. :)
class Node {
constructor(value) {
this.prev = null;
this.next = null;
this.value = value === undefined? null : value;
this.list = null;
}
remove() {
let prev = this.prev;
let next = this.next;
if (prev) {
prev.next = next;
}
if (next) {
next.prev = prev;
}
return this;
}
insert(node) {
let prev = this.prev;
if (prev) {
prev.next = node;
node.prev = prev;
}
this.prev = node;
node.next = this;
}
append(node) {
let next = this.next;
if (next) {
next.prev = node;
node.next = next;
}
this.next = node;
node.prev = this;
}
}
class LinkedList {
constructor() {
this.head = null;
}
get last() {
return this.list.length > 0 ? this.list[this.list.length] : null;
}
get values() {
let node = this.head;
let values = ;
while(node) {
values.push(node.value);
node = node.next;
};
return values;
}
push(node) {
node.prev = null;
node.next = null;
if (this.head) {
this.head.prev = node;
node.next = this.head;
}
this.head = node;
}
find(v) {
let node = this.head;
let fn = v;
if (!(v instanceof Function)) fn = (el) => el.value === v;
while(node && !fn(node)) {node = node.next;};
return node;
}
forEach(fn) {
let node = this.head;
while(node) {fn(node); node = node.next;};
}
}
Usable like so:
let ll = new LinkedList();
let n1= new Node(1);
let n2= new Node(2);
let n3= new Node(3);
ll.push(n1);
ll.push(n2);
ll.find(1).append(n3);
console.log(ll.values);
ll.find(3).remove();
console.log(ll.values);
ll.find(2).append(n3);
console.log(ll.values);
add a comment |
And since we are at it.
Here is a version where you can insert append and remove nodes in ES6. No last node thougth since that would ruin the beauty of it. :)
class Node {
constructor(value) {
this.prev = null;
this.next = null;
this.value = value === undefined? null : value;
this.list = null;
}
remove() {
let prev = this.prev;
let next = this.next;
if (prev) {
prev.next = next;
}
if (next) {
next.prev = prev;
}
return this;
}
insert(node) {
let prev = this.prev;
if (prev) {
prev.next = node;
node.prev = prev;
}
this.prev = node;
node.next = this;
}
append(node) {
let next = this.next;
if (next) {
next.prev = node;
node.next = next;
}
this.next = node;
node.prev = this;
}
}
class LinkedList {
constructor() {
this.head = null;
}
get last() {
return this.list.length > 0 ? this.list[this.list.length] : null;
}
get values() {
let node = this.head;
let values = ;
while(node) {
values.push(node.value);
node = node.next;
};
return values;
}
push(node) {
node.prev = null;
node.next = null;
if (this.head) {
this.head.prev = node;
node.next = this.head;
}
this.head = node;
}
find(v) {
let node = this.head;
let fn = v;
if (!(v instanceof Function)) fn = (el) => el.value === v;
while(node && !fn(node)) {node = node.next;};
return node;
}
forEach(fn) {
let node = this.head;
while(node) {fn(node); node = node.next;};
}
}
Usable like so:
let ll = new LinkedList();
let n1= new Node(1);
let n2= new Node(2);
let n3= new Node(3);
ll.push(n1);
ll.push(n2);
ll.find(1).append(n3);
console.log(ll.values);
ll.find(3).remove();
console.log(ll.values);
ll.find(2).append(n3);
console.log(ll.values);
add a comment |
And since we are at it.
Here is a version where you can insert append and remove nodes in ES6. No last node thougth since that would ruin the beauty of it. :)
class Node {
constructor(value) {
this.prev = null;
this.next = null;
this.value = value === undefined? null : value;
this.list = null;
}
remove() {
let prev = this.prev;
let next = this.next;
if (prev) {
prev.next = next;
}
if (next) {
next.prev = prev;
}
return this;
}
insert(node) {
let prev = this.prev;
if (prev) {
prev.next = node;
node.prev = prev;
}
this.prev = node;
node.next = this;
}
append(node) {
let next = this.next;
if (next) {
next.prev = node;
node.next = next;
}
this.next = node;
node.prev = this;
}
}
class LinkedList {
constructor() {
this.head = null;
}
get last() {
return this.list.length > 0 ? this.list[this.list.length] : null;
}
get values() {
let node = this.head;
let values = ;
while(node) {
values.push(node.value);
node = node.next;
};
return values;
}
push(node) {
node.prev = null;
node.next = null;
if (this.head) {
this.head.prev = node;
node.next = this.head;
}
this.head = node;
}
find(v) {
let node = this.head;
let fn = v;
if (!(v instanceof Function)) fn = (el) => el.value === v;
while(node && !fn(node)) {node = node.next;};
return node;
}
forEach(fn) {
let node = this.head;
while(node) {fn(node); node = node.next;};
}
}
Usable like so:
let ll = new LinkedList();
let n1= new Node(1);
let n2= new Node(2);
let n3= new Node(3);
ll.push(n1);
ll.push(n2);
ll.find(1).append(n3);
console.log(ll.values);
ll.find(3).remove();
console.log(ll.values);
ll.find(2).append(n3);
console.log(ll.values);
And since we are at it.
Here is a version where you can insert append and remove nodes in ES6. No last node thougth since that would ruin the beauty of it. :)
class Node {
constructor(value) {
this.prev = null;
this.next = null;
this.value = value === undefined? null : value;
this.list = null;
}
remove() {
let prev = this.prev;
let next = this.next;
if (prev) {
prev.next = next;
}
if (next) {
next.prev = prev;
}
return this;
}
insert(node) {
let prev = this.prev;
if (prev) {
prev.next = node;
node.prev = prev;
}
this.prev = node;
node.next = this;
}
append(node) {
let next = this.next;
if (next) {
next.prev = node;
node.next = next;
}
this.next = node;
node.prev = this;
}
}
class LinkedList {
constructor() {
this.head = null;
}
get last() {
return this.list.length > 0 ? this.list[this.list.length] : null;
}
get values() {
let node = this.head;
let values = ;
while(node) {
values.push(node.value);
node = node.next;
};
return values;
}
push(node) {
node.prev = null;
node.next = null;
if (this.head) {
this.head.prev = node;
node.next = this.head;
}
this.head = node;
}
find(v) {
let node = this.head;
let fn = v;
if (!(v instanceof Function)) fn = (el) => el.value === v;
while(node && !fn(node)) {node = node.next;};
return node;
}
forEach(fn) {
let node = this.head;
while(node) {fn(node); node = node.next;};
}
}
Usable like so:
let ll = new LinkedList();
let n1= new Node(1);
let n2= new Node(2);
let n3= new Node(3);
ll.push(n1);
ll.push(n2);
ll.find(1).append(n3);
console.log(ll.values);
ll.find(3).remove();
console.log(ll.values);
ll.find(2).append(n3);
console.log(ll.values);
answered Nov 28 '18 at 9:37
JGoodgiveJGoodgive
542413
542413
add a comment |
add a comment |
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53515119%2flinkedlist-creating-function-that-takes-a-value-creates-a-node-and-add-it-to%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
LQI9unddj,JyMDFH5F
1
Is this homework?
– Mawg
Nov 28 '18 at 8:31
1
"The pseudocode itself has an error in it as well" - but you are not going to tell us what it is?
– Mawg
Nov 28 '18 at 8:32
1
What does "` it's pointing to the {
" mean?? Are you getting a compiler error? If so, tell us what it says. How can we help you otherwise? And which
{` ? There are several– Mawg
Nov 28 '18 at 8:33
This is an exercise that I'm doing
– cjl85
Nov 28 '18 at 8:41
1
Added last line to clarify where the error is
– cjl85
Nov 28 '18 at 8:44