PHP CURL Cannot Connect Between 2 Docker Sites
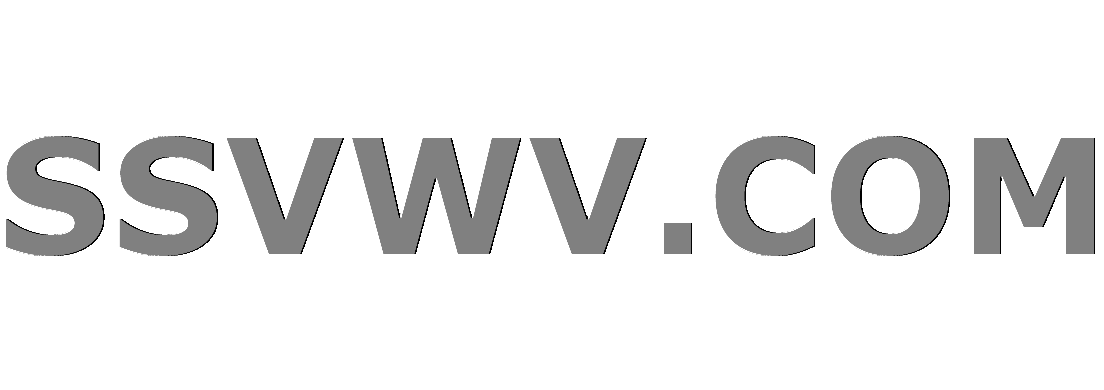
Multi tool use
I am having an issue with two docker sites, site1.example.com and api.example.com. Both sites are hosted on my local machine via docker. I am trying to make a request to api.example.com via curl, like so:
$curl_handle=curl_init();
curl_setopt($curl_handle,CURLOPT_URL,'http://api.example.com');
curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,1000);
curl_setopt($curl_handle, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl_handle, CURLOPT_HEADER, 1);
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl_handle, CURLOPT_PROXY, true);
curl_setopt($curl_handle, CURLOPT_VERBOSE, true);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl_handle);
if($result === false) {
$error = curl_error($curl_handle);
print_r($error);
}
print_r($result);
curl_close($curl_handle);
Here is what happens:
- If I turn-off CURLOPT_PROXY I get a connection refused error.
- I am getting a result = false with proxy on, except it gives no
error message - This is my docker logs:
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Rebuilt URL to: http://api.example.local/?is_deleted=0"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Trying 0.0.0.1..."
172.xx.x.x - 27/Nov/2018:18:23:03 +0000 "GET /index.php" 200
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* TCP_NODELAY set"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Immediate connect fail for 0.0.0.1: Invalid argument"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Closing connection 0"
But the same code and work fine on production. I am on php:7.2-fpm Any idea what is wrong with using curl from docker?
php docker curl
add a comment |
I am having an issue with two docker sites, site1.example.com and api.example.com. Both sites are hosted on my local machine via docker. I am trying to make a request to api.example.com via curl, like so:
$curl_handle=curl_init();
curl_setopt($curl_handle,CURLOPT_URL,'http://api.example.com');
curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,1000);
curl_setopt($curl_handle, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl_handle, CURLOPT_HEADER, 1);
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl_handle, CURLOPT_PROXY, true);
curl_setopt($curl_handle, CURLOPT_VERBOSE, true);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl_handle);
if($result === false) {
$error = curl_error($curl_handle);
print_r($error);
}
print_r($result);
curl_close($curl_handle);
Here is what happens:
- If I turn-off CURLOPT_PROXY I get a connection refused error.
- I am getting a result = false with proxy on, except it gives no
error message - This is my docker logs:
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Rebuilt URL to: http://api.example.local/?is_deleted=0"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Trying 0.0.0.1..."
172.xx.x.x - 27/Nov/2018:18:23:03 +0000 "GET /index.php" 200
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* TCP_NODELAY set"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Immediate connect fail for 0.0.0.1: Invalid argument"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Closing connection 0"
But the same code and work fine on production. I am on php:7.2-fpm Any idea what is wrong with using curl from docker?
php docker curl
add a comment |
I am having an issue with two docker sites, site1.example.com and api.example.com. Both sites are hosted on my local machine via docker. I am trying to make a request to api.example.com via curl, like so:
$curl_handle=curl_init();
curl_setopt($curl_handle,CURLOPT_URL,'http://api.example.com');
curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,1000);
curl_setopt($curl_handle, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl_handle, CURLOPT_HEADER, 1);
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl_handle, CURLOPT_PROXY, true);
curl_setopt($curl_handle, CURLOPT_VERBOSE, true);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl_handle);
if($result === false) {
$error = curl_error($curl_handle);
print_r($error);
}
print_r($result);
curl_close($curl_handle);
Here is what happens:
- If I turn-off CURLOPT_PROXY I get a connection refused error.
- I am getting a result = false with proxy on, except it gives no
error message - This is my docker logs:
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Rebuilt URL to: http://api.example.local/?is_deleted=0"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Trying 0.0.0.1..."
172.xx.x.x - 27/Nov/2018:18:23:03 +0000 "GET /index.php" 200
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* TCP_NODELAY set"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Immediate connect fail for 0.0.0.1: Invalid argument"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Closing connection 0"
But the same code and work fine on production. I am on php:7.2-fpm Any idea what is wrong with using curl from docker?
php docker curl
I am having an issue with two docker sites, site1.example.com and api.example.com. Both sites are hosted on my local machine via docker. I am trying to make a request to api.example.com via curl, like so:
$curl_handle=curl_init();
curl_setopt($curl_handle,CURLOPT_URL,'http://api.example.com');
curl_setopt($curl_handle,CURLOPT_CONNECTTIMEOUT,1000);
curl_setopt($curl_handle, CURLOPT_RETURNTRANSFER, 1);
curl_setopt($curl_handle, CURLOPT_HEADER, 1);
curl_setopt($curl_handler, CURLOPT_FOLLOWLOCATION, true);
curl_setopt($curl_handle, CURLOPT_PROXY, true);
curl_setopt($curl_handle, CURLOPT_VERBOSE, true);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYPEER, false);
curl_setopt($curl_handle, CURLOPT_SSL_VERIFYHOST, false);
$result = curl_exec($curl_handle);
if($result === false) {
$error = curl_error($curl_handle);
print_r($error);
}
print_r($result);
curl_close($curl_handle);
Here is what happens:
- If I turn-off CURLOPT_PROXY I get a connection refused error.
- I am getting a result = false with proxy on, except it gives no
error message - This is my docker logs:
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Rebuilt URL to: http://api.example.local/?is_deleted=0"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Trying 0.0.0.1..."
172.xx.x.x - 27/Nov/2018:18:23:03 +0000 "GET /index.php" 200
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* TCP_NODELAY set"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Immediate connect fail for 0.0.0.1: Invalid argument"
[27-Nov-2018 18:23:04] WARNING: [pool www] child 352 said into stderr: "* Closing connection 0"
But the same code and work fine on production. I am on php:7.2-fpm Any idea what is wrong with using curl from docker?
php docker curl
php docker curl
asked Nov 27 '18 at 18:48
Devin DixonDevin Dixon
3,7591658116
3,7591658116
add a comment |
add a comment |
0
active
oldest
votes
Your Answer
StackExchange.ifUsing("editor", function () {
StackExchange.using("externalEditor", function () {
StackExchange.using("snippets", function () {
StackExchange.snippets.init();
});
});
}, "code-snippets");
StackExchange.ready(function() {
var channelOptions = {
tags: "".split(" "),
id: "1"
};
initTagRenderer("".split(" "), "".split(" "), channelOptions);
StackExchange.using("externalEditor", function() {
// Have to fire editor after snippets, if snippets enabled
if (StackExchange.settings.snippets.snippetsEnabled) {
StackExchange.using("snippets", function() {
createEditor();
});
}
else {
createEditor();
}
});
function createEditor() {
StackExchange.prepareEditor({
heartbeatType: 'answer',
autoActivateHeartbeat: false,
convertImagesToLinks: true,
noModals: true,
showLowRepImageUploadWarning: true,
reputationToPostImages: 10,
bindNavPrevention: true,
postfix: "",
imageUploader: {
brandingHtml: "Powered by u003ca class="icon-imgur-white" href="https://imgur.com/"u003eu003c/au003e",
contentPolicyHtml: "User contributions licensed under u003ca href="https://creativecommons.org/licenses/by-sa/3.0/"u003ecc by-sa 3.0 with attribution requiredu003c/au003e u003ca href="https://stackoverflow.com/legal/content-policy"u003e(content policy)u003c/au003e",
allowUrls: true
},
onDemand: true,
discardSelector: ".discard-answer"
,immediatelyShowMarkdownHelp:true
});
}
});
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53506270%2fphp-curl-cannot-connect-between-2-docker-sites%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
0
active
oldest
votes
0
active
oldest
votes
active
oldest
votes
active
oldest
votes
Thanks for contributing an answer to Stack Overflow!
- Please be sure to answer the question. Provide details and share your research!
But avoid …
- Asking for help, clarification, or responding to other answers.
- Making statements based on opinion; back them up with references or personal experience.
To learn more, see our tips on writing great answers.
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
StackExchange.ready(
function () {
StackExchange.openid.initPostLogin('.new-post-login', 'https%3a%2f%2fstackoverflow.com%2fquestions%2f53506270%2fphp-curl-cannot-connect-between-2-docker-sites%23new-answer', 'question_page');
}
);
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Sign up or log in
StackExchange.ready(function () {
StackExchange.helpers.onClickDraftSave('#login-link');
});
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Sign up using Google
Sign up using Facebook
Sign up using Email and Password
Post as a guest
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
Required, but never shown
s79I jDxmJNURJAViB,AV W3ejOvCXia,ySEd2b6,2yBf